Overview
It’s a device that could help and motivate you to finish your tasks and also work as a nice decorative material for your bleak walls – complete all your tasks for today and find out what constellation you got at the end!
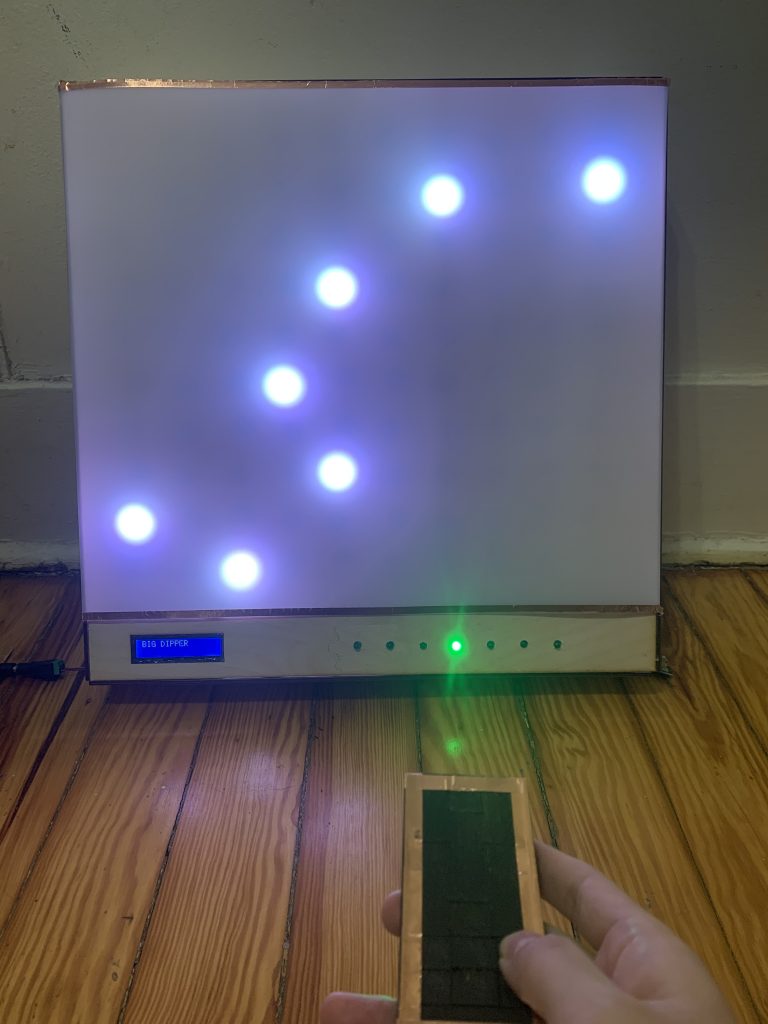
Final Model Showing Big Dipper. Notice the LCD screen showing ‘BIG DIPPER’ and the LED indicating that you have seven tasks is on. (From left to right each LED represents 4, 5, 6, 7, 8, 9, 10 tasks-to-do respectively) The final device is battery-run.
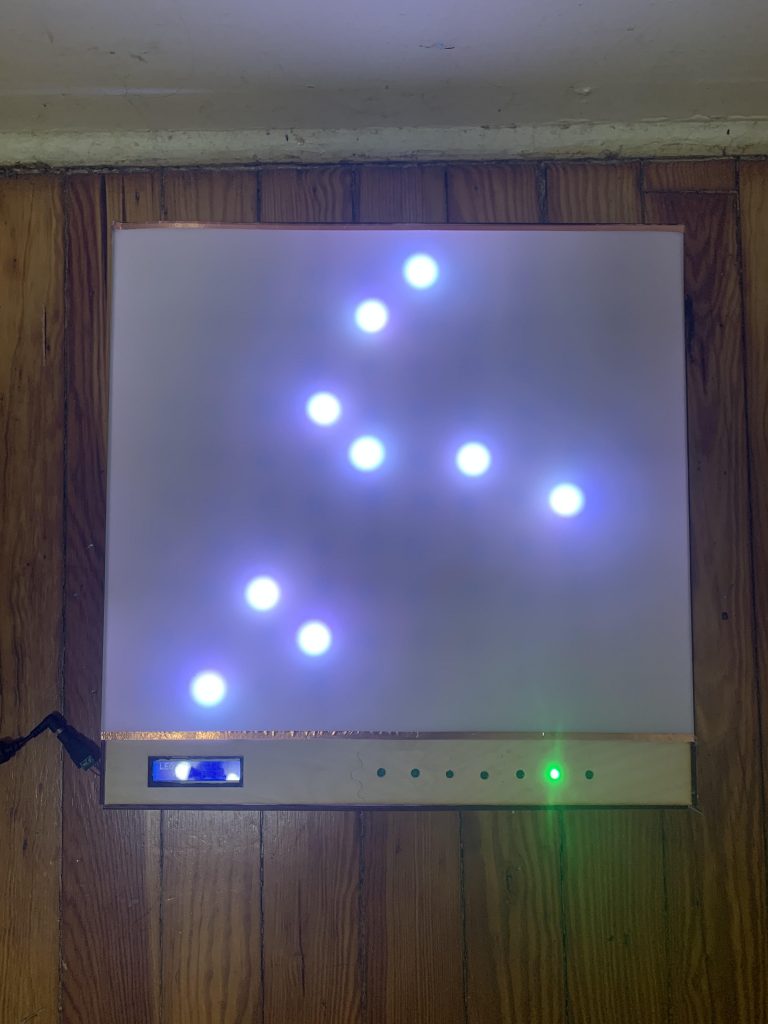
Device showing another constellation (Leo)
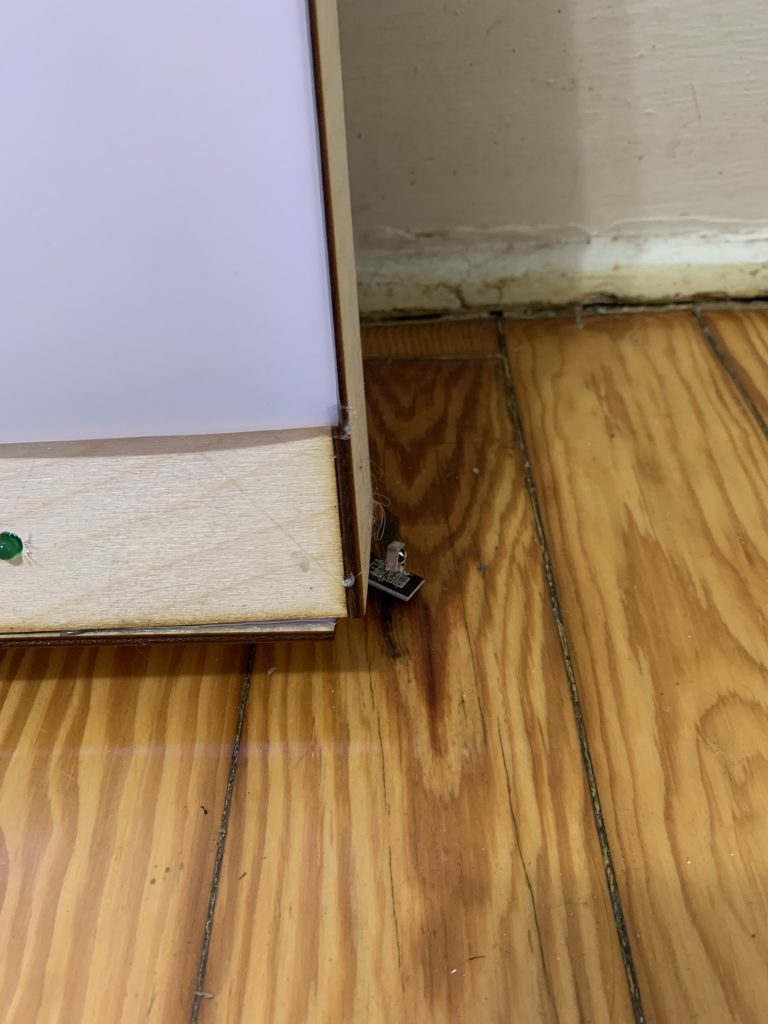
