A device that opens and closes the blinds automatically depending on how bright it is outside.
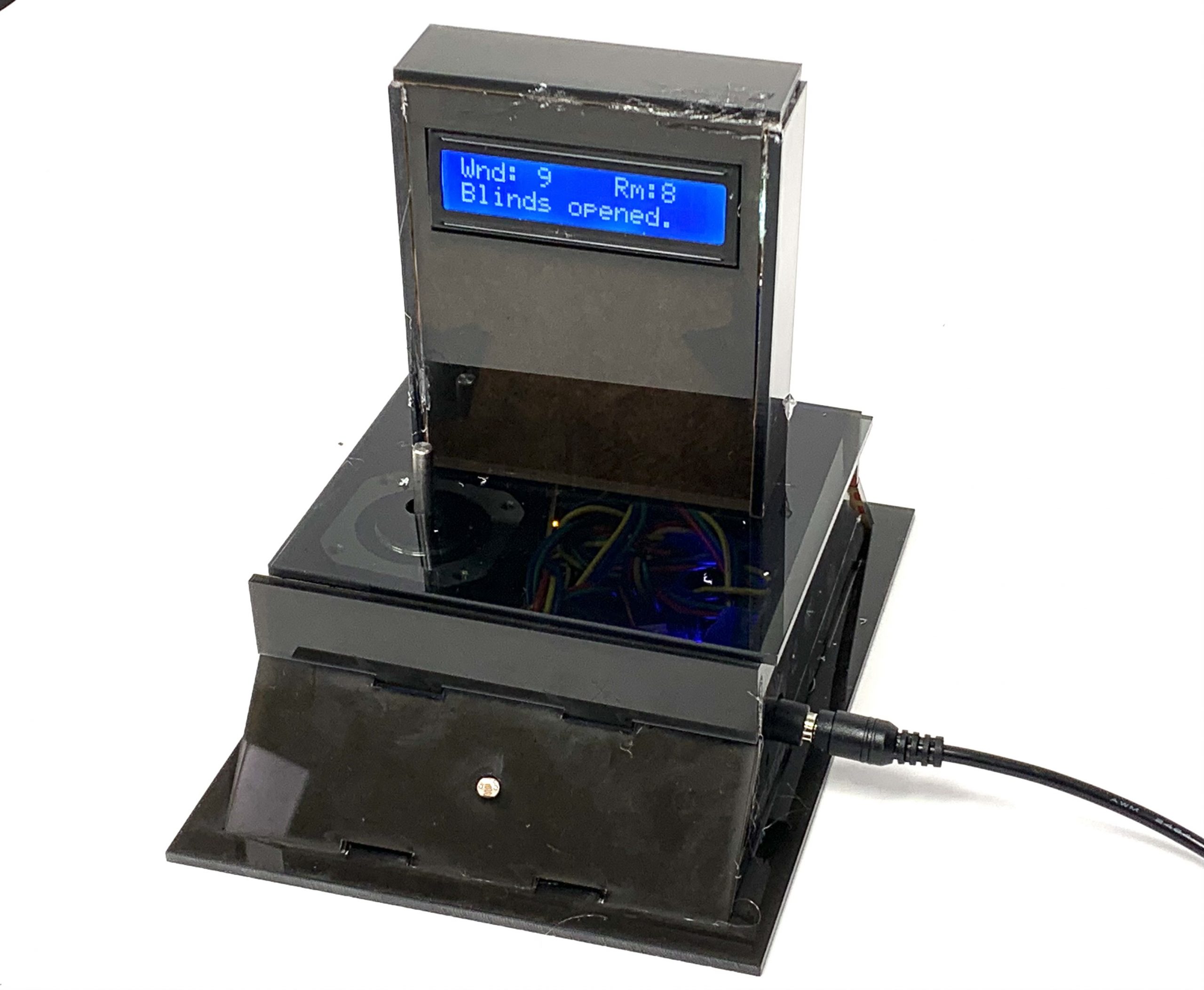
An overall view of the device.
