A device to monitor writing pressure, and complain if it becomes too large.
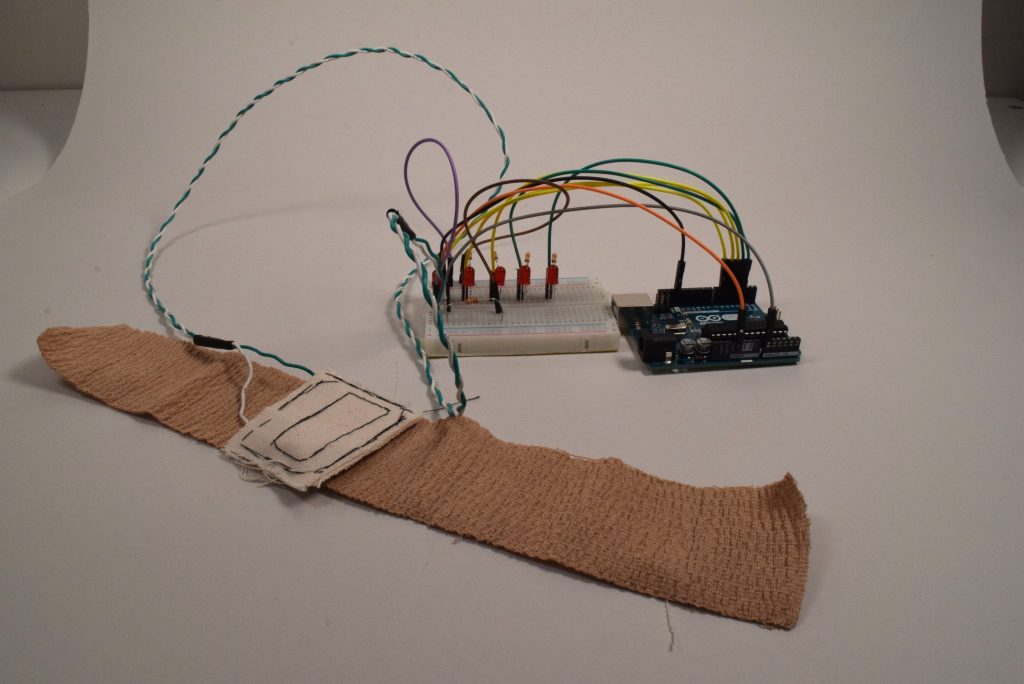
Overall View
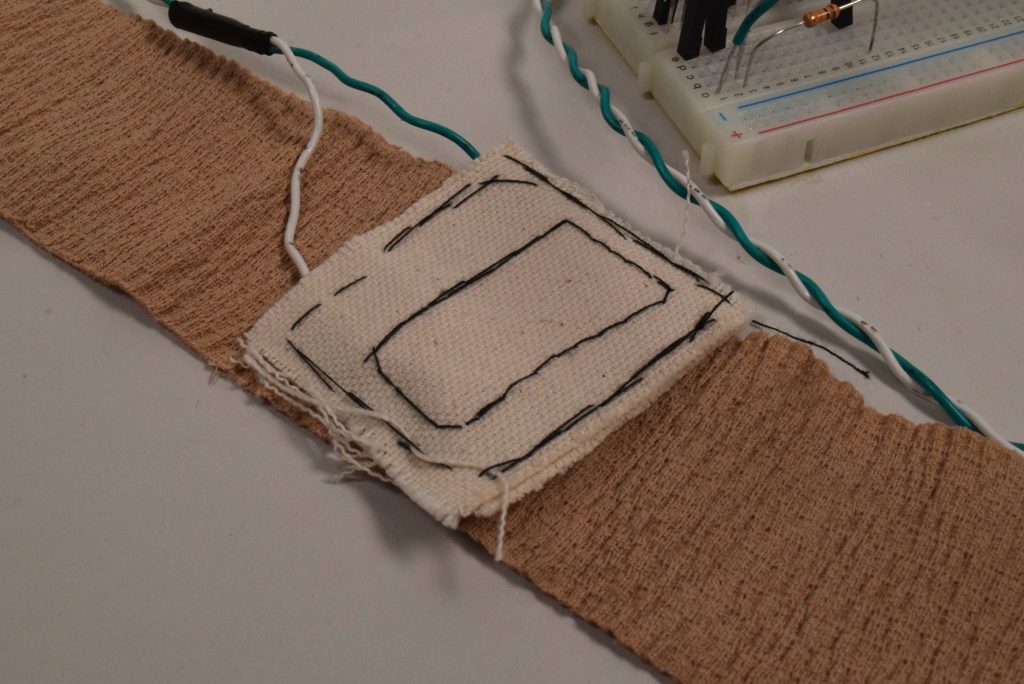
Soft Pressure Sensor
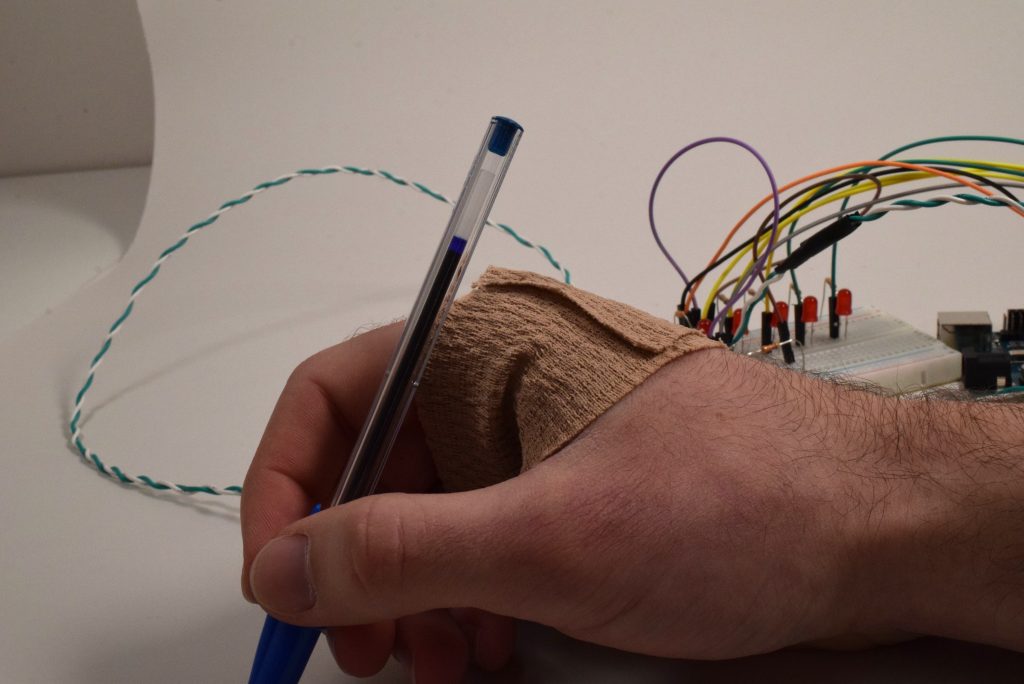
Attached to Hand
Process
Decision Points
I had intended to attach the pressure sensor to a glove, but I needed a glove that was as unobtrusive as possible. As it turns out, when somebody is selling a glove it is generally not for the purpose