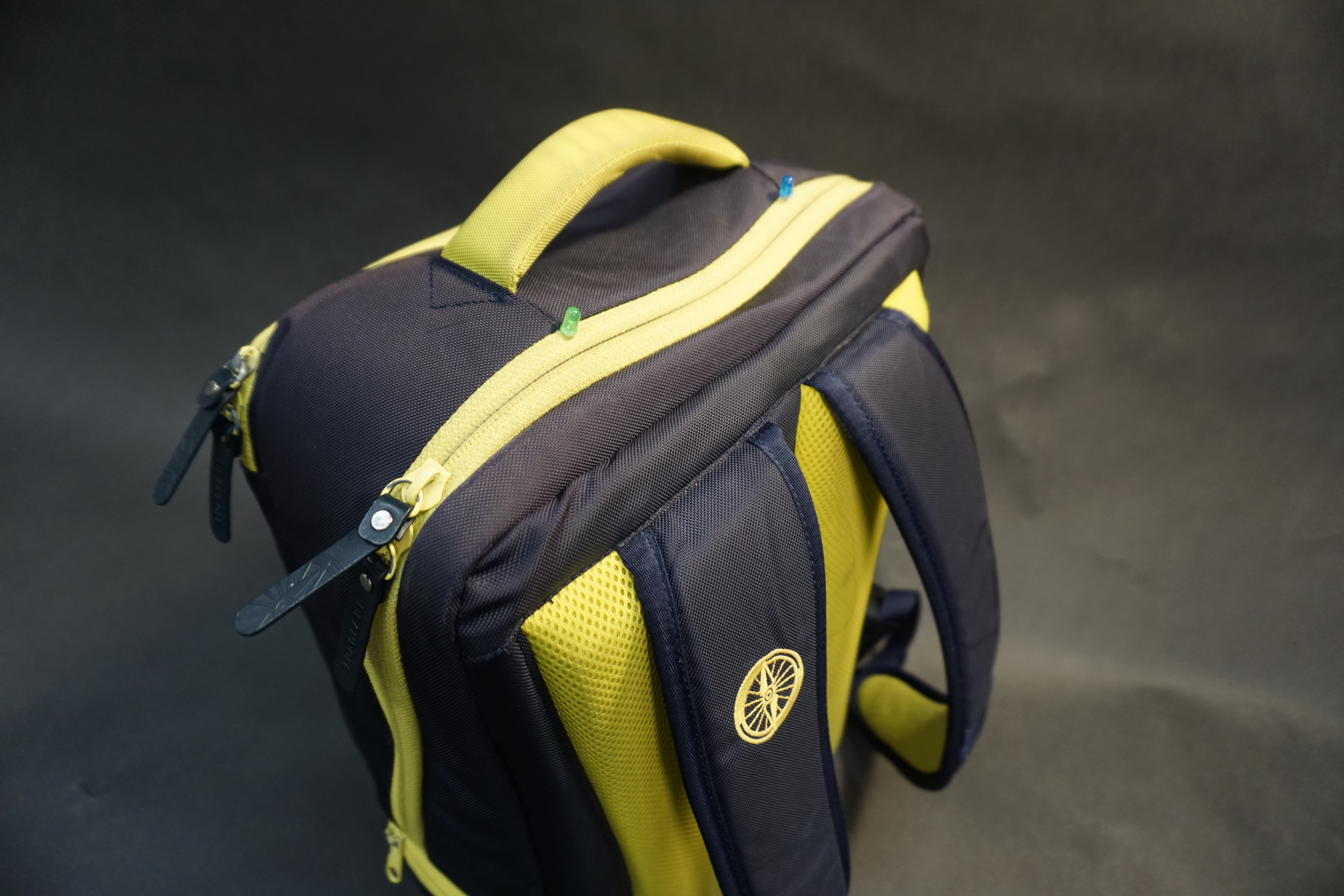
The LEDs poking out indicate if the chargers are inside my backpack in an organized fashion.
This backpack lets me know if I have the chargers I need in my backpack by lighting up the LEDs