Performance Utility Tools¶
Some of the exercise packages include several utility and test programs. These are all standalone scripts using Python 3 and a few additional packages as described in System Requirements.
The following scripts may be browsed in the Python tools directory on the course site.
list_MIDI_ports.py¶
Command-line test program for identifying available MIDI ports by printing a list of the MIDI input and output ports available via python-rtmidi. There are no options, just running it will print a list of port names:
python3 list_MIDI_ports.py
The full code follows, but may also be downloaded from list_MIDI_ports.py.
1#!/usr/bin/env python3
2
3import rtmidi
4midiout = rtmidi.MidiOut()
5midiin = rtmidi.MidiIn()
6
7print("Available output ports:")
8for idx, port in enumerate(midiout.get_ports()):
9 print(" %d: %s" % (idx, port))
10
11print("Available input ports:")
12for idx, port in enumerate(midiin.get_ports()):
13 print(" %d: %s" % (idx, port))
midi_display.py¶
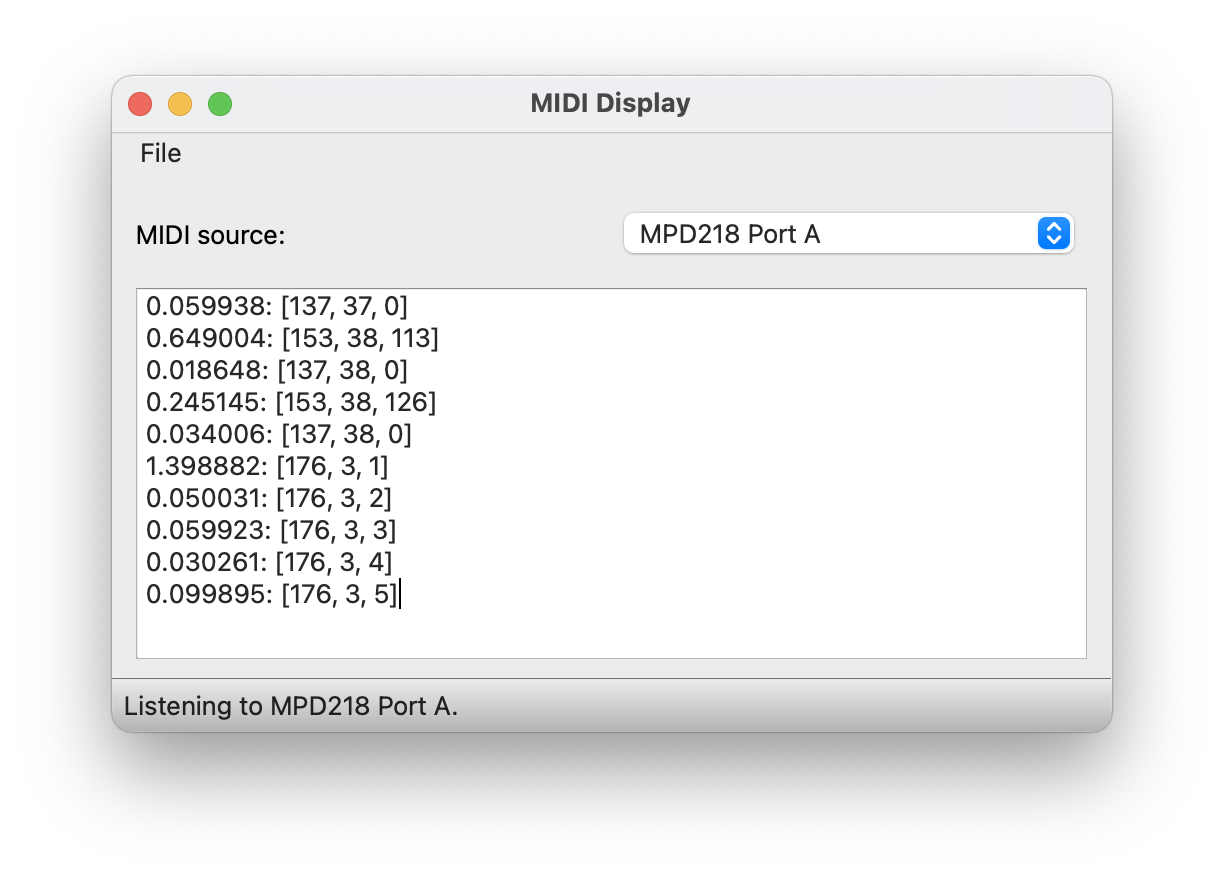
Test program for monitoring MIDI stream, providing a GUI for connecting to a MIDI source and printing all messages.
