Helen’s Vest
The hand pump inflates the heart shaped organ. Inflating the heart tightens the vest, offering physical security to the user.
The motor pump inflates the collar. The inflated collar offers comfort and protection around the neck.
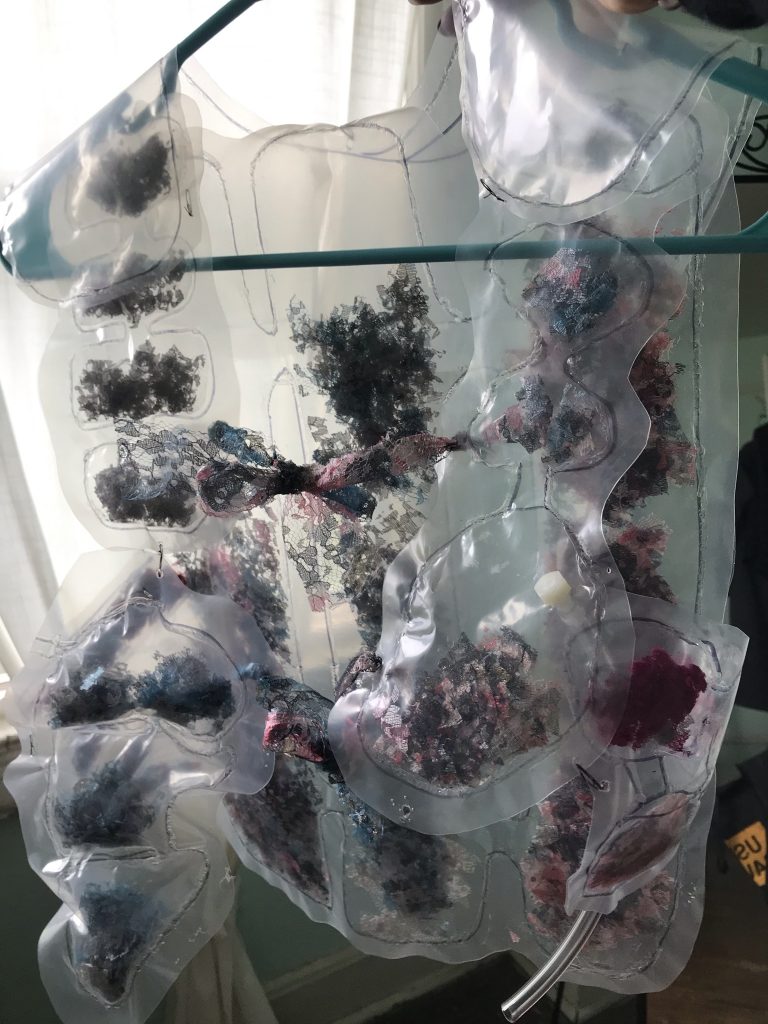
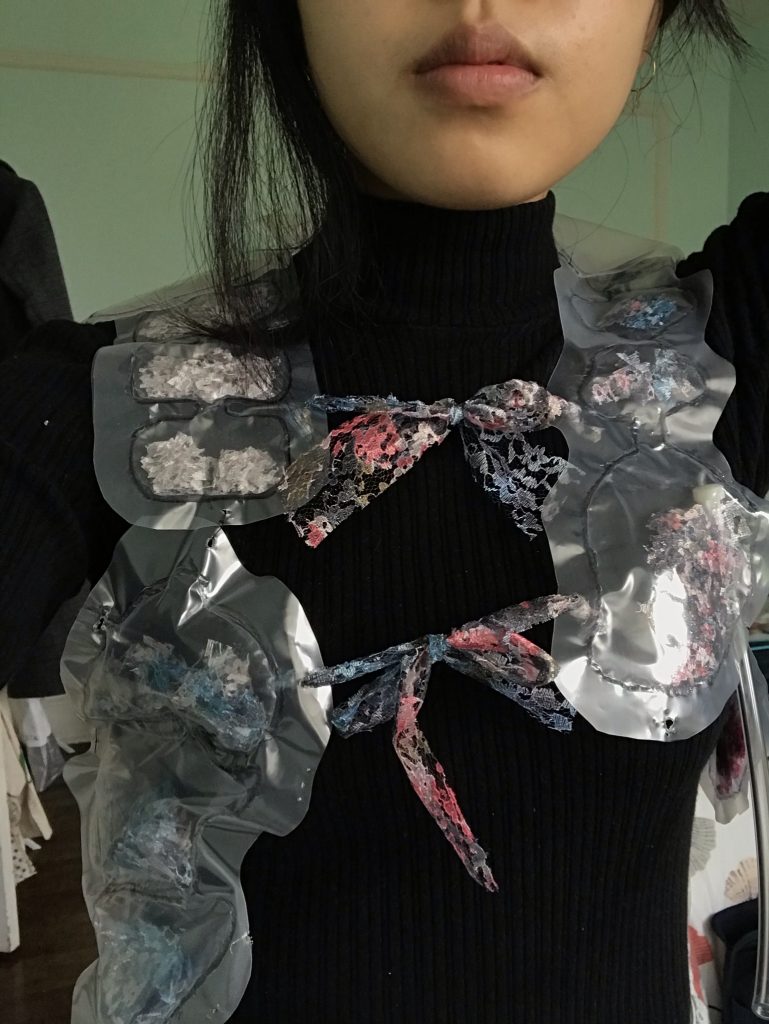
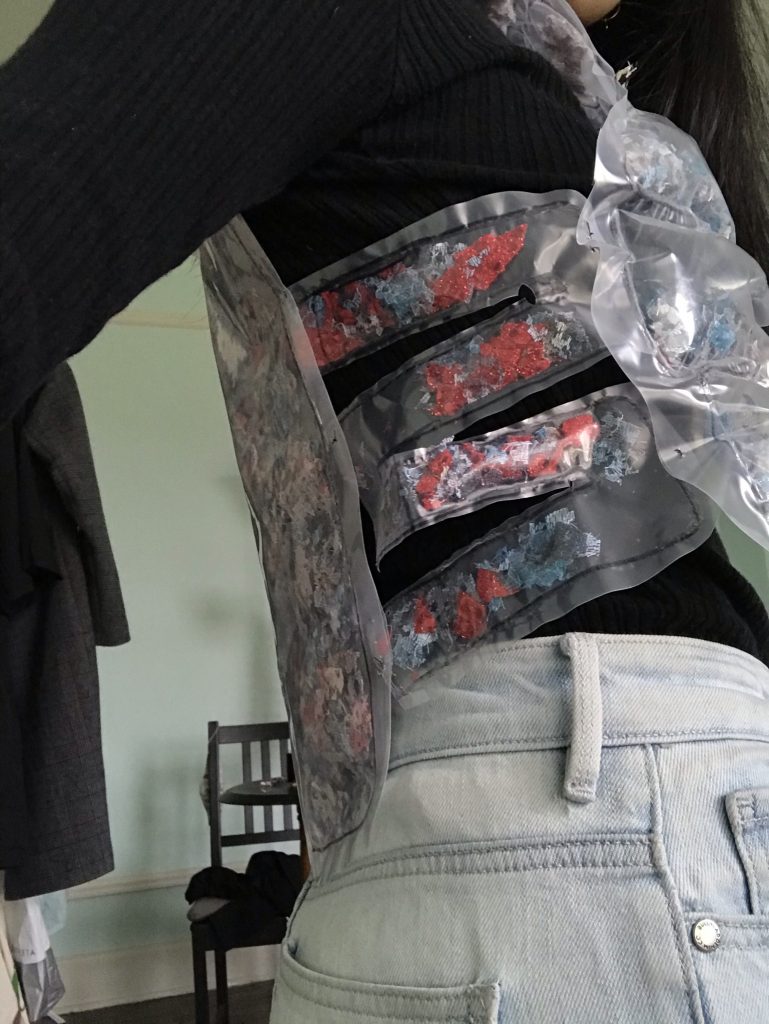
Why inflate the collar? The inflation of the collar creates comfort and protection. The comfort comes from the collar’s similarity to a travel pillow. The protection comes from the fact that the inflated collar shields a vulnerable area. Often when people are anxious, their hand goes to their neck as a subconscious way to protect themselves.
Still to do: add more movement and textiles to the collar to enhance the effect, finishing touches on the body of the vest,
Rebecca’s Vest
I am planning to use an inflatable pouch motor to slowly rise the way a garage door does to reveal the safety kit underneath. See a prototype perform that here:
It travels a considerable vertical distance.
