Overview
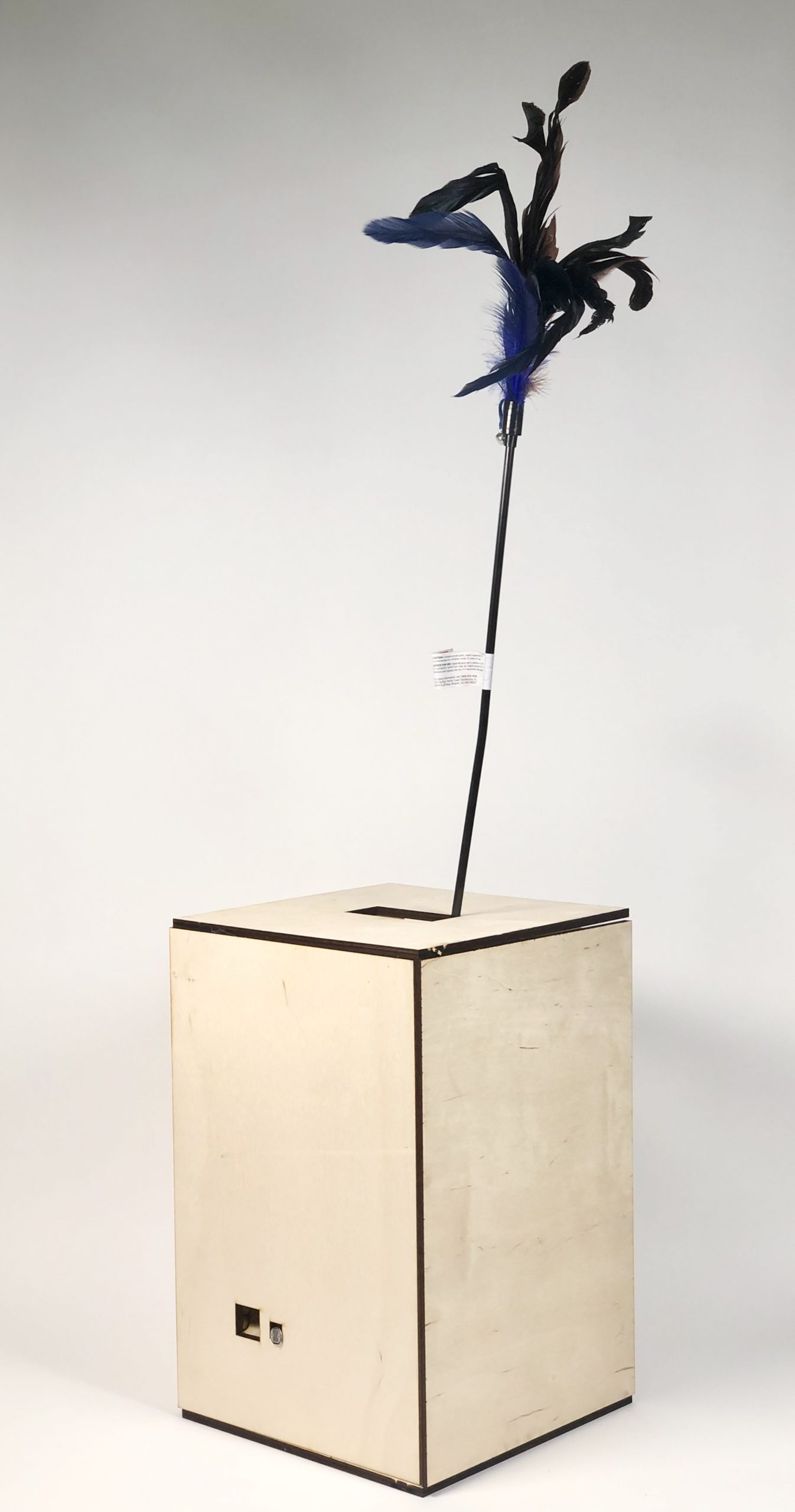
Overview photo.
