Using pointillism for different shapes. Before, I was trying to use different lines, but I wasn’t able to get it to work sadly.
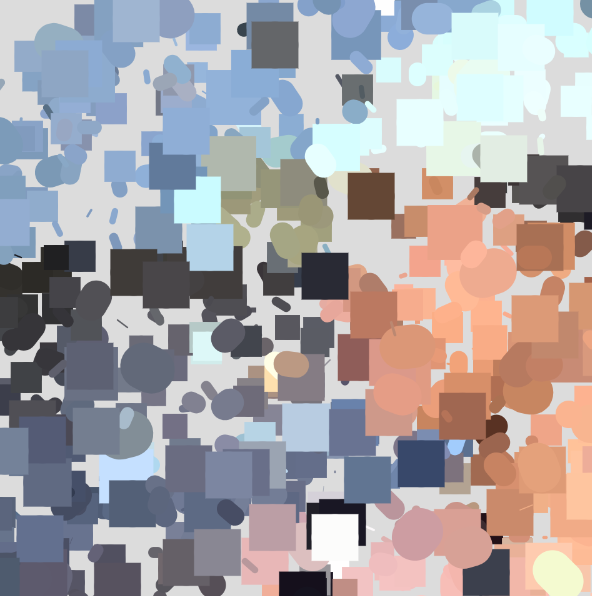
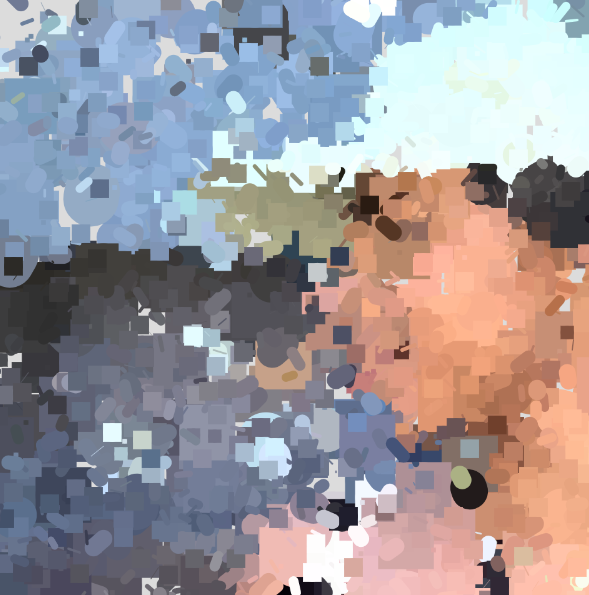
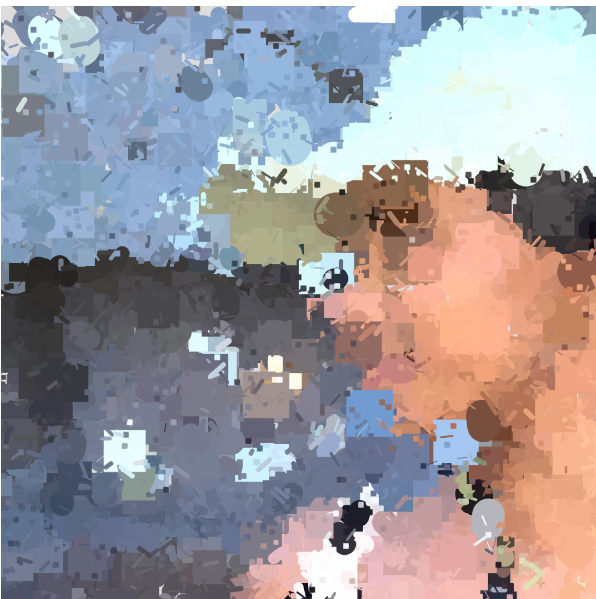
var sonia;
var smallPoint, largePoint;
function preload() {
sonia = loadImage("https://i.imgur.com/3h0u1wQ.jpeg");
}
function setup() {
createCanvas(480, 480);
//creating variables for the smallPoint size and the largePoint size
smallPoint = 4;
largePoint = 20;
noStroke();
background(220);
rectMode(CENTER)
}
function draw() {
//created 6 x and y variables to hold different sizes of widths and heights based on the corresponding variable
var x1 = floor(random(sonia.width));
var y1 = floor(random(sonia.height));
//-10 and +10 so that it would always draw a shorter line, so when I used get, it would get a color closer to the point.
var x2 = floor(random(x1 - 10, x1 + 10));
var y2 = floor(random(y1 -10, y1 + 10));
var x = floor(random(sonia.width));
var y = floor(random(sonia.height));
var lineLength = ((y2 - y1) / (x2 - x1)) / 10;
createMultipleLines(x1,y1, x2,y2);
createMultipleRectangles(x,y);
createMultiplePoints(x,y);
}
function createMultipleLines(x1, y1, x2, y2) {
var pointillize = map(mouseX, 0, width, smallPoint, largePoint);
//gets color
var pix = sonia.get(x1,y1);
var pix1 = sonia.get(x2,y2);
stroke(pix);
//random strokeWeight
strokeWeight(random(0.1, pointillize));
line(x1,y1,x2,y2);
}
function createMultipleRectangles(x,y) {
//creates multiple rectangles using same method
var pointillize = map(mouseX, 0, width, smallPoint, largePoint);
print(sonia.width);
print(sonia.height)
var pix = sonia.get(x,y);
fill(pix);
noStroke();
rect(x,y, pointillize, pointillize);
}
function createMultiplePoints(x,y) {
//creates multiple circular points using same method.
var pointillize = map(mouseX, 0, width, smallPoint, largePoint);
print(sonia.width);
print(sonia.height)
var pix = sonia.get(x,y);
fill(pix);
noStroke();
ellipse(x,y, pointillize, pointillize);
}
// function createTinyLines(x1,y1,x2,y2, lineLength) {
// var pointillize = map(mouseX, 0, width, smallPoint, largePoint);
// for (var i = 0; i< lineLength; i ++) {
// var pix = sonia.get(x1,y1);
// var pix1 = sonia.get(x2,y2);
// stroke(pix);
// line(x1,y1,x2,y2);
// var slopeY = y2 - y1;
// var slopeX = x2 - x1;
// x1 = x2;
// y1 = y2;
// x2 += slopeX;
// y2 += slopeY;
// }
// }
// function createOneLine(x1,y1,x2,y2, lineLength) {
// //var pointillize = map(mouseX, 0, width, smallPoint, largePoint);
// var pix = sonia.get(x1,y1);
// var pix1 = sonia.get(x2,y2);
// stroke(pix);
// for (var i = 0; i< 20; i ++) {
// line(x1,y1,x2,y2);
// var slopeY = y2 - y1;
// var slopeX = x2 - x1;
// x1 == x2;
// y1 == y2;
// x2 += slopeX;
// y2 += slopeY;
// }
// }