Project 2: A Personal Assistive Device for Caleb
The Hot Grog-inator is a personal assistive device that helps me keep track of tea-steeping and prevent mouth-burning.
Demo –
Please note that the device is in demo mode right now, meaning it’s timer functionality is set to activate at 3 seconds and it’s temperature functionality is set to activate when it reaches above 50 degrees Celcius. In practice, the Grog-inator’s timer buzzer will be set to go off at 3 minutes, and the temperature buzzer will go off when reaching below 65 degrees Celcius.
Details –
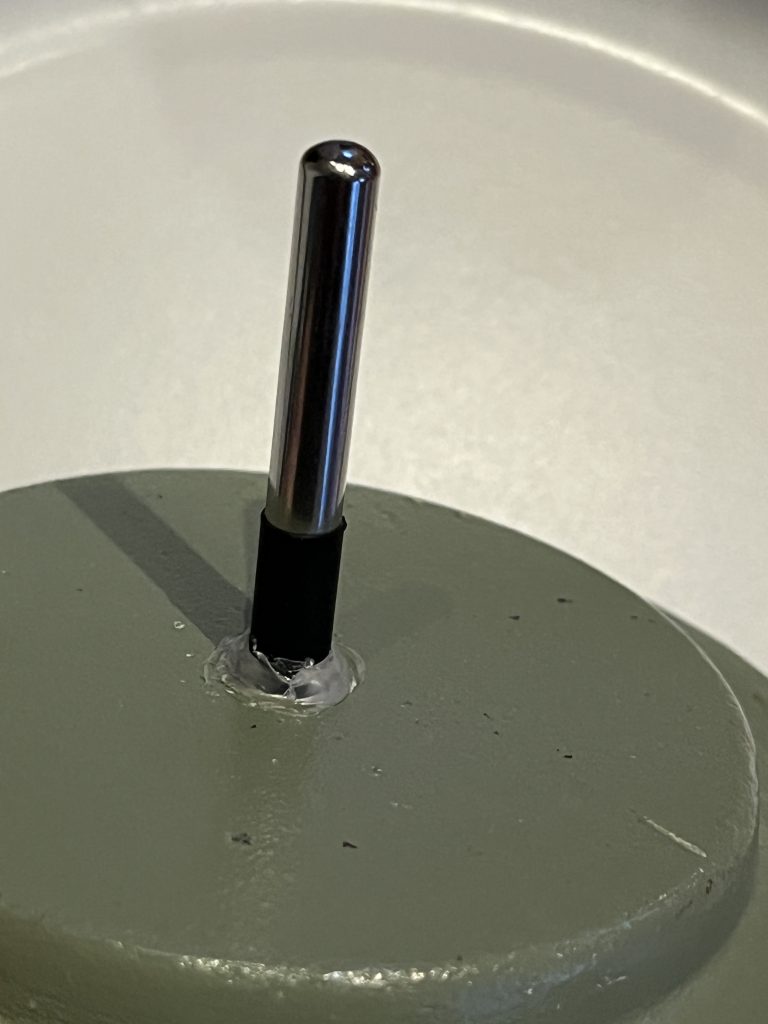
Temperature probe on the underside of the Grog-inator
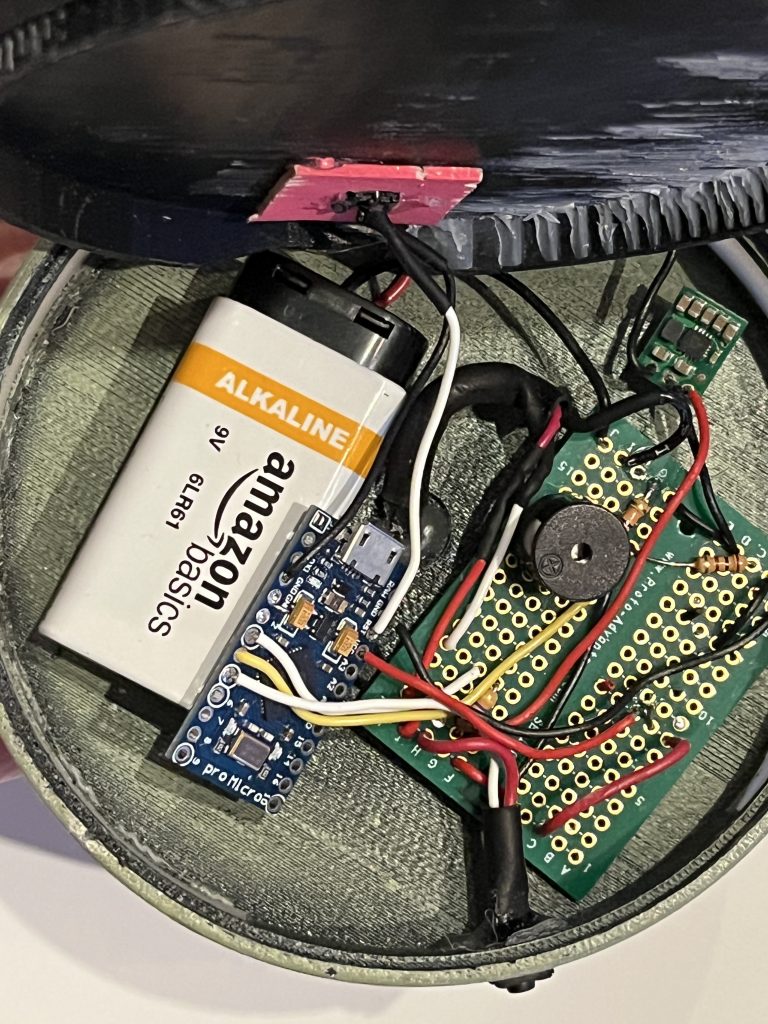
Interior of the Grog-inator
Process –
Initial ideation of the hot-groginator. I was inspired by burning my mouth on tea one too many times, and wanted to make a device that could prevent that from happening.
