Megan Roche & Joey Santillo
The Startle Box is a wooden box with a button on the top face of the box. The box features a button on the top, which when attempted to press, triggers a distance sensor, which in turns opens the lid of the box. The lid of the box opens, revealing a note from within the box, saying “Hi!”.
This project was our first time creating a piece of physical hardware that responded to code, and first tangible result of working with an Arduino. The circuitry is fairly simple as is the concept and the box itself, but it took a lot of effort from us to try and understand how to get the hardware and software to work together. One roadblock that took awhile for us to figure out was how to make the servo motor be able to open the lid of the box. After some researching and playing around, we found that using a popsicle to prop it open worked fairly well. We also used laser printing for the first time, and had to get extensive help with that.
If we had more time with this project, we would probably try to figure out how to make the hinge less obvious, and maybe try to incorporate sound in the final product- we were playing around with having a song play when the lid opened. We could also program the button to do something if someone pressed it, instead of being a dummy. Additionally, we would have like to play around with multiple ultrasonic rangers OR cultivating a better solution for hiding the current ultrasonic ranger.
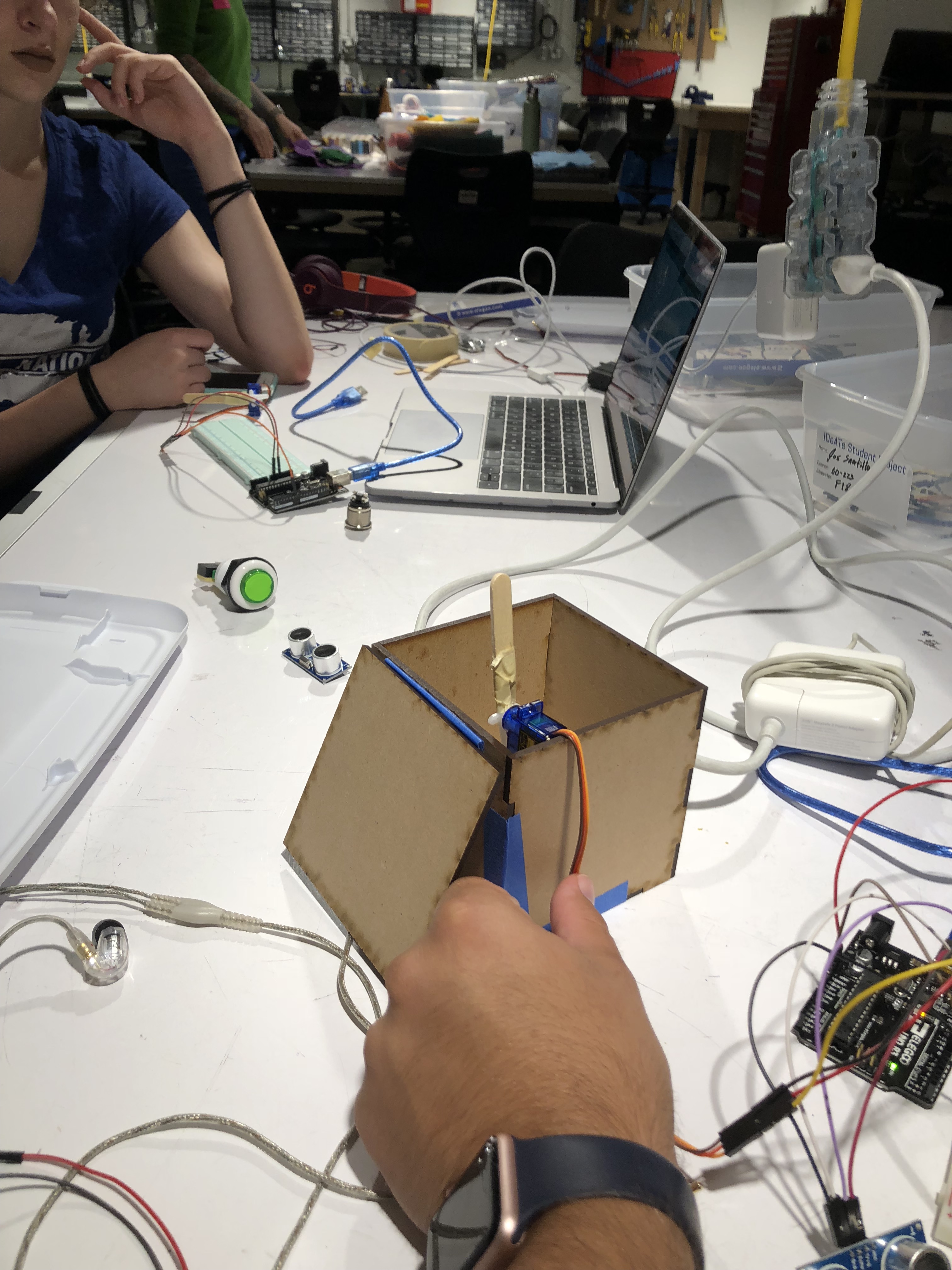
Box, with servo only, pre-hole drilling.
