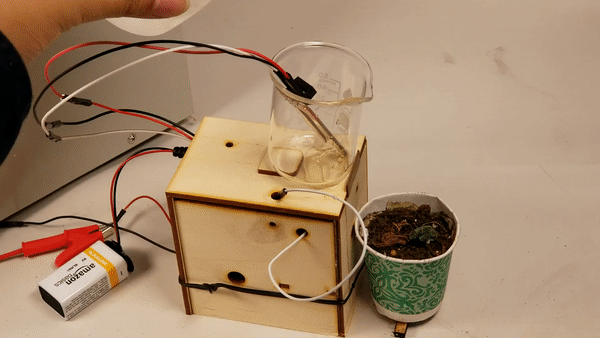
The contraption in action
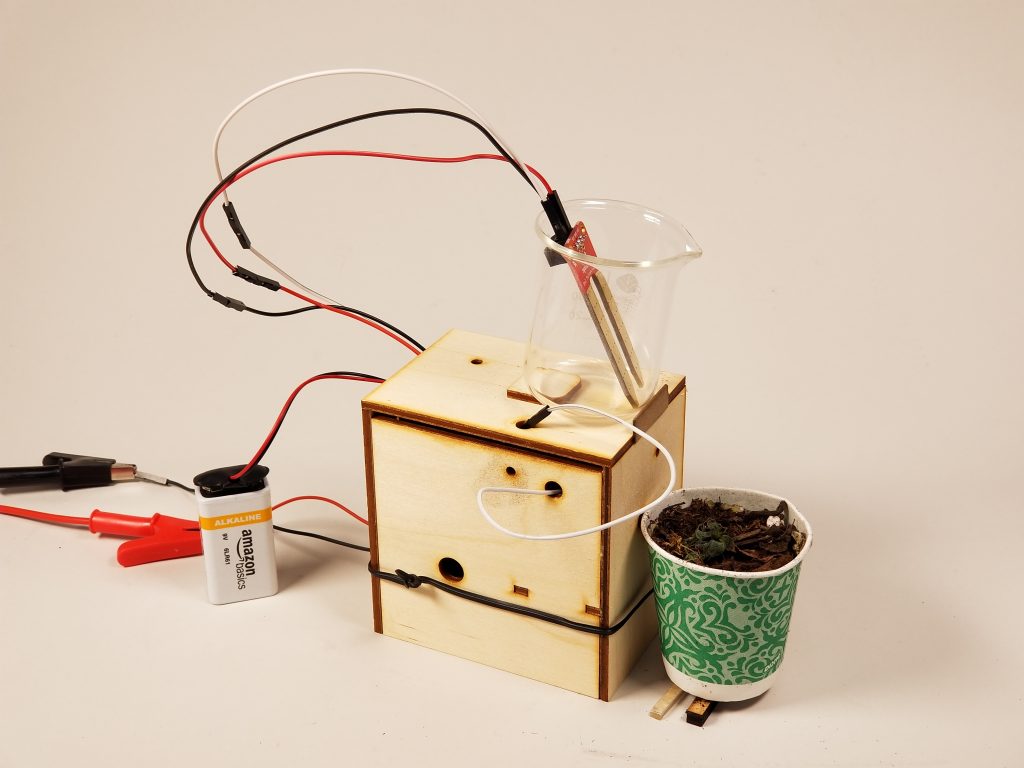
The final contraption without solenoid power source: A beaker rests on top of a wooden box with a moisture sensor and a plant in a cup is placed on the side of the box, covering the solenoid. 9V battery is used to power the Arduino in the box.