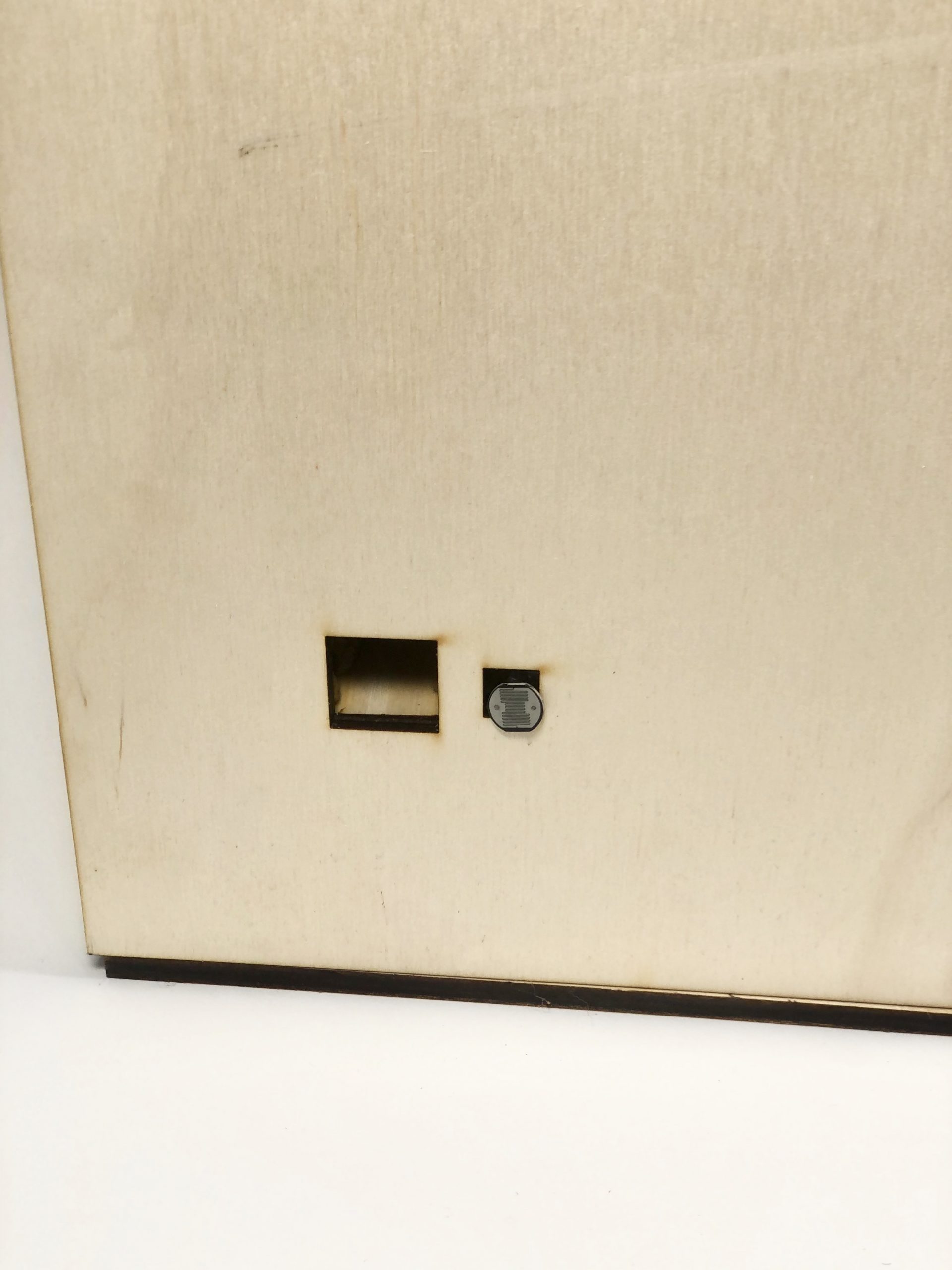
The photocell resistor; senses that the cat is near, then the food is dispensed through the hole.
