//Alicia Kim
//Section B
var terrainHeight = [];
var noiseParam = 0;
var noiseStep = 0.1;
var offset=0;
var maxi=1;
var fish = [];
var loc1 = 600;
var loc2 = 400;
var loc3 = 100;
var loc4 = 230;
var starX=[];
var starY=[];
var r = 144;
var g = 209;
var b = 206;
var by = 240;
var sx =240;
var sy = 60;
var frameCount_=0;
function setup() {
createCanvas(480, 240);
background(144, 209, 206);
frameRate(8);
for (var i=0; i<=width/5; i++) {
noiseParam += noiseStep;
var n = noise (noiseParam);
var value = map (n, 0, 1, 0, height);
terrainHeight.push(value);
noiseParam += noiseStep
}
for (var i=0;i<20;i++){
starX.push(random(0,width));
starY.push(random(0,height-100));
}
}
function draw() {
background(r, g, b);
loc1-=5;
loc2-=5;
loc3-=5;
loc4-=5;
if (loc1<-100){
loc1 = 600;
}
if (loc2<-100){
loc2 = 600;
}
if (loc3<-100){
loc3 = 600;
}
if (loc4<-100){
loc4 = 600;
}
noStroke();
drawSun();
if (frameCount_>50 & frameCount_<103) {
r-=20;
g-=8;
b-=2;
}
if (r<180 & g<180 && b<180) {
stars();
}
if (r<=0 & g<=0 && b<=0) { //reset
r=144;
g=209;
b=206;
sy = 60;
sx = 240;
frameCount_=0;
} else {
r+=6;
g-=0.1;
b-=1;
}
drawCloud(loc1,loc2,loc3,loc4);
drawMountain();
drawLake();
drawBoat();
frameCount_+=1;
}
function drawSun(){
fill (255, 150, 51); //yellow
circle (sx,sy,40,40);
sy+=1.5;
sx+=3;
}
function drawLake(){
fill (60, 111, 166,220); //blue
beginShape();
vertex(0,height);
for (var x = 0; x < width; x++) {
// wave
var theta = offset+0.07*x;
var y = map(sin(theta),-maxi,maxi,height-60,height-70);
vertex(x,y);
}
vertex(width, height);
endShape();
offset += 0.4;
}
function drawBoat(){
fill(235, 100, 52); //orange
strokeWeight(1);
ellipse(width/2,by,300,60);
fill(100);
ellipse(width/2,by,80,30);
// make boat float
if (by <= 210) { // when it gets higher than lake
by += 4;
} else {
by -= 1;
}
}
function drawCloud(loc){
drawCloudHelper(1.3,loc1,-4);
drawCloudHelper(1,loc2,25);
drawCloudHelper(0.8,loc3,15);
drawCloudHelper(1.6,loc4,0);
}
function drawCloudHelper(scale,loc,dy){
fill(255);
circle(loc,30+dy,30*scale);
circle(loc-10,36+dy,25*scale);
circle(loc-20,43+dy,20*scale);
circle(loc-3,40+dy,25*scale);
circle(loc+10,38+dy,25*scale);
circle(loc+21,41+dy,14*scale);
}
function drawMountain(){
terrainHeight.shift();
var n = noise(noiseParam);
var value = map (n, 0, 1, 0, height);
terrainHeight.push(value);
noiseParam += noiseStep
for (var i = 0; i < width/5; i++) {
noStroke();
fill (101, 138, 41); //green
beginShape();
vertex(i*5,terrainHeight[i]);
vertex((i+1)*5, terrainHeight[i+1]);
vertex ((i+1)*5, height-40);
vertex(i*5, height-40);
endShape();
}
}
function stars (){
for (var i=0;i<20;i++){
drawStar(starX[i],starY[i],1,4,5);
}
}
// adapted from p5js website (https://p5js.org/examples/form-star.html)
function drawStar(x, y, radius1, radius2, npoints) {
let angle = TWO_PI / npoints;
let halfAngle = angle / 2.0;
beginShape();
for (let a = 0; a < TWO_PI; a += angle) {
let sx = x + cos(a) * radius2;
let sy = y + sin(a) * radius2;
vertex(sx, sy);
sx = x + cos(a + halfAngle) * radius1;
sy = y + sin(a + halfAngle) * radius1;
vertex(sx, sy);
}
endShape(CLOSE);
}
Author: Alicia
Looking Outwards 11: Societal Impacts of Digital Art
Beeple’s NFT, “Everydays” sold for a whopping price of 69 million dollars to a crypto company. His piece is a piece of digital art relying on the blockchain technology to stay immutable and hold value. This is a new realm of conceptual art. Also, this type of art has a huge social impact. There are people of all ages in all professions making an insane amount of money off NFTs now and no one knows how long this can last and how reliable this is. The whole idea of NFTs rely on the authenticity and the ownership of the art. However, authenticity of digital art is conceptual and only happens when everyone believes in it. It will be interesting to see how many more people will invest in NFTs in the future and how much they will be worth in a few years.
