// isis berymon section D
var buildings = [];
var stars = [];
var streetlights = [];
var ground = 160;
function setup() {
createCanvas(480, 200);
frameRate(10);
//initial scene
for(var i =0; i < 10; i++){
var rx = random(width);
buildings[i] = makeBuilding(rx);
}
for(var i=0; i< 30; i++){
var rx = random(width);
stars[i] = makeStar(rx);
}
for(var i = 0; i<3; i++){
var rx = random(width);
streetlights[i] = makeLight(rx);
}
}
function draw() {
background(81, 61, 128); //dark purple sky
showScene();
updateScene();
//dark grey road
fill(84);
noStroke();
rect(0, ground, width, height-ground);
}
function showScene(){ //draw and move all objects
//show stars
for(var i =0; i< stars.length; i++){
stars[i].move();
stars[i].draw();
}
//show buildings
for(var i = 0; i < buildings.length; i++){
buildings[i].move();
buildings[i].draw();
}
//show streetlights
for(var i= 0; i < streetlights.length; i++){
streetlights[i].move();
streetlights[i].draw();
}
}
function updateScene(){ //remove and add to arrays
//remove stars offscren
var keepstar = [];
for(var i = 0; i < stars.length; i++){
if(stars[i].x + 3 > 0){
keepstar.push(stars[i]);
}
}
stars = keepstar;
//add new stars randomly
var nstr = .1;
if(random(1) < nstr){
stars.push(makeStar(width+3));
}
//remove buildings offscreen
var keepbldg = [];
for(var i = 0; i < buildings.length; i++){
if(buildings[i].x + buildings[i].bw > 0){
keepbldg.push(buildings[i]);
}
}
buildings = keepbldg;
//add new buildings randomly
var nbldg = .03;
if(random(1) < nbldg){
buildings.push(makeBuilding(width));
}
//remove lights offscreen
var keeplight = [];
for(var i = 0; i < streetlights.length; i++){
if(streetlights[i].x + 15 > 0){
keeplight.push(streetlights[i]);
}
}
streetlights = keeplight;
//add new lights randomly
var nlight = .005;
if(random(1) < nlight){
streetlights.push(makeLight(width));
}
}
function makeStar(sx){ //star object constructor
var star = {x: sx,
y: random(ground),
speed: -.7,
move: moveLeft,
draw: drawS}
return star;
}
function drawS(){ //white star
stroke(255);
line(this.x, this.y-3, this.x, this.y+3);
line(this.x-3, this.y, this.x+3, this.y);
}
function makeBuilding(bx){ //building object constructor
var bldg = {x: bx,
bw: round(random(50, 100)),
bh: round(random(30, 120)),
speed: -2,
move: moveLeft,
draw: drawB}
return bldg;
}
function drawB(){
fill(32, 27, 43); //dark purple grey building
noStroke();
rect(this.x, ground-this.bh, this.bw, this.bh);
fill(95, 86, 115); //light purple grey shading
rect(this.x, ground-this.bh, 6, this.bh);
rect(this.x, ground-this.bh, this.bw, 3);
}
function makeLight(lx){
var light = {x: lx,
speed: -2,
move: moveLeft,
draw: drawL}
return light; //light object constructor
}
function drawL(){
fill(112); //mid grey
rect(this.x, 130, 5, 30);
//flickering lights
if(random(1) > .8){