IR Remote sticking from the bottom (Works best when there’s nothing blocking it so I took it out of the casing)
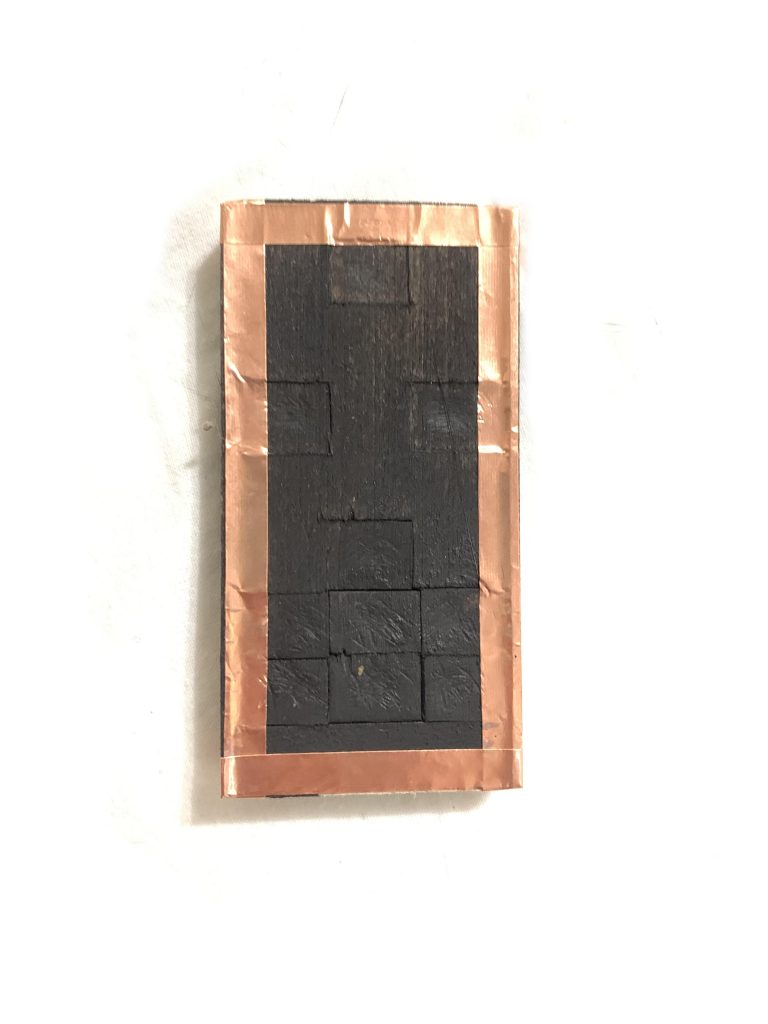
IR remote with a new casing
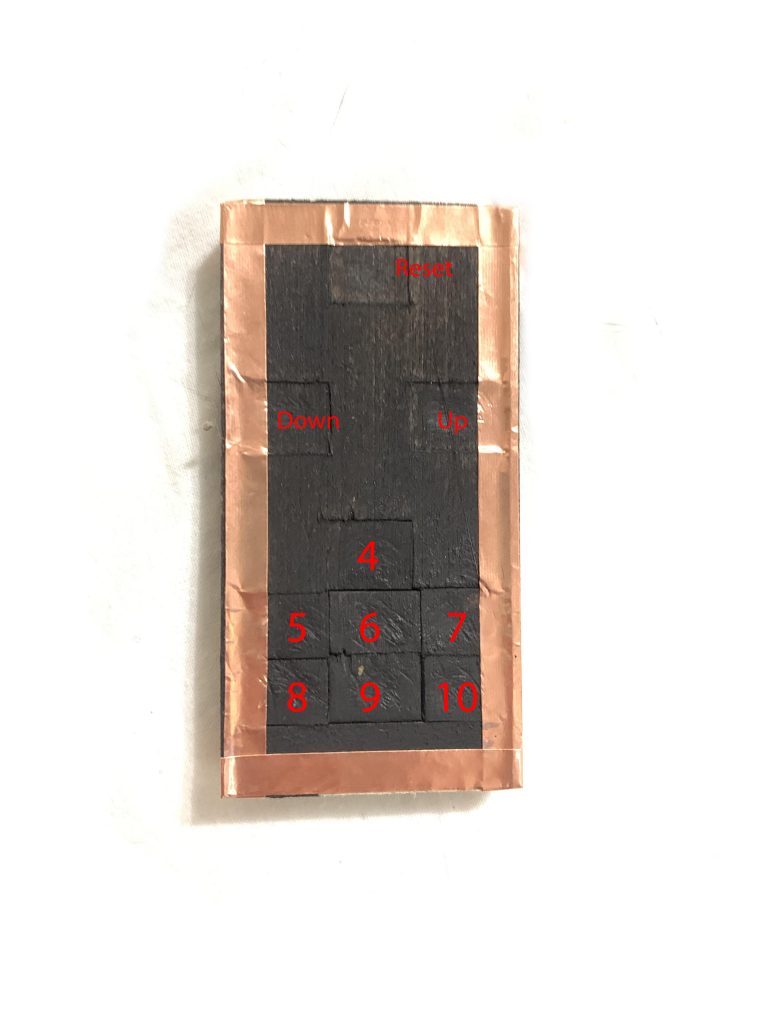
What each button indicates
Process
After some consideration, I came up with an idea to make something that would help me keep track of my tasks – but also something that would motivate me to do so. And since it had to be placed somewhere noticeable in my room, I wanted it to be aesthetically pleasing and work as a decorative material too. At the end, I came up with task-tracker that uses constellations to visualize for your processes.