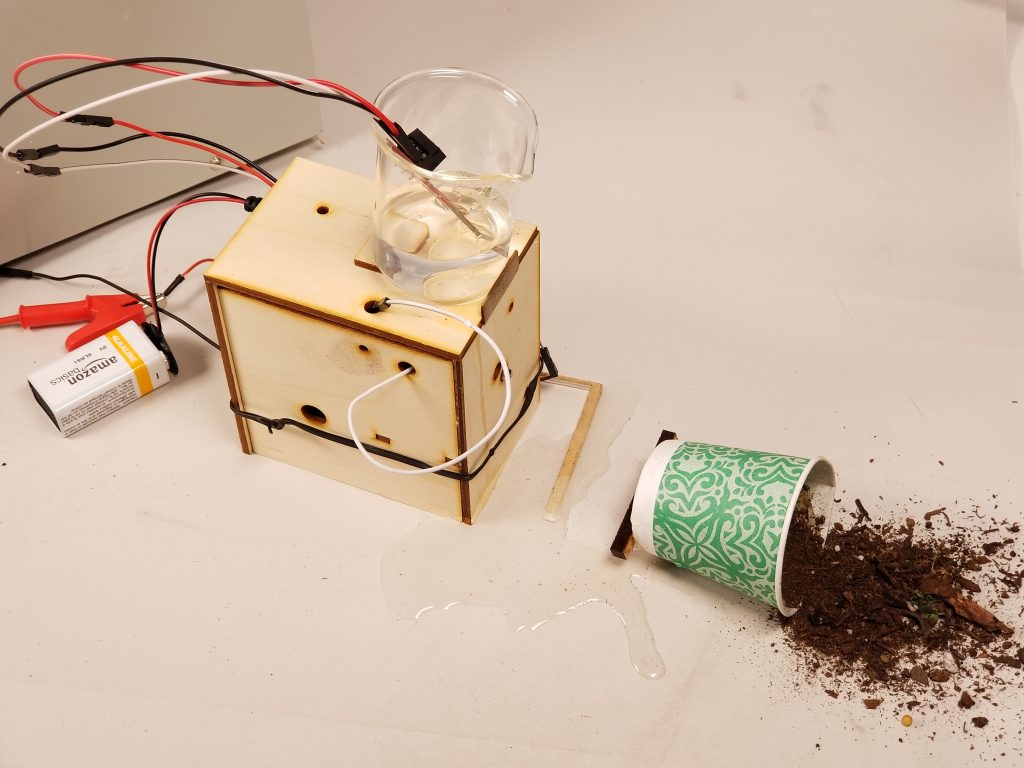
The tragic aftermath: water is added to the beaker, the solenoid has knocked over the plant cup.
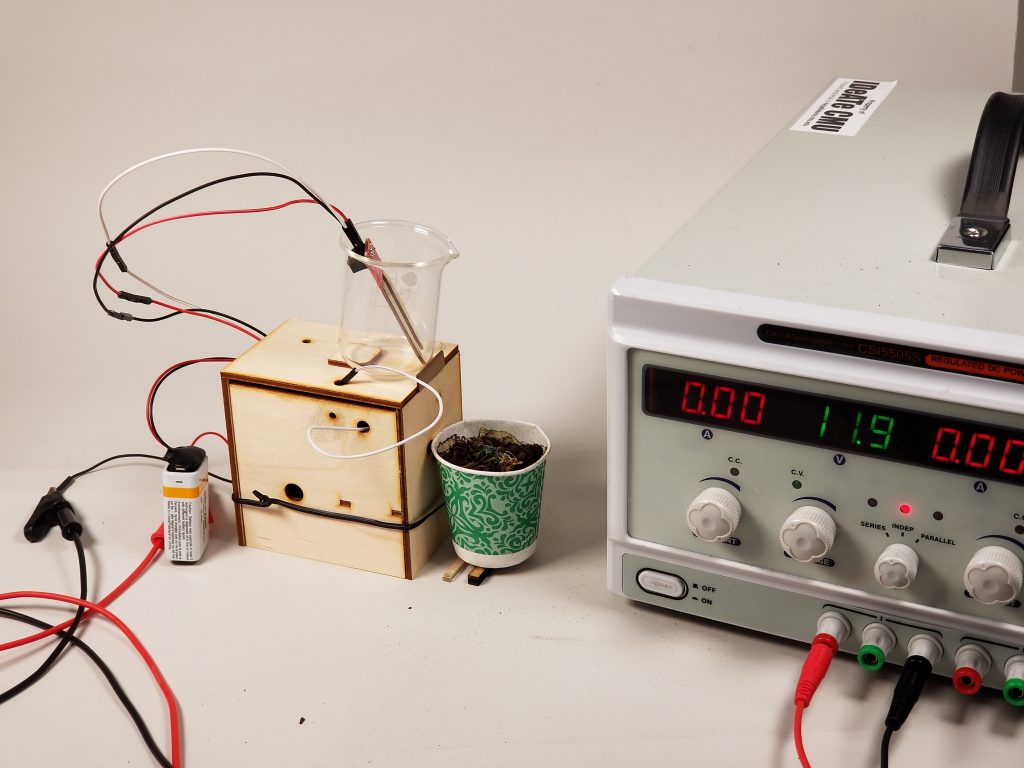
Solenoid needs 12 volts of power to run
This is a box. Not just an ordinary box, but a tiny wooden box with a glass beaker on top, a potted plant to its side, and electronics on the inside. When the user pours water into the beaker, a sensor that is in the beaker detects that there is water in the beaker. This then triggers the solenoid (a small device that punches out a small “arm” with sudden force) which knocks the plant over. The plant must be resting right on the side of the box to both cover the solenoid and be close enough so that the solenoid can reach it.
