this sketch samples pixel colors and draws random quadrilaterals where the pixel was sampled from. mouseX controls the size of the shapes, while pressing the mouse will fill the shapes, rather than using the color as a stroke. press a key to erase the canvas.
below are some earlier variations in which I manually changed the size and fill/stroke boolean, and tested out density using a loop. (this version was not interactive).
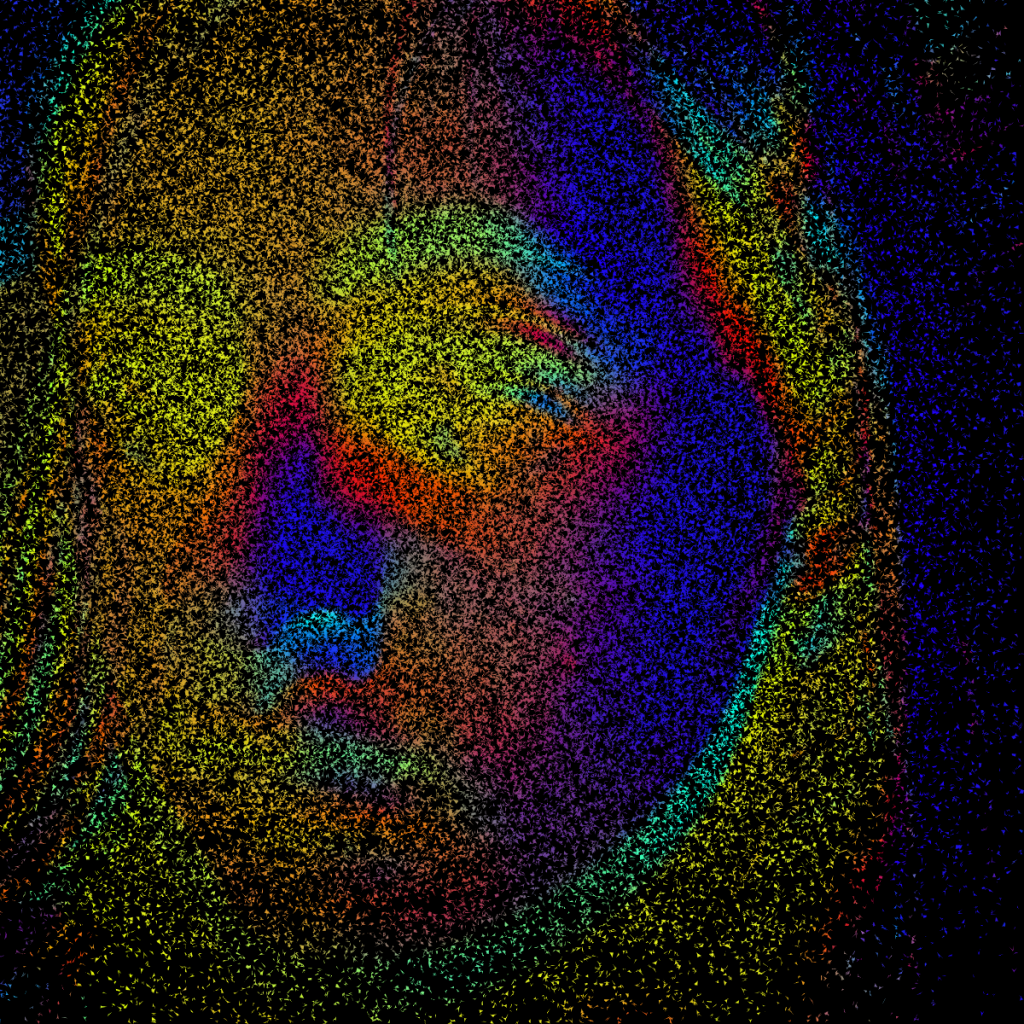
