Today we mapped out our game flow and looked at how our various object interactions would move us through our state machine, and then used another singleton, the GameManger, to help coordinate this journey, then spent a little time at the end making cooler enemies. (You don’t have to do this for your game, but you may want to!)
Part 1: Game Flow
First, we mapped out our game flow so that we know what states we intend to use, and how we can move between them. We implemented some (but not all) of these steps, and those that remain are your responsibility for this week’s assignment.
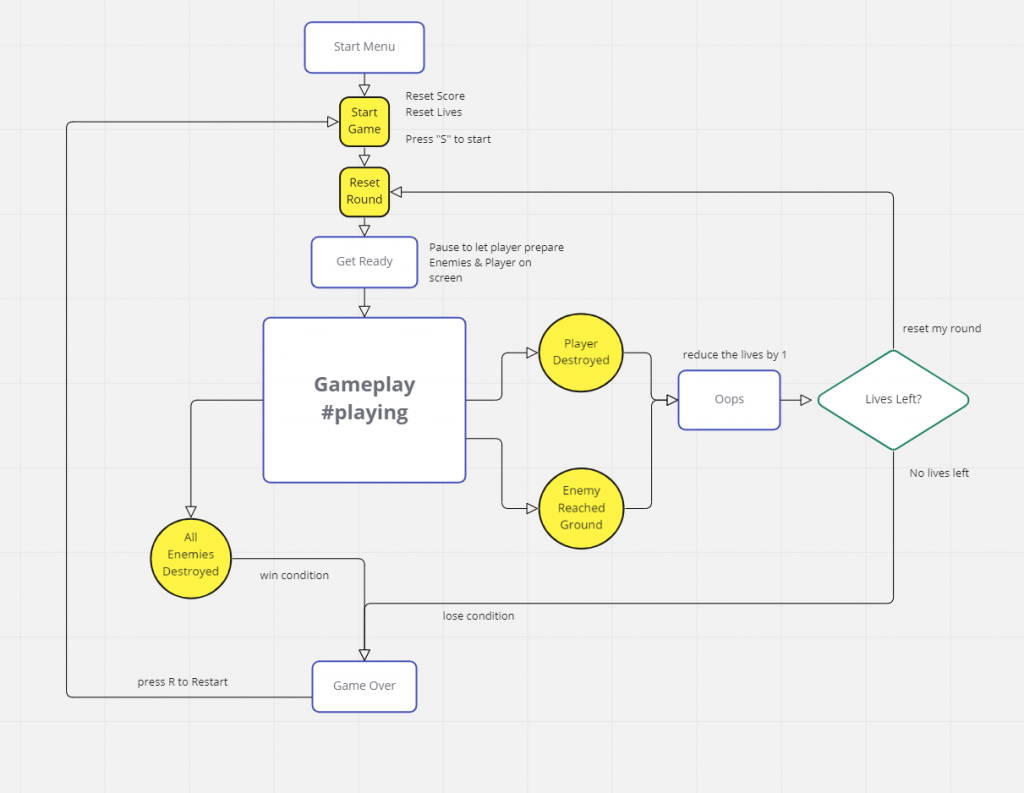
- We start in an inert state that invites us to “Press S to Start”. This is the Menu state and eventually we will replace it with a separate scene with way better UI.
- Pressing S takes us to a new game, where the number of lives are set, and the objects for the first round are prepared. Once ready, we will go into the GetReady state and hold there momentarily.
- Upon completion of the GetReady state, we move into Playing,
- During Playing, the player will have control of the ship and be able to shoot, and the enemies will begin their attack. There are three possible ways to exit this state.
- The first way occurs in case of a win condition – the player destroys all of the enemy ships – and are taken to the GameOver state with a win message.
- The other two methods are losing conditions – the player object is destroyed by an enemy, or the enemy ship reaches the ground. In this case, the player loses a life, and the round is reset after an oops state, or the game is over if the player has run out of lives.
