This tool detects & rewards note-taking, which also functions to mitigate binge snacking through a timed snack dispenser.

Overall View

The inside of the device. A motor controls a door that releases snacks from the top compartment. The sensor, arduino, and all the wiring are within the inside of the box.

The two buttons indicate two modes for the device – snack mode or study mode. The hole behind the box offers two options of connection to a computer for power, or connection to a power outlet.
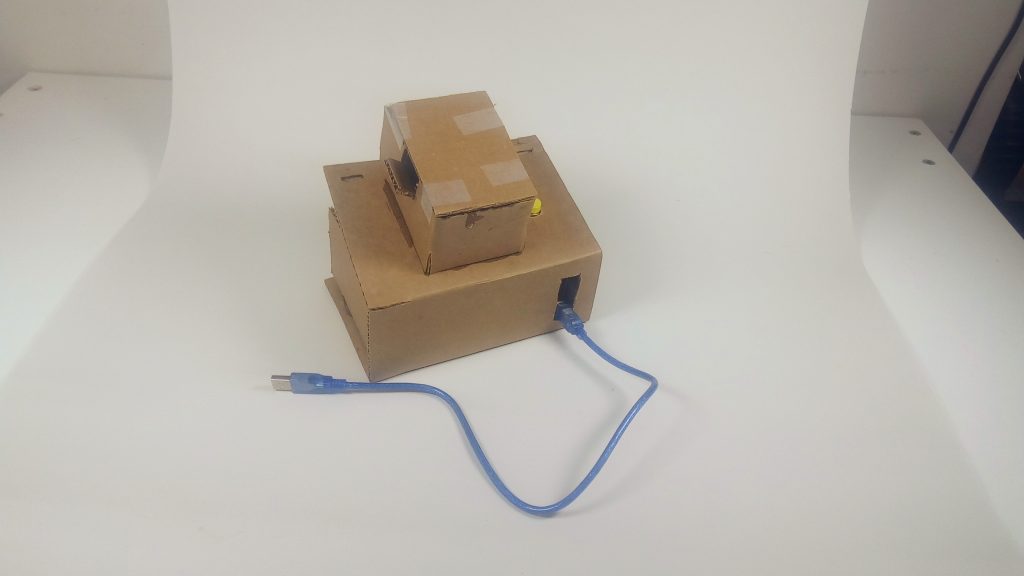
This image displays the slot which is used to add snacks into the top compartment, which is positioned above a trap door.
The design process for the device began with the idea that my attention is often limited when studying, and that a reward or alarm system might be helpful. I was stuck for a long time, deliberating on whether I needed alarms to sound when I wasn’t studying, which sensors to use, and also if serial input would be critical.
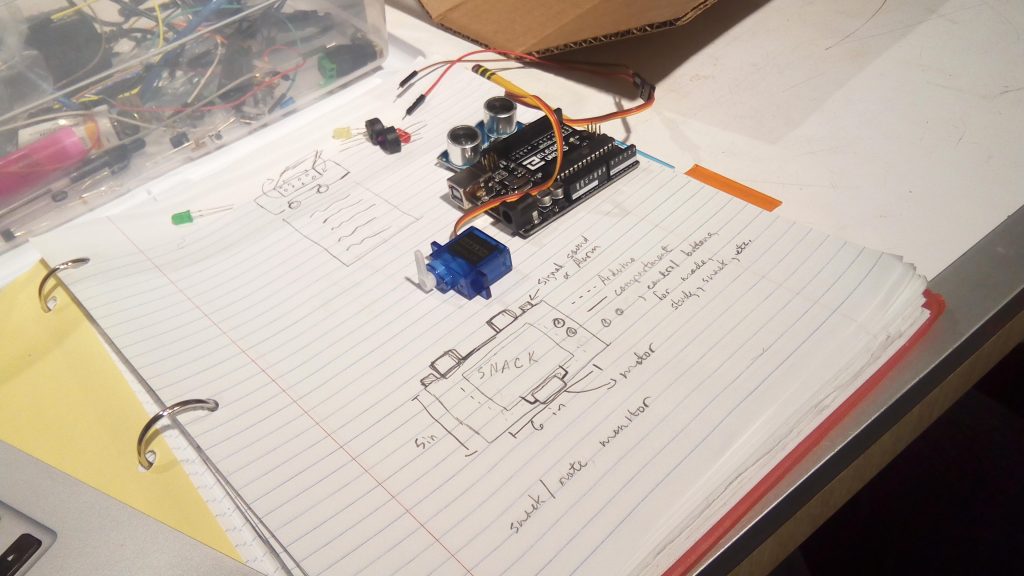
My initial collection of items, and one of multiple sketches involved.
