Child Description
This is a skinny black box about a foot tall, with the same shape as a desktop computer. It has a toothy gap down the left side, and is cut out of a glossy, hard material. The top face has a series of copper pads in the shape of a palm and 5 fingers, with green LEDs above each finger. There’s also a gap in the top-center of the top face, through which a snack on wax paper peeks through.
The hand shape of the top invites you to position your hand over it, and the LEDs light up as each “finger” is touched. Multiple lights can be on at the same time. When a specific set of pads are touched simultaneously, fulfilling a code, the LEDs glow sequentially and a small beep sound is emitted from the interior. Then, a motor whirrs and fruit-by-the-foot is dispensed from the top gap.
Reflection
My project had too much thinking in it. Mainly, I remember spending a lot of time agonizing about what a dominant robot would look like so I could design the vending machine around it, as if I were making a specification for it. As an exploratory design, I should have spent less time nailing down what the actual design would be and instead spent more time iterating over a few designs, and seeing what made sense in the context of a machine user. In the future I’d like to make things that require less thinking, which are more hands-on enjoyable projects. I don’t know how they tricked me, a hands-on pesron, into thinking so much without doing.
As a rather absent-minded person, I forget a lot of relevant things I’ve learned over the years. One is that physical construction is hard and takes time, even if you know exactly what you’re doing. Mistakes take half an hour to correct, which isn’t the case for electronics or programming. I expected the electronics to go quickly, and I finished those in about 2 hours. But when it came to the wheel that actually dispensed the fruit-by-the-foot, the mount for the motor and fruit roll took me way longer to think of and build. By the time I finished adjusting the gap between the motor and the fruit roll, the plastic gears in the gearbox had clean stripped off, and the wheel no longer worked under any load. Unfortunately, I had hot-glued everything into place because I expected it to work, which turned out to be a terrible decision since I couldn’t get in and replace the gearbox. I forget this stuff when I haven’t been building in a while, but I have been reminded. I also re-learned how to use the laser cutters, which is great! Can’t wait to make more enclosures!
I spoke with Erin a bit towards the beginning of the project about how I felt like anything I could make was futile. It would all end up in the landfill, from nice clean parts that anyone could use, to hole-ridden sheets of acrylic that would be left for the moss to decay in 4 billion years. Erin said futility and uselessness were fine byproducts of the system we’d build since many of the parts would be recycled, which I had and have trouble agreeing with. In the future I will try to build more practical things, since many such projects I think of can fit y’all’s descriptions, be artistic, and be useful. Obviously that is hard, but we are practicing so I should feel less bad if I fail. And I won’t have to force myself to work on them, which is a major incentive.
In-Process Photos
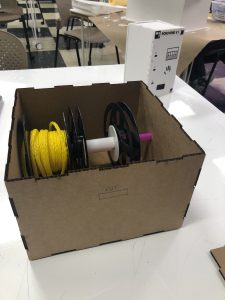
Box prototype for holding reels and dispensing their contents. Maquette in the back
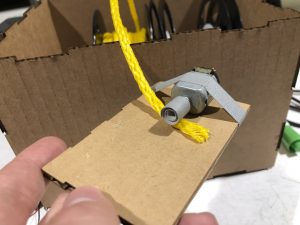
First design of dots dispenser. I tested on string, but that didn’t work really well so I switched it to candy
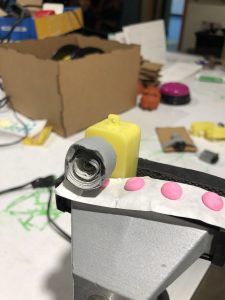
Mounted dots dispenser, loaded up with candy
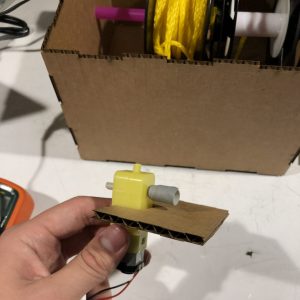
2nd iteration of the dots dispenser. The dots go between the roller and the cardboard
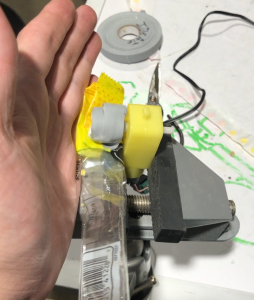
Adding a nub to the wheel allowed better grip to things on the track, so much so that I could pull the flat wrapping of the candy. This also informed my decision to add a track in the final version.