on the top and forces me to organize them at the same time.
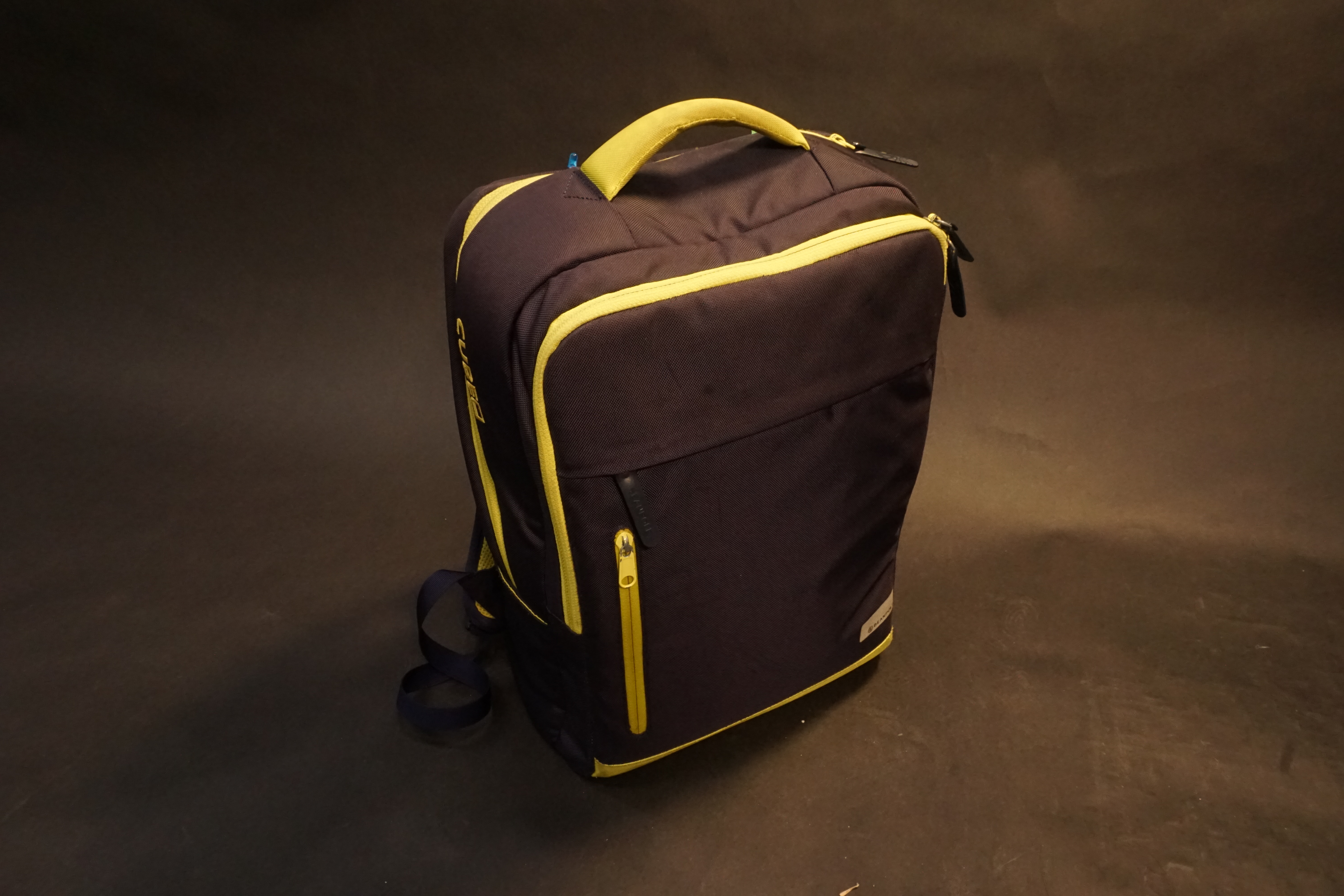
Front view of the Semi Hi-Tech Backpack
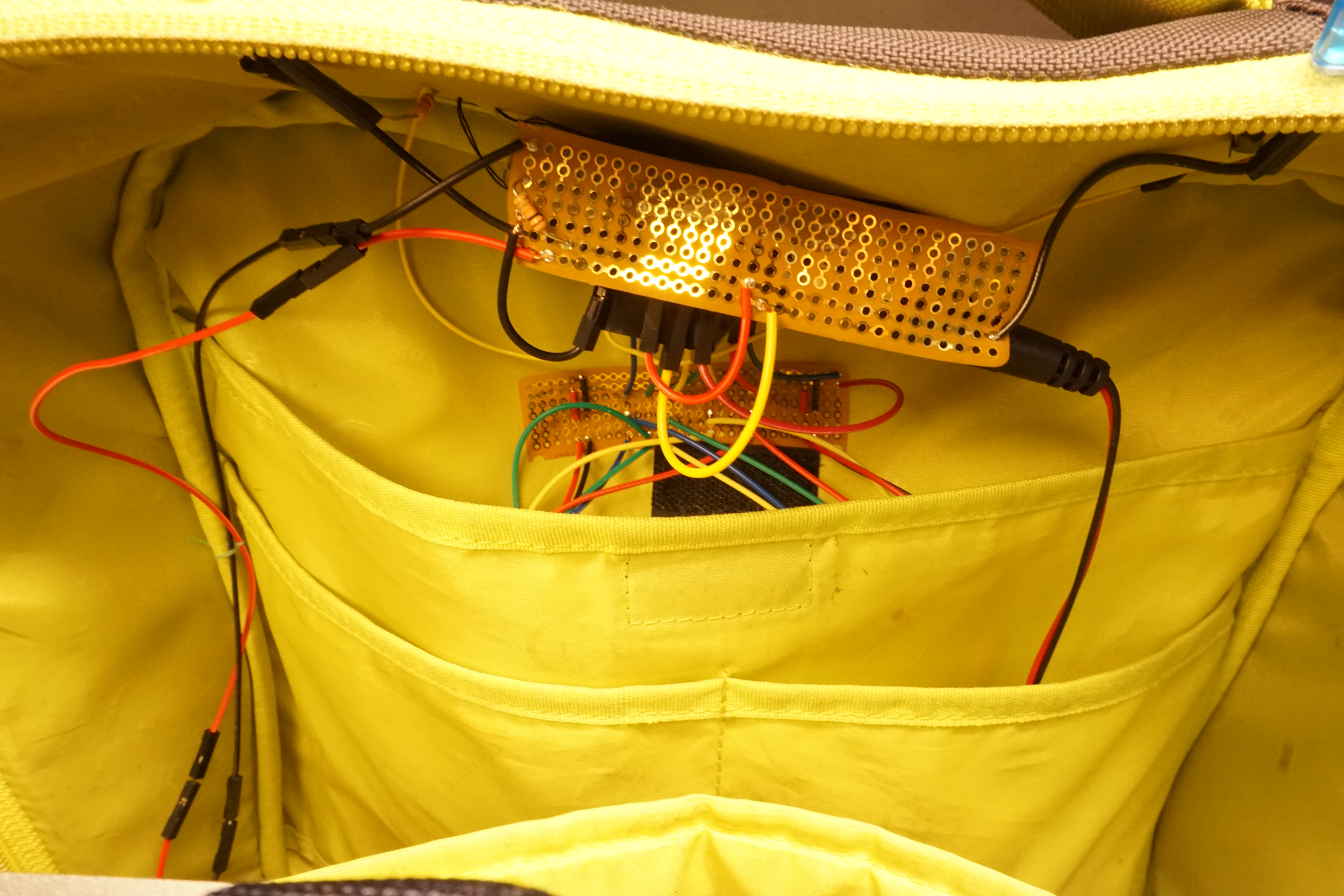
Overview of the electronics inside the backpack