The first step to build out this structure is for us to create our GameManager object (again created from an empty gameobject), and create the GameManager.cs script for it.
We declare our variable to hold the Singleton…
public static GameManager S; // set up the singleton
… and we assign it. This time, we add in some extra protection to make sure that a version of this singleton does not already exist. This can happen when we move between scenes, if we were to preserve our GameManager object, we might enter a scene that already has a GameManager inside of it. We will test for the instance and destroy if we find one already in place.
private void Awake() { if (GameManager.S) { // singleton already exists, destroy this instance Destroy(this.gameObject); } else { // define the singleton S = this; } }
Next we define a number of enumerated values for our various gamestates:
public enum GameState { menu, getReady, playing, oops, gameOver};
To implement Step 1, we set up a GameState variable in our script and test against it at appropriate times. For instance, pressing “S” would mean one thing during our Menu stage (it should be interpreted as the “start” command) but might mean something different during gameplay, if we are using our Input.GetAxis(“Vertical”), which relies on pressing WASD keys. And for the assignment, you can do the same sort of check for the GameOver state looking for a user to press the R key to restart.
void Update() { if (gameState == GameState.menu) { // game is in menu state, press S to start will advance if (Input.GetKeyDown(KeyCode.S)) { StartANewGame(); } } else if (gameState == GameState.gameOver) { // press r to restart the game } }
We can begin to set up the stages. We created a StartNewGame() function that resets the lives left (and later will also reset the score, which is part of this week’s assignment)
We also create ResetRound( ) and StartRound( ) functions. ResetRound( ) is Step 2, and will place the block of enemies in the starting position. We make our Mothership object a prefab, and remove it from the scene, but add the prefab to a GameObject variable in this script. ResetRound instantiates the prefab and stores it in another GameObject variable that is our “currentMothership”. Before we instantiate, we test to see if one already exists. If this returns true, then we probably have arrived here after a player lost a round. We want to reset the enemy objects, so we destroy whatever already exists.
private void ResetRound() { // spawn a new set of enemies if (currentMotherShip) { Destroy(currentMotherShip); } currentMotherShip = Instantiate(motherShipPrefab); // put us in the get ready state gameState = GameState.getReady; // run the GetReadyState coroutine StartCoroutine(GetReadyState()); }
Next we transition to the GetReady state, and kicking off a coroutine that will let us pause for 3 seconds, then trigger StartRound( ), which completes Step 3. StartRound( ) moves us to the Playing state, and we remain here until it is time to end a round.
Now that we can get to the playing state, it is time to adjust some of our object behaviors, and make them state dependent.
First, we want to restrict the player movement so that they can only move and shoot while we are in the Playing state. To accomplish this, we add a gamestate check in the Player’s update function. By wrapping the contents with the following “if” statement, we ensure that motion only occurs while in the Playing state.
if (GameManager.S.gameState == GameState.playing) { ... }
Again, note that there is no need to associate the game manager with any object in our Player script, and no references to be made. “GameManager.S” is available to be called globally.
The next problem is that our enemy object starts moving and shooting during the get ready state. I also don’t like that it continues to move after my player has been destroyed. I want to prevent the possibility of multiple state requests coming in while we are in our Oops state, so I am going to create new scripts in the Enemy object that will be responsible for starting and stopping the coroutines.
