Short description:
This assistive device reminds the user to drink water frequently, with the reminder frequency changing depending on the outside temperature.
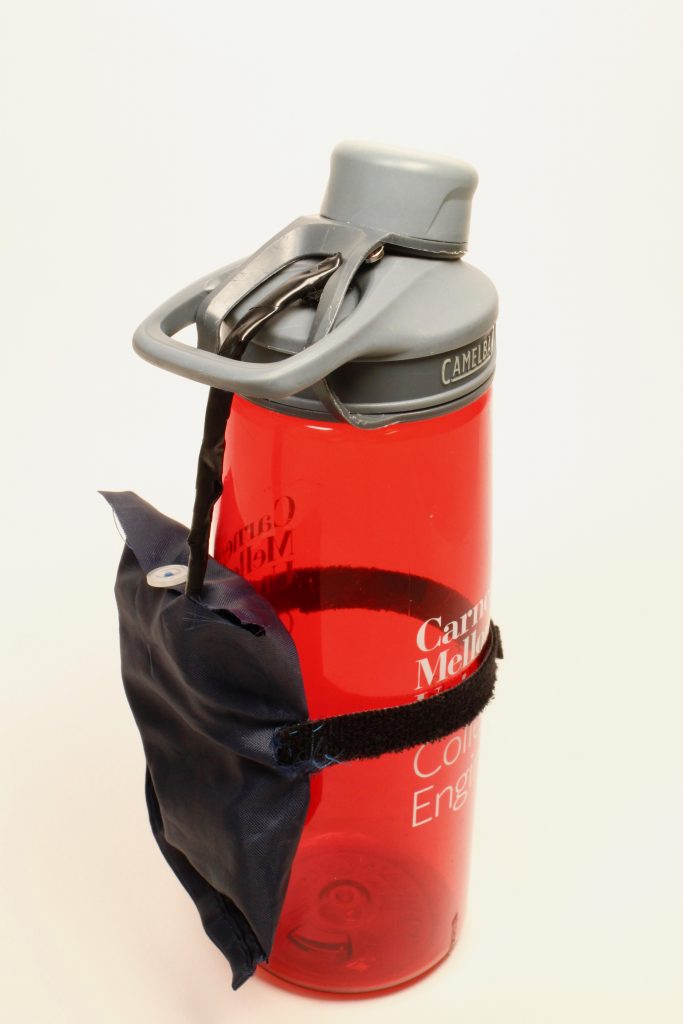
Water bottle with hydration reminder in pouch attached with velcro strip across the middle
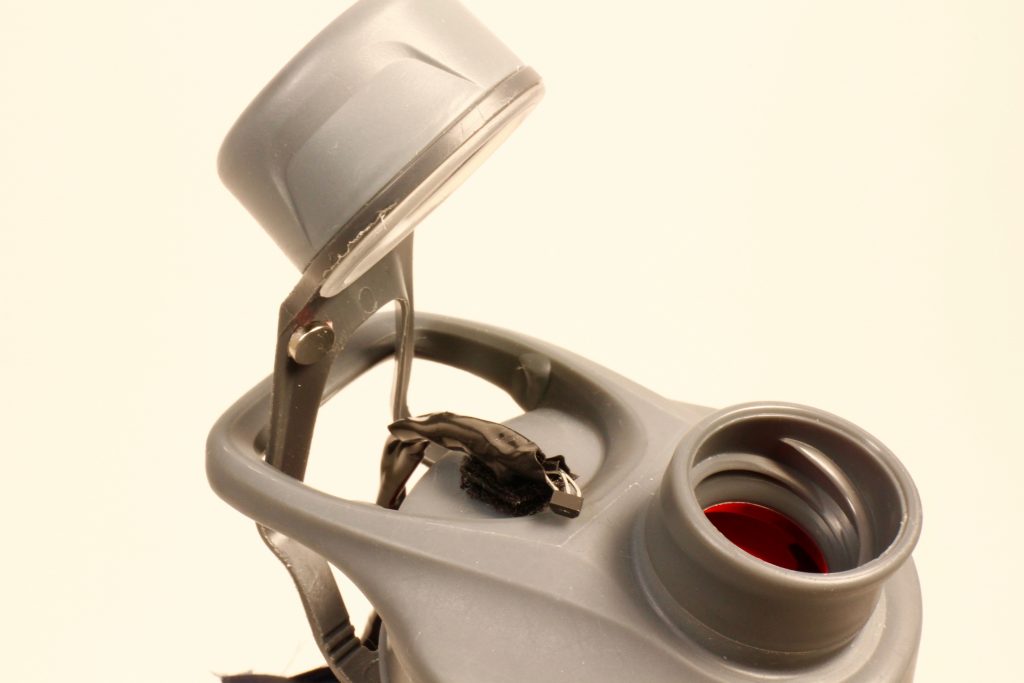