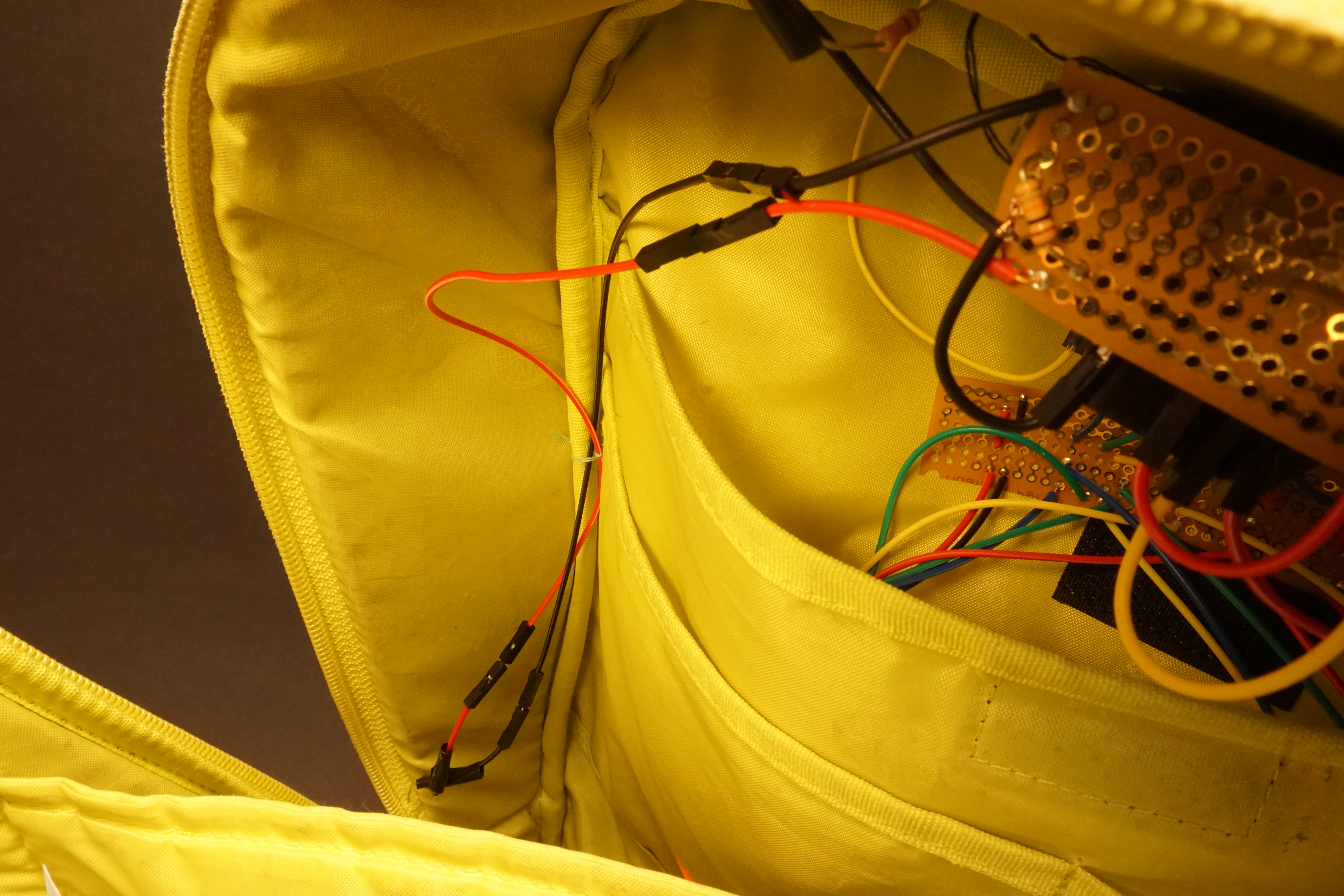
Wire for the pressure sensor on the bottom of the backpack runs down the side.
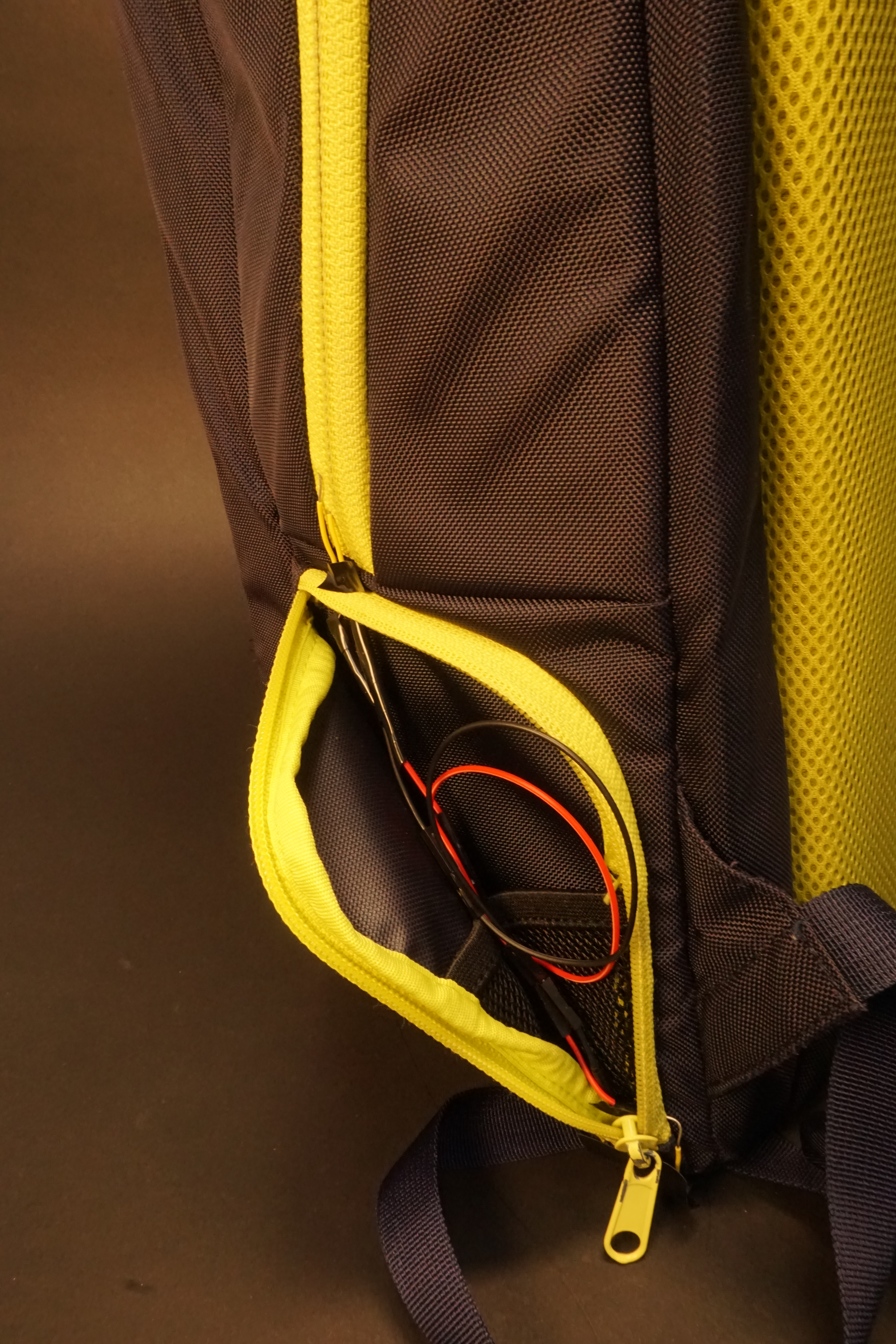
Wire connecting the pressure sensor is hidden using the side pocket.