fill(237, 196, 114); //yellow
} else {
fill(122);
}
rect(this.x+5, 130, 10, 5); //grey streetlight with flickering light
}
function moveLeft(){ //move left every frame
this.x += this.speed;
}
Author: Isis
project 9: computational portrait
i made a computational portrait using perlin noise to randomly draw lines until an image emerges. source image below
// isis berymon section D
//using perlin noise to randomly generate lines
//that over time create an image of my face
var isis; // portrait
var xstep = 0.0; //noise step vars
var ystep = 0.0;
var x1 = 200; //line vars
var y1 = 200;
var x2;
var y2;
//preload image
function preload(){
isis = loadImage("https://i.imgur.com/gspKB1I.jpg");
}
function drawLine(){ //draws continuous line over canvas
//randomly generate line path
x2 = map(noise(xstep), 0, 1, -200, 600);
y2 = map(noise(ystep), 0, 1, -200, 600);
xstep += .08;
ystep += .06;
//use colors from portrait
strokeWeight(2);
stroke(isis.get(x2,y2));
line(x1, y1, x2, y2);
//make 2nd point 1st point and repeat
x1 = x2;
y1 = y2;
}
function setup(){
createCanvas(400, 400);
background(50);
frameRate(60);
}
function draw(){
drawLine();
}
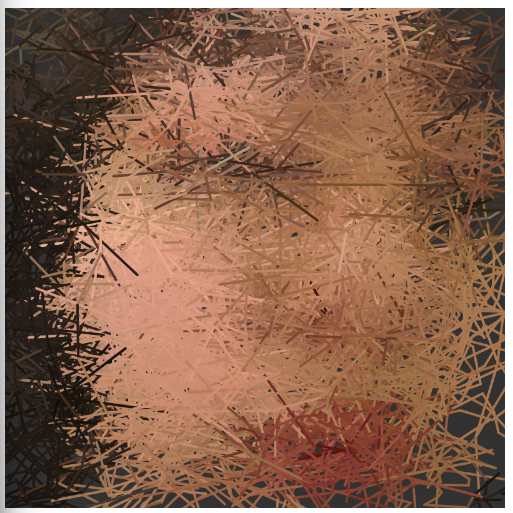
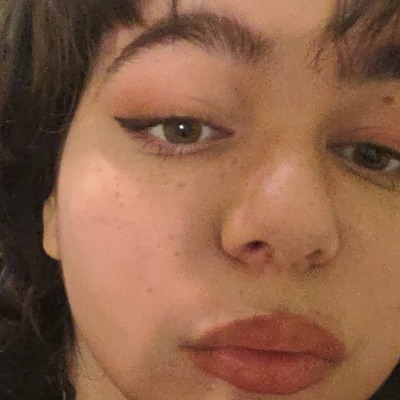
lo: non-men in computational art
One project from a woman artist that caught my eye a while ago is CAre BOt by Caroline Sinders. Sinders is an artist and designer who studies digital spaces and patterns and responds to them with her projects. CAre BOt is sort of an extension or spin-off of another one of her projects – the Social Media Breakup Coordinator – that addresses the irresponsible social media policies concerning harassment and abuse. The chatbot was given dialogue by Sinders that highlights the mistreatment of online victims, backed by her personal research of harassment on social media platforms. While interacting with CAre BOt, you can also learn a lot about that research and how social media sites are poorly designed systems. Many of her projects address system wide flaws in things and who they affect. Her artistic approach to data-driven research and issues allows users like me to learn about the data without being overwhelmed or even traumatized. It’s really interesting to me.
lo: information visualization
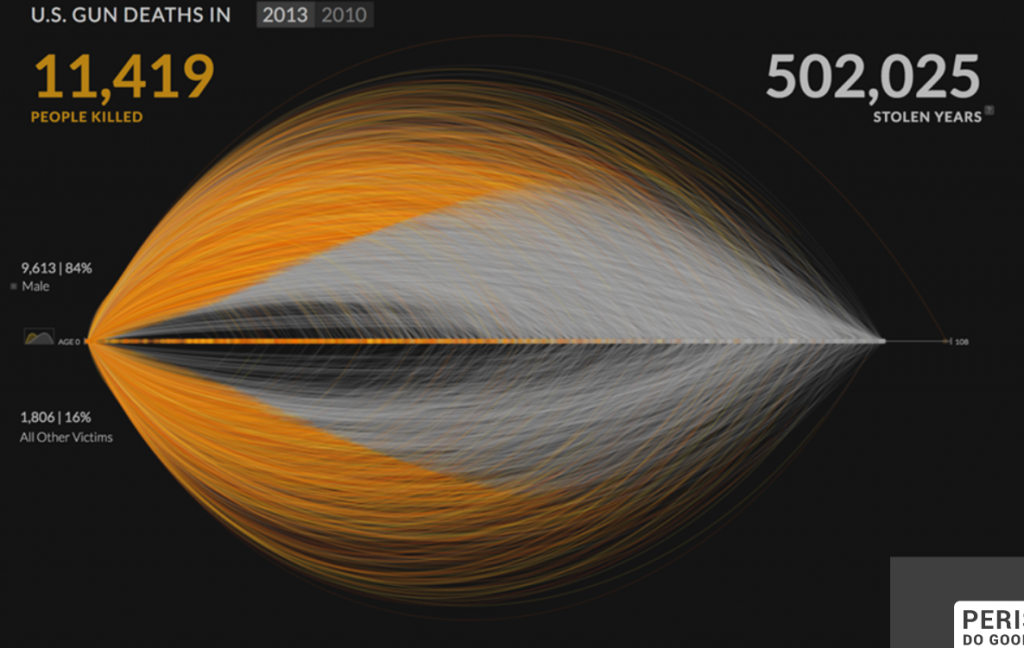
Seeing this prompt for this week, I immediately thought of the firm that made the visualization above years ago about gun violence. Periscopic is a data viz firm that emphasizes their artistic foundation in every project. Their website showcases a lot of their hand drawn process behind every final piece, and each project is developed and computed in a unique way for the cause. As a design student, I really appreciate not only their technical and artistic attention to detail, but their cultural awareness and ability to place their work in context of human lives, which often gets lost when talking about statistics and computer generated pieces.
