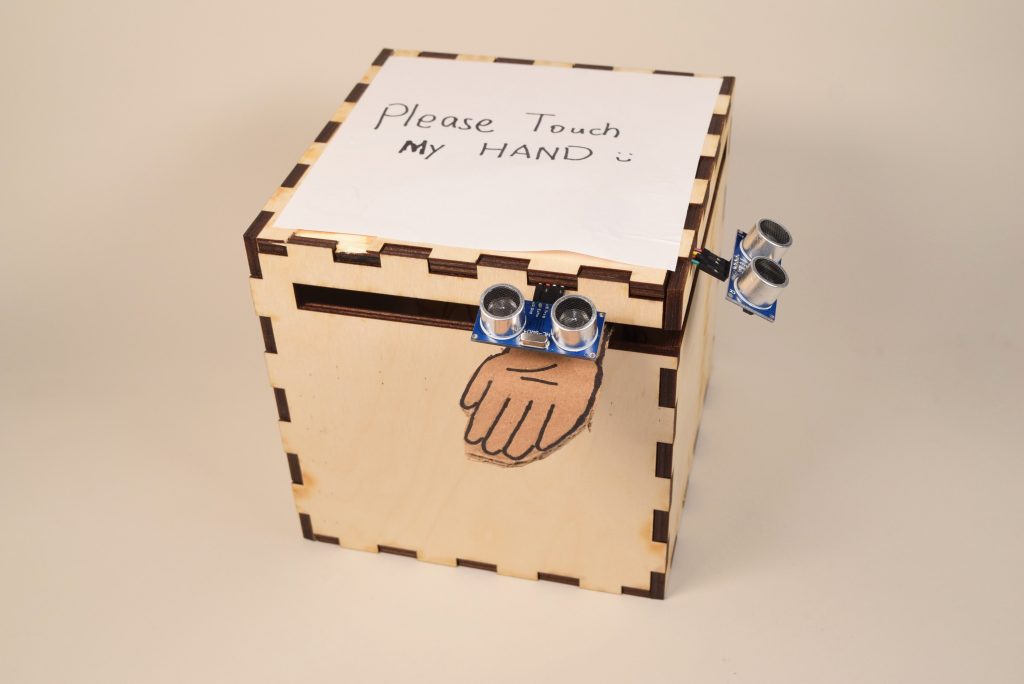
High Five Box
Narrative
A wooden cube with a cardboard hand sticking out of one side. When the user tries the “high-five” the hand, the hand swivels back into the cube and a second hand pops up on a different side of the