AMOR FATI
By Cassie Rausch
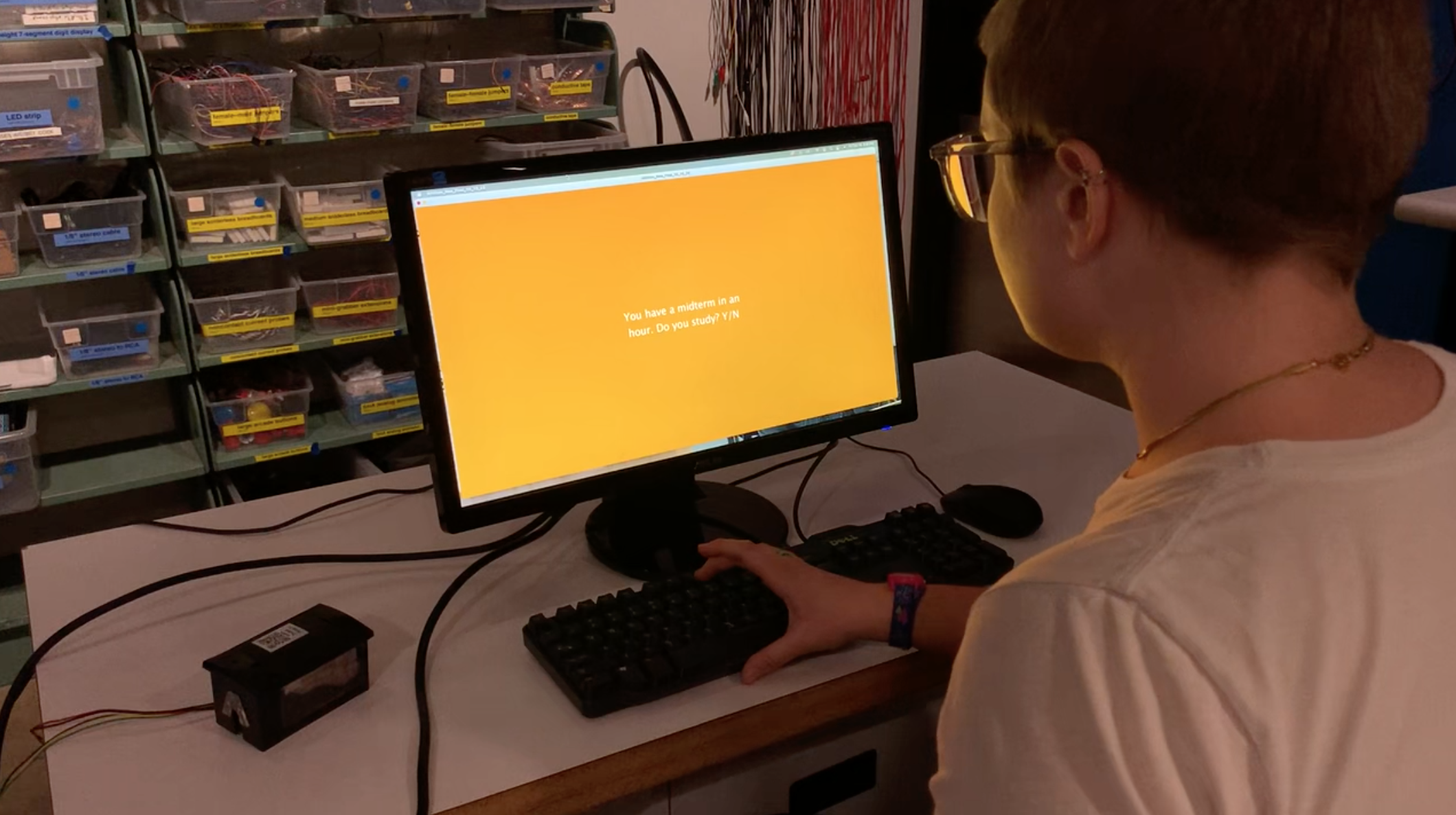
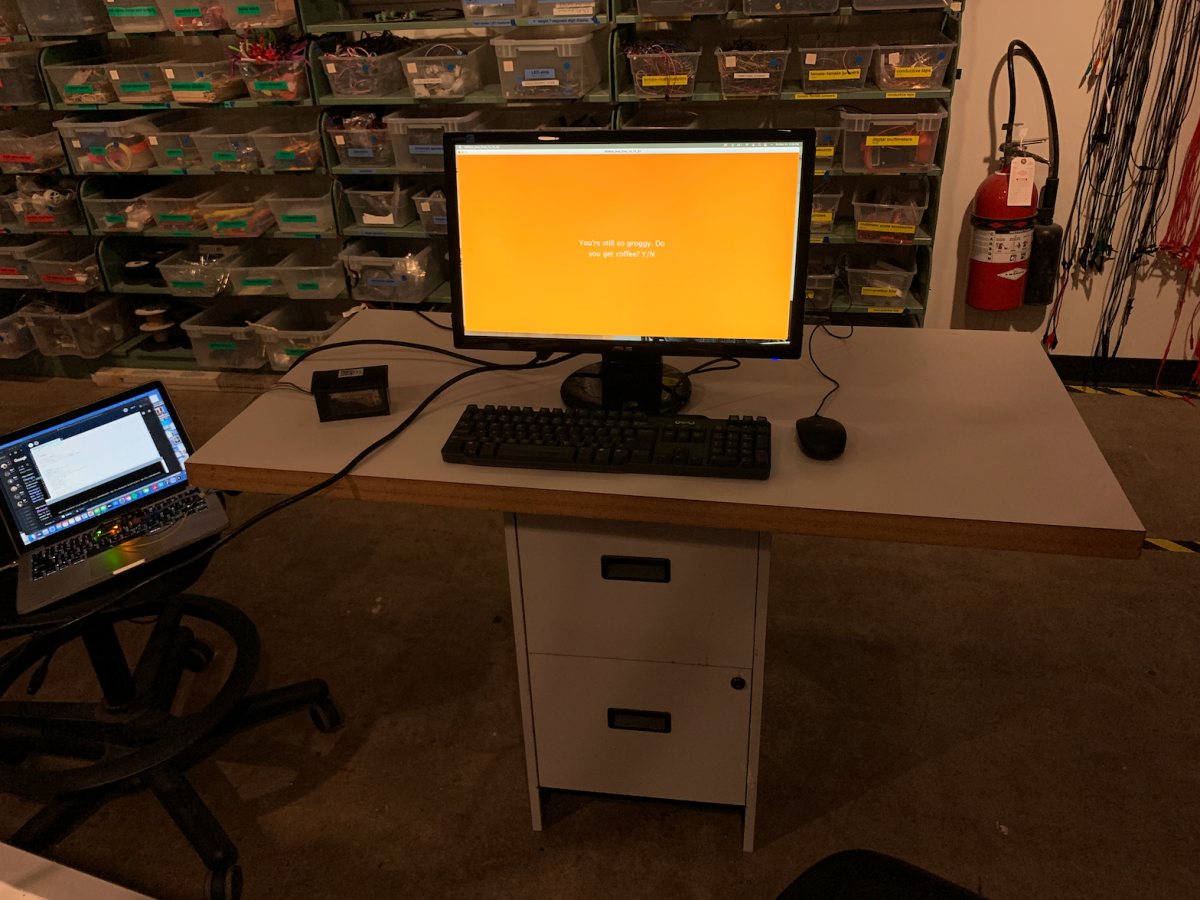
(click images above for videos)
NARRATIVE DESCRIPTION:
This desktop philosophical experience is intended to be placed discretely somewhere in CMU with high foot traffic.
After sitting down, you answer each question as it appears on the screen. The ‘y’ key means yes and the ‘n key means no. All of the questions are