Project 09: Computational Portrait (Custom Pixel)
i used my portrait as an each pixel to create a whole portrait. I used smaller images for face & background section, but bigger and rotated images for hair and eye section to separate the section of my feature.
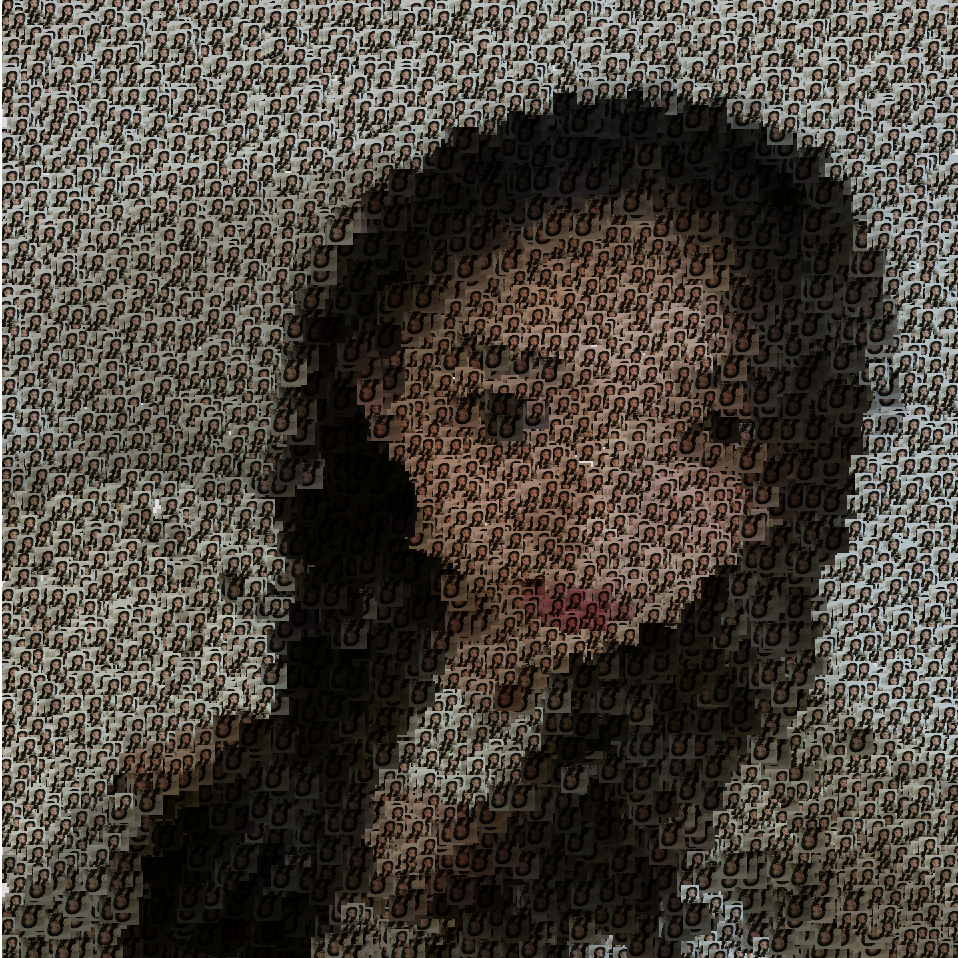
// Alicia Kim
// yoonbink
// Section B
function preload(){ //load the image
alicia = loadImage("https://i.imgur.com/FPEjcyO.jpeg");
}
function setup() {
createCanvas(480, 480);
imageMode(CENTER);
angleMode(DEGREES);
background(220);
alicia.resize(480,480); //resize alicia to canvas size
alicia.loadPixels(); //load pixels
}
function draw() {
var x = floor(random(alicia.width)); //random x-coordinate value
var y = floor(random(alicia.height)); //random y-coordinate value
var pixColor = alicia.get(x,y); //get random coordinate in the canvas
tint(pixColor); //tint with the color of that coordinate
// divide sections by hair and the rest
if (pixColor[0]>130) { //face & background has smaller images
image(alicia,x,y,9,9);
}
if (pixColor[0]<130) { //hair section has bigger & rotated images
push();
translate(x,y);
rotate(180);
image(alicia,0,0,13,13);
pop();
}
}
Looking Outwards 09: A Focus on Women and Non-binary Practitioners in Computational Art
Sinew Flex is a responsive installation by architect Jenny Sabin. Jenny Sabin is an architect using a computational techniques to create experimental form, bringing new perspectives to ways we see structures around us. She studied interdisciplinary visual art for bachelors and architecture for masters degree. Since she specializes in adaptive architecture, material computation, and biomimicry, most of her works contain bioinspired design created through knitting of responsive fibres. Similarly, Sinew Flex, a 2 story