Since I am instantiating the Mothership in GameManager, I have access to the object that is the instance in our scene. I add public functions to StartTheAttack( ) [which starts the coroutines previously held in Start( )] and StopTheAttack( ) in the MotherShip.cs script, and then call those from GameManager using:
currentMotherShip.GetComponent<MotherShip>().StopTheAttack();
In our StopTheAttack( ) function, we call the StopAllCoroutines( ) function. This will cease any coroutines currently running from the component.
public void StopTheAttack() { StopAllCoroutines(); }
Next, we implemented one of the three conditions that will get us out – specifically the case where an enemy bomb hits the player. We created a PlayerDestroyed( ) function and then call it from a OnCollisionEnter that we add to the Player script.
private void OnCollisionEnter(Collision collision) { if (collision.transform.tag == "EnemyBomb") { // make the player explode Destroy(this.gameObject); // make the boom boom noise SoundManager.S.MakePlayerExplosion(); // inform the manager that the player exploded GameManager.S.PlayerDestroyed(); } }
Now when the Player collides with an EnemyBomb, the PlayerDestroyed( ) script is called on the GameManager. PlayerDestroyed removes a life from the count, and then moves us to the Oops state, through a coroutine.
public void PlayerDestroyed() { // remove a life livesLeft--; // go to oops state StartCoroutine(OopsState()); } public IEnumerator OopsState() { // put the game in oops state gameState = GameState.oops; // tell the enemy to stop their attack currentMotherShip.GetComponent<MotherShip>().StopTheAttack(); // pause yield return new WaitForSeconds(2.0f); // decide if we restart or game over if (livesLeft > 0) { // restart our round ResetRound(); } else { // go to game over state gameState = GameState.gameOver; } }
We start the OopsState coroutine by putting the game into the “oops” gamestate, and we the Mothership to stop moving and dropping bombs. Then we yield activity for a few seconds to pause the action. During this time, each object’s Update( ) command will be called on each frame. This is why we move into a different game-state, and have objects test against that state – because the game engine keeps running even when our game flow is taking a rest. We use these variables to help the other objects exhibit the proper behavior.
Part 2: Better Enemies (optional!)
Since we had a little extra time at the end of class, I demonstrated my favorite method for making cool voxel enemies that appear to animate. The process is relatively simple, and when they explode it adds a great juicy quality to the action. Please note, enemies like this are NOT required for your assignment, but if you would like to create some of your own, you should definitely try it out!
Our previous alien attackers were rather primitive – Unity primitives, to be exact. (Thank you, I’ll be here all week!) Now that we have a fancy setting, our enemies should be a little more… dramatic. For this section, we are going to look to the source material and draw some inspiration from the classic pixel-y goodness of the original, and build our enemies out of cubes.
Why cubes? First off, they are super easy to create and work with, and require surprisingly little overhead in terms of rendering and physics calculation. Second, I want to show you how you can create a game using just the tools provided – no need for special 3D modeling/animation software. Third, we are going to use Physics on those blocks to create a satisfying explosion effect on the alien ship that will add some fun to our game.
First, we create a single cube, using GameObject > 3D Object > Cube or Create… > 3D Object > Cube. I’m going to want my bottom center cube to represent the “position” of the object so I will set its position to the origin (0, 0, 0). If yours is not at that location, you can select the settings dropdown from the Transform component and select Reset Transform to do so.
Next we make copies of the cube and offset them by 1 unit increments so that you have a row of 5 cubes with their edges touching. Now copy those rows and move them up by 1 unit increments, until you have a 5×5 cube grid.
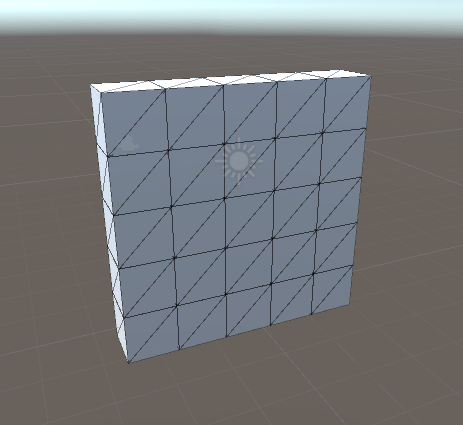
Then, we create an empty game object (GameObject > Create Empty or Create… > Create Empty) also located at (0, 0, 0) and make all of the cubes children of this empty object. Notice that when you add the cubes as children, they now indent under the parent object in the Hierarchy, and the parent object now has a small button that lets you roll-up the list.