modeled after decisions that a CMU student might make on a normal day, some a bit more dramatic than others. After you answer all of the questions, you will get a receipt printed with some of the positive and negative consequences of the choices you made.
PROGRESS IMAGES:
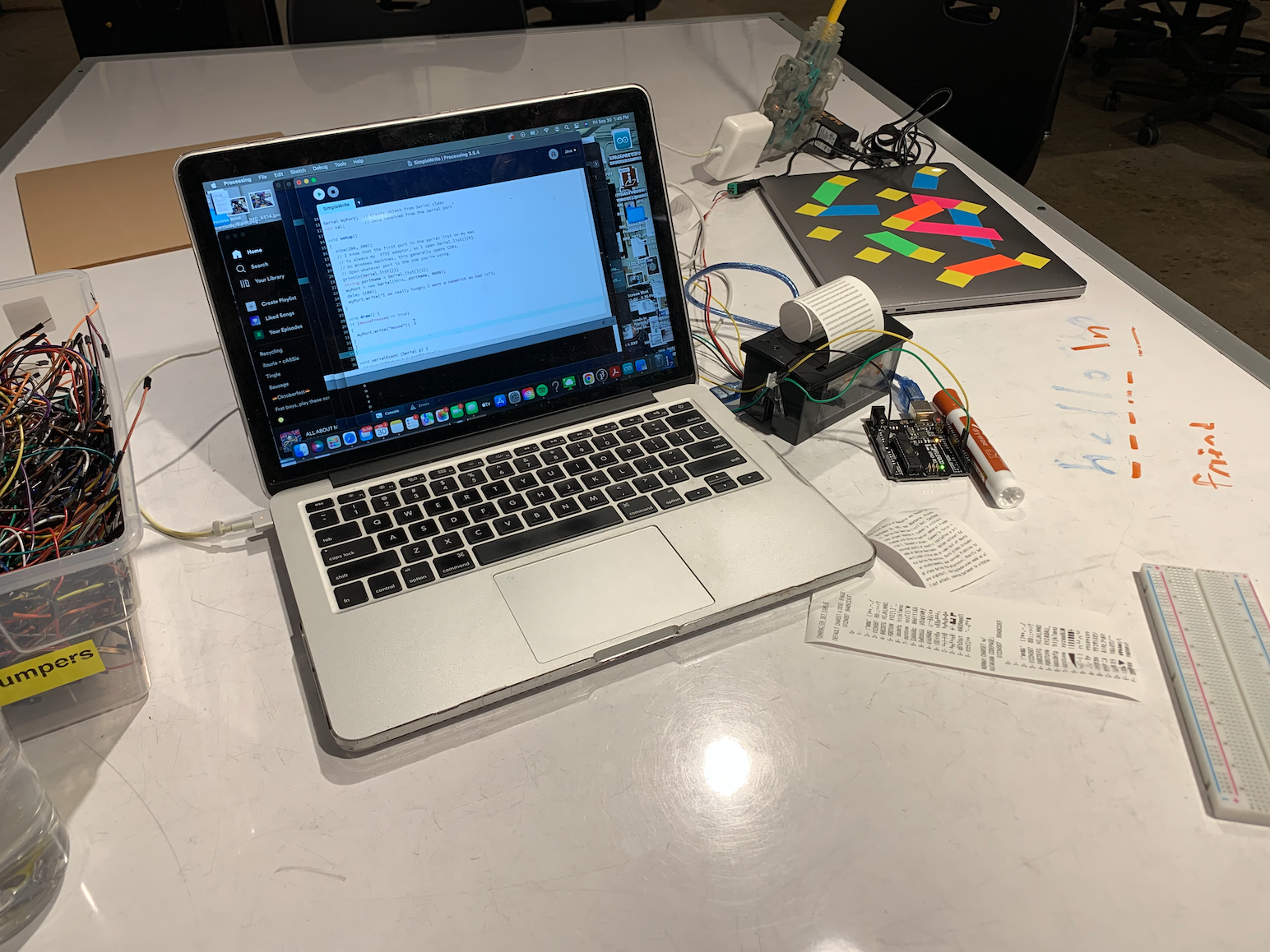
I started my process by working with Zach to learn how to control the receipt printer and how to use Processing.