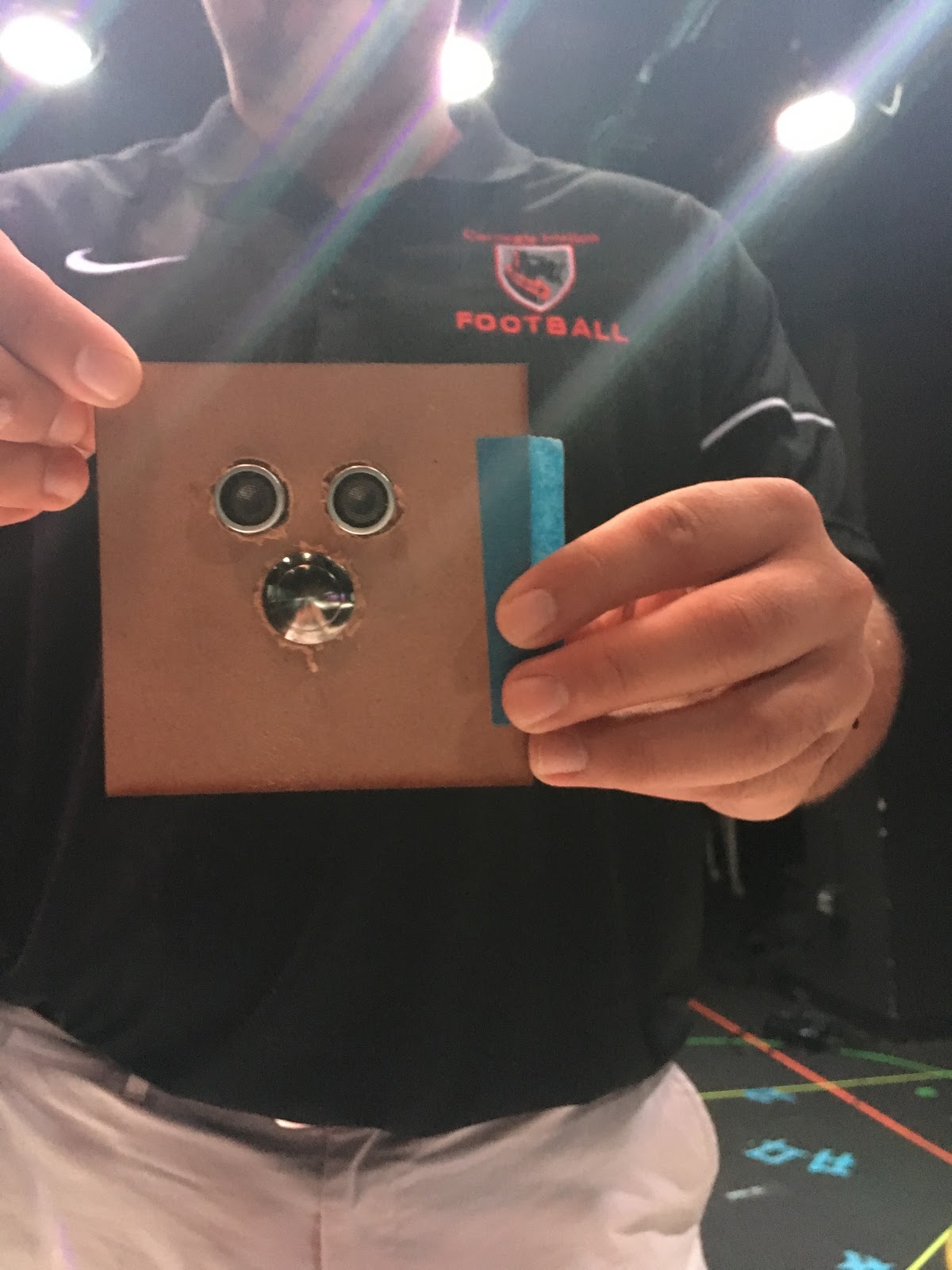
The top of the box, after drilling holes. Initially we thought the drill would create holes large enough for the ultrasonic ranger and button, but we had to spend awhile making the holes bigger.
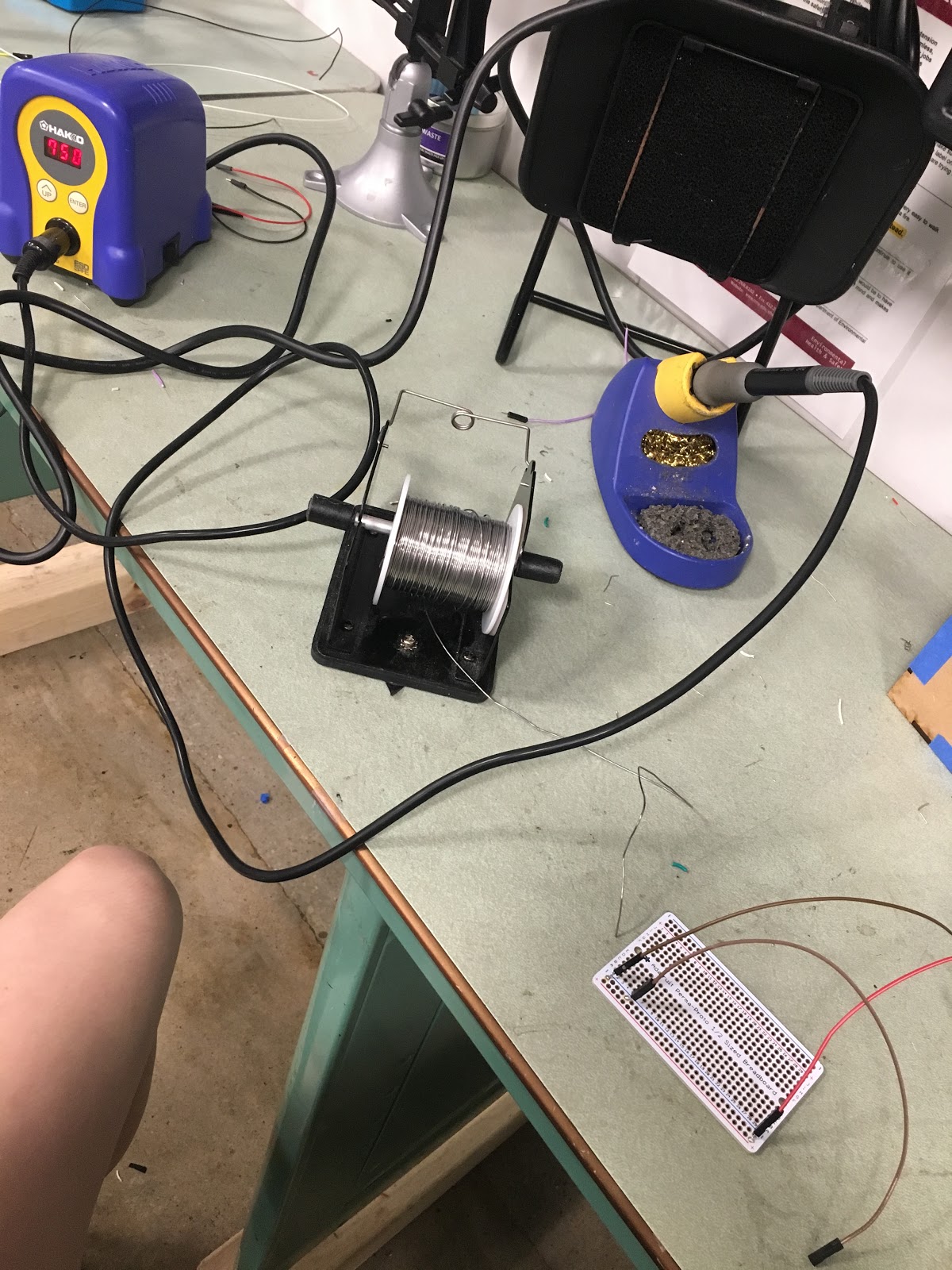
This is the soldering of the bread board we put into the box.
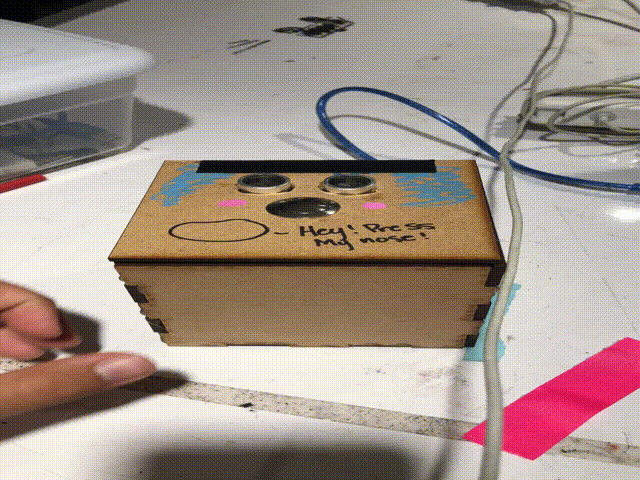
