OVERVIEW
A wooden and clear amber acrylic box with an alarm used to store medication.
I was unable to capture a DSLR video and final image for this project, so these elements will not be included.
PROCESS
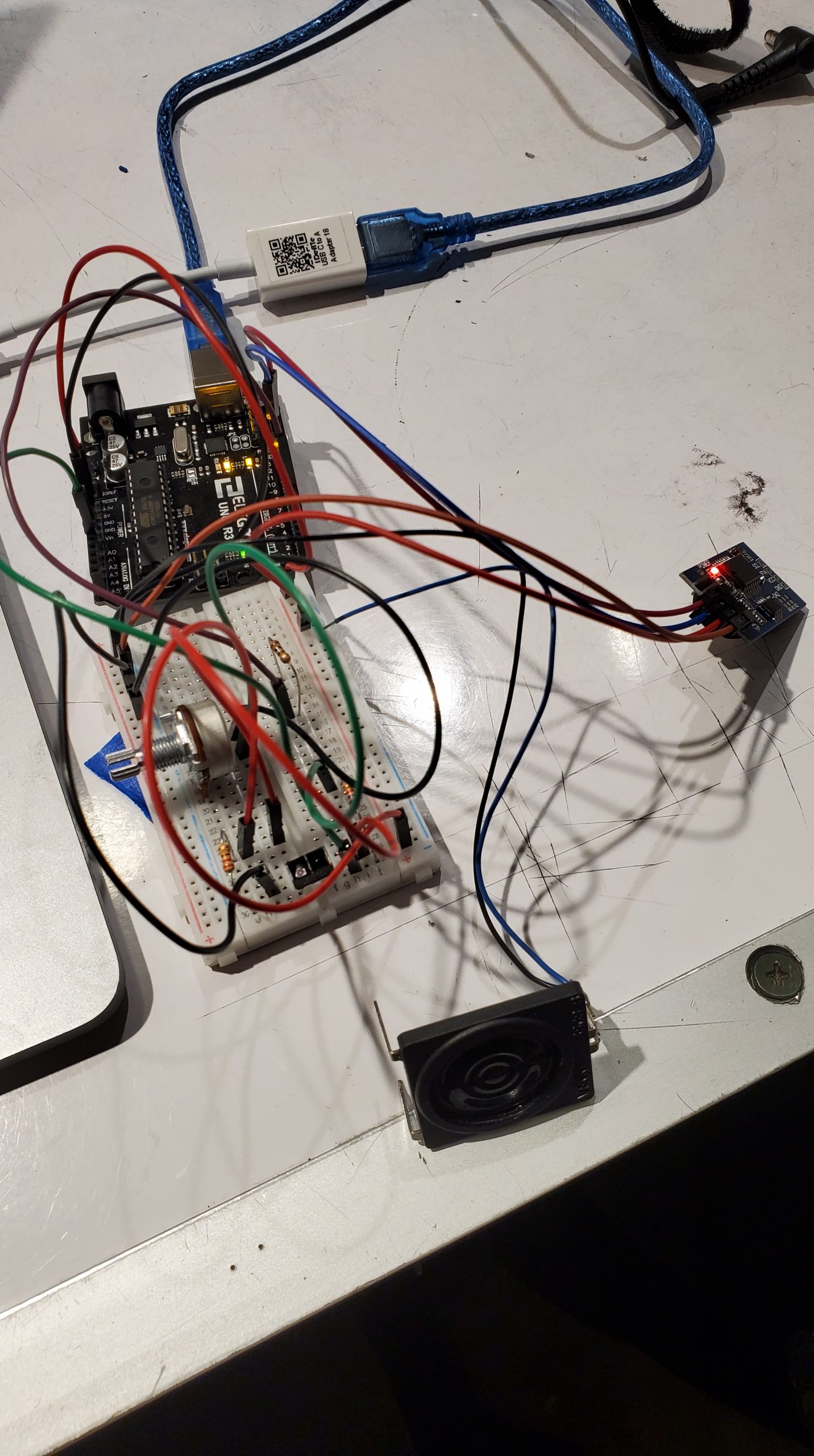
My arduino unit used for my medicine alarm box.
