Over the last week, we made some important decisions regarding the wind motions we are going to achieve. We also progressed quite a bit with CAD designs, and think we can begin to laser cut and prototype this week.
First, we made a rough paper cut out as proof of concept for our light projections as well as programmed the LED strips and tested that.
We were quite impressed with the clarity of the projections and are going to go ahead and make our laser cut pieces for which the CAD looks like this:
We chose patterns based on the season (such as different sized circles to give a snow effect):
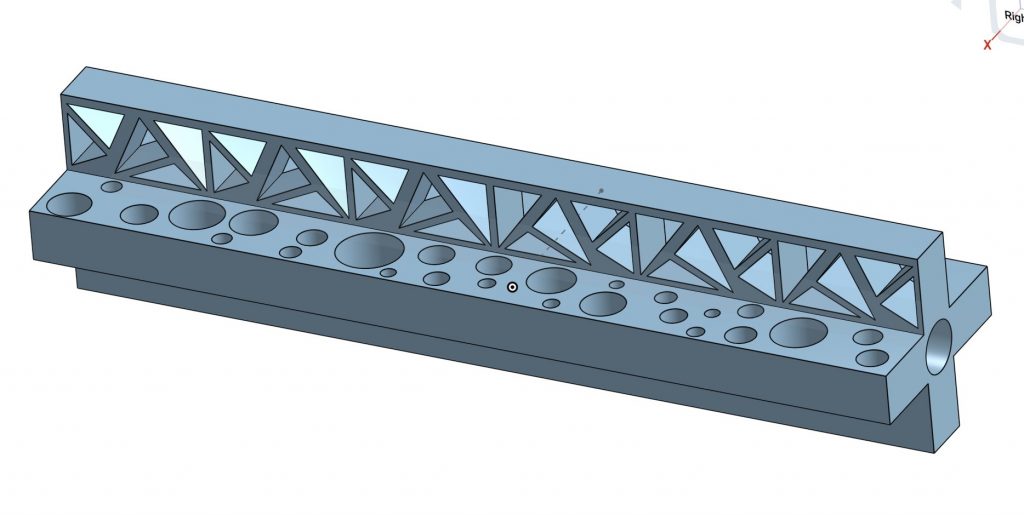
We also worked on more programming, with our next goal to be integrating code for the lights, motors, and fans into the same program. We’re using the FastLED library to control the LED strips.
//Initializing fan Pin int fan1_pin = 3; int fan2_pin = 5; int fan3_pin = 9; int led_pin = 10; void setup() { pinMode(fan1_pin, OUTPUT); pinMode(fan2_pin, OUTPUT); pinMode(fan3_pin, OUTPUT); pinMode(led_pin, OUTPUT); } void loop() { analogWrite(fan1_pin, 254); analogWrite(fan2_pin, 254); analogWrite(fan3_pin, 254); analogWrite(led_pin, 200); delay(1); }
void loop() { ChangePalettePeriodically(); static uint8_t startIndex = 0; startIndex = startIndex + 1; /* motion speed */ FillLEDsFromPaletteColors( startIndex); FastLED.show(); FastLED.delay(1000 / UPDATES_PER_SECOND); } void FillLEDsFromPaletteColors( uint8_t colorIndex) { uint8_t brightness = 255; for( int i = 0; i < NUM_LEDS; ++i) { leds[i] = ColorFromPalette( currentPalette, colorIndex, brightness, currentBlending); colorIndex += 3; } }