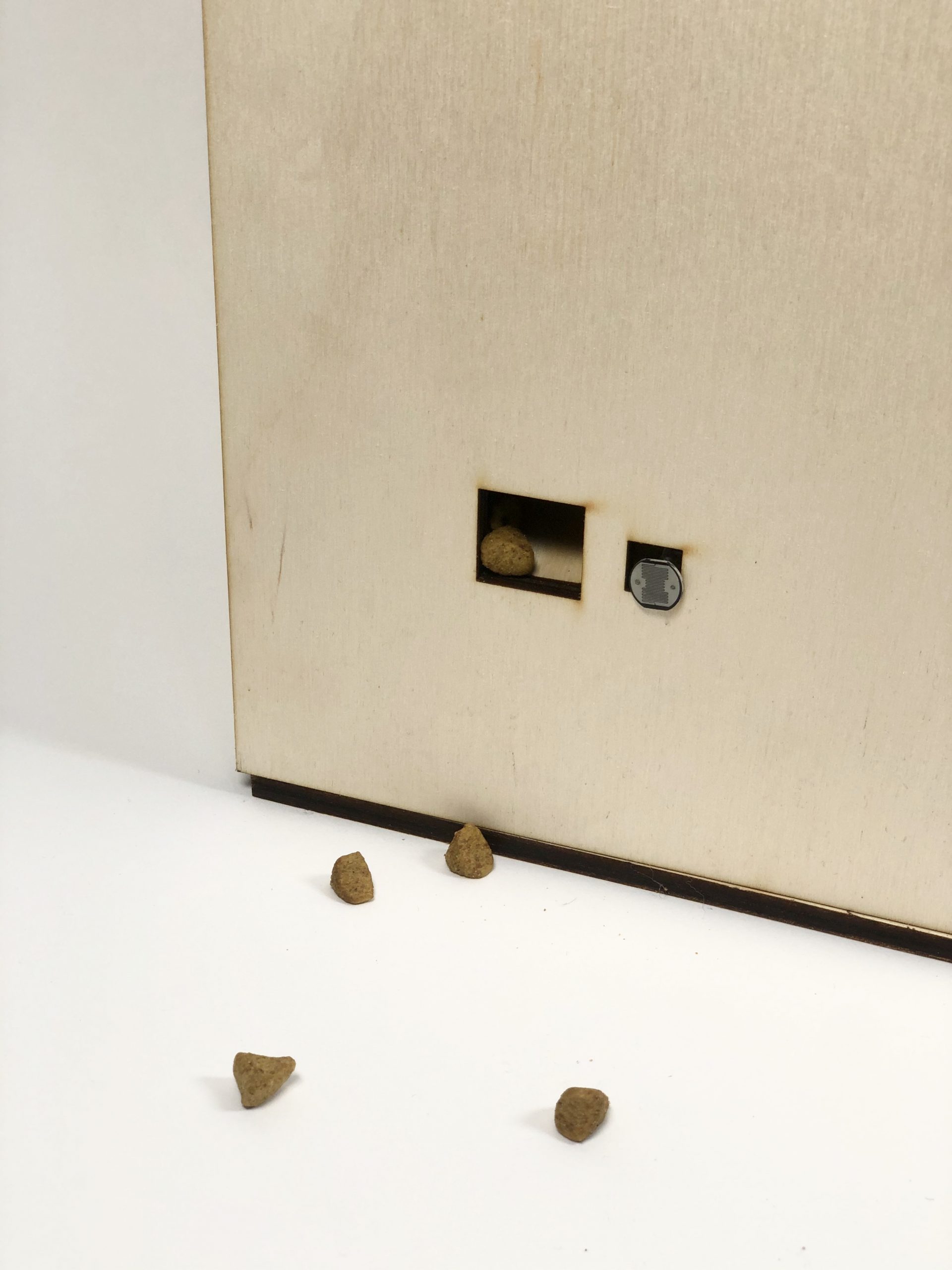
Dispensed cat food.
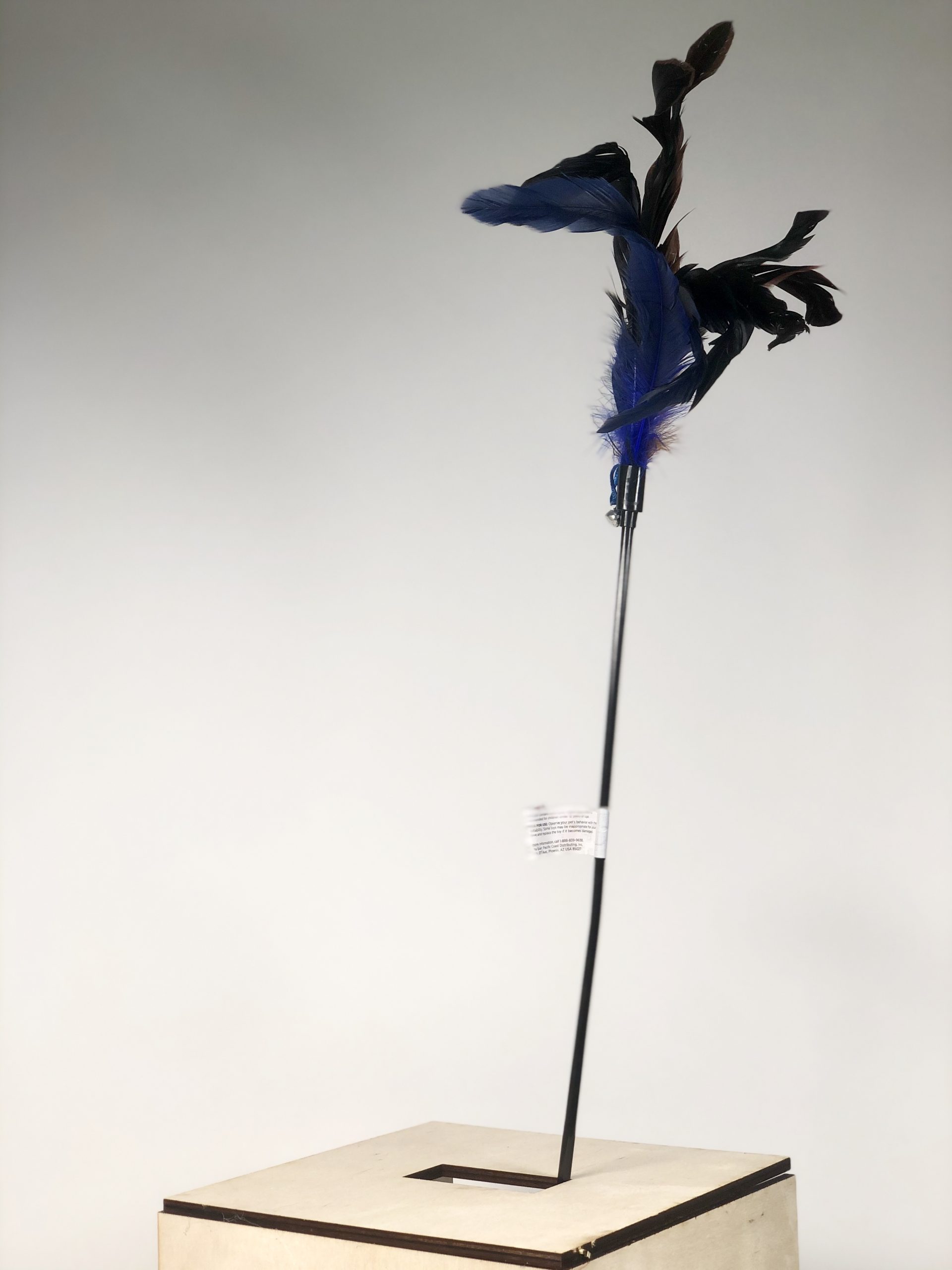
The cat toy; it moves for 30 times when the photocell resistor sends the values.