cube. Now, when the user tries to “high-five” the second hand, it swivels back inside the cube and the first hand flips back out again.
Design details
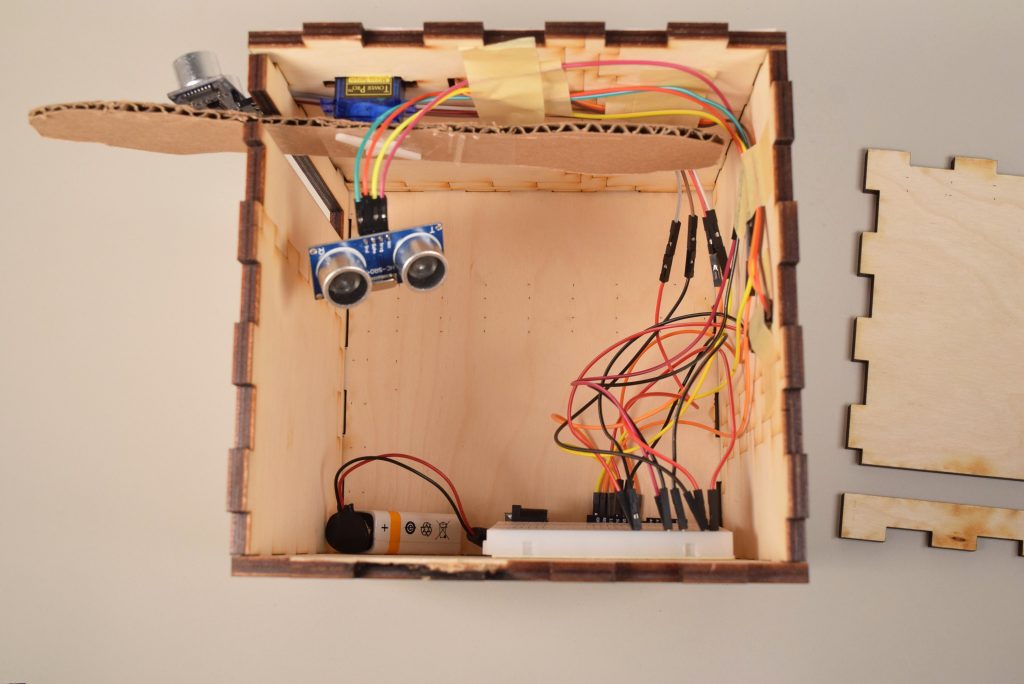
Taped wires inside the box to create turning space for the hand
