Overview
“Task Buddy” is a Tamagotchi-style daily task manager, where completing daily tasks contributes to the overall health of a virtual, portable pet.
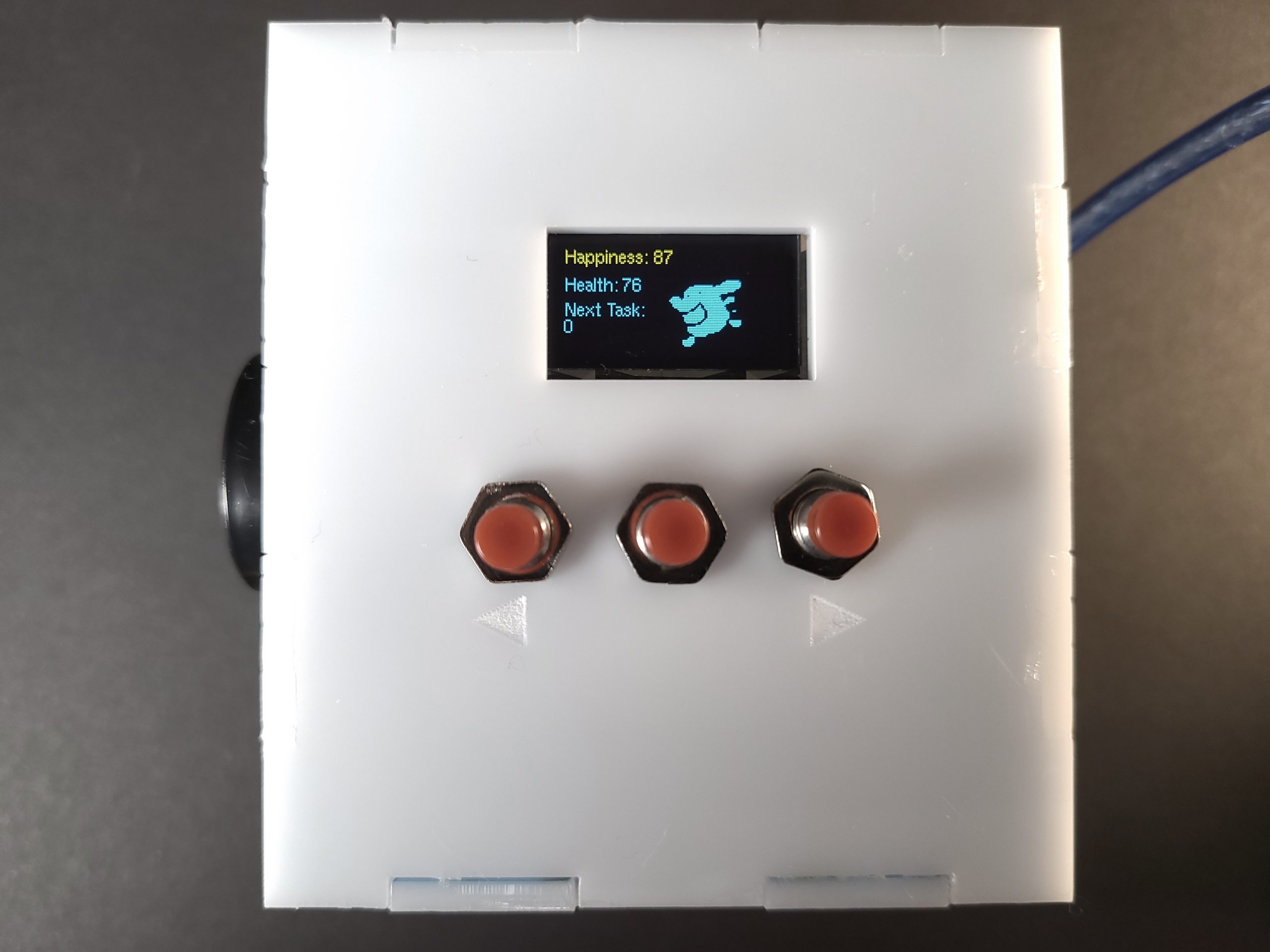
Detail: Front design of the box
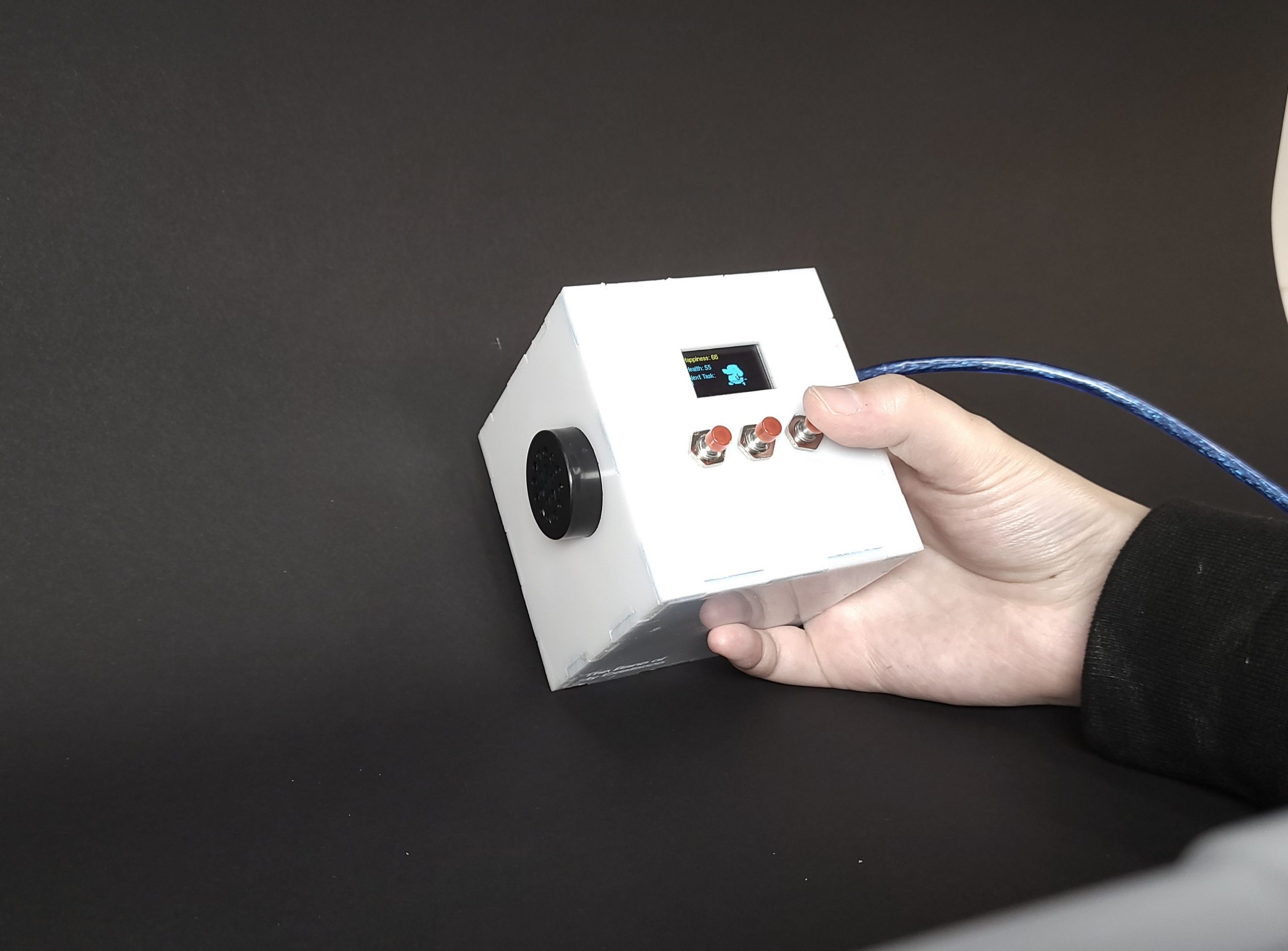
Buddy in use
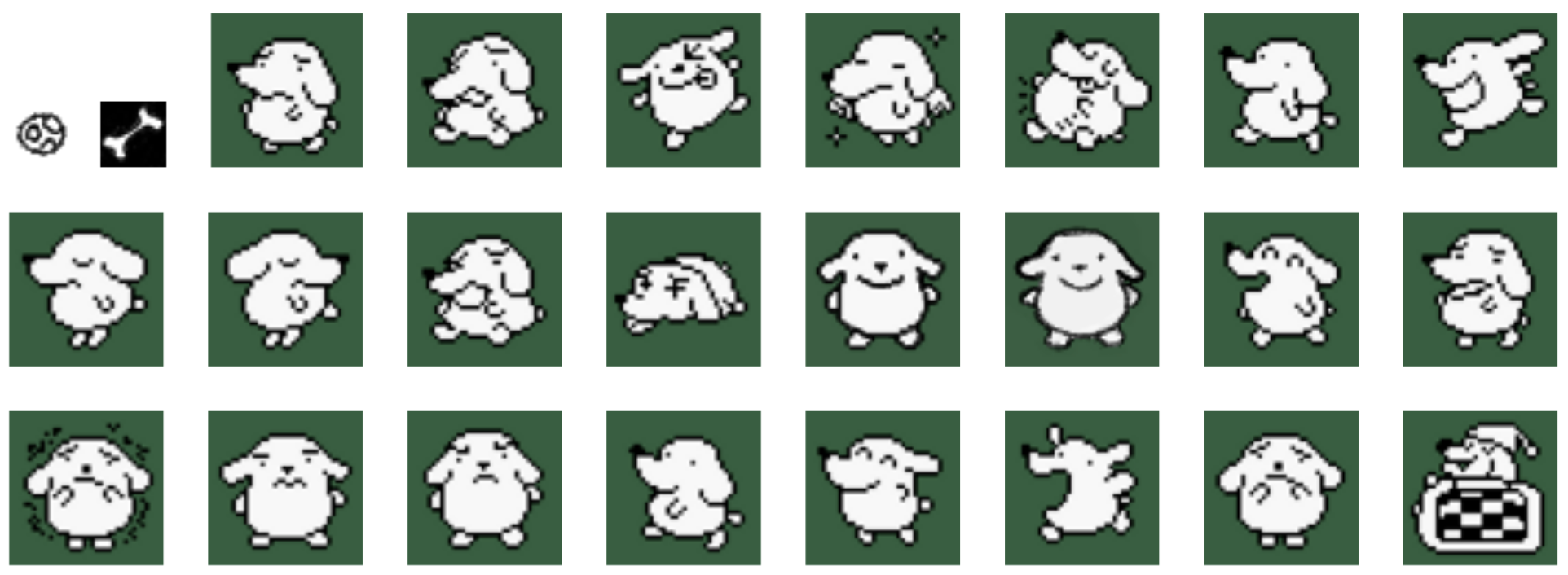
All animation frames of the Buddy used
Usage Videos
Idle animation while Buddy is happy:
Idle animation while Buddy is sad, sped up 2x:
