Here’s a demo of my execution of “Rocking and Rolling”:
Brainstorming and Initial Ideas
Initially, I wanted to create rocking motion that could move a ball bearing from a higher level to a lower level back to a higher level and so on. To clarify, here’s a diagram:
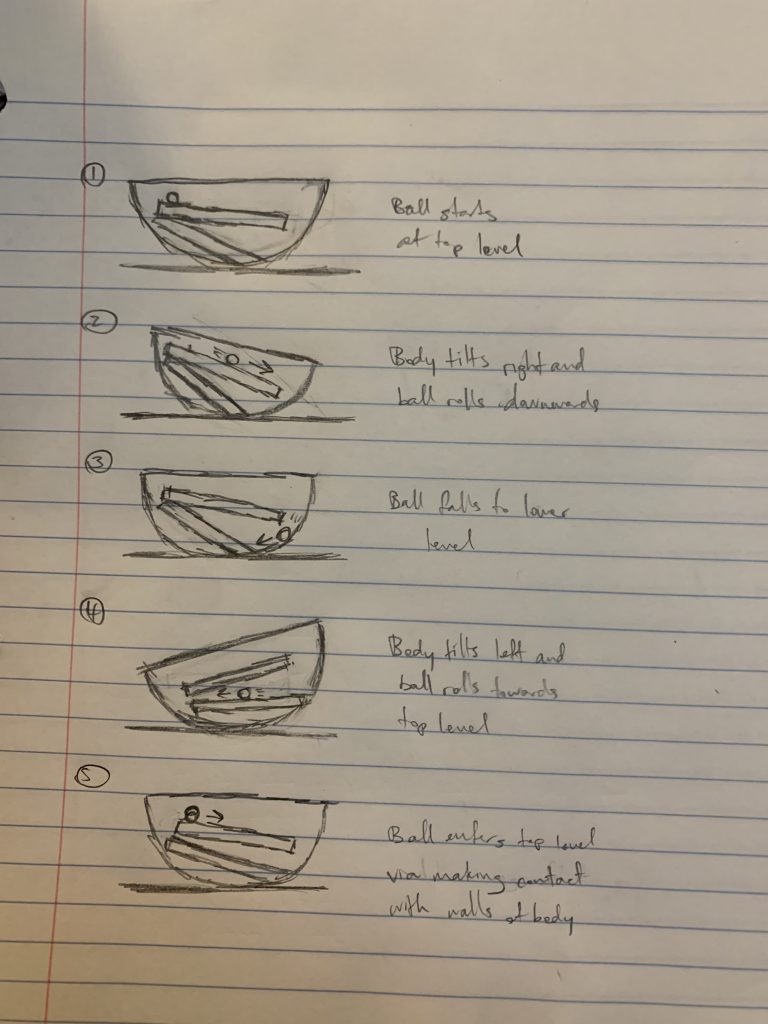
I soon realized that producing such a product was not feasible given how much I had to do this project. So, I came up with the idea to use the rocking motion to move the ball bearing over little “hills,” as shown below:
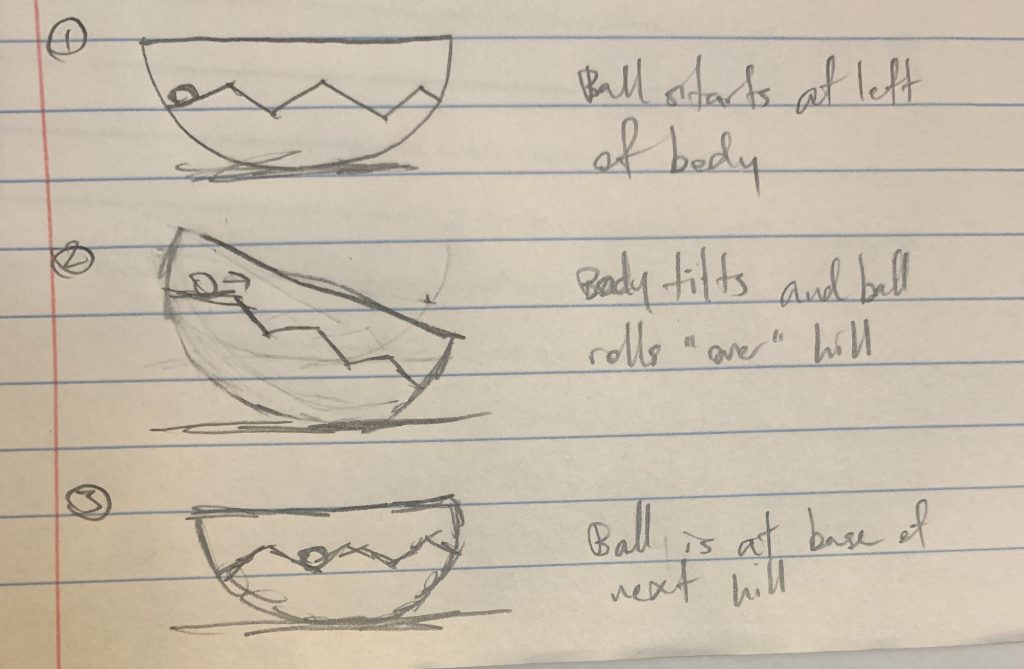
Final Result
I ended up using two semicircles of radius 4.5 inches as my rocking body and 4 short, 1-2 inches long pieces of wood for my “hills.” I also attached a curved arm to the servo arm to add more of a push to the rocking motion. I added paper towel to the shared bottom of the two hills to increase friction so the ball bearing would be able to come to a stop in that area.