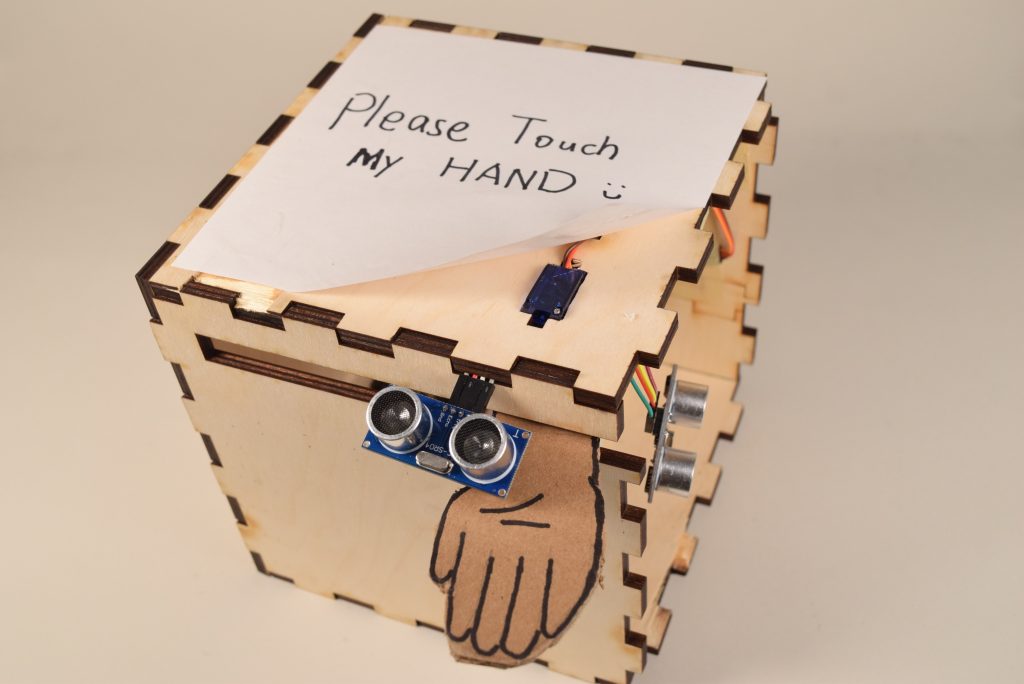
Well-cut hole to fix the motor at the top of the box
How to Trigger the surprise
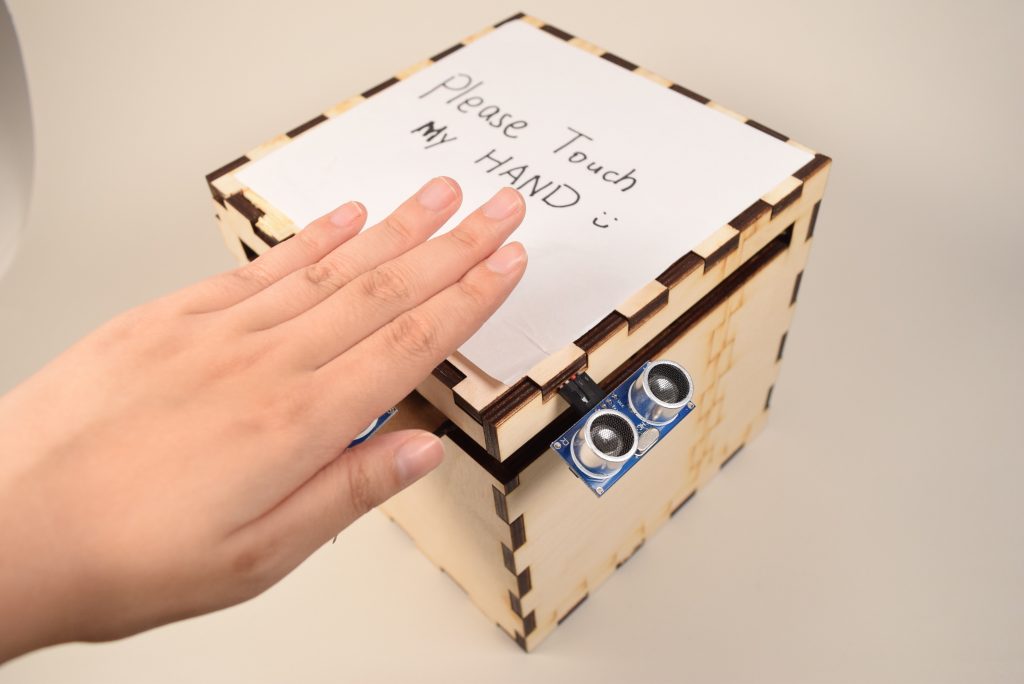
Try to “high five” with the hand
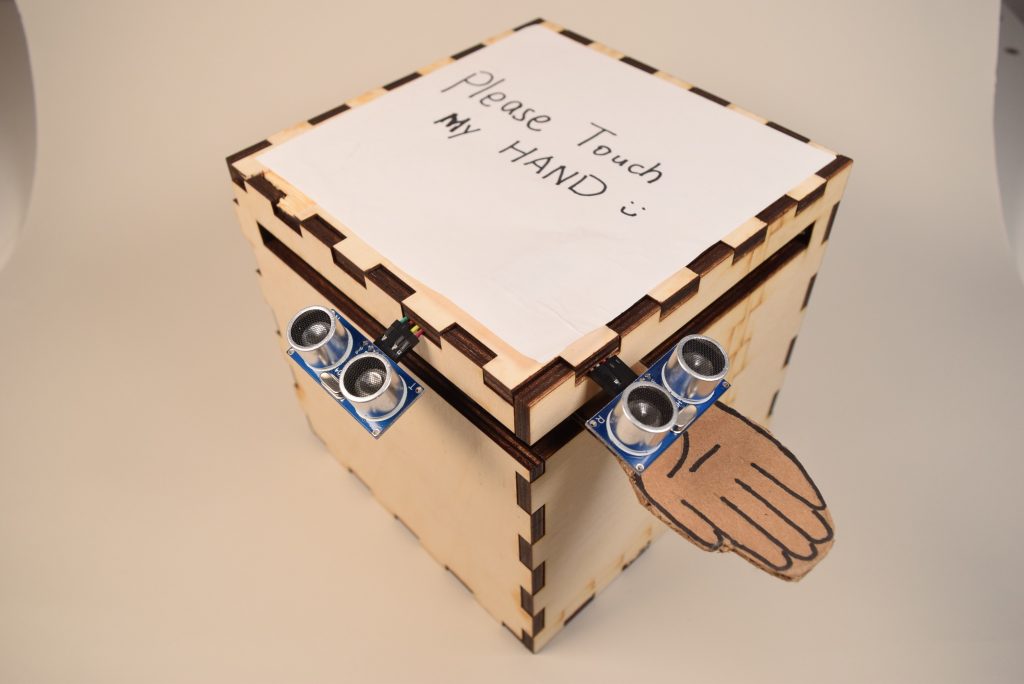
The hand refuses your high five and turns away.