The process
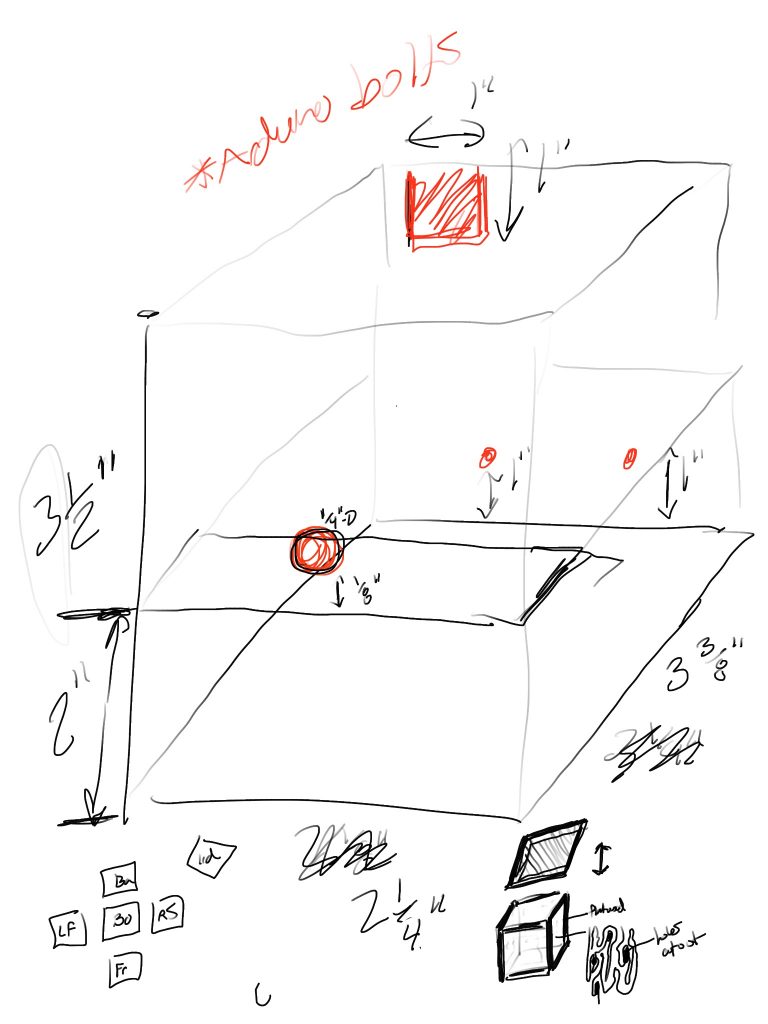
A sketch of the box with measurements for laser cutting. We wanted to make the device as small as we could so every hole and width was measured carefully.