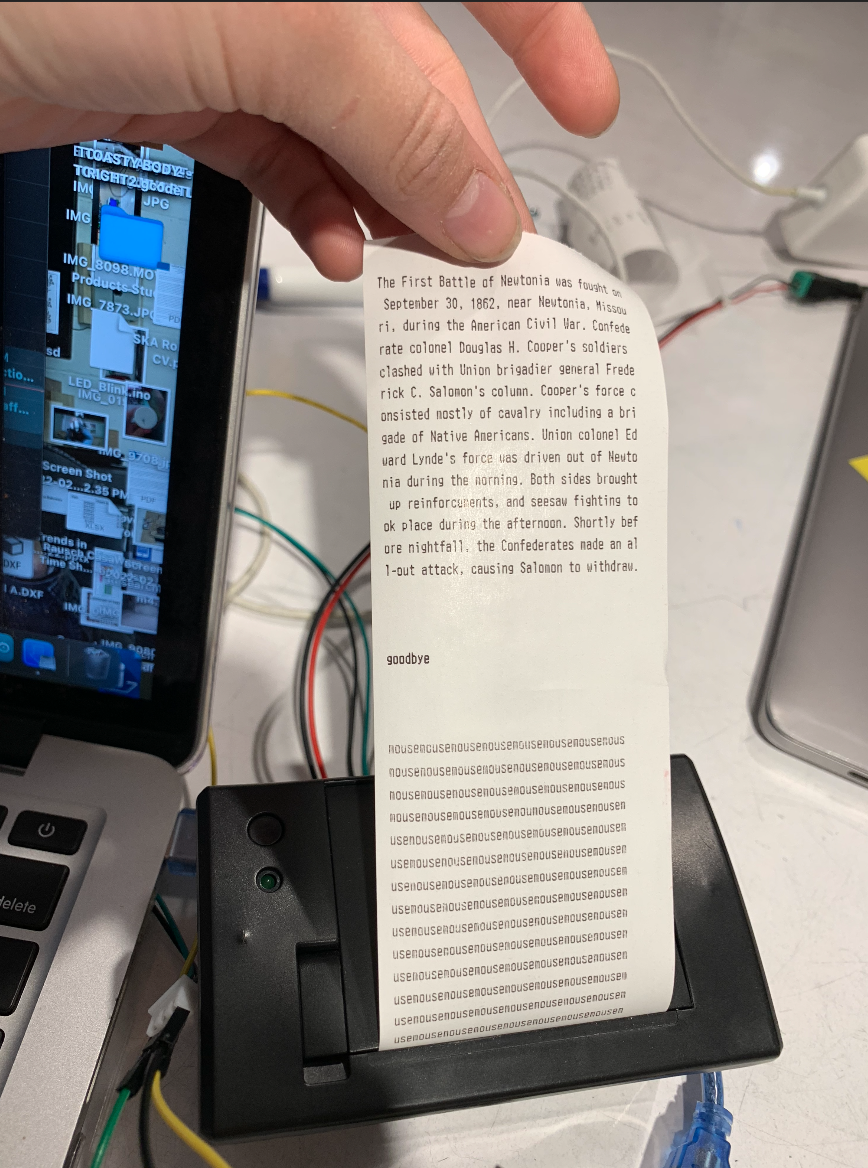
Some of the text that we generated to test the code/printer. It says mouse repeatedly because I spammed the mouse click.
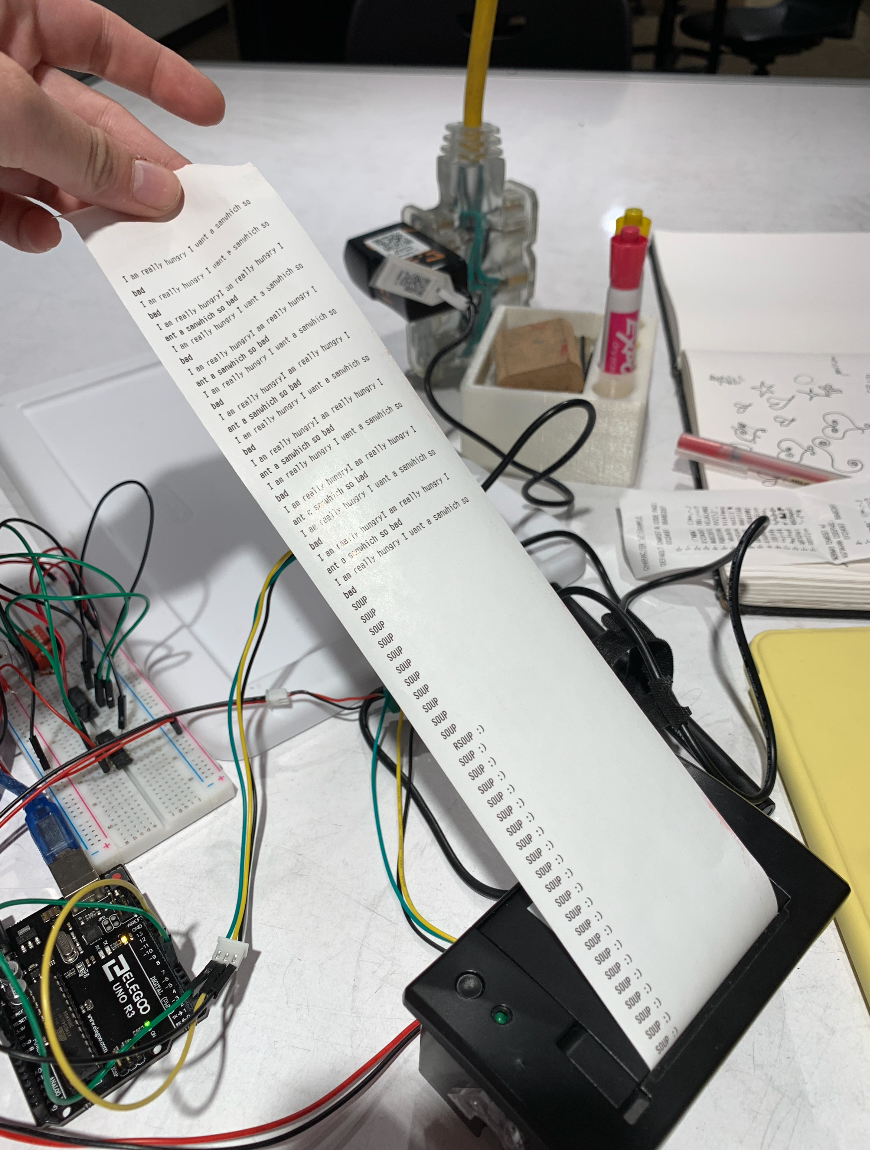
More messing with printing text. Most of this was from my attempts to make a keypress result in text printing.
