Abstract
Our goal was to make a dinosaur that would chew on a wooden ball and spit it out. It would be actuated by a pulley system with strings attached to horns on two servo motors, one on each side of the body. The dino would also sporadically play music.
Our final result did exactly what we promised and performed well during the museum demonstration. It attracted attention and was mostly robust, though towards the end, the wires for the servo started becoming a bit loose.
Overall, the project was very successful.
Objectives
The main objective of our project was to create a dinosaur that would “chew” a wooden ball and then spit and “poop” it out. However, due to the compactness of our initial prototype, we decided to only focus on the spitting portion of the project rather than having both functionalities. This simplified the idea and allowed us to refine and perfect the spitting (and, thinking back, it would have taken much time to get the “pooping” to work as we wanted). Later on, we added a buzzer and found melodies on GitHub (see citations section) to allow the dino to play music and noises before it spits the ball out.
(finished at a responsible time and not the night before the first museum trip)
Implementation
The dino is made of 4 major pieces: one large body (with lower jaw and tail attached), an upper jaw, and two legs.
We decided on using two servo motors move the jaw up and down, which allowed for more chewing power, though, in the end, one servo would have been sufficient.
Since the structure was a bit top heavy, it naturally tipped forward, which was what we wanted. All we needed to figure out was how to get it to stand up after it leaned forward. Initially, we wanted to to have servo motors at the pivot point of the body, but we were worried that the weight of the dinosaur would harm the servo motors. So, we decided on using a pulley system to have the servo horns pull up the dino after it had leaned forward and dropped the ball. The pulley system is shown below.
The tail had a large counter weight inside it to keep the dino from leaning too fast. This weight is hidden in the final implementation.
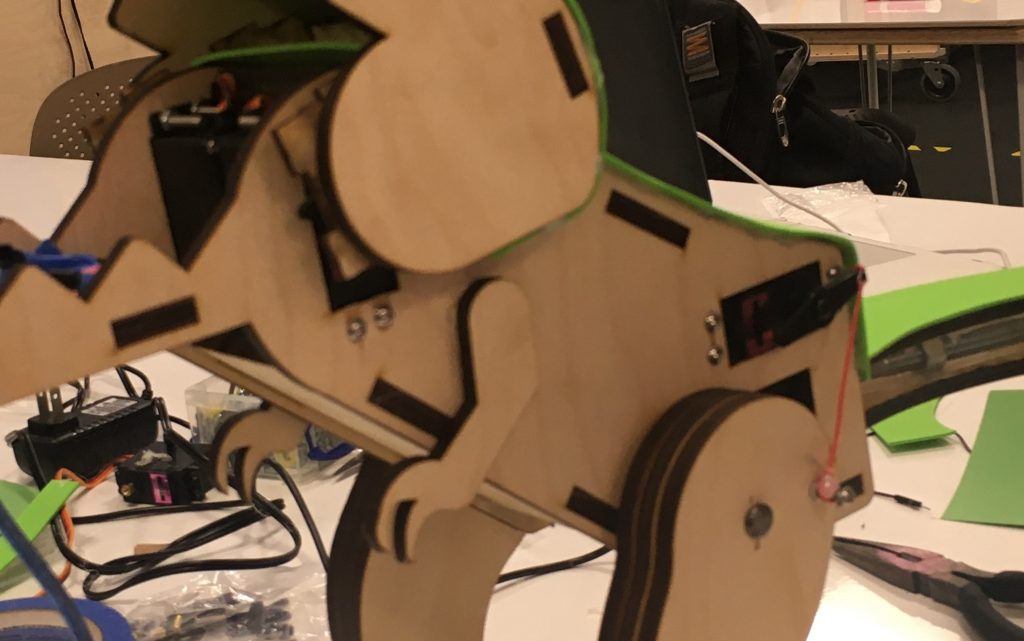
the body tips forward, allowing a ball placed inside to fall out.
We also had two photoreceptor sensors in the lower jaw pointing out to sense the presence of an object in the mouth. When one of them is triggered, the chew and spit sequence initiates. The sequence is: chew for a randomized amount of time, then open the mouth, play a song, lean forward, then return to standing position.