Design Process
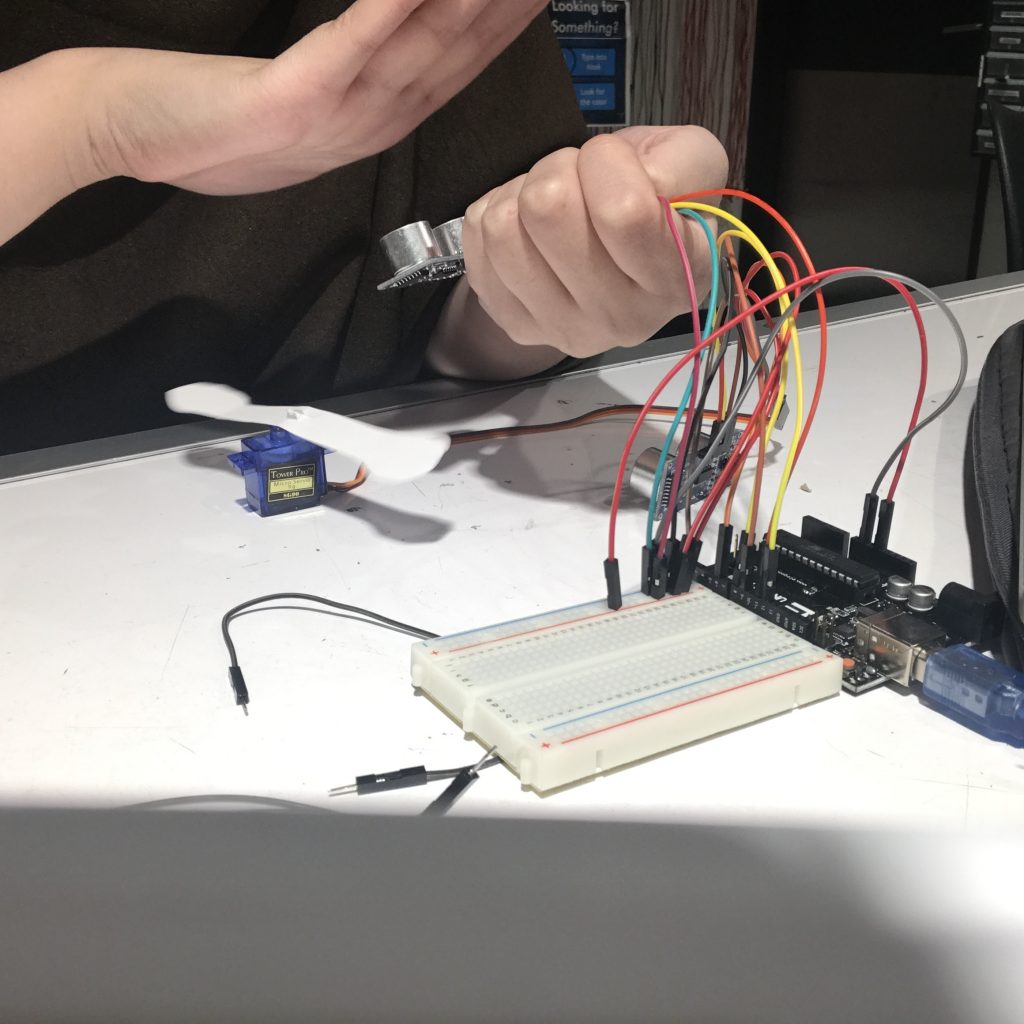
Testing out the appropriate sensing distance for the distance ranger
We started off testing the appropriate distance we should set for the distance ranger.