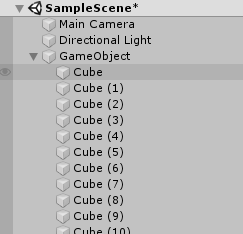
I expect to create a number of aliens using this same block grid, and so I will create a prefab object from it that I can use as a template for future iterations. I name the parent object “EnemyBase” and drag it into my Asset window. I can now use this prefab to override the cubes that are on and off in order to make shapes. Once I have edited this to make an alien shape, I will save this as a different prefab. (This is my path to create these objects, but this is by no means the only path. Do what works for you!)
Once I have unpacked the object, I edit it by de-activating some of the blocks. You can delete these, or simply turn the cube objects off using the check box next to the GameObject name in the Inspector. This way, the block is preserved, in case you want to go back later and edit quickly. Remove some blocks and make an alien shape like so:
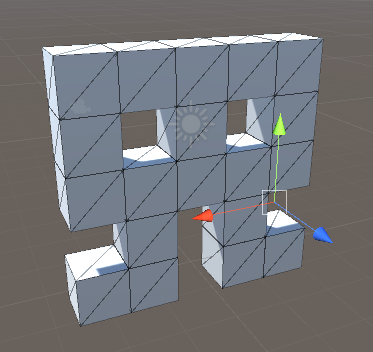
Time to give the parent object a name. I called mine EnemyFrameA, since I will have multiple versions of this, each one representing a frame of animation.
Now I turn off EnemyFrameA, and place a new instance of the EnemyBasetemplate in the scene at the same position. I unpack, edit it to look as though the legs have moved, and name the parent object EnemyFrameB.
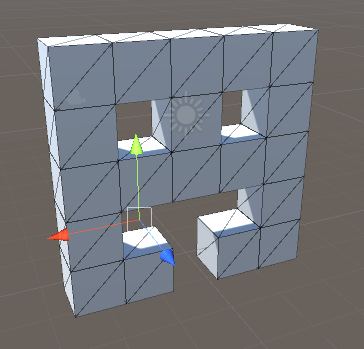
Now I am going to create a duplicate of EnemyA_Frame1, but I will call this one EnemyA_FrameExplode. This object will be out “stunt double”, swapping places with the other frames when it is time for the ship to blow up. To get that great explosive force, add a Rigidbody component to each of the cubes in EnemyA_FrameExplode. (You can simply select all of the cubes at once and go to Add Component).
It is also worth noting that in my objects for frames A & B, I have turned their colliders off. This is because I want to have to worry about managing collisions on the individual blocks – I want these to simply be a part of a cohesive whole.
I’m going to make each one of our alien parent objects a prefab by dragging it down into the Assets folder. Next, I am going to create a new empty game object and parent it to these prefabs, so that it serves as a container to the individual frames. Name this object EnemyTypeA1 and make this object into a prefab as well. I add my Enemy class to this, as well as a Box Collider component which I will resize to fit around my enemy object. (Don’t forget to change your layer designation to “Enemy”!)
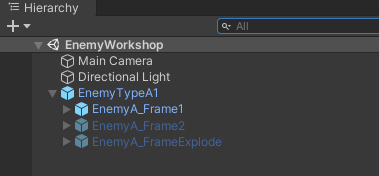
Now, let’s add a little bit of scripting to bring our little attacker to life. We want to open our Enemy script and add variables to hold the three child objects of this particular enemy.
public GameObject FrameA; public GameObject FrameB; public GameObject FrameExplode;
We define three objects (which we must populate with the Inspector), and then on Start( ) we set their conditions so that only the modelOne model is active.
