Today we implemented our game flow using another singleton, the GameManager, to coordinate this journey.
Part 1: Game Flow
Yesterday, we mapped out our game flow so that we know what states we intend to use, and how we can move between them. We implemented some (but not all) of these steps, and those that remain are your responsibility for this week’s assignment.
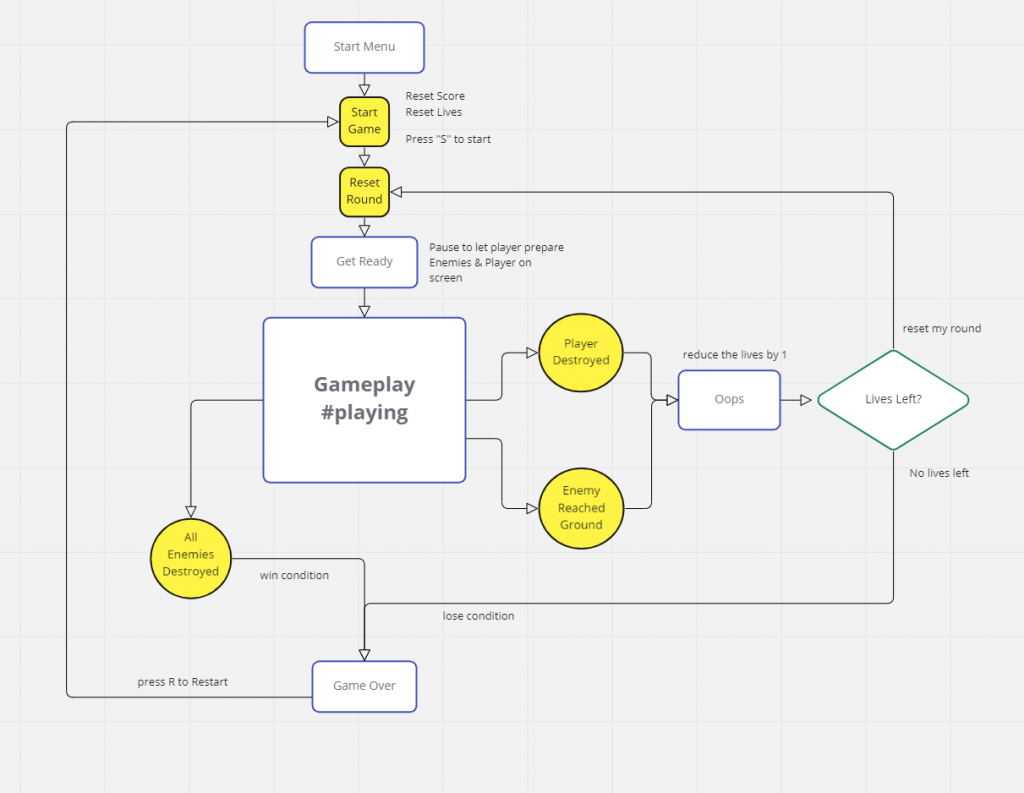
- We start in an inert state that invites us to “Press S to Start”. This is the Menu state and eventually we will replace it with a separate scene with way better UI.
- Pressing S takes us to a new game, where the number of lives are set, and the objects for the first round are prepared. Once ready, we will go into the GetReady state and hold there momentarily.
- Upon completion of the GetReady state, we move into Playing,
- During Playing, the player will have control of the ship and be able to shoot, and the enemies will begin their attack. There are three possible ways to exit this state.
- The first way occurs in case of a win condition – the player destroys all of the enemy ships – and are taken to the GameOver state with a win message.
- The other two methods are losing conditions – the player object is destroyed by an enemy, or the enemy ship reaches the ground. In this case, the player loses a life, and the round is reset after an oops state, or the game is over if the player has run out of lives.
The first step to build out this structure is for us to create our GameManager object (again created from an empty gameobject), and create the GameManager.cs script for it.
We declare our variable to hold the Singleton…
public static GameManager S; // set up the singleton
… and we assign it. This time, we add in some extra protection to make sure that a version of this singleton does not already exist. This can happen when we move between scenes, if we were to preserve our GameManager object, we might enter a scene that already has a GameManager inside of it. We will test for the instance and destroy if we find one already in place.
private void Awake() { if (GameManager.S) { // singleton already exists, destroy this instance Destroy(this.gameObject); } else { // define the singleton S = this; } }
Next we define a number of enumerated values for our various gamestates:
public enum GameState { menu, getReady, playing, oops, gameOver};
To implement Step 1, we set up a GameState variable in our script and test against it at appropriate times. For instance, pressing “S” would mean one thing during our Menu stage (it should be interpreted as the “start” command) but might mean something different during gameplay, if we are using our Input.GetAxis(“Vertical”), which relies on pressing WASD keys. And for the assignment, you can do the same sort of check for the GameOver state looking for a user to press the R key to restart.
