The Sushi Bar Train
For my project I decided to create a restaurant on a train that serves japanese food via a conveyer belt. The dishes include: sashimi, tuna sushi, matcha, miso soup, ramen, and salmon. In the window of the train booth, you can watch as you travel through a bright city at night.
// SRISHTY BHAVSAR
// 15104 SECTION C
//PROJECT 11
var buildings = [];
var fd = [] //
// food items
var ramen; // https://imgur.com/H2R30Wg
var miso; // https://imgur.com/J5EhmuM
var salmon; // https://imgur.com/p6YrdLv
var sashimi; // https://imgur.com/mJjnmVD
var matcha; // https://imgur.com/SErtIMf
var tunasushi; // https://imgur.com/HCUsYNh
var maki; // https://imgur.com/UI5eghR
var food =
["https://i.imgur.com/H2R30Wg.png", "https://i.imgur.com/J5EhmuM.png",
"https://i.imgur.com/p6YrdLv.png", "https://i.imgur.com/mJjnmVD.png",
"https://i.imgur.com/SErtIMf.png" , "https://i.imgur.com/HCUsYNh.png",
"https://i.imgur.com/UI5eghR.png"]; // food contains the images of ramen,miso,salmon,etc.
function preload() {
seats = loadImage("https://i.imgur.com/UEAssld.png");
ramen = loadImage(food[0]); //ramen
miso = loadImage(food[1]); //miso
salmon = loadImage(food[2]); // salmon
sashimi = loadImage(food[3]); //sashimi
matcha = loadImage(food[4]); //matcha
tunasushi = loadImage(food[5]); //tunasushi
maki = loadImage(food[6]); //maki
bg = loadImage("https://i.imgur.com/2zjN6qS.png");
}
function setup() {
createCanvas(480, 480);
background(220);
imageMode(CENTER);
frameRate(17);
// create an initial collection of buildings
for (var i = 0; i < 20; i++) {
var rx = random(width);
buildings[i] = makeBuilding(rx);
}
// collection of dishes
var dist = 0;
for (var i = 0; i <500; i++) {
fd[i] = makeFood(dist);
dist += 150; //distance between dishes
}
print(fd);
}
function draw() {
createCanvas(480, 480);
background(25,25,112);
//buildings
push();
translate(0,-120);
updateAndDisplayBuildings();
removeBuildingsThatHaveSlippedOutOfView();
addNewBuildingsWithSomeRandomProbability();
pop();
//bg
image(bg, 266.4269, 240, 532.8539, 480);
showFood();
//seats
image(seats,240,408.3845,480,143.231);
}
function makeFood(xloc){
var fd = { x: xloc,
speedx: 1.5,
move: foodMove,
food : random([ramen, miso, salmon, sashimi, matcha, tunasushi, maki]),
display: foodDisplay,
}
return fd;
}
function foodDisplay(){
/*the width heights and y location are respective to the
ones mapped out on adobe illustrator. thats why they are typed manually */
//xloc is the speed times 500 and the dist
if (this.food == ramen){
image(ramen,this.x -750*20, 310, 148, 108 ); // ramen
}
if (this.food == miso){
image(miso,this.x -750*20, 310, 119, 115 ); // miso
}
if (this.food == salmon){
image(salmon,this.x -750*20, 318, 174, 126 ); // salmon
}
if (this.food == sashimi){
image(sashimi,this.x -750*20, 309, 203, 147 ); //sashimi
}
if (this.food == matcha){
image(matcha,this.x - 750*20, 324, 119, 86 ); // matcha
}
if (this.food == tunasushi){
image(tunasushi,this.x -750*20, 318, 164, 119); //tuna
}
if (this.food == maki){
image(maki,this.x -750*20, 294, 247, 179 ); //maki
}
}
//speed of moving food
function foodMove() {
this.x += this.speedx;
}
//calling show and move function of dishes
function showFood(){
for( var i = 0; i < fd.length; i++ ) {
fd[i].display();
fd[i].move();
if ( i == fd.length){
textSize(15);
text('Sushi Bar closed. No more food for today!', 100, 200);
}
}
}
function makeBuilding(birthLocationX) {
var bldg = {x: birthLocationX,
breadth: 30,
speed: -1.0,
nFloors: round(random(2,8)),
move: buildingMove,
color: color(random(255),random(255), random(255), 80), // random color buildings with low opacity to look distant
display: buildingDisplay
}
return bldg;
}
function updateAndDisplayBuildings(){
// Update the building's positions, and display them.
for (var i = 0; i < buildings.length; i++){
buildings[i].move();
buildings[i].display();
}
}
function removeBuildingsThatHaveSlippedOutOfView(){
var buildingsToKeep = [];
for (var i = 0; i < buildings.length; i++){
if (buildings[i].x + buildings[i].breadth > 0) {
buildingsToKeep.push(buildings[i]);
}
}
buildings = buildingsToKeep; // remember the surviving buildings
}
function addNewBuildingsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newBuildingLikelihood = 0.007;
if (random(0,1) < newBuildingLikelihood) {
buildings.push(makeBuilding(width));
}
}
// method to update position of building every frame
function buildingMove() {
this.x += this.speed;
}
// draw the building and some windows
function buildingDisplay() {
var floorHeight = 20;
var bHeight = this.nFloors * floorHeight;
fill(this.color);
push();
translate(this.x, height - 40);
strokeWeight(0);
rect(0, -bHeight, this.breadth, bHeight);
for (var i = 0; i < this.nFloors; i++) {
rect(5, -15 - (i * floorHeight), this.breadth - 10, 10);
}
pop();
}
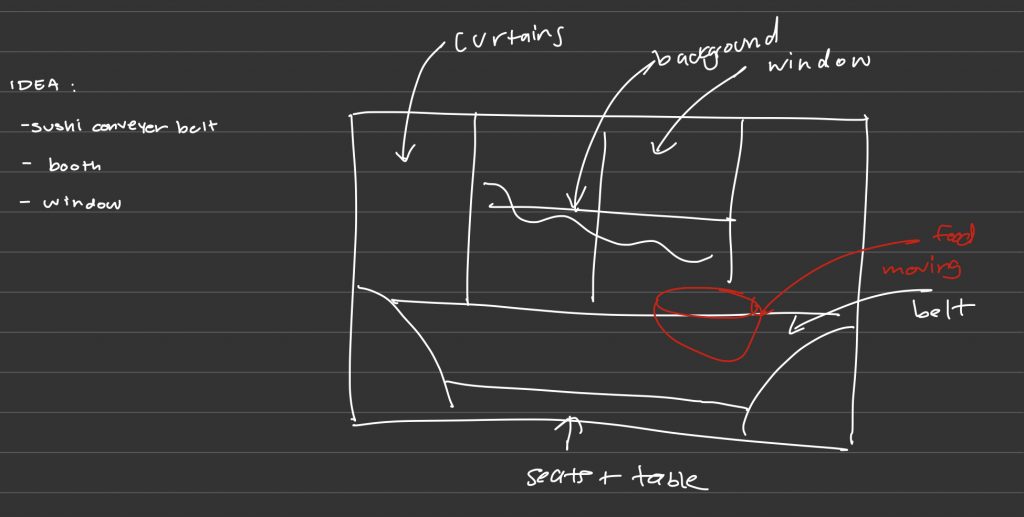