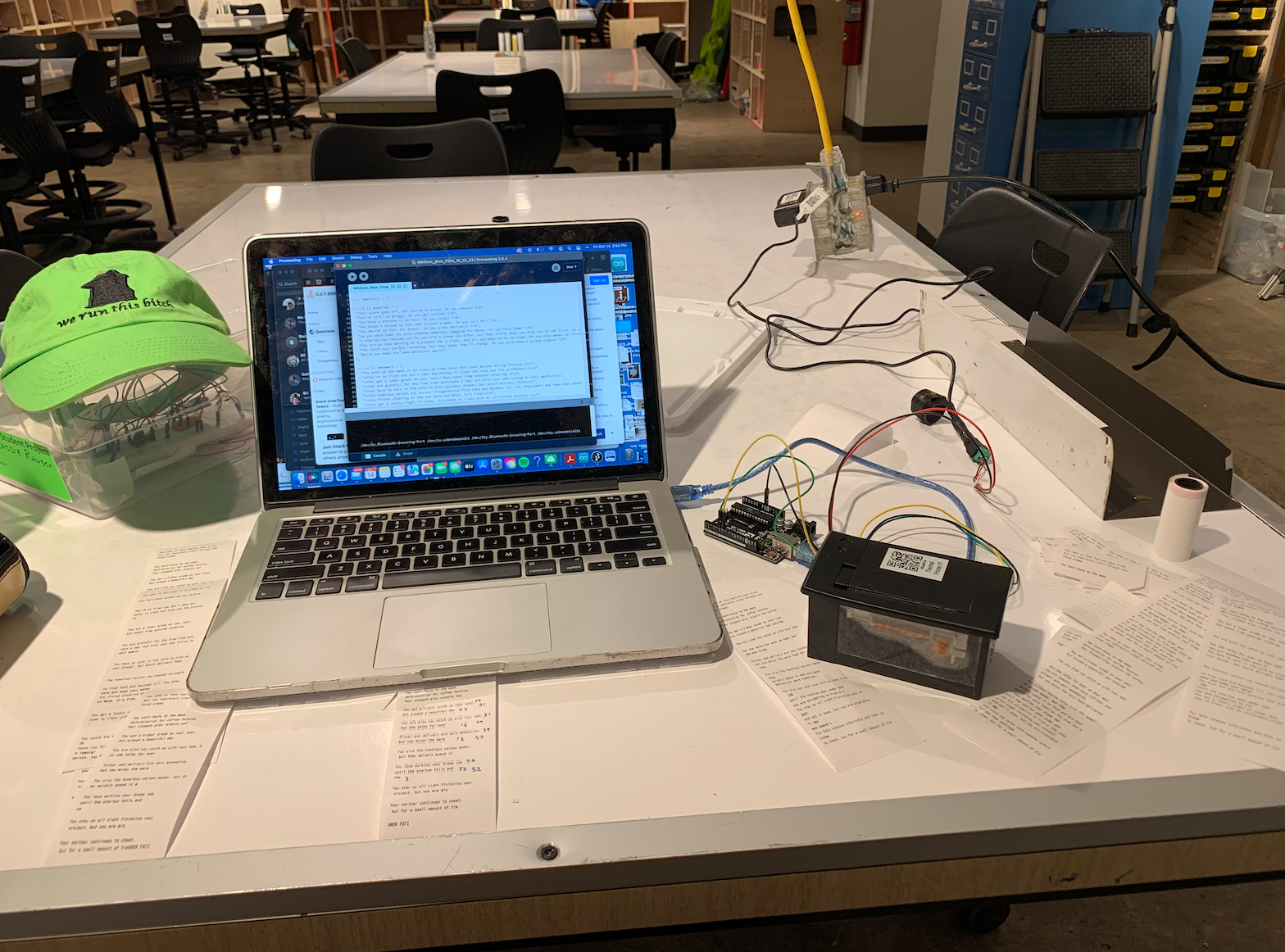
I spent much of my time surrounded by receipts. It took a while to format the text to print well.
