Interactive little desk friends that move back and forth when pet. The desk friend knows when the other is touched and will ‘beg’ for attention so that it can be as loved as the other desk friend.
The friends compare each other based on attention related to touch, presence through sound, and light presence. If one friend is getting more attention, they earn ‘points’ on a counter while the other one will lose points, causing it to beg more fiercely. Any attention, be it through touch, light, or sound, will add and subtract points from the connected pets.
Visualize the counter on the circuit playground through the LEDS (red for negative, green for positive)
If a pet has a significant amount of points more than the other, it may ‘brag’ through dancing.
We both have two servos at our disposal that can generate 2 axis of motion for each of our desk pets. We plan to develop prototypes using these servos to simulate ‘begging’ from the pets. (This might prove to be too complicated). We decided to remove inflatables from our materials list at this current time with the concern that it may be too flimsy for what we hope to achieve.
We both agree that it could be really expressive for the desk friend to be something as simple as a piece of fabric pinned to strings attached to the servos, because as the servos move, the fabric of the desk friend will change and fold in unique ways each time.
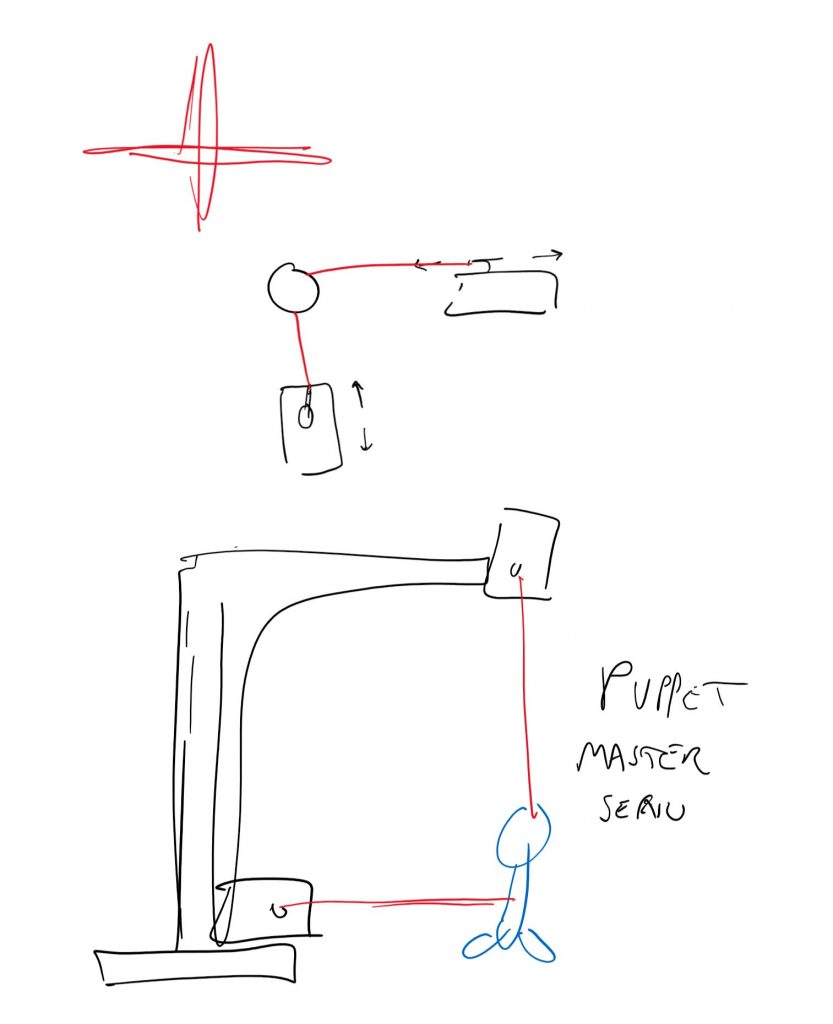
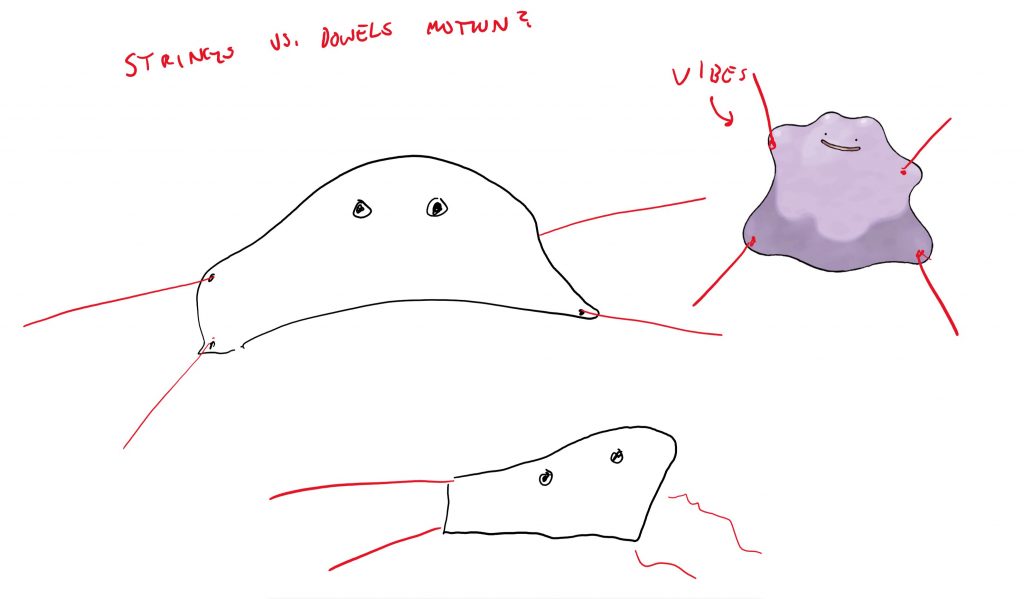
Here’s a preliminary idea for some code that can react differently to different pressure sensitivities – untested for now, but this is a good starting point for us.
# project2.py # Kinetic Fabrics S21 # Kanvi and Yael # some code taken from soft sensor and servo sample files # ----------------------------------------- # Import the standard Python time functions. import time # Import the low-level hardware libraries. import board import digitalio import analogio import pwmio from adafruit_circuitplayground import cp from adafruit_motor import servo # ---------------------------------------------------------------- # Initialize hardware. # Configure the digital input pin used for its