void Update() { if (gameState == GameState.menu) { // game is in menu state, press S to start will advance if (Input.GetKeyDown(KeyCode.S)) { StartANewGame(); } } else if (gameState == GameState.gameOver) { // press r to restart the game } }
We can begin to set up the stages. We created a StartNewGame() function that resets the lives left (and later will also reset the score, which is part of this week’s assignment)
We also create ResetRound( ) and StartRound( ) functions. ResetRound( ) is Step 2, and will place the block of enemies in the starting position. We make our Mothership object a prefab, and remove it from the scene, but add the prefab to a GameObject variable in this script. ResetRound instantiates the prefab and stores it in another GameObject variable that is our “currentMothership”. Before we instantiate, we test to see if one already exists. If this returns true, then we probably have arrived here after a player lost a round. We want to reset the enemy objects, so we destroy whatever already exists.
private void ResetRound() { // spawn a new set of enemies if (currentMotherShip) { Destroy(currentMotherShip); } currentMotherShip = Instantiate(motherShipPrefab); // put us in the get ready state gameState = GameState.getReady; // run the GetReadyState coroutine StartCoroutine(GetReadyState()); }
Next we transition to the GetReady state, and kicking off a coroutine that will let us pause for 3 seconds, then trigger StartRound( ), which completes Step 3. StartRound( ) moves us to the Playing state, and we remain here until it is time to end a round.
Now that we can get to the playing state, it is time to adjust some of our object behaviors, and make them state dependent.
First, we want to restrict the player movement so that they can only move and shoot while we are in the Playing state. To accomplish this, we add a gamestate check in the Player’s update function. By wrapping the contents with the following “if” statement, we ensure that motion only occurs while in the Playing state.
if (GameManager.S.gameState == GameState.playing) { ... }
Again, note that there is no need to associate the game manager with any object in our Player script, and no references to be made. “GameManager.S” is available to be called globally.
The next problem is that our enemy object starts moving and shooting during the get ready state. I also don’t like that it continues to move after my player has been destroyed. I want to prevent the possibility of multiple state requests coming in while we are in our Oops state, so I am going to create new scripts in the Enemy object that will be responsible for starting and stopping the coroutines.
Since I am instantiating the Mothership in GameManager, I have access to the object that is the instance in our scene. I add public functions to StartTheAttack( ) [which starts the coroutines previously held in Start( )] and StopTheAttack( ) in the MotherShip.cs script, and then call those from GameManager using:
currentMotherShip.GetComponent<MotherShip>().StopTheAttack();
In our StopTheAttack( ) function, we call the StopAllCoroutines( ) function. This will cease any coroutines currently running from the component.
public void StopTheAttack() { StopAllCoroutines(); }
Next, we implemented one of the three conditions that will get us out – specifically the case where an enemy bomb hits the player. We created a PlayerDestroyed( ) function and then call it from a OnCollisionEnter that we add to the Player script.
private void OnCollisionEnter(Collision collision) { if (collision.transform.tag == "EnemyBomb") { // make the player explode Destroy(this.gameObject); // make the boom boom noise SoundManager.S.MakePlayerExplosion(); // inform the manager that the player exploded GameManager.S.PlayerDestroyed(); } }
Now when the Player collides with an EnemyBomb, the PlayerDestroyed( ) script is called on the GameManager. PlayerDestroyed removes a life from the count, and then moves us to the Oops state, through a coroutine.
public void PlayerDestroyed() { // remove a life livesLeft--; // go to oops state StartCoroutine(OopsState()); } public IEnumerator OopsState() { // put the game in oops state gameState = GameState.oops; // tell the enemy to stop their attack currentMotherShip.GetComponent<MotherShip>().StopTheAttack(); // pause yield return new WaitForSeconds(2.0f); // decide if we restart or game over if (livesLeft > 0) { // restart our round ResetRound(); } else { // go to game over state gameState = GameState.gameOver; } }
We start the OopsState coroutine by putting the game into the “oops” gamestate, and we the Mothership to stop moving and dropping bombs. Then we yield activity for a few seconds to pause the action. During this time, each object’s Update( ) command will be called on each frame. This is why we move into a different game-state, and have objects test against that state – because the game engine keeps running even when our game flow is taking a rest. We use these variables to help the other objects exhibit the proper behavior.
