Overview
This device effectively reminds you to put in your contacts lenses when you wake up.
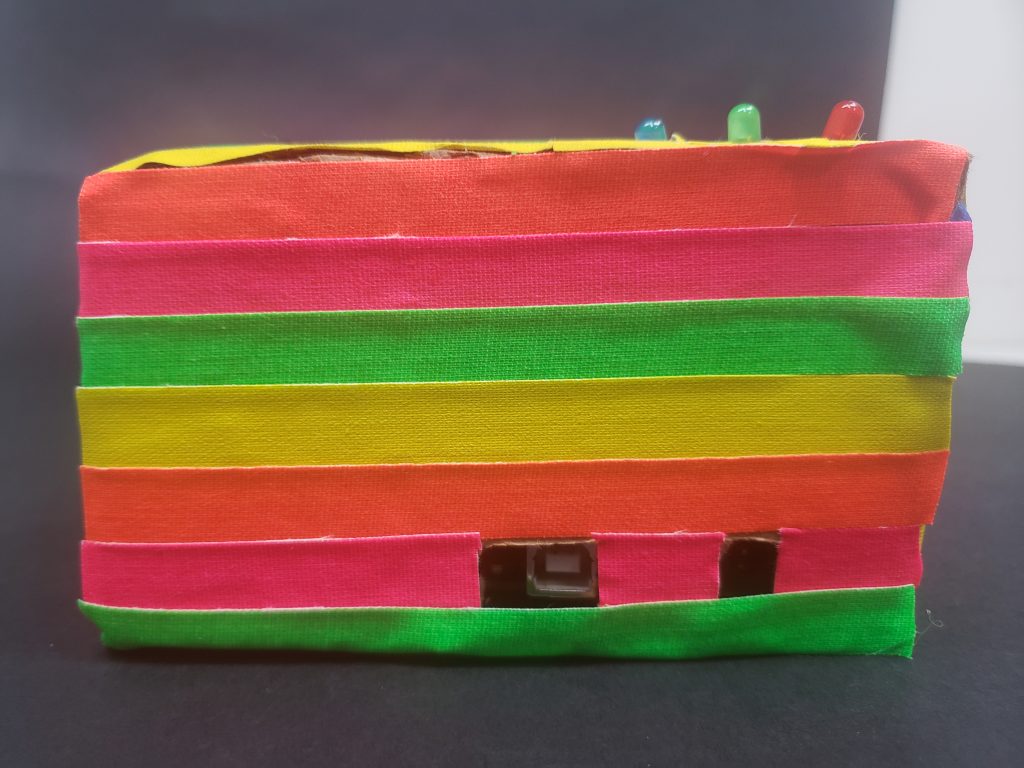
Back View: One port to reprogram and another for power
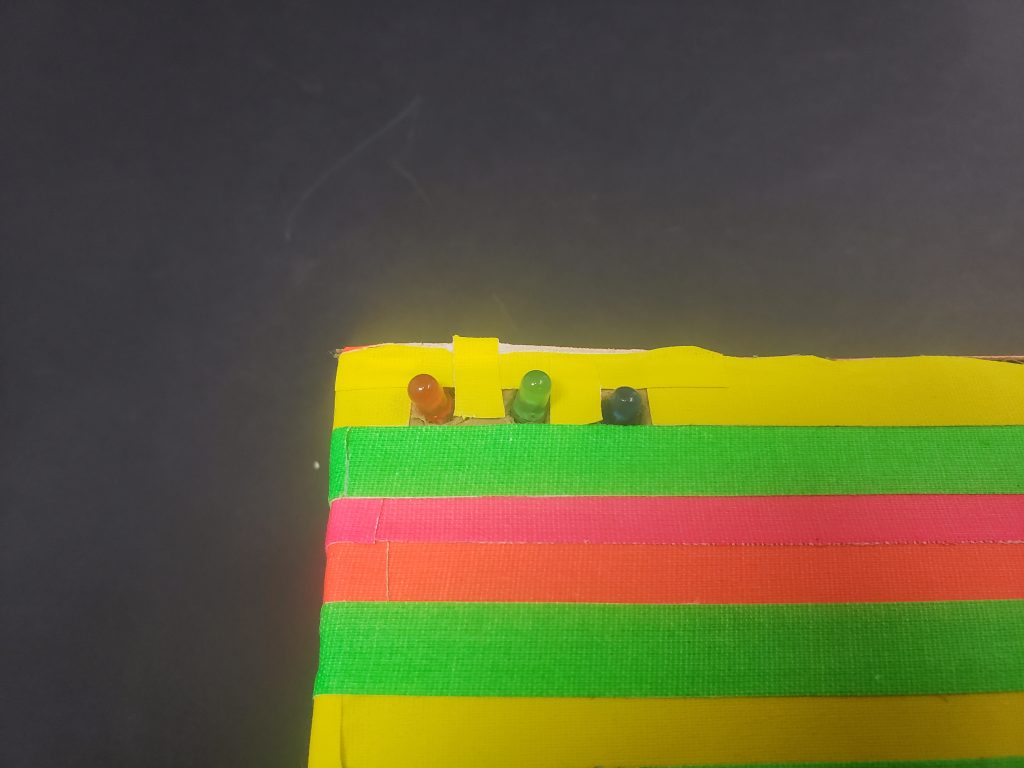
Sample LEDs that blink to remind the user
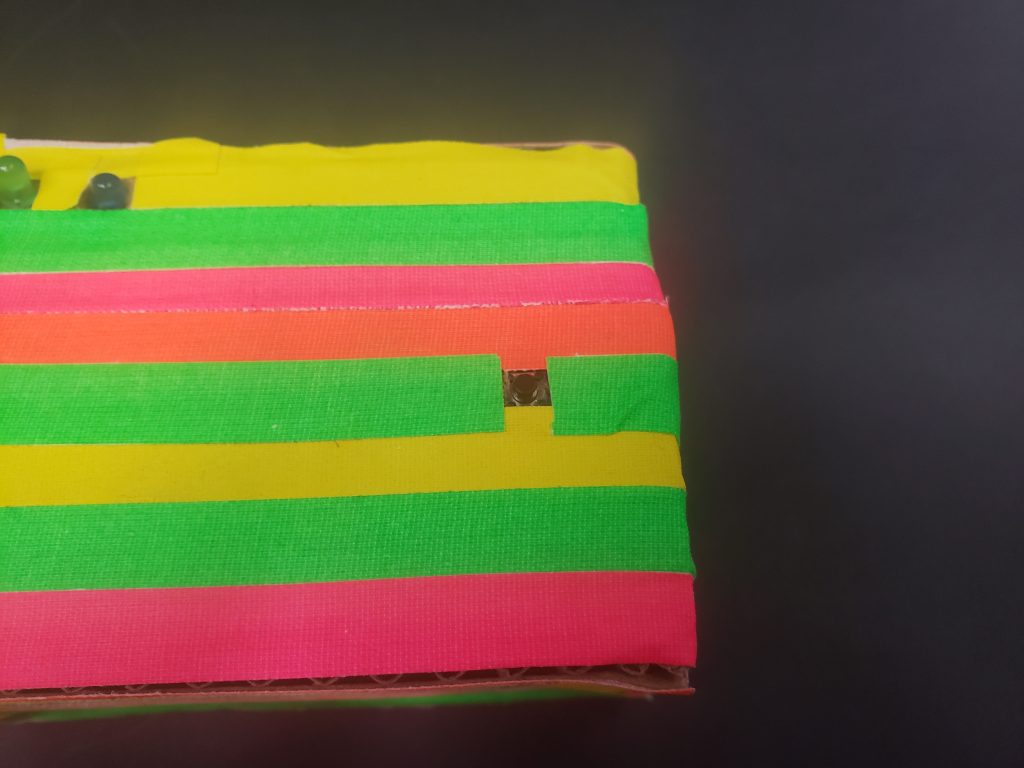
Switch that detects presence of phone
Process Review
One decision point during the process was the choice between using a real time clock or simply another switch to determine when the reminder would be able to activate. If I were to use a switch that the user would have to turn on and off, the device would be more accurate in determining when the user goes to bed, but it’s manual and not automatic which runs the risk of the user forgetting to flip the switch. The real time clock allows the device to automatically know when the user should be asleep based on the time it is set, but the accuracy of the timing relies on the consistency of the user’s sleep schedule. In the end, I decided that the clock has enough accuracy to pinpoint when the user would go to bed, and it’s automatic quality would be more desired.