Decision 1:
Redo the soldering. Due to the micro requiring soldering, I had to solder everything to prototype. I ended up restarting because the wires got too hard to work with. It was a good learning experience on when to give up and when to try to prototype with the least permanent medium as possible.
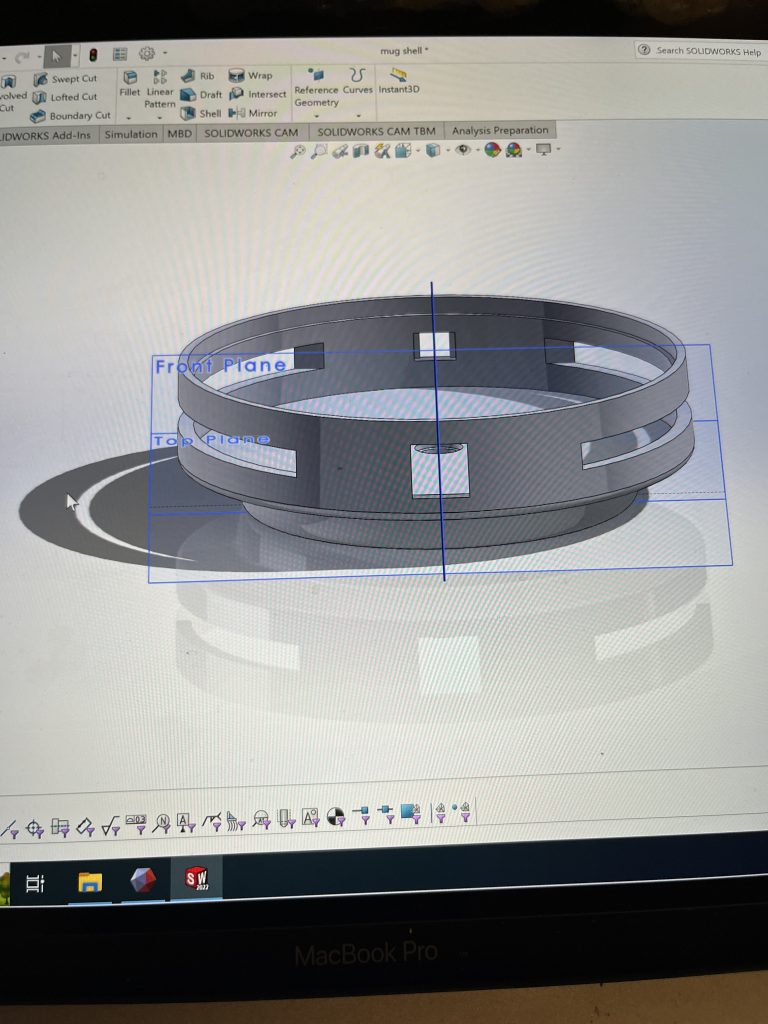
CAD Modeling process
Decision 2:
Give up on the lighting. I made lighting windows that would be connected to the power to indicate a clear on and off state, but they didn’t work well with all the electrical components inside. Additionally, the second light would be much dimmer than the first. I thought it would be a cool decoration, but couldn’t justify it with the issues I was having, so I ended up scrapping the idea. It was a good lesson on knowing the limits of time and electrical capability.
