//hfroling
//section c
var level = 1;
var spot;
var a;
var b;
var lilies = [];
var fishies = [];
var turtles = [];
var heights = [80,140,200,260,320,380,440,500];
var bunny;
function preload() {
bunnyStopped = loadImage("https://i.imgur.com/ASG9W87.png"); //loads image
bunnySitting = loadImage("https://i.imgur.com/fouQhgx.png"); //loads image
bunnyDead = loadImage("https://i.imgur.com/9d2vjo4.png");
lilyPadImg = loadImage("https://i.imgur.com/Z69HCHs.png");
killerFishImg = loadImage("https://i.imgur.com/mRGD4oC.png");
turtleImg = loadImage("https://i.imgur.com/4JEWEFk.png");
}
function setup() {
createCanvas(800,600);
background(120, 150, 81);
imageMode(CENTER)
a = width/2-35;
b = 295;
noStroke();
print(bunnyStopped.width); // displays in console
print(bunnyStopped.height);
for(j=0;j<8;j++){ //lily pad object setup
lilies[j] = new Object();
lilies[j].x = (floor(random(60,width-60)/60)*60)-20;
lilies[j].y = heights[j];
}
for(k=0;k<8;k++){ //fish set up
fishies[k] = new Object();
fishies[k].x = random(width-50);
fishies[k].y = heights[k];
fishies[k].dx = random(1,6);
}
for(l=0;l<8;l++){ //turtles set up
turtles[l] = new Object();
turtles[l].x = random(width-50);
turtles[l].y = heights[l];
turtles[l].dx = random(-3,3);
}
bunny = makeBunny(width/2,320,0);
}
function draw() { //draw the image
background(120, 150, 81);
textSize(18);
textAlign(CENTER, CENTER);
fill('white');
if(frameCount < 150){
text('PRESS UP ARROW KEYS TO HOP ACROSS THE RIVER', width/2, 30);
}
river();
bunny.draw();
bunny.collideLily();
bunny.onTurtle = false;
for(i=0;i<8;i++){
bunny.collideTurtle(turtles[i])
}
for(i=0;i<8;i++){
bunny.collideWater(fishies[i],turtles[i],lilies[i])
}
bunny.dieBunny();
}
function river(){ //make river
var depth = 60;
spot = 50;
if(level == 1){
text('LEVEL 1',100, height-30);
for(var i=1; i<3; i++){
fill('lightblue');
rect(0,spot,width,depth);
fill(208,228,247);
rect(0,spot+depth,width,depth);
spot += depth*2;
}
for(k=0;k<4;k++){ //fishies on river
drawFish(fishies[k]);
fishies[k].x += fishies[k].dx
if (fishies[k].x > width) {
fishies[k].x = -40;
fishies[k].dx = random(1,6);
}
}
for(l=0; l<4; l++){ //turtles on river
drawTurtle(turtles[l]);
turtles[l].x += turtles[l].dx
if (turtles[l].x >= width -25 & turtles[l].dx > 0) {
turtles[l].dx = -turtles[l].dx;
}
if (turtles[l].x <= 25 & turtles[l].dx < 0) {
turtles[l].dx = -turtles[l].dx;
}
}
}
for(j=0;j<4;j++){ //lilies on river
drawLily(lilies[j]);
}
if(level == 2){
text('LEVEL 2',100, height-30);
for(var i=1; i<4; i++){
fill('lightblue');
rect(0,spot,width,depth);
fill(208,228,247);
rect(0,spot+depth,width,depth);
spot += depth*2;
}
for(k=0;k<6;k++){ //fishies on river
drawFish(fishies[k]);
fishies[k].x += fishies[k].dx
if (fishies[k].x > width) {