Close up picture to show that the magnet is not detected by the hall effect sensor when the lid is open
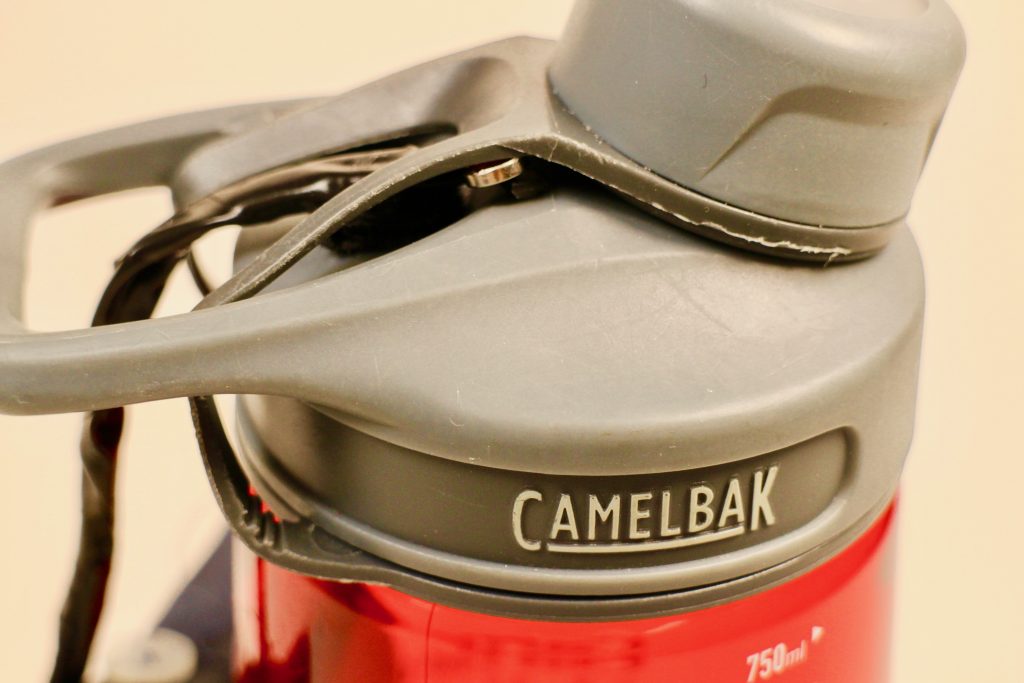
Close up picture to show that that when the lid is closed, the magnet will be detected by the hall effect sensor
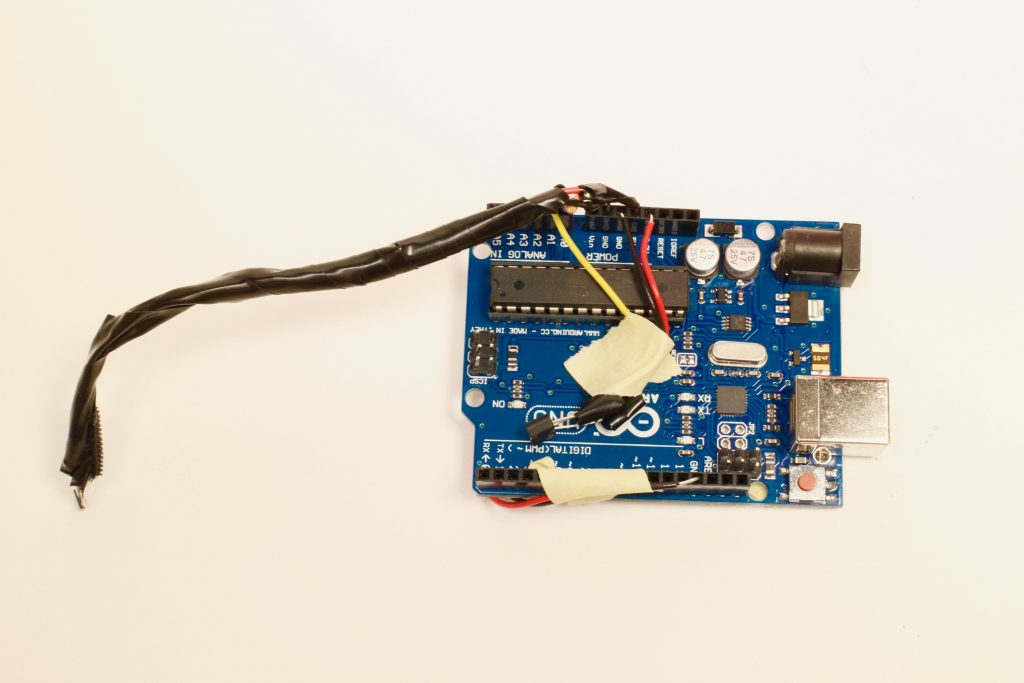
Electronics inside the pouch
Process:
When working on my project, I had two major decision points I made.