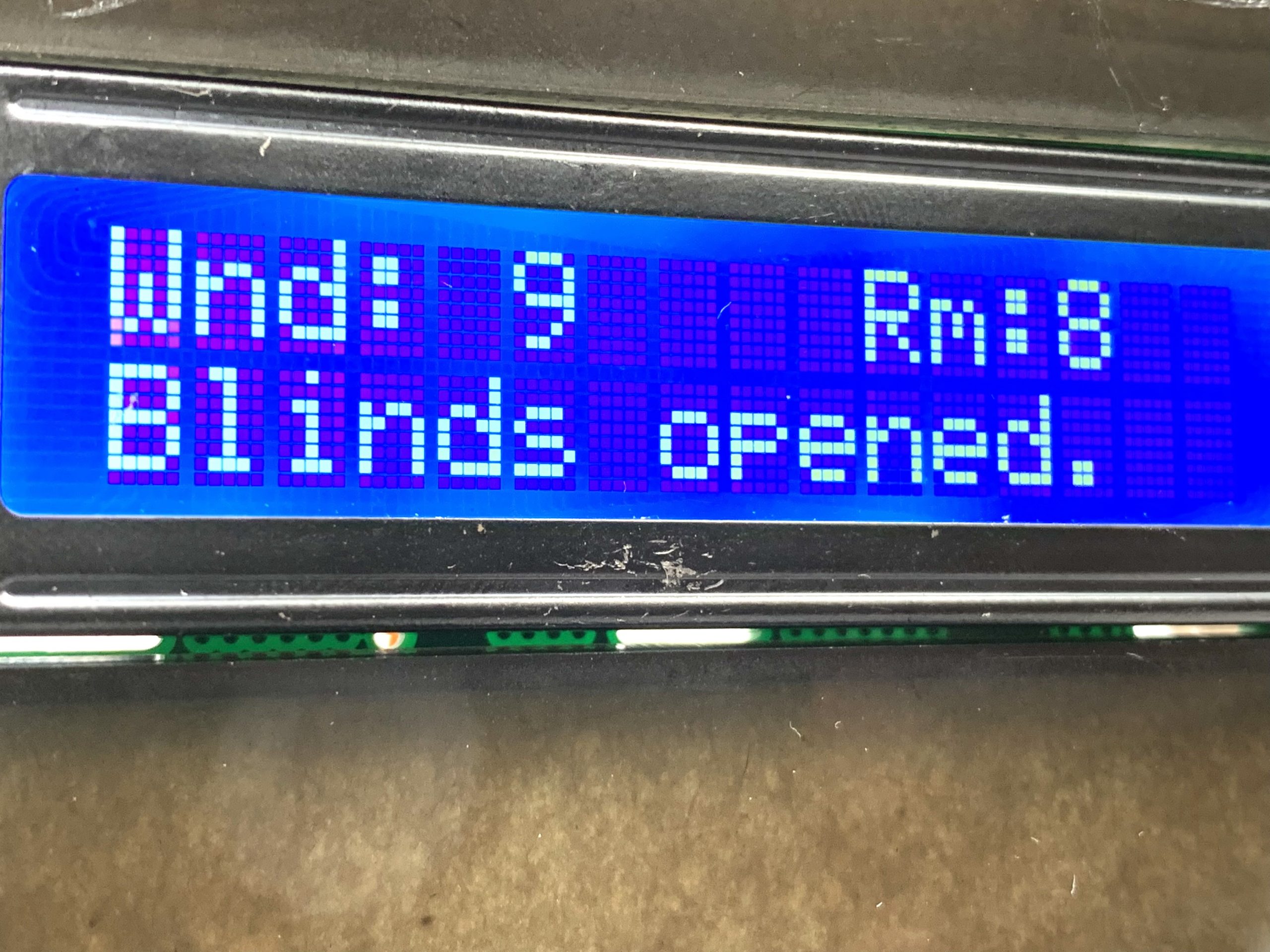
An image of the LED display when the blinds are closed.