fishies[k].x = -40;
fishies[k].dx = random(1,6);
}
}
for(l=0; l<6; l++){ //turtles on river
drawTurtle(turtles[l]);
turtles[l].x += turtles[l].dx
if (turtles[l].x >= width -25 & turtles[l].dx > 0) {
turtles[l].dx = -turtles[l].dx;
}
if (turtles[l].x <= 25 & turtles[l].dx < 0) {
turtles[l].dx = -turtles[l].dx;
}
}
for(j=0;j<6;j++){ //lilies on river
drawLily(lilies[j])
}
}
if(level == 3){
text('LEVEL 3',100, height-30);
for(var i=1; i<5; i++){
fill('lightblue');
rect(0,spot,width,depth);
fill(208,228,247);
rect(0,spot+depth,width,depth);
spot += depth*2;
}
for(k=0;k<8;k++){ //fishies on river
drawFish(fishies[k]);
fishies[k].x += fishies[k].dx
if (fishies[k].x > width) {
fishies[k].x = -40;
fishies[k].dx = random(1,6);
}
}
for(l=0; l<8; l++){ //turtles on river
drawTurtle(turtles[l]);
turtles[l].x += turtles[l].dx
if (turtles[l].x >= width -25 & turtles[l].dx > 0) {
turtles[l].dx = -turtles[l].dx;
}
if (turtles[l].x <= 25 & turtles[l].dx < 0) {
turtles[l].dx = -turtles[l].dx;
}
for(j=0;j<8;j++){ //lilies on river
drawLily(lilies[j])
}
}
}
if(level == 4){
fill('lightblue');
rect(0,0,width,450)
textSize(36);
fill('white')
text('YOU WON!',width/2, height/2-50);
image(bunnySitting,(width/2),450,200,200);
textSize(12);
text('PRESS RELOAD TO PLAY AGAIN!',100, 30);
noLoop();
}
}
function makeBunny(a,b,da){ // make bunny
var bunny = {ba: a, bb: b, bda: da,
draw: drawBunny,
step: stepBunny,
collideLily: collideLily,
onLily: false,
collideTurtle: collideTurtle,
onTurtle: false,
collideWater: collideWater,
dieBunny: dieBunny
}
return bunny;
}
function drawBunny(){
image(bunnyStopped,this.ba,this.bb,50,50);
if(this.bb<=0){
level+=1;
this.bb +=level*120 + 240
}
}
function stepBunny(keyCode){ /// add this .y
if (keyCode === UP_ARROW){
this.bb -= 60;
}
if (keyCode === DOWN_ARROW){
this.bb += 60;
}
if (keyCode === RIGHT_ARROW){
this.ba += 60;
}
if (keyCode === LEFT_ARROW){
this.ba -= 60;
}
}
function collideLily(lily){
this.onLily=false;
for(i=0;i<lilies.length;i++){
if(dist(lilies[i].x,lilies[i].y,this.ba,this.bb)<=10){
this.onLily = true;
this.bda = 0;
console.log("should be on a lily")
}
}
}
function collideWater(fish,turtle,lily){ //work on
if(this.onLily == false & this.onTurtle==false && this.bb < 180+(level*120) && this.bb > 60){
if(dist(this.ba,this.bb,turtle.x,turtle.y)>15||(dist(lily.x,lily.y,this.ba,this.bb)>10)){
this.ba += 1;
}
}
}
function collideTurtle(turtle){
if (this.onLily == false){
if(dist(turtle.x,turtle.y,this.ba,this.bb)<=20){
this.ba += turtle.dx
console.log("on a turtle")
this.onTurtle = true;
}
}
}
function dieBunny(){
if(this.ba > width){
fill(135, 6, 4);
rect(0,0,width,height);
fill(82, 25, 21);
rect(0,330,width,height);
textSize(36);
fill('white')
