Impeller Robot Model¶
The impeller robot model simulates a single-DOF robot which can ‘bat’ objects in the plane. The model is intended as a demonstration testbed for planar juggling.
The link geometry uses only cylinder primitives. The same geometry is referenced to use as bounding objects for collision and automatic calculation of physics parameters. The base object has a NULL Physics object so it does not move, simulating a rigid connection to the ground.
The contact sensor is represented by a yellow cone. It is implemented as a DistanceSensor of limited range, pointing outward along the link axis from the base.
This model is demonstrated in the impeller-demo.wbt
world.
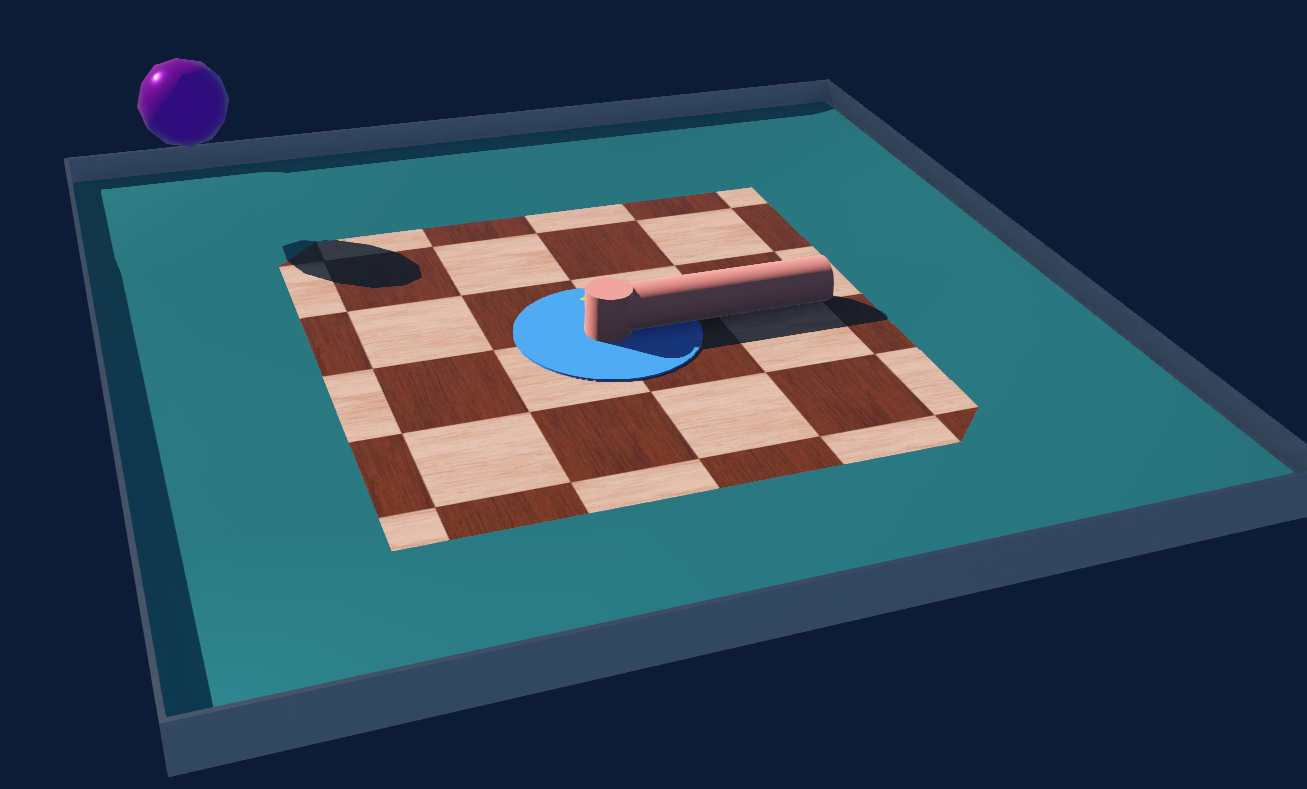
Screenshot of Webots model of impeller robot in an arena with an ElevationGrid slope around the edge.¶
Contents
Sample Control Code¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 | # impeller.py
#
# Sample Webots controller file for driving a single actuator
# attached to an 'impeller' which manipulates objects. The
# example simply moves in a periodic oscillation.
# No copyright, 2020-2021, Garth Zeglin. This file is
# explicitly placed in the public domain.
#------------------------------------------------------------------------------
print("loading impeller.py...")
# Import standard Python modules.
import math
# Import the Webots simulator API.
from controller import Robot
# Define the time step in milliseconds between controller updates.
EVENT_LOOP_DT = 50
#------------------------------------------------------------------------------
# Request a proxy object representing the robot to control.
robot = Robot()
robot_name = robot.getName()
print("%s: controller connected." % (robot_name))
# Fetch handle for the 'base' joint motors.
j1 = robot.getDevice('motor1')
# Connect to the proximity sensor.
sensor = robot.getDevice("endRangeSensor")
sensor.enable(EVENT_LOOP_DT) # set sampling period in milliseconds
#------------------------------------------------------------------------------
# Run loop to execute a periodic script until the simulation quits.
# If the controller returns -1, the simulator is quitting.
while robot.step(EVENT_LOOP_DT) != -1:
# Read simulator clock time.
t = robot.getTime()
# Change the target position target (in radians).
period = 6 # seconds
degrees = 180 * math.sin(t * (2*math.pi / period))
radians = degrees * (math.pi/180.0)
j1.setPosition(radians)
|