Adding a Task:
Deleting a Task:
You can set the Buddy to sleep, and then wake it up (with a penalty!):
When the timer for a task runs out, it autodeletes and there’s a penalty on either health or happiness:
If happiness or health is at zero for too long, the Buddy dies. Revivable with a penalty:
Process Images
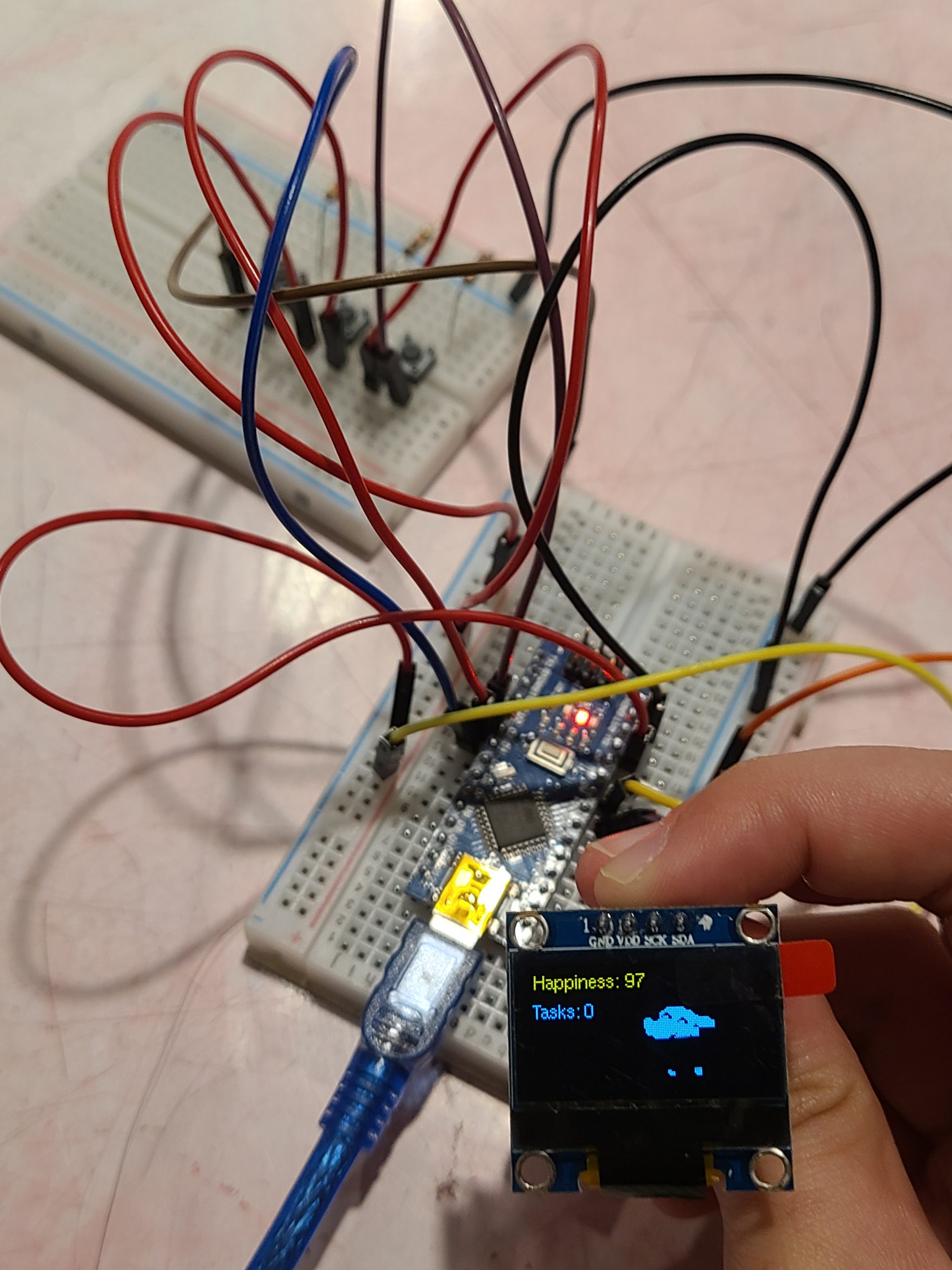
First thing I did: got the OLED screen working with the buttons.