text('YOU LOST!',width/2, 100);
image(bunnyDead,(width/2),350,200,150);
textSize(12);
text('PRESS RELOAD TO PLAY AGAIN!',100, 30);
noLoop();
}
}
function keyPressed() {
bunny.step(keyCode);
}
function drawLily(lily){
image(lilyPadImg, lily.x,lily.y, 50,50);
}
function drawFish(fish){
image(killerFishImg, fish.x, fish.y,50,50);
}
function drawTurtle(turtle){
if(turtle.dx < 0){
push()
translate(turtle.x, turtle.y);
rotate(radians(180));
image(turtleImg, 0, 0, 50,50);
pop()
}
else{
image(turtleImg, turtle.x, turtle.y, 50,50);
}
}
Author: Hazel
Looking Outwards 11
https://ars.electronica.art/aeblog/en/2020/04/10/women-in-media-arts-ai/
As artificial intelligence software that detects, recognizes, and classifies faces becomes increasingly popular, researchers Joy Buolamwini and Timnit Gebru are examining how codified biases in facial recognition software often misgender people who are not white or even fail to recognize their faces completely in their project titled “Gender Shades”. These biased facial recognition softwares are often created by male-dominated teams of computer scientists who lack diversity in ethnicity, race, and gender. Additionally, the data sets that these computer scientists feed their programs also often lack diversity, which is why the software does a poor job of recognizing people who are not white or male. To combat this, Buolamwini and Gebru have created a new standard of data set taken from a diverse group of 1270 parliamentarians from Africa and Europe. This new benchmark dataset for gender and racial diversity will help facial recognition softwares learn to recognize all faces and distinguish between genders and ethnicities without bias.
Looking Outwards Week 09
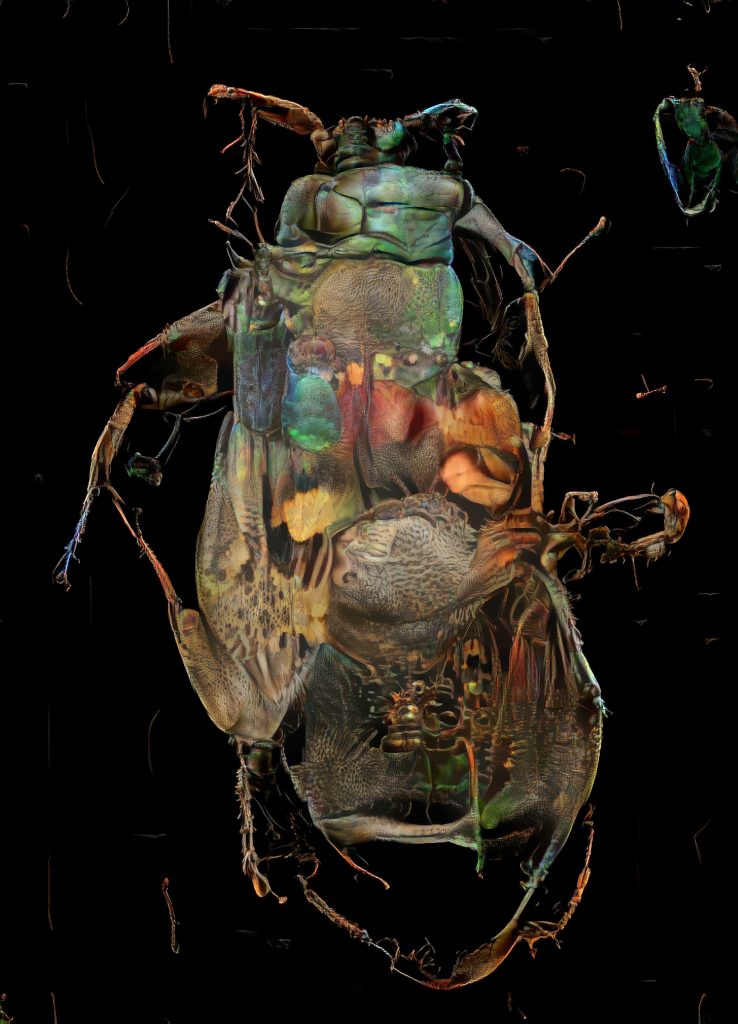
Based in Lisbon Portugal, Sofia Crespo is an accomplished artist and curator who primarily focuses on creating biologically inspired artificial intelligence-generated videos and images. The images are bizarre and ethereal, yet are rooted in familiar systems such as neural pathways, sea creatures, human organs, fungi, and animals. She programs her own project-specific custom AI software through which she runs her base images, using the AI to draw otherworldly connections between the earthly base images, which is incredibly admirable because she is able to seamlessly blend the computer-generated aspects of her work with a more analog process of physically retouching the images. While studying at Miami Art Direction School, she developed her love for AI generative art practices, and combined this passion with her interest in biology, resulting in her current technique. She no teaches workshops, gives talks, and does residencies in addition to making her own artistic works.