Code for the project:
/* Project Title: Semi Hi-Tech Backpack Description: The project uses two RFID sensors to read RFID tags and compare it to the IDs of known tags. When the correct RFID tag is read by the reader, respective LEDs would turn on to indicate that the RFID tag has been sensed. This entire code will only run when the force sensitive resistor reads pressure, which is equal to when the backpack is put on the floor. RST Pin 5 MOSI Pin 11 MISO Pin 12 SCK Pin 13 */ //include libraries #include <SPI.h> #include <MFRC522.h> //define important rfid reader pins #define RST_PIN 5 #define SS_1_PIN 9 //same to reader 0 #define SS_2_PIN 10 //same to reader 1 //some necessary RFID stuff #define NUM_OF_READERS 2 MFRC522 mfrc522[NUM_OF_READERS]; //Create MFRC522 instance //set "simpler" pins const int PRESSUREPIN = A0; const int LED1PIN = 2; const int LED2PIN = 3; //"simpler" variables int force; void setup() { //Initiate SPI bus SPI.begin(); //Initiate each MFRC522 card mfrc522[0].PCD_Init(SS_1_PIN, RST_PIN); mfrc522[1].PCD_Init(SS_2_PIN, RST_PIN); //set up "simpler" pins pinMode (PRESSUREPIN, INPUT); pinMode (LED1PIN, OUTPUT); digitalWrite (LED1PIN, LOW); pinMode (LED2PIN, OUTPUT); digitalWrite (LED2PIN, LOW); } void loop() { //read pressure force = analogRead(PRESSUREPIN); //IDs I'm looking for byte card1[4] = {69, 96, 94, 201}; byte card2[4] = {181, 70, 94, 201}; //backpack is resting on the floor 🙂 if (force > 100) { //create variables for rfids that the reader finds byte readCard1[4]; byte readCard2[4]; //Sensing the stickers if (mfrc522[0].PICC_IsNewCardPresent() && mfrc522[0].PICC_ReadCardSerial()) { byte readCard1[4]; for (uint8_t i = 0; i < 4; i++) { //put the id number that the reader found into the variable readCard1[i] = mfrc522[0].uid.uidByte[i]; } //compare the wanted id and found id if (compareArrays(readCard1, card1)) { digitalWrite(LED1PIN, HIGH); } } if (mfrc522[1].PICC_IsNewCardPresent() && mfrc522[1].PICC_ReadCardSerial()) { byte readCard2[4]; for (uint8_t i = 0; i < 4; i++) { readCard2[i] = mfrc522[1].uid.uidByte[i]; } if (compareArrays(readCard2, card2)) { digitalWrite(LED2PIN, HIGH); } } //Halt PICC mfrc522[0].PICC_HaltA(); mfrc522[1].PICC_HaltA(); //Stop encryption on PCD mfrc522[0].PCD_StopCrypto1(); mfrc522[1].PCD_StopCrypto1(); } //if backpack is off the floor, reset every value else { digitalWrite(LED1PIN, LOW); digitalWrite(LED2PIN, LOW); } } bool compareArrays(byte array1[], byte array2[]) { for (int i = 0; i < 4; i++) { if (array1[i] != array2[i]) return false; } return true; }
(Schematic photo)
Process
The problem I needed to solve was dealing with the monstrosity of chargers I carried everyday. I usually carry four chargers, which are for my laptop, iPad, cellphone, and wireless earbuds. I had to carry them all the time because otherwise it would be a painful day to have a laptop or phone not working. On top of that, unfortunately, none of the chargers were the same, so I had to carry four different wires which eventually tangled with each other and created a big mess in my backpack.
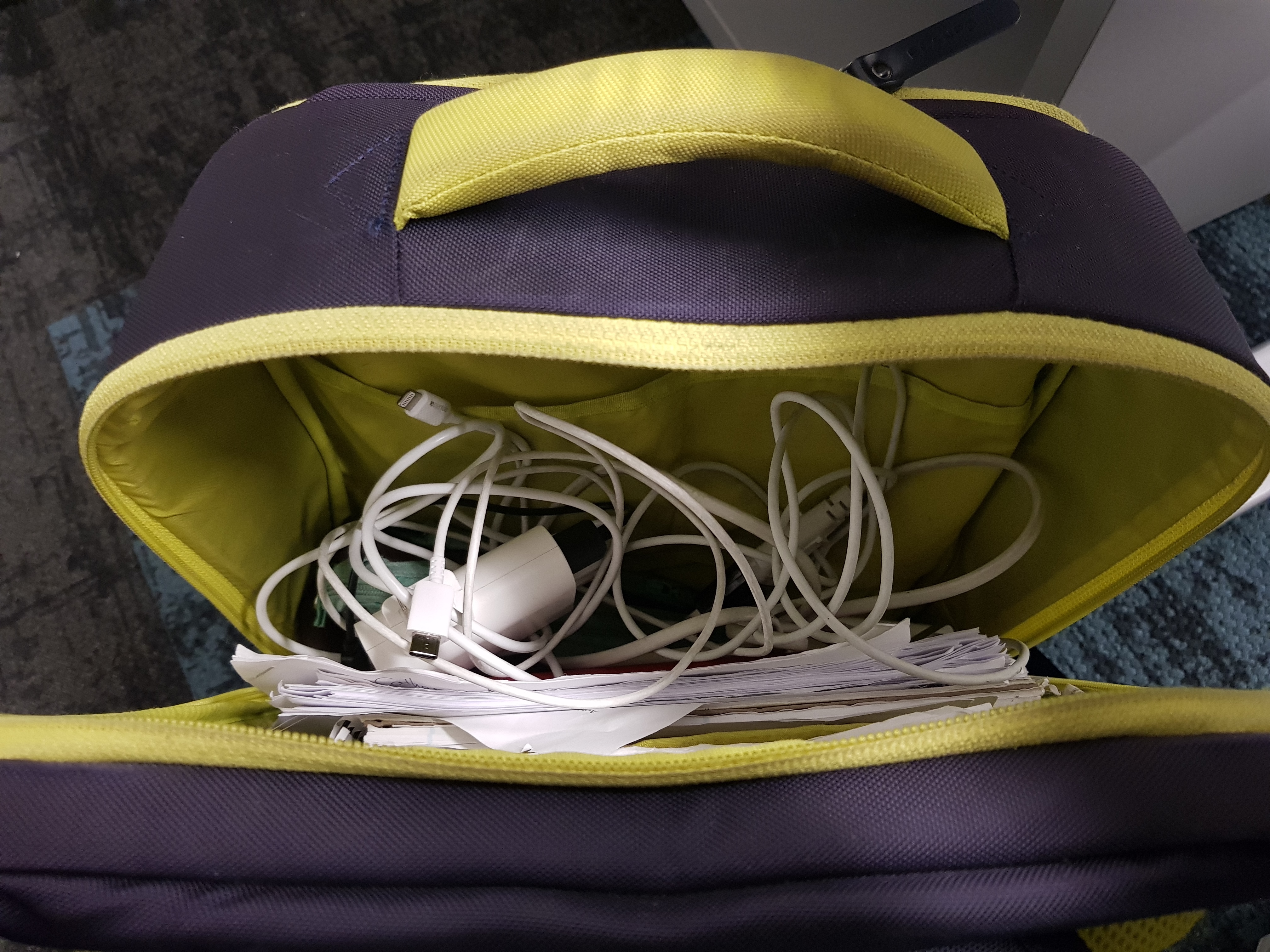
Chargers inside my backpack are all tangled up.