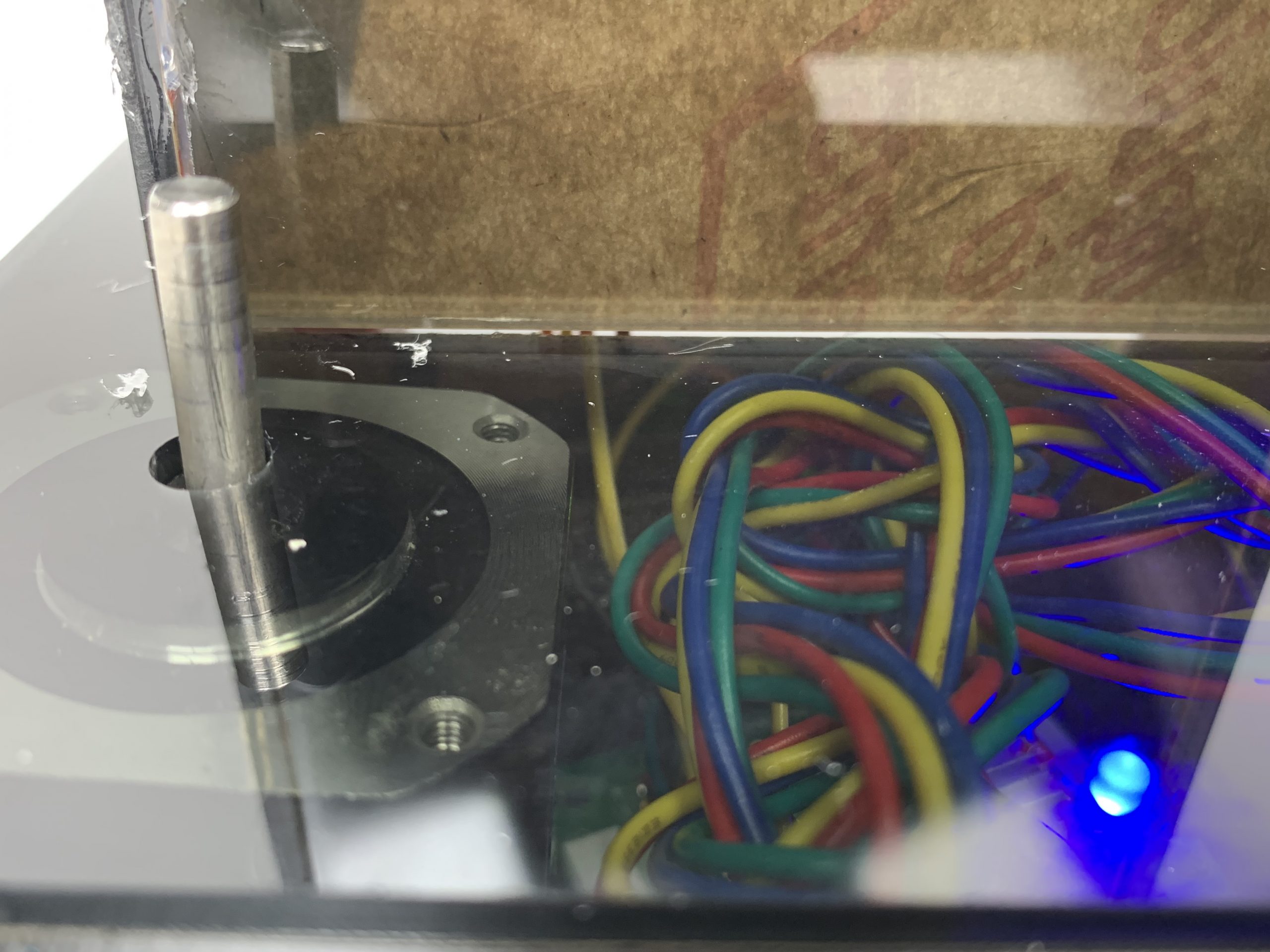
An image of some of the inner parts of the device including the motor, power light, and some wiring.
