Interaction Analysis
For our project, we had a whale that chomped anyone who was careless enough to try to steal its food and treasure. At first, many children were actually afraid of the whale. The relatively sharp teeth and sudden bite was scary for them. However, after some incentive from either parents or teachers, children eventually tried to save fish and retrieve coins from the whale’s mouth. Most of them realized fairly quickly that if they were careful enough when getting whatever was inside the whale’s mouth, they would not be chomped. Even if they did trigger the pressure plate, there was a small wind up gap in which the whale opened its mouth slowly and children could escape.
There were a couple interactions which we found surprising during the museum trip. First, the children associated retrieving the fish and the gold coins to a game, and thus asked whether they had ‘won’ after they got everything out of the whale’s mouth. After winning the game, children were kind enough to feed the whale by themselves (though sometimes Caio had to challenge the children to feed the whale), when they also enjoyed throwing the fish and coins into the mouth. Second, there was one boy in particular who played with our whale for a long time, discovering a new way to play with the whale which he really liked. He would have his hand bitten on purpose so he could pretend to ‘grow’ his hand back.
In order to make this a more delightful interaction, we would like to add sound to the whale’s actions. We believe this would create a more concrete experience, while the sound together with the mouth slowly opening and then quickly closing would also make for a more vivid experience. For the complete project, we are likely maintaining our current basic interaction (retrieving fish and coins without getting chomped), since children seemed to have a lot of fun with it.
Engineering Analysis
Fortunately, we had few issues with our design during the visit. Although there was a minor setback with an unplugged wired when we arrived at the museum, the whale functioned consistently throughout the whole duration of our trip. Our only mechanical failure was the upper-teeth-to-jaw connection, which was basically made through hot glue. Because some children found delight in the anxious experience of getting their hand bitten, the teeth had to withstand more impacts than we anticipated. As such, the glue could not take it and thus the teeth came off close to the conclusion of our stay.
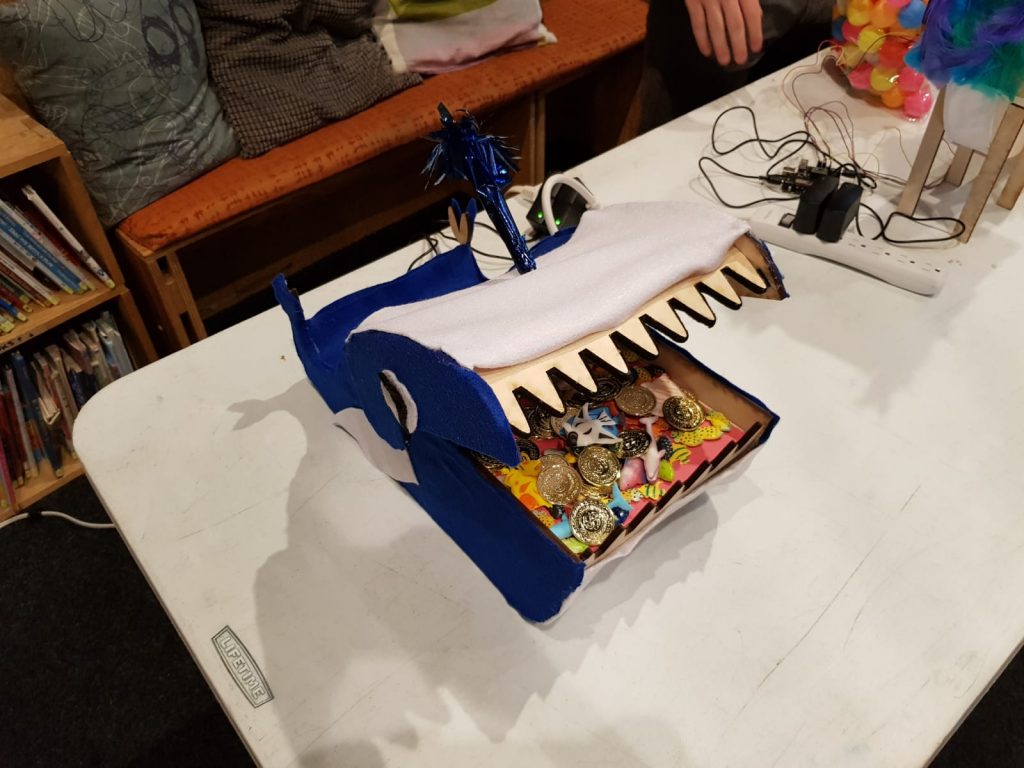
In terms of operational details, we realized that the whale was scary in its behavior. Initially, the biting patterns were random, which meant that more often than not children would get chomped as soon as they triggered the scale. They were afraid, so Caio had to change the code in order to be more consistent and create a window for escape. After the changes, the whale always opened its mouth wide before chomping, so children could read the whale’s behavior and anticipate the incoming bites. These changes certainly made the toy more attractive. However, we do like the sudden and ‘jumpscary’ chomps, thus we will try to think of ways in which we can incorporate those on our final design, as the interactions would also be more diverse.