double canopy structure connected with a central bifurcated tubular form, is mathematically generated and activated by digital knitting process and light.The responsive fibres used in the structure change color and glow in response to an integrated lighting system and changing programs. She employs artificial intelligence to translate the data from environment into an immersive and interactive light and color. Also, the inhabitable structure provides various points of engagement; transformational views when audience descends the stairs, pause, productivity, and reflection at the base of the sculpture. I admire how she breaks down the barriers of disciplines and create a integrated work of engineering, architecture, biology, and mathematics. Especially, how she branches “knitting” into structural and architectural applications is very creative and compelling as it’s not a common material used in architecture.
https://www.jennysabin.com/sinewflex
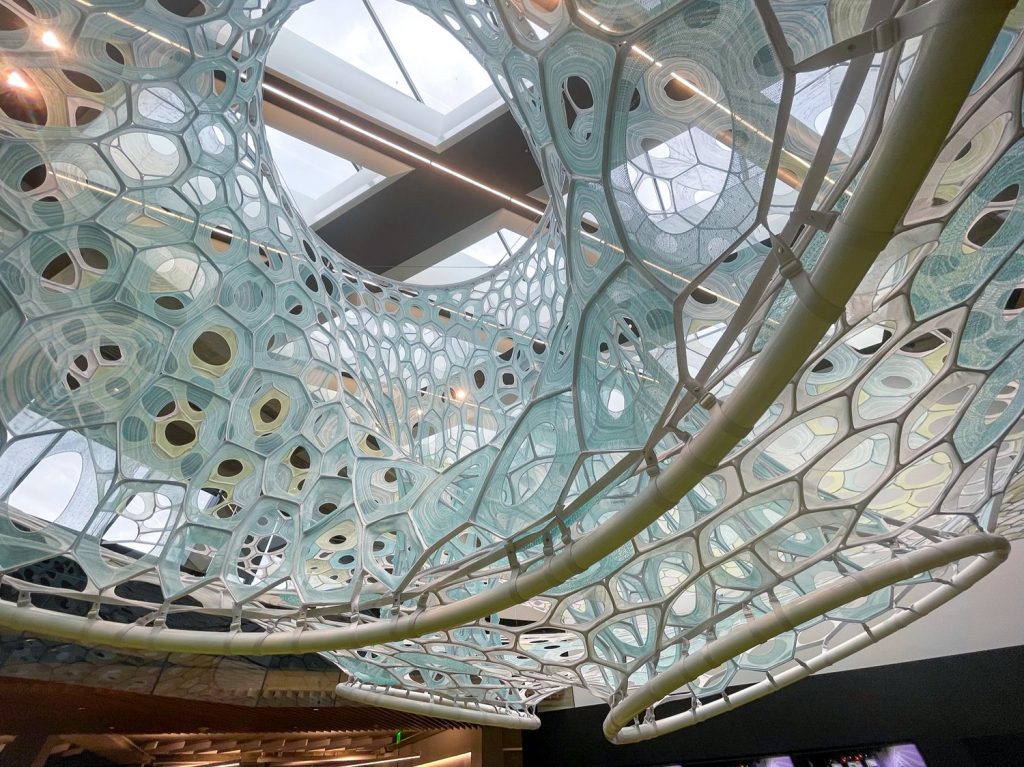
Looking Outwards 08: The Creative Practice of an Individual
Christina Phazero Curlee is a game designer, specializing in emotional impactful and narrative driven Art Games. She describes herself as a “multi-disciplinary game designer” since she works seamlessly in multiple 3D editors, and does script coding, and uses her traditional art foundation in her game design process. She studied traditional art in college and even formerly worked as painte but also she is self-taught in game design, 3D Art, and programming. Her visual art foundation and self-teaching gave her an unusual framework as a game developer as she blends a principle, techniques, and subject matters found in fine arts with game conventions to create her own design style and language in game development.
