Exercise: Servo Motion in Tinkercad¶
The most convenient device for creating movement in the course kit is the hobby servo. These are feedback-controlled motors which move an output shaft to a specified angular position. The chief limitation is a limited range of travel, typically 180 degrees of rotation.
This exercise is as much about programming style as actuation. Tinkercad supports several actuators, but since there is no means to connect mechanism, demos are limited to visual movement. So the main objective is to practice structuring a program as parameterized functions.
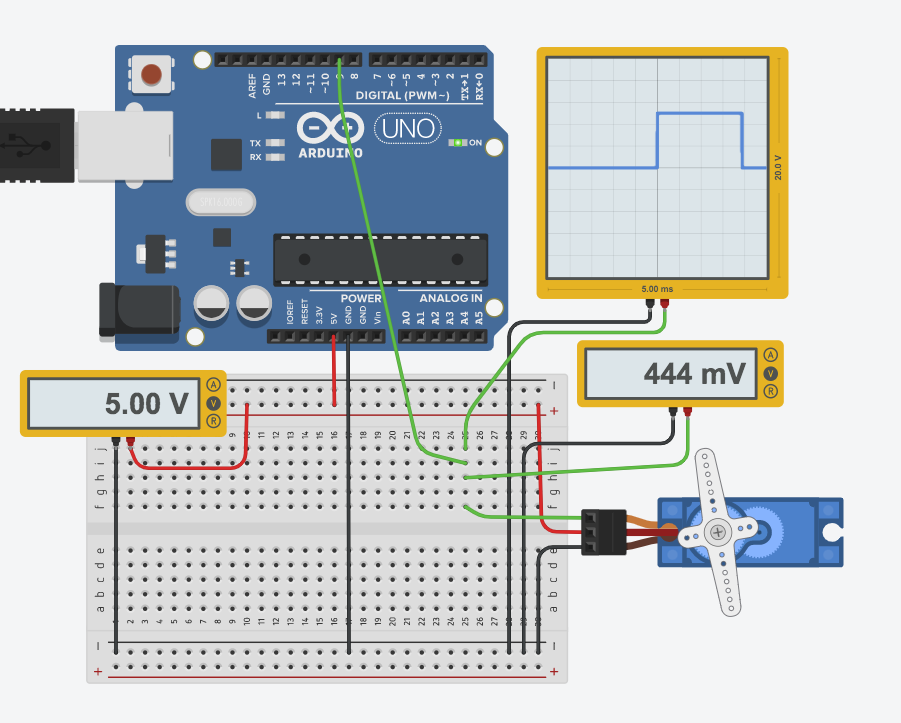
A reference circuit for the exercise. The oscilloscope is set to 500 microseconds/division horizontally and is showing 2 Volts/division vertically. This version includes two additional voltmeters to show the supply voltage and the average PWM voltage.¶
Objectives¶
After this exercise, you should be able to:
Control a hobby servomotor module.
Structure a scripted action sequence as parameterized functions.
Write code with integrated debugging using the serial port for user console communication.
Reference Guides
Please review the following reference guides as needed:
Assigned video lecture clips, including specific commentary on this exercise.
servos section of the Lecture Sample Code
Programming section of the course Reference Guide
Steps and observations¶
The overall objective is to create a choreographed servo movement using a program structured around parameterized functions.
Please construct a circuit with the required minimum components as listed in the Deliverables section: an Arduino, a hobby servo, and an oscilloscope plotting the servo control signal. The sample code generally uses D9 to control the servo, but any output from D2 to D13 is fine.
Please note that Tinkercad has several different servo models and they follow different pin ordering conventions, so please be sure to observe the pin labels carefully (i.e. pop-up rollover labels). The servo can be powered from the Arduino +5V, and please remember to connect the ground.
For this exercise, there is no prescribed user input, the movements will be performed autonomously over time.
Please set up the shell of a text-based Arduino program to support your hardware, including:
importing the Servo library
declaring a Servo object
attaching the Servo object to a hardware pin
initializing the Serial port for debugging
For this exercise, I require that your structure your program around parameterized functions. The best choices of structure depend upon your objectives, but here are some suggestions:
Functions that produce a short motion sequence to use as a compositional phrase or primitive. The parameters might relate to position, rate, or repeat count.
Functions that produce an algorithmically generated movement, i.e. one based on iterated calculations without scripting data.
Functions that produce a pseudo-random motion (see random()). The parameters might relate to the position, scale, tempo, or duration.
Functions that produce stillness. It’s an artistic question whether that is pure motionlessness or an active quiet.
Functions that represent larger units of choreography and only call motion primitive functions instead of directly issuing servo commands.
Please implement your ideas in code, then test and iterate. Please add debugging output to the Serial Monitor as needed.
Deliverables¶
The result of your explorations should include:
a single circuit sketch including code, submitted as a URL to Canvas
a brief paragraph describing your choreographic intent and outcome, submitted as text to Canvas
The circuit sketch should contain the following components:
Arduino Uno
Micro Servo
Oscilloscope
The Arduino should contain a text-based program including the following elements:
A hobby-servo choreography controller.
Multiple parameterized functions.
When it is time to submit your result for the assignment, you’ll need to click Share, then Invite people. You may then copy the generated sharing URL and submit it to Canvas.
N.B. As of Fall 2020, Tinkercad has a sharing bug, please note the following. Tinkercad Circuits sketch sharing links which include the term ‘tenant=circuits’ appear consistently to return a 404 error instead of accessing the sketch. The first workaround appears to be to exit and reload the sketch at least once before generating the share link. Alternatively, an existing link can be fixed by editing out the tenant=circuits clause, e.g. delete the text ‘?tenant=circuits’.
Challenges¶
If you would like to explore more, please consider the following optional challenge questions.
Try adding user inputs.
Try adding additional servos with coordinated movements.
Can you structure your program to issue commands to multiple servos asynchronously?