lo: randomness
Rather than a specific project or artist, I took interest in the random generator tools at the disposal to artists. One great example that I’ve used many times before is coolors.co . It randomly generates color palettes, with the ability to make palettes based on certain colors, color modes, and gradients. Tools like this strike a balance between true randomness and useful brainstorming in the creative process. Obviously, some colors are visually and mathematically better palettes than other, but within that bias there’s still an element of randomness, which can help with things like creative block and monotony in work. Other similar tools I’m familiar with as a design student are AI generated image and typography services, which strike a similar balance. I find all of these tools to be more interesting than truly random pieces of art, because they can be amazing aids in making new, but purposeful, art.
project 6: abstract clock
I chose to make a clock that signifies time visually with the symbols of the 12 constellations. I entertained the idea of a similar 12 hour system, but rather than using numbers, it aligned with what people see in the sky over the course of a year. I also switched what moved (the counters rotate over time vs a traditional clock where the hands do) just for fun.
// isis berymon section D
function setup() {
createCanvas(480, 480);
background(55, 56, 87); //dark blue
}
function draw() {
var h = hour();
var m = minute();
var s = second();
push();
translate(240, 240);
if(h > 11){ //12 hour clock
h = h-12;
}
rotate(radians((m+1)/-2+(-30*h))); //moves ccw every minute
background(55, 56, 87); //dark blue
clock();
pop();
if(s%2==0) { //pointer blinks every second
noStroke();
fill(240, 222, 91); // pale gold
triangle(240, 30, 250, 0, 230, 0);
}
}
function clock(){
var angle = 0;
stroke(240, 222, 91) //pale gold
noFill();
circle(0, 0, 480);
for(var i = 0; i < 12; i++) { //12 spokes
push();
angle +=30;
rotate(radians(angle));
line(0, 0, 240, 0);
pop();
}
//aries symbol
push();
rotate(radians(-315)); //center in between spokes
beginShape();
vertex(-10, -200);
vertex(-20, -210);
vertex(-10, -210);
vertex(0, -200);
vertex(0, -180);
vertex(0, -200);
vertex(10, -210);
vertex(20, -210);
vertex(10, -200);
endShape();
pop();
//taurus
push();
rotate(radians(-285));
circle(0, -190, 20);
beginShape();
vertex(-15, -210);
vertex(-10, -210);
vertex(0, -200);
vertex(10, -210);
vertex(15, -210);
endShape();
pop();
//gemini
push();
rotate(radians(-255));
beginShape(LINES);
vertex(-10, -210);
vertex(10, -210);
vertex(-10, -190);
vertex(10, -190);
vertex(-4, -210);
vertex(-4, -190);
