12.2. OneInOneOutGUI Example¶
This module provides agraphical interface to an Arduino running the OneInOneOutASCII Arduino Sketch.
It is built using several abstract classes implementng reusable tools for creating simple graphical user interfaces for Arduino programs using the PyQt4 library. It may optionally use the PyQwt library to render a dynamic plot window.
The full code can be found in several files in OneInOneOutGUI or downloaded as a single zip file OneInOneOutGUI.zip
12.2.1. Screenshot¶
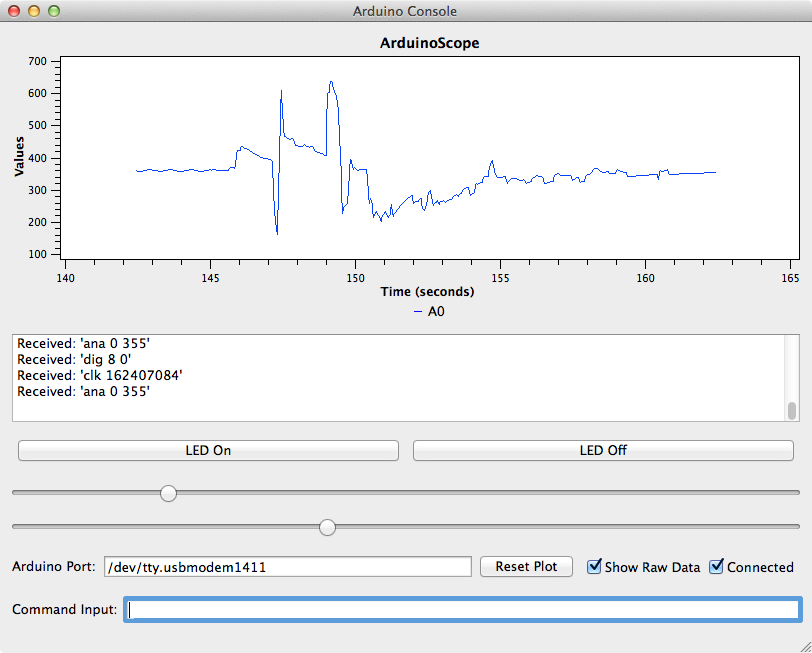
12.2.2. OneInOneOutGUI.py¶
OneInOneOutGUI.py
GUI controller for the OneInOneOutASCII Arduino sketch. This interface shell communicates over a serial port to an Arduino running a sketch which includes line-oriented text input and output.
Copyright (c) 2015, Garth Zeglin. All rights reserved. Licensed under the terms of the BSD 3-clause license.
-
class
OneInOneOutGUI.OneInOneOutGUI.
OneInOneOutGUIController
(port=None, **kwargs)[source]¶ Application-specific control object to manage the GUI for OneInOneOutASCII. This class creates a generic ArduinoConsole GUI, adds application-specific GUI controls, and manages basic I/O.
Parameters: - port – the name of the serial port device
- kwargs – collect any unused keyword arguments
Callback function activated to disable the LED.
Callback function activated to enable the LED.
12.2.3. run_gui.py¶
This script launches the GUI.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 | #!/usr/bin/env python
"""\
run_gui.py
Sample script to create and operate a graphical user interface for an Arduino
program. This interface shell communicates over a serial port to an Arduino
running a sketch which includes line-oriented text input and output.
Copyright (c) 2015, Garth Zeglin. All rights reserved. Licensed under the terms
of the BSD 3-clause license.
"""
from __future__ import print_function
import os, sys, argparse
# This requires the PyQt4 module to interface to the Qt GUI toolkit. For
# documentation on the PyQt4 API, see http://pyqt.sourceforge.net/Docs/PyQt4/index.html
from PyQt4 import QtGui
# Import the custom GUI class.
from OneInOneOutGUI import OneInOneOutGUIController
################################################################
# Main script follows. This sequence is executed when the script is initiated from the command line.
if __name__ == "__main__":
# process command line arguments
parser = argparse.ArgumentParser(description = """Run the GUI for the OneInOneOutGUIASCII Arduino sketch.""")
parser.add_argument( '-v', '--verbose', action='store_true', help='Enable more detailed output.' )
parser.add_argument( '-p', '--port', default='/dev/tty.usbmodem1411', \
help='Specify the name of the Arduino serial port device (default is /dev/tty.usbmodem1411).')
args = parser.parse_args()
# initialize the Qt system itself
app = QtGui.QApplication(sys.argv)
# create the interface window
window = OneInOneOutGUIController(port=args.port)
print("""Starting up OneInOneOutGUI.
Toggle the 'Connected' switch to open the Arduino serial port.
Click 'Enable' while connected to enable motor drivers.