My initial idea was to create a small box in which I could organize all of my chargers inside. Since I had four wires, the idea was to have a RFID tag on each of the wires and have four RFID readers to sense that each wire has been placed in the right section of the box. The readers would look for the tag that they have been assigned to, and once they find the correct tag, they would turn on the LED on the outside and all I would need to grab would be the box with the LED lit up.
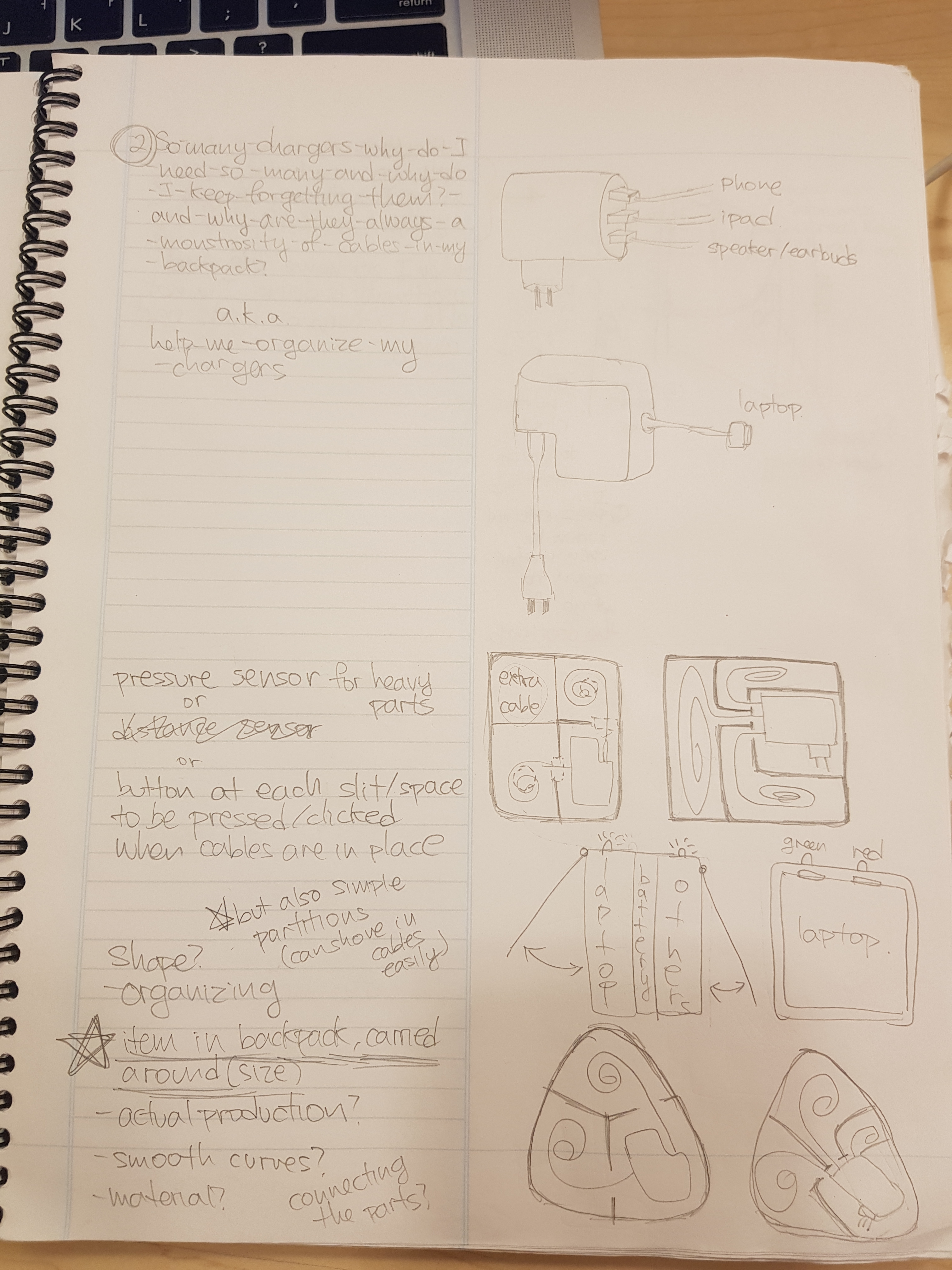
Initial idea for charger organizer box on the bottom right corner
It also involved exploring the RFID tags I could use, which ranged from cards and key fobs to little circular stickers.
But this seemed to be unnecessary and less rational at some point because if I had a box, all I would need to do is open it to check if I have all chargers. Plus, the smallest RFID tag I could find, the sticker, was still too large to put on a wire, which basically had no flat surface. The biggest problem I had to solve was if I always carry my chargers in my backpack, so creating another item that I potentially may forget to throw into my backpack seemed like it was not the ultimate solution. Thus, I had to come up with an alternative which would fundamentally tackle the situation.