Feedback –
Can’t wait to see it all come together a little scared of condensation accumulating on electronics, but I think your casing will protect it.
I definitely considered how the electronics are going to be close to hot water. It’s something that’s hard to contend with given the limitations of my fabrication skills, but I believe that by limiting the use of the lid to just a short part of the life cycle of a hot drink, and enclosing the electronics in a sealed case that will be finished with water-proof material should suffice. Ideally, of course, it would be created using cast plastic if actually put into production.
What are the housing considerations for the electronics? Given the scale of the drawings, it seems like it would be hard to fit everything.
I’m going to use an Arduino Micro and a 9-volt battery for the brains and power of the grog-inator, and that should limit the footprint of the electronic components significantly. While designing the housing, I just need to make sure to accommodate for everything.
Self Critique –
I’m pretty satisfied with the Hot Grog-inator. I think that given the scope and timeline of the project, the fidelity that I got the device to felt appropriate. It’s self-sufficient and doesn’t require being plugged into the computer, and is programmed fairly robustly, being able to be reset with a button. Overall, though, this feels like a proof-of-concept prototype that I’m very happy with.
Learning –
I learned a lot about coding through this project. I think this project was relatively simple electronically all things considered. However, coding the right behavior and making it reliable and robust when not having the ability to access or reset the Arduino was a significant challenge. I wanted to make the device reset automatically when it was powered on, and when that didn’t work I tried to have it reset every 30 minutes. When that didn’t work, I ended up just adding a button to trigger a reset.
Another thing I learned was to not get ahead of myself with fabrication. I ended up having to reprint the lid twice due to being impatient and not measuring things correctly, and still having very little room to work with. Seeing as this was my first time 3D printing, it’s kind of to be expected, but should still be a more thought-out step next time.
Next Steps –
I think if I had more time I would try to make the interior more neat, in that I would try to use a smaller protoboard and divide the wires up so that they weren’t as confusing as before. I would also make the housing more fit to different cups, with a more dramatic cone-line bottom. Currently the grog-inator doesn’t fit all of my cups and that significantly decreases usability. I’d also try to create more exact housing that secures the protoboards and batteries into place, so that everything isn’t just clunking around in the device.
Finally, I would also not paint over the entire device. I like the color I chose, but it’s definitely not something that helped the final fidelity and the imperfect paint job bothers me endlessly.
Block Diagram –
Schematic Diagram –
Code –
//Hot Grog-inator Code //Caleb Sun //Code activates buzzer to beep 3 times 3 minutes after button is pressed to indicate tea-doneness, and 5 times after temperature sensor detects that drink is at safe drinking temperature (65 degrees C) #include <OneWire.h> #include <DallasTemperature.h> #define tempPin 3 #define buzzerPin 4 #define buttonPin 5 OneWire oneWire(tempPin); DallasTemperature sensors(&oneWire); int teaTemp = 0; int buttonState = 0; int buzzCounter3 = 0; int buzzCounter5 = 0; const int threeMin = 180000; const int BuzzDelay = 300; unsigned long buzzerTimer = 0; unsigned long threeMinTimer = 0; bool buzzerState = LOW; void setup(){ pinMode(tempPin,INPUT); pinMode(buzzerPin,OUTPUT); pinMode(buttonPin,INPUT); sensors.begin(); // Start up the library Serial.begin(9600); } void loop() { if (millis() == 10000){ buzzCounter5 = 0; } sensors.requestTemperatures(); teaTemp = sensors.getTempCByIndex(0); if (teaTemp < 65){ if (((millis() - buzzerTimer) >= BuzzDelay) && (buzzCounter5 < 5)){ buzzerState = !buzzerState; buzzerTimer = millis(); buzzCounter5 = buzzCounter5 +1; } else { buzzerState = LOW; } digitalWrite(buzzerPin,buzzerState); } buttonState = digitalRead(buttonPin); if (buttonState == HIGH) { threeMinTimer = millis(); buzzCounter3 = 0; } if (((millis() - threeMinTimer) > threeMin) && (threeMinTimer != 0)) { if (((millis() - buzzerTimer) >= BuzzDelay) && (buzzCounter3 < 3)) { buzzerState = !buzzerState; buzzerTimer = millis(); buzzCounter3 = buzzCounter3 + 1; } else { buzzerState = 0; } digitalWrite(buzzerPin,buzzerState); } }