virtual_mpd218.py¶
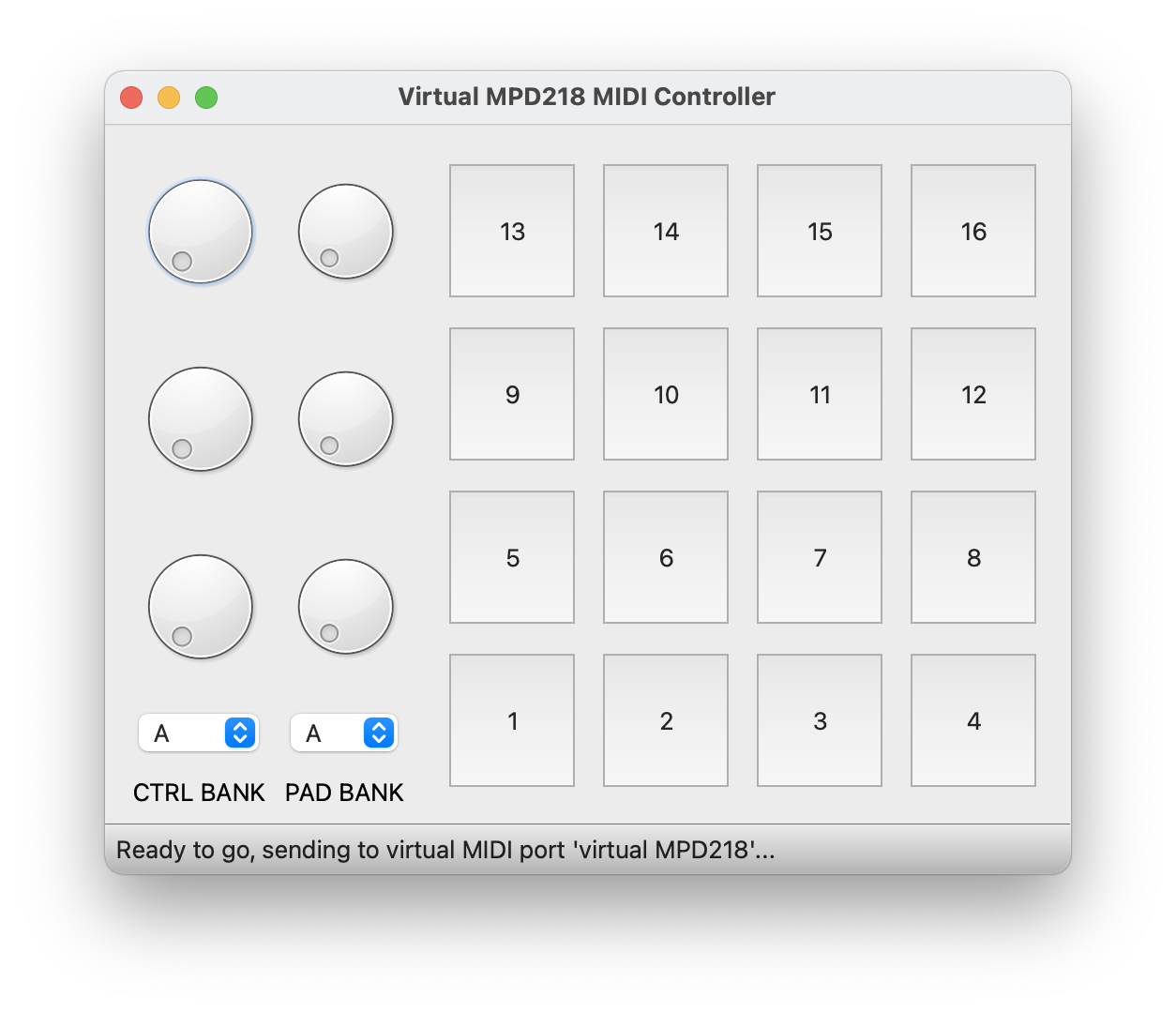
Emulation of an Akai MPD218 Drum Pad Controller, generating local MIDI events and control changes.