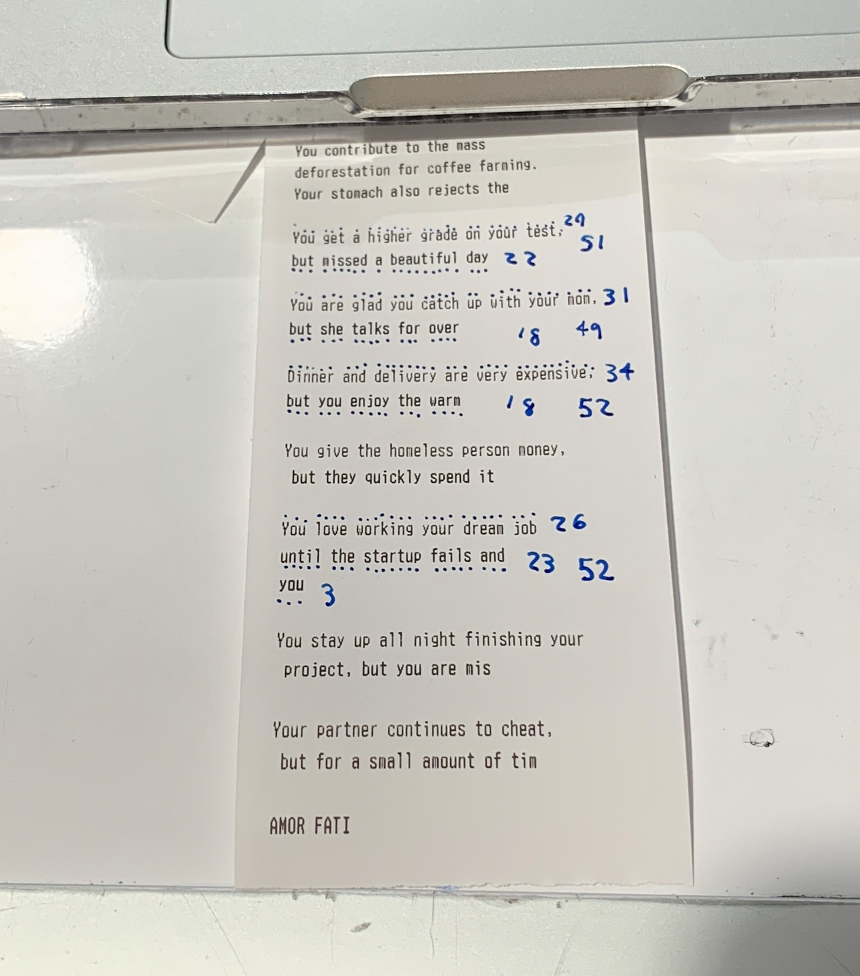
This is when I was trying to figure out why it printed some responses but not others.
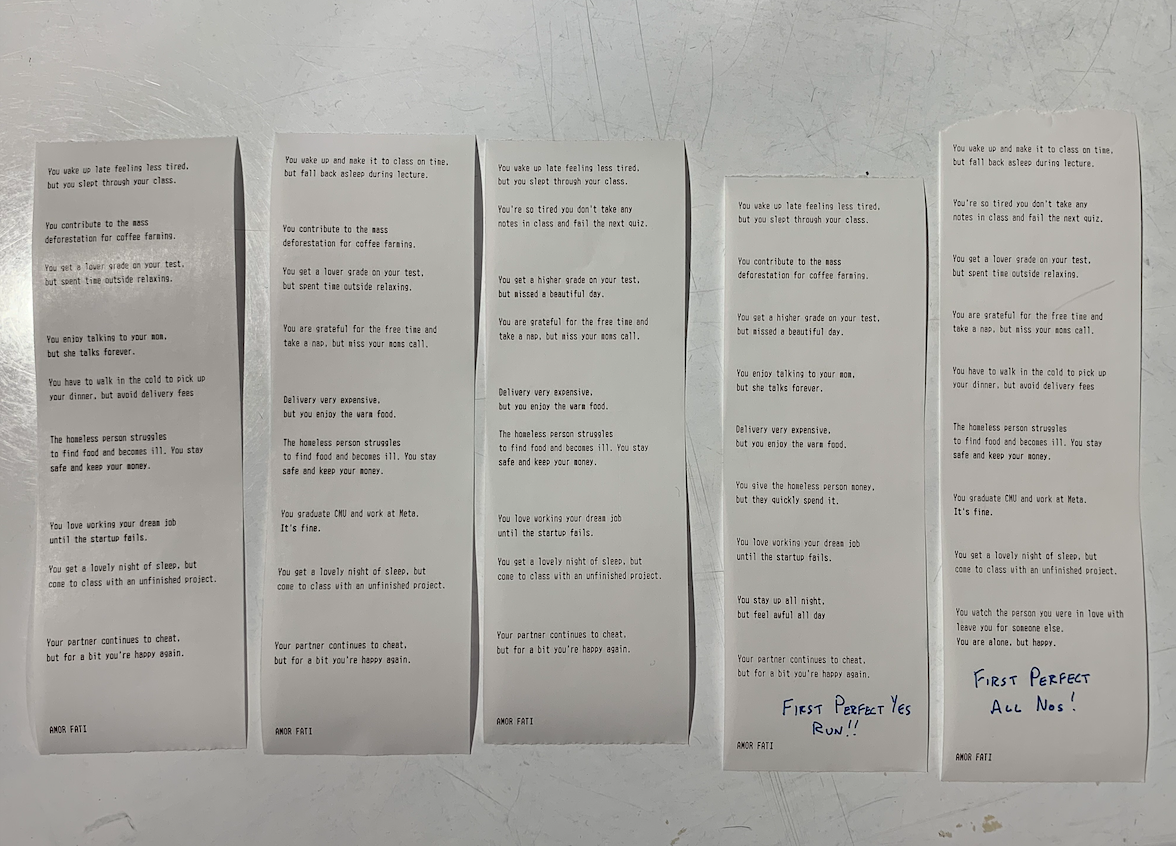
A spread of some of my first successful runs! I started by making all of the yes responses print properly then the no responses.