NOTE: I should probably use Awake so that this is set instantly, just in case something else is going to access our models when the Start( ) event runs, but for today, Start will do.
private void Start() { FrameA.SetActive(true); FrameB.SetActive(false); FrameExplode.SetActive(false); // start the swapping StartCoroutine(NextStage()); }
When Start( ) does arrive, we use that to launch a Coroutine that I have created called NextStage( ) that will call my SwapFrames( ) command once per second. It accomplishes this by nesting the call and the 1.0 second yield inside of a “while” loop that will run continuously for the duration of the object because the condition is set to “true”.
public IEnumerator NextStage() { while (true) { SwapFrames(); yield return new WaitForSeconds(1.0f); } }
The SwapFrames( ) command simply set the active state of both frames to the opposite of its current value. (I get that current value with the GameObject property activeSelf)
public void SwapFrames() { FrameA.SetActive(!FrameA.activeSelf); FrameB.SetActive(!FrameB.activeSelf); }
NOTE
In previous versions of this assignment, I would tend to use the GameObject’s “active” property, getting and setting that directly. But over time, Unity started to become mad at this, because this particular interaction is being phased out. So instead the proper method is used, utilizing the GameObject.SetActive( ) command, and reading the value using the “activeSelf” property.
Once this is done, I am free to go back into my main scene and replace the MotherShip prefab enemies with our new advanced enemy prefabs.
Part 3: Bigger Explosions!
For this next part, we want to test out our explosion effect – where we swap out our model frames with our “stunt double” and then apply an explosive force to the individual boxes. We do this by creating an Explode( ) command in our Enemy, like so:
private void Explode() { // make an explosion Instantiate(enemyExplosionPrefab, transform.position, Quaternion.identity); // activate explosion frame FrameA.SetActive(false); FrameB.SetActive(false); FrameExplode.SetActive(true); Rigidbody[] cubes; // variable to hold rigidbodies // get every rigidbody inside the explosion object. cubes = FrameExplode.GetComponentsInChildren<Rigidbody>(); // apply an explosive force foreach (Rigidbody rb in cubes) { rb.AddExplosionForce(250f, (FrameExplode.transform.position + Vector3.forward), 10f); } FrameExplode.transform.parent = null; }
Once we have turned off our models and turned on our “stunt double” object, our goal is to apply the Rigidbody.AddExplosionForce( ) to each cube in the object. This method takes three arguments – a force to exert, an origination point for the explosion, and a radius within which the explosion will have an effect. We create public float objects for “explosionForce” and “explosionRadius” in the class declaration, and then set those values.
In our explode command, you see that we declared a variable as “Rigidbody[ ] cubes”. These brackets mean that we expect to receive more than one result – an array of Rigidbody components. We then generate this list using the GetComponentsInChildren<>( ) method which returns the array of all child objects for “modelExplosion” that have a Rigidbody component in their backpack. Finally we use a “foreach” loop that lets us cycle through each result in our array. In each pass of the loop (that is, for each Rigidbody object in cubes[ ]) we set that object to the variable “rb”, and then apply our explosive force to that Rigidbody. By cycling through all of these we ensure that every object is affected by the force.
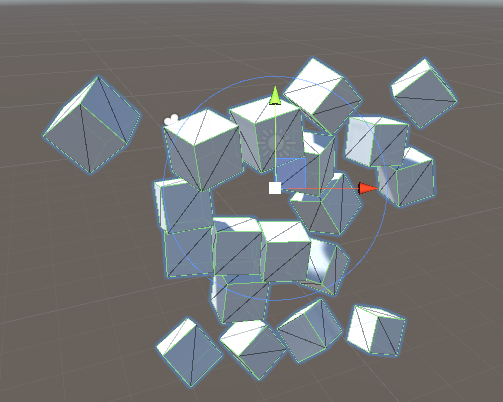
Now, this creates a problem, because we previously set the Enemy object to self-destruct as soon as it collided with the bullet. If we destroy the object, the children will be destroyed as well, along with our “stunt double”. Everything will just blink out of existence. But what if our stunt double was not a child of the enemy object anymore? To accomplish this, we are going to emancipate our stunt double from it’s parent object, making it an orphan (or as I like to call it, a “child of the world”). We do this by setting the parent of that object to “null”.