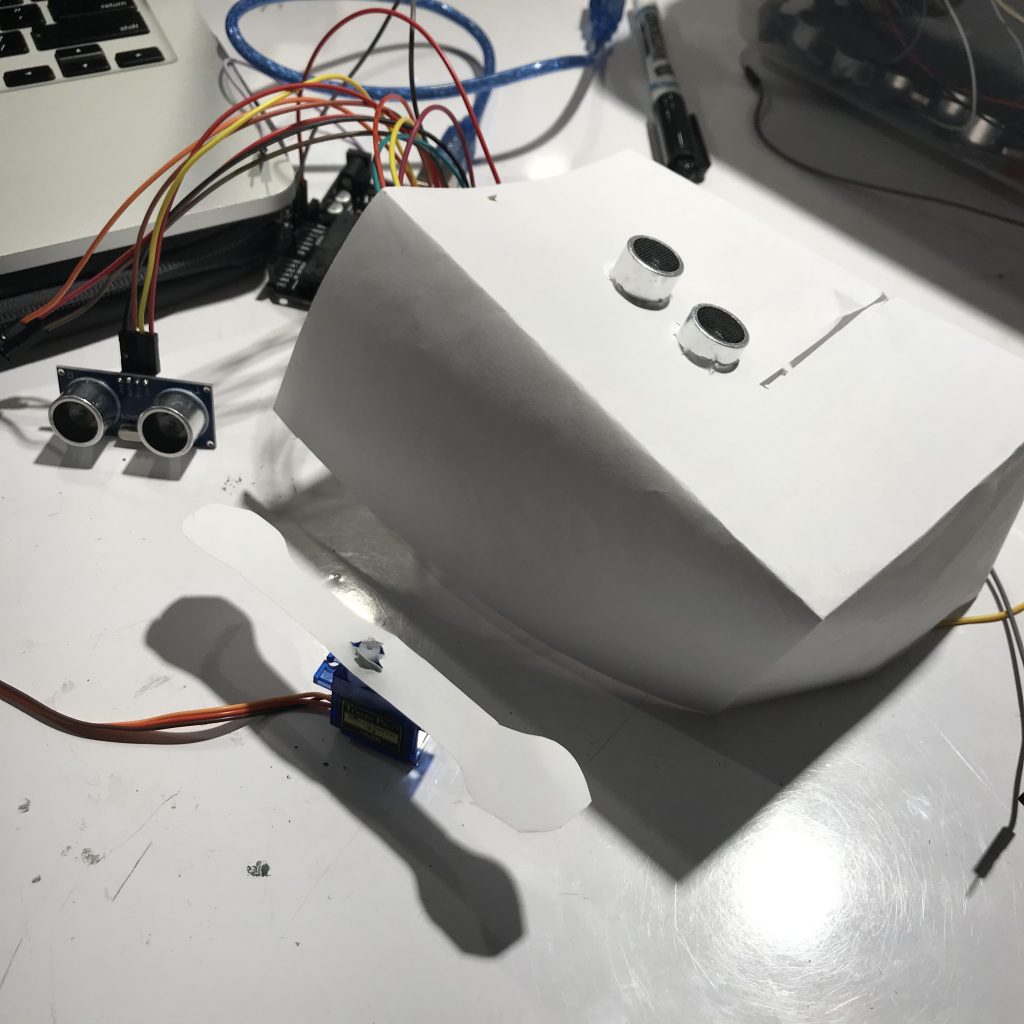
Paper Box Prototype
Later, when Roy was lazer-cutting the box, Caroline tried to figure out the turning angle of the hand by testing the motor and sensor in a paper box. We thought about putting the hand and the sensor on the same surface so that we can hide sensor and forces users to approach the sensor if they want to do high-five. This is why the paper box has . It turns out that the sensor can only have a correct detection of distance when it is facing up, so we eventually changed our plan to let both sensors stick out and face up.