One of them was during the initial brainstorming process, when I was trying to decide how I would allow the Arduino to identify when the user was drinking water or not. I initially had the idea of using an accelerometer, so drinking process would be defined as the tilting of the water bottle. However, considering the context of a water bottle being carried around in the user’s bag, I decided that this was an unnecessary feature. Additionally, I would still require a mechanism to indicate whether the water bottle lid is open and the user is actually drinking water. Therefore, I decided to go with the simpler option, where a hall effect sensor and a magnet attached to the lid would be the indicator for whether the user has opened the lid and is drinking water.
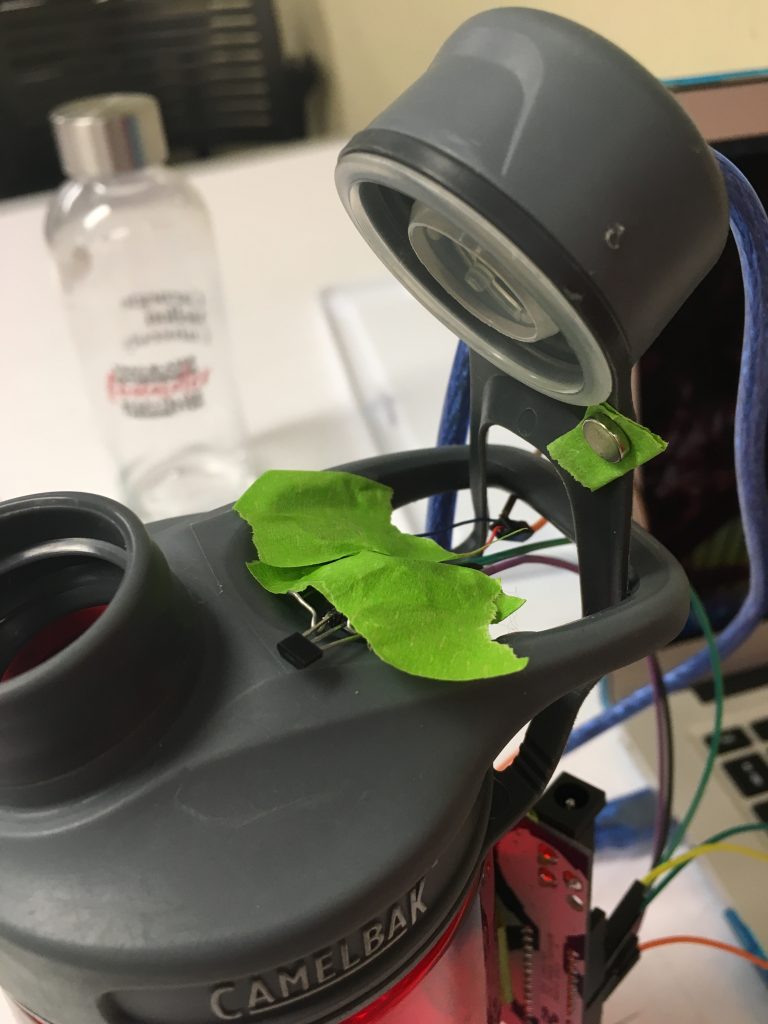
Picture to show when I was trying to attach the hall effect sensor and the magnet to the water bottle. Exact positioning was crucial to ensure interaction between the magnet and the sensor.
Another pivot point I encountered was when I was trying to attach all the electronics to the water bottle, when I realized that with the Arduino, 9V battery for power, and the breadboard with all sensors wired in, it was becoming too bulky for the user to carry around. Moreover, none of the electronics were waterproof, and would be problematic if there was water dripping down the side of the bottle. Based on these observations, I decided to first of all remove the breadboard, soldering the wires to the sensors and wiring them directly into the Arduino pins. This allowed me to decrease the amount of space that the appliances occupy significantly. I also made the decision to sew together a nylon pouch to contain the electronics, for aesthetic reasons, and since nylon is water resistant. With the 9V battery and the velcro strips attached, this allows the user to use the device for any water bottle of their liking.