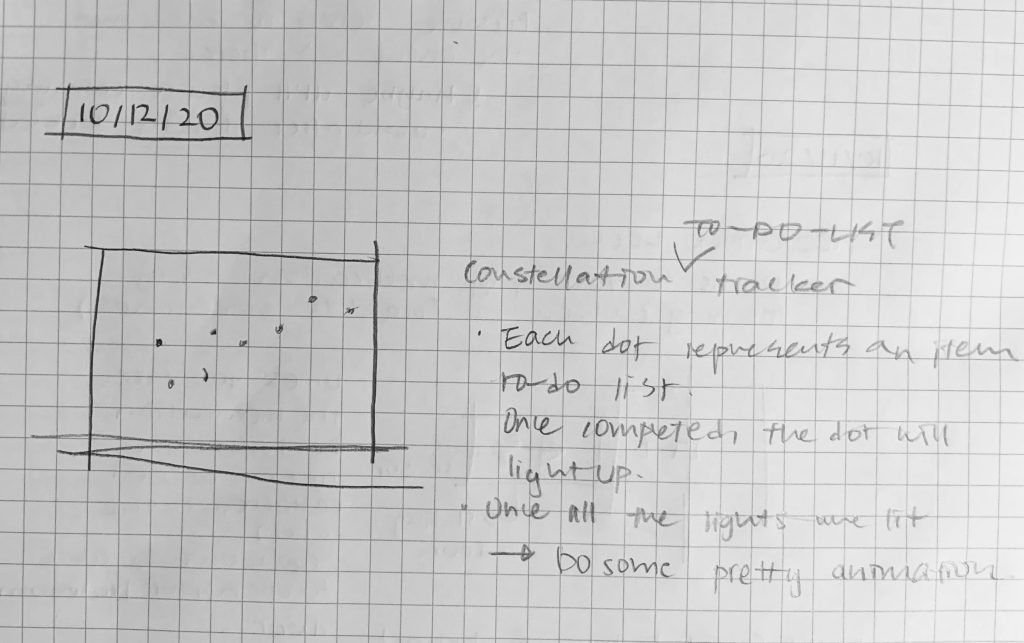
Initial Ideation
I wanted it to have an element of surprise with what constellation I would get at the end, which would require me to input a variety of constellations with a different number of stars. All these constellations have different positions for stars and I thought it would be appropriate to use an addressable LED strip instead of individual LEDs. Working with the LED strip was pretty easy since there were a lot of tutorials for how to program it. For this specific project I used the WS2811 addressable LED strip and the FastLED library.
I began prototyping by testing how I could input the fact that I have finished off a task and how that could translate to an LED being lit up. For this I used a bush button and a state-change code to light up the LED every time I pressed the button as shown in the video below.
However I thought it would be somewhat inconvenient for me to get up every time I finish a task to go find the device (that’s hanging on the wall on the opposite side of the room) and press the button, I thought it would be better if I could control it remotely. So I began looking into how to use the IR remote and receiver and the IRremote library. The library translates the signal received from the remote to a certain code and also includes a function that allows you to command what action would happen when you press a certain button on the remote. At first it wasn’t working. I wasn’t able to figure out if there was a problem in the remote, the receiver, or the library – but it worked fine after I switched to another Arduino board. The video below shows me turning the LED on and off using the IR remote.
After figuring out how to work the individual components – it was time to write the code! I made arrays for seven groups of three constellations (that have 4, 5, 6, 7, 8, 9, 10 stars) and wrote the code to store each star’s location on a 10 x 10 matrix of LED lights. And then I wrote the code to turn one star (LED) at a time using the up/down button on the remote as well as the one to turn off all the lights and reset the device using the IR remote.
After the electronics came the mechanics. I laser-cut some wood to make a casing and also a board to hold the LEDs. Each of these holes are a bit offset so that the constellations would appear more organic. I made some holes on the bottom to hold the LCD panel and the green LED lights that indicate how many tasks you have at the moment. (from left to right: 4, 5, 6, 7, 8, 9, 10) Then I put the diffusing material on top to cover up the LED’s (ended up putting two layers), connected the Arduino to a battery, placed everything in the bottom space and covered it up with another piece of wooden board.
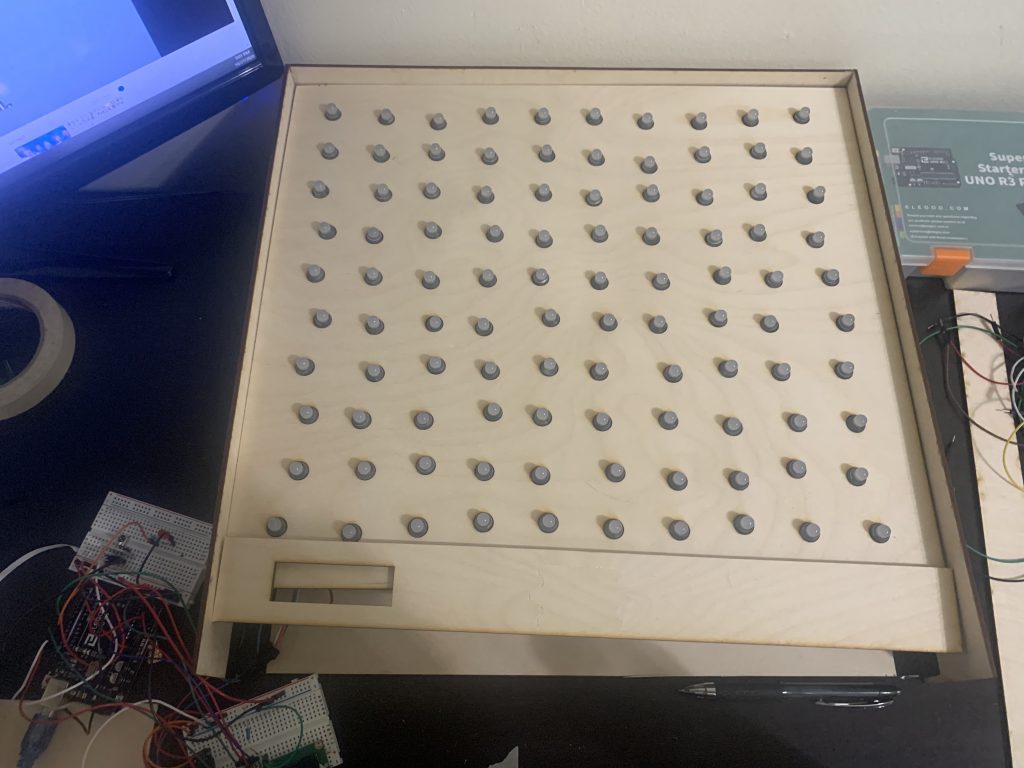
Fitting in the LED strip into the laser-cut parts.