Final Product Gif!
Finished product;
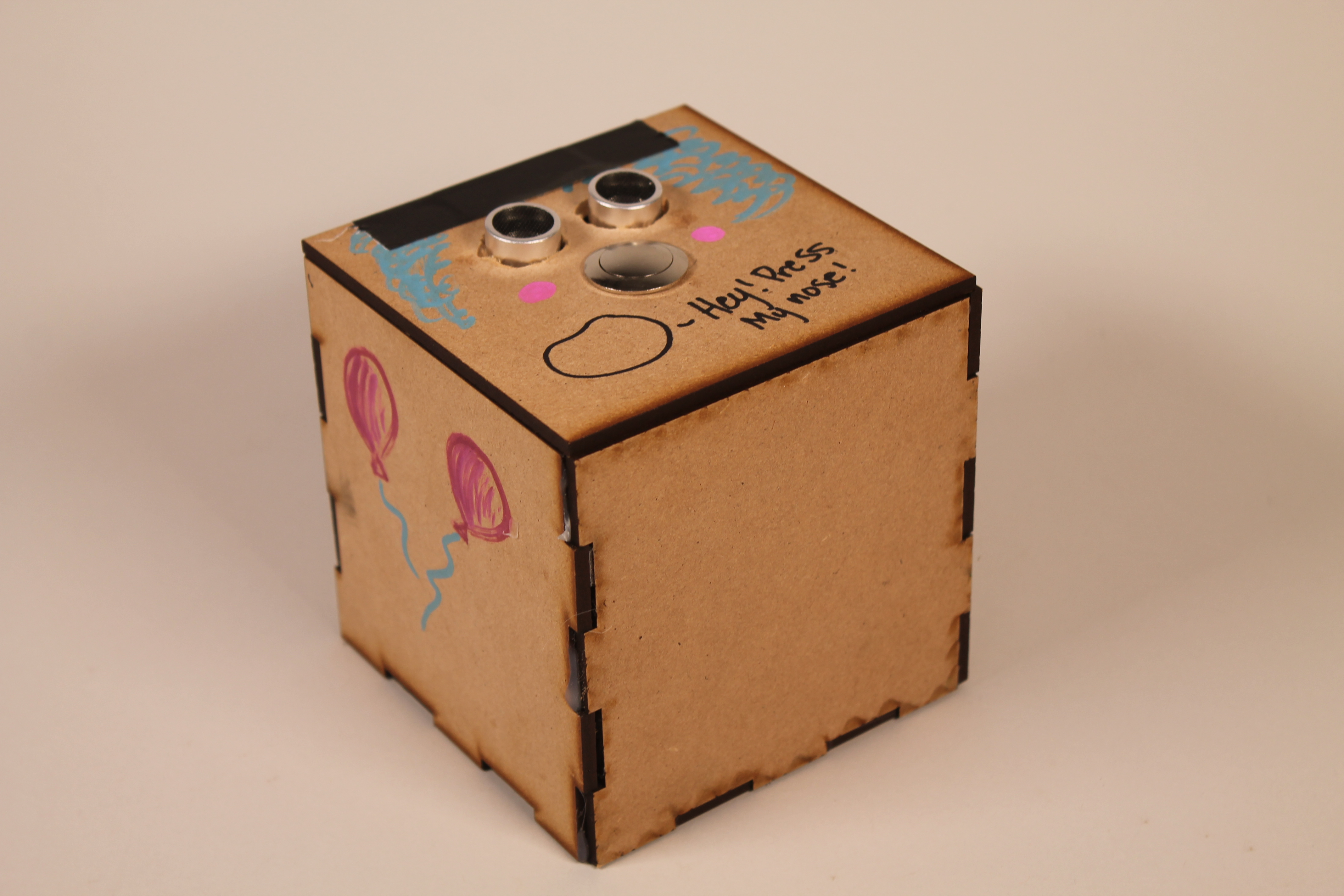
The Startle Box!
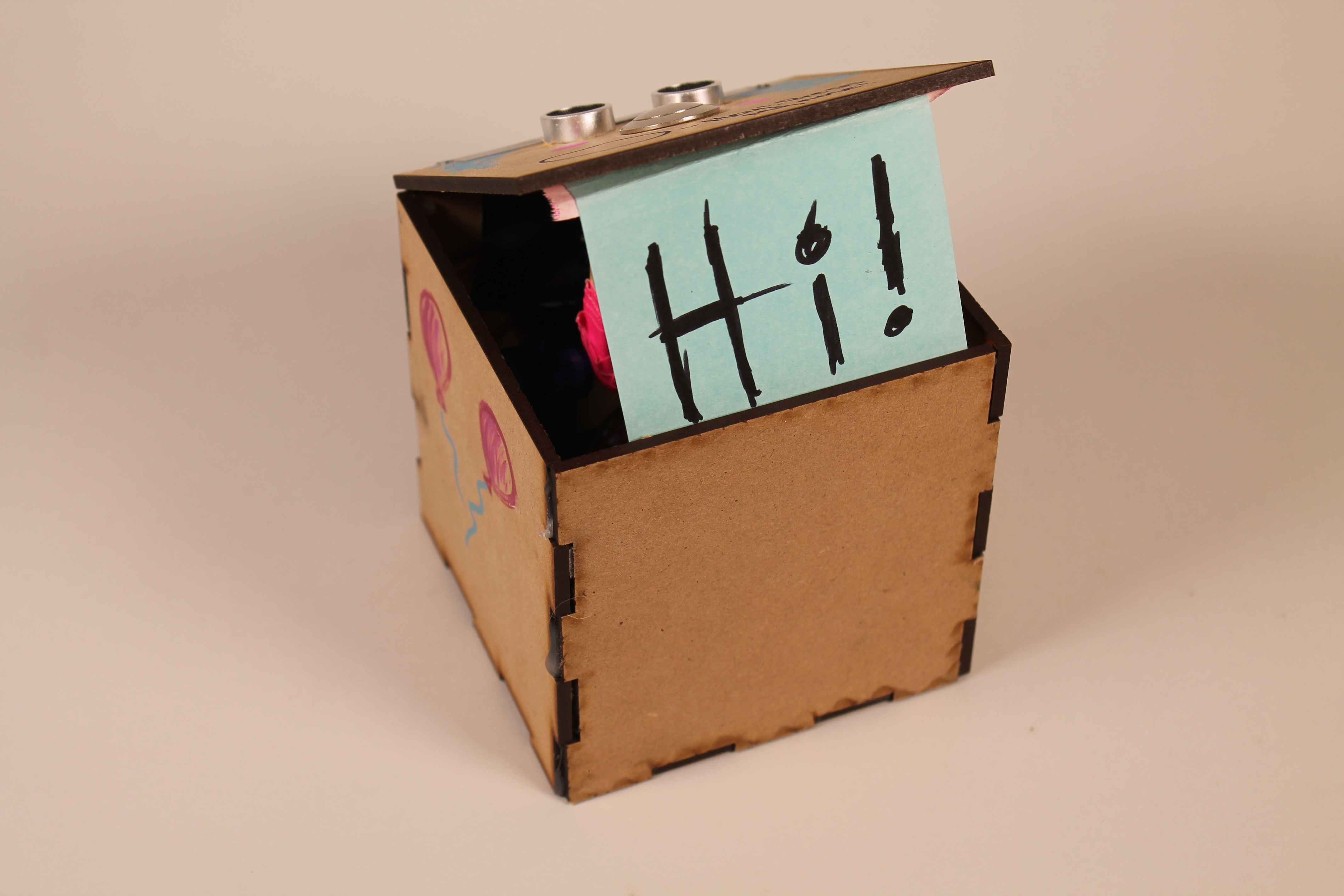
The Startle Box, after being attempted to be touched, diagonal angle.
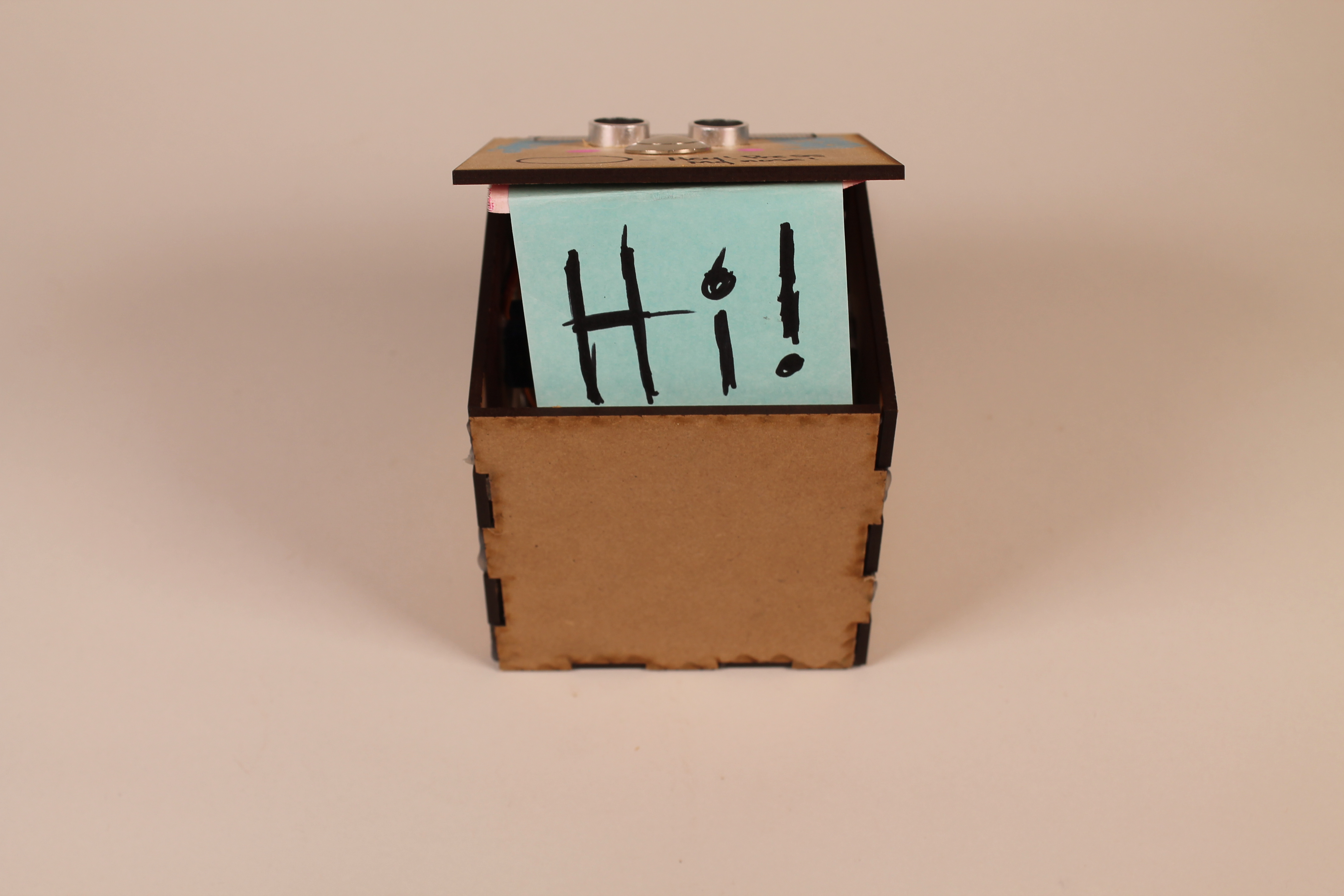
The Startle Box, after being attempted to be touched, front view.
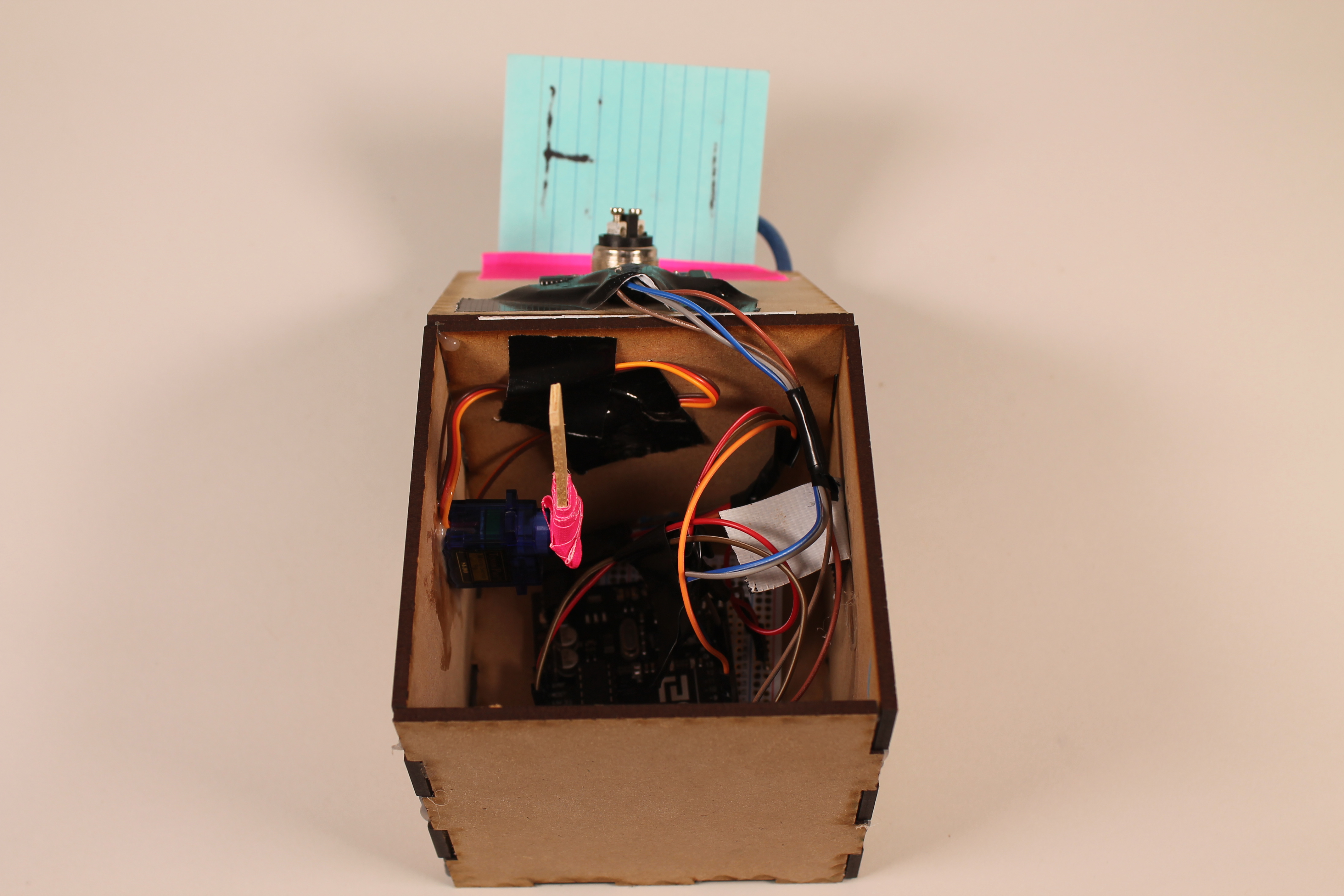