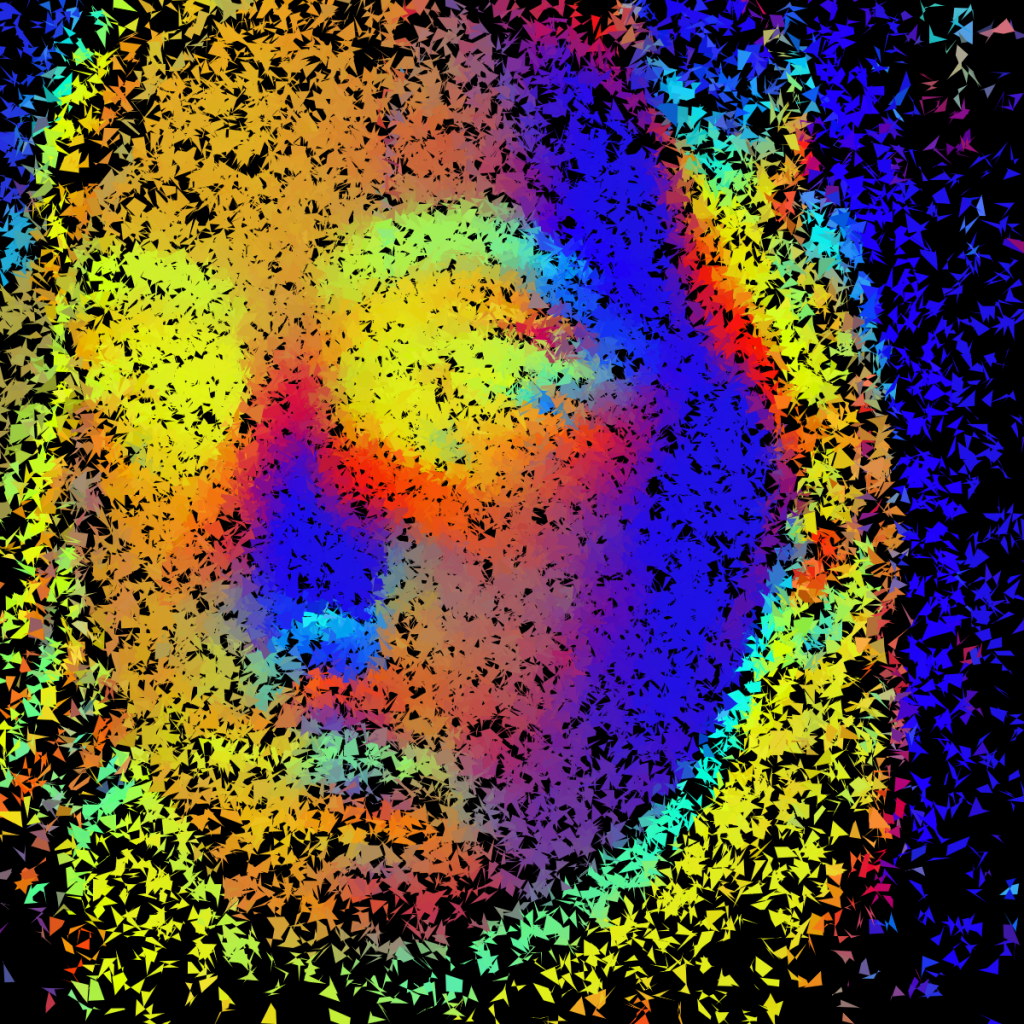
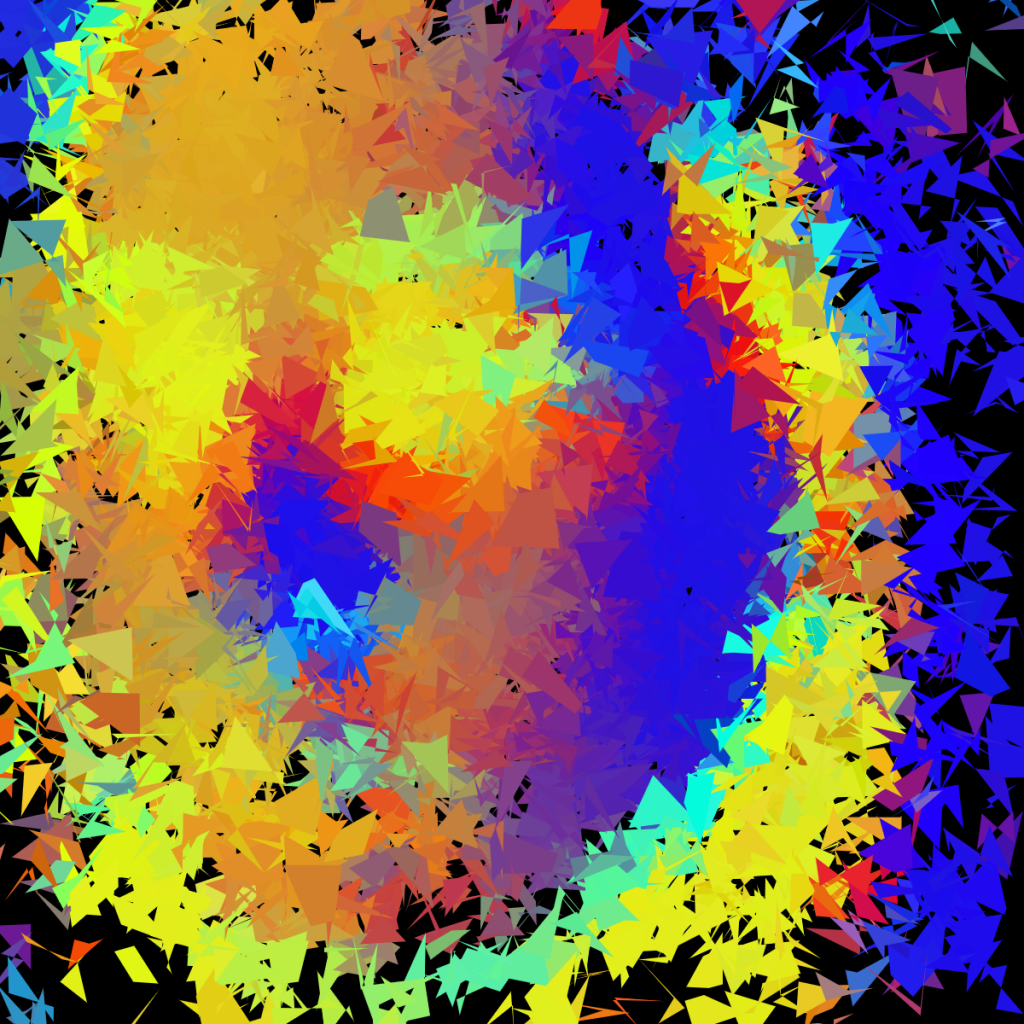
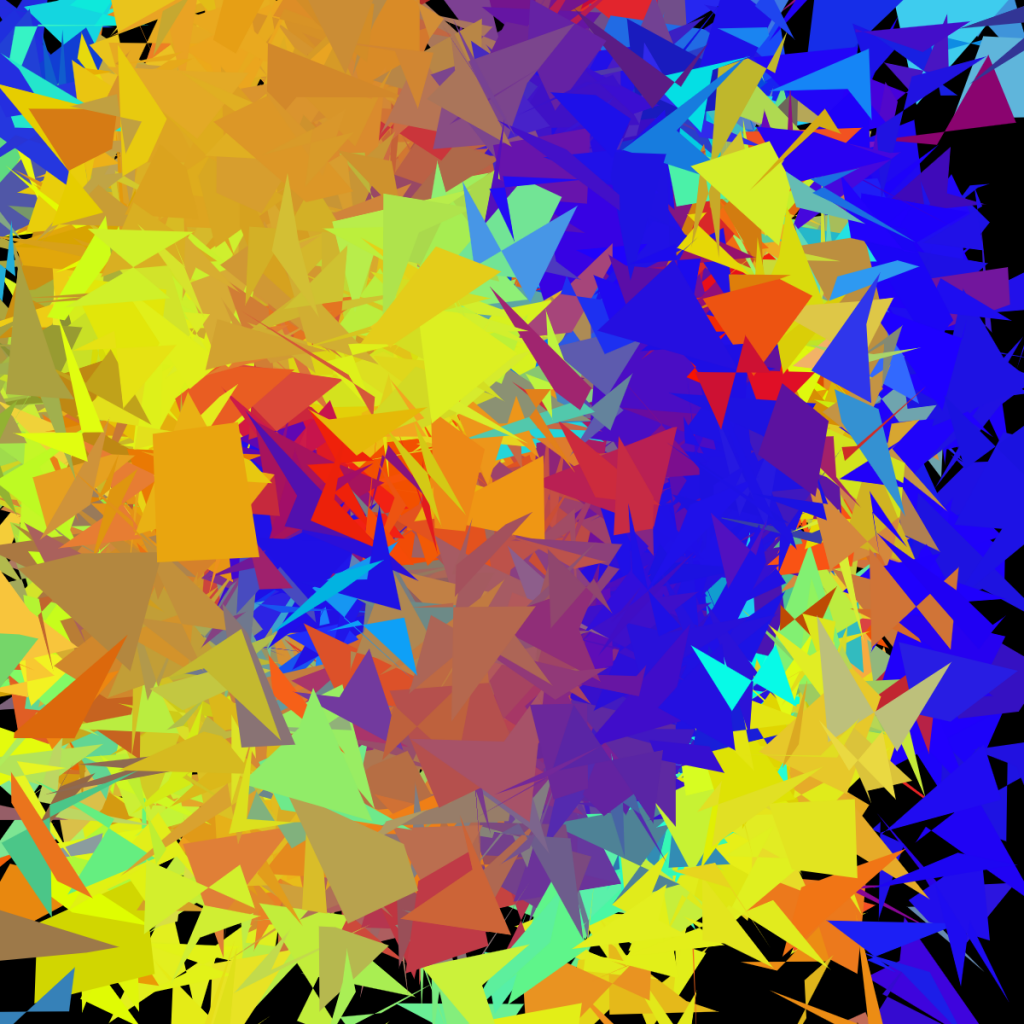
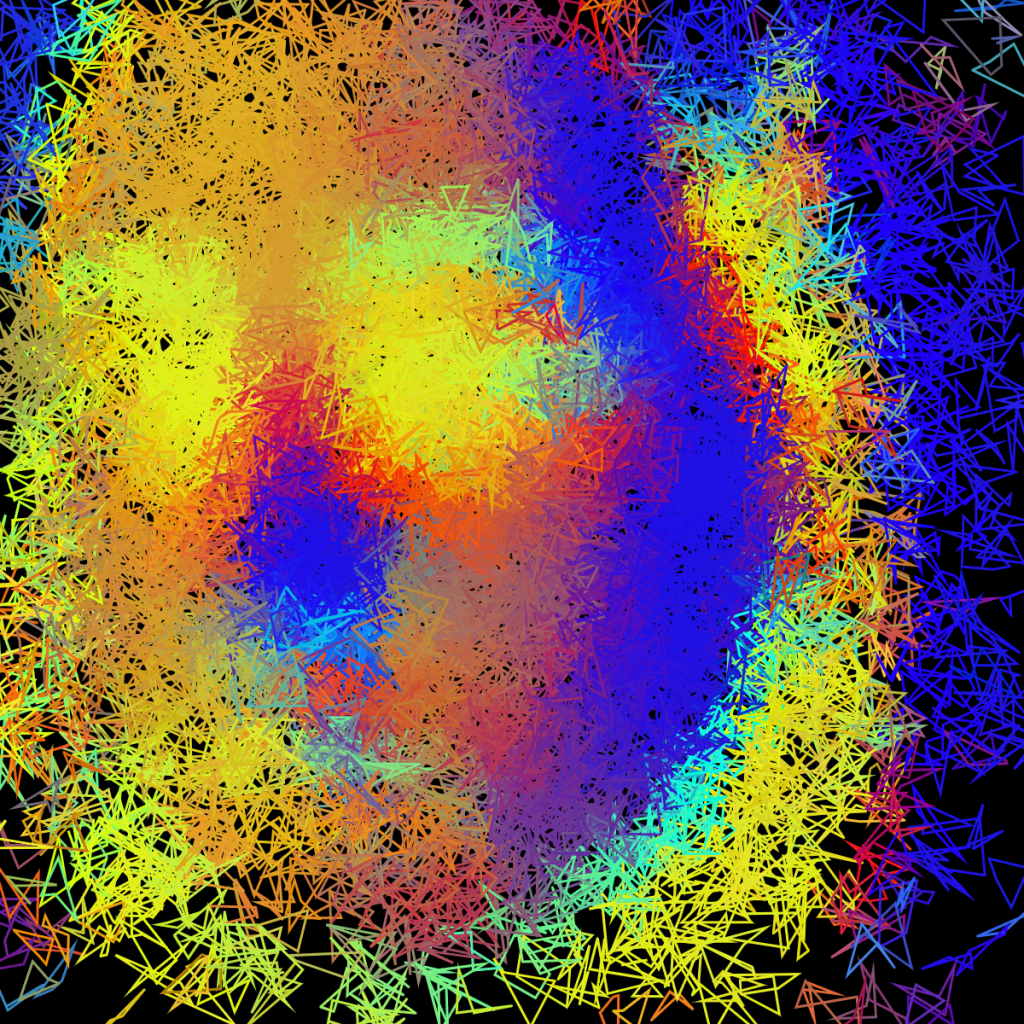
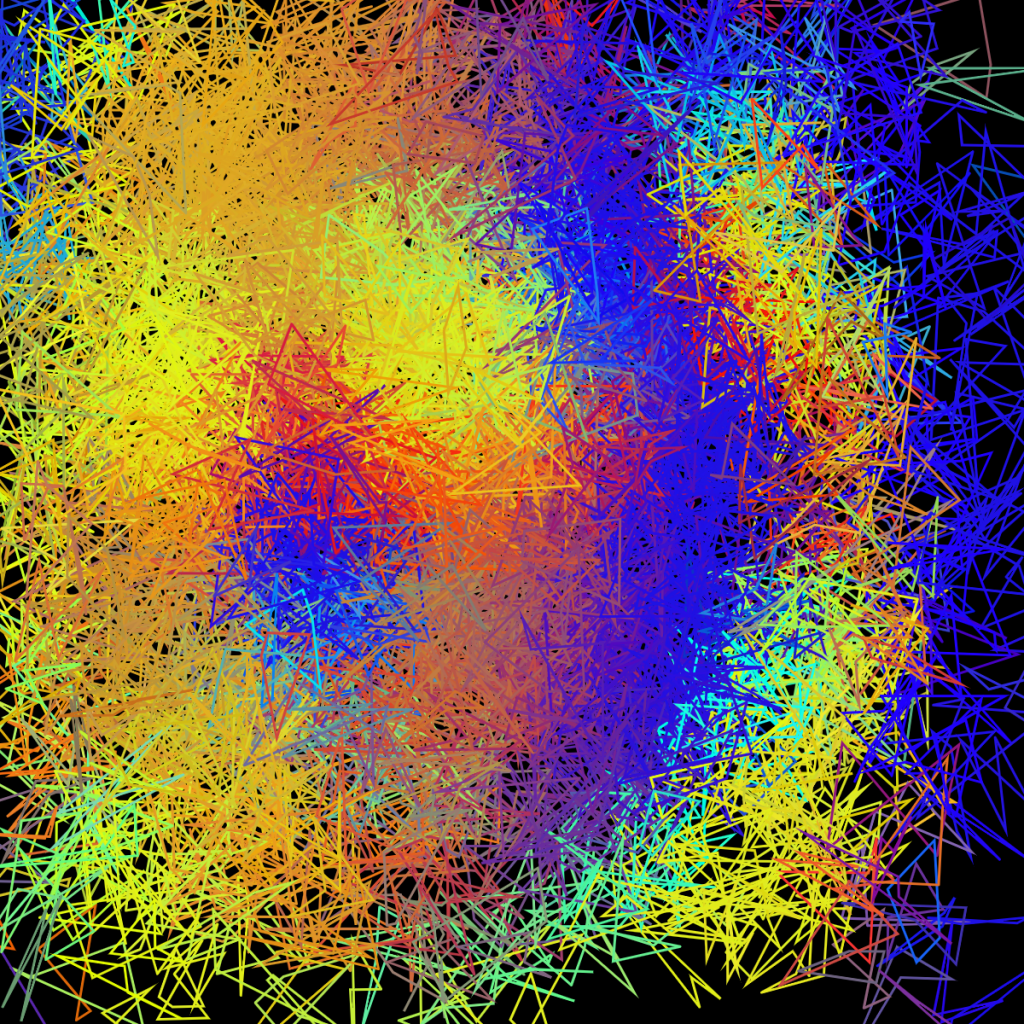
sketch
// jaden luscher
// jluscher@andrew.cmu.edu
// section a
// project 09
// initially i had tried making a chuck close-style portrait with a pixel matrix,
// then i shifted to funky shapes instead. the ineraction was added at the end
