Suitcase Robot Model¶
The suitcase robot model simulates a testbed for mounting actuation inside a suitcase. It corresponds closely to the physical prototype. It is intended as a basis for exploring hardware to add into the suitcase.
This model is demonstrated in the suitcase-demo.wbt
world.
This model makes use of several objects defined in proto files: suitcase.proto, pedestal.proto, and ball_array.proto. These make use of embedded Lua scripting to parametrically generate world objects.
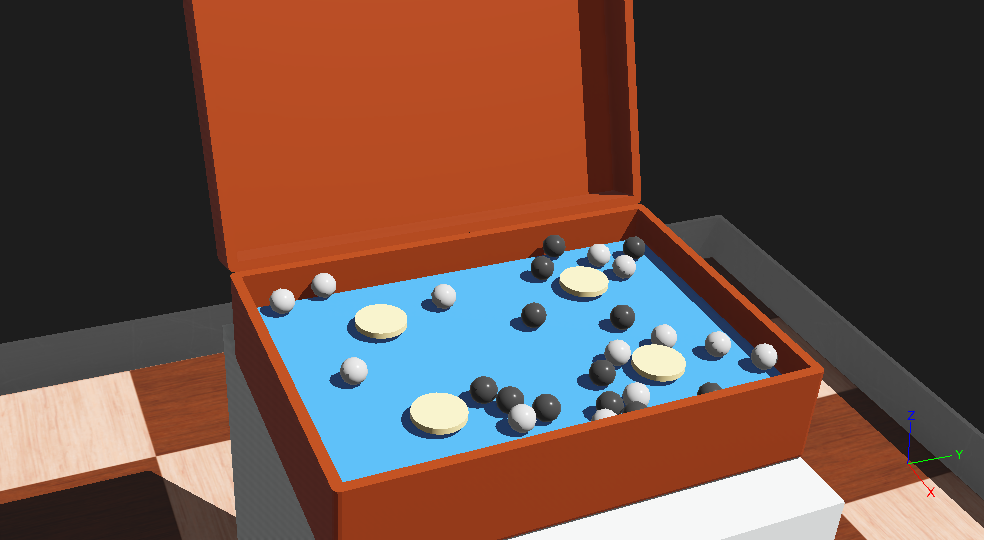
Screenshot of Webots model of actuated suitcase sitting on a gallery pedestal.¶
Sample Control Code¶
1# suitcase.py
2#
3# Sample Webots controller file for driving the four-motor suitcase actuator insert.
4# No copyright, 2021, Garth Zeglin. This file is explicitly placed in the public domain.
5
6print("loading suitcase.py...")
7
8# Standard Python imports.
9import math, random
10
11# Import the Webots simulator API.
12from controller import Robot
13
14# Define the time step in milliseconds between controller updates.
15EVENT_LOOP_DT = 200
16
17################################################################
18
19# The sample controller is defined as an class which is then instanced as a
20# singleton control object. This is conventional Python practice and also
21# simplifies the implementation of the Arduino interface which connects this
22# code to physical hardware.
23
24class Suitcase(Robot):
25 def __init__(self):
26
27 # Initialize the superclass which implements the Robot API.
28 super().__init__()
29
30 robot_name = self.getName()
31 print("%s: controller connected." % (robot_name))
32
33 # Fetch handle for the four stepper motors.
34 j1 = self.getDevice('motor1')
35 j2 = self.getDevice('motor2')
36 j3 = self.getDevice('motor3')
37 j4 = self.getDevice('motor4')
38
39 # Convenience list of all actuators.
40 self.motors = [j1, j2, j3, j4]
41
42 # Configure the motors for velocity control by setting
43 # the position targets to infinity.
44 for j in self.motors:
45 j.setPosition(float('inf'))
46 j.setVelocity(0)
47
48 return
49
50 def run(self):
51 # Run loop to execute a periodic script until the simulation quits.
52 # If the controller returns -1, the simulator is quitting.
53
54 # Time until next control event in milliseconds.
55 event_timer = 0
56 state_index = 'rest'
57
58 while self.step(EVENT_LOOP_DT) != -1:
59 # Read simulator clock time.
60 t = self.getTime()
61
62 # Change the target velocity in a cycle with a two-second period.
63 event_timer -= EVENT_LOOP_DT
64 if event_timer < 0:
65
66 if state_index == 'rest':
67 state_index = 'moving'
68 event_timer += 1500
69 for j in self.motors:
70 vel = math.pi * (1 - 2*random.random())
71 j.setVelocity(vel)
72
73 else:
74 state_index = 'rest'
75 event_timer += 500
76 for j in self.motors:
77 j.setVelocity(0)
78
79 print(f"Switched to state {state_index}")
80
81################################################################
82# If running directly from Webots as a script, the following will begin execution.
83# This will have no effect when this file is imported as a module.
84if __name__ == "__main__":
85 robot = Suitcase()
86 robot.run()