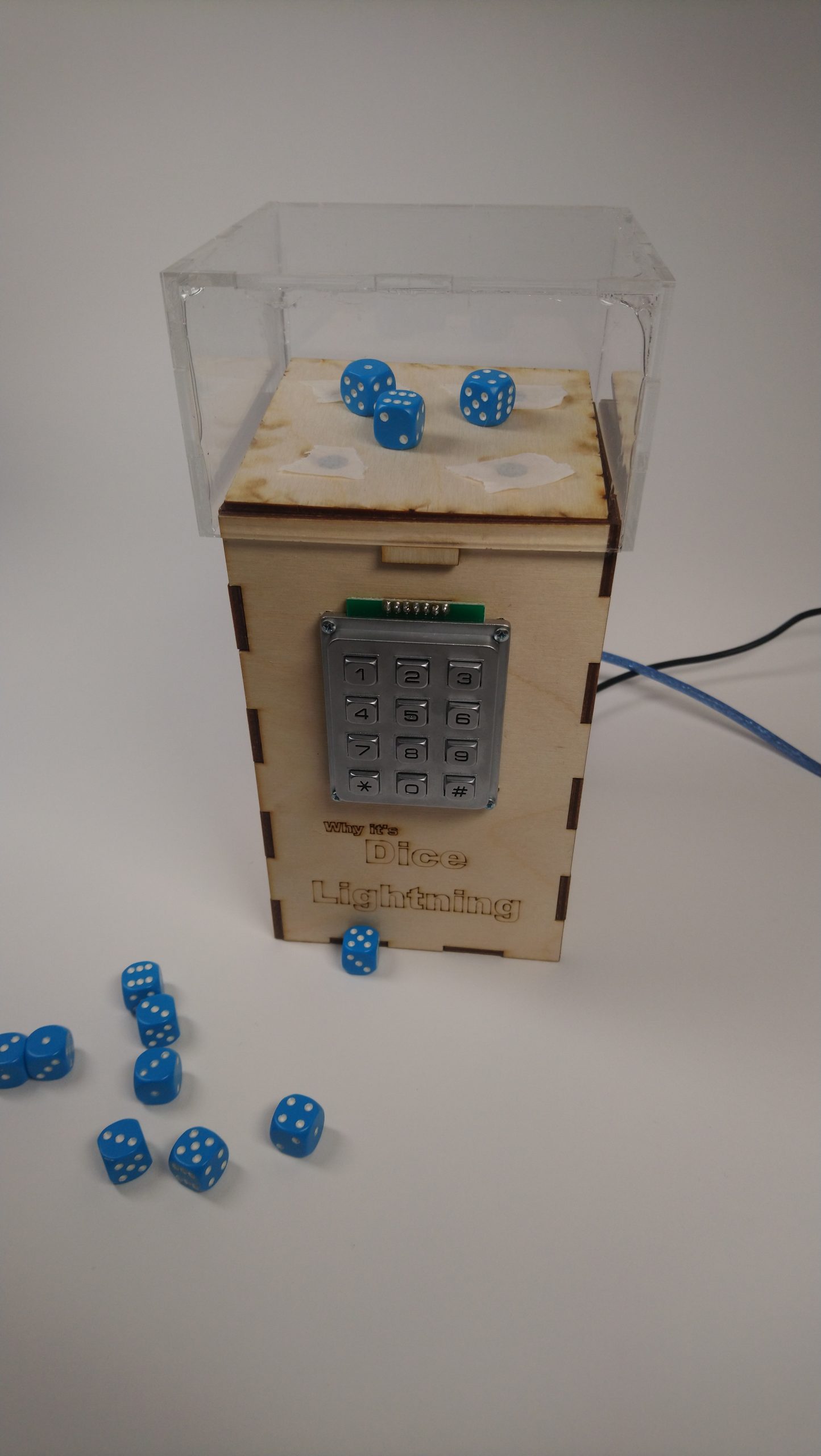
Final completed project
Highlighted Part
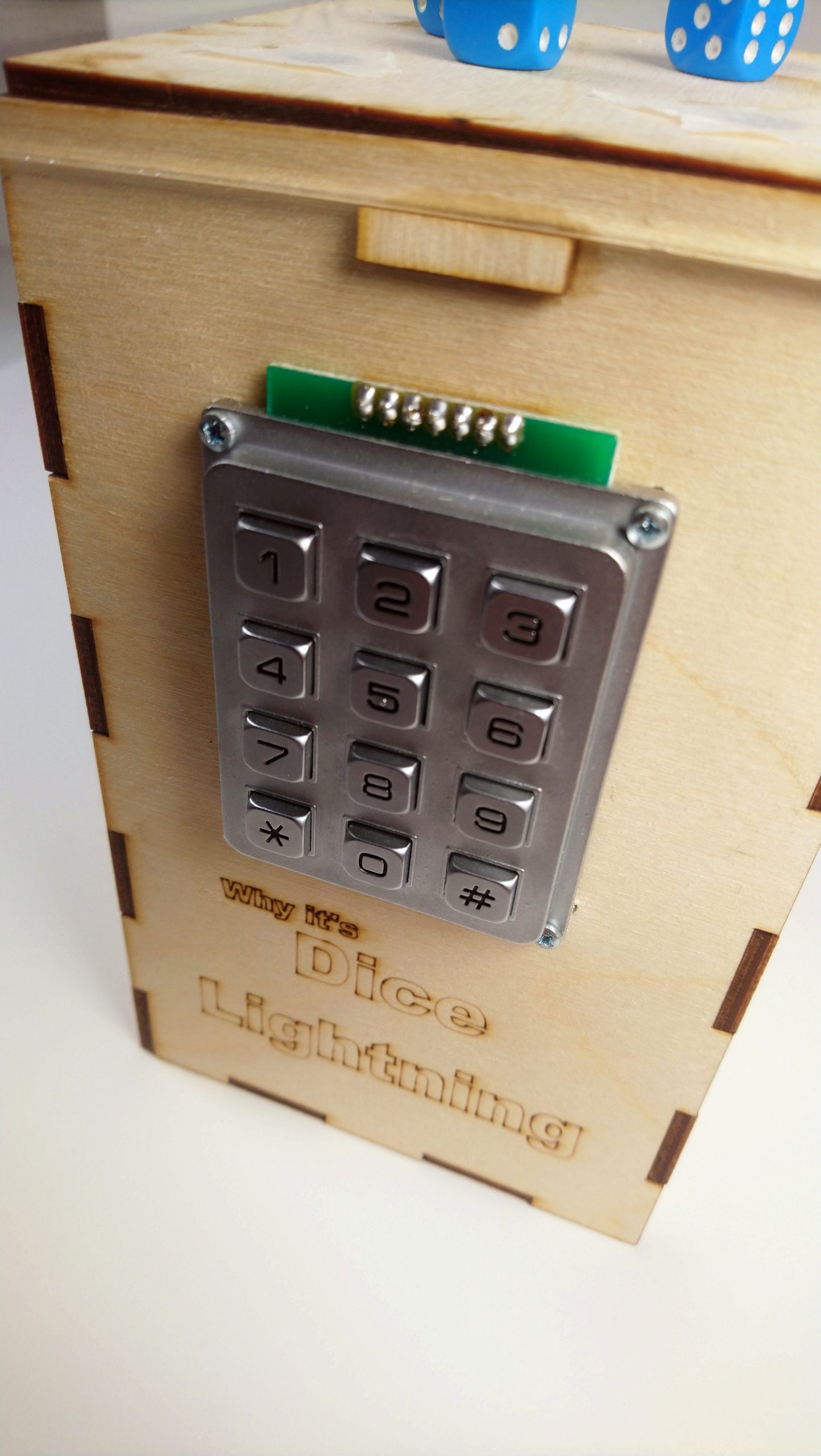
Here is the keypad which I soldered and screwed onto the wood board
Item in Action