""")
# run the event loop until the user is done
sys.exit(app.exec_())
|
12.2.4. ArduinoConsole.py¶
ArduinoConsole.py
PyQt4 window implementing a generic control console for an Arduino sketch, including command line, plot window, and serial port controls. Functions are provide to allow extending the basic interface with custom buttons and sliders without needing to use Qt Designer.
Copyright (c) 2013-2015, Garth Zeglin. All rights reserved. Licensed under the terms of the BSD 3-clause license as included in LICENSE.
-
class
OneInOneOutGUI.ArduinoConsole.
ArduinoConsole
(view=None)[source]¶ A custom window which inherits both from the QMainWindow class and the custom Ui_ArduinoConsoleWindow defined using Qt Designer.
-
ArduinoConnectToggled
(flag)[source]¶ Callback invoked whenever the Arduino Connect checkbox changes state.
-
ArduinoPortEntered
()[source]¶ Callback invoked whenever text is entered into the Arduino Port field.
-
addButton
(title, callback)[source]¶ Add a button to the general-purpose button area of the interface. The title argument should be a short string to appear on the face of the button. The callback function is invoked whenever the button is pressed.
-
addScopeChannel
(*args, **kwargs)[source]¶ Add a named channel to the ArduinoScope plotting widget. The arguments are passed unchanged to add_channel().
-
addScopeSamples
(name, y, t)[source]¶ Add a set of new samples to a given named channel to the ArduinoScope plotting widget. The arguments are passed unchanged to add_samples().
-
addSlider
(title, callback)[source]¶ Add a horizontal slider to the controls area. The range is fixed over [0,1.0).
Parameters: - title – string used as a tooltip
- callback – function receiving floating slider value
-
attachCommandCallback
(callback)[source]¶ Set the callback function to be invoked when the user enters a command.
Parameters: callback – callback( command_string )
-
attachConnectCallback
(callback)[source]¶ Set the callback function to be invoked when the user requests a connect or disconnect from the Arduino.
Parameters: callback – callback( port_name, flag ) flag is True for connect, False for disconnect
-
newInputMonitor
(fd, callback)[source]¶ Convenience function to create a notification callback when input is available on a file descriptor.
Parameters: - fd – integer file descriptor
- callback – one-argument function callback(fd) to be called with the file descriptor
Returns: the underlying QSocketNotifier object
-
newPeriodicTimer
(interval, callback)[source]¶ Convenience function to create a periodic timer which calls a function at the given interval.
Parameters: - interval – interval in milliseconds
- callback – no-argument function callback() to be called at intervals
Returns: the underlying QTimer object
-
newSingleShotTimer
(interval, callback)[source]¶ Convenience function to set up a single-shot timer which calls a function once after the given interval.
Parameters: - interval – interval in milliseconds
- callback – no-argument function callback() to be called once after a delay
-
12.2.5. ArduinoScope.py¶
ArduinoScope.py : Qt/Qwt widget to show a real-time oscilloscope-like display of signals
Copyright (c) 2015, Garth Zeglin. All rights reserved. Licensed under the terms of the BSD 3-clause license.
-
class
OneInOneOutGUI.ArduinoScope.
ArduinoScope
(*args)[source]¶ Plot widget to show real-time data like a multichannel oscilloscope. Implemented as a subclass of the QwtPlot widget provided in the Qwt5 package. Requires Qwt5 and PyQwt5.
-
add_channel
(name, *args, **kwargs)[source]¶ Add a new channel to the oscilloscope. The parameters are passed to ScopeChannel.init.
Parameters: - name – short name with which to identify the channel internally
- title – optional title string to use on plot (default is to use the name value)
- color – optional color name string. Examples: ‘red’, ‘purple, ‘#80ff30’ (default is random)
- duration – optional limit on the length of the time history to store
-
add_samples
(name, y, t)[source]¶ Add one or more data samples to the buffer in the channel. If a maximum duration was specified during creation, the data buffer will be truncated to hold the specified maximum amount of history. After all channels have been updated, call the replot() method to update the display.
Parameters: - name – channel name as supplied during creation
- y – list or ndarray of signal values
- t – list or ndarray of times, must be same length as y
-
-
class
OneInOneOutGUI.ArduinoScope.
ScopeChannel
(name, title=None, color=None, duration=None)[source]¶ Internal class to represent the state of one channel in an ArduinoScope widget. See ArduinoScope.add_channel() for arguments.
-
add_samples
(y, t)[source]¶ Add one or more data samples to the buffer in the channel. If a maximum duration was specified during creation, the data buffer will be truncated to hold the specified maximum amount of history.
Parameters: - y – list or ndarray of signal values
- t – list or ndarray of times, must be same length as y
-
12.2.6. ArduinoConsoleWindow.py¶
This file is generated from an XML interface description using the
pyuic interface compiler. The XML description represents the basic
interface layout, created and edited using Qt Designer and saved as the XML file
ArduinoConsoleWindow.ui
.