I first began with the arduino unit. This unit included an Arduino Uno R3, a DS3231 Real Time Clock, an 8ohm speaker, and an IR sensor with potentiometer. The RTC was included in order to set two time points for my alarm that would let me know when to take my medication. The speaker was used to produce the alarm sound. The IR sensor was used in order to detect when the box was opened or closed as a mechanism to switch off the alarm once it began ringing. If the box was closed and the alarm rang, the alarm would continue to do so until the box is opened.
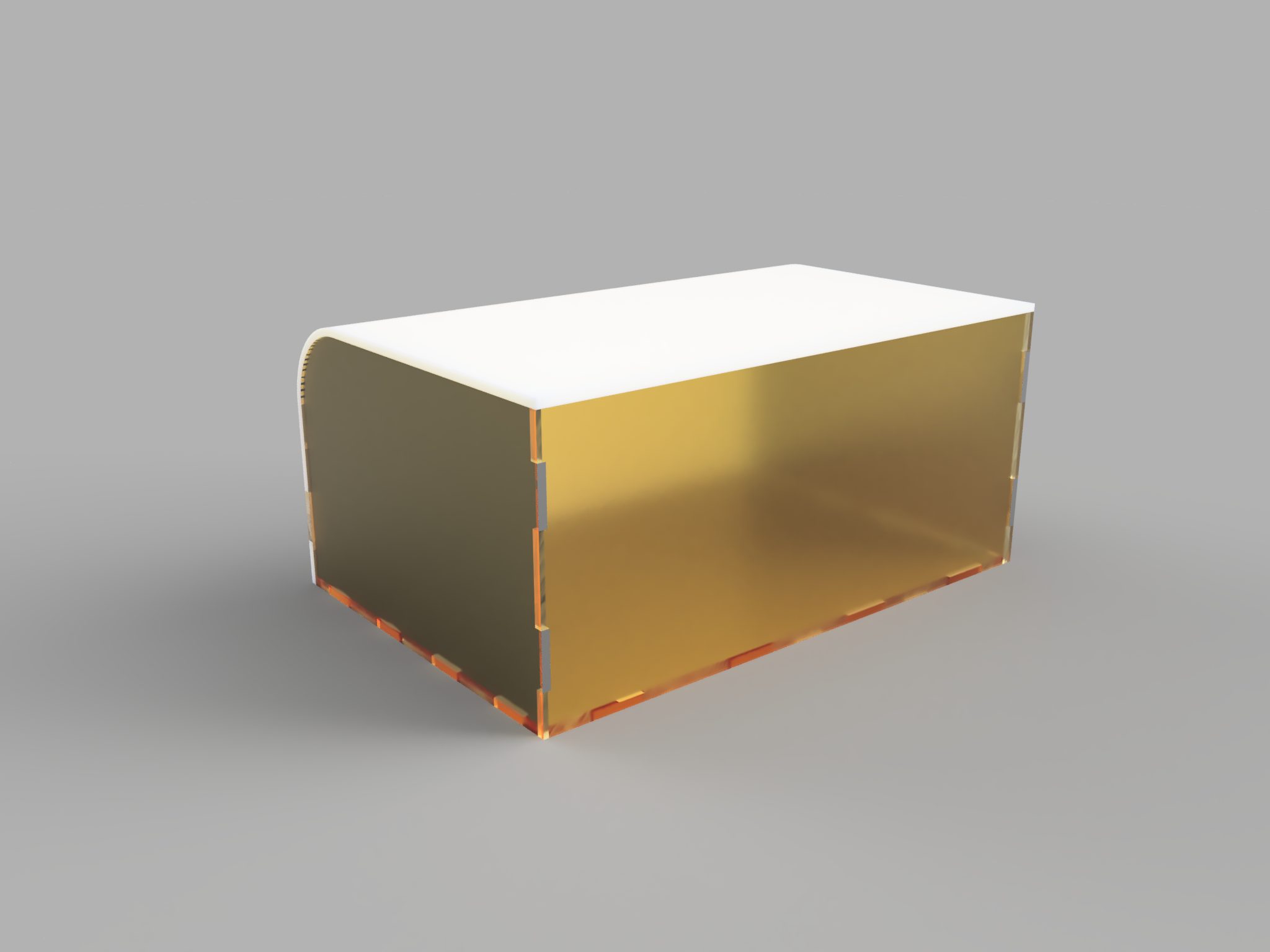
Fusion 360 render of medicine alarm box.