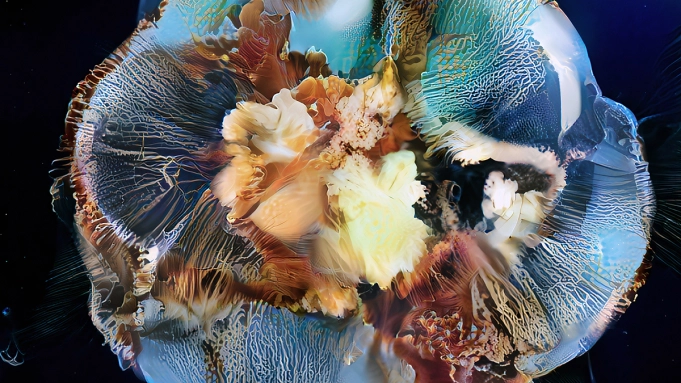
Looking Outwards 08
Paul Soulellis
An artist and professor at RISD interested in the meaning of crisis and Urgent Craft, as well as permanence, Paul Soulellis introduces himself as a queer artist and educator. As a child and young adult, Soulellis grappled with the self-hatred that came with being gay in the 70s and 80s as the AIDS crisis swept the nation. Since then, his queerness has deeply impacted his artistic practice. Although he recognizes his privileges, the difficult process of becoming secure in his sexuality was deeply entwined with a sense of urgency. Yet, Soulellis argues that slowing down in today’s society is inherently queer because it goes against the cultural fixation with acceleration. In response to this idea, he created the body of work: QUEER.ARCHIVE.WORK (2013-2018), which is a vast series of printed web publications from queer artists, aiming to immortalize the fast-paced culture of internet art. Since this first foray into printing websites, Soulellis has created many collections of printed internet images, published books of redacted tweets from politicians, zines of digital protests, and many other examples of printed literature. Although the digital world is ever-expansive, I greatly admire that Soulellis continues to create physical copies of the art and protest material that is so indicative of our generation.
Looking Outwards 07
http://www.stefanieposavec.com/
Stefanie Posavec is an artist, designer, writer, teacher, and data visualizer who finds satisfaction in representing large amounts of data in physical and experiential artworks. Her artistic practice involves collecting data manually and gradually, and although laborious, I personally really admire artists who enjoy working manually in conjunction with digitally produced components, in contrast to those who find the quickest (often fully digital) approach. Posavec’s process is extremely experimental and takes many forms, whether that is through dance, hopscotch, fine art, sculpture, auditory or wearable art, she never is tied down to one singular style or medium. However, her overarching goal, woven into each project, is to make the layperson understand and connect to data, even if they have never had an interest or experience with any data previously.
Looking Outwards 06
Randomly Generated Poetry
Although the world of random visual art is vast, as is the genre of randomly generated music, I found that I was drawn toward the idea of randomly generated poetry. In a recent project from two students at Bard College, an algorithm was written that created randomly generated poetry based on the stylistic choices of a chosen poets such as Dickenson and Shakespear. In terms of their process, they begin by writing an algorithm that reads poetry from the writer and creates a numerical value based on how often the word s used(its usage probability). Next, the bot randomly chooses a word from the list. The choice is truly random but constrained to words used by the writer. The algorithm then goes on to gauge the probability of other words used in conjunction with the first word, and chooses the next word accordingly, and so on. So although it is not truly random, the code is not always choosing the words that are most likely to be used together. I really admire this work because it is created for the public by students like ourselves, and the poetry generated is really beautiful.