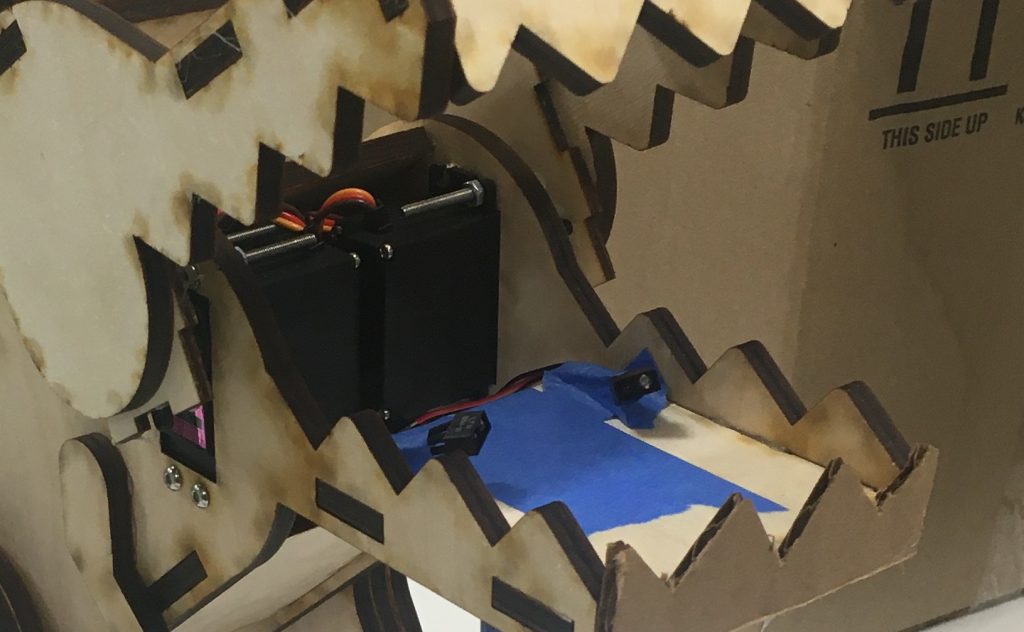
Outcomes
Our outcomes of our final demo were overall quite successful. The Dino attracted attention and held it for a decent while. The learning curve was mostly in the timing. Some people tried to feed it, while it was spitting out the balls but it was overall quite intuitive. Especially considering during the first museum demo the Dino broke down immediately, it was so relieving to see our final iteration work the whole day and survive the kids and adults who tried to play with it. We were very proud of how robust the final product was and its ability to work consistently under real world conditions.
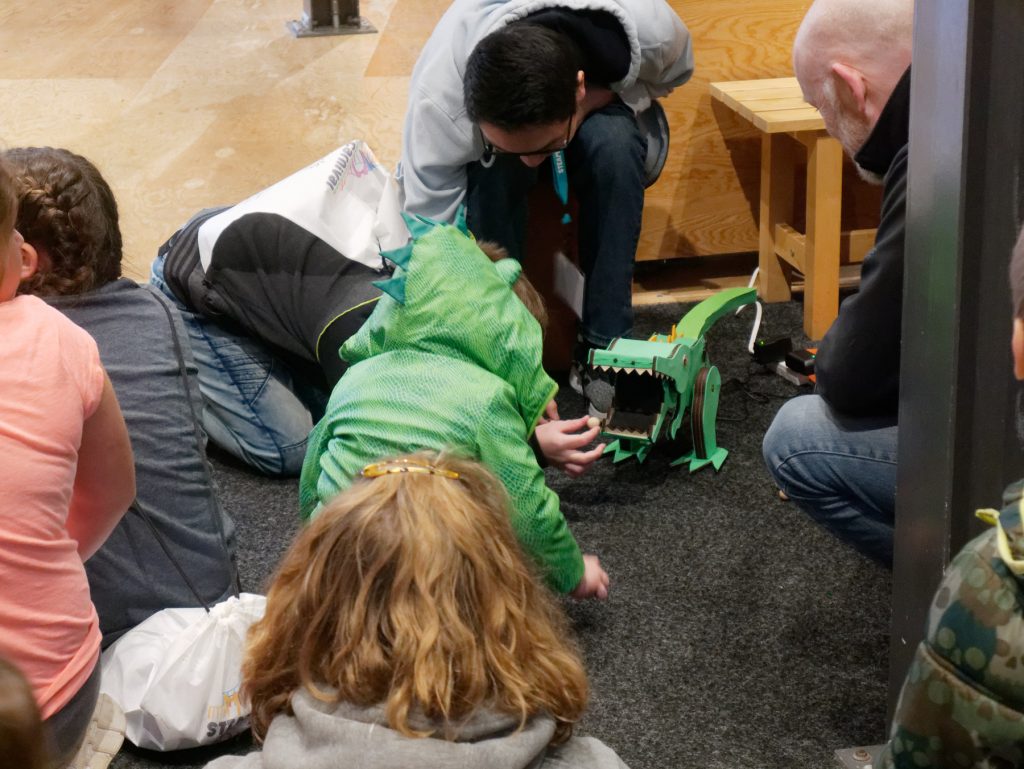
Future Work
The next steps for the Dining Dino would be to implement more diverse functionality. There are a few immediate fixes that should be implemented to make it more effective
First, we would make the buzzer louder. The music the Dino played was a simple last minute addition but it added a whole lot. Getting a wider range of sounds, including songs more recognizable to our target audience, would improve the fun and reward of the noisemaker.