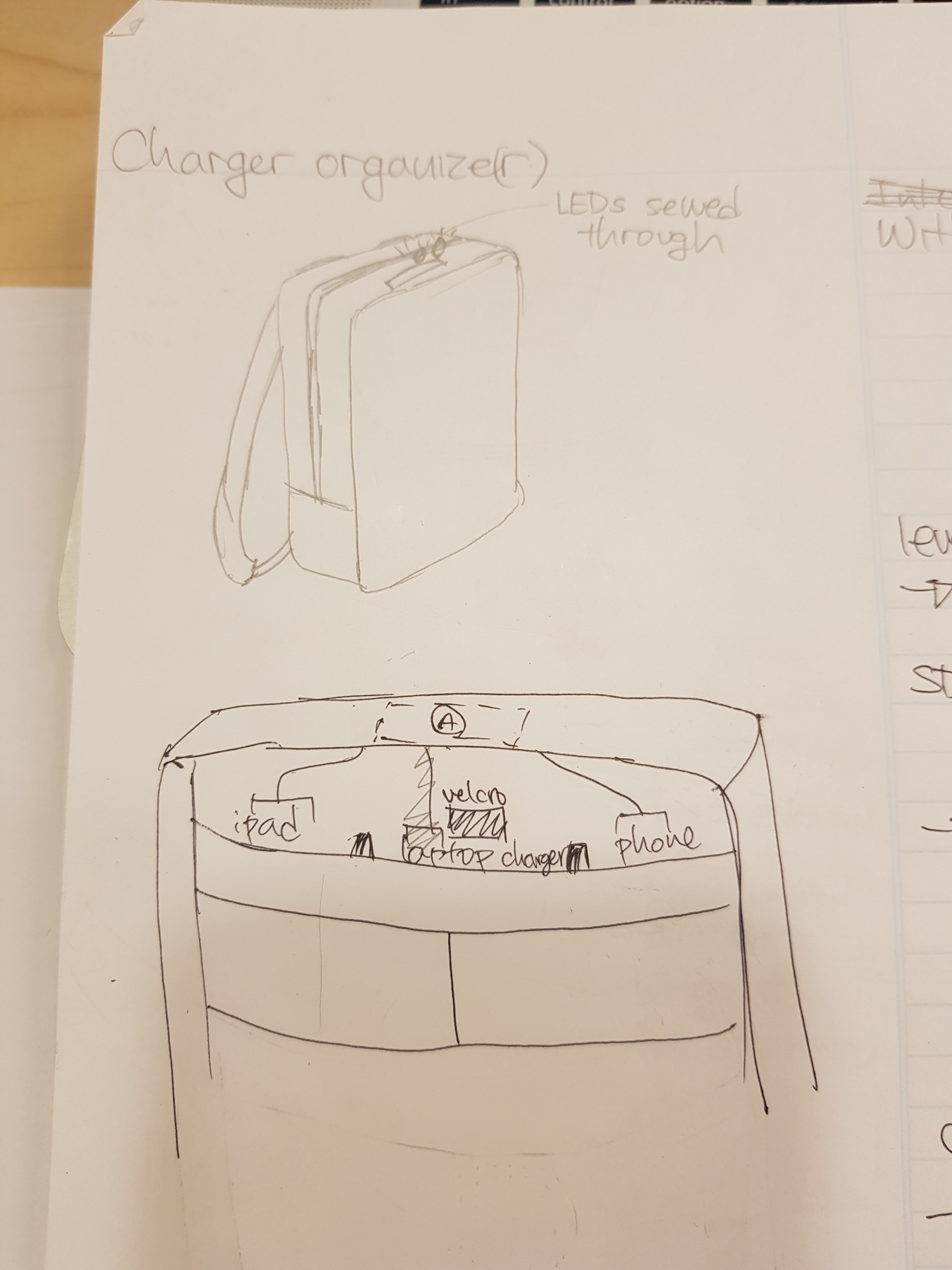
Electronics incorporated into backpack, initial sketch
A new iteration was to incorporate the electronics into my backpack. The core objective was to make my backpack know if it had all of my chargers. So all I had to do was make the RFID readers a part of the backpack and have LEDs poke out so that I can see it easily without opening it. I was able to convince myself that this was plausible because; 1. by pure luck I learned that I could pierce through the fabric of the zippers with the LED; 2. I had pockets in my backpack that I never used but was large just enough that I would have to really force myself to organize the wires before I put them in; and 3. my backpack has a rather stiff box shape which would make it easier to organize and attach the electronics and keep them in place.
For the electronics I went through the process of learning how RFID readers and tags work, learning how to connect two RFID readers to one Arduino Uno, learning what code is used with the RFID readers, and then adding two more readers. I also realized that I may have battery issues if the LEDs were on all the time, so I also added a force sensitive resistor to the bottom of the backpack that would initiate the entire program when the FSR would be pressed by the backpack, which means when it is put down on the ground, and would reset the entire program when there is no pressure on it. In the midst of this, I ran into multiple problems in every facet, which can be summarized to:
- The function dump_byte_array that was used in an example sketch was difficult to decipher and mold into the way I wanted to code to run. I tried multiple ways I could think of but it just simply did not give the output that I wanted it to. This was harder especially when I had two readers. I could already know that if I could not figure it out with two readers, I definitely would not be able to do it with four readers.
- Connecting four RFID readers and two controlled LEDs to one Arduino Uno board was rough. I was basically running out of pins. Even when I shared every pin that could be shared, I was using all of the digital pins with a bunch of wires, which would be difficult to organize in my backpack.
Four RFID readers involved lots of wires and lots of pins.
- Placing four RFID readers in my backpack also became an issue. My backpack has one big pocket and two smaller pockets, so I was planning to divide the big pocket and place one reader in each pocket, but that meant some readers could possibly overlap with each other, which sometimes made the entire electronics stop working.
- I still had the problem of putting a circular sticker on wires which had no big enough surface to put the sticker on.
To resolve this, I decided to stick to two RFID readers and two RFID tags only. One tag was put on my laptop charger and the other was put on an adaptor where I could plug all three of my chargers into one outlet. This simplified the electronics immensely and also solved the problem of placing an RFID tag on each charger.
