THE MUSICAL BUG

Front View of the Musical Bug

Closeup of Musical Buttons

Suprise Flag

Musical Bug as Suprise is Triggered

Musical Bug After Suprise
Narrative
This robot looks like a car with piano keys, making the user think that the songs and wheels might work together somehow. However, if the user hits a specific note the robot enters an infinite loop of a theme song and a flag breaks the top of the robot and shows a surprised face. This also surprises the user and renders the piano unusable. After that point, the “Bug” breaks and the keyboard no longer works.
Process & Discussion
Initially, the piano was designed to hide the wheels of the robot and if the user hit the wrong note the robot would run away. However, after much trial and error with motors that didn’t work and wires that weren’t connecting, we decided that movement was an unreliable source to depend on for our surprise. Thus, we decided to still incorporate the original structure but come up with a different idea for the surprise. The flag still gives the same idea as the robot running away as well as being completely unexpected. Because the robot looks so complex, the result of such a simple and useless reaction is surprising.
Some design challenges we had was how to deliver the surprise. The tissue paper was used to have an easy barrier for the flag to break. This component was decided after testing multiple different fabrics and materials such as regular paper, tape, thin fabric, paper towels, and a lid. We decided that the most reliable barrier was to use tissue paper to ensure that the flag would be able to break through. Another consideration was the form of the device. After experimenting with paper, plastic boxes, and different forms of cardboard boxes, we decided to stick with a regular cardboard box to keep the mystery of the device.
It was challenging to come up with a good idea for this project, and we ended up thinking about things we would be surprised seeing ourselves. We also learned to test motors before attaching them to the robot to ensure that they will work. Moving forward, we could better this project by concealing the surprise flag so that it does not stick out the bottom of the device. Or, to encourage the idea that the device is a piano, we could make the form of the device mimic a traditional piano. Thus, after a note or sequence of notes is pressed, the device would pop a flag out and then play an endless loop of a song. We also could have thought of a more unexpected thing that a piano could do such as run away.
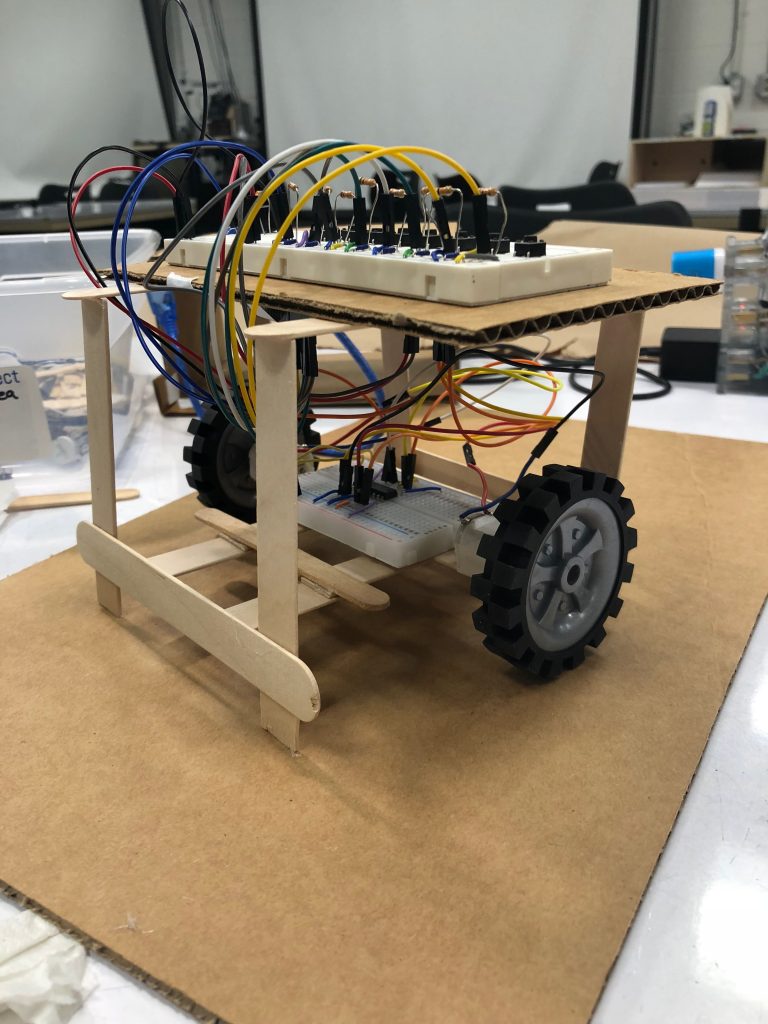
Originally, we worked with two motors and concentrated on building a structure that encloses the wheels and wires. We ran into multiple issues with this. The biggest were: 1- there was too much friction between the ground and anything that touched it that was not the wheels. 2- the 2 motors seemed too weak to carry the entire components.