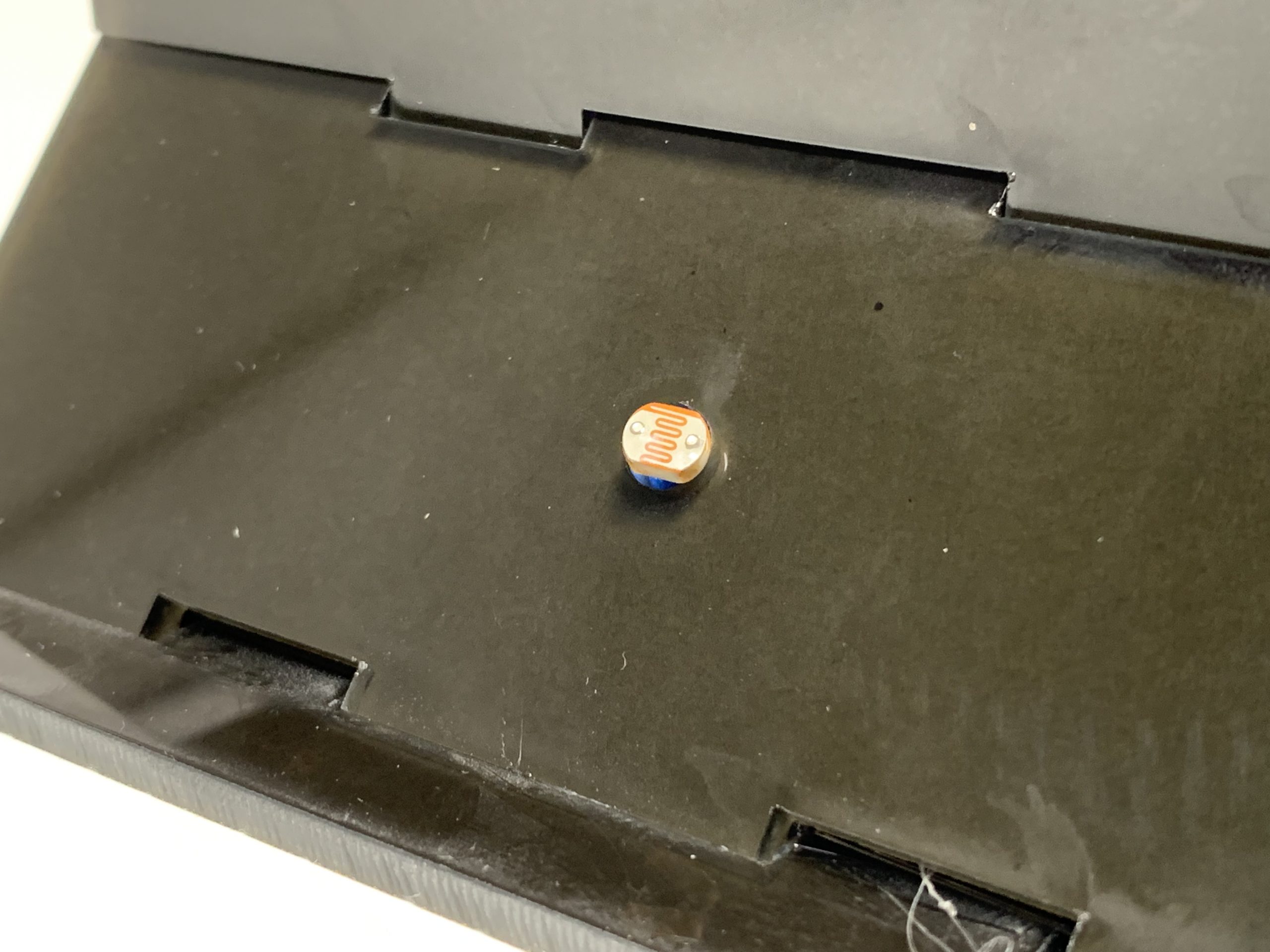
An image of the photoresistor being used to detect light on the inside of the room.