osc_display.py¶
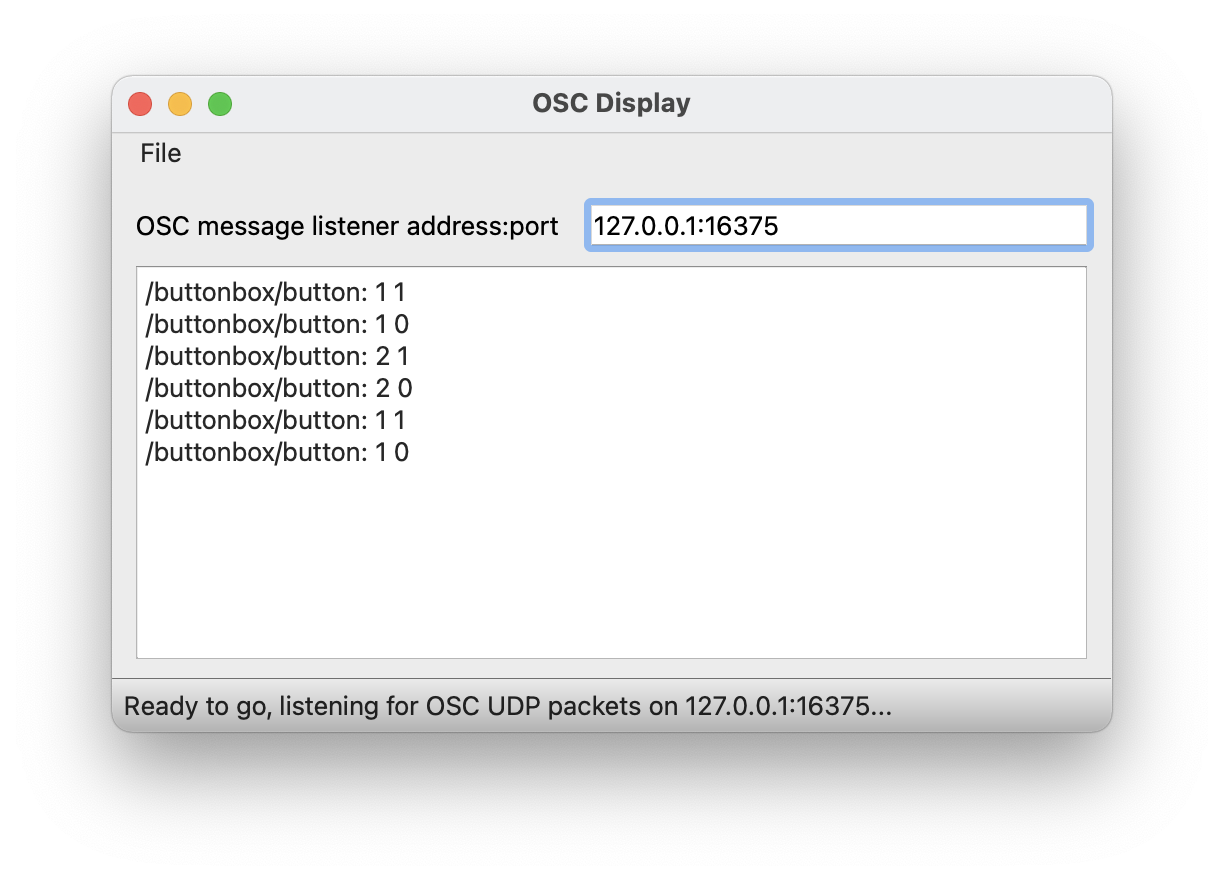
Test program for monitoring an OSC stream, providing a GUI for receiving OSC UDP packets and printing all contents.