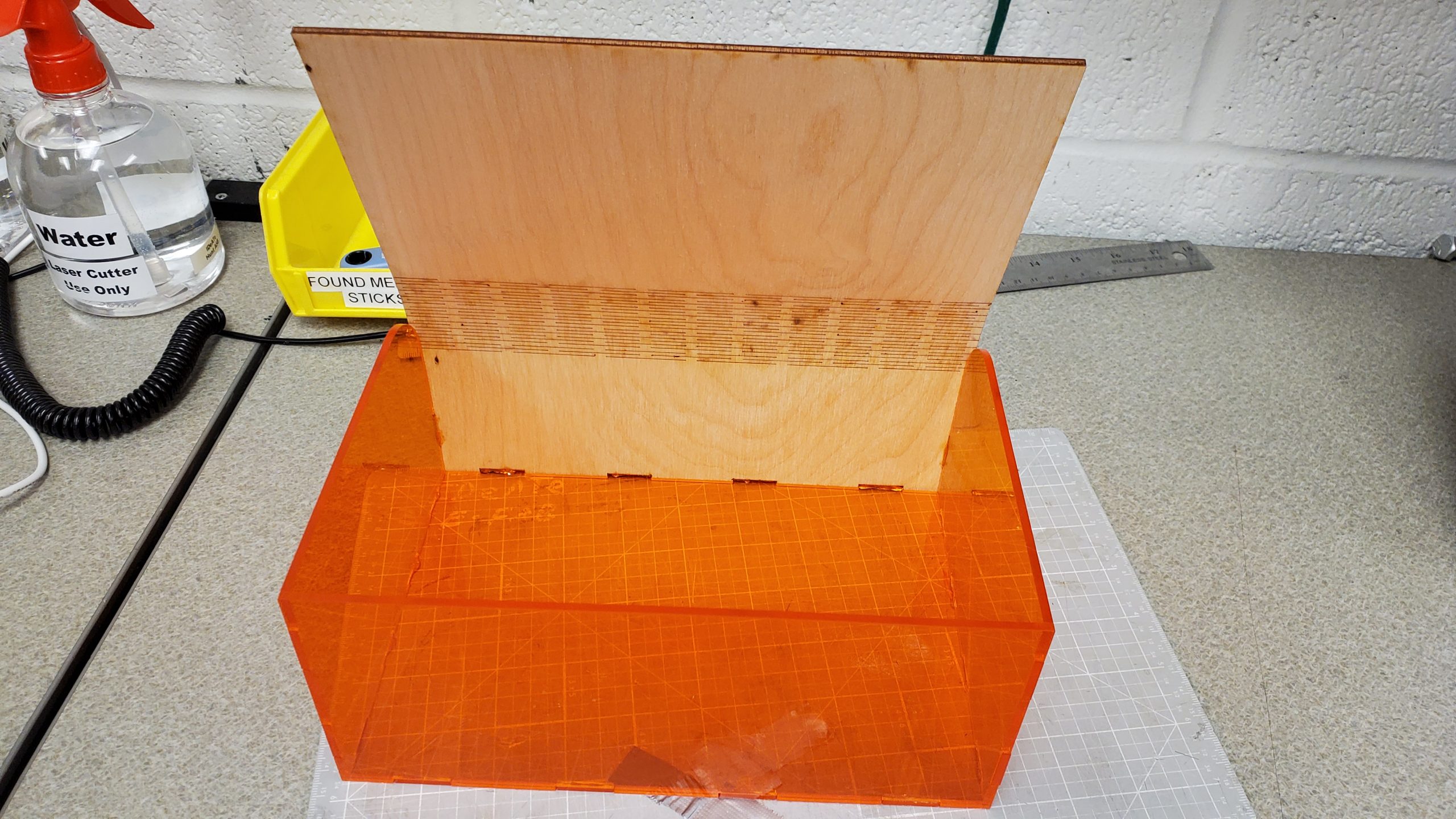
Open medicine alarm box without Arduino unit.
