Overview: This device reminds the user to take their medicine before going to bed.
- Lights are turned on when the force sensor detects user going to bed. A speaker starts 1 minute (demonstration 3 seconds) after the lights turn on if box hasn’t been opened.
- A dimmer interior light turns on when the box is opened (detected by proximity sensor) in case it’s too dark to see.
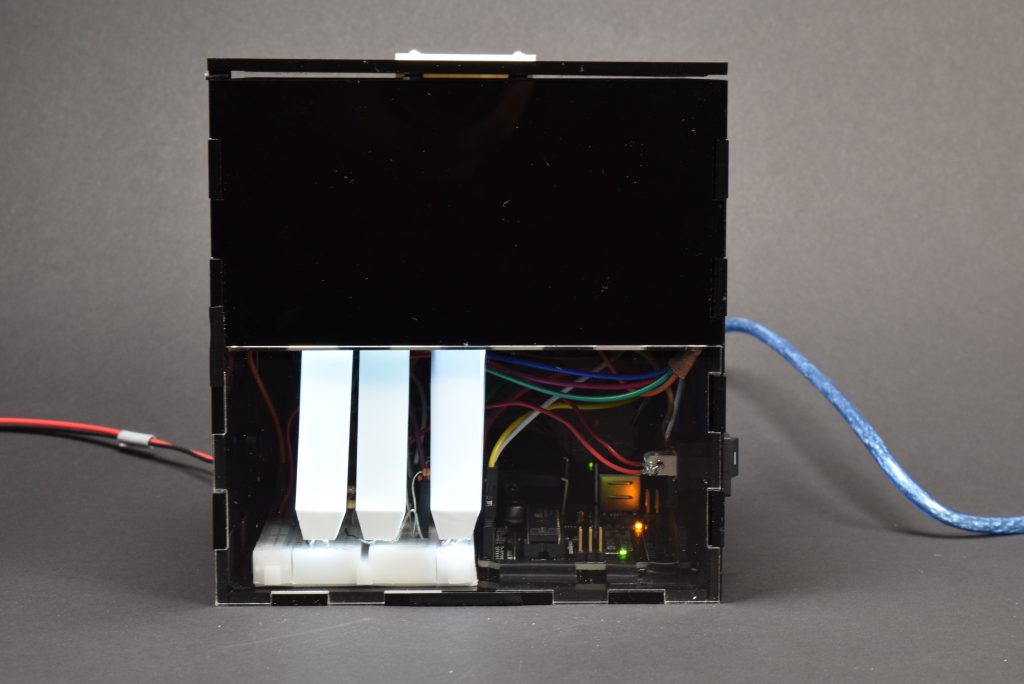
Front view: different materials allow the light to alert user and display wiring, but keep the contents of the box hidden
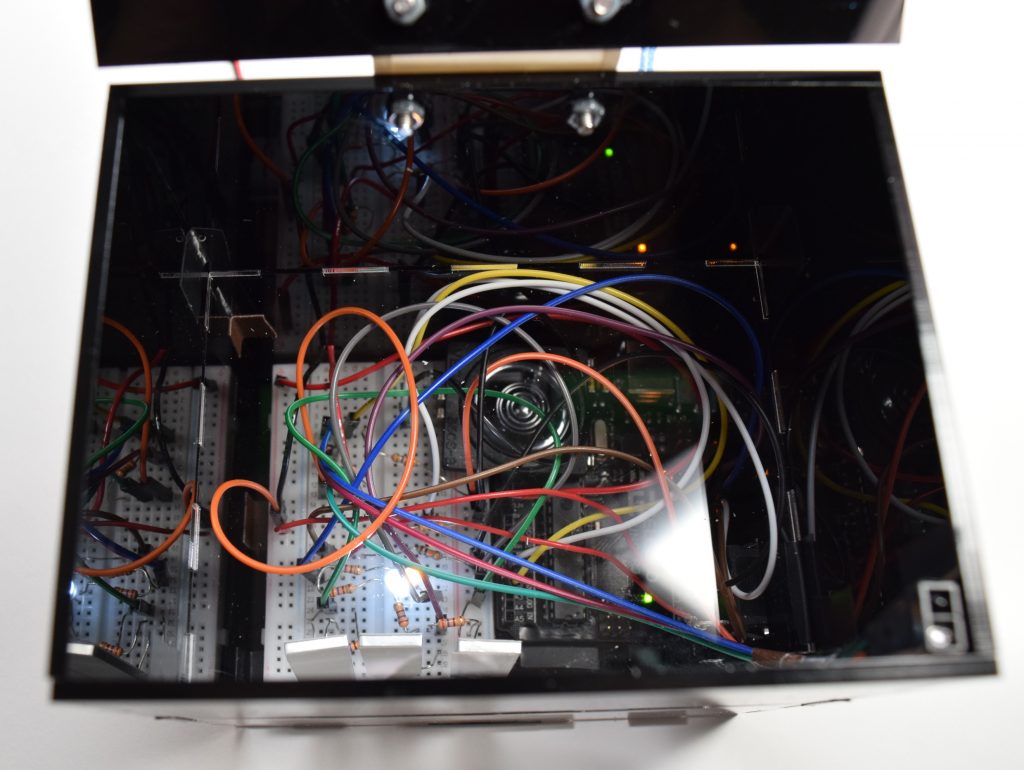
Top view of the inside: clear acrylic shows the electronics below and allows interior light to shine through
Process:
I’d originally planned to have LED strips on the exterior of the front and along the border of the top that would light up when the lid was opened. The acrylic ended up being too thin to embed the lights properly, so I changed direction.