Looking outwards 05
Designer and art director Santi Zoraidez blends impossibly clean computer-generated animations and images with physical products to create highly original and captivating digital campaigns for brands such as Apple, Ikea, and Nike. Although not much is known about his highly coveted artistic process, it is clear that he depends heavily on 3D modeling software to create the “physical” objects of his art. Then a phenomenal rendering software is used to give the objects materiality, texture, transparency, shine, and luminance. I really admire that the images Zoraidez creates are clean and modern, and embody a kind of playfulness that is rare in marketing. Similarly, his animations are bright and full of forms so realistic you want to reach into the screen and grab them.
week 04 project
for this project, I wanted to make a heart shape using string art!
var dx1;
var dy1;
var dx2;
var dy2;
var tx1;
var ty1;
var tx2;
var ty2;
var qx1;
var qy1;
var qx2;
var qy2;
var numLines = 50;
var numLinesb = 50;
var numLinesc = 50;
function setup() {
createCanvas(400, 400);
background(255,240,250);
//corresponds to bottom part of heart
dx1 = (-250)/numLines; //bottom left x
dy1 = (600)/numLines; //bottom left y
dx2 = (300)/numLines; // top right x
dy2 = (-700)/numLines; // top right y
//corresponds to top right part of heart
tx1 = (0)/numLinesb; //bottom left x
ty1 = (-600)/numLinesb; //bottom left y
tx2 = (200)/numLinesb; // top right x
ty2 = (700)/numLinesb; // top right y
//corresponds to top left part of heart
qx1 = (-100)/numLinesc; //bottom left x
qy1 = (500)/numLinesc; //bottom left y
qx2 = (0)/numLinesc; // top right x
qy2 = (-600)/numLinesc; // top right y
}
function draw() {
//following is bottom part of heart
stroke(200,100,100); //pink lines
var x1 = 0; //left x
var y1 = 200; //left y
var x2 = 400; //right x
var y2 = 600; //right y
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
} //following is right upper part of heart
stroke(255,100,200); // hot pink lines
var a1 = 200; //left x
var b1 = 250; //left y
var a2 = 400; //right x
var b2 = 0; //right y
for (var i = 0; i <= numLinesb; i += 1) {
line(a1, b1, a2, b2);
a1 += tx1;
b1 += ty1;
a2 += tx2;
b2 += ty2;
} // following is left upper part of heart
var c1 = 0; //left x
var d1 = 0; //left y
var c2 = 200; //right x
var d2 = 250; //right y
for (var i = 0; i <= numLinesc; i += 1) {
line(c1, d1, c2, d2);
c1 += qx1;
d1 += qy1;
c2 += qx2;
d2 += qy2;
}
stroke(240,100,240); //pink 3
for (var i = 2; i <= 100; i += 4){ //right side of heart
line(320,0,2.5*i+350,200);
line(2.5*i+350,200,320,400);
}
for (var i = 2; i <= 100; i += 4){ //left side of heart
line(80,0,2.5*i-220,200);
line(2.5*i-220,200,80,400);
}
noLoop();
}
Week 04 Blog – Brian Eno
Just as a song has a beginning, so too must it come to an end. Or so I thought before discovering the world of generative music several years ago. Popularized by musician Brian Eno in the late 70s, this genre defies norms by challenging the idea that a song or an album needs to end. Rather, this type of ambient music has the ability to generate and grow on its own, ever-expanding, yet unfortunately, there hasn’t been a way to release never-ending music to the public until recently. The project I wish to discuss today is the app that Eno released in 2016, called “Reflection”. “Reflection” is a culmination of all his work with computer-generated colored light displays and installation art, and most importantly, it has the capacity to play an endless loop of music that will never repeat itself. The app is designed by Eno and uses his secret programming methods to produce the music, as well as the visual light displays that he coded himself using a basic Arduino board. Eno considered himself more of a scientist than an artist and loves the accessibility that science offers, and tries to convey this accessibility in his music, which he approaches in a very physically intuitive manner, trying to isolate the things that “work” and continue to do them. In his project “Reflection” he uses code that randomizes the notes played, as well the delays, volume of notes, and rhythm of the piece. The code he creates also allows him to use a function to correct any notes that clash with the key. The code then generates the music and once it begins playing, Eno adds additional rules to form the music into how he likes it, though he never imagines the result beforehand.