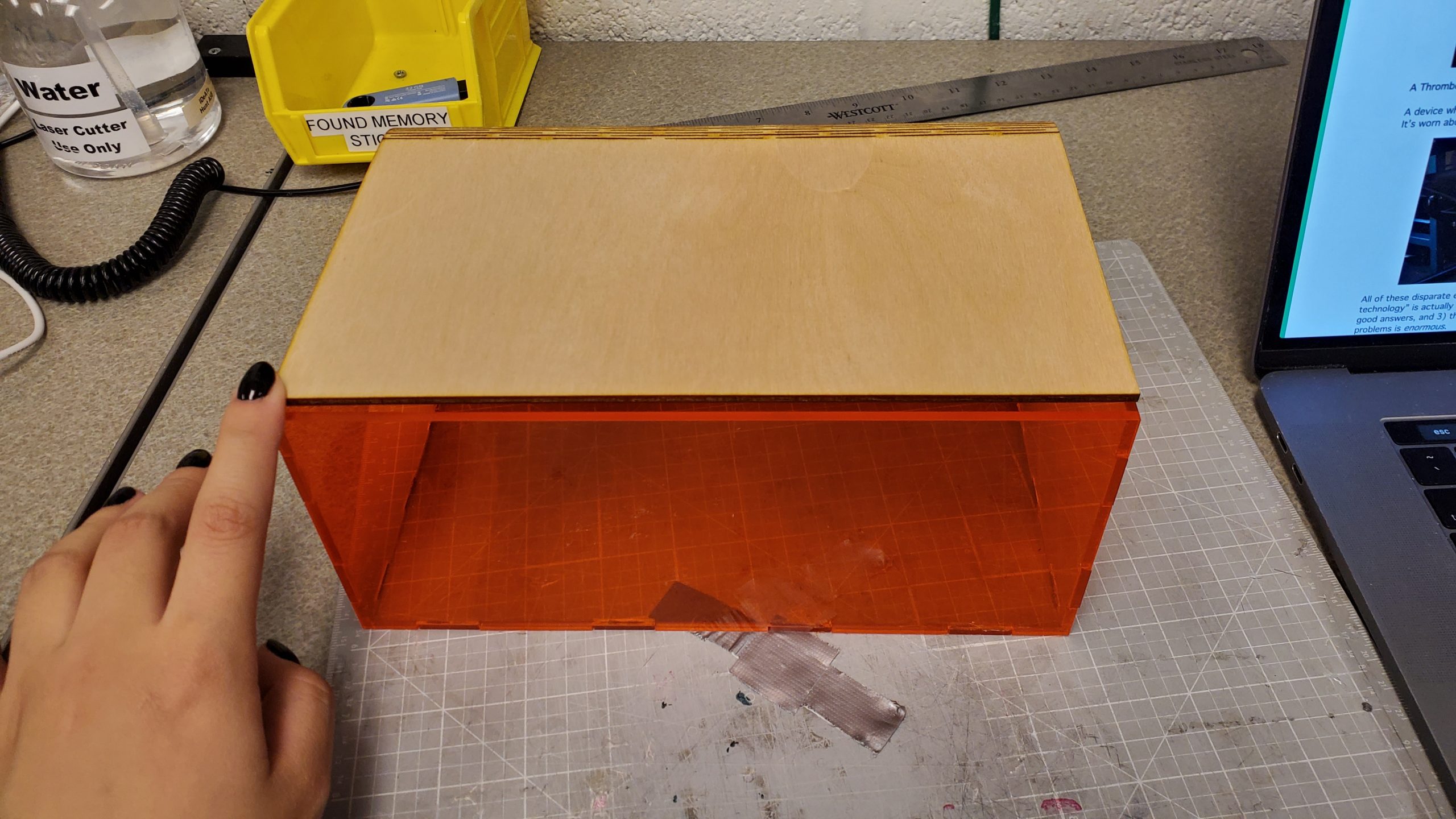
Closed medicine alarm box without Arduino unit.
For the box component, I chose to laser cut a box with an acrylic body and wooden lid. Originally, I had planned for the entire box to be made of acrylic, but due to the living hinge, I opted for a wooden lid instead as it would be less likely to break upon movement. For the living hinge, I used premade files from https://obrary.com/products/living-hinge-patterns. For the rest of the body, I modeled it from the ground up.