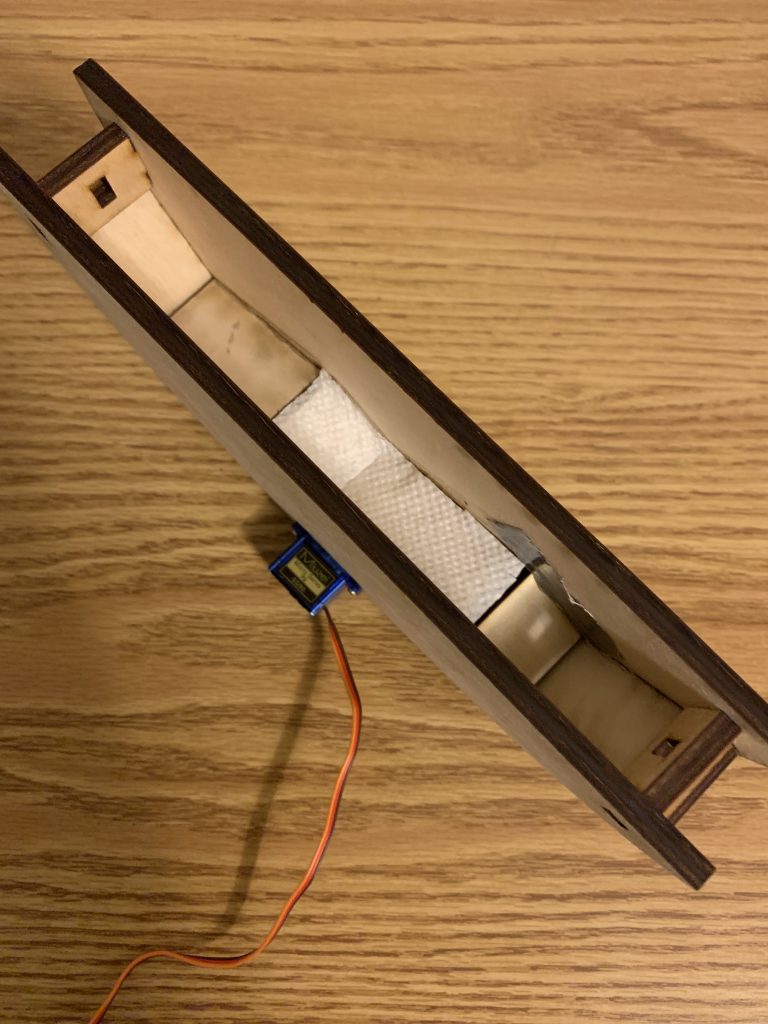
Overall, I’m happy with how my project turned out. However, I found it very hard to make the ball bearing consistently stop at the shared bottom of the two hills. If I were to take a go at this project again, I’d probably spend more time looking into how to get the ball bearing to consistently stay put at the bottom of the hills.
Code
#include <Servo.h> Servo ser; // create servo object to control a servo // twelve servo objects can be created on most boards int pos = 95; // variable to store the servo position void setup() { Serial.begin(9600); ser.attach(9); // attaches the servo on pin 9 to the servo object ser.write(pos); } void loop() { for(pos = 95; pos <= 180; pos += 2){ ser.write(pos); delay(50); } delay(500); for(pos = 180; pos >= 95; pos -= 12){ ser.write(pos); delay(50); } delay(2000); for(pos = 95; pos <= 180; pos += 2){ ser.write(pos); delay(50); } delay(2000); for(pos = 180; pos >= 95; pos -= 2){ ser.write(pos); delay(50); } delay(2000); for(pos = 95; pos >= 50; pos -= 2){ ser.write(pos); delay(50); } delay(2000); for(pos = 50; pos <= 95; pos += 2){ ser.write(pos); delay(50); } }
Leave a Reply
You must be logged in to post a comment.