Process Images
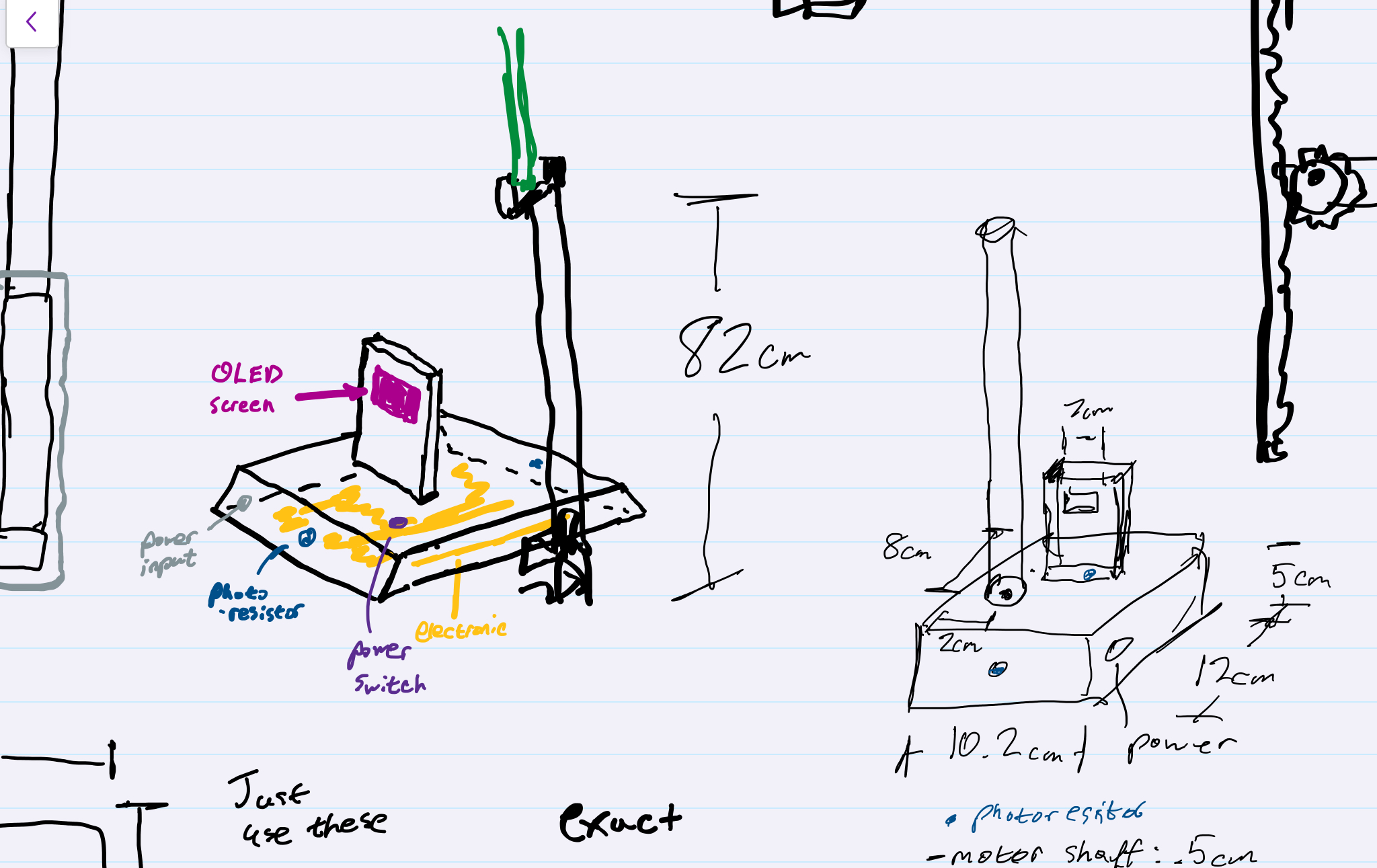
These are sketches of my device during early ideation stages. I had a few designs made, but I decided to go with the one on the left because I wanted a sophisticated look without having to build something too complex.