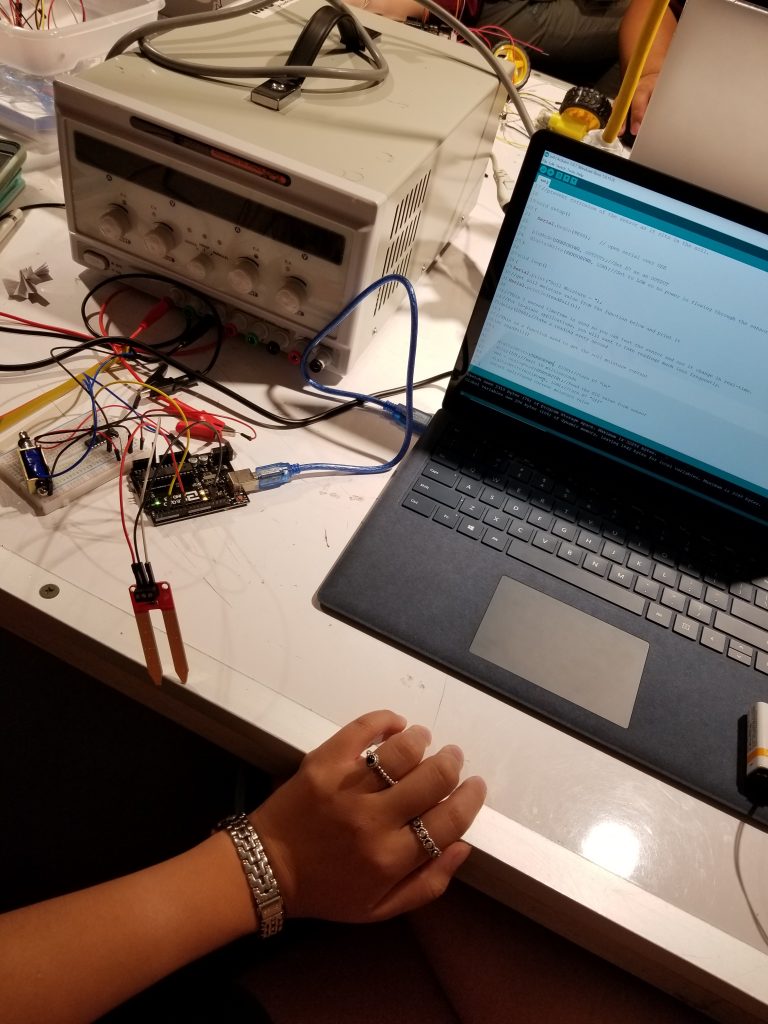
We couldn’t find out why our code wasn’t working to power the solenoid but it turned out to be a wiring problem instead.
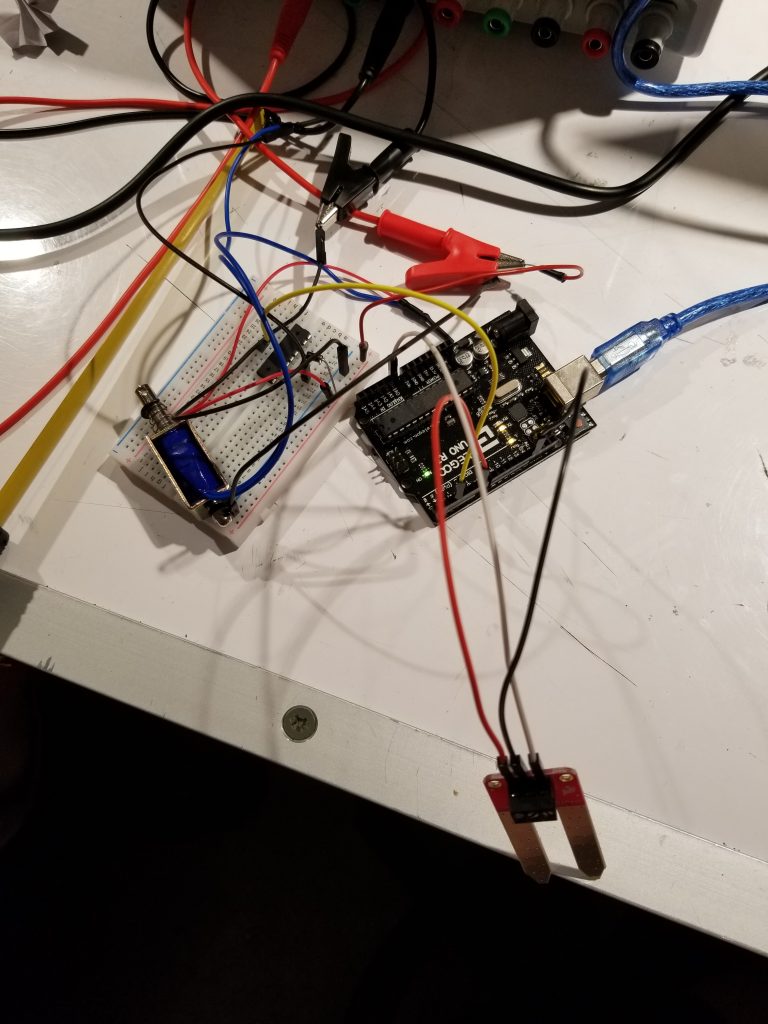
The parts that are on the inside of the box, before we made the box.