I was browsing though previous semesters’ projects that used clear acrylic and found that I like being able to see the wiring and electronics. But I wanted to keep the contents of the box hidden for privacy. Deciding to use clear acrylic allowed me to changed my original plan of have lights on the outside of the box and along the top of the interior.
For the lid, I wanted to make it a sliding lid that would fit into a groove when I realized the hinge would be hard to work with (the plastic’s tendency to hold its position made it hard to get the lid precisely against the top), but again I found the acrylic too thin to do that. I compromised the smooth exterior by placing the hinge on the outside.
Discussion:
Overall, I am satisfied with the way my project turned out, because the project did achieve my goal of being a proficient reminder device, but I wish I’d added some more advanced features. I’m glad I took into account details such as taking naps, having “light as a gentle nudge, the sound as a second reminder”, and the interior light.
Comments in my critique were also positive about the design, choice of material, and thought process. One written critique that I completely agree with was “I would suggest a longer wire to connect the box to the pressure sensor that would be placed under the mattress.” This would definitely be a change I would make next time. Another problem with the design was the plastic hinge. It sometimes pops the lid open slightly, so in a future iteration, I would laser cut the acrylic to imbed a magnet to keep the lid shut. The plastic hinge also has to be “trained” to hold its shut position and is more difficult to bend, so I would also use a metal pinned hinge instead.
I found designing the box was surprisingly time-consuming, and I got hung up with the blue/black lines in the fusion 360 software. But I did learn a lot about constraints and dimensions and gained useful experience for future designing. Seth helped me with laser cutting, and from him, I learned a lot about using delete overlap and combine curve to allow the laser to move in one continuous line.
Another comment was: “This project looks super aesthetically pleasing! A suggestion to maybe add a user interface option to change the pressure threshold instead of having to go into the code.” I think this is a great idea, and in another iteration, I would add a potentiometer and button to be able to set the pressure threshold so the device would be able to be used for example by an adult and a toddler. Another way I would incorporate user interaction would be to have color changing options for the lights.
In discussion, we talked about how the device could be useful for people with Alzheimers. A next step could be to develop a way to connect it to a phone where the user could customize reminders.
Schematic:
Code:
/* Medicine Taking Reminder This device reminds the user to take their medicine before going to bed, using a force sensor to detect it. Reminder cues are light and sound. Pin mapping: pin | mode | description ------|--------|------------ 12 output Outside LED 10 output Inside LED 8 input Switch A1 input Force sensor A2 input IR sensor 9 output Speaker Libraries Used: Volume3 */ #include "Volume3.h" const int EXLEDPIN = 12; const int INLEDPIN = 10; const int SWITCHPIN = 8; const int FORCEPIN = A1; const int IRPIN = A2; const int SPEAKERPIN = 9; // how long to wait until sensor is on // 10 seconds for demo, 72000000 (20 hours) in use const unsigned long RESTARTTIME = 10000; const int PRESSURE = 400; bool on = true; bool newDay = true; bool closed = true; unsigned long newDayTimer = 0; unsigned long exLEDtimer = millis(); void setup() { pinMode(EXLEDPIN, OUTPUT); pinMode(INLEDPIN, OUTPUT); pinMode(SWITCHPIN, INPUT); pinMode(IRPIN, INPUT); pinMode(FORCEPIN, INPUT); pinMode(SPEAKERPIN, OUTPUT); Serial.begin(9600); } void loop() { // is device turned on? if (digitalRead(SWITCHPIN) == LOW) { on = false; } else { on = true; } // has it reached restart time for sensor to be on? // 10 seconds for demo, 72000000 (20 hours) in use if (millis() - newDayTimer >= RESTARTTIME) { newDay = true; } bool start = false; while (on && newDay) { unsigned long exLEDtimer; int force = analogRead(FORCEPIN); if (force > PRESSURE && !start) { if (!start) { exLEDtimer = millis(); } start = true; } while (start) { digitalWrite(EXLEDPIN, HIGH); //haven't opened box in longer than 1 minute //demonstration will be 5 seconds Serial.print("millis - exled"); Serial.println(millis()); if (millis() - exLEDtimer >= 5000 && closed) { vol.tone(SPEAKERPIN, 220, 500); } //is box opened int distance = analogRead(IRPIN); if (distance < 50) { closed = false; newDay = false; start = false; vol.tone(SPEAKERPIN, 220, 0); newDayTimer = millis(); } } while (!closed) { digitalWrite(INLEDPIN, HIGH); digitalWrite(EXLEDPIN, LOW); if (analogRead(IRPIN) >= 50) { closed = true; digitalWrite(INLEDPIN, LOW); vol.tone(SPEAKERPIN, 220, 0); } } } }
Comments are closed.