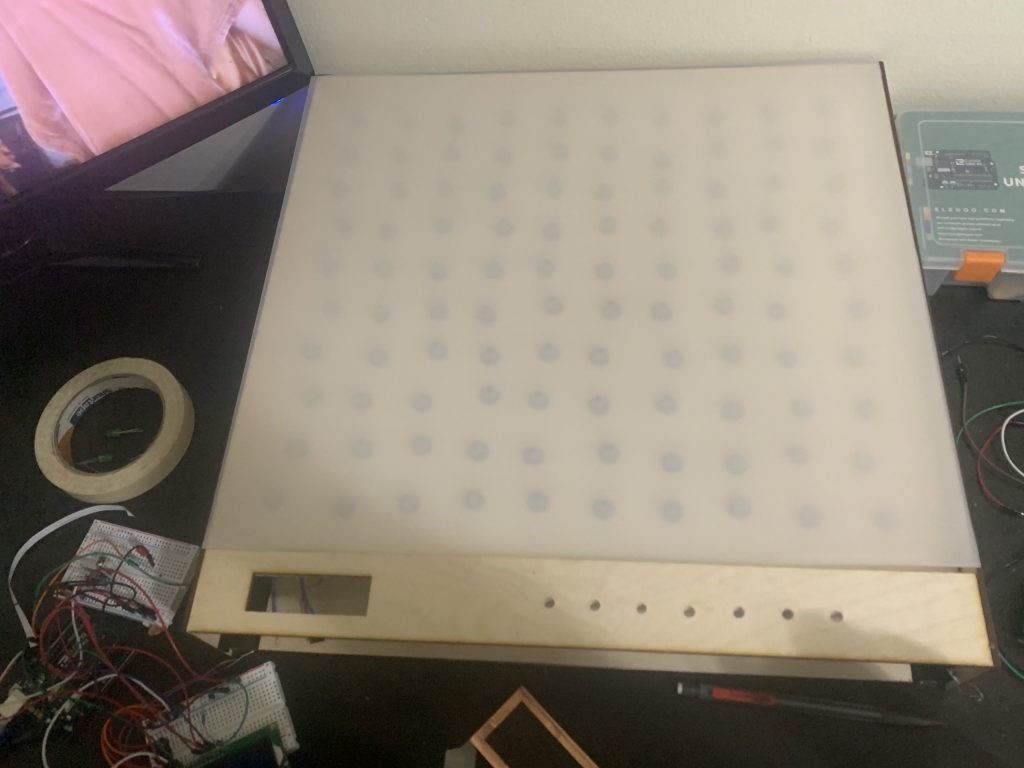
Putting the diffusing material on top
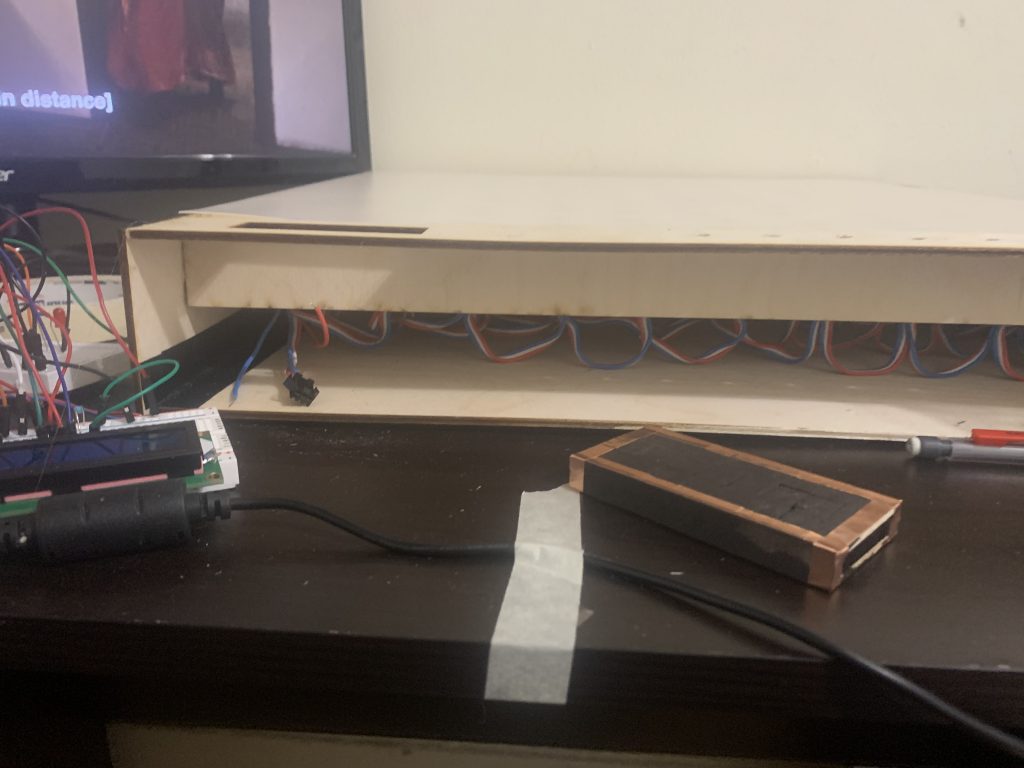
Space on the bottom where all the electronics will go into
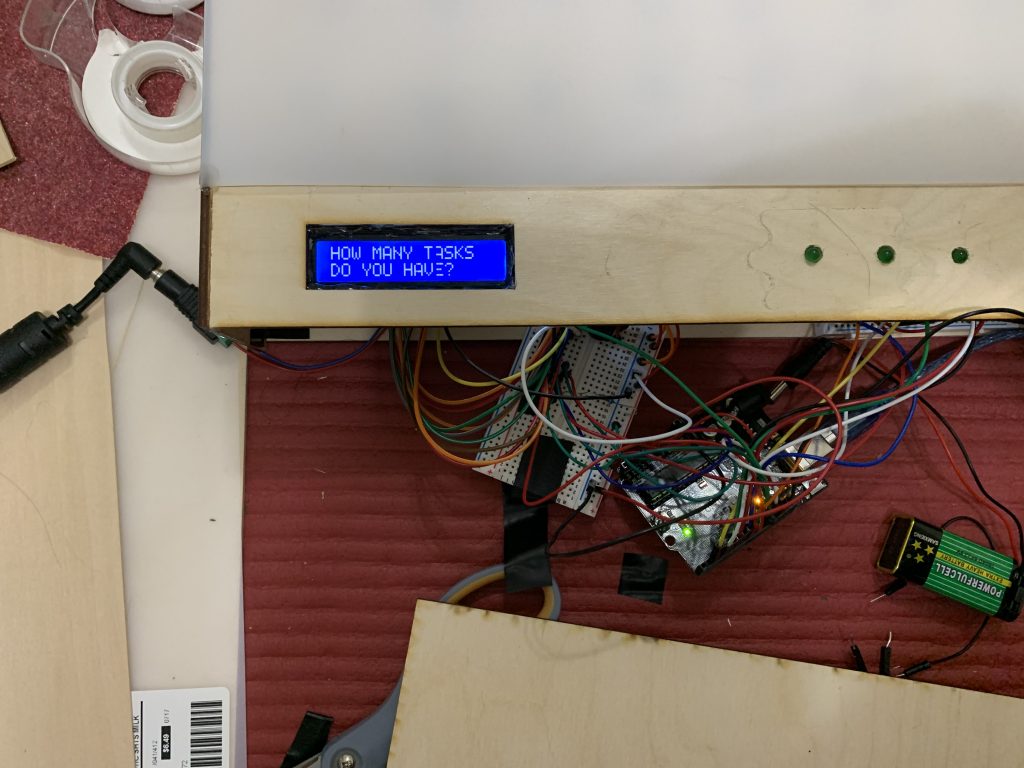
At the beginning and every time you press the reset button the LCD panel will say: HOW MANY TASKS DO YOU HAVE?