I decided to focus on making a minimum viable product, because I suffered from analysis paralysis. I decided to stop considering buzzers and lights until I was confident about the organization of my device. This is one reason why my device is made of cardboard – I needed room to experiment.
Surprisingly, it took significant consideration to decide where I wanted the trap door and snack compartment to be.
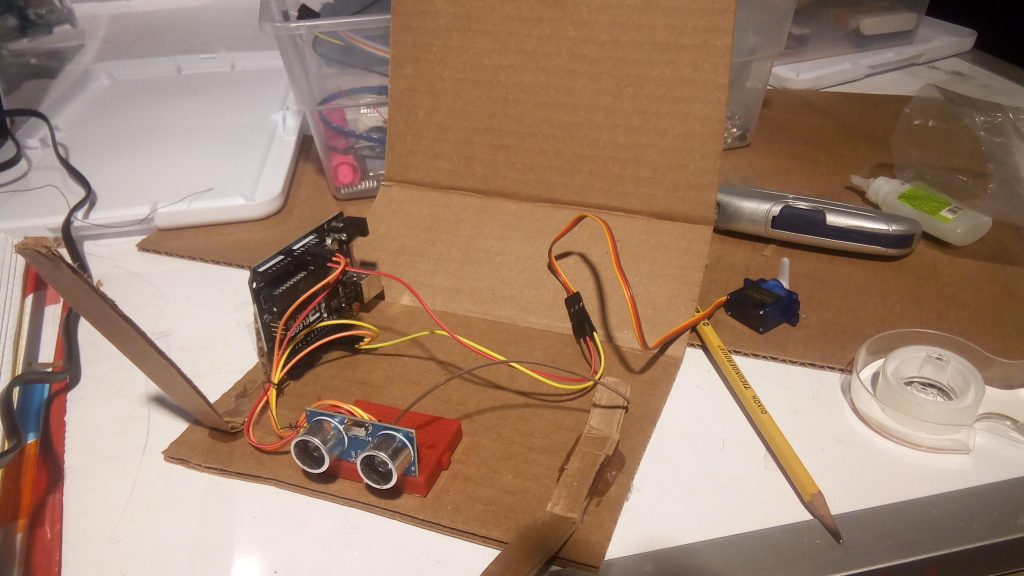
The sensor and the motor were the most essential parts of my device, so I assumed everything would naturally grow from this initial organization.
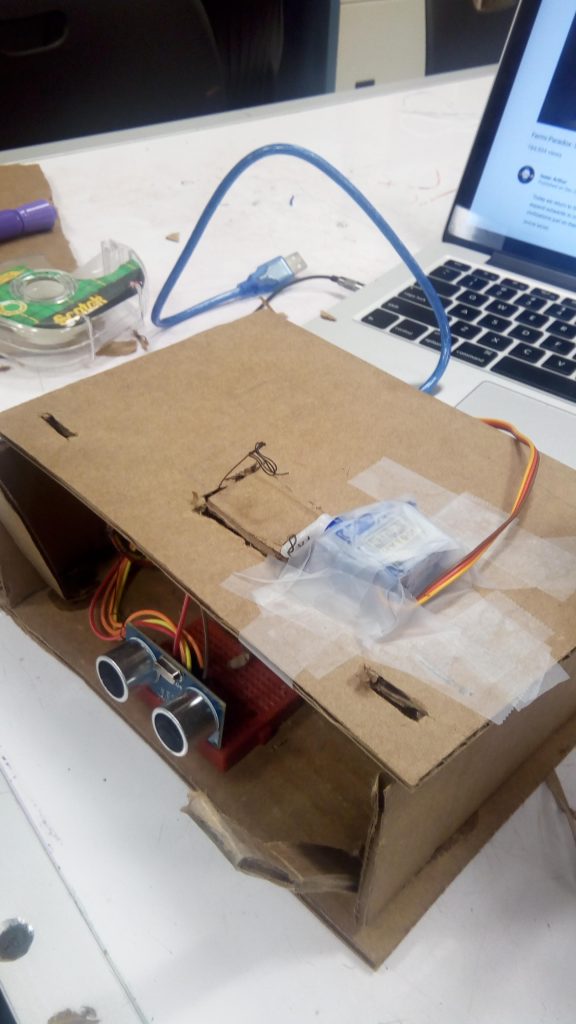
This image shows some of my experimentation with motor position; I realized that placing the motor below was much more convenient.