After getting the screen working, I spent a large portion of my time programming the game logic and each of the screens, working out how tasks would be added in and deleted, and how I would affect the Buddy. I also created each of the animation frames in this time.
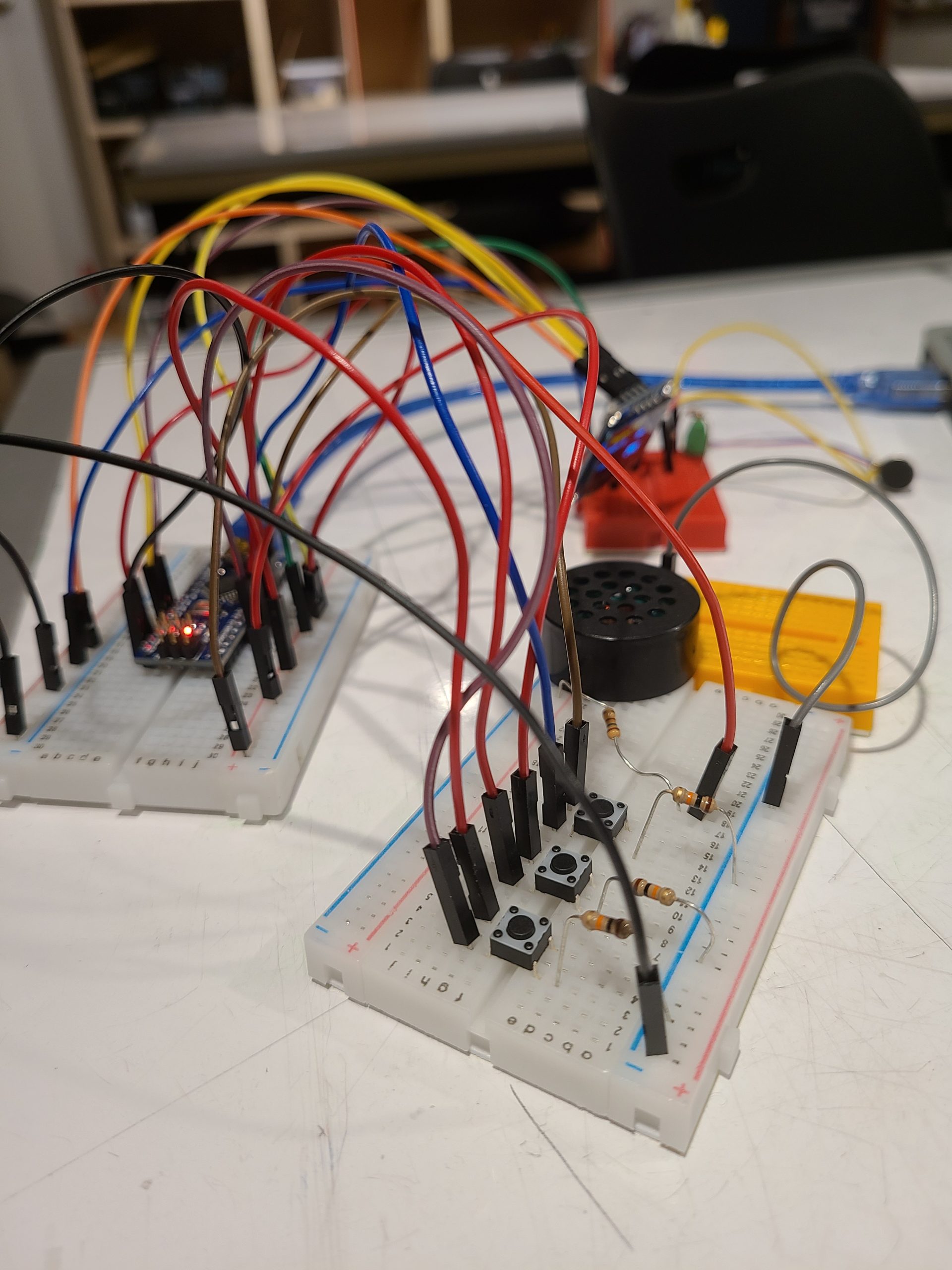