Discussion
I am pretty satisfied with the outcome of this project even though the initial concept may have lacked practicality. I was able to solve all the problems I faced and make it work exactly how I wanted it. However there were some points that were brought up during crit which I completely agree with: the diffusing material is white which is not what the night sky looks like exactly, and sometimes it is hard to even remember to turn on the light every time you finish the task. And you may want to keep up with your tasks NOT exactly because it is motivational, but because it is fun to play with (which is the reason why it would work so great with kids). I also enjoyed learning to control the LED strip and IR remote for the first time during this project. I think both of them are going to be useful tools in the future electronics project. Making the casing was also fun and I am glad that it turned out nicely at the end despite the lack of proper tools and materials (wood glue, wood finish, black fabric that I intended to use as a diffusing material at first).
There was one thing that bothered me and that is how long the code was. After completing I tried tweaking it to make it shorter but it just didn’t work the same way I wanted it to. It might be because I do not have sufficient knowledge about it. I think this a skill though that I can develop over time as I familiarize myself with coding.
As a next iteration, I was thinking about adding the IoT component to it to automate the task-tracking component and make it easier too. With the Particle Board and IFTTT I think it would be possible to link it to either your reminder app or the calendar app on your phone to update the number of tasks and keep track of them automatically even when you’re far away from the device.
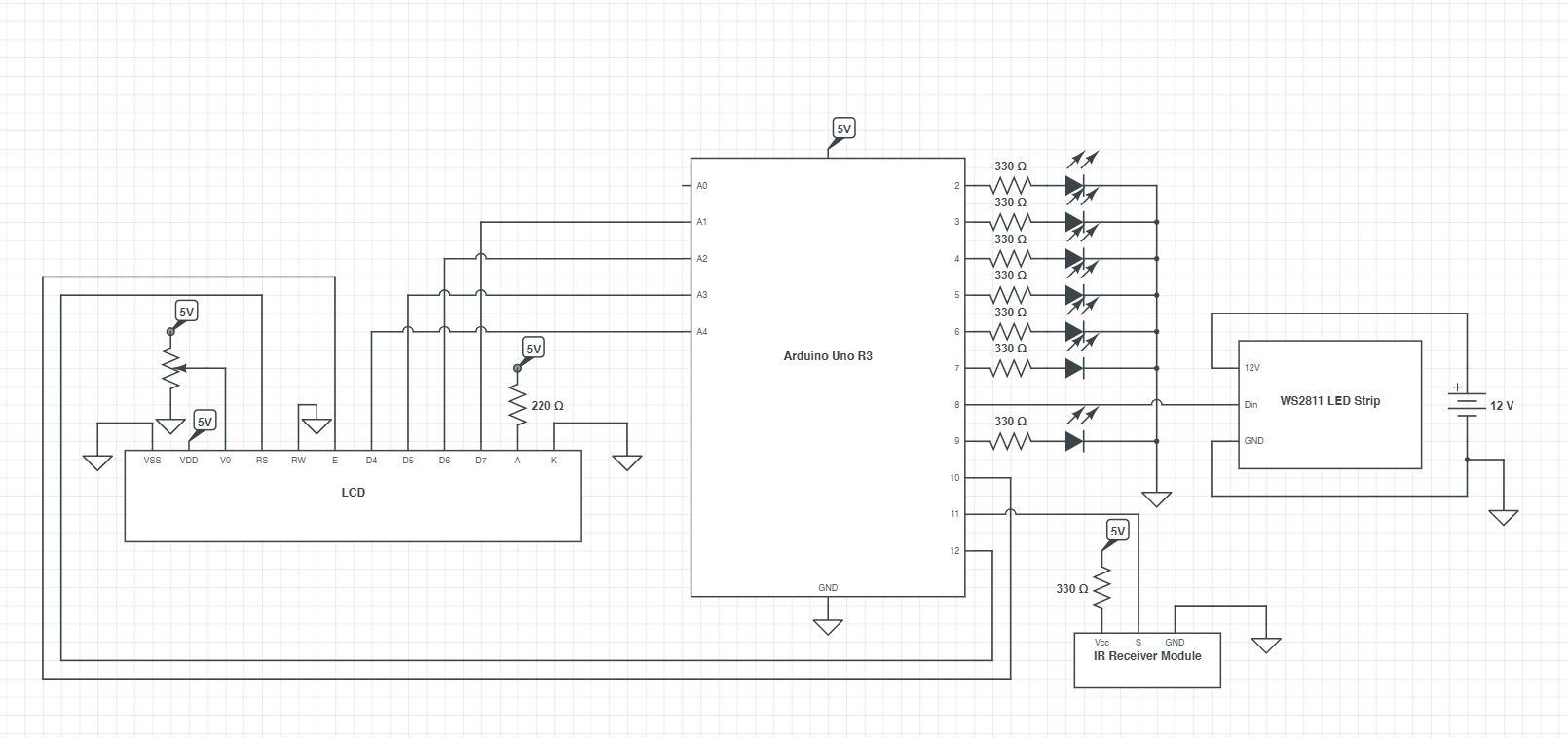
Schematic
/* * Project 2: Assistive Technology * Claire Koh * * Collaboration: FastLED tutorial (https://www.instructables.com/Basic-of-FastLED/) * IR Remote Control tutorial (https://www.circuitbasics.com/arduino-ir-remote-receiver-tutorial/) * * Challenge: Learning how to work the IR remote and the LED strip using respective libraries * that were both new to me. * * Next Time: I would change the diffusing material to black so it would have more of the 'night sky' feeling. * Also I hope once I learn more about coding I could figure out how to shorten this code.. which is very very long. * * * Description: It helps you keep track of the tasks you have finished and also motivates you to do so * by making a complete constellation at the end. * First you start by inputting the number of tasks you have - you can do that by clicking the corresponding * button on the IR remote (4,5,6,7,8,9,10) * Then Arduino will pick a random constellation whose number of stars matches the input number of tasks. * Every time you finish a task you press the up button and a star will light up. You can press the down button * to take it back if you've pressed the up button by accident. * Once you've completed all the tasks the constellation will be complete - and it will do a little animation. * You can go to sleep by looking at the constellation you've completed and feeling accomplished. * It could also work as a general wall art. * * Pin Mapping: * * pin | mode | description * ----|------|------------- * 2 OUTPUT Task indicator LED (10) * 3 OUTPUT Task indicator LED (9) * 4 OUTPUT Task indicator LED (8) * 5 OUTPUT Task indicator LED (7) * 6 OUTPUT Task indicator LED (6) * 7 OUTPUT Task indicator LED (5) * 8 OUTPUT To program the LED strip * 9 OUTPUT Task indicator LED (4) * 10 OUTPUT LCD Panel (EPIN) * 11 INPUT IR remote signal receiver * 12 OUTPUT LCD Panel (RSPIN) * A0 INPUT To get a random number * A1 OUTPUT LCD Panel (D7PIN) * A2 OUTPUT LCD Panel (D6PIN) * A3 OUTPUT LCD Panel (D5PIN) * A4 OUTPUT LCD Panel (D4PIN) */ #include <IRremote.h> #include <FastLED.h> #include <LiquidCrystal.h> // Signal Pin of IR receiver to Arduino Digital Pin 11 const int receiver = 11; // LED's to indicate how many tasks you have to do. const int FOURLED = 9; const int FIVELED = 7; const int SIXLED = 6; const int SEVENLED = 5; const int EIGHTLED = 4; const int NINELED = 3; const int TENLED = 2; // Parameters for the LED strip const int NUM_LEDS = 100; const int DATA_PIN = 8; // For the LCD screen const int RSPIN = 12; const int EPIN = 10; const int D4PIN = A4; const int D5PIN = A3; const int D6PIN = A2; const int D7PIN = A1; // Integer to store how many tasks you've done. int plusCount = -1; // Integers used to indicate which function is on at the moment. // At the beginning of a function one of these would be set to 1 and the rest would be 0. int booleancaelum = 0; int booleancrux = 0; int booleansagitta = 0; int booleanaries = 0; int booleancassiopeia = 0; int booleancorvus = 0; int booleancancer = 0; int booleanlyra = 0; int booleanauriga = 0; int booleanbigdipper = 0; int booleancepheus = 0; int booleancygnus = 0; int booleanorion = 0; int booleanlynx = 0; int booleanlibra = 0; int booleanleo = 0; int booleancapricorn = 0; int booleanbootes = 0; int booleangemini = 0; int booleancanismajor = 0; int booleanvirgo = 0; int LEDstate = LOW; // Arrays to store the position of leds that correspond to the position of the stars in each constellation int caelumArray[] = {82, 73, 45, 26}; int cruxArray[] = {56, 72, 84, 26}; int sagittaArray[] = {98, 54, 12, 30}; int ariesArray[] = {60, 83, 32, 12, 6}; int cassiopeiaArray[] = {79, 96, 55, 47, 8}; int corvusArray[] = {27, 47, 37, 96, 86}; int cancerArray[] = {94, 65, 54, 23, 27, 8}; int lyraArray[] = {75, 38, 23, 73, 87, 71}; int aurigaArray[] = {23, 32, 51, 87, 83, 58}; int bigdipperArray[] = {19, 2, 24, 43, 64, 86, 89}; int cepheusArray[] = {48, 47, 74, 82, 57, 23, 24}; int cygnusArray[] = {83, 64, 33, 27, 41, 72, 88}; int orionArray[] = {2, 43, 82, 94, 73, 54, 13, 44}; int lynxArray[] = {1, 18, 37, 35, 54, 53, 87, 90}; int libraArray[] = {59, 57, 63, 95, 88, 48, 26, 13}; int leoArray[] = {48, 16, 1, 22, 53, 55, 63, 84, 94}; int capricornArray[] = {33, 26, 37, 60, 61, 63, 65, 71, 88}; int bootesArray[] = {29, 31, 26, 54, 84, 97, 78, 43, 5}; int geminiArray[] = {80, 61, 75, 85, 89, 69, 35, 22, 18, 2}; int canismajorArray[] = {20, 38, 22, 3, 25, 55, 73, 84, 93, 69}; int virgoArray[] = {42, 75, 53, 34, 15, 