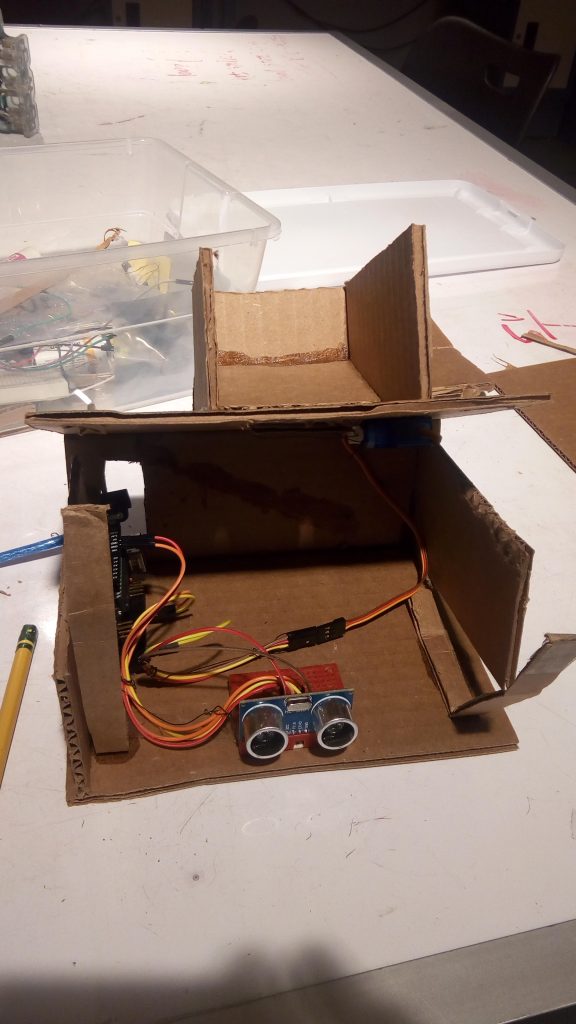
This image depicts part process of deciding on placement for an internal ramp.
As far as my project goes, I am not a fan of its aesthetic. It looks too shabby, but it reflects the indecision I had during the process of design. The sensor works accurately, and I am very satisfied of the sensation of the buttons – figuring out how to solder them, attach them to the roof, and wire them to the Arduino was a process. More planning and foresight would have allowed me to focus on expanding functionality, after a basic final design was settled on.
In my project, I expected some inaccuracy from the ultrasonic sensor. Initially, I thought that this was the case, but then it turned out that two wires were in the wrong location. Everything was generally as expected. However, positioning the movements of the motor was tricky. I had to test the angles at which it moved and stopped. The movements of the motor also disturbed the structure of my device as I was testing it, which made the process more vexing. The two strap-like structures were made so that I could easily open and close the device, so that I had an intuitive understanding of how to position everything within.
I mentioned at an earlier paragraph that my design was shoddy. Right now, I lack laser-cutting experience, and I may have been able to make a more appealing design with laser cut pieces. Cardboard should stick to prototyping, but with creativity, I could have made the cardboard more pleasing to the eye. Ultimately, my indecision about how I wanted the project to turn out was a significant limiting factor.
If I were to build a second iteration of the project, I would focus on laser cut pieces, or more elegant cardboard design. I’d get rid of the upper compartment, to create something visually simple. Concerns about wiring, and putting the project together caused me to use the upper compartment as a quick solution.. An ideal version would have an actual hinge for the trap door, less tape, and possibly a battery pack. Adding peizo buzzers as alarms to indicate laziness would also be in a second iteration.