#include <Servo.h> const int SERVO_PIN_1 = 8; const int SERVO_PIN_2 = 4; const int PHOTO_PIN_1 = A0; const float open_angle1 = 120; const float open_angle2 = 73; const float wideness = 30; const float closed_angle1 = open_angle1 - wideness; const float closed_angle2 = open_angle2 + wideness; Servo servo1, servo2; void reset_all() { servo1.write(open_angle1); servo2.write(open_angle2); } void slow_open(int angle, float cur1, float cur2) { float i = 0; while (i <= angle){ servo1.write(cur1 + i); servo2.write(cur2 - i); i += 0.25; // 0.25 and delay(10) super smooth, delay(15) also good delay(15); } Serial.print("Servo 1 ended at "); Serial.println(cur1+(i-0.25)); Serial.print("Servo 2 ended at "); Serial.println(cur2-(i-0.25)); delay(250); } void slow_close(int angle, float cur1, float cur2) { float i = 0; while (i <= angle){ servo1.write(cur1 - i); servo2.write(cur2 + i); i += 0.25; // 0.25 and delay(10) super smooth, delay(15) also good delay(15); } Serial.print("Servo 1 ended at "); Serial.println(cur1-(i-0.25)); Serial.print("Servo 2 ended at "); Serial.println(cur2+(i-0.25)); delay(250); } void regular_open(int angle, float cur1, float cur2) { servo1.write(cur1 + angle); servo2.write(cur2 - angle); Serial.print("Servo 1 ended at "); Serial.println(cur1+angle); Serial.print("Servo 2 ended at "); Serial.println(cur2-angle); delay(250); // reset_all(); } void regular_close(int angle, float cur1, float cur2) { servo1.write(cur1 - angle); servo2.write(cur2 + angle); Serial.print("Servo 1 ended at "); Serial.println(cur1-angle); Serial.print("Servo 2 ended at "); Serial.println(cur2+angle); delay(250); // reset_all(); } void setup() { // put your setup code here, to run once: servo1.attach(SERVO_PIN_1); servo2.attach(SERVO_PIN_2); reset_all(); pinMode(PHOTO_PIN_1, INPUT); Serial.begin(9600); } void loop() { // put your main code here, to run repeatedly: // reset_all(); int photo_read = analogRead(PHOTO_PIN_1); int threshold = 800; static float current_angle1 = open_angle1; static float current_angle2 = open_angle2; static int last_read = -1; Serial.println(photo_read); if (last_read > 0 && abs(last_read-photo_read) > 3){ int chance = random(1, 101); int angle = random(10, wideness+1); angle = wideness; chance = 5; if (chance < 10) { // yawn int oang = random(20, 31); slow_open(oang, current_angle1, current_angle2); current_angle1 += oang; current_angle2 -= oang; delay(100); regular_close(angle+oang, current_angle1, current_angle2); current_angle1 -= (angle+oang); current_angle2 += (angle+oang); delay(100); regular_open(oang, current_angle1, current_angle2); current_angle1 += angle; current_angle2 -= angle; delay(100); int chomps = random(2, 5); for (int i = 0; i < chomps; i++){ regular_close(angle, current_angle1, current_angle2); current_angle1 -= angle; current_angle2 += angle; regular_open(angle, current_angle1, current_angle2); current_angle1 += angle; current_angle2 -= angle; } delay(100); } } last_read = photo_read; }
(the code above includes last-minute changes, so it does not have the best style)
Revision Plan
Our basic experience intentions seemed to work pretty well. Children did enjoy playing with the whale and even competed against each other to see who could retrieve more. However, we had to delete the sudden chomping during the demo in order to make it less scary. We will think of ways in which we can incorporate these more scary bites because we believe they will make the interactions more diverse. Maybe some sort of petting that will calm the whale.
Regarding technical limitations, our current design is not very robust, as the jaw is supported by two servos. For our next iteration, we will incorporate a lever mechanism so that the servo is responsible only for providing torque to the jaw. Regarding the teeth which fell off, we will use tabs to keep them in place for the next structure, so that they can resist stronger (and more frequent) impacts. Also, the teeth should be smoother and rounder, i.e. less scary.
For our next design, we would like to add sound and petting. When the whale moves its jaw, there should be some sort of sound that accompanies the movement, in order to create a more realistic experience. Also, if we include petting capabilities which would improve the whale’s ‘mood’, we can work with the more sudden chomps as long as children understand that they must calm down the whale.
Concerning new skills, we will have to design a robust way to detect petting. We imagine that something similar to our pressure plate could work if we put it under the head’s felt. Also, it would be helpful to crimp the wires so that we can completely dismiss the breadboard and create more reliable electrical connections.
Design review list
- Robust mechanical structure for the servo
- More sensitive pressure plate, so it is harder to save the fish
- Better attachment for the teeth
- Robust electrical connections
- Add sound
- Smoother teeth
- Petting mechanism
Leave a Reply
You must be logged in to post a comment.