Prototyping the wiring for the buttons, vibration motor, and speakers
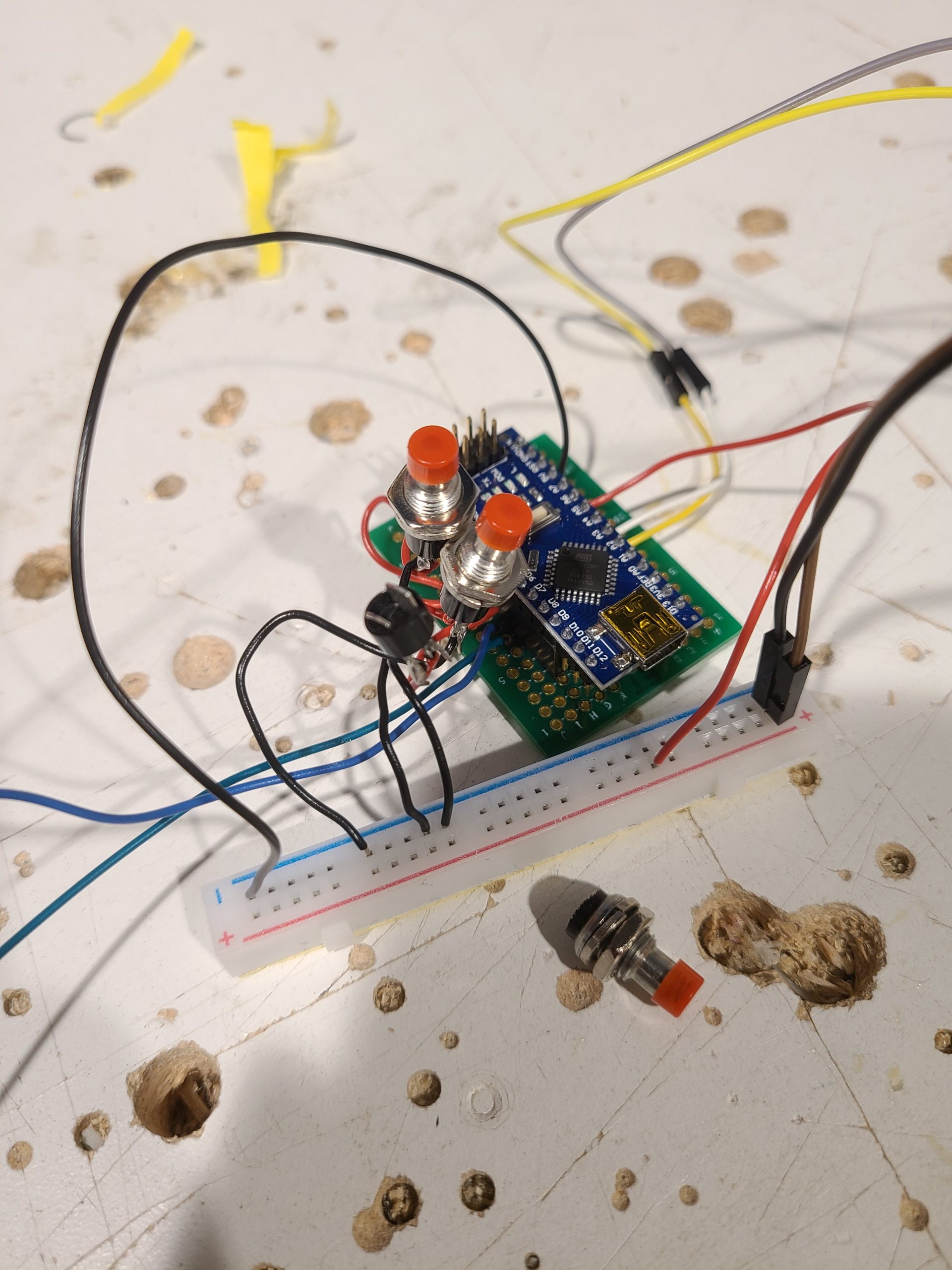
Attaching the buttons to the board
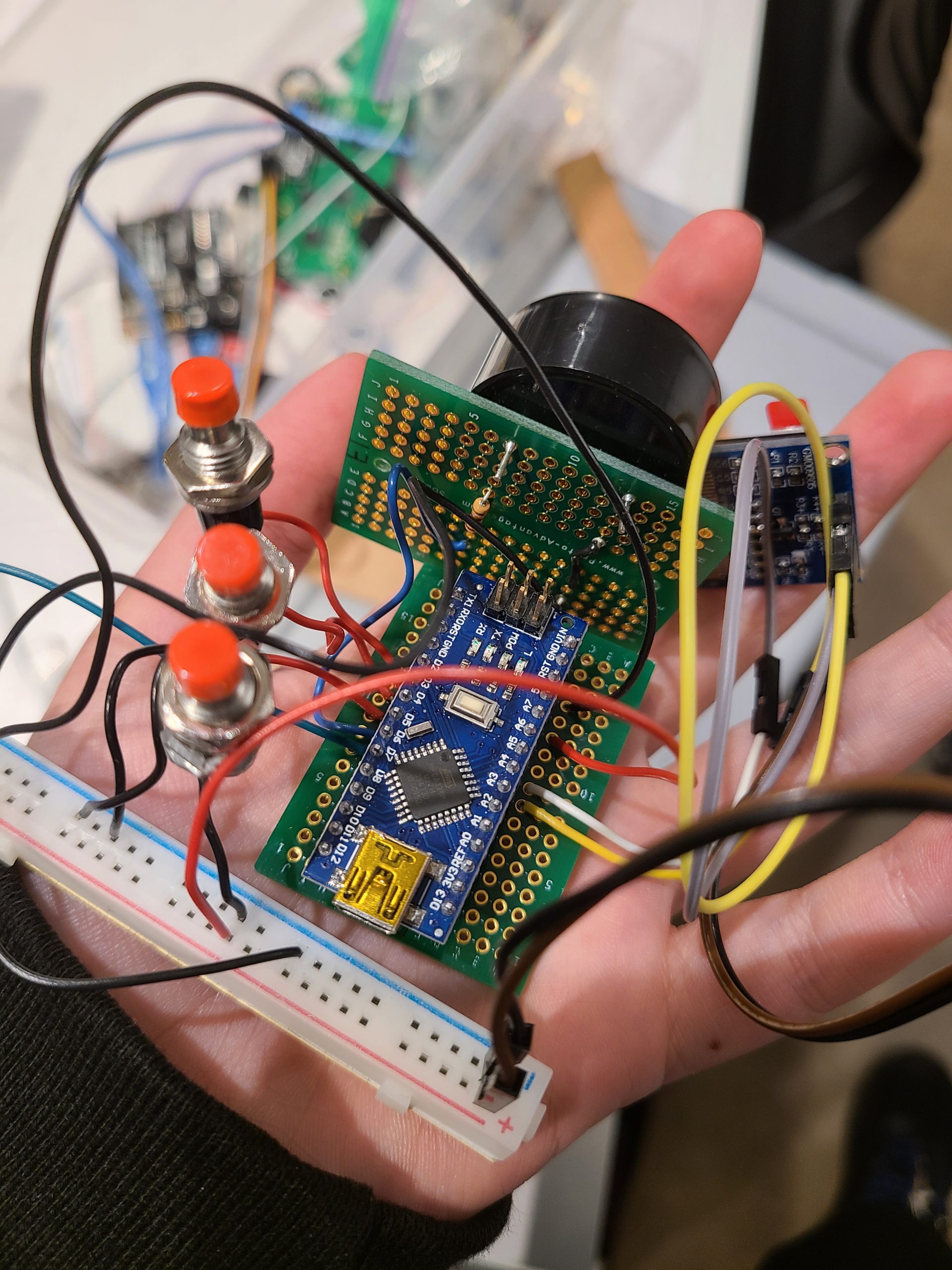
Soldering it all together
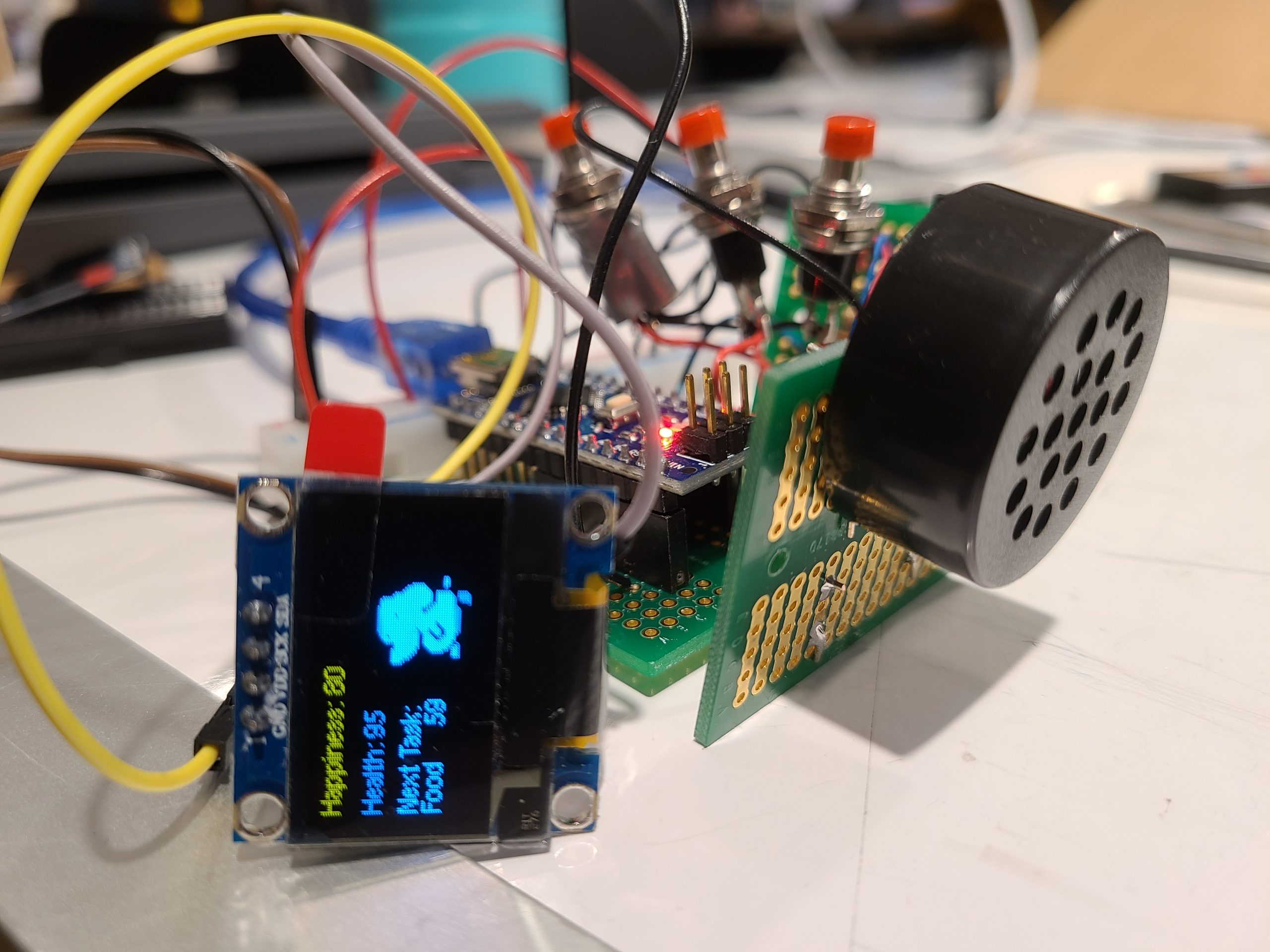
More soldering done