pullup resistor bias voltage. bias = digitalio.DigitalInOut(board.D10) bias.switch_to_input(pull=digitalio.Pull.UP) # Configure the analog input pin used to measure the sensor voltage. sensor = analogio.AnalogIn(board.A2) scaling = sensor.reference_voltage / (2**16) # Create a PWMOut object on pad SDA A5 to generate control signals. pwm = pwmio.PWMOut(board.A5, duty_cycle=0, frequency=50) # Create a Servo object which controls a hobby servo using the PWMOut. actuator = servo.Servo(pwm, min_pulse=1000, max_pulse=2000) threshold1 = 0.2 threshold2 = 0.4 threshold3 = 0.6 threshold4 = 0.8 # ---------------------------------------------------------------- # Begin the main processing loop. while True: # Read the integer sensor value and scale it to a value in Volts. volts = sensor.value * scaling # Every handmade sensor will have different properties, so the # interpretation of the voltage value will usually need to be individually # calibrated. One way to accomplish this is by measuring the voltage # response under different conditions, then applying additional scaling and # offset to produce a normalized signal (possibly inverted). For example, # if the observed voltage ranges between 1.4 and 0.1 Volts, the input signal # could be normalized to a [0,1] range as follows: pressure = (1.4 - volts) / (1.4 - 0.1) # Print the voltage reading and normalized value on the console. print(f"({volts}, {pressure})") if pressure > threshold4: for angle in range(0, 180, 5): actuator.angle = angle time.sleep(0.02) for angle in range(180, 0, -5): actuator.angle = angle time.sleep(0.02) elif pressure > threshold3: for angle in range(0, 180, 5): actuator.angle = angle time.sleep(0.04) for angle in range(180, 0, -5): actuator.angle = angle time.sleep(0.04) elif pressure > threshold2: for angle in range(0, 180, 5): actuator.angle = angle time.sleep(0.06) for angle in range(180, 0, -5): actuator.angle = angle time.sleep(0.06) elif pressure > threshold1: for angle in range(0, 180, 5): actuator.angle = angle time.sleep(0.1) for angle in range(180, 0, -5): actuator.angle = angle time.sleep(0.1) else: time.sleep(0.1)
Leave a Reply
You must be logged in to post a comment.