Finished Photos
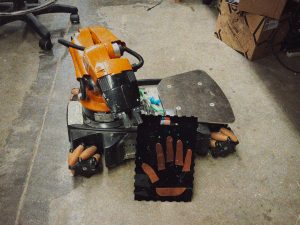
Part of the mythos of the Robovend is its robotic creator, who wanted a vending machine that both humans and factory floor robots could benefit from
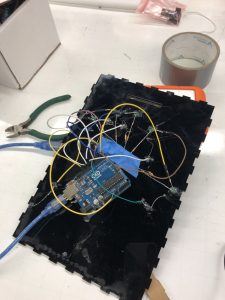
Wiring of the LEDs and touch responsive pads. The pads were connected to the Arduino through a small hole in the top acrylic panel
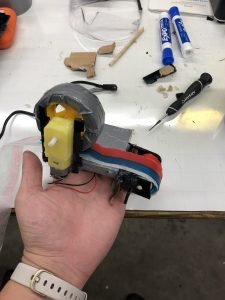
The fruit by the foot dispensing mechanism. This final mechanism worked until the gearbox broke itself
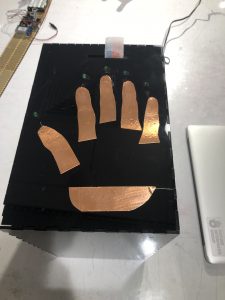
Robovend’s pads are all touch responsive
Video
High Res Version: https://www.youtube.com/watch?v=8N-5hp1FVPY
Code
/* RoboVend - A vending machine for humans and robots * An ideate project by Andy Kong * * This code detects touches on its input pins, turns on LEDs * according to which pads are touched, and recognizes * a preprogrammed code to turn the motor driver on. * * Shoutout to FastTouch, really made my life easier for doing touch detection */ #include <FastTouch.h> int ledpins[] = {2,3,4,5,6}; int touchpins[] = {7,8,9,10,11}; const int numtouchpins = 5; int lastTouches[numtouchpins]; int palmPin = 11; // GND the palm pin int motPin = 13; // Pin that controls the transistor that powers the food dispenser void setup() { Serial.begin(115200); // Set up LED pins for (int i = 0; i < numtouchpins; i++){ pinMode(ledpins[i], OUTPUT); } pinMode(motPin, OUTPUT); pinMode(palmPin, OUTPUT); } // Create capacitive touch threshold for the fastTouchRead() int touchMargin = 1; void loop() { Serial.println("New"); // Read from all touch pads, and trigger their LED if they're past the touch threshold for (int i = 0; i< numtouchpins; i++){ lastTouches[i] = fastTouchRead(touchpins[i]); Serial.print("\t Pin "); Serial.print(i); Serial.print(": "); Serial.print(lastTouches[i]); if (lastTouches[i] >= touchMargin){ digitalWrite(ledpins[i], HIGH); // Debuggery // Serial.println("pin "); // Serial.print(touchpins[i]); // Serial.println(" turning on HIGH"); } else{ digitalWrite(ledpins[i], LOW); if (i == 0) digitalWrite(motPin, LOW); } } // Blink if the combination of pads is correct if (lastTouches[0] >= touchMargin && lastTouches[1] >= touchMargin && lastTouches[2] == 0 && lastTouches[3] == 0 && lastTouches[4] >= touchMargin){ // Turning the motor for food dispenser-y digitalWrite(motPin, HIGH); // Beeping code for (int i = 0; i < numtouchpins; i++){ digitalWrite(ledpins[i], LOW); } delay(50); digitalWrite(motPin, LOW); delay(50); digitalWrite(motPin, HIGH); delay(50); digitalWrite(motPin, LOW); delay(50); digitalWrite(motPin, HIGH); delay(50); digitalWrite(motPin, LOW); // Blinking code for (int i = 0; i < numtouchpins; i++){ if (i != 0){ digitalWrite(ledpins[i-1], LOW); } digitalWrite(ledpins[i], HIGH); delay(100); } digitalWrite(ledpins[numtouchpins-1], LOW); for (int i = 0; i < numtouchpins; i++){ if (i != 0){ digitalWrite(ledpins[i-1], LOW); } digitalWrite(ledpins[i], HIGH); delay(100); } } else { digitalWrite(motPin, LOW); } delay(10); }