I was alternating between the ideas of inflating one large vacuum and inflating a bunch of smaller pouches. To create each small pouch, I added an extra segment that would hold the bolt connector and be soldered off after inflating the pouch with the motor pump. However, after spending too much time troubleshooting each pouch and looking for holes and accidentally soldering off vinyl, I decided to commit to the idea of creating a one-piece vest.
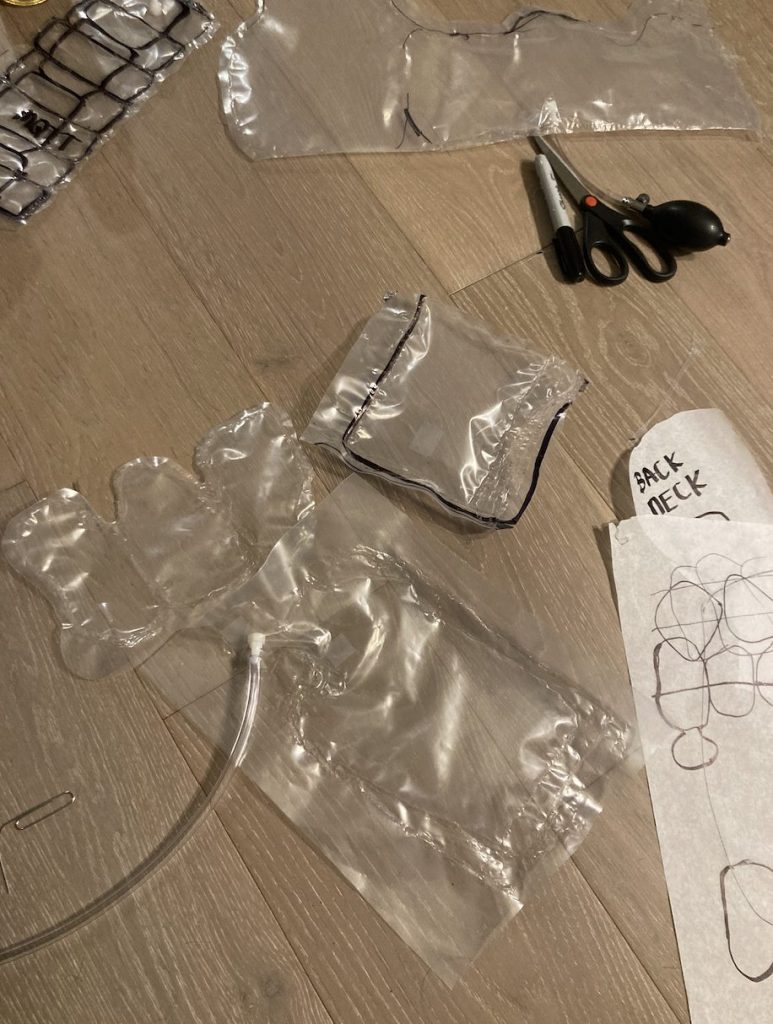
Following up on the last check-in post, I created “darts” in a one piece vest to form folds around the bends of the body. With the dart:
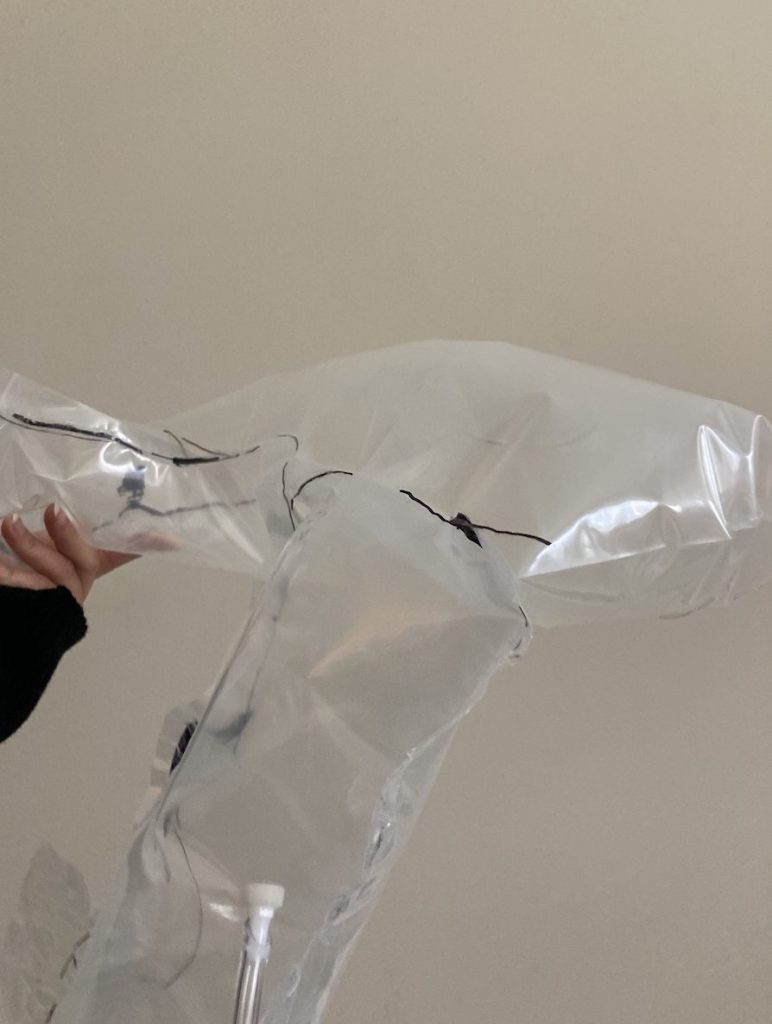
Without the dart:
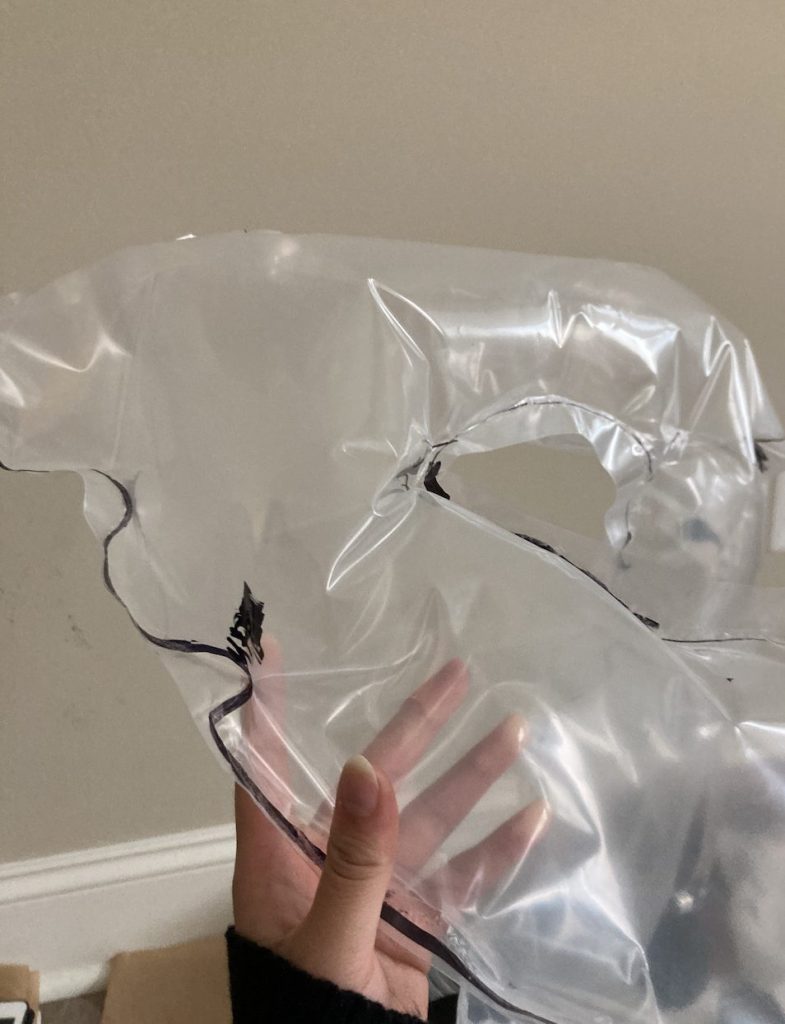
Because there is only one motor pump which is going to inflate the pouch motor shown in the video, I considered using the heart pump to inflate the one-vacuum vest. I quickly discarded that idea.