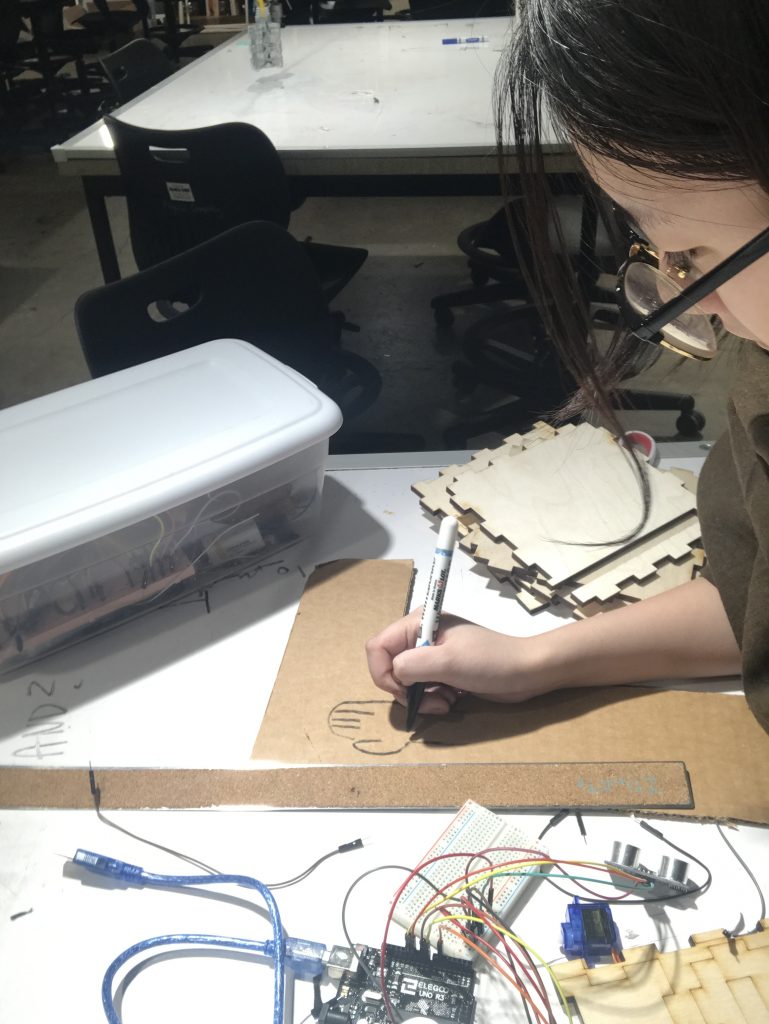
Creating the Hand
After testing the sensor and motor, we started cutting the cupboard hand to fit onto the motor. We made several cupboard hands, since the initial design was too large to fit into the box. .
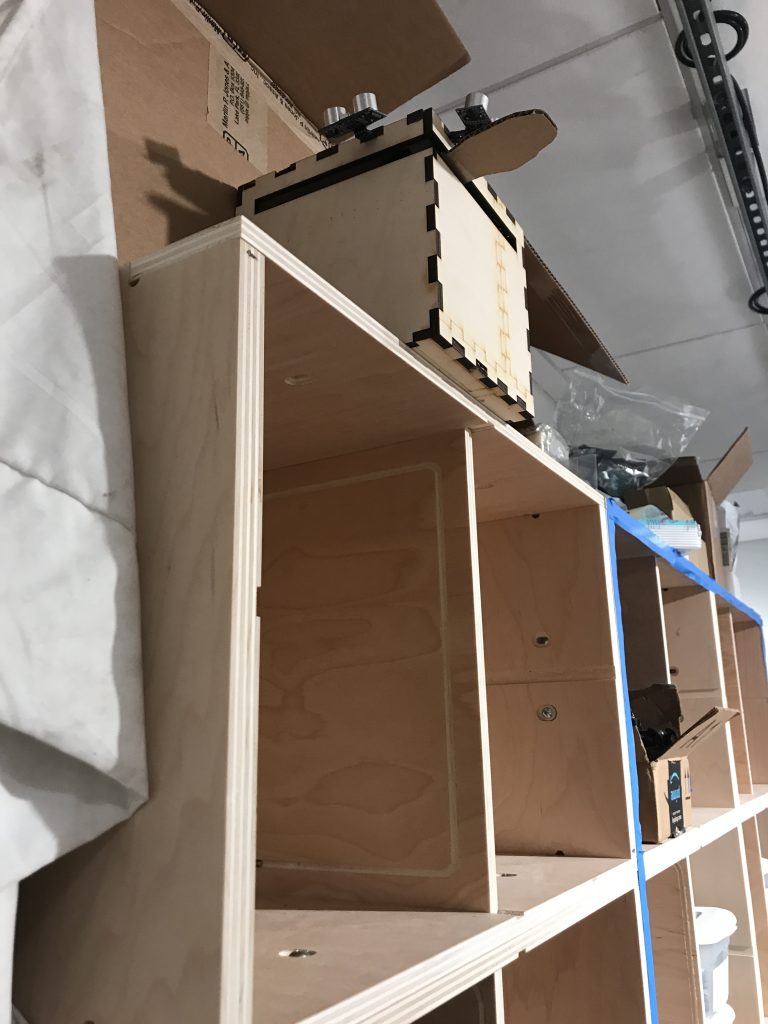
Box On Top of Shelf
One interesting thing happened before our presentation is that because we set the sensor to be so sensitive that if we placed it in a cube it would turn forever until running out of battery, so we had to place it at the top of the shelf.
