This is a drawing machine which allows you to control the brush by sweeping your hands in front of the sensor. As the hand sweeps towards the sensor, the brush will move left, and vise versa. As the brush move to the right-most position, a tab attached to the pen holder will touch the switch, which makes the pen stop for a second.
The biggest challenge while testing it is its unstableness. A subtle change in angles will result in the shaking of the brush. In addition, it is hard for the tab to touch the switch because of the shivering. However, I like the way that shaking adds a lot of interesting texture to the brushstrokes.
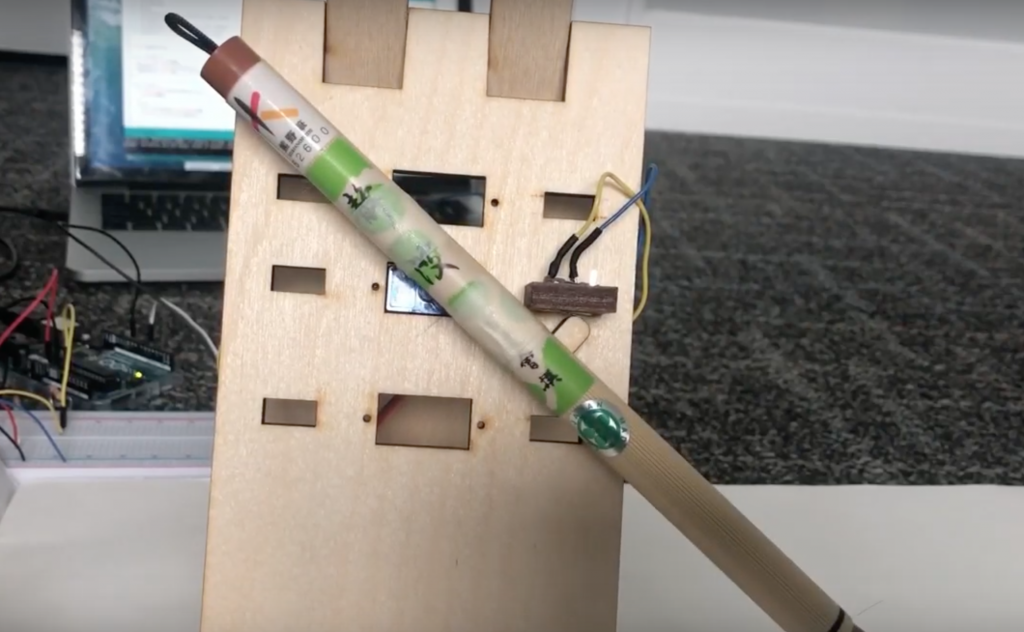
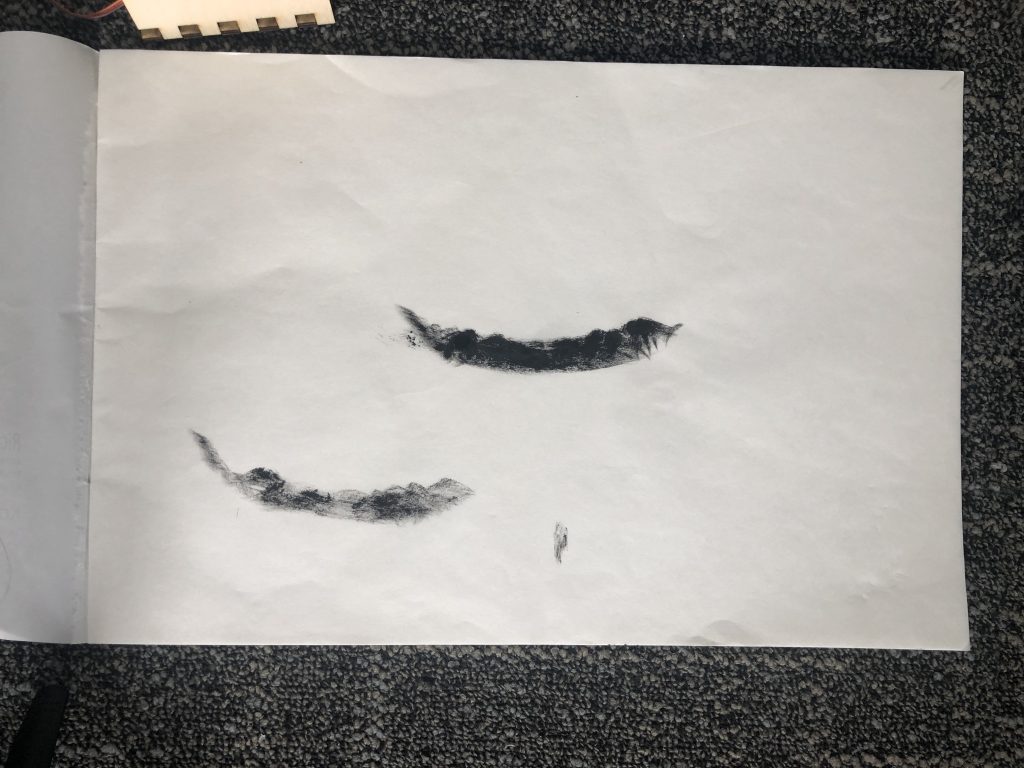
Code:
#include <Servo.h> //variables int servoPin=5; int switchPin=9; int prevSenVal=0; //the previous sensor value int prevAngle=0; int unitAngle=1; //the angle the servo rotates int maxAngle=83; //the maximum rotate angle Servo hobbyServo; void setup() { Serial.begin(115200); hobbyServo.attach(servoPin); hobbyServo.write(0); // initiate with 0 degree of angle } void loop() { if (digitalRead(switchPin)==1){ //when hit the switch, stop for 1 sec delay(1000); } else{ int currSenVal=analogRead(A0); Serial.println(currSenVal); //compare the current sensor value to the previous one // if larger, move to right; if smaller, move to left if (currSenVal==prevSenVal) { //stop hobbyServo.write(prevAngle); } else if (currSenVal>prevSenVal){ //servo move counterclockwise int currAngle=prevAngle+unitAngle; if (currAngle>=maxAngle){ //stop hobbyServo.write(maxAngle); } else { prevAngle=currAngle; //set previous angle to be the current angle prevSenVal=currSenVal; //set previous sensor value to be the current one hobbyServo.write(currAngle); } } else if (currSenVal<prevSenVal){ //servo move clockwise int currAngle=prevAngle-unitAngle; if (currAngle<=0){ hobbyServo.write(0); } else { prevAngle=currAngle; prevSenVal=currSenVal; hobbyServo.write(currAngle); } } } }
Leave a Reply
You must be logged in to post a comment.