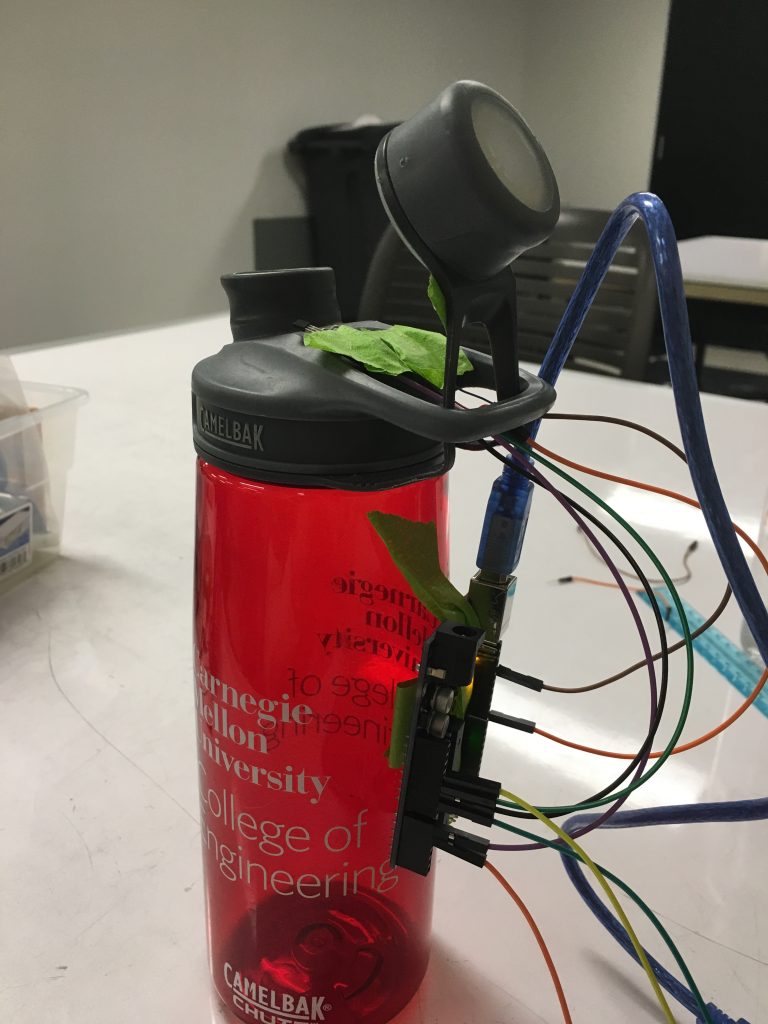
Picture to show exposed electronics on the water bottle. This would have been problematic since water may be dripping down the side, and would be harder for the user to hold.
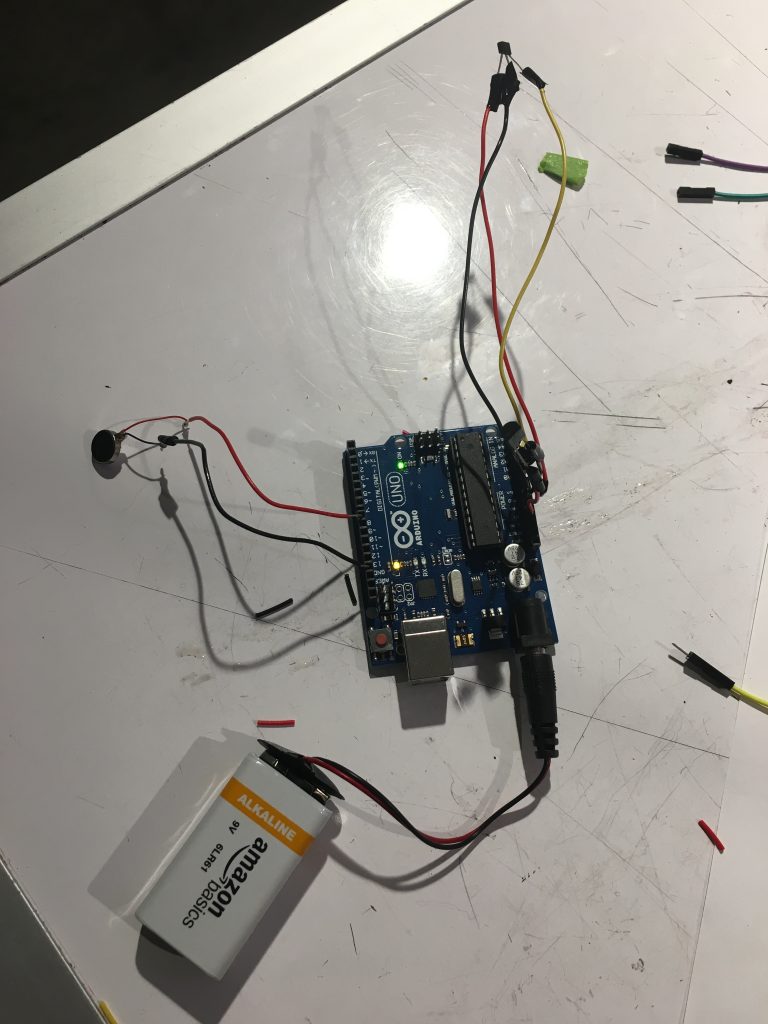
Picture to show how the electronics were wired after removing the breadboard and soldering everything together.
