Video
Description
Our goal was to create two robots that would “talk” to each other by passing a ball bearing back and forth between them.
The initial idea was simple: we would have two identical robots that would seesaw back and forth. The ball would get from one robot to the next by rolling over a ramp.
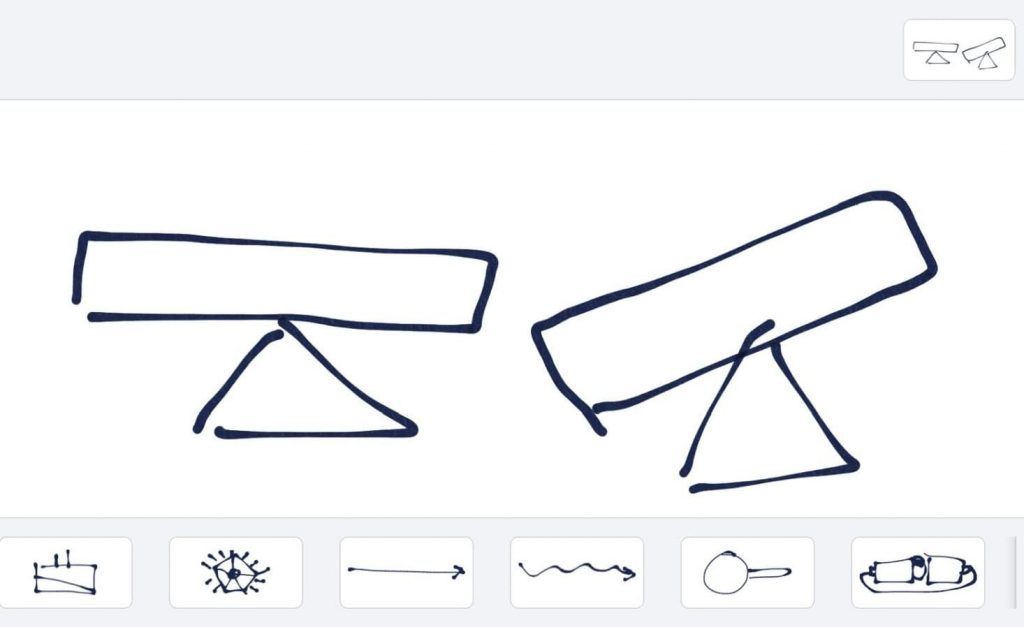
The main challenges we encountered when putting the project together were:
- The angle of the ramp: we were unsure what the angle of the ramp would be, so we taped cardboard to the assembly in various positions and tested them out until we found the perfect angle.
- Threshold of the photoreflector: The threshold of the photoreflector kept changing as we tested depending on where in the room we had the robots and the angle of the ramp. After we settled on the angle of the ramp, we could figure out the threshold.
- The robots sometimes had trouble catching the ball. We solved this by holding the robots next to a flat surface so they stayed in one spot. We also discovered that it was a fun game to move the robots around to catch the ball bearings.
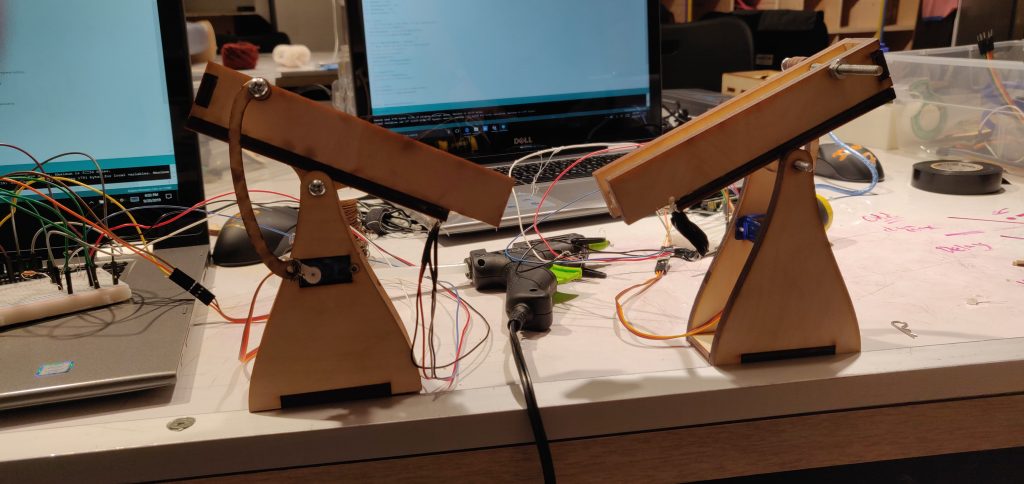
Pattern of Interaction
This is the interaction loop, as soon as the robot goes through the odd sequence it becomes the even sequence and vice versa.
