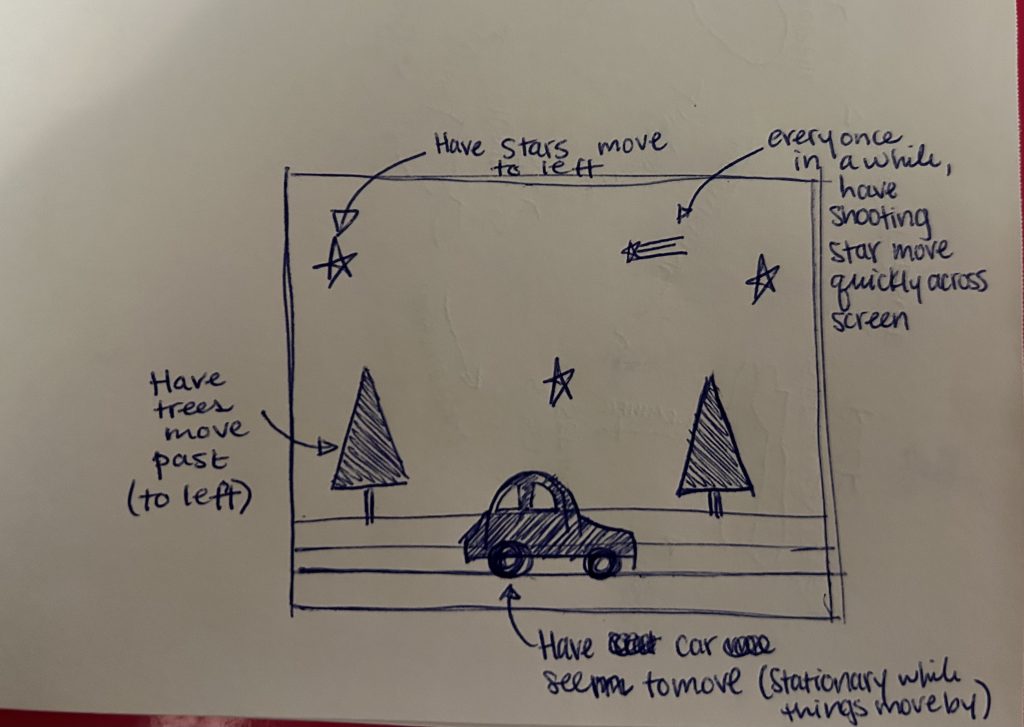
It was fun to figure out how to create a whole landscape without having to define absolutely everything and making it more interesting with randomness. I added a shooting star with a very small creation probability to create a little surprise within the landscape every once in a while.
// Rachel Legg / rlegg / Section C
//have trees and stars move by
//every once in a while, a surprising shooting star
//car in the middle (moving past trees)
var stars = [];
var trees = [];
var shootingStars = [];
var car;
function preload(){
car = loadImage("https://i.imgur.com/C7SXV3W.png");
}
function setup() {
createCanvas(450, 300);
frameRate(10);
//initial stars and trees
for(var i = 0; i < 6; i++){
var sx = random(width);
stars[i] = makeStar(sx);
}
for(var i = 0; i < 10; i++){
var sx = random(width);
trees[i] = makeTree(sx);
}
//random shooting star
for(var i = 0; i < 1; i++){
var sx = random(width);
shootingStars[i] = makeShootingStar(sx);
}
}
function draw() {
//sky - dark blue
background(10, 52, 99);
//ground
fill("darkgreen");
noStroke();
rect(0, 240, 500, 75);
//road
fill("gray");
rect(0, 260, 495, 10)
updateAndDisplayStar();
removeStarOutOfView();
addNewStar();
updateAndDisplayShootingStars();
removeShootingStarOutOfView();
addNewShootingStars();
updateAndDisplayTree();
removeTreeOutOfView();
addNewTree();
//car stays stationary as scenery moves by
//drew out car in illustrator & uploaded to imgur
image(car, width/2 - 60, 207, 165, 65);
}
//stars object
function makeStar(starLocationX){
var star = {x : starLocationX,
y : random(0, 170),
starScale : random(0.1, 0.5),
speed : -1,
move : moveStar,
display : displayStar}
return star;
}
function displayStar(){
push();
translate(this.x, this.y);
fill(255);
noStroke();
scale(this.starScale);
beginShape();
vertex(50, 18);
vertex(61, 37);
vertex(83, 43);
vertex(69, 60);
vertex(71, 82);
vertex(50, 73);
vertex(29, 82);
vertex(31, 60);
vertex(17, 43);
vertex(39, 37);
endShape();
pop();
}
//update star positions and display
function updateAndDisplayStar(){
for(var i = 0; i < stars.length; i++){
stars[i].move();
stars[i].display();
}
}
function addNewStar(){
var newStarProbability = 0.01;
if (random(0, 1) < newStarProbability){
stars.push(makeStar(width));
}
}
function removeStarOutOfView (){
//if star goes off left edge, remove from array
var starsToKeep = [];
for (var i = 0; i < stars.length; i++){
if (stars[i].x + 50 > 0) {
starsToKeep.push(stars[i]);
}
}
//stars left
stars = starsToKeep;
}
function moveStar(){
this.x += this.speed;