dmx_controller.py¶
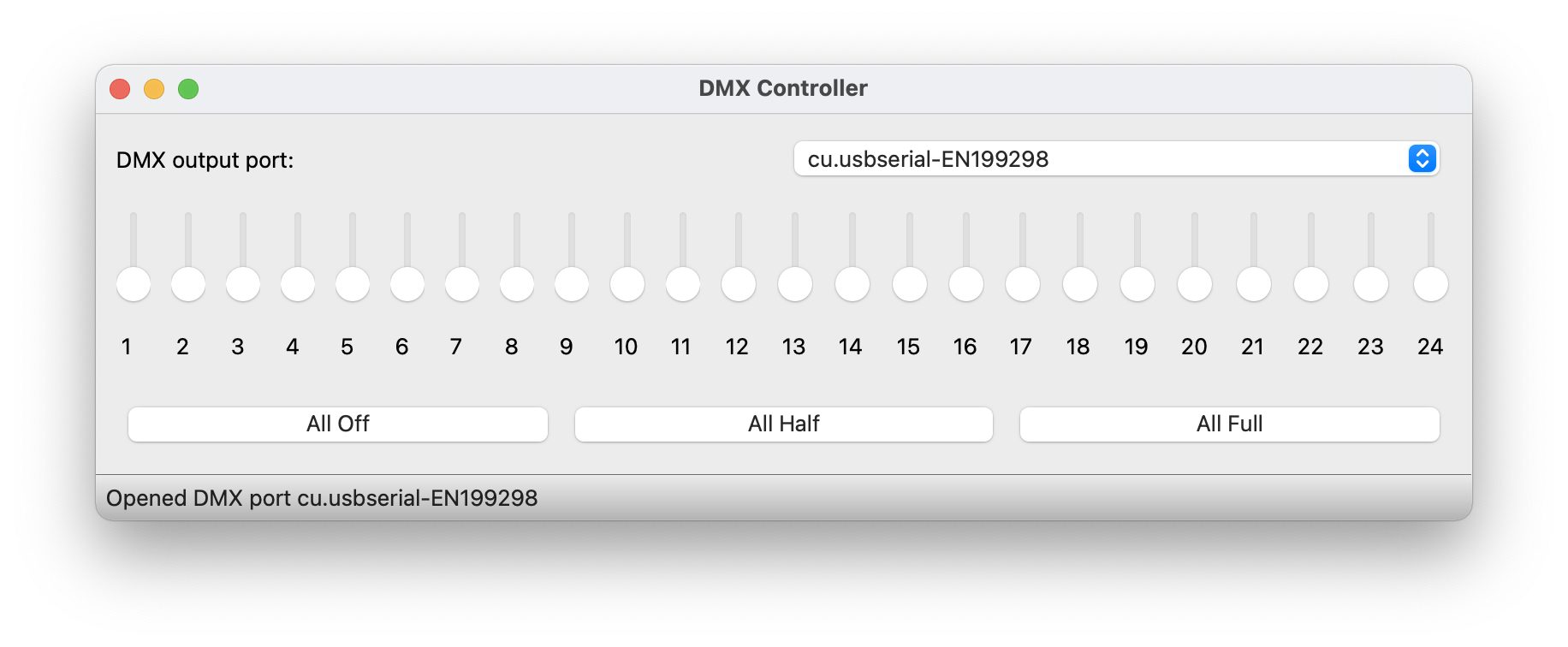
General purpose control interface for lighting control, providing a GUI for directly connecting to a single Enttec DMXUSB Pro and manipulating output.