Second, the wires we used for our pulley system will need to be secured better or converted into solid linkages so they don’t loosen over time. While this wasn’t a huge problem, we could tell by the end of the day that the base angle of the Dino was a bit lower. Having a more robust solution is an ideal upgrade.
Third, although not a huge change, it would be quite beneficial to make our Dino a little bigger. Out on the test floor, it looked a little lost in the massive crowds. It is quite the balancing act however, because making the Dino bigger would also make it heavier and although our system was quite strong, there is a limit to how big we could go and still work effectively.
Lastly, if we had more time to work on this product, it may be a good idea to go back to the drawing board. Since we went through so many changes and iterations, the product became a plaything with a muddled conglomeration of a purpose. Taking the time to revisit what we really want to get across and how the Dino will affect people can really allow us to hone in on certain elements and push them to the max.
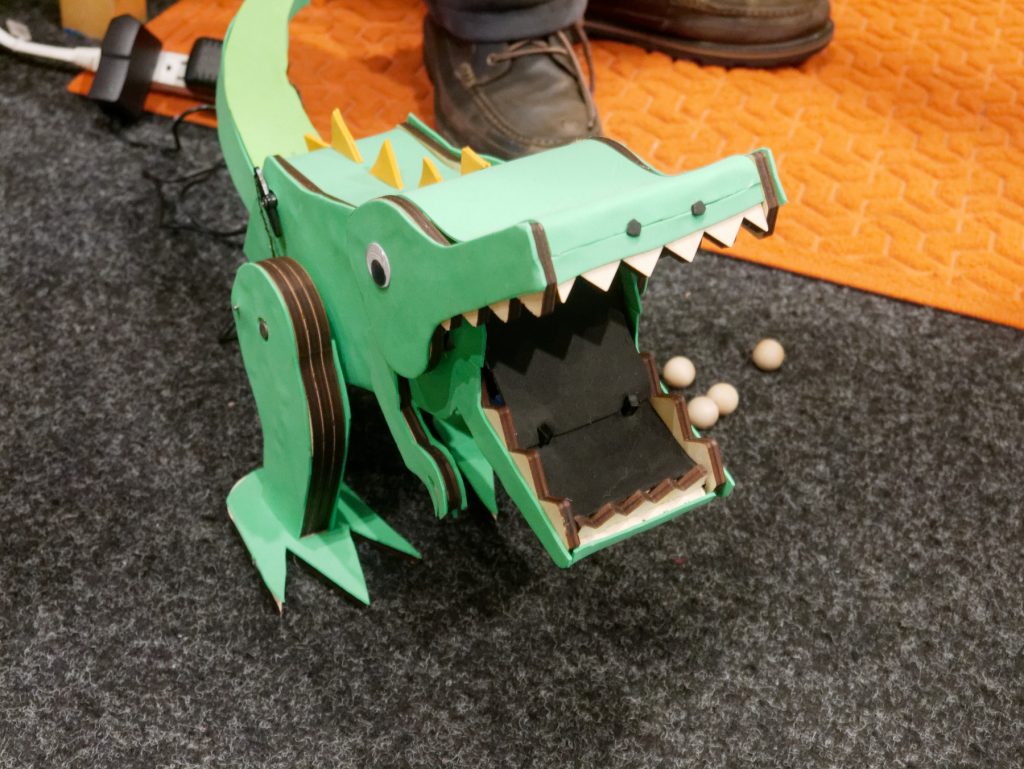
Contributions
Both authors made equal contributions in manufacturing the Dino: cutting parts, decorating, and testing. Edward made considerable bounds in updating code and finding online music sources while Quincy continued base testing functionality.