40, 1, 96, 68, 90}; // Pin to get a random value. Will be used to assign a random constellation. const int RANDOMPIN = A0; IRrecv irrecv(receiver); // create instance of 'irrecv' decode_results results; // create instance of 'decode_results' CRGB leds[NUM_LEDS]; LiquidCrystal lcd(RSPIN,EPIN,D4PIN,D5PIN,D6PIN,D7PIN); void setup() { pinMode(FOURLED, OUTPUT); pinMode(FIVELED, OUTPUT); pinMode(SIXLED, OUTPUT); pinMode(SEVENLED, OUTPUT); pinMode(EIGHTLED, OUTPUT); pinMode(NINELED, OUTPUT); pinMode(TENLED, OUTPUT); pinMode(RANDOMPIN, INPUT); randomSeed(analogRead(RANDOMPIN)); FastLED.addLeds<WS2811, DATA_PIN, RGB>(leds, NUM_LEDS); // Start the LED strip irrecv.enableIRIn(); // Start the receiver Serial.begin(9600); allOff(); } void loop() { if (irrecv.decode(&results)) { // Have we received an IR signal? translateIR(); // Then translate the signal to the corresponding code. irrecv.resume(); // And then receive the next value FastLED.show(); } } // Takes action based on IR code received // Describing Remote IR codes void translateIR() { int randomnumber; switch(results.value) { case 0xFF18E7: // Will be used as "4" Button allOff(); digitalWrite(FOURLED, HIGH); LEDstate = HIGH; randomnumber = random(0,3); if (randomnumber == 0) { caelum(); } if (randomnumber == 1) { crux(); } if (randomnumber == 2) { sagitta(); } FastLED.show(); break; case 0xFF10EF: Serial.println("4"); // Will be used as "5" Button allOff(); digitalWrite(FIVELED, HIGH); LEDstate = HIGH; randomnumber = random(0,3); if (randomnumber == 0) { aries(); } if (randomnumber == 1) { cassiopeia(); } if (randomnumber == 2) { corvus(); } FastLED.show(); break; case 0xFF38C7: Serial.println("5"); // Will be used as "6" Button allOff(); digitalWrite(SIXLED, HIGH); LEDstate = HIGH; randomnumber = random(0,3); if (randomnumber == 0) { cancer(); } if (randomnumber == 1) { lyra(); } if (randomnumber == 2) { auriga(); } FastLED.show(); break; case 0xFF5AA5: Serial.println("6"); // Will be used as "7" Button allOff(); digitalWrite(SEVENLED, HIGH); LEDstate = HIGH; randomnumber = random(0,3); if (randomnumber == 0) { bigdipper(); } if (randomnumber == 1) { cepheus(); } if (randomnumber == 2) { cygnus(); } FastLED.show(); break; case 0xFF42BD: Serial.println("7"); // Will be used as "8" Button allOff(); digitalWrite(EIGHTLED, HIGH); LEDstate = HIGH; randomnumber = random(0,3); if (randomnumber == 0) { orion(); } if (randomnumber == 1) { lynx(); } if (randomnumber == 2) { libra(); } FastLED.show(); break; case 0xFF4AB5: Serial.println("8"); // Will be used as "9" Button allOff(); digitalWrite(NINELED, HIGH); LEDstate = HIGH; randomnumber = random(0,3); if (randomnumber == 0) { leo(); } if (randomnumber == 1) { capricorn(); } if (randomnumber == 2) { bootes(); } FastLED.show(); break; case 0xFF52AD: Serial.println("9"); // Will be used as "10" Button allOff(); digitalWrite(TENLED, HIGH); LEDstate = HIGH; randomnumber = random(0,3); if (randomnumber == 0) { gemini(); } if (randomnumber == 1) { canismajor(); } if (randomnumber == 2) { virgo(); } FastLED.show(); break; case 0xFF629D: Serial.println("VOL+"); // Would be used as a reset button allOff(); digitalWrite(FOURLED, LOW); digitalWrite(FIVELED, LOW); digitalWrite(SIXLED, LOW); digitalWrite(SEVENLED, LOW); digitalWrite(EIGHTLED, LOW); digitalWrite(NINELED, LOW); digitalWrite(TENLED, LOW); plusCount = -1; Serial.println("reset"); Serial.println(plusCount); break; // Following case is used as an up button // It will light up one LED at a time for the called function case 0xFF906F: Serial.println("UP"); plusCount ++; Serial.println(plusCount); if (booleancaelum == 1) { leds[caelumArray[plusCount]] = CRGB(255,255,255); FastLED.show(); caelum(); } if (booleancrux== 1) { leds[cruxArray[plusCount]] = CRGB(255,255,255); FastLED.show(); crux(); } if (booleansagitta == 1) { leds[sagittaArray[plusCount]] = CRGB(255,255,255); FastLED.show(); sagitta(); } if (booleanaries == 1) { leds[ariesArray[plusCount]] = CRGB(255,255,255); FastLED.show(); aries(); } if (booleancassiopeia == 1) { leds[cassiopeiaArray[plusCount]] = CRGB(255,255,255); FastLED.show(); cassiopeia(); } if (booleancorvus == 1) { leds[corvusArray[plusCount]] = CRGB(255,255,255); FastLED.show(); corvus(); } if (booleancancer == 1) { leds[cancerArray[plusCount]] = CRGB(255,255,255); FastLED.show(); cancer(); } if (booleanlyra == 1) { leds[lyraArray[plusCount]] = CRGB(255,255,255); FastLED.show(); lyra(); } if (booleanauriga == 1) { leds[aurigaArray[plusCount]] = CRGB(255,255,255); FastLED.show(); auriga(); } if (booleanbigdipper == 1) { leds[bigdipperArray[plusCount]] = CRGB(255,255,255); FastLED.show(); bigdipper(); } if (booleancepheus == 1) { leds[cepheusArray[plusCount]] = CRGB(255,255,255); FastLED.show(); cepheus(); } if (booleancygnus == 1) { leds[cygnusArray[plusCount]] = CRGB(255,255,255); FastLED.show(); cygnus(); } if (booleanorion == 1) { leds[orionArray[plusCount]] = CRGB(255,255,255); FastLED.show(); orion(); } if (booleanlynx == 1) { leds[lynxArray[plusCount]] = CRGB(255,255,255); FastLED.show(); lynx(); } if (booleanlibra == 1) { leds[libraArray[plusCount]] = CRGB(255,255,255); FastLED.show(); libra(); } if (booleanleo == 1) { leds[leoArray[plusCount]] = CRGB(255,255,255); FastLED.show(); leo(); } if (booleancapricorn == 1) { leds[capricornArray[plusCount]] = CRGB(255,255,255); FastLED.show(); capricorn(); } if (booleanbootes == 1) { leds[bootesArray[plusCount]] = CRGB(255,255,255); FastLED.show(); bootes(); } if (booleangemini== 1) { leds[geminiArray[plusCount]] = CRGB(255,255,255); FastLED.show(); gemini(); } if (booleancanismajor == 1) { leds[canismajorArray[plusCount]] = CRGB(255,255,255); FastLED.show(); canismajor(); } if (booleanvirgo== 1) { leds[virgoArray[plusCount]] = CRGB(255,255,255); FastLED.show(); virgo(); } break; // Does the opposite of the UP button case 0xFFE01F: Serial.println("DOWN"); if (booleancaelum == 1) { leds[caelumArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; caelum(); } if (booleancrux == 1) { leds[cruxArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; crux(); } if (booleansagitta == 1) { leds[sagittaArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; sagitta(); } if (booleanaries == 1) { leds[ariesArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; aries(); } if (booleancassiopeia == 