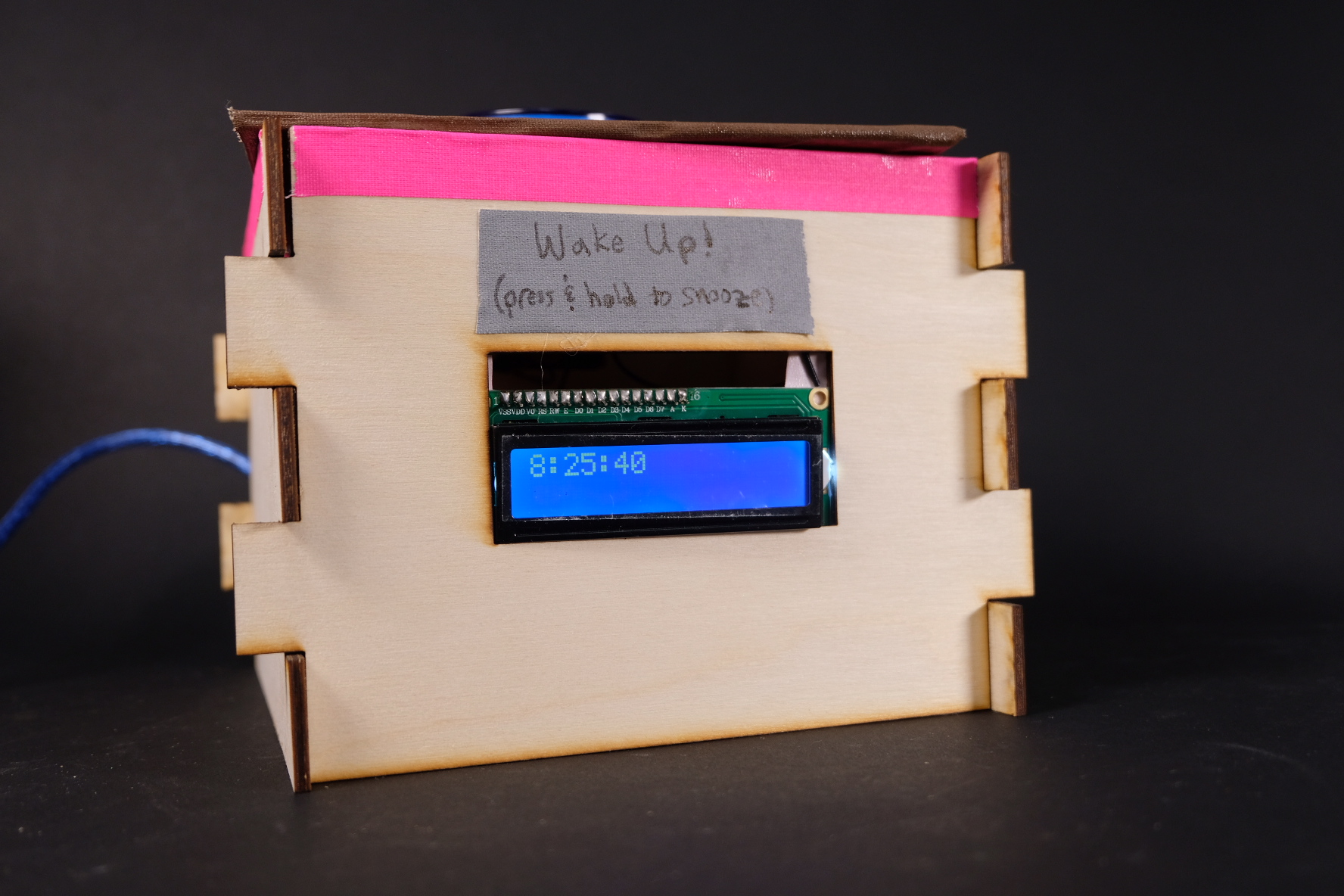
The “alarm clock”
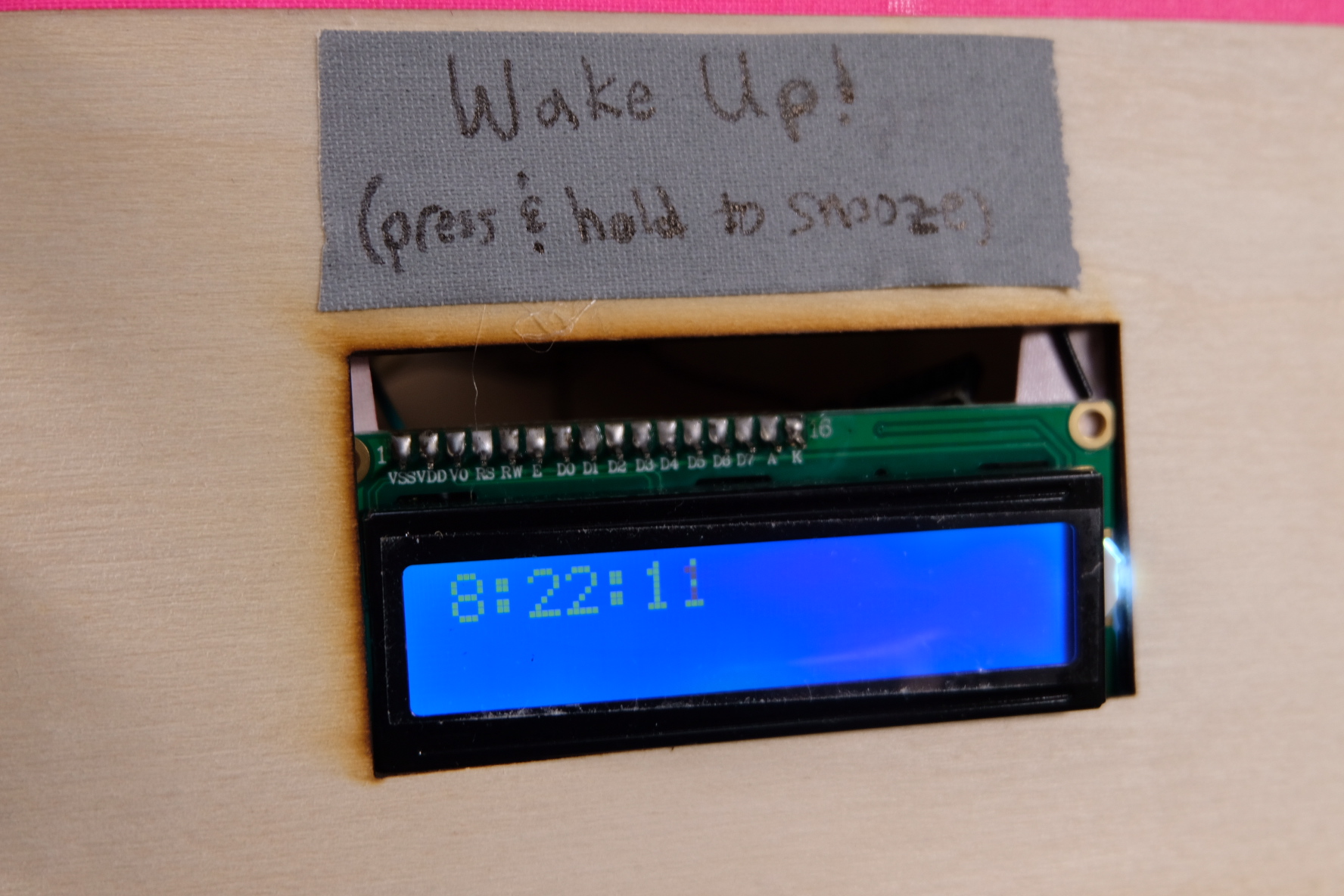
Clock display
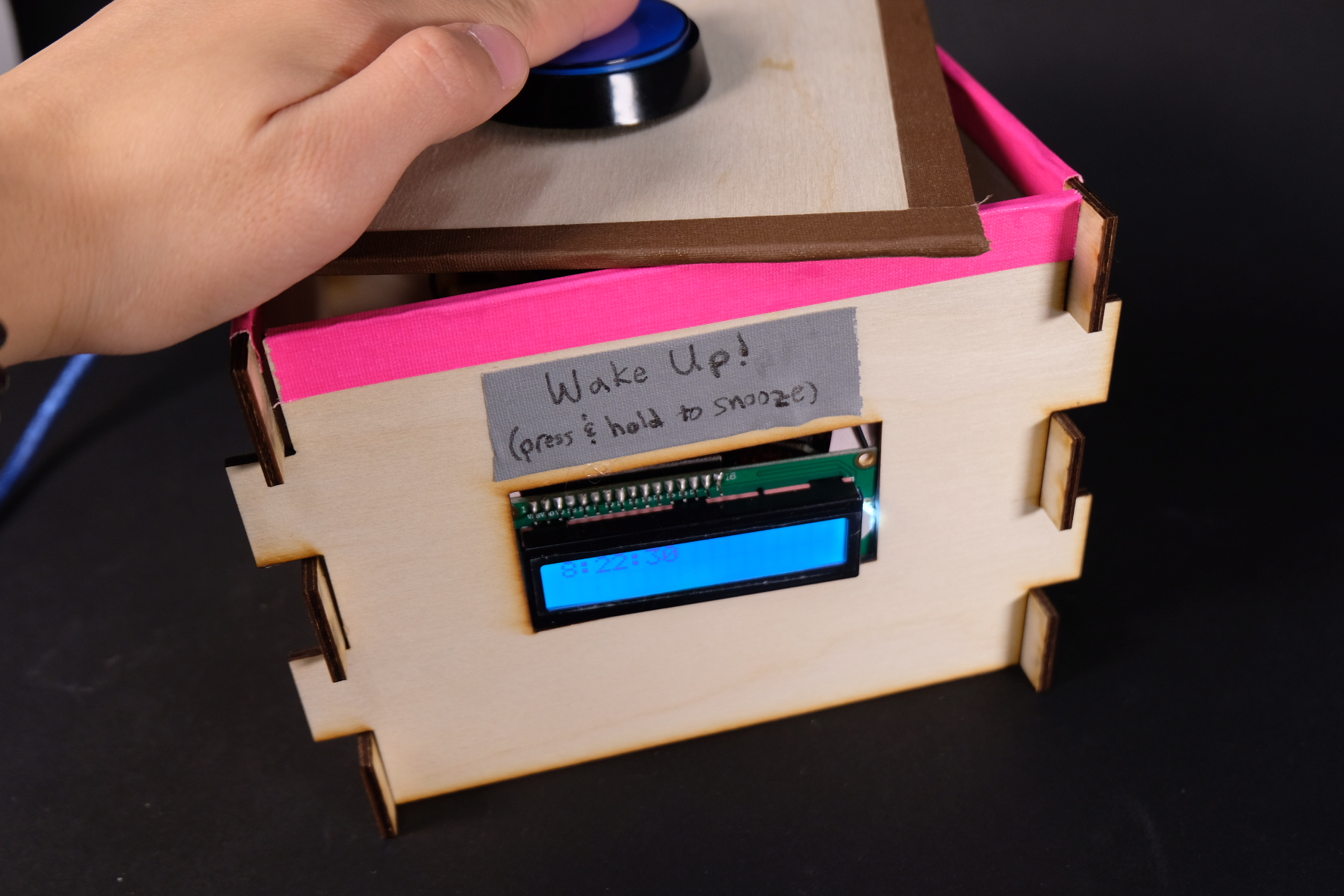
Top of the box with button and some circuitry showing inside
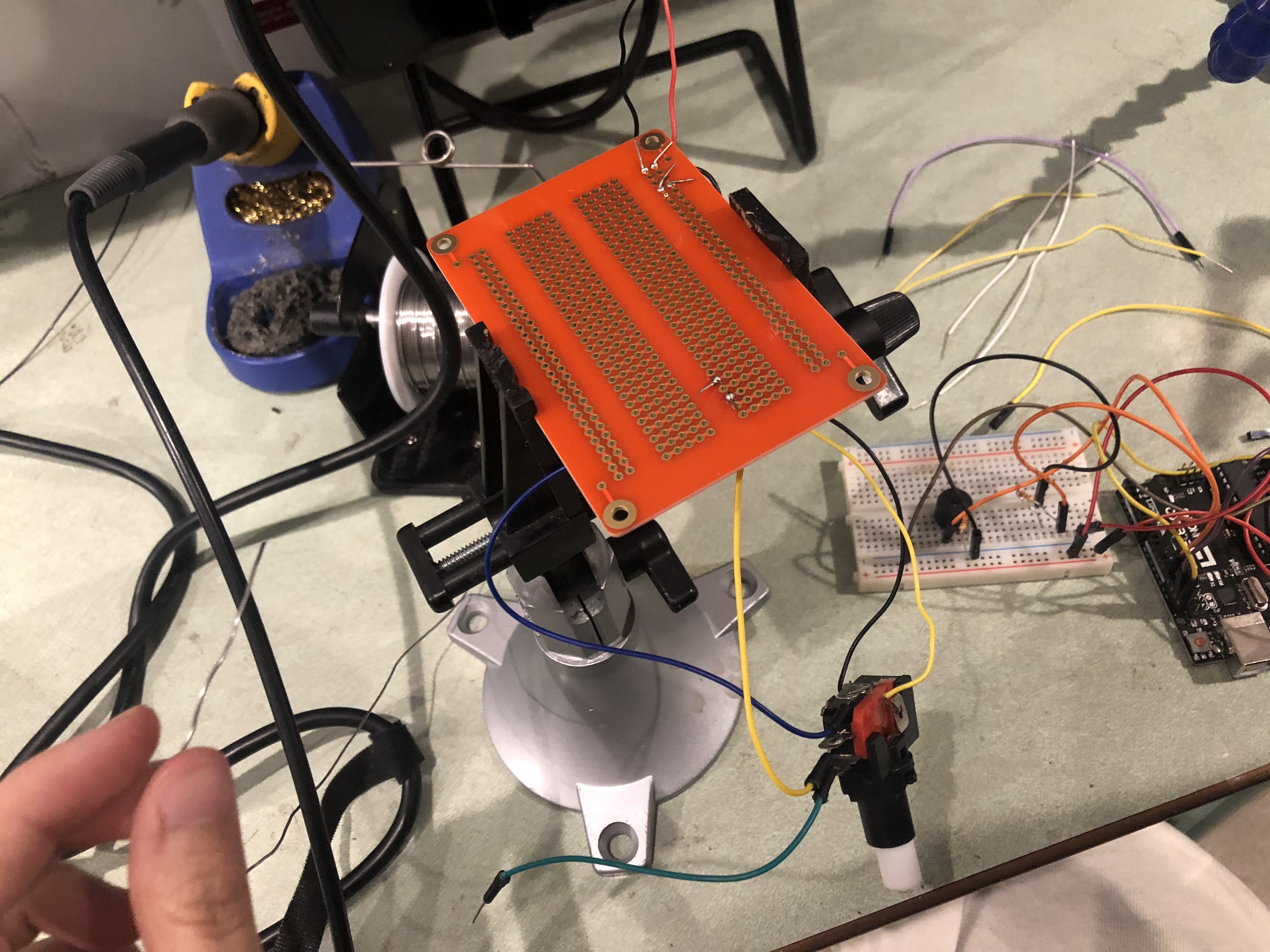
the soldering of the project
Project Description:
The screen on the front displays the time. The box will make a buzzer sound when the display reaches “8:30:0” and there is a button on the top of the box that silences the alarm. The display of time now increases much faster than before.
#include <Adafruit_LiquidCrystal.h> #include <Wire.h> #include <LiquidCrystal_I2C.h> LiquidCrystal_I2C lcd(0x3F,16,2); int hourTime = 8; int minTime = 29; int secTime = 55; int currDelay = 1000; bool alarmOn = false; const int BUZZER_PIN = 13; const int SNOOZE_PIN = 10; void setup() { Serial.begin(9600); lcd.begin(); lcd.backlight(); pinMode(BUZZER_PIN, OUTPUT); pinMode(SNOOZE_PIN, INPUT); digitalWrite(BUZZER_PIN, LOW); } void loop() { lcd.setCursor(0,0); DisplayDateTime(); Serial.println(alarmOn); if(hourTime == 8 && minTime == 30 && secTime == 0){ alarmOn = true; digitalWrite(BUZZER_PIN, HIGH); Serial.println("turn on alarm"); } if(digitalRead(SNOOZE_PIN) == LOW){ alarmOn = false; digitalWrite(BUZZER_PIN, LOW); currDelay = 200; Serial.println("speed up"); } delay(currDelay); lcd.clear(); } void DisplayDateTime(){ secTime += 1; if(secTime == 60){ secTime = 0; minTime += 1; } if(minTime == 60){ minTime = 0; hourTime += 1; } lcd.print(hourTime); lcd.print(":"); lcd.print(minTime); lcd.print(":"); lcd.print(secTime); }
start constructing the wire conncection with a buzzer
working through external libarary of the clock
LiquidCrystal screen works!
running test
laser cut a box for it
inside the box
testing (change the button)
Schematic
testing video (buzzer are replaced by LED)
Discussion:
In creating this project we ran into some trouble with learning the liquidCrystal library, but after fiddling for a bit, we figured out which functions we needed and how we could use them efficiently. The circuitry of the alarm is pretty simple so that wasn’t too hard because we had gone over how to wire up most of these components in class.
In the crit, there was some great input of how we could make this project even better. One specific example was to better implement the snooze button as a “snooze” button – the alarm would go, the person would snooze the alarm and go back to sleep, but the alarm would go off much sooner than the snooze interval because of the sped up time. Another interesting idea was to have the clock be more sneaky with its time jumps – the clock would just skip some numbers every so often instead of visibly running faster.
We liked working on this project because it makes us think about things we take for granted like small appliances – lights, clocks, table, boxes, etc. We purely expect them to work properly because they’re so simple, but when they don’t, then, surprise!
Leave a Reply
You must be logged in to post a comment.