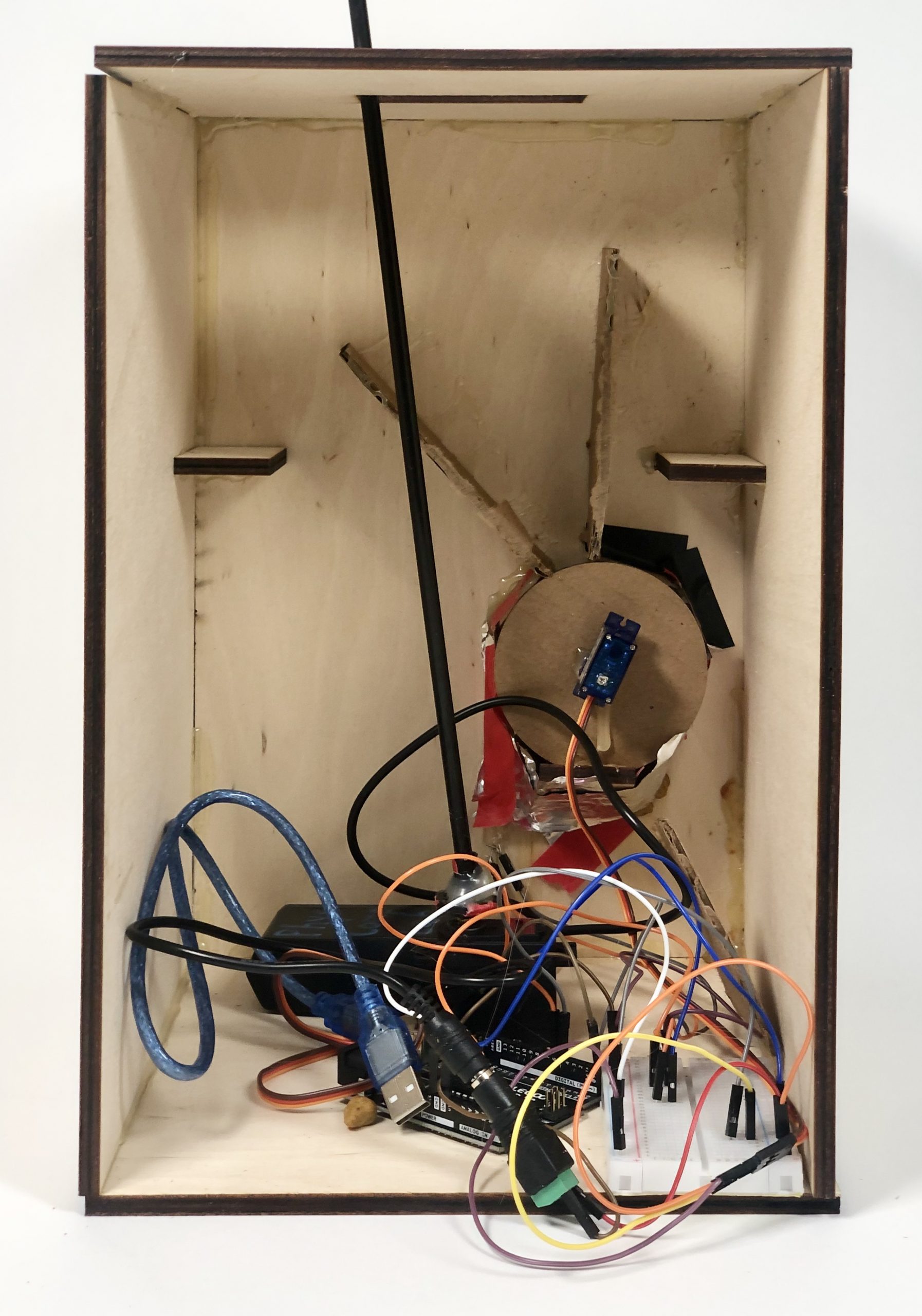
The inside view of it– it is normally blocked with another wood panel to hide it.
Process images and review