FrameExplode.transform.parent = null;
Now our enemy object destroys itself, but not before throwing the stunt double out into the world and applying the explosion just before it disappears. Because our Explode( ) command runs prior to the destruction of the Enemy object, our stunt double makes it out alive (but not for long).
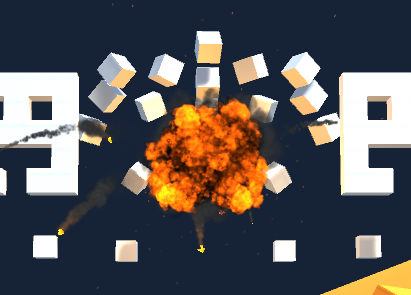
Tomorrow we will look at improved UI fonts using TextMeshPro, and at the “build” process, where we compile our game that only runs in an editor into it’s own standalone executable.
GameManager.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; public enum GameState { menu, getReady, playing, oops, gameOver}; public class GameManager : MonoBehaviour { public static GameManager S; // set up the singleton public GameState gameState; // game variables private int score; private int livesLeft; private int LIVES_AT_START = 3; // mothership variable private GameObject currentMotherShip; public GameObject motherShipPrefab; private void Awake() { // Singleton Definition if (GameManager.S) { // singleton already exists, destroy this instance Destroy(this.gameObject); } else { // define the singleton S = this; } } // Start is called before the first frame update void Start() { // set the gamestate gameState = GameState.menu; } // Update is called once per frame void Update() { if (gameState == GameState.menu) { // game is in menu state, press S to start will advance if (Input.GetKeyDown(KeyCode.S)) { StartANewGame(); } } else if (gameState == GameState.gameOver) { // press r to restart the game } } void StartANewGame() { // reset our lives livesLeft = LIVES_AT_START; // reset our score score = 0; // go to the first round ResetRound(); } private void ResetRound() { // spawn a new set of enemies if (currentMotherShip) { Destroy(currentMotherShip); } currentMotherShip = Instantiate(motherShipPrefab); // put us in the get ready state gameState = GameState.getReady; // run the GetReadyState coroutine StartCoroutine(GetReadyState()); } private void StartRound() { // start the attack currentMotherShip.GetComponent<MotherShip>().StartTheAttack(); // put this into the playing state gameState = GameState.playing; } public IEnumerator GetReadyState() { // turn on the get ready message // wait for a few seconds yield return new WaitForSeconds(3.0f); // turn off the get ready message // start the round StartRound(); } public void PlayerDestroyed() { // remove a life livesLeft--; // go to oops state StartCoroutine(OopsState()); } public IEnumerator OopsState() { // put the game in oops state gameState = GameState.oops; // tell the enemy to stop their attack currentMotherShip.GetComponent<MotherShip>().StopTheAttack(); // put up destroyed message // pause yield return new WaitForSeconds(2.0f); // decide if we restart or game over if (livesLeft > 0) { // restart our round ResetRound(); } else { // go to game over state gameState = GameState.gameOver; // game over lose message } } }
Enemy.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Enemy : MonoBehaviour { public GameObject enemyBombPrefab; public GameObject enemyExplosionPrefab; public GameObject FrameA; public GameObject FrameB; public GameObject FrameExplode; private void Start() { FrameA.SetActive(true); FrameB.SetActive(false); FrameExplode.SetActive(false); // start the swapping StartCoroutine(NextStage()); } public void SwapFrames() { FrameA.SetActive(!FrameA.activeSelf); FrameB.SetActive(!FrameB.activeSelf); } public IEnumerator NextStage() { while (true) { SwapFrames(); yield return new WaitForSeconds(1.0f); } } public void DropABomb() { // make a bomb Instantiate(enemyBombPrefab, (transform.position + Vector3.down), Quaternion.identity); } private void Explode() { // make an explosion