of attaching sensors, and so it has padding, seams, &c.. Eventually, I fell back to using a strip of roller bandage.
I started off using a buzzer to warn me when I was pressing too hard, but I realized that if I was going to use the device in lecture I couldn’t have a buzzer going of every few seconds, so I switched to LEDs.
Progress Images
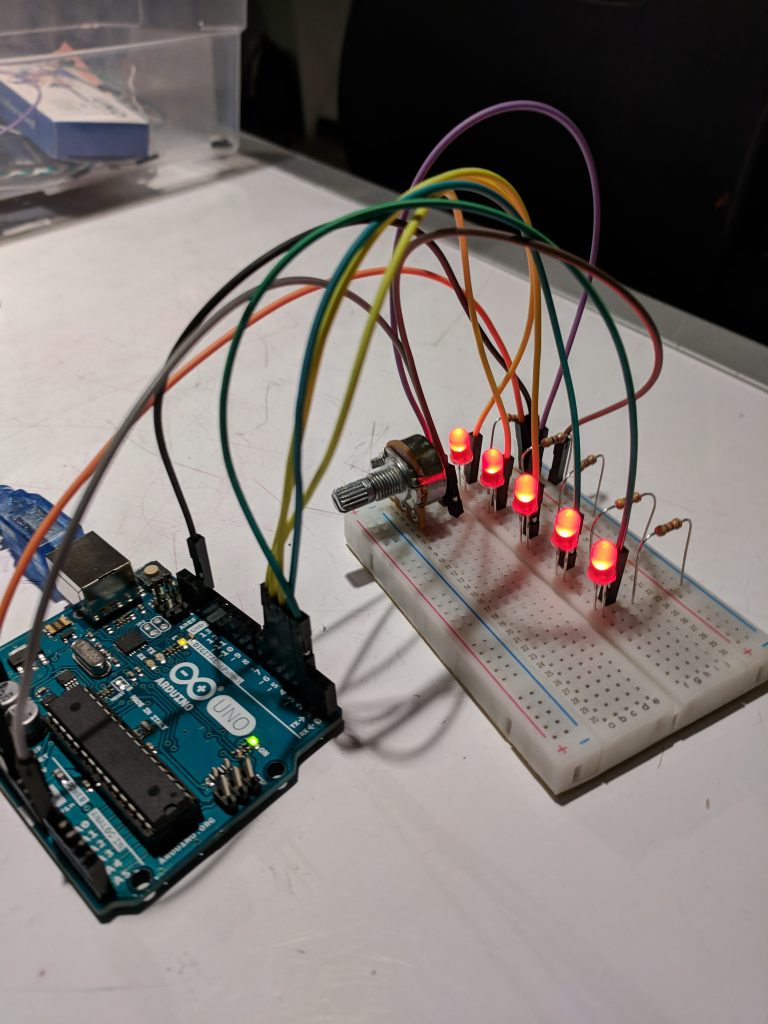
Initial testing of the LED indicator, using a potentiometer in place of the pressure sensor.