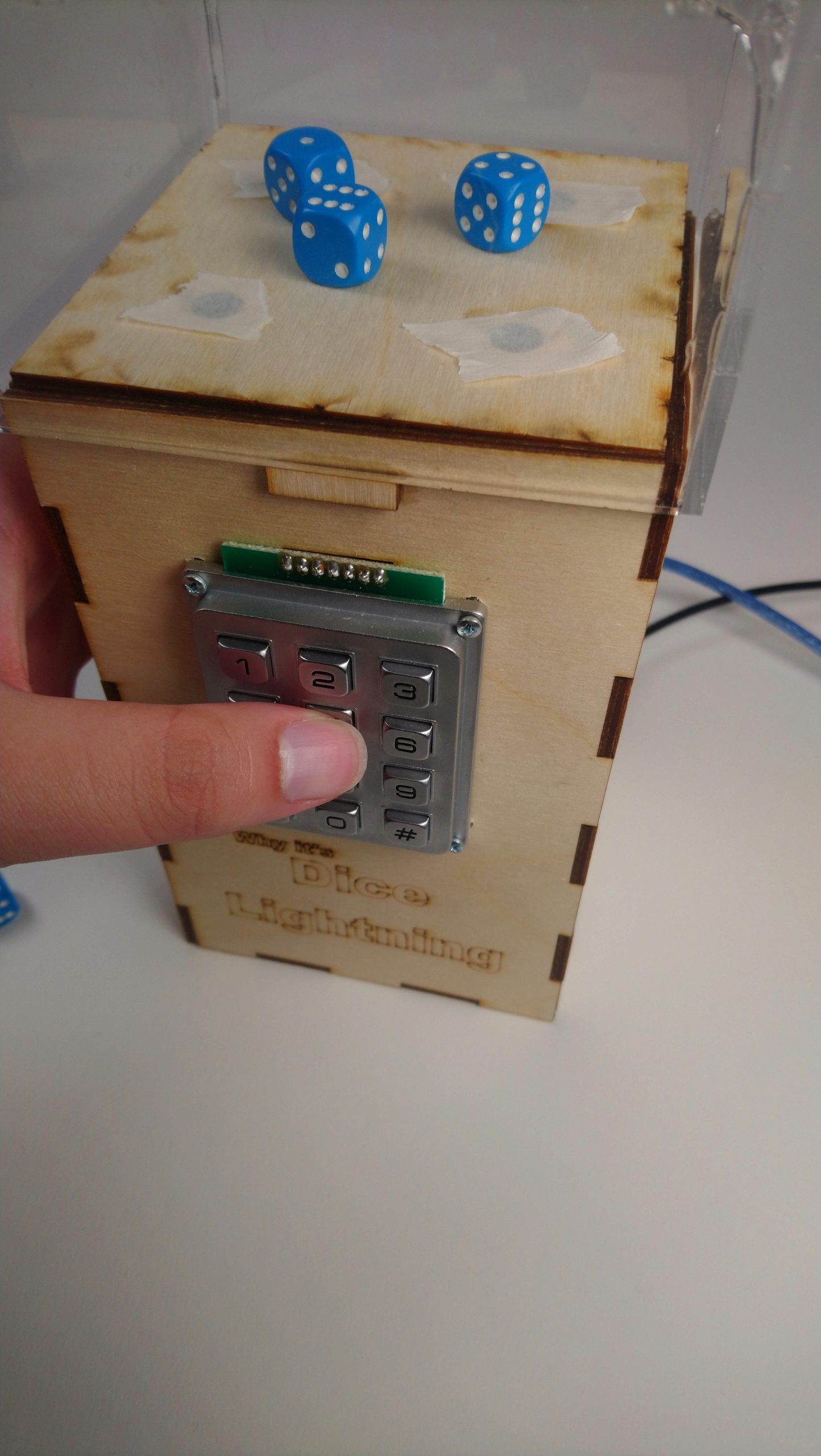
Pushing the 5 button to set the number of kicks
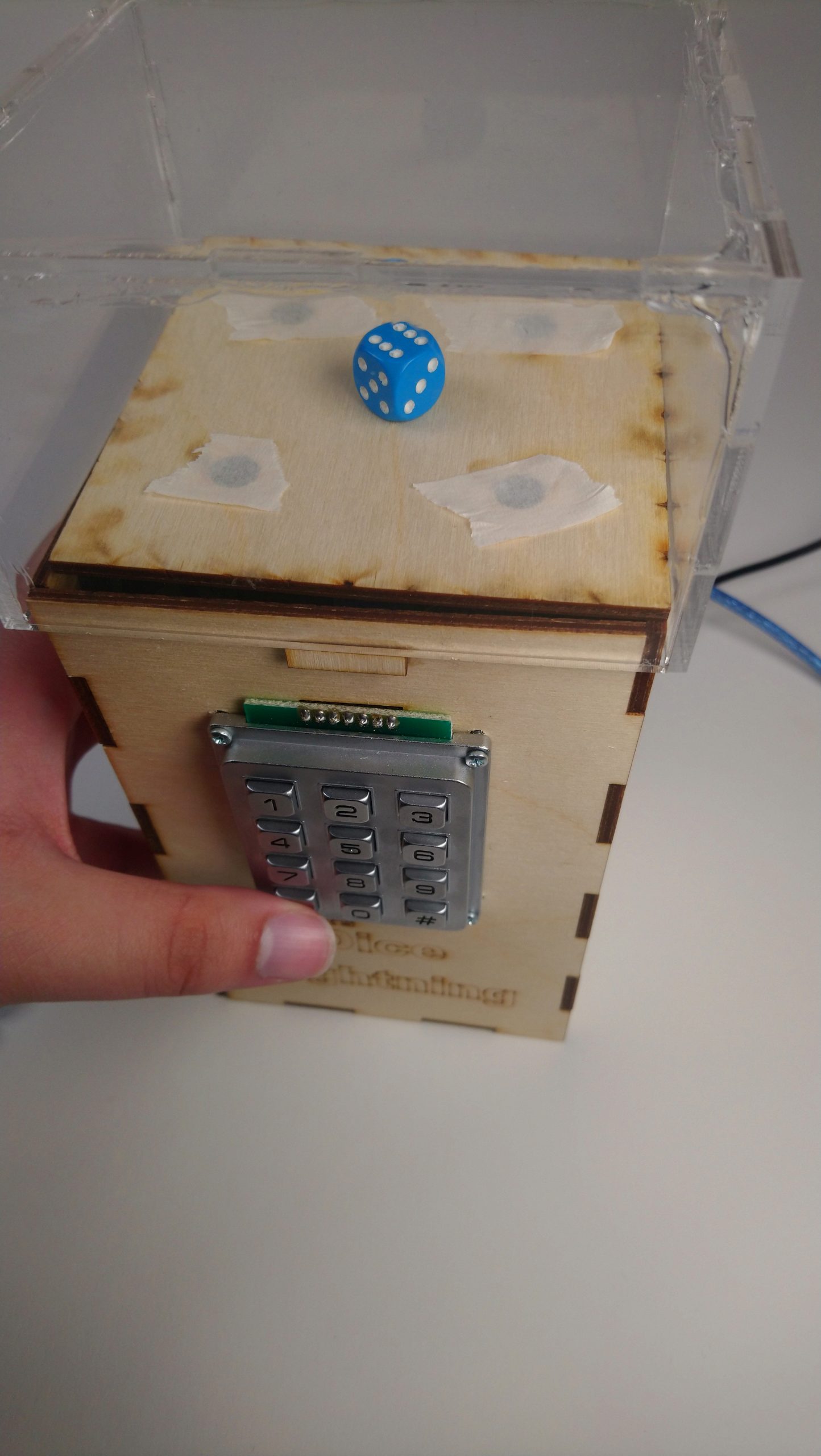
Pushing the * button to initiate kicking
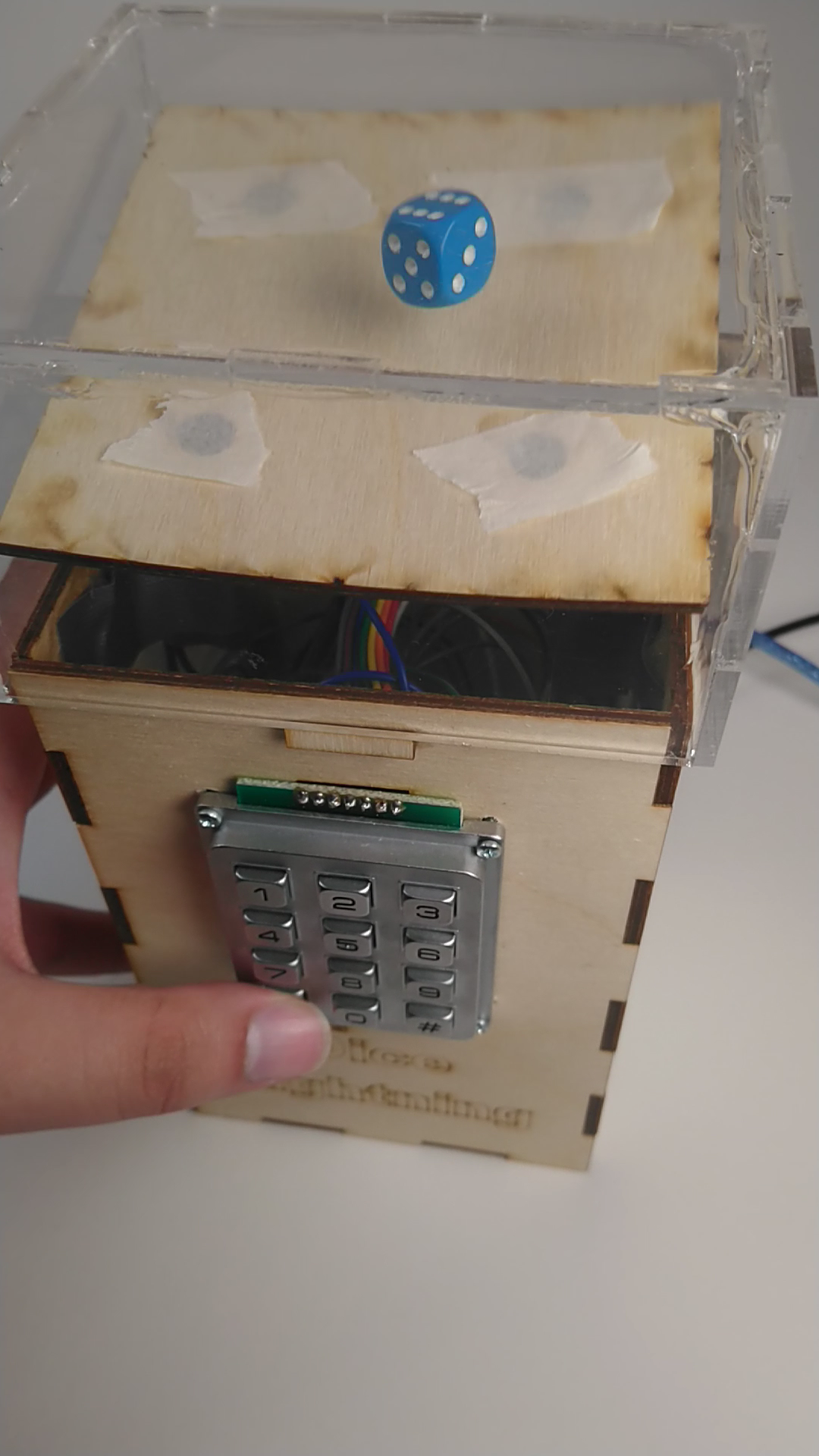
Here is the first kick with the dice in the air
Decisions that affected Output
The first big decision that changed the outcome significantly was the change from IR remote and IR sensor for wireless input to the metal keypad. This was because I felt like the keypad would be more fun to use and since this device is going to e used really close by to me anyways so there really isn’t any need for the wireless device.