}
//trees object
function makeTree(treeLocationX){
var tree = {xt : treeLocationX,
yt : 240,
treeScale : random(0.1, 0.5),
treeColor : color(0, random(75, 255), 0),
speedT : -3,
moveT : moveTree,
displayT : displayTree}
return tree;
}
function displayTree(){
push();
translate(this.xt + 60, this.yt); //add 60 so transitions onto screen
fill(255);
noStroke();
scale(this.treeScale);
//tree!
noStroke();
fill("brown");
rect(0, 0, 10, -50);
rect(1, 0, -10, -50);
fill(this.treeColor);
triangle(-60, -50, 60, -50, 0, -400);
pop();
}
//update tree positions and display
function updateAndDisplayTree(){
for(var i = 0; i < trees.length; i++){
trees[i].moveT();
trees[i].displayT();
}
}
function addNewTree(){
var newTreeProbability = 0.04;
if (random(0, 1) < newTreeProbability){
trees.push(makeTree(width));
}
}
function removeTreeOutOfView (){
//if star goes off left edge, remove from array
var treesToKeep = [];
for (var i = 0; i < trees.length; i++){
if (trees[i].xt + 100 > 0) {
treesToKeep.push(trees[i]);
}
}
//trees left
trees = treesToKeep;
}
function moveTree(){
this.xt += this.speedT;
}
//shooting star object
function makeShootingStar(locationX){
var shootingStar = {xs : locationX,
ys : random(75, 125),
shootingStarScale : random(0.1, 0.3),
speedS : -8,
moveS : moveShootingStar,
displayS : displayShootingStar}
return shootingStar;
}
function displayShootingStar(){
push();
translate(this.xs + 60, this.ys); //add 60 so transitions onto screen
fill("yellow");
noStroke();
scale(this.shootingStarScale);
//shooting star
beginShape();
vertex(50, 18);
vertex(61, 37);
vertex(83, 43);
vertex(69, 60);
vertex(71, 82);
vertex(50, 73);
vertex(29, 82);
vertex(31, 60);
vertex(17, 43);
vertex(39, 37);
endShape();
stroke("yellow");
strokeWeight(2);
line(60, 37, 100, 37);
line(60, 47, 110, 47);
line(60, 57, 120, 57);
pop();
}
//update tree positions and display
function updateAndDisplayShootingStars(){
for(var i = 0; i < shootingStars.length; i++){
shootingStars[i].moveS();
shootingStars[i].displayS();
}
}
function addNewShootingStars(){
var newShootingStarsProbability = 0.008;
if (random(0, 1) < newShootingStarsProbability){
shootingStars.push(makeShootingStar(width));
}
}
function removeShootingStarOutOfView (){
//if star goes off left edge, remove from array
var shootingStarsToKeep = [];
for (var i = 0; i < shootingStars.length; i++){
if (shootingStars[i].xs + 100 > 0) {
shootingStarsToKeep.push(shootingStars[i]);
}
}
//trees left
shootingStars = shootingStarsToKeep;
}
function moveShootingStar(){
this.xs += this.speedS;
}