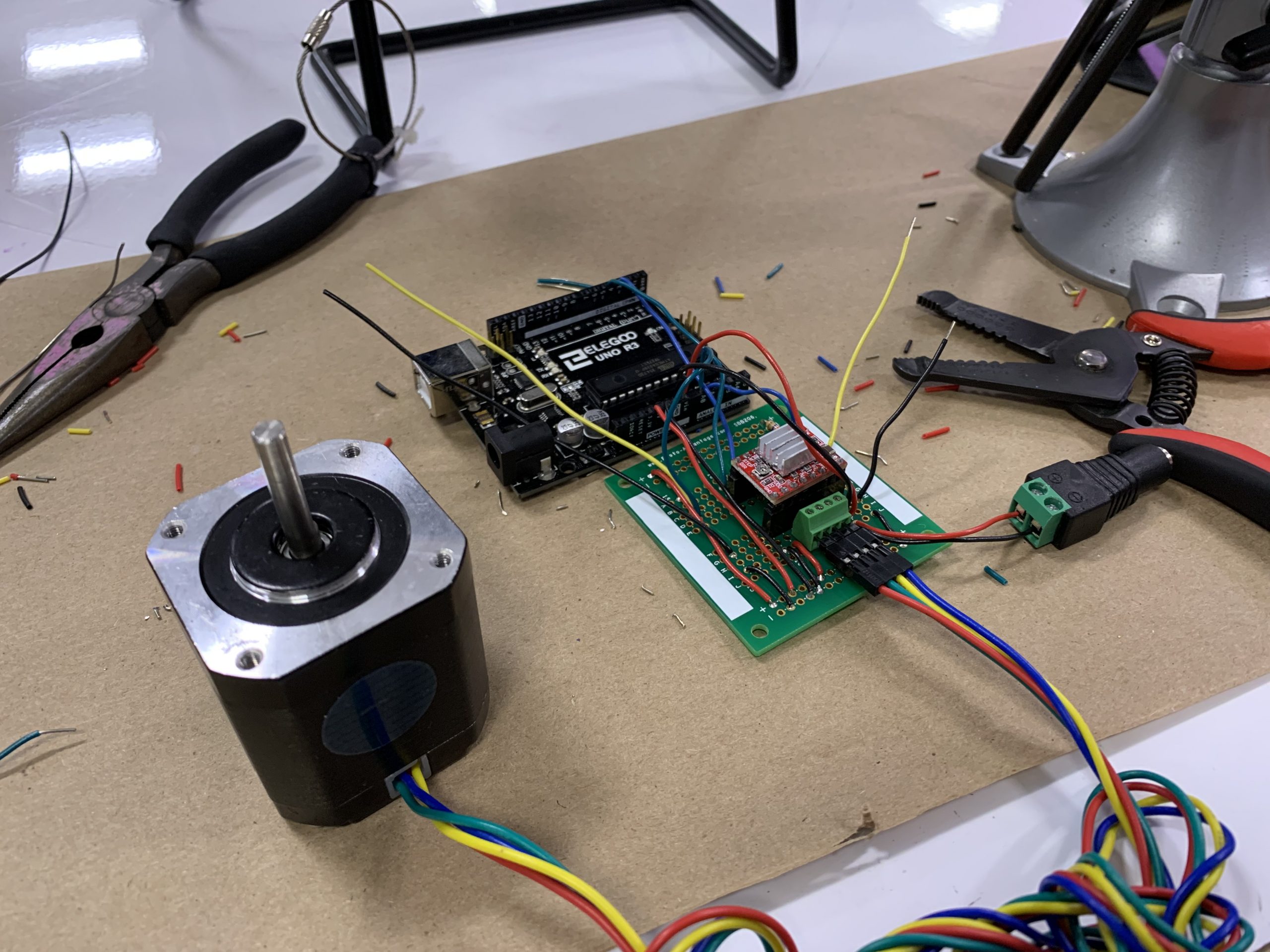
This is a picture of my electronics being soldered. I was considering using a breadboard because I knew that there were a lot of connections that had to be made, but at the same time I didn’t want anything to come undone while piecing everything together.