The second big decision was the removal of the wooden prongs that would hold the top dice platform in place when it got kicked up. As I was putting the wooden pieces in they weren’t placed perfectly and created friction with the wooden platform preventing it from kicking up as hard as it could. It was also making the glueing of the device really difficult since I had put them in before glueing the whole device together.
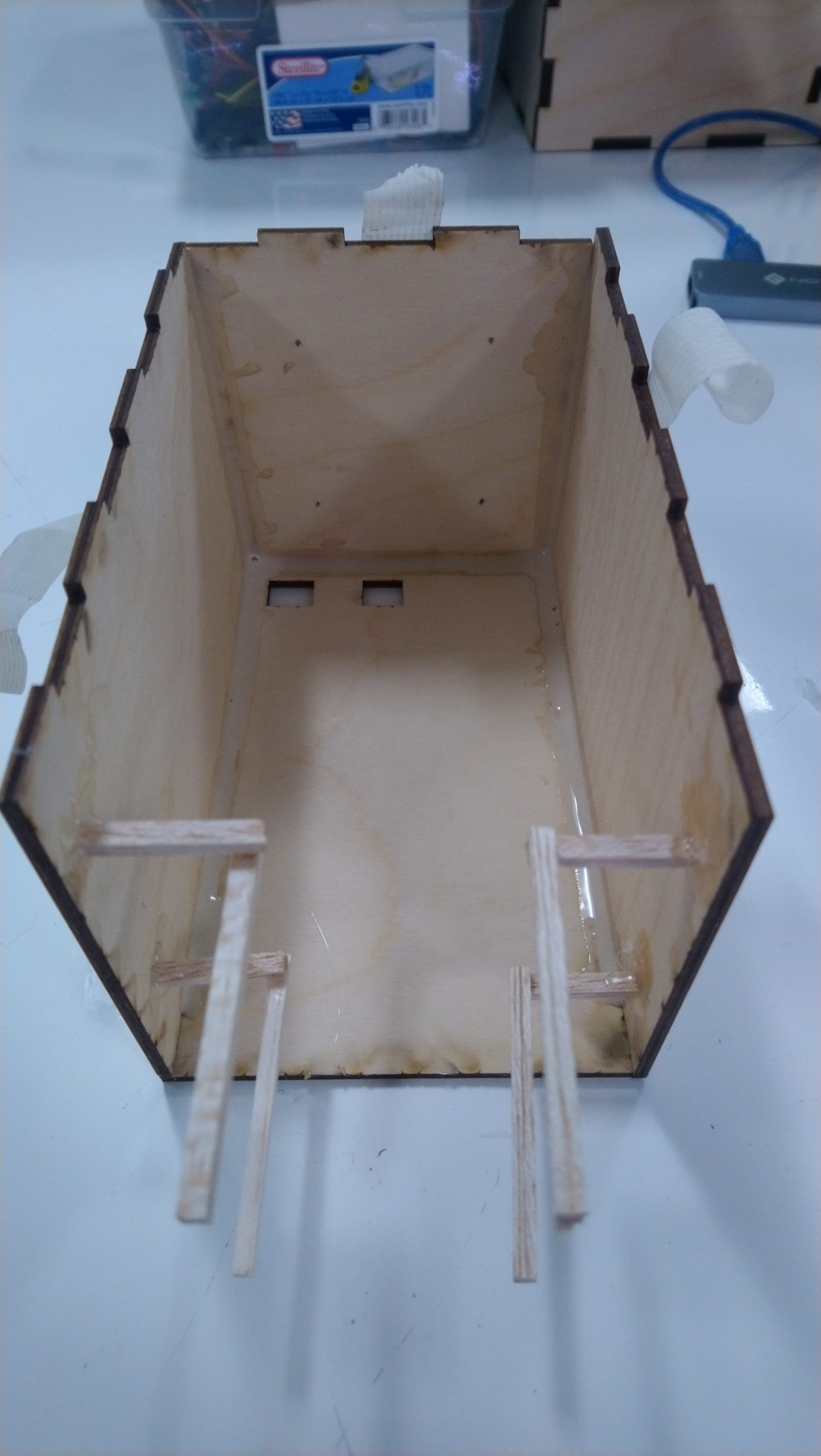
Here is what it looked like with the wooden rods in
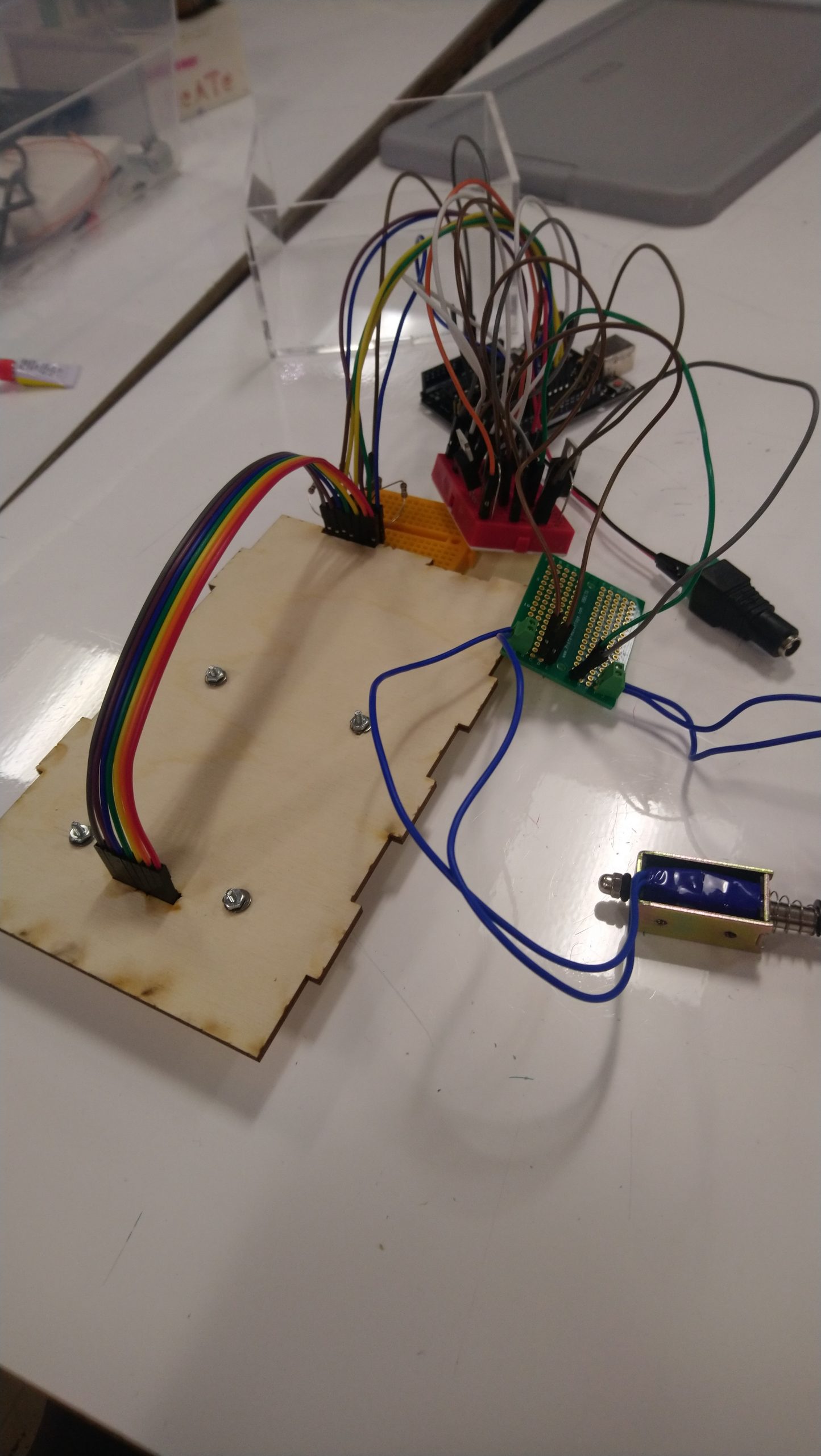
This is the attachment of the keypad to the rest of the circuit with the big rainbow cable
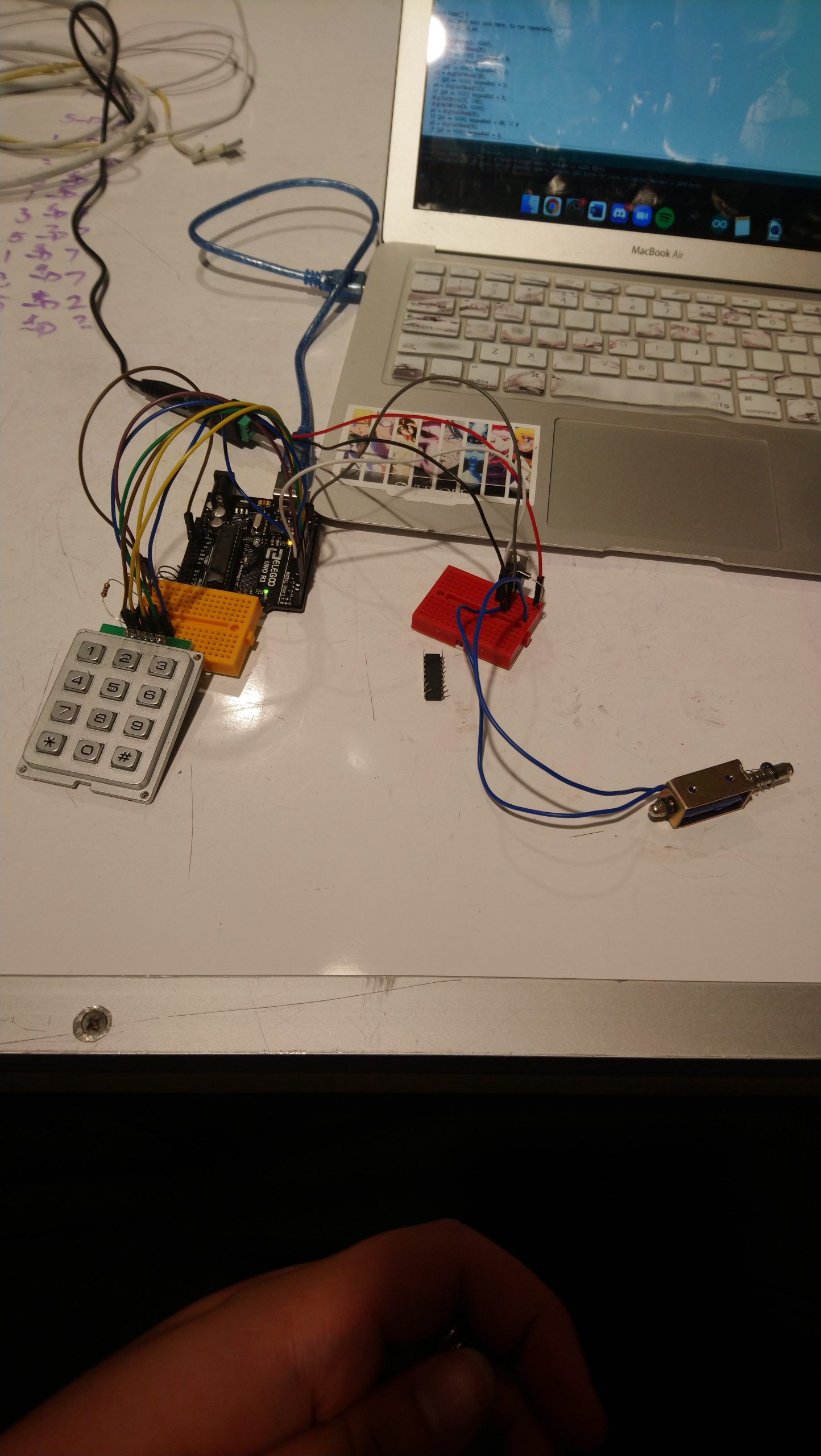
This is the first prototype of the project before any external components were added
Discussion
“I think some additional stability for the upper platform would help a lot for actually flipping dice so that it does not tilt, as well as some more height with the plastic