Naturally, our next step was to build the entire structure to see how much it would weigh and using a larger power source, and stronger motors. We kept tweaking the box and the amount of material that touched the ground. Still no luck. It was too heavy and the friction was too high.

This is when we decided to use 4 wheels and 4 motors, that way all the objects touching the ground were movable, which reduced the friction by a lot. At this point, the robot functioned the way it was supposed to and moved really well. However, when we came back to our next work session to build the encasement for the robot, we realized two out of four of the motors were not working. One was just not working and the other had a faulty wire. We soldered new wires to the motor, but it still was not working properly. We replaced the other motor with a working one, and tried to make-do with 3/4 working motors, but the movement was not as smooth as before. After a lot of trial and error, we got to a point where 2/4 motors were not reliable, and one motor would occasionally work and occasionally not. At that point, we decided to switch courses and try something else that is surprising. We already had the wheels built and wired, so we decided to use that as an optical illusion, making the users think it is a functional moving robot with wheels.
Schematic

Schematic for Musical Bug
Code:
- // Musical Bug
- // Jenny Han
- // Ghalya Alsanea
- // This code needs an Arduino, servo, speaker, and 7 buttons.
- // This device assigns each button to a note and plays music.
- // If the button G is pressed, the rest of the buttons become
- // disabled and the device releases a flag while playing a song
- // on repeat.
- #include<Servo.h>
- Servo servo1;
- const int SERVO;
- const int SERVOPIN = 11;
- int SPEAKER = 9;
- //Setting the tones for the scale
- int BUTTONC = 8;
- int C = 261;
- int BUTTOND = 7;
- int D = 294;
- int BUTTONE = 6;
- int E = 329;
- int BUTTONF = 5;
- int F = 349;
- int BUTTONG = 4;
- int G = 392;
- int BUTTONA = 3;
- int A = 440;
- int BUTTONB = 2;
- int B = 492;
- void setup() {
- //initialize inputs and outputs
- pinMode(SPEAKER, OUTPUT);
- pinMode(BUTTONC,INPUT);
- pinMode(BUTTOND,INPUT);
- pinMode(BUTTONE,INPUT);
- pinMode(BUTTONF,INPUT);
- pinMode(BUTTONG,INPUT);
- pinMode(BUTTONA,INPUT);
- pinMode(BUTTONB,INPUT);
- servo1.attach(SERVOPIN);
- servo1.write(0);
- Serial.begin(9600);
- }
- void loop() {
- int buttonC = digitalRead(BUTTONC);
- int buttonD = digitalRead(BUTTOND);
- int buttonE = digitalRead(BUTTONE);
- int buttonF = digitalRead(BUTTONF);
- int buttonG = digitalRead(BUTTONG);
- int buttonA = digitalRead(BUTTONA);
- int buttonB = digitalRead(BUTTONB);
- //play music except for button G, the sequence starts
- if (buttonC) {
- tone(SPEAKER,C,100); //C
- Serial.println("pressed!");
- }
- if (buttonD) {
- tone(SPEAKER,D,100); //D
- }
- if (buttonE) {
- tone(SPEAKER,E,100); //E
- }
- if (buttonF) {
- tone(SPEAKER,F,100); //F
- }
- //if note hit then play song and release flag
- if (buttonG) {
- tone(SPEAKER,G,100); //G
- Serial.println("do a song!");
- servo1.write(180);
- song();
- }
- if (buttonA) {
- tone(SPEAKER,A,100); //A
- }
- if (buttonB) {
- tone(SPEAKER,B,100); //B
- }
- }
- //song sequence that plays when hit a certain note
- void song()
- {
- while (true)
- {
- tone(SPEAKER,F,100); //F
- delay(300);
- tone(SPEAKER,E,100); //E
- tone(SPEAKER,F,100); //F
- delay(200);
- tone(SPEAKER,A,100);
- tone(SPEAKER,B,100); //F
- delay(400);
- tone(SPEAKER,D,100);
- delay(200);
- tone(SPEAKER,C,100);
- delay(200);
- tone(SPEAKER,E,100);
- delay(100);
- tone(SPEAKER,G,100);
- delay(200);
- tone(SPEAKER,A,100);
- delay(200);
- tone(SPEAKER,A,100);
- delay(300);
- tone(SPEAKER,F,100);
- }
- }
Leave a Reply
You must be logged in to post a comment.