Schematic
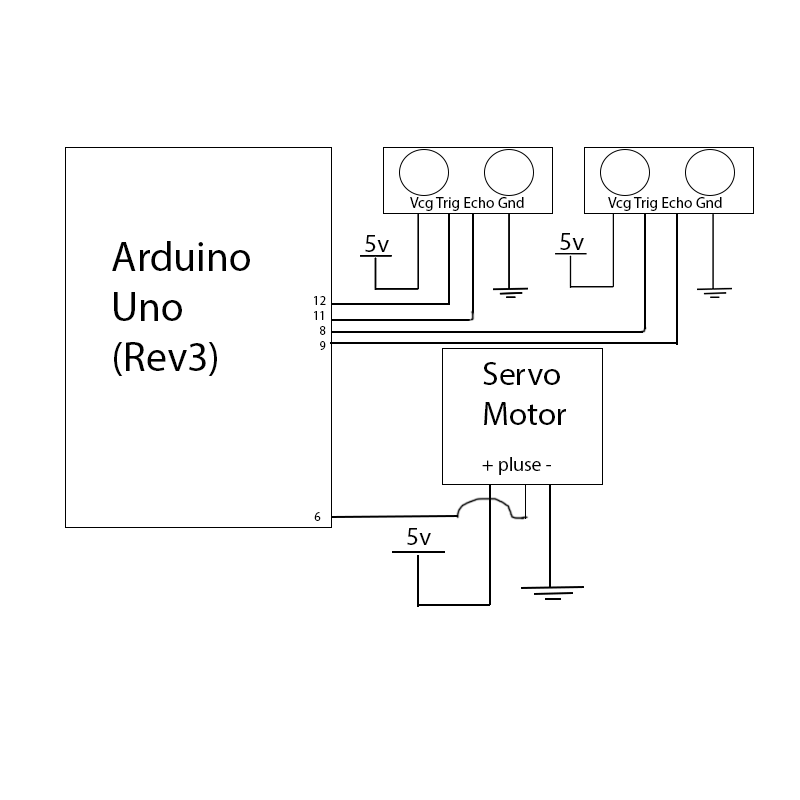
High Five Box Schematic
LINK TO CODE
#include <NewPing.h> #include<Servo.h> boolean isFirstHand = true; #define TRIGGER_PIN1 12 // Arduino pin tied to trigger pin on the ultrasonic sensor. #define ECHO_PIN1 11 // Arduino pin tied to echo pin on the ultrasonic sensor. #define MAX_DISTANCE1 200 // Maximum distance we want to ping for (in centimeters). Maximum sensor distance is rated at 400-500cm. #define TRIGGER_PIN2 8 // Arduino pin tied to trigger pin on the ultrasonic sensor. #define ECHO_PIN2 9 // Arduino pin tied to echo pin on the ultrasonic sensor. #define MAX_DISTANCE2 200 // Maximum distance we want to ping for (in centimeters). Maximum sensor distance is rated at 400-500cm. #define TOOCLOSE 20 #define HANDSPIN 6 NewPing sonar1(TRIGGER_PIN1, ECHO_PIN1, MAX_DISTANCE1); // NewPing setup of pins and maximum distance. NewPing sonar2(TRIGGER_PIN2, ECHO_PIN2, MAX_DISTANCE2); Servo hands; void setup() { Serial.begin(115200); // Open serial monitor at 115200 baud to see ping results. hands.attach(HANDSPIN); } void loop() { int dataNum = 5; int distance1[dataNum]; int distance2[dataNum]; //Collect some data from each sensor for(int i = 0;i<dataNum;i++){ delay(20); distance1[i] = sonar1.ping_cm(); distance2[i] = sonar2.ping_cm(); } //Get rid of the highest and lowest data for each sensor //and get the average of the remaining data int distanceSum1 = 0; int distanceSum2 = 0; int distancemax1 = -1; int distancemax2 = -1; int distancemin1 = 10000; int distancemin2 = 10000; for(int i = 0; i<dataNum;i++){ distanceSum1 = distanceSum1 + distance1[i]; distanceSum2 = distanceSum2 + distance2[i]; if(distance1[i]<distancemin1){ distancemin1 = distance1[i]; } if(distance2[i]<distancemin2){ distancemin2 = distance2[i]; } if(distance1[i]>distancemax1){ distancemax1 = distance1[i]; } if(distance2[i]>distancemax2){ distancemax2 = distance2[i]; } } double distanceAvg1 = (double)(distanceSum1 - distancemin1 - distancemax1)/(double)(dataNum-2); double distanceAvg2 = (double)(distanceSum2 - distancemin2 - distancemax2)/(double)(dataNum-2); //Print out the data Serial.print("Ping1: "); Serial.print(distanceAvg1); // Send ping, get distance in cm and print result (0 = outside set distance range) Serial.println("cm"); Serial.print("Ping2: "); Serial.print(distanceAvg2); // Send ping, get distance in cm and print result (0 = outside set distance range) Serial.println("cm"); //If something approaches sensor to a certain distance, //the motor would be turned if(distanceAvg1<= TOOCLOSE){ if(isFirstHand){ isFirstHand = false; hands.write(0); delay(500); } } if(distanceAvg2<=TOOCLOSE){ if(!isFirstHand){ isFirstHand = true; hands.write(80); delay(500); } } }