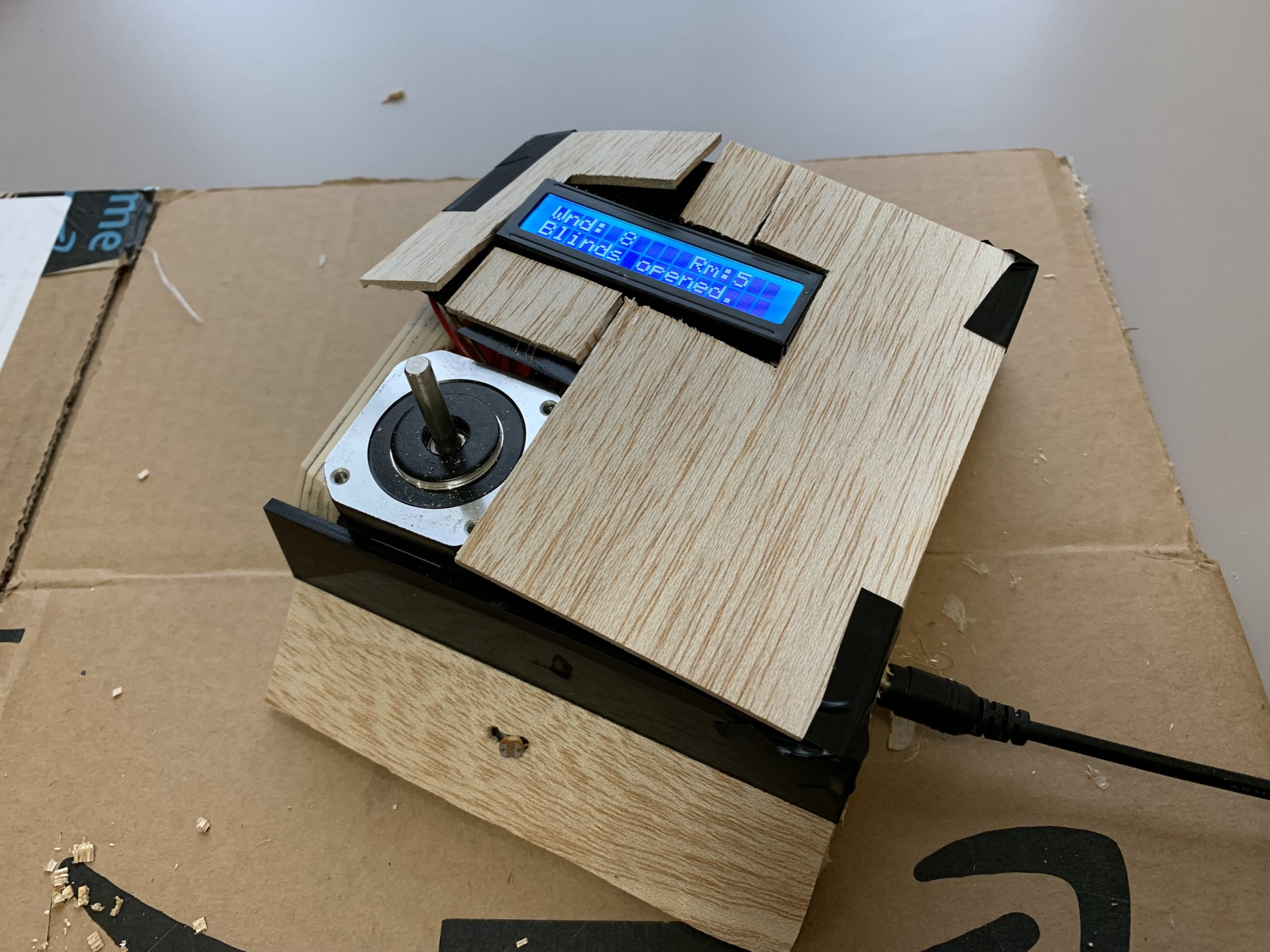
This is a picture of how my project orignially looked once I fabricated it. I had tried to form the shell by hand, but since I was inexperienced, I made a lot of mistakes that ended up having a huge effect on stability and aesthetic. I was reluctant to use a laser cutter, but it turned out for the better.
Discussion
After reflecting with my friend about all we had gone through with our projects, I realized a lot about my skills and deficiencies.
One huge realization is that I’m a lot better at conceptualizing than physically fabricating. I got supportive feedback about my functionality like, “Even though you went through a lot of design changes I think it still works well,” and, “I like the idea of contrasting light sources and rotating based on that,” but nobody commented on my structure or design except for one, “oof the wobbling stick.” One person even commented, “overall love the idea and would def purchase…,” and I genuinely think they would, just not my model. Even I could tell that my device had poor aesthetic!