Her body of work usually explores emotional experiences, marginalized identities, and digital consciousness. I admire her work “Artifacts II” the most as her passion in emotional storytelling is well brought out in this game. Artifacts II is a surreal narrative game about personal discovery and trauma such as chdildhood neglect; The game is set in a surreal storybook-like series of environments, representing memory spaces in the character’s mind. The character engtangles trauma in the mind and body and go through process of introspection and self evaluation as he/she plays. I admire the way she utilizes symbols to convey her personal experiencs, making an game environment conceptually rich, imaginative, and compelling.
To effectively present her work, she uses symbols such as enemies, scores, and timers to represent the concepts of love, fear, and defense mechanisms. Also, Artifact II uses elements of exploration (walking sims), RPG, and survival MDA (mechanics, dynamics, and aesthetics) to effectively reveal the fragments of character’s past memories.
Project-07: Composition with Curves
//Alicia Kim
//yoonbink@andrew.cmu.edu
//Section B
var nPoints = 100;
var r;
function setup() {
createCanvas(480, 480);
background(220);
r=0;
}
function draw() {
// gradient background (adapted from my project 6)
for(var j=0; j<height; j+=1) {
stroke(lerpColor(color(127,255,212) ,color(255,253,208),j/height));
line(0,j,width,j);
}
noStroke();
push();
translate (width/2, height/2);
noFill();
if (r<=15){
r+=0.17;
}
else{r=0}
drawHeart2(r);
drawDevil();
pop();
drawHeart1();
}
function drawDevil() {
// https://mathworld.wolfram.com/DevilsCurve.html
// adapted from drawCranoid example from Wk 7 Deliverable example
var x;
var y;
fill(250,250,210);
beginShape();
for (var i = 0; i < nPoints; i++) {
var a = constrain((mouseX / width), -16.0, 16.0);
var b = constrain((mouseY / height), -16.0, 16.0);
var t = map(i, 0, nPoints, 0, TWO_PI);
x = 100*cos(t)*sqrt((sq(a)*sq(sin(t))-(sq(b)*sq(cos(t)))/(sq(sin(t))-sq(cos(t)))));
y = 100*sin(t)*sqrt((sq(a)*sq(sin(t))-(sq(b)*sq(cos(t)))/(sq(sin(t))-sq(cos(t)))));
vertex(x, y);
}
endShape();
}
//cursor heart
function drawHeart1(){
var x2;
var y2;
fill(250);
beginShape();
for (var j = 0; j < nPoints; j++) {
var t2 = map(j, 0, nPoints, 0, TWO_PI);
x2 = mouseX+2*16*pow(sin(t2),3);
y2 = 2*(13*cos(t2)-5*cos(2*t2)-2*cos(3*t2)-cos(4*t2));
y2= mouseY-y2
vertex(x2, y2);
}
endShape();
}
//background heart
function drawHeart2(r){
var x3;
var y3;
// var r;
fill(173,216,230);
beginShape();
// r=10;
// r+=1;
for (var j = 0; j < nPoints; j++) {
var t2 = map(j, 0, nPoints, 0, TWO_PI);
x3 = r*16*pow(sin(t2),3);
y3 = -r*(13*cos(t2)-5*cos(2*t2)-2*cos(3*t2)-cos(4*t2));
vertex(x3, y3);
}
endShape();
}
Looking Outwards 07: Information Visualization
I am inspired by the project, Unnumbered Sparks by Aaron Koblin. This interactive sculpture installation is a combination of art and technology based on custom software.