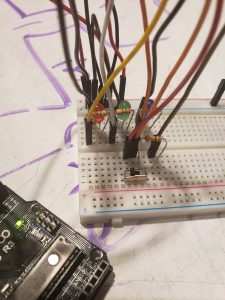
Here is an early prototype with the switch
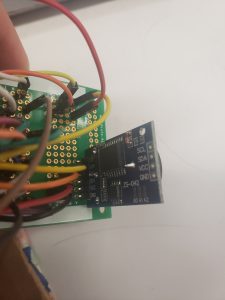
This is part of the final build with the DS3231 module (Clock) attached to protoboard
Another decision point was how the phone button would be optimally implemented into the final build of the device without creating flaw in the design. This was significant because if the button wasn’t efficient in detecting the phone, the whole device would be compromised. I luckily discovered that I could use the inter-structure of cardboard to have space for wires and a place for the button to show to be pressed. This allowed the button to be fully functional without any wires showing.
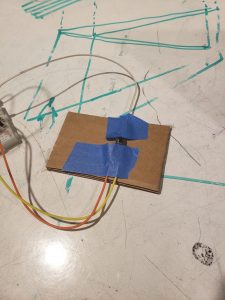
Very early build of phone button
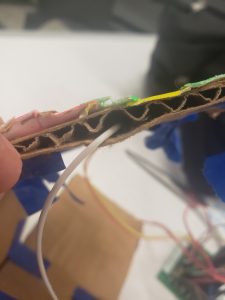
One side of the fed through wire for phone button
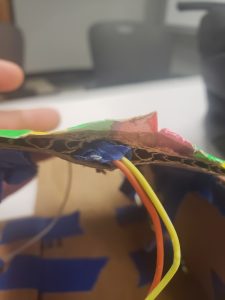
Another side of wire fed through cardboard for phone button
Progress Pictures (cont.)
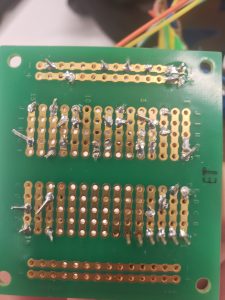
Backside of protoboard
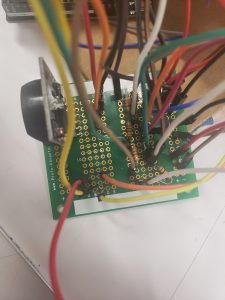
Front side of protoboard
Discussion
Responses:
“I think that the form of the box could be modified to fit the shape and size of the phone more. Is there a way to set the way that the LEDs blink on the fly? The rapid flashing seems like it would be a lot at 8AM.”
Now that I’m looking back on it, a holder for the phone would have been extremely useful and convenient. This would help prevent the phone from somehow falling off or moved enough where the button isn’t triggered anymore. The point of the presence of a phone triggering the LEDs rather than simply at a set time was to increase accuracy in determining when the LEDs turn on. My thinking was that everyone goes everywhere with their phone, and we often have varying schedules, so your phone would act as a better key when you wake rather than time. The purpose of the clock was implemented to automatically “set” the device and to “unset”. Also, the rapid flashing of the LEDs was intended to cause as much annoyance as possible to force the user to remember to put in their contact lenses.