Schematic
Code
int val = 0; //value for storing moisture value const int SENSORPIN = A0;//Declare a variable for the soil moisture sensor int SENSORPWR = 7;//Variable for Soil moisture Power const int SOLENOID = 3; void setup() { Serial.begin(9600); // open serial over USB pinMode(SENSORPWR, OUTPUT);//Set Sensor power and solenoid as an OUTPUT pinMode(SOLENOID, OUTPUT); pinMode(SENSORPIN, INPUT);//sensor pin reads input digitalWrite(SENSORPWR, LOW);//Set to LOW so no power is flowing through the sensor digitalWrite(SOLENOID, LOW); } void loop() { int moisture = readMoist(); Serial.print("Moisture = "); //test that moisture sensor is working //get soil moisture value from the function below and print it Serial.println(moisture); if(moisture > 800){//turn solenoid on if moisture detected, but after 3 seconds delay(3000); digitalWrite(SOLENOID, HIGH); Serial.println("solenoid on"); delay(50); digitalWrite(SOLENOID, LOW); Serial.println("solenoid off"); } delay(1000);//take a reading every second } //This is a function used to get the moisture content int readMoist() { digitalWrite(SENSORPWR, HIGH);//turn SENSORPWR "On" delay(10);//wait 10 milliseconds val = analogRead(SENSORPIN);//Read the SIG value form sensor digitalWrite(SENSORPWR, LOW);//turn SENSORPWR "Off" return val;//send current moisture value }
Description
The first challenge we had to face was thinking about what we wanted to build for our project. Our concept was to make something that appeared benign and harmless but had the opposite effect. We thought of “harming” a plant because plants are things that are to be cared for, so you would naturally want to nurture it. We came up with the idea of appearing to care for the plant by giving it water, but the act of watering would punch the plant and knock it over.
In order to do this, we needed a moisture sensor and a solenoid. We chose to work with a solenoid because of its speed and power. However, the solenoid required 12V of power, so we needed an external power source which was big and bulky and detracted from the aesthetic of the piece a little bit. The wiring was a little difficult, and we had to be extra careful because we were working with the 12V power source. Writing the code wasn’t difficult, but we couldn’t figure out what was wrong with it when we ran it. It turned out there was nothing wrong with the code, but we had missed connecting one of the ground wires.
One thing I would modify is to only have the solenoid go off once after the water is poured into the cup, but it could be repeated without having to press restart. The solenoid just kept going off repeatedly 3 seconds after detecting moisture (we created this delay to not have the solenoid go off immediately) until it stopped detecting moisture, which maybe wasn’t the best. It is an easy fix: I could tweak the code so that it keeps track of how many times the solenoid went off, stop after one, and reset the count when the moisture sensor gets back to 0.
The box was laser cut and carefully measured to have all the holes aligned precisely, and be as compact as possible. The potted plant sits on a thin piece of wood so that the solenoid can easily and swiftly knock it over. However, this instability cause some accidental spills. The cup with the plant is also mostly empty with soil only on the very top, again to make it light. We also added some wires on the outside connecting the sides to the top to make it look like the beaker was going to do something, but the wires were not connected to anything on the inside.
Leave a Reply
You must be logged in to post a comment.