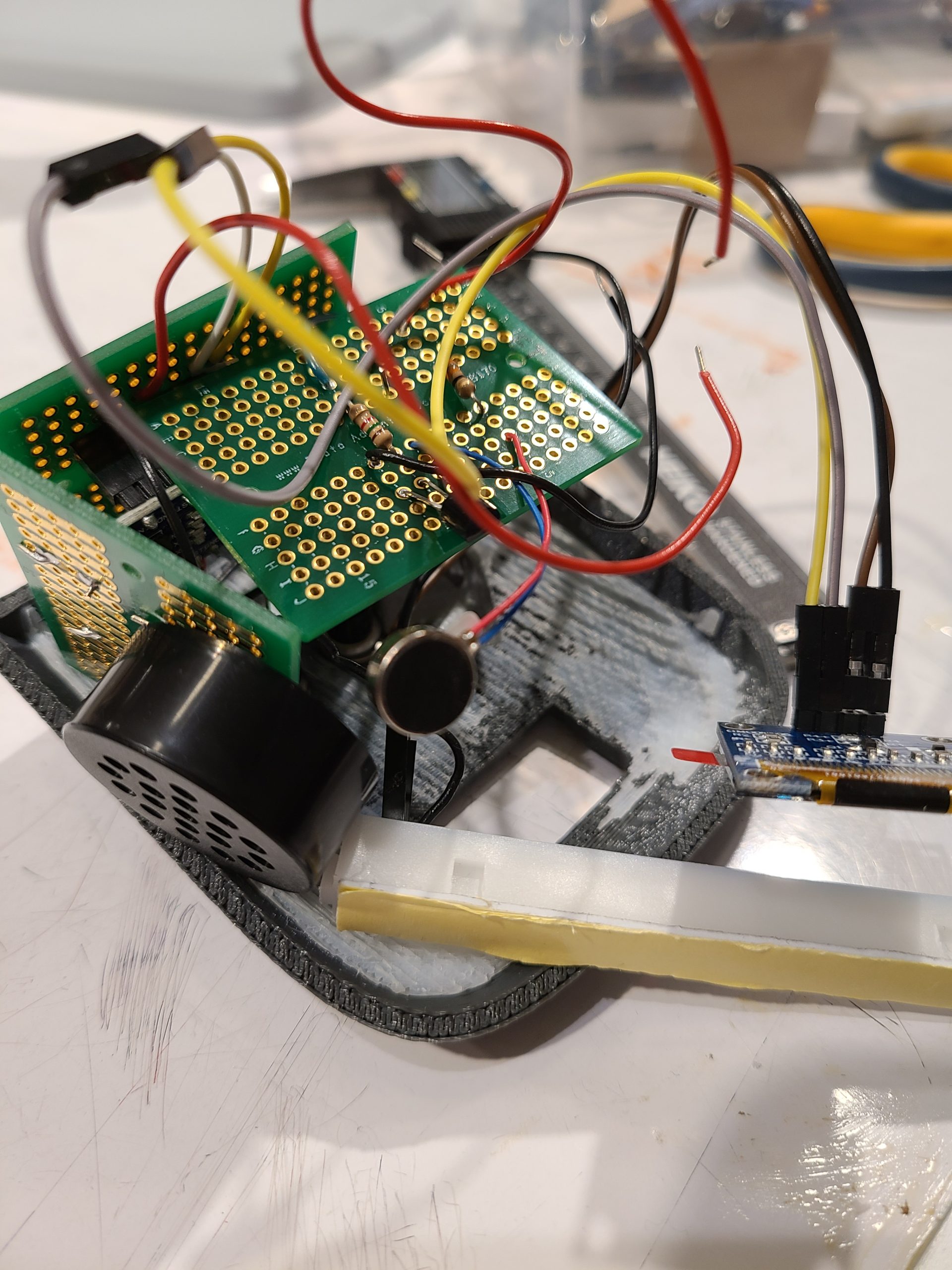
After finishing all the wiring, all I needed was a case. Originally, I planned to 3D print a case, but the plan didn’t pan out. As a result…
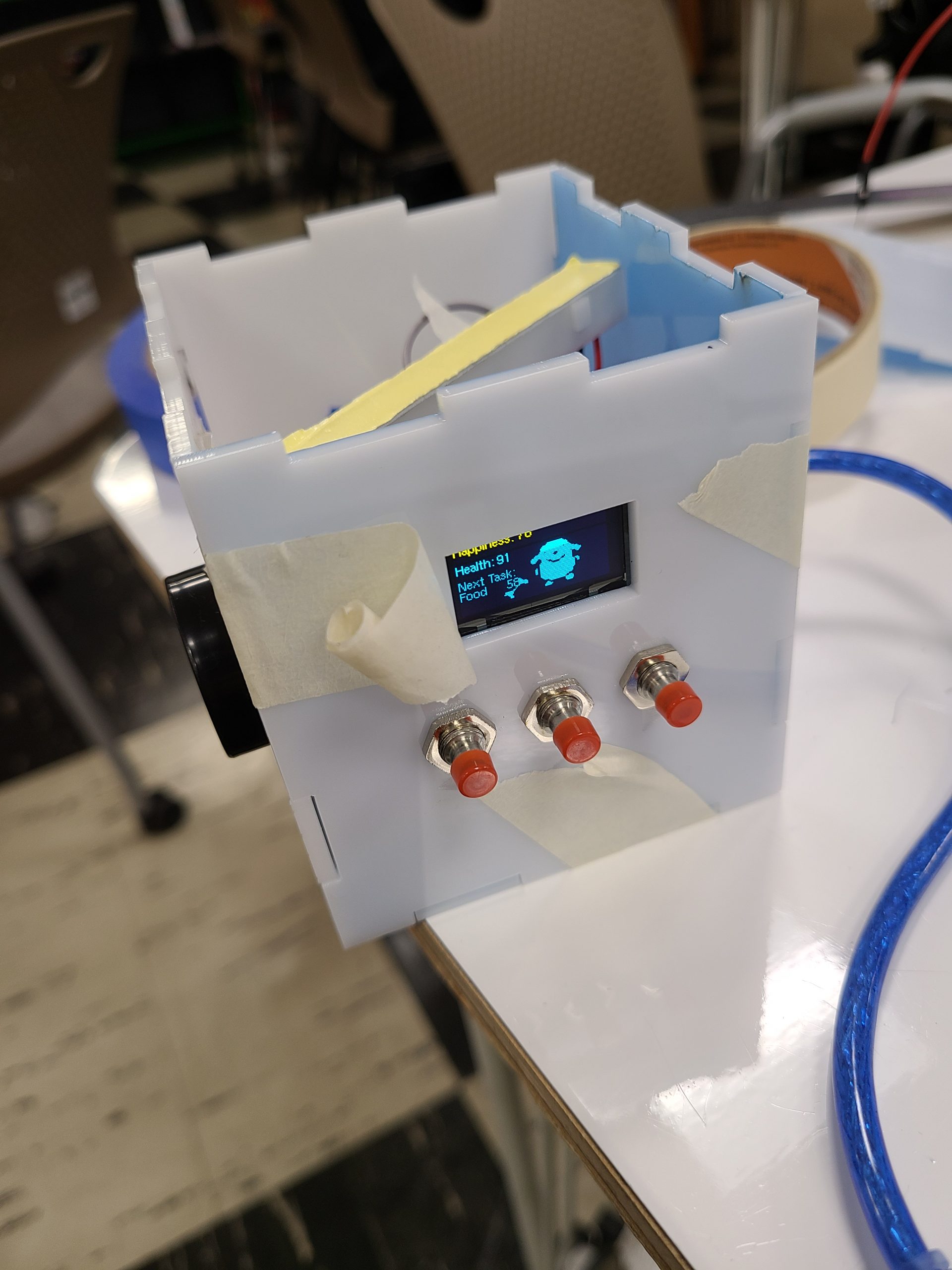
Lasercut the final box and casing to put the entire setup within!