GameManager.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; public enum GameState { menu, getReady, playing, oops, gameOver}; public class GameManager : MonoBehaviour { // singleton declaration public static GameManager S; // set the initial gamestate public GameState gameState = GameState.menu; // set score and lives public int score = 0; private int livesLeft = 0; private int LIVES_AT_START = 3; // prefabs & objects for mothership public GameObject motherShipPrefab; private GameObject currentMotherShip; private void Awake() { if (S) { // destroy the duplicate Destroy(this.gameObject); } else { // define the singleton S = this; } } // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (gameState == GameState.menu) { // game is in menu state, can use press S to advance if (Input.GetKeyDown(KeyCode.S)) { // start a new game StartANewGame(); } } else if (gameState == GameState.gameOver) { // in game over state, can press R to restart // YOUR CODE GOES HERE } } private void StartANewGame() { Debug.Log("Entering Start A New Game"); // reset lives and score score = 0; livesLeft = LIVES_AT_START; // reset for the first round ResetRound(); } private void ResetRound() { Debug.Log("Resetting Round"); // spawn the enemy (instatiate) if (currentMotherShip) { Destroy(currentMotherShip); } // destroy the old instance if it still exists. currentMotherShip = Instantiate(motherShipPrefab); // spawn the player (your work here) // put us into the get ready state gameState = GameState.getReady; // start the get ready coroutine StartCoroutine(GetReadyState()); } public IEnumerator GetReadyState() { Debug.Log("In GetReady State"); // set up some messages on the screen to get ready // pause for a few seconds yield return new WaitForSeconds(3.0f); // turn off the get ready message // start the round StartRound(); } private void StartRound() { Debug.Log("Starting Round"); // start the attack currentMotherShip.GetComponent<MotherShip>().StartTheAttack(); // set the gamestate to playing gameState = GameState.playing; } public void PlayerDestroyed() { // go to oops state StartCoroutine(OopsState()); } public IEnumerator OopsState() { // change to the oops state gameState = GameState.oops; // reduce the lives by 1 livesLeft--; Debug.Log("Lives Remaining = " + livesLeft); // put up the oops message // tell the mothership to stop the attack currentMotherShip.GetComponent<MotherShip>().StopTheAttack(); // pause yield return new WaitForSeconds(2.0f); // decide if game over or reset round if (livesLeft > 0) { // restart the round ResetRound(); } else { // go to the game over state gameState = GameState.gameOver; // GameOver(); } } }
Player.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Player : MonoBehaviour { public float speed; public float MAX_OFFSET; public GameObject bulletPrefab; // Update is called once per frame void Update() { if ((GameManager.S.gameState == GameState.playing) || (GameManager.S.gameState == GameState.getReady)) { // move the player object Vector3 currentPosition = transform.position; currentPosition.x = currentPosition.x + (Input.GetAxisRaw("Horizontal") * speed * Time.deltaTime); // clamp our value currentPosition.x = Mathf.Clamp(currentPosition.x, -MAX_OFFSET, MAX_OFFSET); // return the final position transform.position = currentPosition; if ((Input.GetKeyDown(KeyCode.Space)) && (GameManager.S.gameState == GameState.playing)) { FireBullet(); } } } private void FireBullet() { Instantiate(bulletPrefab, (transform.position + Vector3.up), Quaternion.identity); } private void OnCollisionEnter(Collision collision) { if (collision.transform.tag == "EnemyBomb") { // make the player explode // Destroy(this.gameObject); // make the explosion noise SoundManager.S.PlayerExplosion(); // inform the game manager that the player has been destroyed GameManager.S.PlayerDestroyed(); } } }
MotherShip.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; public class MotherShip : MonoBehaviour { public int stepsToSide; public float sideStepUnits; public float downStepUnits; public float timeBetweenSteps; public float timeBetweenBombs; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { } public void StartTheAttack() { StartCoroutine(MoveMother()); StartCoroutine(SendABomb()); } public void StopTheAttack() { StopAllCoroutines(); } public IEnumerator MoveMother() { // define the side step vector Vector3 moveVector = Vector3.right * sideStepUnits; // repeat the movement while this object has children while (transform.childCount > 0) { // move to the side (sequence) for (int i = 0; i < stepsToSide; i++) { // move to the side transform.position = transform.position + moveVector; // wait for the next move yield return new WaitForSeconds(timeBetweenSteps); } // now move down transform.position += (Vector3.down * downStepUnits); yield return new WaitForSeconds(timeBetweenSteps); // flip the direction moveVector *= -1.0f; } SoundManager.S.StopTheMusic(); Debug.Log("End of MoveMother script reached"); } public IEnumerator SendABomb() { // set the running flag bool isRunning = true; while(isRunning) { // see how many child objects exist int enemyCount = transform.childCount; // if there are children if (enemyCount > 0) { // pick one at random int enemyIndex = Random.Range(0, enemyCount); // get the chosen object Transform thisEnemy = transform.GetChild(enemyIndex); // make sure this has the Enemy component Enemy enemyScript = thisEnemy.GetComponent<Enemy>(); if (enemyScript) { // drop a bomb enemyScript.DropABomb(); } } else { // there are no children isRunning = false; } // wait for that time yield return new WaitForSeconds(timeBetweenBombs); } } }