cover so the dice can bounce/flip.” I agree about the additional stability for the platform but the way I was implementing was not a viable option because the order that I did things in, but the plastic cover doesn’t need any more height added to it since the solenoids don’t kick it high enough to ever hit the ceiling anyways.
“Doesn’t seem to actually flip the dice consistently, but that could be fixed with a little more iteration.” This is true as the solenoids struggle with the weight of the wooden platform and the dice on it, this could be solved by adding more solenoids to simply kick the platform up with more force.
I am extremely proud and happy with the project, for the most part it does everything I want it to and it looks really nice. I would have liked for it to only need one cable connecting it instead of two on the back since having two makes it a little annoying to use. I would also make the top platform out of a lighter wood like balsa without having to use a laser cutter. Other than that though the project works exactly as intended and is really fun to use and look at.
I really enjoyed using the laser cutters. Working with the wood and acrylic and putting the pieces together was a lot of fun and I think I’ll definitely be doing it again in the future. If I could go back I would tell myself to start with the box and then put the circuitry inside the box and go from there instead of the other way around since I just shoved all of my circuits in the box haphazardly instead of putting everything in a nice spot to make it look good.
If I were to build another iteration I would for sure add more solenoids to make it kick harder since that was the main issue that I was having. I would also add some sort of voltage transformer to the circuit to power the Arduino inside so that I don’t have to have two cables coming out of the back of the project to make it easier to use.