The wiring within the Startle Box.
// --------------------------------------------------------------------------- // Example NewPing library sketch that does a ping about 20 times per second. // --------------------------------------------------------------------------- /* This code will create a box that will "surprise" its user when it opens up as the user gets their hand close. It senses that the user is getting close by using an ultrasonic ranger, and moves the box up by using a servo motor. */ #include <NewPing.h> #include<Servo.h> #define TRIGGER_PIN 12 // Arduino pin tied to trigger pin on the ultrasonic sensor. #define ECHO_PIN 11 // Arduino pin tied to echo pin on the ultrasonic sensor. #define MAX_DISTANCE 200 // Maximum distance we want to ping for (in centimeters). Maximum sensor distance is rated at 400-500cm. NewPing sonar(TRIGGER_PIN, ECHO_PIN, MAX_DISTANCE); // NewPing setup of pins and maximum distance. Servo June; const int JUNEPIN = 7; void setup() { June.attach(JUNEPIN); pinMode(13, OUTPUT); Serial.begin(115200); // Open serial monitor at 115200 baud to see ping results. } void loop() { int handDistance = rangeCheck(); Serial.print("Ping: "); Serial.print(handDistance); // Send ping, get distance in cm and print result (0 = outside set distance range) Serial.println("cm"); if (handDistance <= 30 && handDistance != 0) { // If hand is close June.write(20); // Close box delay(2000); June.write(0); } else { June.write(170); delay(100); } } int rangeCheck() { delay(50); // Wait 50ms between pings (about 20 pings/sec). 29ms should be the shortest delay between pings. int distance = sonar.ping_cm(); if (sonar.ping_cm() < 20) { digitalWrite(13, HIGH); } else { digitalWrite(13, LOW); } return distance; }
Leave a Reply
You must be logged in to post a comment.