Basically, the sculpture is made up of a complex matrix of hand and machine-made knots and braided fiber, and it’s spanned between the city’s existing architectures. To create a geometric and structural design for the rope network, the artist utilized a custom software, implementing an “adaptive form finding” algorithm. He also used the custom 3D software by Autodesk to model the sculpture and test its feasibility by exploring density, shape, and scale in greater detail. What makes this giant aerial netted sculpture special and admirable is the dynamic interaction between visitors and the artwork; The work visualizes visitors’ physical gestures on mobile devices in real-time through multi-colored light projection on the sculpture. The creator’s artistic sensibilities are manifested in his pattern design of the structural ropes and the aesthetic installation of it that well blends into the city environment.
http://www.aaronkoblin.com/project/unnumbered-sparks/
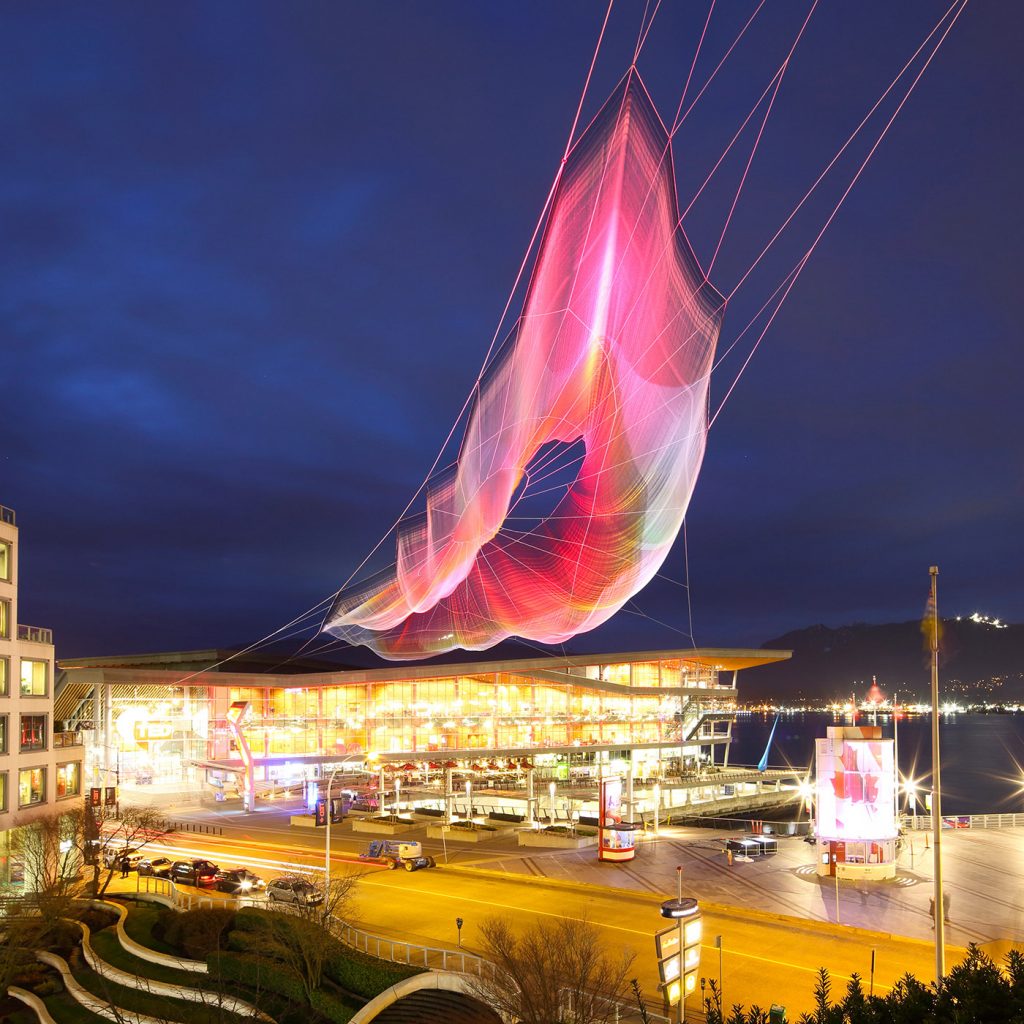
Project 06 – Abstract Clock
I created a boba clock which is milk tea with boba from noon to midnight and juice with lychee jelly from midnight to noon. The number of Jelly/Boba inside the cup, including one inside the straw, represents the hour. Juice/milk shrinks per second, and the full amount disappears per minute. Lastly, a Jelly/Boba stuck inside the straw moves upward per minute and got out from the straw each hour.