So, I have to create three separate compartments: one pouch motor, one segment to inflate with the heart pump so I can send Helen my signal, and the body of the vest (which will hold my safety kit).
Code
""" Project 2: Active Proxy Body Helen Yu (heleny1), Rebecca Kim Written by Helen Yu Last Updated: 5/4/21 Summary: When you clench your pump and inflate your vest, your partner's vest leaps into defense mode. A hobby servo helps control the defense mechanism, be it spikes, projectiles, webs, etc. Inputs: Soft Touch Sensor on A1 Outputs: Motor Pump on A2 """ # ---------------------------------------------------------------- # Import any needed standard Python modules. import time, math, sys # Import the board-specific input/output library. from adafruit_circuitplayground import cp # Import the low-level hardware libraries. import board import digitalio import analogio import pwmio # Import the Adafruit helper library. from adafruit_motor import servo # Import the runtime for checking serial port status. import supervisor # ---------------------------------------------------------------- # Initialize hardware. # Configure the digital input pin used for its pullup resistor bias voltage. bias = digitalio.DigitalInOut(board.D10) # pad A3 bias.switch_to_input(pull=digitalio.Pull.UP) # Configure the analog input pin used to measure the sensor voltage. sensor = analogio.AnalogIn(board.A1) scaling = sensor.reference_voltage / (2**16) # Create a PWMOut object (motor pump) on pad A2 to generate control signals. pwm = pwmio.PWMOut(board.A2, duty_cycle=0, frequency=2000) # ---------------------------------------------------------------- # Initialize global variables for the main loop. remote_touch = [0] # Measure the time since the last remote move message, and reset after a period without data. remote_touch_timer = False # Convenient time constant expressed in nanoseconds. second = 1000000000 # Integer time stamp for the next console output. sensing_timer = time.monotonic() # Integer time stamp for next behavior activity to begin. next_activity_time = time.monotonic_ns() + 2 * second # Flag to trigger motion. inflate_state = False phase_angle = 0.0 cycle_duration = 12 # seconds per cycle phase_rate = 2*math.pi / cycle_duration # radians/second # Integer time stamp for next servo update. next_pump_update = time.monotonic_ns() # The serial port output rate is regulated using the following timer variables. serial_timer = 0.0 serial_interval = 0.5 # ---------------------------------------------------------------- # Begin the main processing loop. while True: # Read the current integer clock. now = time.monotonic() #---- soft sensor input and display ----------------------------- # Read the integer sensor value and scale it to a value in Volts. volts = sensor.value * scaling # Normalize the soft sensor reading. Typically you'll need to adjust these values to your device. low_pressure_voltage = 0.65 high_pressure_voltage = 0.20 pressure = abs((high_pressure_voltage - volts) / (high_pressure_voltage - low_pressure_voltage)) # Check the serial input for new line of remote data if supervisor.runtime.serial_bytes_available: line = sys.stdin.readline() tokens = line.split() if len(tokens) == 1: try: remote_touch = [int(token) > 0 for token in tokens] remote_touch_timer = 4.0 except ValueError: pass #---- periodic console output ----------------------------------- # Poll the time stamp to decide whether to emit console output. if now >= sensing_timer: sensing_timer += 100000000 #0.1sec if pressure > 0.5: remote_touch_timer = now + 4000000000 # 4 sec timeout if now >= serial_timer: serial_timer += serial_interval touch = ["1" if pressure > 0.5 else "0"] print(pressure) print(" ".join(touch)) # If a slow movement has been received, sweep twice at a constant speed if inflate_state is True: pwm.duty_cycle = 2**16-1 print("Defense activated.") time.sleep(10) pwm.duty_cycle = 0 print("Defense deactivated") inflate_state = False if any(remote_touch): # Check whether there was any remote movement if remote_touch[0]: inflate_state = True print("Engage defense") remote_touch = [False] #---- periodic servo motion commands ---------------------------- # If the time has arrived to update the servo command signal: if now >= next_pump_update: next_pump_update += 20000000 # 20 msec in nanoseconds (50 Hz update)
Leave a Reply
You must be logged in to post a comment.