In this project, we want to capture the natural motion of a plant responding to sunlight and watering. When the flower is being watered, it will bloom. However, when the sunlight is being covered, it will wilt or unbloom. In addition, in order to attract kids to interact with it, we also added a heartbeat shaking to the flower when it is neither being covered nor watered.
Being connected together with fishing wires which are then bundled to connect to the servo, the pedals and leaves are able to perform the same motion. A photosensor allows the flower to sense whether its sunlight is blocked or not. In order to sense the watering motion, an accelerometer and a distance sensor are embedded in the water pot.
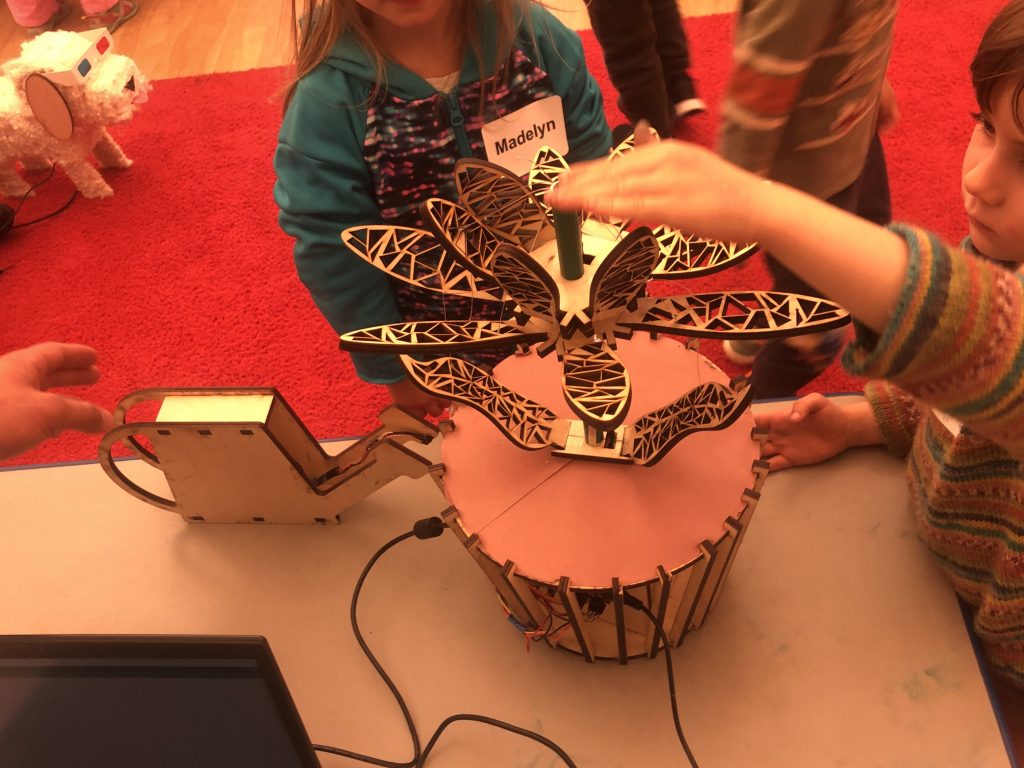
This device was brought to Children’s School on Friday for testing. In general, the interacting time was about 40 seconds to 1 minute. Some children were interacting with it for a long time and were very curious about how we made it; some found it boring because the motions were too slow and gentle. We also observed some unexpected behaviors such as watering on top of the flower instead of the pot, trying to use the water pot to water the other group’s dog, and blooming the flower physically which destroyed the pedals.
We really appreciated the opportunity that we can demo our device on the spot. The observations we made were very important lessons for us to understand our audience and make improvements next time.
Code
#include <Servo.h> Servo myservo; int SERVO_PIN=8; int SENSOR_PIN=A0; int pos=0; float now = millis(); void shake(){ for (int i = 0; i < 2; i++){ myservo.write(min(pos + 10, 100)); delay(200); myservo.write(max(pos-10, 45)); delay(600); } now = millis(); } void setup() { myservo.attach(SERVO_PIN); Serial.begin(115200); myservo.write(100); // initialize the flower to be closed } void loop() { float sensorVal=analogRead(SENSOR_PIN); //Serial.println(sensorVal); Serial.println(isTipped()); if (sensorVal<=920){ //sense the shadow -- unbloom now = millis(); if (pos<100){ pos+=1; myservo.write(pos); } else if (pos==100){ myservo.write(pos); } } else if (isTipped()==true ){ // water the flower -- bloom now = millis(); if (pos>45){ pos-=1; myservo.write(pos); } else if (pos==45){ myservo.write(pos); } } if (millis() - now > 5000){ shake(); } delay(15); } // watercup float sampleA0(){ float full = 0.0; for (int i = 0; i < 50; i++){ full = full + analogRead(A3); } return full/50; } float sampleA1(){ float full = 0.0; for (int i = 0; i < 50; i++){ full = full + analogRead(A4); } return full/50; } float sampleA2(){ float full = 0.0; for (int i = 0; i < 50; i++){ full = full + analogRead(A5); } return full/50; } float isTipped(){ if (sampleA0() > 750 && sampleA1() < 770 && sampleA2() < 1000){ return true; } else{ return false; } }
Leave a Reply
You must be logged in to post a comment.