//Alicia Kim
//Section B
var x = [];
var y = [];
var angle = [];
function setup() {
createCanvas(300, 400);
for (var i=0; i<(hour()%12-1); i+=1) {
x[i] = random (120,190);
y[i] = random (200,340);
angle[i] = random(360);
}
for(var j=0; j<height; j+=1){
stroke(lerpColor(color(255,232,239) ,color(255,253,208),j/height));
line(0,j,width,j);
}
}
function draw() {
var s = second();
var min = minute();
stroke(0);
//juice with lychee jelly from midnight to noon
//& milk tea with boba from noon to midniht
push();
if (hour()>12){
drawMilk(s);
}
else if (hour()<=12){
drawJuice(s);
}
pop();
push ();
drawCup();
pop();
push();
drawStraw();
pop();
push();
if (hour()>12){
drawBoba();
strawBoba(min);
}
else if (hour()<=12){
drawJelly();
strawJelly(min);
}
pop();
}
// juice inside the cup shrinks per sec
function drawJuice(s){
fill(255,203,164); //orange
quad(70,75,230,75,215,350,85,350); //juice
//cover
fill(255);
quad(70,75,230,75,230-(15/60)*s,75+s*(275/60),70+(15/60)*s,75+s*(275/60));
stroke(255,178,120); //dark orange
fill(255,203,164);
ellipse (width/2, 75+(275/60)*s, 160-(0.5)*s, 60);
}
function drawCup(){
// bigger circle
fill(224,255,255); //light cyan
ellipse (width/2, 75 , 160, 60);
// smaller circle
push();
if (hour()<=12){
fill(255,203,164);
}
else if (hour()>12){
fill(247,231,200);
}
ellipse (width/2, 350, 130, 50);
pop();
//connecting lines
line (70, 75, 85, 350);
line (230, 75, 215, 350);
}
function drawStraw(){
// straw body
push();
fill(0,191,255,50); //sky blue
rect(140,30,20,300);
pop();
// straw holes
ellipse(width/2,30,20,10);
ellipse(width/2,330,20,10);
fill(224,255,255); //light cyan
arc (width/2, 75 , 160, 60, 0, PI, OPEN);
}
// number of jelly inside the cup represents the hour
function drawJelly(){
for (var i=0; i<(hour()%12-1); i++) {
push();
translate(x[i],y[i]);
rotate(radians(angle[i]));
noStroke();
fill(249,246,231,150); //lychee
rect (0,0,10,30); //lychee jelly
pop();
}
}
// jelly inside the straw moves up per minute
function strawJelly(m){
noStroke();
fill(255,255,142);
rect (width/2-5,300-(225/60)*m,10,30);
}
// milk inside the cup shrinks per sec
function drawMilk(s){
stroke(236,220,194); //dark milk color
fill(247,231,200); //milk color
quad(70,75,230,75,215,350,85,350); //juice
//cover
fill(255);
quad(70,75,230,75,230-(15/60)*s,75+s*(275/60),70+(15/60)*s,75+s*(275/60));
fill(247,231,200); //milk color
ellipse (width/2, 75+(275/60)*s, 160-(0.5)*s, 60);
}
// number of boba inside the cup & straw represents the hour
function drawBoba(){
for (var i=0; i<(hour()%12-1); i++) {
push();
stroke(70, 51, 51,100); //darker brown
fill(88,59,57,150); //dark brown
ellipse (x[i],y[i],20,20); //boba
pop();
}
}
// boba inside the straw moves up per minute
function strawBoba(m){
noStroke();
fill(88,59,57);
ellipse (width/2,300-(225/60)*m,20,20);
}
Looking Outwards 06: Randomness
I am inspired by the project, Gush by Adam Ferriss. This piece of digital, algorithmic art is created by the random noise function called Perlin noise. The noise is a pseudo-random function for generating values, which are deterministic sequences and reproducible. The function adds realistic randomness to the color and composition of the piece. Ferriss still has control over some parameters such as the speed of the color change, allowing him to generate images with gradual gradients and in turn, create crazy psychedelic transitions. What I admire about this project is that the piece is not entirely automated, while image-making itself is. Ferriss’s artistic sensibilities could be still manifested in the final piece as he adjusts the parameter’s values until he gets an aesthetically satisfying and completely new type of work.