Problems with electronics or programming were much more manageable than the ones that propped up during fabrication. I felt frustrated since it seemed like everyone else’s project seemed to come together cleanly while mine was a mess. I realized, however, that even though my fabrication skills weren’t where I wanted them to be, I could troubleshoot electronics and code pretty well. That was a relief for me to realize because I couldn’t celebrate the fact that my device worked since it’s look had me feeling like I completely failed.
This project has also helped me to be easier on myself. I hardly have any experience with fabrication; I hardly took any art classes where these skills were taught, I rarely ever engaged in personal projects that required this sort of skill, and I was generally unprepared for fabrication. Even now, my Mechanical Engineering curriculum is preparing me to be an extremely adept problem solver, but so far it’s been lacking in developing me as a designer/manufacturer. Regardless, I have a lot more respect for myself as a problem solver and can now see my blind spots.
If I were to build my device again, I would go with the exact same model. This time however, I would be much more intentional about assembling the parts in a way that was aesthetically pleasing. Overall though, I’m happy with the way everything turned out and I’m excited to apply what I learned to my next project.
Technical Information
Functional Block Diagram & Schematic
Code
// Automatic Blind Opener // David Oladosu // Turns a stepper motor to a certain position if the light on one // photoresistor is higher than the other. // INITIALIZE #include <Wire.h> #include <LiquidCrystal_I2C.h> #include <AccelStepper.h> const int STEP_PIN = 3; const int DIR_PIN = 4; const int WINDOWSENSOR = A0; const int ROOMSENSOR = A1; int motorPos = 0; int blindsOpen = 3; // Value that keeps track of whether or not blinds are open int currentWindowBrightness = 0; int currentRoomBrightness = 0; AccelStepper myMotor(1, STEP_PIN, DIR_PIN); LiquidCrystal_I2C screen(0x27, 16, 2); void setup() { Serial.begin(9600); pinMode(WINDOWSENSOR, INPUT); pinMode(ROOMSENSOR, INPUT); myMotor.setMaxSpeed(1000); myMotor.setAcceleration(500); screen.init(); screen.backlight(); } void loop() { int windowVal = analogRead(WINDOWSENSOR)* .78; //This value may have to be adjusted depending on the value of int roomVal = analogRead(ROOMSENSOR); //the photoresistor you use. int windowBrightness = map(windowVal, 0, 970, 9, 1); //analogRead values mean nothing, but brightness on a int roomBrightness = map(roomVal, 0, 970, 9, 1); //scale from 1-9 is useful. 1 is dark, 9 is bright. //The following conditionals have nested conditionals so that the LED screen only changes it's display when //it needs to. Otherwise, data constantly being sent to the screen slows down the stepper motor. if( roomBrightness > windowBrightness){ if( blindsOpen != 0 ){ //If blinds aren't opened, open them and run this block of code blindsOpen = 0; currentWindowBrightness = windowBrightness; currentRoomBrightness = roomBrightness; screen.clear(); screen.home(); screen.print((String) "Wnd: " + windowBrightness); screen.setCursor(10,0); screen.print((String) "Rm:" + roomBrightness); screen.setCursor(0,1); screen.print("Closing blinds"); } motorPos = 1000; myMotor.moveTo(motorPos); myMotor.run(); if (myMotor.distanceToGo() == 0){ screen.setCursor(0,1); screen.print("Blinds closed."); } } else{ if( blindsOpen != 1 ){ blindsOpen = 1; currentWindowBrightness = windowBrightness; currentRoomBrightness = roomBrightness; screen.clear(); screen.home(); screen.print((String) "Wnd: " + windowBrightness); screen.setCursor(10,0); screen.print((String) "Rm:" + roomBrightness); screen.setCursor(0,1); screen.print("Opening blinds"); } motorPos = 0; myMotor.moveTo(motorPos); myMotor.run(); if (myMotor.distanceToGo() == 0){ screen.setCursor(0,1); screen.print("Blinds opened."); } } //If the numbers on the LED display should change but they haven't, change them. if(currentWindowBrightness != windowBrightness || currentRoomBrightness != roomBrightness){ currentWindowBrightness = windowBrightness; currentRoomBrightness = roomBrightness; screen.home(); screen.print((String) "Wnd: " + windowBrightness); screen.setCursor(10,0); screen.print((String) "Rm:" + roomBrightness); } myMotor.run(); }