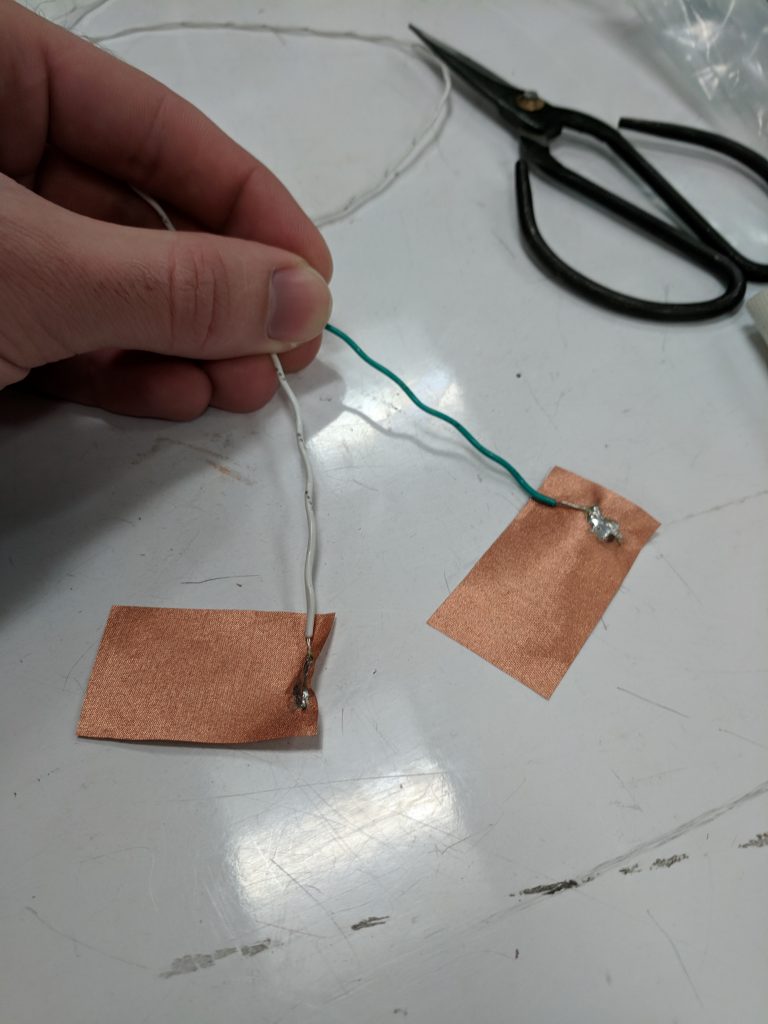
Leads soldered onto conductive fabric. There is a hole from shaking and touching the iron directly to the fabric.