https://www.itsnicethat.com/articles/adam-ferriss
https://www.itsnicethat.com/articles/adam-ferriss
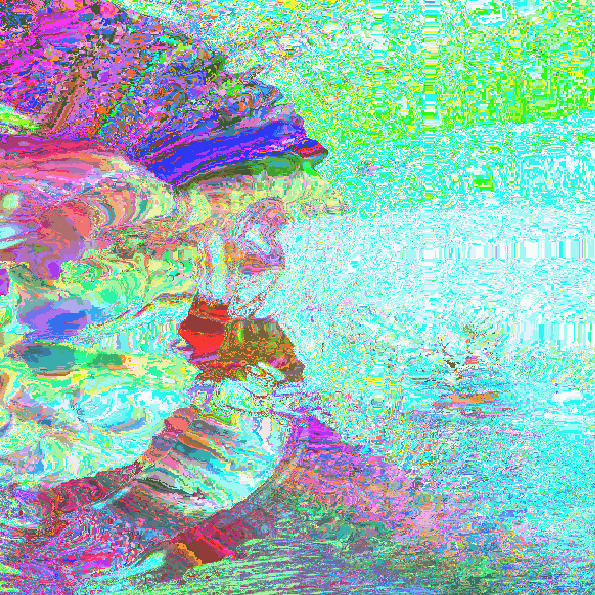
Project 5: Wallpaper
//Alicia Kim
//Section B
//yoonbink@andrew.cmu.edu
//Project-05
function setup() {
createCanvas(420, 420);
background(175,238,238);
}
function draw() {
for (r=0;r<8;r++){
for(c=0;c<8;c++){
if (r%2==0 & c%2==0){
drawFlower(r*60,c*60,255,209,193);
}
else{
drawFlower(r*60,c*60,255,250,245);
}
}
}
noLoop();
}
function drawFlower (x,y,fillR,fillG,fillB) {
// leaves
push();
noStroke();
translate(x+30,y+25);
var randomR=floor(random(360));
for (var j=0;j<3;j++){
fill(144,238,144); //light green
rotate(radians(randomR));
ellipse(13,13,10,20);
}
pop();
// stem
push();
noStroke();
translate(x+30,y+25);
var randomS=floor(random(360));
fill(60,179,113,191); //sea green
rotate(radians(randomS));
rect(13,13,12,4.5);
print(randomS);
pop();
// flower petals
push();
noStroke();
translate(x+30,y+25);
fill(fillR,fillG,fillB); // lavender blush
var petal = floor(random(3,7));
for (var i=0 ; i<petal ;i++){
ellipse(0,0,25,50);
rotate(2*PI/petal);
}
pop();
noLoop();
// circle in the middle
push();
noStroke();
fill(249,139,136); //pink
circle (x+28,y+28,20);
fill(255,209,193); //peach
circle(x+30,y+25,12);
//small circles
fill(128,128,0); //olive
circle(x+28,y+23,4);
circle(x+32,y+25,3);
circle(x+28,y+28,4.5);
circle(x+29,y+27,2.5);
circle(x+23,y+23,3);
pop();
}