Discussion
“Screen is really small, making text hard to read (I’m old)” Agreed! One of the main issues I originally had with the whol”e thing was finding a suitable screen; before I found the OLED, I was going to just use blinking lights and other indicators. With the introduction of the OLED screen and its limitation, I tried to create something focused around the size of this screen, so it would look proportional in relation to it. However, I wasn’t able to make the device as small as I liked, and so the screen feels very small in comparison to the whole thing.
“Really letting the cute thing die.” Assuming this is a criticism on having there be stronger consequences for the buddy dying, I considered having a sort of randomly generated new buddy entirely different from the original or having the program keep track of number of deaths and make it harder to keep the buddy alive with each death, but I ended up scraping both ideas due to time.
I’m fairly happy with how the project turned out, especially in aspects of figuring out how to gamify the process of finishing tasks. However, I would improve it on many different fronts. For example, I would have liked to shrink the size of the casing even more and make it more portable, since currently it is a little unwieldy. I’d also like to add even more kinds of interactions by connecting it to an SD card, and perhaps allow the whole thing to be completely independent of external power sources, instead powering it with something like a battery. In particular, I learned how tricky the process of fabrication is, and to devote more time to it in the future.
If I built another iteration, I think I would focus on shrinking the design of it even further, spending more time mapping out all the wiring and able to figure out how to utilize my space most efficiently.
Technical information
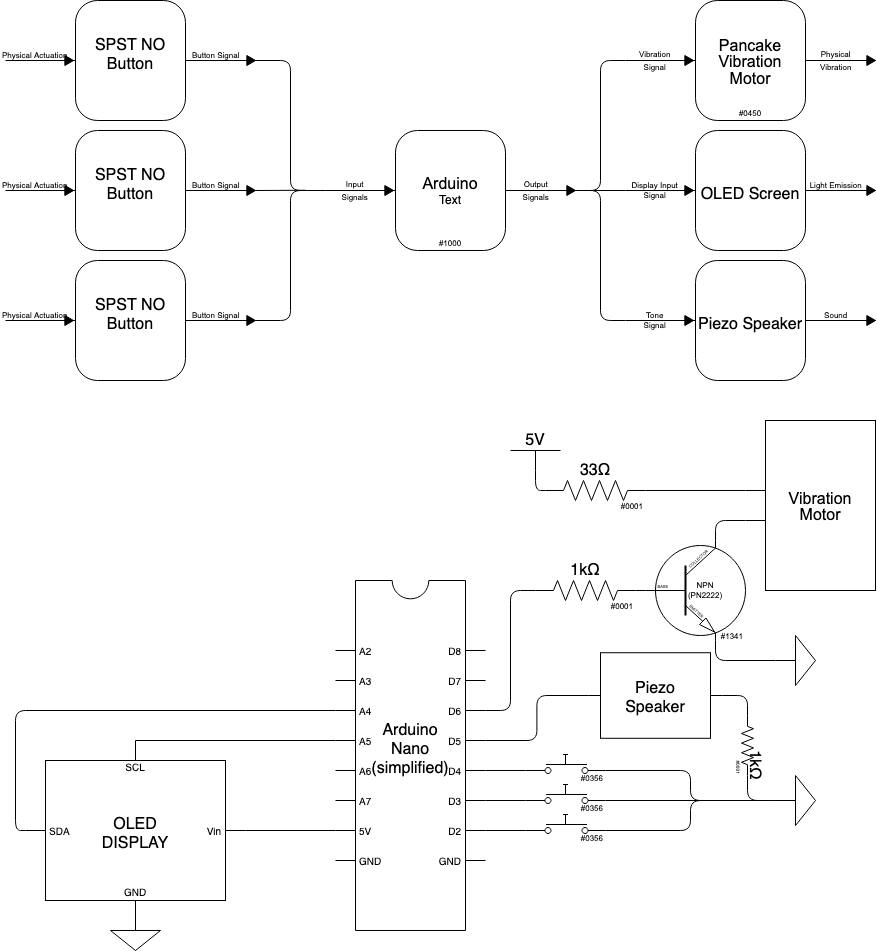
Block Diagram and Schematic
Main Code File:
/* * Task Buddy * * This code does all the animations, takes in inputs from * buttons, and outputs speaker sounds and vibrations * accordingly. * * PINS: * button 1 = D4; * button 2 = D3; * button 3 = D2; * speaker = D5; * motor = D6; * * Thank you to: * This tool for converting images to byte arrays for the OLED: * https://javl.github.io/image2cpp/ * */ #include "U8glib.h" #include "pitches.h" U8GLIB_SSD1306_128X64 u8g(U8G_I2C_OPT_DEV_0|U8G_I2C_OPT_NO_ACK|U8G_I2C_OPT_FAST); // Fast I2C / TWI const unsigned char happy_happy0 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xdf, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x07, 0xdf, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x7f, 0xff, 0xc7, 0xff, 0xfe, 0x00, 0x01, 0xff, 0xff, 0x87, 0xff, 0xff, 0x80, 0x03, 0xff, 0xff, 0xcf, 0xff, 0x7f, 0x80, 0x03, 0xf3, 0xff, 0xff, 0xff, 0x8f, 0x00, 0x03, 0xe1, 0xff, 0xff, 0xff, 0x86, 0x00, 0x01, 0xc1, 0xef, 0xff, 0xdf, 0x80, 0x00, 0x00, 0x01, 0xf1, 0xff, 0x1f, 0x80, 0x00, 0x00, 0x03, 0xf8, 0xfe, 0x3f, 0x80, 0x00, 0x00, 0x07, 0xfe, 0x01, 0xff, 0x80, 0x00, 0x00, 0x07, 0xff, 0x03, 0xff, 0x80, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0xdc, 0x00, 0x00, 0x27, 0xff, 0xff, 0xff, 0xee, 0x00, 0x00, 0x67, 0xff, 0xff, 0xff, 0xe6, 0x00, 0x00, 0x67, 0xff, 0xff, 0xff, 0xe6, 0x00, 0x00, 0x67, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x67, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x67, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xc7, 0x00, 0x00, 0x00, 0x00, 0x30, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00, 0x78, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00, 0xfe, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00, 0xfc, 0x00, 0x1f, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char happy_happy1 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xdf, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x07, 0xdf, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x7f, 0xff, 0xc7, 0xff, 0xfe, 0x00, 0x01, 0xff, 0xff, 0x87, 0xff, 0xff, 0x80, 0x03, 0xff, 0xff, 0xcf, 0xff, 0x7f, 0x80, 0x03, 0xf3, 0xff, 0xff, 0xff, 0x8f, 0x00, 0x03, 0xe1, 0xff, 0xff, 0xff, 0x86, 0x00, 0x01, 0xc1, 0xef, 0xff, 0xdf, 0x80, 0x00, 0x00, 0x01, 0xf1, 0xff, 0x1f, 0x80, 0x00, 0x00, 0x03, 0xf8, 0xfe, 0x3f, 0x80, 0x00, 0x00, 0x07, 0xfe, 0x01, 0xff, 0x80, 0x00, 0x00, 0x07, 0xff, 0x03, 0xff, 0x80, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0xdc, 0x00, 0x00, 0x27, 0xff, 0xff, 0xff, 0xee, 0x00, 0x00, 0x67, 0xff, 0xff, 0xff, 0xe6, 0x00, 0x00, 0x67, 0xff, 0xff, 0xff, 0xe6, 0x00, 0x00, 0x67, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x67, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x67, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xc7, 0x00, 0x00, 0x00, 0x00, 0x30, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00, 0x78, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00, 0xfe, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00, 0xfc, 0x00, 0x1f, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char happy_happy2 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x1b, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x7e, 0x1f, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xfd, 0xff, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x01, 0xff, 0xbf, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xbf, 0xf0, 0x00, 0x00, 0x00, 0x00, 0xff, 0x3f, 0xf0, 0x00, 0x00, 0x00, 0x7f, 0xff, 0x3f, 0xf0, 0x00, 0x00, 0x00, 0xff, 0xff, 0x3f, 0xe0, 0x00, 0x00, 0x00, 0xff, 0xff, 0x9f, 0xc0, 0x00, 0x00, 0x00, 0xff, 0xf3, 0xcf, 0x80, 0x00, 0x00, 0x01, 0xff, 0xf3, 0x80, 0x00, 0x00, 0x00, 0x01, 