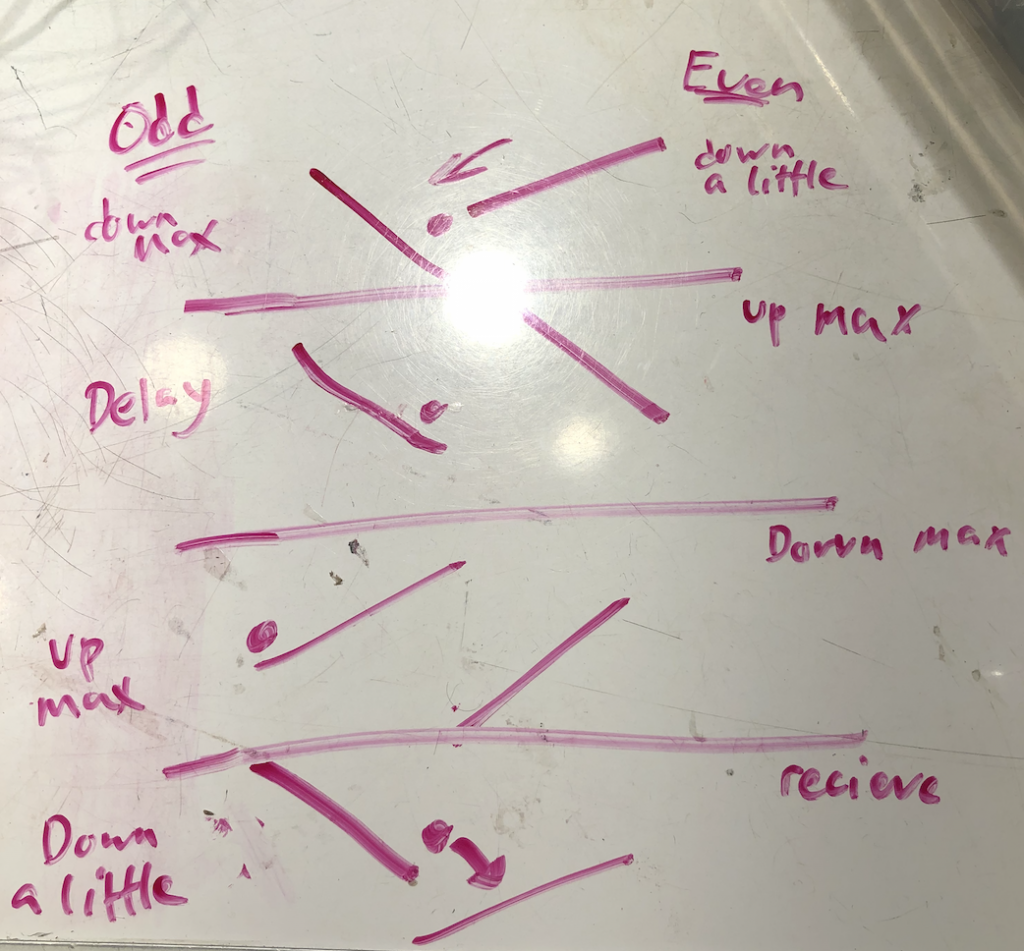
Mechanism Design
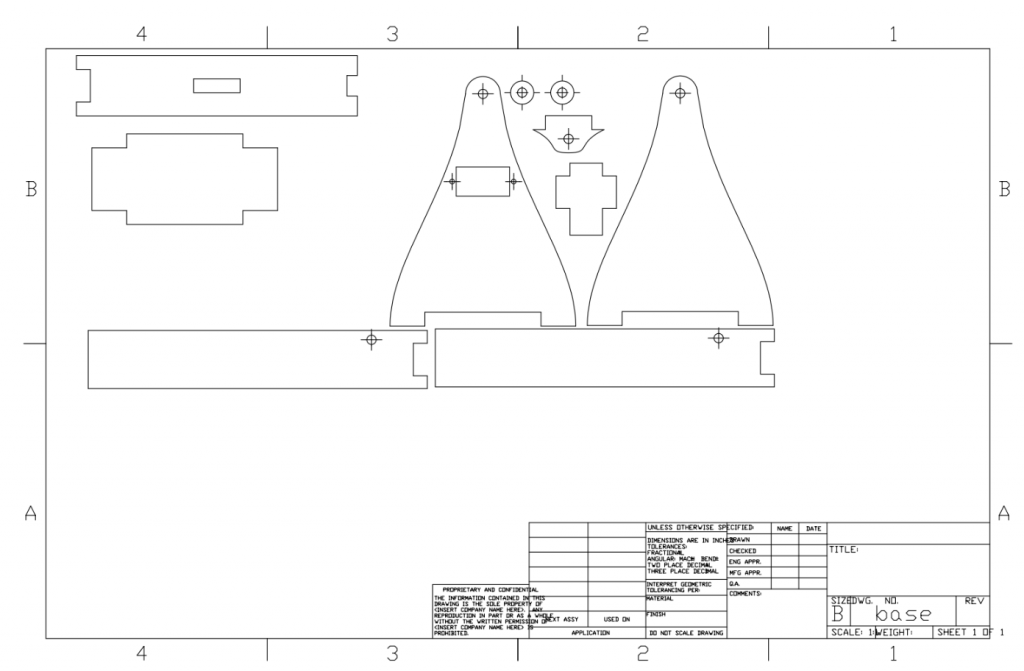
Code
#include <Servo.h>; Servo robot; const int photoPin = A0; void setup() { robot.attach(9); Serial.begin(115200); while (! Serial); Serial.println("BEGIN"); } int count = 0; int thresh = 750; int upAngle = 150; int downAngleSend = 20; int downAngle = 20; int photoPinRead = 0; void loop() { // put your main code here, to run repeatedly: Serial.println("TEST"); photoPinRead = analogRead(photoPin); Serial.println(photoPinRead); while (photoPinRead > thresh) { // When there is ball not on photosensor Serial.println("count"); Serial.println(count); if(count%2==1){ robot.write(downAngleSend); Serial.println("sending"); } if(count%2==0){ robot.write(downAngle); Serial.println("receiving"); } delay(1000); photoPinRead = analogRead(photoPin); Serial.println(photoPinRead); } while (photoPinRead<thresh){ // when there is no ball on the photosensor count = count + 1; robot.write(upAngle); delay(1000); photoPinRead = analogRead(photoPin); Serial.println(photoPinRead); } delay(500); }
Leave a Reply
You must be logged in to post a comment.