vertex(4, -210);
vertex(4, -190);
endShape();
pop();
//cancer
push();
rotate(radians(-225));
circle(-10, -195, 10);
circle( 10, -185, 10);
line(-10, -200, 10, -200);
line(10, -180, -10, -180);
pop();
//leo
push();
rotate(radians(-195));
circle(-10, -190, 10);
beginShape();
vertex(-5, -190);
vertex(-8, -210);
vertex(5, -210);
vertex(0, -185);
vertex(10, -195);
endShape();
pop();
//virgo
push();
rotate(radians(-165));
beginShape();
vertex(-20, -210);
vertex(-15, -205);
vertex(-15, -190);
vertex(-15, -205);
vertex(-10, -210);
vertex(-5, -205);
vertex(-5, -190);
vertex(-5, -205);
vertex(0, -210);
vertex(5, -205);
vertex(5, -190);
endShape();
beginShape();
vertex(5, -200);
vertex(10,-195);
vertex(0, -185);
endShape();
pop();
//libra
push();
rotate(radians(-135));
line(-10, -190, 10, -190);
beginShape();
vertex(-10, -195);
vertex(-2, -195);
vertex(-8, -205);
vertex(0, -210);
vertex(8, -205);
vertex(2, -195);
vertex(10, -195);
endShape();
pop();
//scorpio
push();
rotate(radians(-105));
beginShape();
vertex(-20, -210);
vertex(-15, -205);
vertex(-15, -190);
vertex(-15, -205);
vertex(-10, -210);
vertex(-5, -205);
vertex(-5, -190);
vertex(-5, -205);
vertex(0, -210);
vertex(5, -205);
vertex(5, -190);
vertex(10, -190);
endShape();
pop();
//sagittarius <3
push();
rotate(radians(-75));
line(-10, -190, 10, -210);
line(-5, -205, 5, -195);
line(0, -208, 10, -210);
line(10, -210, 10, -200);
pop();
//capricorn
push();
rotate(radians(-45));
beginShape();
vertex(-15, -210);
vertex(-10, -205);
vertex(-10, -190);
vertex(-10, -205);
vertex(0, -210);
vertex(5, -205);
vertex(5, -190);
vertex(10, -190);
vertex(7, -195);
vertex(0, -190);
endShape();
pop();
//aquarius
push();
rotate(radians(-15));
beginShape();
vertex(-10, -200);
vertex(-5, -210);
vertex(0, -200);
vertex(5, -210);
vertex(10, -200);
endShape();
beginShape();
vertex(-10, -190);
vertex(-5, -200);
vertex(0, -190);
vertex(5, -200);
vertex(10, -190);
endShape();
pop();
//pisces
push();
rotate(radians(15));
line(-10, -210, 0, -200);
line(0, -200, -10, -190);
line(5, -200, 15, -190);
line(5, -200, 15, -210);
line(-5, -200, 10, -200);
pop();
}
lo: 3D computer graphics
The work of Son Taehung, and company 37th Degree as a whole, is very interesting to me and I wanted to look into it. 37th Degree is a production company that executes computer generated graphics in films and videos very well. Son Taehung in particular works in their South Korean sector with entertainment company SM, working on various K-pop videos. I couldn’t find a lot on their process itself, but the blending of mediums and techniques in their projects is intriguing and shows their level of expertise and artistic vision. This kind of seamless mixing and matching of reality and virtual assets wouldn’t be possible without computer generated graphics.