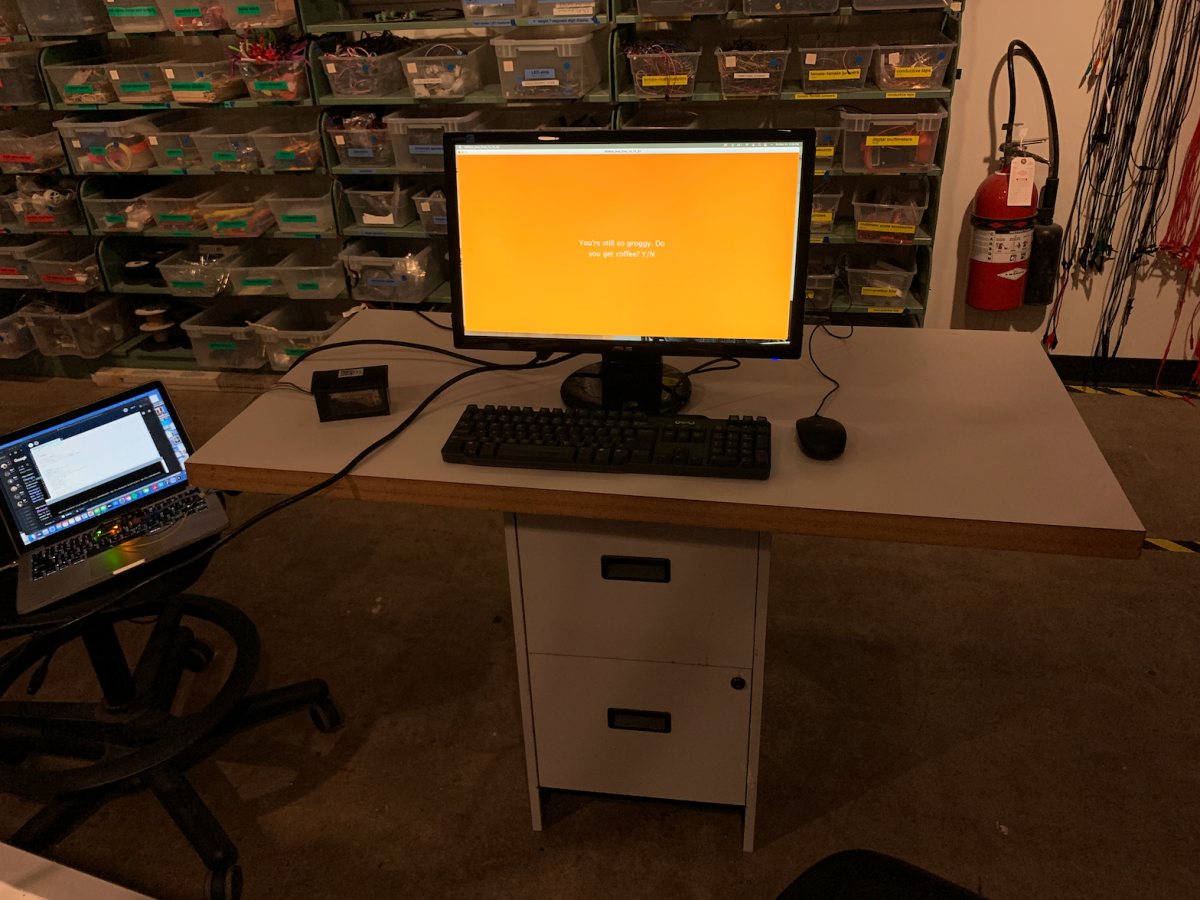
Running my project through another monitor to see how it would display.
PROCESS REFLECTION:
This project was a success in my opinion, because my main goal was to learn more about philosophy and Processing. Taking a philosophy that is new to me and trying to convey it through an experience for a stranger to understand that philosophy was harder than I imagined. If I were to redo this project, I would have spent much more time debugging my code and considering the experience of my project and less studying philosophy. Experience design is not something new to me, but this was my first attempt at an experiential art piece. Something I learned was how much more you are trying to influence the users emotions and thoughts and less so the actions like I typically do in my products.
Processing was vaguely familiar to me from a basic high school course I took in java, but I learned so much from this project and re-familiarized myself with this coding language. Zach was a huge help in clarifying basic concepts for me again, and even more so in making the more complex parts of my code work. I learned a lot about strings and how finicky they can be, and how to connect processing to Arduino. The receipt printer was also new for me to use, but I’d love to work with it more in the future. The idea of walking away from a digital experience with something physical is important to me, because it crosses the boundary from being a thought experiment to something that users will hold onto.
CODE:
//this code is designed to recieve input from a user as a key press of either 'y' or 'n'
//in response to a displayed question and then compile correlating responses
//to this input and print a receipt with the responses generated
int qcounter = 0; //counter that tracks the question you are on
String [] receipt = {" ", " ", " ", " ", " ", " ", " ", " ", " ", " "}; //string that holds all of the responses to be printed
char results[] = {' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '}; //string that holds all of the users input
"Your alarm goes off, but you're so tired. Do you snooze? Y/N",
"You're still so groggy. Do you get coffee? Y/N",
"You have a midterm in an hour. Do you study? Y/N",
"You haven't talked to your mom in over a week. Do you call her? Y/N",
"You decide on Thai for dinner. Do you order delivery? Y/N",
"As you walk home you pass someone homeless, begging for money. Do you help them? Y/N",
"A startup has reached out to you with a dream job offer, but they insist that you drop out of CMU first. Do you do it? Y/N",
"You are up late working on a project for a class, but all you want to do is sleep. Do you stay awake to finish it? Y/N",
"You catch your partner cheating, but they swear they'll change. Do you give them a second chance? Y/N",
"Would you make the same decisions again?",
"You wake up and make it to class on time,\nbut fall back asleep during lecture.\n\n",
"\nYou're so tired you don't take any\nnotes in class and fail the next quiz.\n\n",
"\nYou get a lower grade on your test,\nbut spent time outside relaxing.\n\n",
"\nYou are grateful for the free time and\ntake a nap, but miss your moms call.\n\n",
"\nYou have to walk in the cold to pick up\nyour dinner, but avoid delivery fees\n\n",
"\nThe homeless person struggles\nto find food and becomes ill. You stay\nsafe and keep your money.\n\n",
"\nYou graduate CMU and work at Meta.\nIt's fine.\n\n",
"\nYou get a lovely night of sleep, but\ncome to class with an unfinished project.\n\n",
"\nYou watch the person you were in love with\nleave you for someone else.\nYou are alone, but happy.\n\n\n\n\n\n",
"You wake up late feeling less tired,\nbut you slept through your class.\r\n",
"\n\nYou contribute to the mass\ndeforestation for coffee farming.\r\n",
"\n\nYou get a higher grade on your test,\nbut missed a beautiful day.\r\n",
"\n\nYou enjoy talking to your mom,\nbut she talks forever.\r\n",
"\n\nDelivery very expensive,\nbut you enjoy the warm food.\r\n",
"\n\nYou give the homeless person money,\nbut they quickly spend it.\r\n",
"\n\nYou love working your dream job\nuntil the startup fails.\r\n",
"\n\nYou stay up all night,\nbut feel awful all day\r\n",
"\n\nYour partner continues to cheat,\nbut for a bit you're happy again.\r\n\n\n",
import processing.serial.*;
Serial myPort; // Create object from Serial class
int val; // Data received from the serial port
String portName = Serial.list()[1];
myPort = new Serial(this, portName, 9600);
void draw() { // drawing background and text
background (240, 130, 0);
textAlign(CENTER, CENTER);
String printresults = new String(results);
// text(printresults, 10, 10);
// text(qcounter, 10, 20);
if (qcounter < 10) text(question[qcounter], width/2, height/2, 500, 300);