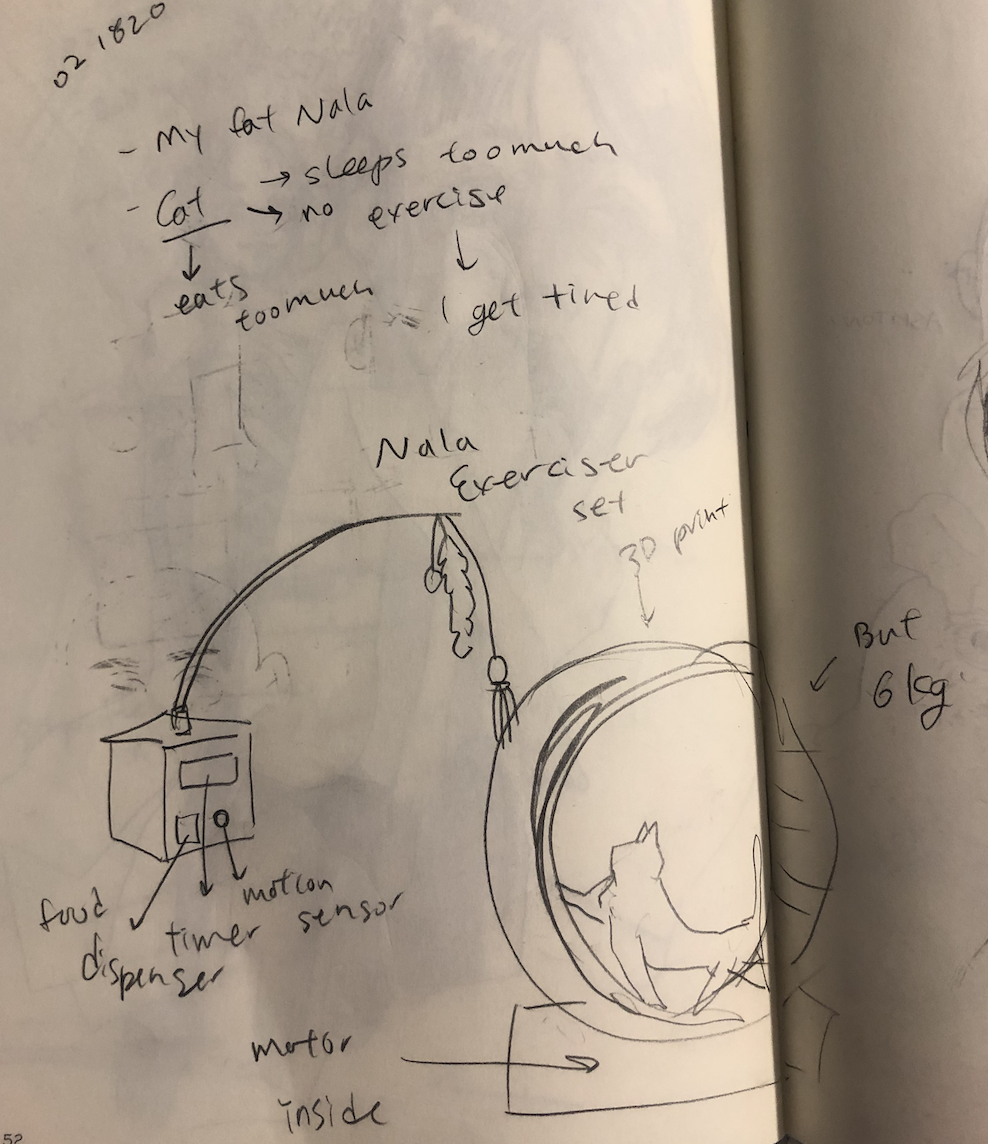
A sketch of the original idea. The main challenge was how sturdy it had to be and the food dispensing chamber.