Schematic and Block Diagram
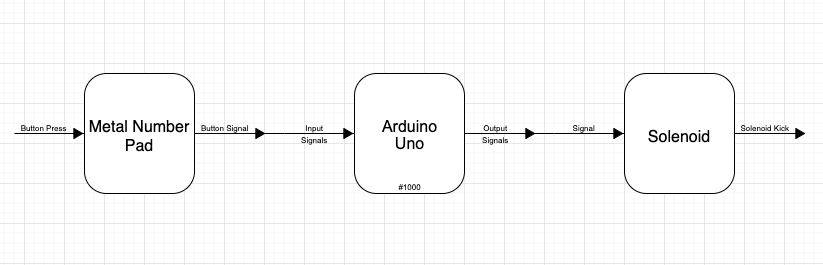
Block diagram
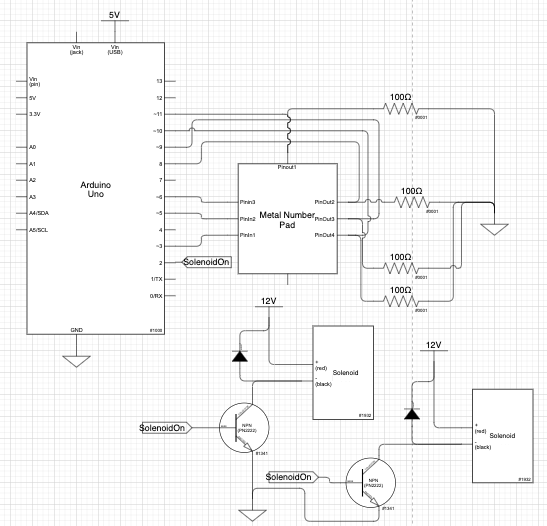
Circuit schematic
Code
/* * Dice Lightning * * Team: Ronald Gonzalez * * Description: * The code takes turns turning on the input pins * of the keypad and then depending on where it * read an output it knows what the key pressed * was. * * Then it will call the solenoid function which * based on the number in keypadVal will turn off * and on the solenoid so that it starts kicking * the dice around. * * Arduino Pin | Description * ------------|--------------------- * 2 | SolenoidOn * 3 | KeypadIn1 * 5 | KeypadIn2 * 6 | KeypadIn3 * 8 | KeypadOut1 * 9 | KeypadOut2 * 10 | KeypadOut3 * 11 | KeypadOut4 * */ // Solenoid Control #define SOLENOIDON 2 // Keypad Input Signals #define KEYPADIN1 3 #define KEYPADIN2 5 #define KEYPADIN3 6 // Keypad Output Signals #define KEYPADOUT1 8 #define KEYPADOUT2 9 #define KEYPADOUT3 10 #define KEYPADOUT4 11 // Delay for Button Pushes #define DELAY 250 // Keeps track of number being pressed unsigned int keypadVal = 0; // Tells the solenoid to start firing bool start = false; void setup() { // Keypad setup pinMode(KEYPADIN1, OUTPUT); pinMode(KEYPADIN2, OUTPUT); pinMode(KEYPADIN3, OUTPUT); pinMode(KEYPADOUT1, INPUT); pinMode(KEYPADOUT2, INPUT); pinMode(KEYPADOUT3, INPUT); pinMode(KEYPADOUT4, INPUT); // Solenoid setup pinMode(SOLENOIDON, OUTPUT); } //Makes the solenoid kick keypadVal number of times void solenoid(){ start = false; //reset start so that it doesn't keep firing if(keypadVal == 0) return; for(int i = 0; i < keypadVal; i++) { digitalWrite(SOLENOIDON, HIGH); delay(2 * DELAY); digitalWrite(SOLENOIDON, LOW); delay(3 * DELAY); } keypadVal = 0; //reset keypadVal } // Reads from the keys on the keypad and changes keypadVal // or start depending on the button pressed void key(int row){ int keys[4]; int sig; //signal being sent to key /* * Based on the row of the keypad that we are * working with the keys and where we send the * signal to change * */ if(row == 1) { int keys[] = {11, 1, 4, 7}; sig = KEYPADIN3; } else if(row == 2) { int keys[] = {0, 2, 5, 8}; sig = KEYPADIN1; } else if(row == 3) { int keys[] = {12, 3, 6, 9}; sig = KEYPADIN2; } // Send a signal to the keypad digitalWrite(sig, HIGH); // Read from all keypadOuts to see what key is being pressed if(digitalRead(KEYPADOUT1) == HIGH) { if(row == 1) start = true; // * key, also the start else if(row == 2) keypadVal = 0; // # key, also the reset else if(row == 3) keypadVal * 10; // 0 key delay(DELAY); } if(digitalRead(KEYPADOUT2) == HIGH) { keypadVal = keypadVal * 10 + keys[1]; delay(DELAY); } if(digitalRead(KEYPADOUT3) == HIGH) { keypadVal = keypadVal * 10 + keys[2]; delay(DELAY); } if(digitalRead(KEYPADOUT4) == HIGH) { keypadVal = keypadVal * 10 + keys[3]; delay(DELAY); } // Turn signal back off digitalWrite(sig, LOW); } // Send all signals to the keypad in order void loop() { for(int i = 1; i <= 3; i++) key(i); // If the start button has been pressed the solenoid will fire if(start) solenoid(); }