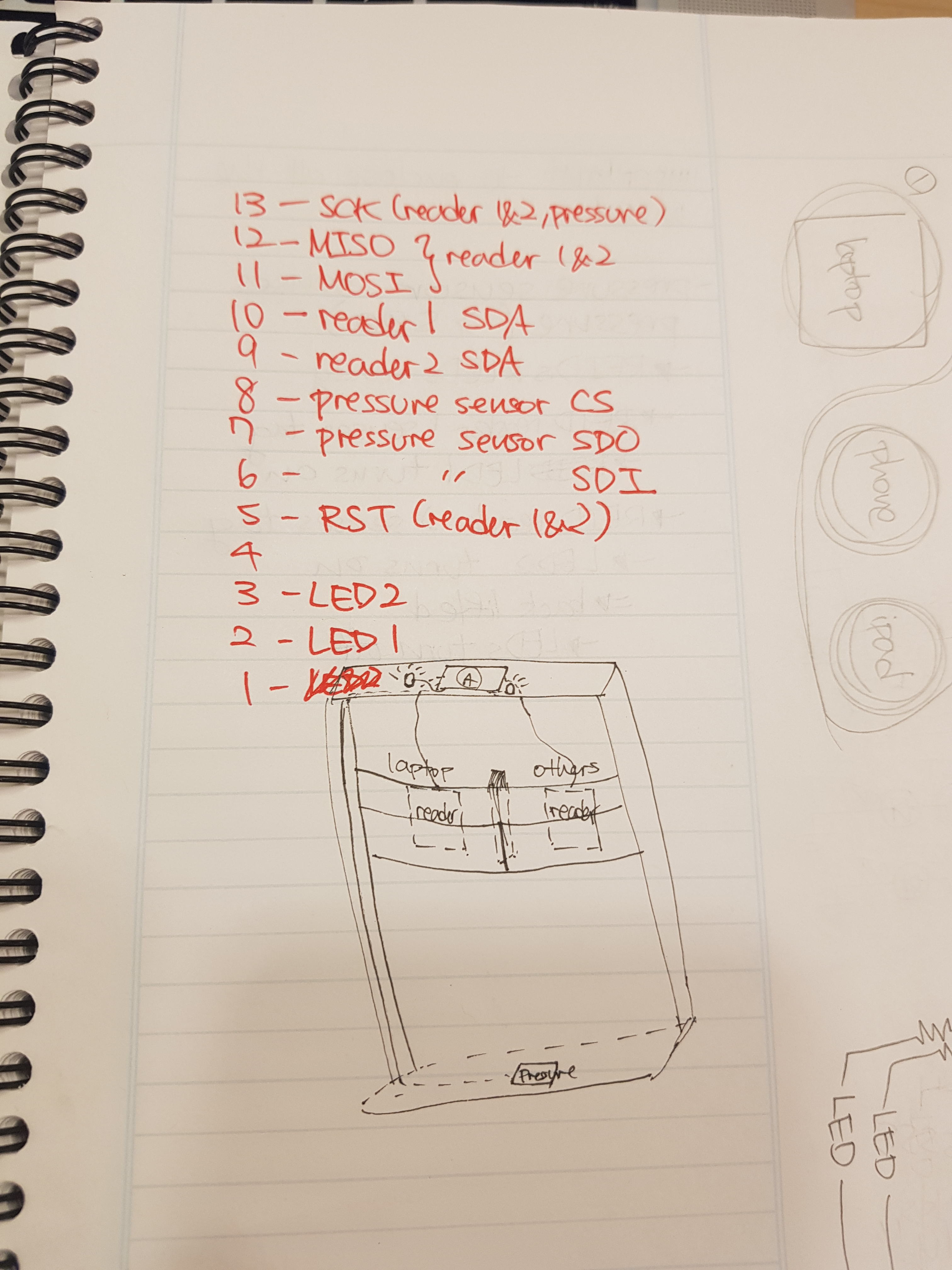
Refined sketch of two RFID readers and a pressure sensor on the bottom
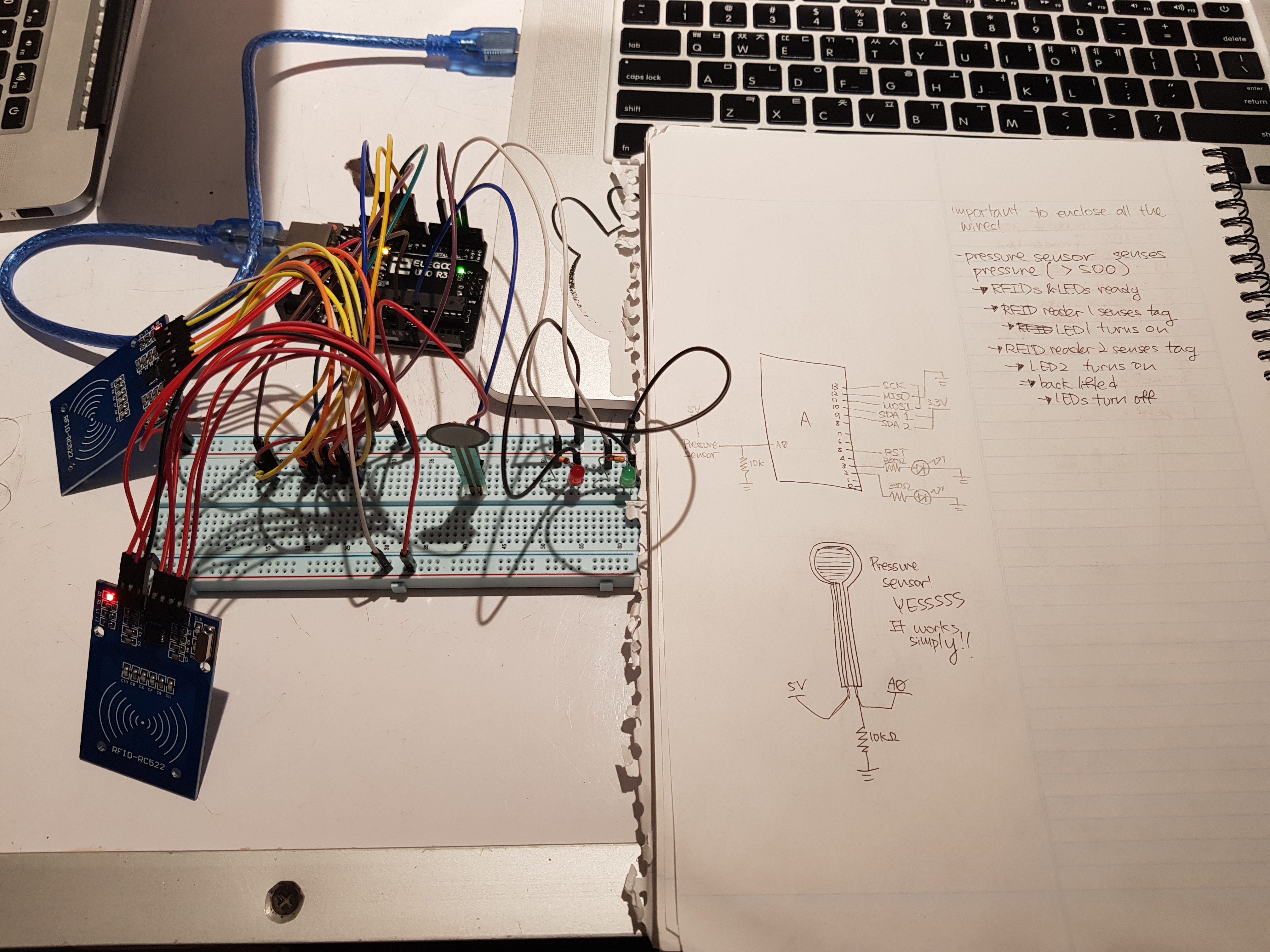
Resolved to two RFID readers with two LEDs and an FSR
Discussion
As for the final product, I am proud of how I incorporated electronics into a pre-existing object but frustrated about the ‘finicky’ nature RFID readers. As I was planning and sketching for the final product, I did expect that I inevitably would have to solder electronics while they are inside my backpack, regardless of planning the wire plot as much as I can.