1) { leds[cassiopeiaArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; cassiopeia(); } if (booleancorvus == 1) { leds[corvusArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; corvus(); } if (booleancancer == 1) { leds[cancerArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; cancer(); } if (booleanlyra == 1) { leds[lyraArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; lyra(); } if (booleanauriga == 1) { leds[aurigaArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; auriga(); } if (booleanbigdipper == 1) { leds[bigdipperArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; bigdipper(); } if (booleancepheus == 1) { leds[cepheusArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; cepheus(); } if (booleancygnus == 1) { leds[cygnusArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; cygnus(); } if (booleanorion == 1) { leds[orionArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; orion(); } if (booleanlynx == 1) { leds[lynxArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; lynx(); } if (booleanlibra == 1) { leds[libraArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; libra(); } if (booleanleo == 1) { leds[leoArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; leo(); } if (booleancapricorn == 1) { leds[capricornArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; capricorn(); } if (booleanbootes == 1) { leds[bootesArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; bootes(); } if (booleangemini == 1) { leds[geminiArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; gemini(); } if (booleancanismajor == 1) { leds[canismajorArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; canismajor(); } if (booleanvirgo == 1) { leds[virgoArray[plusCount]] = CRGB(0,0,0); FastLED.show(); plusCount = plusCount -1; virgo(); } break; } delay(200); // Do not get immediate repeat FastLED.show(); } //END translateIR // Function to turn off all LED's and reset the count numbers to -1 void allOff() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("HOW MANY TASKS"); lcd.setCursor(0,2); lcd.print("DO YOU HAVE?"); plusCount = -1; for (int i = 0; i <=NUM_LEDS; i++) { leds[i] = CRGB(0,0,0); } digitalWrite(FOURLED, LOW); digitalWrite(FIVELED, LOW); digitalWrite(SIXLED, LOW); digitalWrite(SEVENLED, LOW); digitalWrite(EIGHTLED, LOW); digitalWrite(NINELED, LOW); digitalWrite(TENLED, LOW); FastLED.show(); } // From here will be functions to store the constellations void caelum() { booleancaelum = 1; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==3) { leds[caelumArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); caelumComplete(); FastLED.show(); } } void caelumComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("CAELUM"); for (int i = 0; i<=3; i++) { leds[caelumArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[caelumArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void crux() { booleancaelum = 0; booleancrux = 1; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==3) { leds[cruxArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); cruxComplete(); FastLED.show(); } } void cruxComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("CRUX"); for (int i = 0; i<=3; i++) { leds[cruxArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[cruxArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void sagitta() { booleancaelum = 0; booleancrux = 0; booleansagitta = 1; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==3) { leds[sagittaArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); sagittaComplete(); FastLED.show(); } } void sagittaComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("SAGITTA"); for (int i = 0; i<=3; i++) { leds[sagittaArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[sagittaArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void aries() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 1; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==4) { leds[ariesArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); ariesComplete(); FastLED.show(); } } void ariesComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("ARIES"); for (int i = 0; i<=4; i++) { leds[ariesArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[ariesArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void cassiopeia() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 1; booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==4) { leds[cassiopeiaArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); cassiopeiaComplete(); FastLED.show(); } } void cassiopeiaComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("CASSIOPEIA"); for (int i = 0; i<=4; i++) { leds[cassiopeiaArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[cassiopeiaArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void corvus() { booleancaelum =0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 1; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==4) { leds[corvusArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); corvusComplete(); FastLED.show(); } } void corvusComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("CORVUS"); for (int i = 0; i<=4; i++) { leds[corvusArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[corvusArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void cancer() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 1; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==5) { leds[cancerArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); cancerComplete(); FastLED.show(); } } void cancerComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("CANCER"); for (int i = 0; i<=5; i++) { leds[cancerArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[cancerArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void lyra() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 1; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==5) { leds[lyraArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); lyraComplete(); FastLED.show(); } } void lyraComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("LYRA"); for (int i = 0; i<=5; i++) { leds[lyraArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[lyraArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void auriga() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 1; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==5) { leds[aurigaArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); aurigaComplete(); FastLED.show(); } } void aurigaComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("AURIGA"); for (int i = 0; i<=5; i++) { leds[aurigaArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[aurigaArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void bigdipper() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 1; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==6) { leds[bigdipperArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); bigdipperComplete(); FastLED.show(); } } void bigdipperComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("BIG DIPPER"); for (int i = 0; i<=6; i++) { leds[bigdipperArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[bigdipperArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void cepheus() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 1; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==6) { leds[cepheusArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); cepheusComplete(); FastLED.show(); } } void cepheusComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("CEPHEUS"); for (int i = 0; i<=6; i++) { leds[cepheusArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[cepheusArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void cygnus() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 1; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==6) { leds[cygnusArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); cygnusComplete(); FastLED.show(); } } void cygnusComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("CYGNUS"); for (int i = 0; i<=6; i++) { leds[cygnusArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[cygnusArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void orion() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 1; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==7) { leds[orionArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); orionComplete(); FastLED.show(); } } void orionComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("ORION"); for (int i = 0; i<=7; i++) { leds[orionArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[orionArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void lynx() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 1; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==7) { leds[lynxArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); lynxComplete(); FastLED.show(); } } void lynxComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("LIBRA"); for (int i = 0; i<=7; i++) { leds[lynxArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[lynxArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void libra() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 1; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==7) { leds[libraArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); libraComplete(); FastLED.show(); } } void libraComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("LIBRA"); for (int i = 0; i<=7; i++) { leds[libraArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[libraArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void leo() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 1; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==8) { leds[leoArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); leoComplete(); FastLED.show(); } } void leoComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("LEO"); for (int i = 0; i<=8; i++) { leds[leoArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[leoArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void capricorn() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 1; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==8) { leds[capricornArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); capricornComplete(); FastLED.show(); } } void capricornComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("CAPRICORN"); for (int i = 0; i<=8; i++) { leds[capricornArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[capricornArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void bootes() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 1; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==8) { leds[bootesArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); bootesComplete(); FastLED.show(); } } void bootesComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("BOOTES"); for (int i = 0; i<=8; i++) { leds[bootesArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[bootesArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void gemini() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 1; booleancanismajor = 0; booleanvirgo = 0; if (plusCount ==9) { leds[geminiArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); geminiComplete(); FastLED.show(); } } void geminiComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("GEMINI"); for (int i = 0; i<=9; i++) { leds[geminiArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[geminiArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void canismajor() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 1; booleanvirgo = 0; if (plusCount ==9) { leds[canismajorArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); canismajorComplete(); FastLED.show(); } } void canismajorComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("CANIS MAJOR"); for (int i = 0; i<=9; i++) { leds[canismajorArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[canismajorArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } } void virgo() { booleancaelum = 0; booleancrux = 0; booleansagitta = 0; booleanaries = 0; booleancassiopeia = 0;booleancorvus = 0; booleancancer = 0; booleanlyra = 0; booleanauriga = 0; booleanbigdipper = 0; booleancepheus = 0; booleancygnus = 0; booleanorion = 0; booleanlynx = 0; booleanlibra = 0; booleanleo = 0; booleancapricorn = 0; booleanbootes = 0; booleangemini = 0; booleancanismajor = 0; booleanvirgo = 1; if (plusCount ==9) { leds[virgoArray[plusCount]] = CRGB(255,255,255); plusCount = -1; FastLED.show(); delay(1000); virgoComplete(); FastLED.show(); } } void virgoComplete() { lcd.begin(16,2); lcd.setCursor(0,0); lcd.print("VIRGO"); for (int i = 0; i<=9; i++) { leds[virgoArray[i]] = CRGB(0,0,0); FastLED.show(); delay(100); leds[virgoArray[i]] = CRGB(255,255,255); delay(100); FastLED.show(); } }