0xff, 0xfb, 0x80, 0x00, 0x00, 0x00, 0x01, 0xff, 0xf9, 0xcf, 0x80, 0x00, 0x00, 0x01, 0xff, 0xf9, 0xcf, 0x80, 0x00, 0x00, 0x01, 0xff, 0xfc, 0x1f, 0x80, 0x00, 0x00, 0x00, 0xff, 0xfe, 0x3f, 0x9e, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0x8e, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0x80, 0x00, 0x00, 0x03, 0x3f, 0xff, 0xff, 0x00, 0x00, 0x00, 0x0f, 0x8f, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x0f, 0xc1, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x0f, 0xf0, 0xff, 0xc3, 0x80, 0x00, 0x00, 0x03, 0xe0, 0x00, 0x07, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char happy_happy3 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x0d, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x1f, 0x8f, 0xff, 0xff, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xfe, 0xff, 0x80, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x3f, 0xbf, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0xff, 0x9f, 0xf8, 0x00, 0x00, 0x00, 0x1f, 0xff, 0x9f, 0xf0, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xcf, 0xe0, 0x00, 0x00, 0x00, 0x7f, 0x3f, 0xe7, 0xc0, 0x00, 0x00, 0x00, 0xfe, 0x7f, 0xf0, 0x00, 0x00, 0x00, 0x00, 0xfc, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0xf9, 0xe7, 0xff, 0xc0, 0x00, 0x00, 0x00, 0xf9, 0xcf, 0xff, 0xc0, 0x00, 0x00, 0x00, 0xfc, 0x1f, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x7e, 0x3f, 0xff, 0xcf, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xc7, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xff, 0x80, 0x00, 0x00, 0x03, 0xc7, 0xff, 0xff, 0x00, 0x00, 0x00, 0x03, 0xe0, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x03, 0xf0, 0x7f, 0xe1, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char happy_happy4 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x73, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x61, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x5c, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x70, 0x7c, 0xf9, 0xff, 0xf8, 0x00, 0x00, 0xf8, 0xff, 0xf0, 0xff, 0xfc, 0x00, 0x00, 0xff, 0xff, 0xee, 0x7f, 0xff, 0x00, 0x00, 0xff, 0xff, 0xfe, 0x7f, 0xff, 0x00, 0x00, 0xff, 0xff, 0xff, 0xfb, 0xff, 0x00, 0x00, 0xff, 0xff, 0xff, 0xf1, 0xff, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xdf, 0xfe, 0x00, 0x00, 0x00, 0x07, 0xfe, 0x3f, 0xde, 0x00, 0x00, 0x00, 0x03, 0xfc, 0x7f, 0x8e, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xf6, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xf2, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0xf6, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0xef, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x38, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x38, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x08, 0x00, 0x1e, 0x00, 0x00, 0x00, 0x00, 0x0c, 0x00, 0x1e, 0x00, 0x00, 0x00, 0x00, 0x0f, 0x00, 0x1e, 0x00, 0x00, 0x00, 0x00, 0x07, 0x00, 0x0e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char happy_happy5 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x0e, 0x7f, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x1c, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x18, 0x39, 0x9f, 0xfe, 0x00, 0x00, 0x00, 0x3c, 0x39, 0x9f, 0x3f, 0x00, 0x00, 0x00, 0x7e, 0x3b, 0xde, 0x1f, 0x80, 0x00, 0x00, 0x7f, 0xff, 0xfc, 0xcf, 0xe0, 0x00, 0x00, 0x7f, 0xff, 0xfc, 0xcf, 0xf0, 0x00, 0x00, 0x7f, 0xff, 0xfd, 0xef, 0xfe, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xfd, 0xff, 0x00, 0x00, 0x1f, 0xf3, 0xff, 0xf9, 0xff, 0x00, 0x00, 0x0f, 0xe1, 0xff, 0xfd, 0xff, 0x00, 0x00, 0x00, 0x00, 0xff, 0xfe, 0xff, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0x7e, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0x3c, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xff, 0x80, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xf9, 0xc0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xf9, 0xe0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfc, 0xe0, 0x00, 0x00, 0x01, 0xff, 0xff, 0x9e, 0x60, 0x00, 0x00, 0x02, 0xff, 0xff, 0xce, 0x64, 0x00, 0x00, 0x02, 0x7f, 0xff, 0xe0, 0xe0, 0x00, 0x00, 0x02, 0x7f, 0xff, 0xf1, 0xe0, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x07, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x18, 0xff, 0xf1, 0xc0, 0x00, 0x00, 0x00, 0x3f, 0x00, 0x03, 0xe0, 0x00, 0x00, 0x00, 0x3f, 0x00, 0x03, 0xe0, 0x00, 0x00, 0x00, 0x3f, 0x00, 0x01, 0xe0, 0x00, 0x00, 0x00, 0x1e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char happy_happy6 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0x80, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x03, 0xff, 0x9f, 0xff, 0xc0, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0xff, 0xbf, 0xff, 0xc0, 0x00, 0x00, 0x00, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0xdf, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x78, 0xff, 0xff, 0xfc, 0x3c, 0x00, 0x00, 0xfd, 0xff, 0xff, 0xfc, 0x7c, 0x00, 0x01, 0xff, 0xff, 0xff, 0xfe, 0xfc, 0x00, 0x00, 0xff, 0xff, 0xbf, 0xff, 0xe0, 0x00, 0x00, 0x7f, 0xff, 0x1f, 0xff, 0x80, 0x00, 0x00, 0x7f, 0xfe, 0x4f, 0xff, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0xcf, 0xff, 0x80, 0x00, 0x00, 0x0f, 0xf1, 0xe7, 0xff, 0x80, 0x00, 0x00, 0x07, 0xe3, 0xf3, 0xff, 0xc6, 0x00, 0x00, 0x00, 0x1f, 0xf3, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x3f, 0xf3, 0xff, 0xe0, 0x00, 0x00, 0x01, 0xff, 0xf3, 0xff, 0xe0, 0x00, 0x00, 0x00, 0xff, 0xf3, 0xff, 0xe0, 0x00, 0x00, 0x00, 0xff, 0xe7, 0xff, 0xc0, 0x00, 0x00, 0x00, 0xff, 0xcf, 0xff, 0x9f, 0xc0, 0x00, 0x00, 0x7f, 0x1f, 0xff, 0x9f, 0xc0, 0x00, 0x00, 0x00, 0x3f, 0xff, 0x8f, 0xc0, 0x00, 0x00, 0x01, 0xff, 0xff, 0x07, 