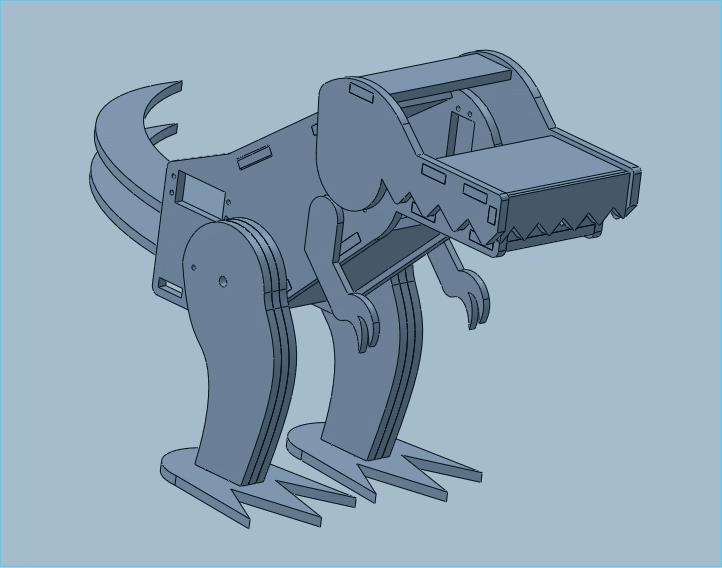
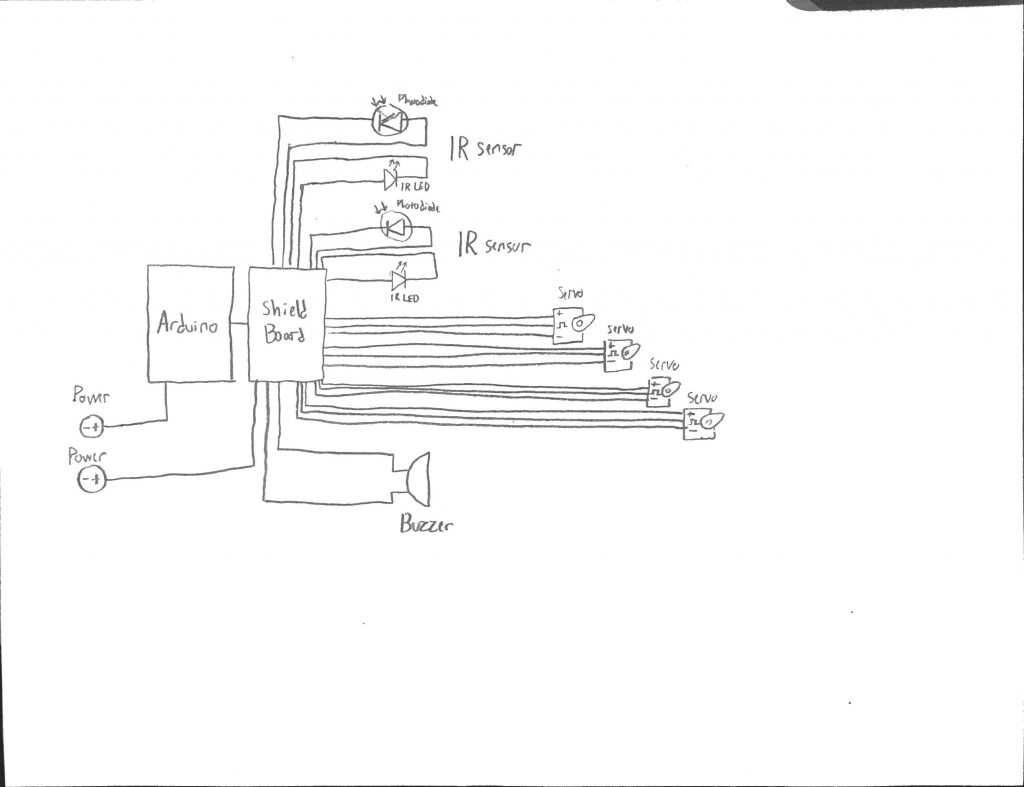
Citations
Melodies – https://github.com/robsoncouto/arduino-songs
Files
#include <Servo.h> const int SEN_PIN1 = A0; const int SEN_PIN2 = A1; const int MOUTH_SVO_PIN1 = 8; const int MOUTH_SVO_PIN2 = 7; const int LEFT_LEG_SVO_PIN = 2; const int RGHT_LEG_SVO_PIN = 4; Servo mouth_svo1, mouth_svo2, l_leg_svo, r_leg_svo; const int SVO1_OPEN = 80; const int SVO2_OPEN = 100; const int SVO1_CLOSE = 130; const int SVO2_CLOSE = 50; const int SEN1_THRES = 900; const int SEN2_THRES = 900; long chew_t = 0; long chew_start = 0; void setup() { Serial.begin(9600); mouth_svo1.attach(MOUTH_SVO_PIN1); mouth_svo2.attach(MOUTH_SVO_PIN2); l_leg_svo.attach(LEFT_LEG_SVO_PIN); r_leg_svo.attach(RGHT_LEG_SVO_PIN); open_mouth(); l_leg_svo.write(70); r_leg_svo.write(110); } long start_t = millis(); void loop() { long curr_t = millis(); int sen1_val = analogRead(SEN_PIN1); int sen2_val = analogRead(SEN_PIN2); Serial.print(chew_t); Serial.print("\t"); Serial.print(""); Serial.print("\t"); Serial.print(sen1_val); Serial.print("\t"); Serial.println(sen2_val); if ((sen1_val < SEN1_THRES) || (sen2_val < SEN2_THRES)) { if (curr_t - start_t > 500) { start_t = curr_t; if (chew_t < 10) { chew_t = curr_t; chew_start = chew_t; close_mouth(); delay(100); } } } else if (random(10000) == 0) { close_mouth(); delay(1000); open_mouth(); delay(1000); } else if (random(10000) == 0) { lean_forward(); } if (500 < chew_t) { chew(curr_t); chew_t = curr_t; if (chew_t - chew_start > 5000) { chew_t = 0; open_mouth(); delay(500); lean_forward(); } } } void chew(long curr_t) { int a = 6; int x0 = 55; mouth_svo2.write(a*sinf((float)curr_t/150) + x0); mouth_svo1.write(-a*sinf((float)curr_t/150) + (180-x0)); } void open_mouth() { mouth_svo1.write(SVO1_OPEN); mouth_svo2.write(SVO2_OPEN); } void close_mouth() { mouth_svo1.write(SVO1_CLOSE); mouth_svo2.write(SVO2_CLOSE); } void lean_forward() { l_leg_svo.write(140); r_leg_svo.write(30); delay(random(2000, 4000)); l_leg_svo.write(70); r_leg_svo.write(110); delay(1000); }
Leave a Reply
You must be logged in to post a comment.