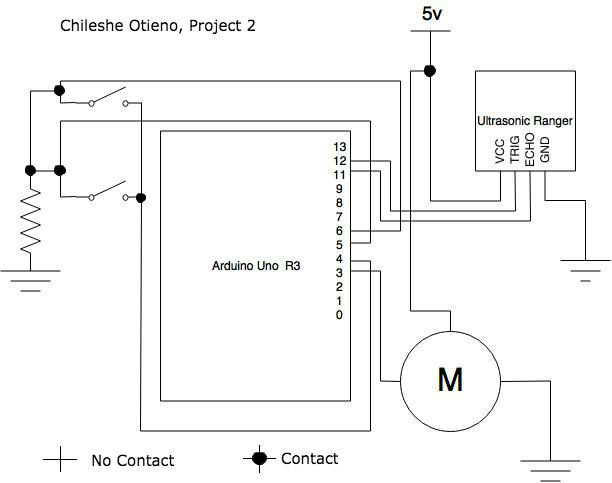
Schematic
//author: Chileshe Otieno //references: Robert Zacharias, 60-223 Physical Computing #include NewPing.h; #include Servo.h; // this allows you to use the Servo library Servo myLittleMotor; // servo name int servoPin = 3; // pin the servo data line is plugged into #define TRIGGER_PIN 12 // Arduino pin tied to trigger pin on the ultrasonic sensor. #define ECHO_PIN 11 // Arduino pin tied to echo pin on the ultrasonic sensor. #define MAX_DISTANCE 100 // Maximum distance we want to ping for (in centimeters). Maximum sensor distance is rated at 400-500cm. #define LIGHT 9 //Never used this, spaghetti code for potential light signal int power = 4; // powers buttons int yellowButton = 5; // Arduino pin connected to signal yellow button click int blueButton = 6; //Arduino pin connected to signal blue button click int studyDistance = 30; // number of cm used as a guide for studying activity area long lastActivity = 0; // amount of time since last movement long candyTimer = 5; //time between candy releases long lastTimerValue = 0; //num seconds counted int activeDuration = 5; bool study_mode = false; bool snack_mode = false; long distanceTimer = 0; //measures last time since significant motion NewPing sonar(TRIGGER_PIN, ECHO_PIN, MAX_DISTANCE); // NewPing setup of pins and maximum distance. void setup() { Serial.begin(115200); // Open serial monitor at 115200 baud to see ping results. myLittleMotor.attach(servoPin); // set up the servo on that data pin snackTime(); pinMode(power, OUTPUT); pinMode(yellowButton, INPUT); pinMode(blueButton, INPUT); pinMode(LIGHT, OUTPUT); } void loop() { // Wait 50ms between pings (about 20 pings/sec). 29ms should be the shortest delay between pings. /* * delay(50); *Serial.print("Ping: "); Serial.print(sonar.ping_cm()); // Send ping, get distance in cm and print result (0 = outside set distance range) Serial.println("cm");*/ digitalWrite(power, HIGH); if ((digitalRead(yellowButton) == HIGH) && (digitalRead(blueButton) == LOW)) { Serial.println("We are studying"); study_mode = true; } else if ((digitalRead(yellowButton)) == LOW && (digitalRead(blueButton) == HIGH)) { snack_mode = true; } if (study_mode == true) { studyMode(); } if (snack_mode == true) { Serial.println("Snack Time"); snackMode(); } } void snackTime() { myLittleMotor.write(70); delay(400); myLittleMotor.write(270); delay(400); } void studyMode() { int dist = sonar.ping_cm(); if ((millis()-lastTimerValue) > 1000){ //decrements the candy timer release time by seconds if ((millis() - lastActivity) < 1000) { candyTimer -= 1; } lastTimerValue = millis(); } if ((dist < studyDistance) && (dist != 0)) // checks when last activity occurred { lastActivity = millis(); } if ((millis() - lastActivity) > activeDuration*1000) //checks if time since last activity is excessive { //alert digitalWrite(LIGHT, HIGH); Serial.println("You should be studying, dude"); candyTimer = 5; } else if (candyTimer == 0) { digitalWrite(LIGHT, LOW); snackTime(); candyTimer = 5; } } void snackMode() { int dist = sonar.ping_cm(); if ((millis()-lastTimerValue) > 1000){ //decrements the candy timer release time by seconds candyTimer -= 1; lastTimerValue = millis(); } if (candyTimer == 0) { snackTime(); candyTimer = 5; } }
Leave a Reply
You must be logged in to post a comment.