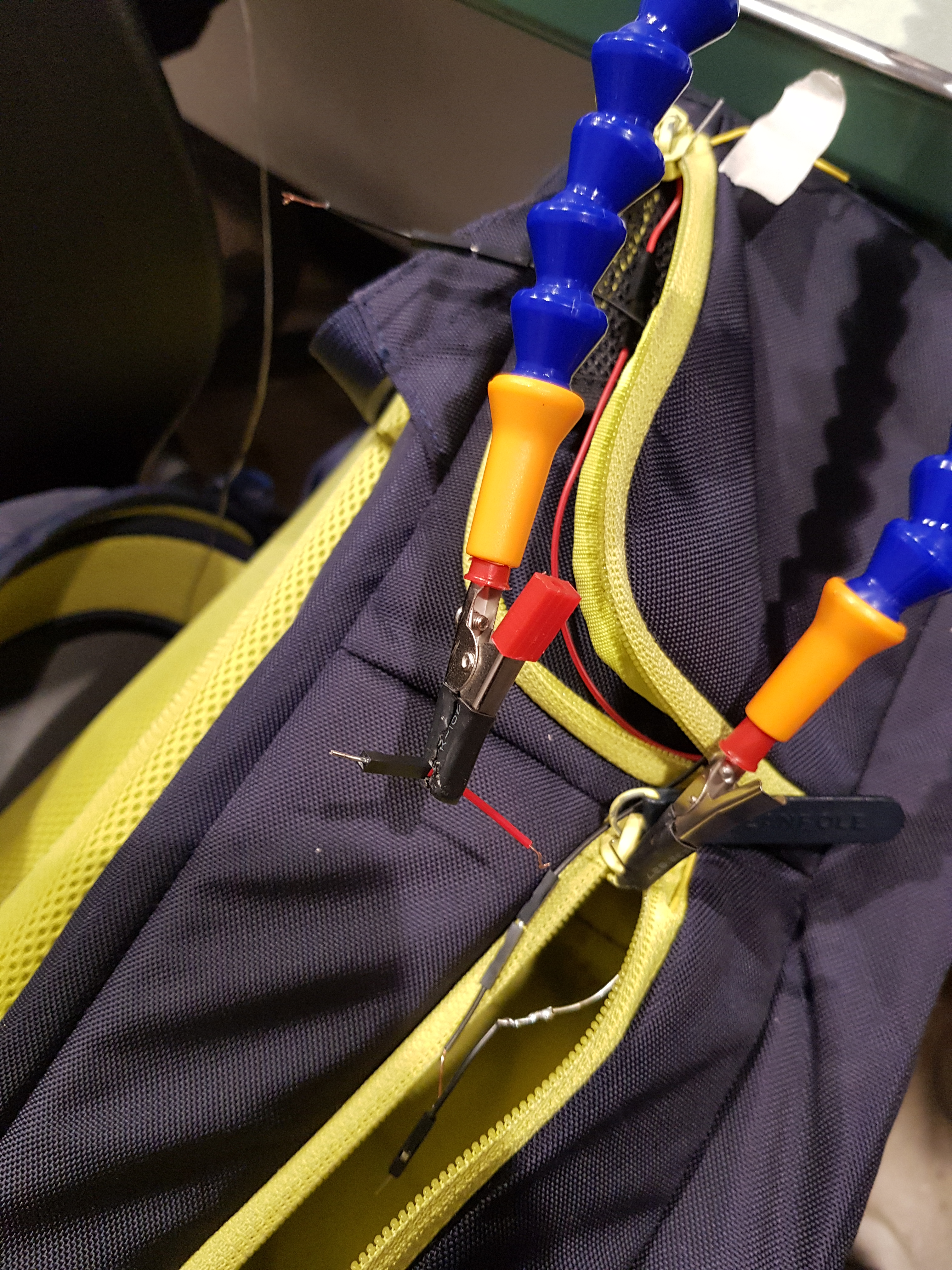
Soldering as wires are piercing through the backpack’s fabric
I definitely would allocate more time into soldering things because it took more time than I expected. I think I focused too much on the code, which was also difficult not to mention. The physical restraints of soldering very close to fabric or soldering in a smaller space were potentially dangerous (and I was very nervous about it too), but in the end I soldered everything into place without causing any hazards or resulting in any unconnected electronics. This success allowed my backpack, the project, to look really natural from the outside, and this specifically brought a lot of positive feedback such as; “Super minimalistic and subtle but still gets the job done!”, “From the outside your backpack looks normal, which is cool too”, and “really cool how you integrated it into the backpack and you can’t see any wires from the outside!” I’m particularly glad and proud that the majority of the feedback I received was about how it was integrated nicely into the object.
There also was a followup feedback about tidying the inside saying “maybe make wires on inside neater?” and “For very far off improvement, it would be cool if it was weather-proof. Since you put a lot of items inside your backpack, I’m worried about durability”, which I totally agree on. This falls into my initial wish of allocating more time into soldering and tidying up the insides. The wires in the current state do get in the way, so I would need to do a better job on sewing the wires more tightly and tucking them away to a corner.
For the frustration with the readers, I want to research more into it and find a way to fix it. The sad part for the critique was that it did not work, and I was tired of it. So, the RFID readers apparently would ‘get tired’ (as I like to call it) after a few runs of reading the tags. It would work perfectly as intended, but all of a sudden it would not respond. And when I ran the example code for using two readers, the serial feedback would say that communication failed and I would need to check the connection. The problem was odd because if I switched out the reader to a different one several times, then it was fine. When the new reader ‘got tired’ and I switched it back to the first reader I was using, it would work perfectly fine again. After I soldered everything the same problem happened, and this time it was much harder to switch out the readers because they were supposed to be tucked away inside the deep pocket. I really want to fix this part and make it work without any weird stops.
Leave a Reply
You must be logged in to post a comment.