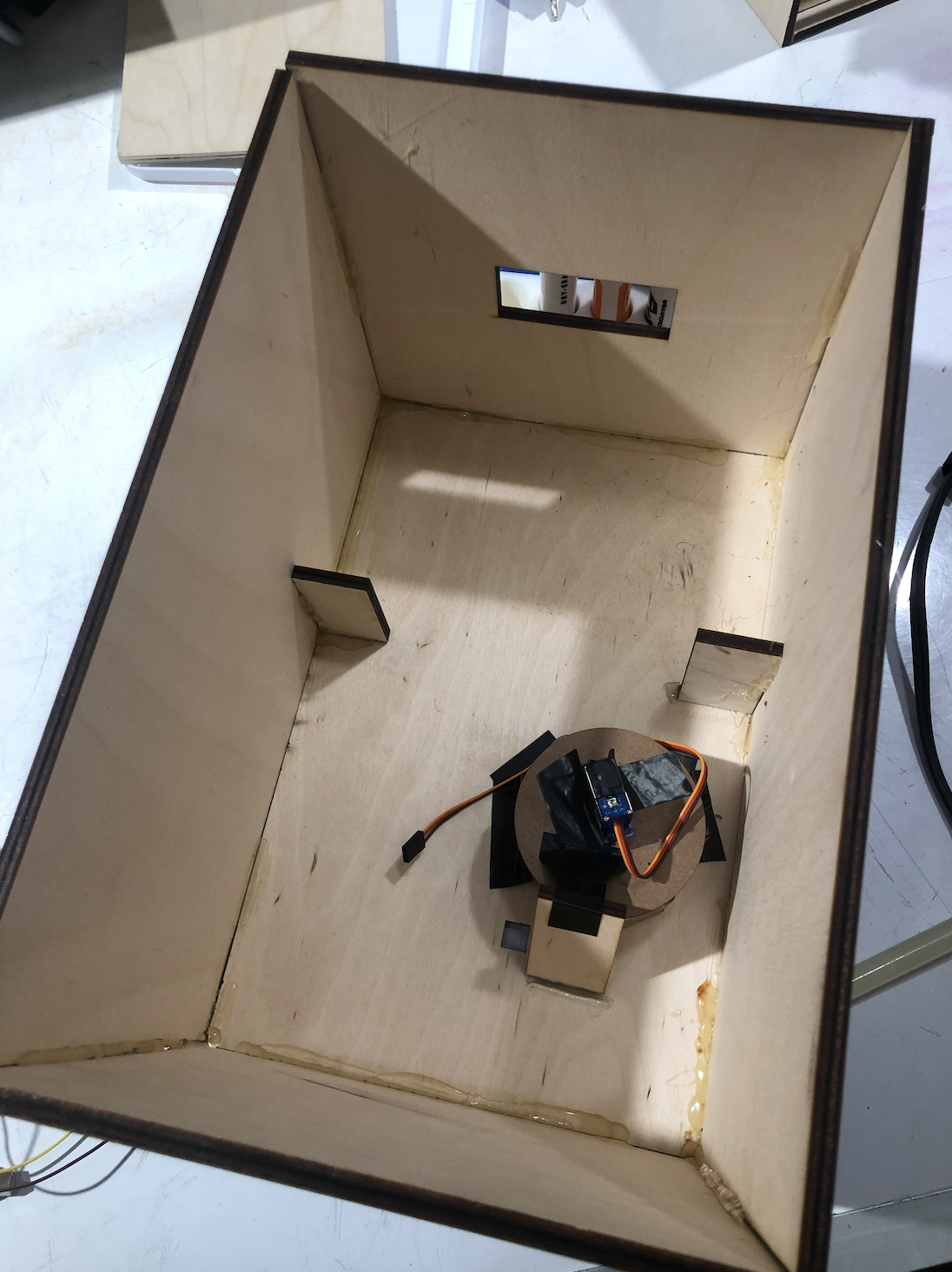
The turning chamber of the food dispenser. The box was originally made in cardboard, yet thought it would be sturdier with wood panels. Also, scratch-proof!