Not pictured is the final box. I drilled holes in the upper left front corner of the box in order to mount the speaker, as I had forgotten to measure out and place holes in the 3D modeling of the box. The IR sensor was hot glued to the upper front middle of the box where the edge of the lid met the edge of the front wall. Right under the lid on the very edge, I also hot glued a metal rod in order to keep the lid closed, as the second process image showed that the lid remained open when resting.
DISCUSSION
“Next time think about putting compartments in to allow better organization and separation of items and wires.”
While I did have some ideas to implement this originally, I’m not actually sure why I did not do so. I think this definitely would’ve been a good idea to make compartments and at least close off the wires from the rest of the box so that things don’t get tangled up if I ever had to move the box around.
“I appreciate that the aesthetic decisions were driven by an intention to mirror the appearance of a medicine bottle, but I think if that were the case, why not go all the way and have a more bottle-shaped whole? “
I like this idea of having a pill bottle shaped box, but I kind of wonder how easy it would be to make something cylindrical while keeping the orange acrylic body. I think I could use a heat gun to bent the acrylic, but I then would have an issue of the edges of the original acrylic piece possibly not connecting well enough. I think I could make an illusion of roundness by possibly making the box hexagonal, but as I had difficulty making the cut out of the rectangular box with the notches to combine the acrylic pieces, that would be more difficult to produce with a hexagonal box. But I really like the idea of having a pill bottle-esque appearance.
~
I think although overall this project was successful in achieving the functionality of the box, it was very simple and could’ve had more features. Originally I had wanted to include a 20×4 LCD screen that could display the time like a clock, as well as which medication I would need to take next, as well as pill count for the number of pills I had left in the box. I had difficulty with setting up the LCD, so I was unable to implement all of these features. Definitely with a next iteration of this project, I would like to actually be able to put these features in.