Looking Outwards 03
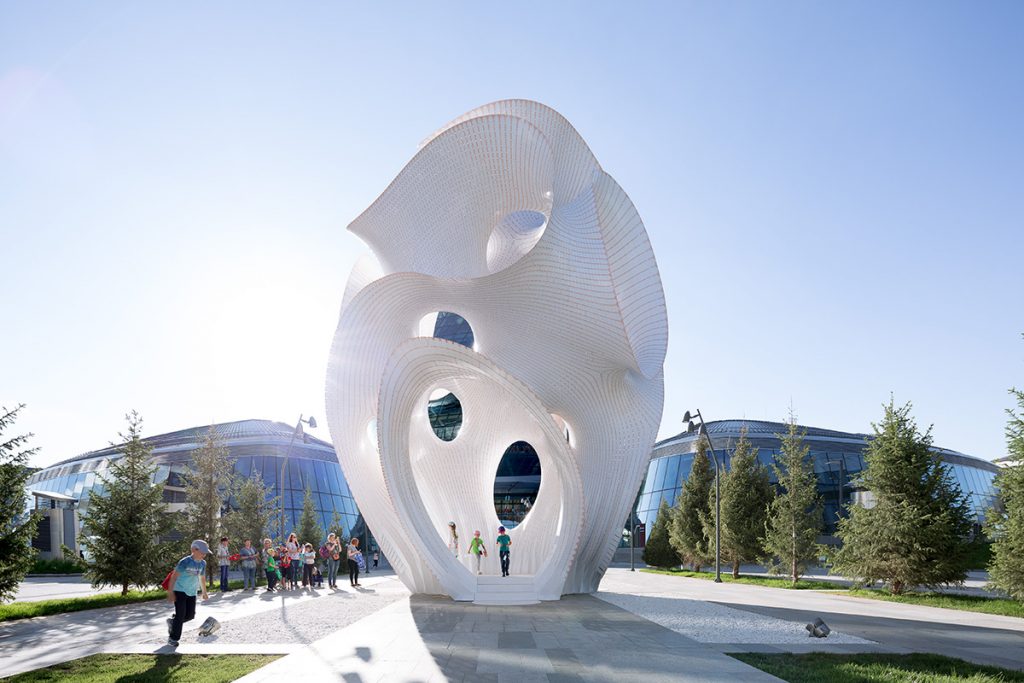
https://www.archdaily.com/879626/minima-maxima-marc-fornes-theverymany
Marc Fornes’ parametric pavilion, entitled “MINIMA|MAXIMA” is a 2017 project created by the research collective THEVERYMANY for the 2017 World Expo in Astana, Kazakhstan. This 43’ tall pavilion is designed using parametric architecture techniques including Rhinoscripting. Not only does Grasshopper (the generative modeling tool in the software Rhino) allow one to easily manipulate dimensions and shapes as well as the size of the individual panels, it can be used in conjunction with Python to create more complex geometries. Although the sculptural piece consists of only curves, the individual pieces of ultra-thin material are all created as flat surfaces, and only when interconnected do they take on their curved form. Although the construction of the pavilion is similar to the construction process of carbon fiber assembly, the structure is made of 6mm thick sheets of aluminum, stacked together to make a sandwich of white, pink, and white. Fornes and his collaborators continue to develop their research regarding the material and assembly tchniques used in their pavilions and treat each structure as a research opportunity. The cutting-edge assembly techniques used by THEVERYMANY, as well as the uniquely thin yet self-supporting construction material, are two incredibly admirable aspects of the pavilion.