The challenge was with the food dispenser. The servo motor’s knob was very shallow, therefore it was keep getting detached from the chamber from the inside. Moreover, the size of the cat food mattered. If it was too small, it would get jammed inside. Therefore, it took me a while to plan dimensions and technicalities over and over again.
Prototype of the chamber working. First practiced dispensing with fruit loop cereals.
Food Dispenser test code:
#include <Servo.h> //dispenses food in every set time interval const int FOODPIN = 5; Servo foodMotor; unsigned long taskTimer = 0; unsigned long INTERVAL = 5000; //18000000; void setup() { foodMotor.attach(FOODPIN); Serial.begin(9600); } void loop() { // if (millis() - taskTimer >= INTERVAL) { //do the scheduled task foodMotor.write(100); // taskTimer = millis(); // } }
Cat Toy test code:
#include <Servo.h> //moves cat toy const int CATPIN = 6; Servo catMotor; void setup() { catMotor.attach(CATPIN); } void loop() { catMotor.write(0); delay(500); catMotor.write(180); delay(500); }
Photocell Resistor test code:
//prints out the values of photocell resistor to test the range of light and dark const int PHOTOPIN = A3; void setup() { pinMode(PHOTOPIN, INPUT); Serial.begin(9600); } void loop() { Serial.println(analogRead(PHOTOPIN)); }
Discussion
Response:
“ Loud noise is scary to a cat – are there softer motors that you can use?“
“I will love to see this in action with a cat. “
“I like how the project considered the cat’s needs quite well — rather than just making the cat toy automated, I enjoyed how the cat would be attracted to the toy through a treat dispenser as well. Fun and interesting concept. “
“I love the box design, very compact.“
In this project, I learned a lot about not only coding and wiring, but also with presenting my work. The design matters, and understanding the needs of a cat and purpose of the device was the main challenge. I was very happy that I got positive responses from our classmates. I was glad that many have noticed my design of the box. It was a last-minute change in material from cardboard to wood, yet I do not regret that I made it scratch-proof, because during the action of this device, the cat will definitely try to scratch the box. However, with this purpose, someone has mentioned about putting a scratch post next to the device, and make a whole set. I do agree. It would be nice to see how a cat sharpens his claw on the post then plays with the device. Also, the other concern that was mentioned was the sound of the servo motor that the cat toy was attached to. It was making a very loud noise, and I totally forgot about how cats get scared of loud motor noises. I have taken that comment and the other comment about actually testing it with the cat, and I have tried:
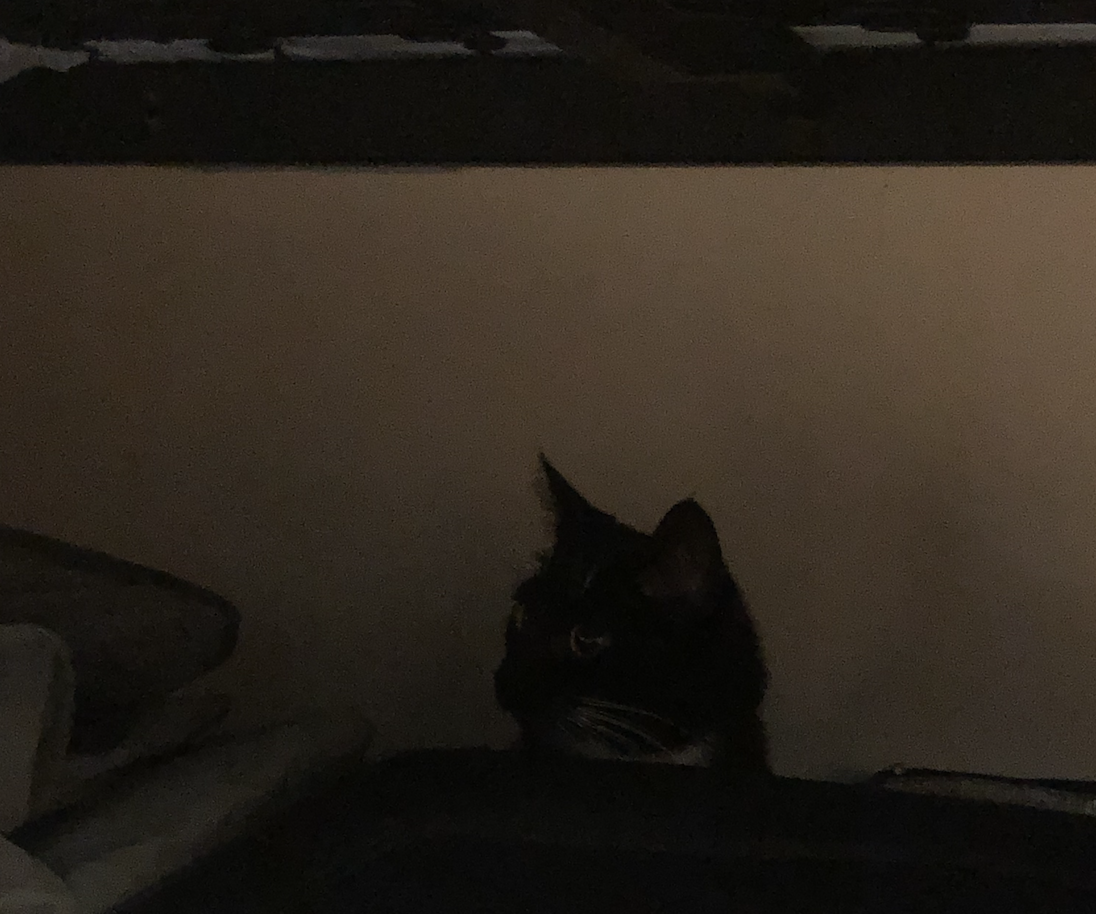