void receiptbuilder () { // function to build all of the responses to be printed based on user input
for (int counter = 0; counter < 10; counter++) {
if (results[counter] == 'y') {
receipt[counter] = yanswers[counter];
} else if (results[counter] == 'n') {
receipt[counter] = nanswers[counter];
myPort.write(receipt[0]);
myPort.write(receipt[1]);
myPort.write(receipt[2]);
myPort.write(receipt[3]);
myPort.write(receipt[4]);
myPort.write(receipt[5]);
myPort.write(receipt[6]);
myPort.write(receipt[7]);
myPort.write(receipt[8]);
myPort.write(receipt[9]);
void serialEvent (Serial p) {
String inString = p.readString();
//AMOR FATI
//by Cassie Rausch
//this code is designed to recieve input from a user as a key press of either 'y' or 'n'
//in response to a displayed question and then compile correlating responses
//to this input and print a receipt with the responses generated
int qcounter = 0; //counter that tracks the question you are on
String [] receipt = {" ", " ", " ", " ", " ", " ", " ", " ", " ", " "}; //string that holds all of the responses to be printed
char results[] = {' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '}; //string that holds all of the users input
String [] question = {
"Your alarm goes off, but you're so tired. Do you snooze? Y/N",
"You're still so groggy. Do you get coffee? Y/N",
"You have a midterm in an hour. Do you study? Y/N",
"You haven't talked to your mom in over a week. Do you call her? Y/N",
"You decide on Thai for dinner. Do you order delivery? Y/N",
"As you walk home you pass someone homeless, begging for money. Do you help them? Y/N",
"A startup has reached out to you with a dream job offer, but they insist that you drop out of CMU first. Do you do it? Y/N",
"You are up late working on a project for a class, but all you want to do is sleep. Do you stay awake to finish it? Y/N",
"You catch your partner cheating, but they swear they'll change. Do you give them a second chance? Y/N",
"Would you make the same decisions again?",
};
String [] nanswers = {
"You wake up and make it to class on time,\nbut fall back asleep during lecture.\n\n",
"\nYou're so tired you don't take any\nnotes in class and fail the next quiz.\n\n",
"\nYou get a lower grade on your test,\nbut spent time outside relaxing.\n\n",
"\nYou are grateful for the free time and\ntake a nap, but miss your moms call.\n\n",
"\nYou have to walk in the cold to pick up\nyour dinner, but avoid delivery fees\n\n",
"\nThe homeless person struggles\nto find food and becomes ill. You stay\nsafe and keep your money.\n\n",
"\nYou graduate CMU and work at Meta.\nIt's fine.\n\n",
"\nYou get a lovely night of sleep, but\ncome to class with an unfinished project.\n\n",
"\nYou watch the person you were in love with\nleave you for someone else.\nYou are alone, but happy.\n\n\n\n\n\n",
"\n\nAMOR FATI \n\n\n\n"
};
String [] yanswers = {
"You wake up late feeling less tired,\nbut you slept through your class.\r\n",
"\n\nYou contribute to the mass\ndeforestation for coffee farming.\r\n",
"\n\nYou get a higher grade on your test,\nbut missed a beautiful day.\r\n",
"\n\nYou enjoy talking to your mom,\nbut she talks forever.\r\n",
"\n\nDelivery very expensive,\nbut you enjoy the warm food.\r\n",
"\n\nYou give the homeless person money,\nbut they quickly spend it.\r\n",
"\n\nYou love working your dream job\nuntil the startup fails.\r\n",
"\n\nYou stay up all night,\nbut feel awful all day\r\n",
"\n\nYour partner continues to cheat,\nbut for a bit you're happy again.\r\n\n\n",
"\n\nAMOR FATI \n\n\n\n"
};
import processing.serial.*;
Serial myPort; // Create object from Serial class
int val; // Data received from the serial port
void setup() {
size(1300, 700);
println(Serial.list());
String portName = Serial.list()[1];
myPort = new Serial(this, portName, 9600);
}
void draw() { // drawing background and text
background (240, 130, 0);
textSize(25);
rectMode (CENTER);
textAlign(CENTER, CENTER);
String printresults = new String(results);
if (qcounter == 10) {
delay (1000);
receiptbuilder ();
delay (5000);
qcounter = 0;
}
// text(printresults, 10, 10);
// text(qcounter, 10, 20);
if (qcounter < 10) text(question[qcounter], width/2, height/2, 500, 300);
}
void keyPressed () {
if (qcounter < 10) {
if (key == 'y') {
results[qcounter] = 'y';
qcounter++;
}
if (key == 'n') {
results[qcounter] = 'n';
qcounter++;
}
}
}
void receiptbuilder () { // function to build all of the responses to be printed based on user input
for (int counter = 0; counter < 10; counter++) {
if (results[counter] == 'y') {
receipt[counter] = yanswers[counter];
} else if (results[counter] == 'n') {
receipt[counter] = nanswers[counter];
}
}
myPort.write(receipt[0]);
delay (2000);
myPort.write(receipt[1]);
delay (2000);
myPort.write(receipt[2]);
delay (2000);
myPort.write(receipt[3]);
delay (2000);
myPort.write(receipt[4]);