I had a lot of fun 3D modeling the box, although things like the living hinge were a bit of trouble. In my modeling, however, I miscalculated the length I would need for the lid. This didn’t end up affecting my project in any way, but for aesthetic purposes, I would rather have a lid that’s slighly longer than needed than slightly shorter like what had happened this time. In another iteration, I would need to remeasure more accurately to ensure things are the correct length.
As mentioned before in the feedback response, I should’ve put compartments to separate my medication from my Arduino unit. I could easily laser cut some acrylic dividers and glue them in for another iteration of this project.
During the critique, I also received a comment about the potentiometer that was connected to my IR proximity sensor. In theory, this would allow for different lighting environments, but it was unnecessary for my project and ended up being just a dead part. I would definitely remove this for another iteration.
TECHNICAL
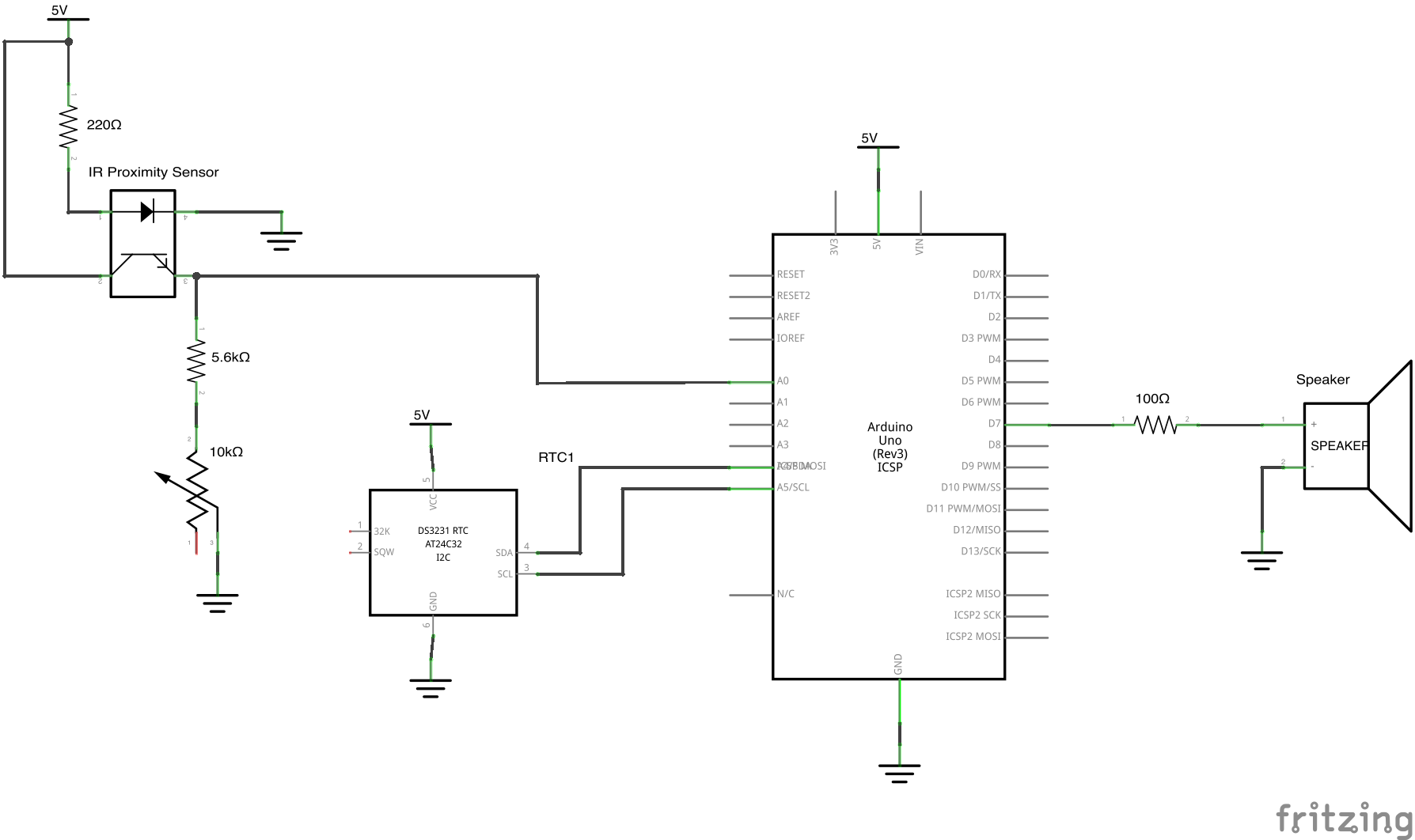
Schematic for medicine alarm box
/* * Medicine Alarm Box * * Sets an alarm for 9am and 9pm, reads if box is open or closed * in order to turn off alarm. * * RTC code was taken from RTClib.h examples * https://github.com/adafruit/RTClib/tree/master/examples * */ unsigned long alarmTimer = 0; const int ALARMINTERVAL = 700; #include <RTClib.h> RTC_DS3231 rtc const int IRSENSORPIN = A0; const int SPEAKERPIN = 7; bool medAlarm = false; void setup() { pinMode(IRSENSORPIN, INPUT); pinMode(SPEAKERPIN, OUTPUT); Serial.begin(9600); // RTC code from RTClib.h examples if (! rtc.begin()) { Serial.println("Couldn't find RTC"); while (1); } if (rtc.lostPower()) { Serial.println("RTC lost power, lets set the time!"); // following line sets the RTC to the date & time this sketch was compiled rtc.adjust(DateTime(F(__DATE__), F(__TIME__))); // This line sets the RTC with an explicit date & time, for example to set // January 21, 2014 at 3am you would call: // rtc.adjust(DateTime(2014, 1, 21, 3, 0, 0)); } //end RTC code } void loop() { // read of IR sensor int irRead = analogRead(IRSENSORPIN); // variable to read clock DateTime now = rtc.now(); // alarm rings at 9pm if (String(now.hour(),DEC) == "21" && String(now.minute(),DEC) == "0" && String(now.second(),DEC) == "0"){ medAlarm = true; } // alarm also rings at 9am else if (String(now.hour(),DEC) == "9" && String(now.minute(),DEC) == "0" && String(now.second(),DEC) == "0"){ medAlarm = true; } // when alarm is on if (medAlarm){ //if the IR read is less than 20 aka if box open, shut off alarm if (irRead < 20){ medAlarm = false; } // otherwise continue playing alarm else if(millis() - alarmTimer > ALARMINTERVAL){ tone(SPEAKERPIN, 440, 400); alarmTimer = millis(); } } }
Comments are closed.