Discussion
. So wiring the circuit was rather quick and simple. We predicted that writing the code would be easy as well, however, it was more complex than we anticipated because of the unreliability of the ultrasonic sensors. The sensors would read erratic distances so we had to implement a filtering function so that the distances the Arduino was receiving were more accurate. Essentially, we took the median of every five distance readings and this helped the accuracy of the ultrasonic sensors.
Another process that turned out to be more difficult than we thought it would be was the fabrication of the final setup. Designing the cube so that the servo and sensors would be in the right locations required a lot of measuring and relatively tight tolerances. The process of laser cutting the wood was also more difficult than it should have been because the laser cutter we first used didn’t perform reliably to our inputs.
From doing this project we learned that we can’t assume everything we use is going to function perfectly. The ultrasonic sensors’ unreliability and the malfunctioning laser cutter added a lot of complications that we didn’t originally account for. If we were to do the project again, we would probably add a feature to the box that hid the ultrasonic sensors to increase the element of surprise. Or we might even have considered different sensors that could operate from inside the cube. There are some other small things that could have be done to increase the surprise factor so if we had spent less time in the idea generation phase and more time in making the actual project then we could have made a more surprising device. One thing we could have done to trick the user is add a phony functionality that the user could see but it didn’t actually do anything. For example, we could have put the cube on wheels so the user would think that the cube would move at some point but we would keep the motors completely passive. We had a lot of fun doing this project and it made us realize all the devices you can create with relatively simple circuits and hardware.
Leave a Reply
You must be logged in to post a comment.