The cat was scared by the sound of the servo motor, so he hid under my bed.
The cat was interested in his food and everything yet when as soon as the motor started to move, the cat got scared by the noise and hid under my bed. The other challenge was the photocell resistor. It did function, yet I wish I had more time and replaced it with an infrared sensor. Then it would have been more accurate, and easier to deal with moving animals. The time was the biggest constraint of this project. I wanted to score designs on to the box, or paint it– some sort of surfacing to the project. Also, I really wanted to use a nail gun to put the panels together to make it look clean, yet I was scared that the nail would poke through the panels since it is quite thinner than half-inch revolution plywood that I normally use. I am not completely satisfied with this project, yet I think it was a good opportunity to broaden up my spectrum in bringing my idea to life. I think this project can go in many directions. It could be used solely as a food dispenser, or automatic cat toy, or even attaching more functions to it so it can activate multiple toys.
Technical Information
Schematic:
Code submission:
/* Title: The Catxerciser by Chloe Cho Purpose: The millis function sends how many milliseconds have passed since the arduino board was on, and when the value of milliseconds equals to the INTERVAL, it moves the foodMotor which is attached to the food chamber to dispense food. Then the photocell resistor senses the light value when cat is infront of it, then the catMotor moves the cat toy. Something to consider: unsigned long is better than other variable types to store long time interval values. */ #include <Servo.h> //sets up pines for each input and outputs const int FOODPIN = 5; const int CATPIN = 6; const int PHOTOPIN = A3; Servo catMotor; Servo foodMotor; unsigned long taskTimer = 0; //time interval for the food dispenser. I set it up for 10 seconds(10000 milliseconds) just to show, yet one can change the value to whatever they want. unsigned long INTERVAL = 10000; void setup() { catMotor.attach(CATPIN); foodMotor.attach(FOODPIN); pinMode(PHOTOPIN, INPUT); Serial.begin(9600); } void loop() { if (millis() - taskTimer >= INTERVAL) { //compares the arduino's up-time with the INTERVAL value. If it is equal, it does the task below. //the food dispenser starts to turn. foodMotor.write(90); foodMotor.write(130); foodMotor.write(90); //the photocell resistor starts to compare values to 800, which is the range I got from range testing. 800 is usually when there is light out. if (analogRead(PHOTOPIN) < 800) { for (int i = 0; i < 30; i++) { //the cat toy starts to turn when there is low light value. catMotor.write(0); delay(500); catMotor.write(180); delay(500); } } //resets. taskTimer = millis(); } }
Comments are closed.