Instantiate(enemyExplosionPrefab, transform.position, Quaternion.identity); // activate explosion frame FrameA.SetActive(false); FrameB.SetActive(false); FrameExplode.SetActive(true); Rigidbody[] cubes; // variable to hold rigidbodies // get every rigidbody inside the explosion object. cubes = FrameExplode.GetComponentsInChildren<Rigidbody>(); // apply an explosive force foreach (Rigidbody rb in cubes) { rb.AddExplosionForce(250f, (FrameExplode.transform.position + Vector3.forward), 10f); } FrameExplode.transform.parent = null; } private void Update() { if (Input.GetKeyDown(KeyCode.B)) { DropABomb(); } } private void OnCollisionEnter(Collision collision) { if (collision.transform.tag == "PlayerBullet") { // make the explosion effect Explode(); // tell the bullet to destroy itself Destroy(collision.gameObject); // destroy this object Destroy(this.gameObject); // make the explosion noise SoundManager.S.MakeEnemyExplosionSound(); } } }
Player.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Player : MonoBehaviour { public float speed; public float MAX_OFFSET; public GameObject bulletPrefab; // Update is called once per frame void Update() { // move our player object Vector3 currentPosition = transform.position; currentPosition.x = currentPosition.x + (Input.GetAxis("Horizontal") * speed * Time.deltaTime); // restrict motion to range currentPosition.x = Mathf.Clamp(currentPosition.x, -MAX_OFFSET, MAX_OFFSET); // update the position transform.position = currentPosition; if (GameManager.S.gameState == GameState.playing) { if (Input.GetKeyDown(KeyCode.Space)) { FireBullet(); } } } void FireBullet() { // instantiate a bullet Instantiate(bulletPrefab, (transform.position + Vector3.up), Quaternion.identity); } private void OnCollisionEnter(Collision collision) { if (collision.transform.tag == "EnemyBomb") { // make the player explode Destroy(this.gameObject); // make the boom boom noise SoundManager.S.MakePlayerExplosion(); // inform the manager that the player exploded GameManager.S.PlayerDestroyed(); } } }
MotherShip.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; public class MotherShip : MonoBehaviour { public int stepsToSide; public float sideStepUnits; public float downStepUnits; public float timeBetweenSteps; // Start is called before the first frame update void Start() { // StartCoroutine(MoveMother()); // StartCoroutine(SendABomb()); } // Update is called once per frame void Update() { } public void StartTheAttack() { StartCoroutine(MoveMother()); StartCoroutine(SendABomb()); } public void StopTheAttack() { StopAllCoroutines(); } public IEnumerator MoveMother() { // define our side step vector Vector3 moveVector = Vector3.right * sideStepUnits; // repeat this while (transform.childCount > 0) { // side move sequence for(int i = 0; i < stepsToSide; i++) { // move to the side transform.position = transform.position + moveVector; // wait for the next move yield return new WaitForSeconds(timeBetweenSteps); } // move down transform.position += (Vector3.down * downStepUnits); yield return new WaitForSeconds(timeBetweenSteps); // flip the direction moveVector *= -1; } } public IEnumerator SendABomb() { float timeBetweenBombs = 8.7f; bool isRunning = true; while (isRunning) { // see how many child objects there are int enemyCount = transform.childCount; // if there are children... if (enemyCount > 0) { // pick one at random int enemyIndex = Random.Range(0, enemyCount); // get the object Transform thisEnemy = transform.GetChild(enemyIndex); // make sure it has the Enemy component Enemy enemyScript = thisEnemy.GetComponent<Enemy>(); if (enemyScript) { // drop the bomb enemyScript.DropABomb(); } } else { // .. and if no children, stop running isRunning = false; } yield return new WaitForSeconds(timeBetweenBombs); } } }