Goals:
A problem I have in my everyday life is dealing with the inconvenience of my laundry situation; I live on the third floor and the washer and dryer are in the basement. I often find myself making multiple trips up and down the stairs only to find the laundry isn’t done, or I’ve forgotten to grab quarters. My aim with this project was to address both of these issues.
Solution:
This project gives me a way to both store and keep track of how many quarters I have, and to monitor how long there is left in the laundry cycle. The device can display your current balance in quarters, has an illuminated progress bar to indicate how much time is left on the laundry, and LEDs to show whether you’re timing washing or drying, and to notify you when the timer is done. A sliding switch toggles between washing and drying modes, a button dispenses coins one at a time, and a pair of buttons sets and resets the timer.
Materials:
- Arduino Uno
- Laser cut wood housing
- 3x 8-way MUX
- 4-digit 7-segment display
- 10-bar LED bank
- 3x LED
- 3x push button
- Bump switch
- Sliding Potentiometer
- 4x 6.4v Zener diodes
- 5x 330 ohm resistors
- 4x 10k ohm resistors
- Servo motor
Challenges:
I faced many challenges in the design and building of this device. The primary challenges being driving 3 7-segment digits, and finding an element way to dispense the coins. The chief barrier that prevented the device from being ready on time though was the electrical complexity that arose from designing the solutions to the aforementioned problems.
The solution to the display problem went through several iterations before arriving at what I think is the best way to do it. First, the idea was to use zener diodes and a lot of muxes to bounce back and forth between all the necessary inputs. Here’s an early schematic of this design:
The obvious problem with this design I found early on was it became very, very complex very fast. The design pictured only had 1/8 of the necessary functionality and was already hugely complex.
A second iteration used fewer muxes, and had worked off of the idea that only certain digits needed to be displayed at certain times and I could bounce back and forth between them quickly in software. But the hardware execution of it ended up using upwards of a dozen zener diodes and the wiring would have been almost impossible to get right on a breadboard. Also visible in the diagram below is the table used to determine the mux outputs.
The third, and final, iteration of the design was a radical departure and put much of the burden in the software to deal with the control. It uses a system of 3 muxes which drive both the LED bank, and the 7-segment display. The idea is that depending on what digits need to be displayed, the software will drive one segment, of one digit at a time, and rapidly bounce between them. The first mux (top in the schematic) is used to run the LED bank, and its lower 2 outputs are the selector bits for the digit selecting mux (bottom). The middle mux is used to select which segment is being driven in a given digit.
Schematic:
Notes:
- All resistors not labeled are 330 ohms
- The outputs of the middle mux are labeled corresponding to their connection to the display for clarity’s sake
Progress Images:
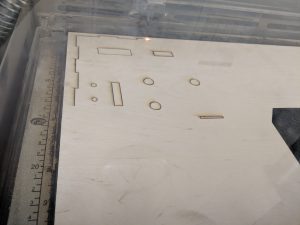
laser cutting the housing
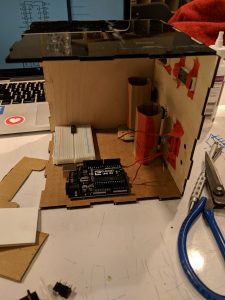
initial plans for coin dispenser
Final Thoughts + code:
I think that for such a simple concept, I really underestimated the complexity of the solution. The biggest problem for me, wasn’t necessarily coming up with a design to solve it, but actual implementation of such a complex circuit was challenging. I spend hours and hours soldering, and redoing connections on the breadboard, and doing it all in such a small space too. Tackling a coin dispenser or digital timer would’ve been enough of a challenge, and trying to do both I ended up getting neither working.
I waited to post the code at the bottom because of its length. Something to note about it are the case statements which is how I cycle quickly based on the loop cycle to drive each separate segment.