“The minimalistic interface does keep it simple for users, which is always a plus, especially if the users are tired, college students.”
The key characteristics I wanted to highlight in this device were simplicity and effectiveness, so I’m glad that this response and many others indicated the key characteristics. The purpose of implementing these key aspects was because I myself am a tired college student, and lets be honest, I probably wouldn’t need a device to remind me of self-care if I wasn’t a tired college student. That being said I wanted a device where I could roll out of bed and without using my brain, be able to remember to put my contact lenses in, and I think this device does that pretty well.
This device is simple, and it works. I’m satisfied in the aspect that it does everything I wanted it to do. However, after going through the process and gaining feedback, there are many things about my device that could be improved. The core design of the device is good, but each aspect that make up the core design can be improved to produce a much better overall device. I learned how useful peer feedback is. As I went through the process, I had an idea of how to improve parts, but I couldn’t quite make the connection in my head. When I heard feedback from others, I was able to finalize exactly how to improve certain parts. On my next project, I’ll be sure to reach out to more peers during the process of building the device to catch more flaws. The most difficult part of making the device was the design part. This was especially true on the placement of the phone button which I was lucky enough to find a creative spot to put it. I felt like that the design was where I put most of my time into, and in the end, it was the area that could have used the most improvements. I think next time I should make more frequent and less time consuming prototype design builds in order to eliminate more flaws. I don’t plan on building another finalization of this device, but I know how I would improve it if I did. First, I would design a holder to keep the phone in place when placed onto the button. Also, I would change the button placement to be under the middle of the phone rather than on one of its sides. Finally, some of the parameters should be modified in the software to more accurately determine when the device needs to be set. Right now, it only takes in account the hour, but it could also consider the minutes of the hour.
Schematic
Code
// Contact Lens Reminder // Leland Mersky // // This code runs a reminder system in the morning (or any desired // time) that will blink LED's once you take your phone off it to // get up and on with your day. It'll shut off at a set time when // you're not expected to be at home and have no use for it. // // Digital // Red LED Pin 2 // Green LED Pin 3 // Blue LED Pin 4 // Phone Button Pin 5 #include <DS3231.h> DS3231 rtc(SDA, SCL); const int RED_LED_PIN = 2; const int GREEN_LED_PIN = 3; const int BLUE_LED_PIN = 4; const int PHONE_BUTTON_PIN = 5; void setup() { pinMode(RED_LED_PIN, OUTPUT); pinMode(GREEN_LED_PIN, OUTPUT); pinMode(BLUE_LED_PIN, OUTPUT); pinMode(PHONE_BUTTON_PIN, INPUT); Serial.begin(9600); Serial.begin(115200); rtc.begin(); //starts the real time clock rtc.setDOW(TUESDAY); //set the day of the week rtc.setTime(0,20,00); //set the time in hours:minutes:seconds rtc.setDate(10,9,2019); //set the date months/days/year } void loop() { String mytime = rtc.getTimeStr(); //gets the time in a string mytime = mytime.substring(0,2); //only gets the hours part of string int hour = mytime.toInt(); //turns hours part into an int type int PhoneButtonState; PhoneButtonState = digitalRead(PHONE_BUTTON_PIN); if (((hour<=7) and (PhoneButtonState == LOW))){ //set hour to when you want it to be activated //this is set to turn on no later than 7:59 AM //because hours can be from 0 to 7 digitalWrite(RED_LED_PIN,HIGH); delay(100); digitalWrite(RED_LED_PIN,LOW); digitalWrite(GREEN_LED_PIN,HIGH); delay(100); digitalWrite(GREEN_LED_PIN,LOW); digitalWrite(BLUE_LED_PIN,HIGH); delay(100); digitalWrite(BLUE_LED_PIN,LOW); } }
Comments are closed.