// when i kept wanting to tweak the size and fill/stroke modes.
var jaden; // original image
var x1;
var y1;
var dx = 0; // noise step
var dy = 0;
var size; // bounds of shape
var yesFill = false;
function preload() {
jaden = loadImage("https://i.imgur.com/BRUtdb2.png") // original portrait
}
function setup() {
createCanvas(400, 400);
background(0);
frameRate(60);
jaden.loadPixels();
}
function draw() {
size = int(map(constrain(mouseX, 0, width), 0, width, 5, 50));
if (mouseIsPressed) { // toggle fill mode
yesFill = true;
} else { // reset to line drawing mode
yesFill = false;
}
if (keyIsPressed) { // erase drawing
background(0);
}
drawShape();
print(yesFill);
}
function drawShape() {
x1 = int(map(noise(dx), 0, 1, -150, width + 150));
y1 = int(map(noise(dy), 0, 1, -150, height + 150));
dx += random(0.1, 1.0);
dy += random(0.1, 1.0);
if (yesFill == true) {
noStroke();
fill(jaden.get(x1, y1)); // returns pixel from canvas
} else if (yesFill == false) {
strokeWeight(1);
stroke(jaden.get(x1, y1)); // returns pixel from canvas
noFill();
}
beginShape(QUADS); // generate random polygons
vertex(random(-1 * size, size) + x1, random(-1 * size, size) + y1);
vertex(random(-1 * size, size) + x1, random(-1 * size, size) + y1);
vertex(random(-1 * size, size) + x1, random(-1 * size, size) + y1);
vertex(random(-1 * size, size) + x1, random(-1 * size, size) + y1);
endShape();
}