Code:
const int coinSwitch = 2; const int dispPin = 3; const int startPin = A3; const int resetPin = A4; const int slider = A5; const int washLED = 4; const int dryLED = 5; const int alarmLED = 6; const int clockMuxA = 7; const int clockMuxB = 8; const int clockMuxC = 9; const int barMuxA = 10; const int barMuxB = 12; const int barMuxC = 13; const int servoPin = 11; /*####### GLOBALS ###### */ int coinCount = 0; long timer = 0; bool counting = true; bool wash = true; const long wash_time = 1800000; const long dry_time = 2 * wash_time; bool prevDisp = false; bool currDisp; bool prevStart = false; bool currStart; bool prevReset = false; bool currReset; bool prevCoin = false; bool currCoin; void writeBars(){ if (!counting) return; if (timer <= 0){ digitalWrite(alarmLED,HIGH); } else { timer--; } int frac = wash ? (timer/wash_time) : (timer/dry_time); int numBars = frac * 5; //selecting on MUX which LEDs to light up switch (numBars) { case 0: digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,LOW); digitalWrite(barMuxC,HIGH); break; case 1: digitalWrite(barMuxA,LOW); digitalWrite(barMuxB,LOW); digitalWrite(barMuxC,LOW); break; case 2: digitalWrite(barMuxA,LOW); digitalWrite(barMuxB,LOW); digitalWrite(barMuxC,HIGH); break; case 3: digitalWrite(barMuxA,LOW); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,LOW); break; case 4: digitalWrite(barMuxA,LOW); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,HIGH); break; case 5: digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,LOW); digitalWrite(barMuxC,LOW); break; } } long loopCounter = 0; void writeCount(){ loopCounter++; int quarters = coinCount % 4; long oneMod = loopCounter % 4; int twoMod = loopCounter % 3; int threeMod = loopCounter % 5; int thisMod = loopCounter % 2; switch (quarters){ case 0: digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,HIGH); digitalWrite(clockMuxC,LOW); if (loopCounter%2 == 0){ digitalWrite(barMuxA,LOW); digitalWrite(barMuxB,LOW); digitalWrite(barMuxC,LOW); } else { digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,HIGH); } break; case 1: if (oneMod == 0){ digitalWrite(clockMuxA,LOW); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,HIGH); digitalWrite(barMuxA,LOW); digitalWrite(barMuxB,LOW); digitalWrite(barMuxC,LOW); } else if (oneMod == 1){ digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,LOW); digitalWrite(barMuxA,LOW); digitalWrite(barMuxB,LOW); digitalWrite(barMuxC,LOW); } else if (oneMod == 2){ digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,HIGH); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,HIGH); } else if (oneMod == 3){ digitalWrite(clockMuxA,LOW); digitalWrite(clockMuxB,HIGH); digitalWrite(clockMuxC,LOW); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,HIGH); } break; case 2: if (twoMod == 0){ digitalWrite(clockMuxA,LOW); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,HIGH); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,HIGH); } else if (twoMod == 1){ digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,LOW); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,HIGH); } else if (twoMod == 2){ digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,HIGH); digitalWrite(clockMuxC,LOW); digitalWrite(barMuxA,LOW); digitalWrite(barMuxB,LOW); digitalWrite(barMuxC,LOW); } break; case 3: if (threeMod == 0){ digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,HIGH); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,HIGH); } else if (threeMod == 1){ digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,LOW); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,HIGH); } else if (threeMod == 2){ digitalWrite(clockMuxA,LOW); digitalWrite(clockMuxB,HIGH); digitalWrite(clockMuxC,HIGH); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,HIGH); } else if (threeMod == 3){ digitalWrite(clockMuxA,LOW); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,HIGH); digitalWrite(barMuxA,LOW); digitalWrite(barMuxB,LOW); digitalWrite(barMuxC,LOW); } else if (threeMod == 4){ digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,LOW); digitalWrite(barMuxA,LOW); digitalWrite(barMuxB,LOW); digitalWrite(barMuxC,LOW); } break; } int dollars = coinCount / 4; switch (dollars) { case 0: digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,HIGH); digitalWrite(clockMuxC,LOW); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,LOW); break; case 1: if (threeMod == 0){ digitalWrite(clockMuxA,LOW); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,LOW); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,LOW); } else if (threeMod == 1){ digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,HIGH); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,LOW); } else if (threeMod == 2){ digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,HIGH); digitalWrite(clockMuxC,LOW); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,LOW); } else if (threeMod == 3){ digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,LOW); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,LOW); } else if (threeMod == 4){ digitalWrite(clockMuxA,LOW); digitalWrite(clockMuxB,HIGH); digitalWrite(clockMuxC,HIGH); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,LOW); } break; case 2: if (thisMod == 0){ digitalWrite(clockMuxA,HIGH); digitalWrite(clockMuxB,LOW); digitalWrite(clockMuxC,HIGH); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,LOW); } else if (thisMod == 1){ digitalWrite(clockMuxA,LOW); digitalWrite(clockMuxB,HIGH); digitalWrite(clockMuxC,LOW); digitalWrite(barMuxA,HIGH); digitalWrite(barMuxB,HIGH); digitalWrite(barMuxC,LOW); } break; } } void setup() { pinMode(coinSwitch,INPUT); pinMode(dispPin,INPUT); pinMode(startPin, INPUT); pinMode(resetPin,INPUT); pinMode(slider,INPUT); pinMode(washLED,OUTPUT); pinMode(dryLED,OUTPUT); pinMode(alarmLED,OUTPUT); pinMode(clockMuxA,OUTPUT); } void loop() { int washdry = analogRead(slider); wash = (washdry < 512); if (wash){ digitalWrite(washLED,HIGH); digitalWrite(dryLED,LOW); } else { digitalWrite(washLED,LOW); digitalWrite(dryLED,HIGH); } //add a coin currCoin = digitalRead(coinSwitch); if (currCoin && !prevCoin){ coinCount++; } //dispense a coin currDisp = digitalRead(dispPin); if (currDisp && !prevDisp){ //move the servo up for a hot sec coinCount--; } //reset the timer currReset = digitalRead(resetPin); if (currReset && !prevReset){ counting = false; timer = wash ? wash_time : dry_time; } //start the timer currStart = digitalRead(startPin); if (currStart && !prevStart){ counting = true; } writeBars(); writeCount(); }
Leave a Reply
You must be logged in to post a comment.