project 5: wallpaper
// isis berymon section D
function setup() {
createCanvas(600, 600);
background(206, 214, 204); //light green
}
function draw() {
var x = -20; //negative so pattern doesnt stop at edge
var y = -20;
for(var i = 0; i < 6; i++){
for(var j = 0; j < 6; j++){
tile(x, y);
x+=120;
}
y+=120;
x = -20;
}
}
function tile(x, y) {
push();
translate(x, y);
//branches
stroke(120, 105, 80); //brown
line(26, 0, 38, 12); //touches top
line(40, 19, 20, 39);
line(0, 27,14, 14); //touches left 1
line(4, 24, 54, 73);
line(54, 73, 70, 56);
line(47, 66, 33, 80);
line(24, 74, 13, 63);
line(13, 63, 0, 75); //touches left 2
line(27, 120, 15, 108); //touches bottom
line(15, 108, 29, 94);
line(29, 94, 41, 106);
line(41, 106, 64, 83);
line(64, 83, 84, 102);
line(84, 102, 93, 93);
line(92, 120, 115, 97);
line(115, 97, 91, 73);
line(91, 73, 104, 59);
line(104, 59, 120, 75); //touches right 2
line(97, 66, 88, 57);
line(88, 57, 98, 46);
line(120, 27, 108, 37); //touches right 1
line(108, 37, 88, 17);
line(88, 17, 91, 13);
//flowers
flower(41, 20);
flower(102, 42);
flower(18, 46);
flower(73, 53);
flower(28, 79);
flower(63, 88);
flower(12, 110);
flower(93, 124);
pop();
}
function flower(x, y) {
noStroke();
fill(187, 166, 212); //purple petals
circle(x, y-6, 10);
circle(x+6, y, 10);
circle(x, y+6, 10);
circle(x-6, y, 10);
circle()
fill(240, 228, 188); //yellow center
circle(x, y, 6);
}
lo: sound art
Rather than one specific project, I’d like to talk about a genre that’s very dependent on digital production to experiment and push the boundaries of music. Hyperpop is a fairly new genre in mainstream culture and is defined by its exaggerated and dramatic nature compared to contemporary pop music. Many of my personal favorite songs include larger than life basslines, quirky sound effects, and almost nonsensical lyrics, if any. Much of this experimentation is possible due to digital workstations and tools, making and mixing sounds that would not naturally happen in traditional music. Certain artists, like SOPHIE, also viewed music making as an entirely different process than people of the past would have. She described her process as something similar to building a sculpture – where each sound mimicked a certain material and sensation. While there isn’t one specific way these artists generate music, I think this subculture encapsulates how the advent of technology in the musical space has broadened their possibilities.
project 4: string art
I made my piece about flowers. I used functions to be able to place flowers wherever I want on the canvas, although there are only two right now
// isis berymon section D
var dx;
var dy;
var numLines;
function setup() {
createCanvas(400, 300);
background(84, 111, 82); //green
}
function draw() {
//center flower
stroke(134, 190, 243); //light blue
petal(150, 100, 250, 100, 200, 150);
petal(250, 100, 250, 200, 200, 150);
petal(250, 200, 150, 200, 200, 150);
petal(150, 100, 150, 200, 200, 150);
stroke(144, 115, 245); //light purple
petal(125, 75, 175, 75, 200, 150);
petal(125, 75, 125, 125, 200, 150);
petal(225, 75, 275, 75, 200, 150);
petal(275, 75, 275, 125, 200, 150);
petal(275, 175, 275, 225, 200, 150);
petal(275, 225, 225, 225, 200, 150);
petal(175, 225, 125, 225, 200, 150);
petal(125, 225, 125, 175, 200, 150);
flowerCenter(200, 150);
//right flower
stroke(134, 190, 243); //light blue
petal(350, 0, 400, 50, 400, 0);
stroke(144, 115, 245); //light purple
petal(300, -25, 350, 25, 400, 0);
petal(375, 50, 425, 75, 400, 0);
flowerCenter(400, 0);
noLoop();
}
function flowerCenter(x, y) {
stroke(245, 231, 115); //light yellow
numLines = 50;
for(var i = 0; i <= numLines; i++){
push();
translate(x, y);
rotate(radians(i*360/numLines)) // draws lines in a circle
line(0, 0, 0, 25);
pop();
}
}
function petal(x1, y1, x2, y2, centerX, centerY) {
numLines = 10;
dx = (x2-x1)/numLines;
dy = (y2-y1)/numLines;
var drawX1 = x1;
var drawY1 = y1;
for(var i = 0; i <= numLines; i++){
line(drawX1, drawY1, centerX, centerY); //lines all come from center
drawX1 += dx;
drawY1 += dy;
}
}