Discussion:
In terms of satisfying the goal of creating an assistive device for someone, I think I was able to succeed. Hydration is an action that is so vital for our well-being, but many people tend to ignore its importance. By creating a device to assist better health, especially for people living in hotter climates, I believe I was able to achieve the goal of assisting someone’s life. However, I believe the technical performance of the device could be further improved, considering how simple the mechanism is. This was what surprised me when working on the project. On testing day, the hall effect sensor fired up like crazy without a magnet in near proximity because the resistor between the power and the output broke off. It reminded me that even one resistor could affect how the device works, as the sensors are sensitive objects. Also I found difficulties positioning the hall effect sensor near the lid and the magnet on the lid, so the device would operate with a high reliability.
One thing I did not realize until later on was the complication related to soldering. As mentioned earlier in my second pivot point, I wanted to have everything of my device contained in the pouch, so the user can easily attach and detach the device. In order to do this, I got rid of the breadboard and soldered all resistors onto the wires and the sensors together. However, the joints would become weak, susceptible to outside forces, with a higher chance of the wires snapping. Also, the chance of the power/output/ground wires touching increased since the wires were exposed, so I had to put electrical tape around every metal part.
Through this project, I learnt that coding and debugging was not too big of an issue, as the concept of tracking the time using millis() and different states, was very similar to the problems we attempted in class. However, I would like to work on improving the aesthetics of my project, as a clean exterior would ensure that the mechanism is more user friendly, and possibly reduce complications related to exposed electronics.
This also applies to the following steps I would like to take if I were to improve this project further. First of all, I would like to explore different mechanisms for detecting when the water bottle is open or not, so I can have all electronics contained in minimum space. This ensures an improved exterior for both aesthetics and usability. I would also want to look at different soldering techniques to make sure that the wires will be reliably intact even without the breadboard.
Technical Information
Schematic – Drawn using Fritzing
Code
unsigned long lastOpened = 0; unsigned long threshold = 0; const unsigned long thresh1 = 30ul * 60ul * 1000ul; const unsigned long thresh2 = 60ul * 60ul * 1000ul; const unsigned long thresh3 = 90ul * 60ul * 1000ul; int MAGNETPIN = A0; int TEMPPIN = A1; int BUZZERPIN = 8; int lidState = 1; //0 is closed, 1 is open int lastLidState = 0; bool buzzerState = LOW; void setup() { pinMode(MAGNETPIN, INPUT); pinMode(TEMPPIN, INPUT); pinMode(BUZZERPIN, OUTPUT); Serial.begin(9600); } void loop() { int lidState = digitalRead(MAGNETPIN); float tempv = analogRead(TEMPPIN); float temp = (tempv*5000/1024-500)/10; //in celcius //set different threshold for time closed depending on outside temp if (temp >= 30){ threshold = thresh1; } else if (temp >= 20 && temp < 30){ threshold = thresh2; } else if (temp < 20){ threshold = thresh3; } if (lidState == 0){ //water bottle is closed if (lidState != lastLidState){ //just closed lastOpened = millis(); } if ((millis() - lastOpened) > threshold){ //Water bottle has not been opened for too long buzzerState = HIGH; } } else if (lidState == 1){ //water bottle is open buzzerState = LOW; } digitalWrite(BUZZERPIN, buzzerState); lastLidState = lidState; }
Leave a Reply
You must be logged in to post a comment.