delay (2000);
myPort.write(receipt[5]);
delay (2000);
myPort.write(receipt[6]);
delay (2000);
myPort.write(receipt[7]);
delay (2000);
myPort.write(receipt[8]);
delay (2000);
myPort.write(receipt[9]);
}
void serialEvent (Serial p) {
String inString = p.readString();
// println (inString);
}
//AMOR FATI
//by Cassie Rausch
//this code is designed to recieve input from a user as a key press of either 'y' or 'n'
//in response to a displayed question and then compile correlating responses
//to this input and print a receipt with the responses generated
int qcounter = 0; //counter that tracks the question you are on
String [] receipt = {" ", " ", " ", " ", " ", " ", " ", " ", " ", " "}; //string that holds all of the responses to be printed
char results[] = {' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '}; //string that holds all of the users input
String [] question = {
"Your alarm goes off, but you're so tired. Do you snooze? Y/N",
"You're still so groggy. Do you get coffee? Y/N",
"You have a midterm in an hour. Do you study? Y/N",
"You haven't talked to your mom in over a week. Do you call her? Y/N",
"You decide on Thai for dinner. Do you order delivery? Y/N",
"As you walk home you pass someone homeless, begging for money. Do you help them? Y/N",
"A startup has reached out to you with a dream job offer, but they insist that you drop out of CMU first. Do you do it? Y/N",
"You are up late working on a project for a class, but all you want to do is sleep. Do you stay awake to finish it? Y/N",
"You catch your partner cheating, but they swear they'll change. Do you give them a second chance? Y/N",
"Would you make the same decisions again?",
};
String [] nanswers = {
"You wake up and make it to class on time,\nbut fall back asleep during lecture.\n\n",
"\nYou're so tired you don't take any\nnotes in class and fail the next quiz.\n\n",
"\nYou get a lower grade on your test,\nbut spent time outside relaxing.\n\n",
"\nYou are grateful for the free time and\ntake a nap, but miss your moms call.\n\n",
"\nYou have to walk in the cold to pick up\nyour dinner, but avoid delivery fees\n\n",
"\nThe homeless person struggles\nto find food and becomes ill. You stay\nsafe and keep your money.\n\n",
"\nYou graduate CMU and work at Meta.\nIt's fine.\n\n",
"\nYou get a lovely night of sleep, but\ncome to class with an unfinished project.\n\n",
"\nYou watch the person you were in love with\nleave you for someone else.\nYou are alone, but happy.\n\n\n\n\n\n",
"\n\nAMOR FATI \n\n\n\n"
};
String [] yanswers = {
"You wake up late feeling less tired,\nbut you slept through your class.\r\n",
"\n\nYou contribute to the mass\ndeforestation for coffee farming.\r\n",
"\n\nYou get a higher grade on your test,\nbut missed a beautiful day.\r\n",
"\n\nYou enjoy talking to your mom,\nbut she talks forever.\r\n",
"\n\nDelivery very expensive,\nbut you enjoy the warm food.\r\n",
"\n\nYou give the homeless person money,\nbut they quickly spend it.\r\n",
"\n\nYou love working your dream job\nuntil the startup fails.\r\n",
"\n\nYou stay up all night,\nbut feel awful all day\r\n",
"\n\nYour partner continues to cheat,\nbut for a bit you're happy again.\r\n\n\n",
"\n\nAMOR FATI \n\n\n\n"
};
import processing.serial.*;
Serial myPort; // Create object from Serial class
int val; // Data received from the serial port
void setup() {
size(1300, 700);
println(Serial.list());
String portName = Serial.list()[1];
myPort = new Serial(this, portName, 9600);
}
void draw() { // drawing background and text
background (240, 130, 0);
textSize(25);
rectMode (CENTER);
textAlign(CENTER, CENTER);
String printresults = new String(results);
if (qcounter == 10) {
delay (1000);
receiptbuilder ();
delay (5000);
qcounter = 0;
}
// text(printresults, 10, 10);
// text(qcounter, 10, 20);
if (qcounter < 10) text(question[qcounter], width/2, height/2, 500, 300);
}
void keyPressed () {
if (qcounter < 10) {
if (key == 'y') {
results[qcounter] = 'y';
qcounter++;
}
if (key == 'n') {
results[qcounter] = 'n';
qcounter++;
}
}
}
void receiptbuilder () { // function to build all of the responses to be printed based on user input
for (int counter = 0; counter < 10; counter++) {
if (results[counter] == 'y') {
receipt[counter] = yanswers[counter];
} else if (results[counter] == 'n') {
receipt[counter] = nanswers[counter];
}
}
myPort.write(receipt[0]);
delay (2000);
myPort.write(receipt[1]);
delay (2000);
myPort.write(receipt[2]);
delay (2000);
myPort.write(receipt[3]);
delay (2000);
myPort.write(receipt[4]);
delay (2000);
myPort.write(receipt[5]);
delay (2000);
myPort.write(receipt[6]);
delay (2000);
myPort.write(receipt[7]);
delay (2000);
myPort.write(receipt[8]);
delay (2000);
myPort.write(receipt[9]);
}
void serialEvent (Serial p) {
String inString = p.readString();
// println (inString);
}