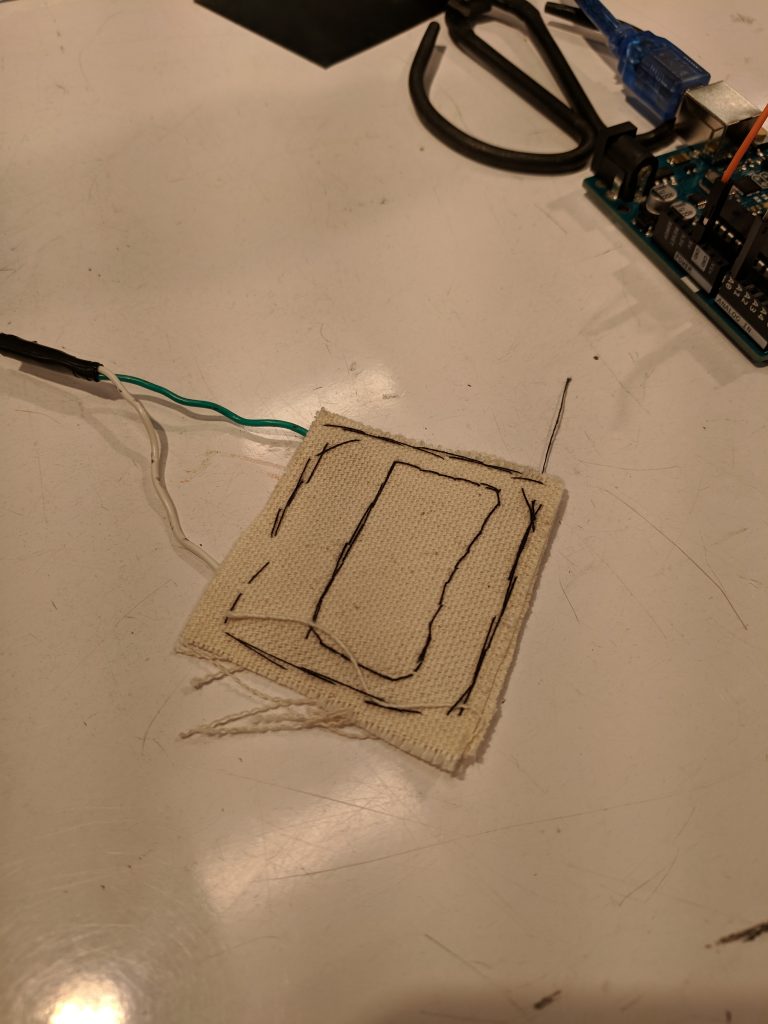
Soft sensor sewn together. The stitching is a mess.
Discussion
Self Critique
The project is a good proof-of-concept to show that the technical aspects are not particularly challenging. The form, however, needs work. The roller bandage is less comfortable than I had hoped, both in that it does not conform to the hand very well, and that it sticks to the paper. Additionally, the sensor is quite thick, and interrupts my natural writing posture. The LEDs are also fairly easy to ignore: some form of touch feedback would work better.
Surprises
I was surprised by the range of resistances provided by the Velostat: I was expecting a range of a few hundred Ohms at most, but it was actually a range of tens of kOhms.
What I Learned
Sewing a functional sensor is easier than I had expected: sewing a beautiful sensor is harder than I had expected. I would want to practice a few times on scrap fabric before trying another sensor, or use a machine.
Next Steps
I would like to try building a second iteration of the device. I would build my own glove with the pressure sensor directly integrated into the side to reduce the fabric overhead that makes the current model uncomfortable. I would also use a vibration motor touching the back of my hand to provide feedback. Additionally, I would re-route the wires to be more comfortable, and place all of the electronics, and a battery, in a pouch on the back of the glove.
Technical Information
Schematic
Code
/* * Copyright 2018, Nathan Serafin. * * This program is free software: you can redistribute it and/or modify * it under the terms of the GNU General Public License as published by * the Free Software Foundation, either version 3 of the License, or * (at your option) any later version. * * This program is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * GNU General Public License for more details. * * You should have received a copy of the GNU General Public License * along with this program. If not, see <http://www.gnu.org/licenses/>. */ constexpr int LED1_PIN = 2; constexpr int LED2_PIN = 3; constexpr int LED3_PIN = 4; constexpr int LED4_PIN = 5; constexpr int LED5_PIN = 6; constexpr int SENSOR_PIN = A0; constexpr int THRESH1 = 650; constexpr int THRESH2 = 670; constexpr int THRESH3 = 690; constexpr int THRESH4 = 710; constexpr int THRESH5 = 730; void setup() { pinMode(LED1_PIN, OUTPUT); pinMode(LED2_PIN, OUTPUT); pinMode(LED3_PIN, OUTPUT); pinMode(LED4_PIN, OUTPUT); pinMode(LED5_PIN, OUTPUT); pinMode(SENSOR_PIN, INPUT); } void loop() { int sensor_val = analogRead(SENSOR_PIN); int led1 = LOW; int led2 = LOW; int led3 = LOW; int led4 = LOW; int led5 = LOW; if (sensor_val >= THRESH1) { led1 = HIGH; } if (sensor_val >= THRESH2) { led2 = HIGH; } if (sensor_val >= THRESH3) { led3 = HIGH; } if (sensor_val >= THRESH4) { led4 = HIGH; } if (sensor_val >= THRESH5) { led5 = HIGH; } digitalWrite(LED1_PIN, led1); digitalWrite(LED2_PIN, led2); digitalWrite(LED3_PIN, led3); digitalWrite(LED4_PIN, led4); digitalWrite(LED5_PIN, led5); }
Leave a Reply
You must be logged in to post a comment.