0x80, 0x00, 0x00, 0x3f, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1c, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3c, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char happy_happy7 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x23, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xfe, 0x00, 0x78, 0x00, 0x00, 0x00, 0x1f, 0xff, 0x00, 0xf8, 0x00, 0x00, 0x00, 0x3f, 0xff, 0x87, 0xf8, 0x00, 0x00, 0x70, 0x37, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x78, 0x7f, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x7f, 0xff, 0xfd, 0xfe, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x3f, 0x83, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf8, 0xf8, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf3, 0xfc, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x01, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0x38, 0x00, 0x00, 0x00, 0x07, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xff, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0x20, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0x60, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfe, 0x70, 0x00, 0x00, 0x00, 0xff, 0xff, 0xfe, 0x78, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xf8, 0xf8, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xf1, 0xf8, 0x00, 0x00, 0x03, 0xc7, 0xff, 0x80, 0xf0, 0x00, 0x00, 0x03, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; // Array of all bitmaps for convenience. (Total bytes used to store images in PROGMEM = 3328) const unsigned char* happy_allArray[8] = { happy_happy0, happy_happy1, happy_happy2, happy_happy3, happy_happy4, happy_happy5, happy_happy6, happy_happy7 }; const unsigned char sad_sad0 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0x80, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x3f, 0xfe, 0xfb, 0xff, 0xff, 0x00, 0x00, 0x7f, 0xff, 0x03, 0xff, 0xff, 0x00, 0x00, 0xff, 0xff, 0x87, 0x9f, 0xff, 0x00, 0x00, 0xff, 0xff, 0xff, 0x9f, 0xff, 0x00, 0x00, 0xff, 0xff, 0xff, 0xcf, 0xff, 0x00, 0x00, 0xff, 0xff, 0xff, 0xe7, 0xff, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xf1, 0xfe, 0x00, 0x00, 0x3f, 0xfc, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x1f, 0xf8, 0x7f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x03, 0x3f, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x07, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x00, 0xff, 0xfe, 0xe7, 0xf0, 0x00, 0x00, 0x00, 0xff, 0xff, 0x73, 0xf3, 0x00, 0x00, 0x00, 0xff, 0xff, 0x33, 0xf3, 0x00, 0x00, 0x00, 0xff, 0xff, 0x33, 0xf3, 0x00, 0x00, 0x00, 0xff, 0xff, 0x87, 0xe0, 0x00, 0x00, 0x00, 0xff, 0xff, 0xcf, 0xc0, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0x80, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x01, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x61, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0xe3, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x01, 0xe7, 0x80, 0x00, 0x00, 0x00, 0x00, 0x01, 0xc7, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char sad_sad1 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0xff, 0xff, 0xdf, 0x7f, 0xfc, 0x00, 0x00, 0xff, 0xff, 0xc0, 0xff, 0xfe, 0x00, 0x00, 0xff, 0xf9, 0xe1, 0xff, 0xff, 0x00, 0x00, 0xff, 0xf9, 0xff, 0xff, 0xff, 0x00, 0x00, 0xff, 0xf3, 0xff, 0xff, 0xff, 0x00, 0x00, 0xff, 0xe7, 0xff, 0xff, 0xff, 0x00, 0x00, 0x7f, 0x8f, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x1f, 0xff, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x7f, 0xfe, 0x1f, 0xf8, 0x00, 0x00, 0x00, 0x7f, 0xfc, 0xc0, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xfb, 0x9f, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xfd, 0xcf, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xfc, 0xcf, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xfc, 0xcf, 0xd8, 0x00, 0x00, 0x03, 0xff, 0xfe, 0x1f, 0xbc, 0x00, 0x00, 0x03, 0xff, 0xff, 0x3f, 0x3c, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfe, 0x3c, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x00, 0x00, 0x01, 0x87, 0x80, 0x00, 0x00, 0x00, 0x00, 0x03, 0x8f, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0x9e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0x1c, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char sad_sad2 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xc0, 0x80, 0x00, 0x00, 0x00, 0x07, 0xff, 0xe1, 0xc0, 0x00, 0x00, 0x03, 0x03, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xf7, 0xf0, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0xf8, 0x00, 0x00, 0xff, 0xef, 0xff, 0xff, 0xff, 0x00, 0x03, 0xff, 0xff, 0xe1, 0xff, 0xff, 0xc0, 0x03, 0xff, 0xff, 0xe3, 0xff, 0xff, 0xc0, 0x03, 0xff, 0xff, 0xf7, 0xff, 0xdf, 0xc0, 0x03, 0xf8, 0xff, 0xff, 0xff, 0xe3, 0x80, 0x03, 0xe0, 0xff, 0xdf, 0x7f, 0xe0, 0x00, 0x01, 0xc0, 0xff, 0x8e, 0x3f, 0xe0, 0x00, 0x00, 0x00, 0xff, 0x71, 0xdf, 0xe0, 0x00, 0x00, 0x01, 0xff, 0xfb, 0xff, 0xe0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xe6, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xf7, 0x00, 0x00, 0x19, 0xff, 0xff, 0xff, 0xf3, 0x80, 0x00, 0x39, 0xff, 0xff, 0xff, 0xf3, 0x80, 0x00, 0x39, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x39, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x39, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xf1, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xc0, 0x00, 0x00, 0x00, 0xfc, 0x00, 0x0f, 0xe0, 0x00, 0x00, 0x00, 0xfc, 0x00, 0x07, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char sad_sad3 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0x70, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x39, 0xff, 0x0e, 0x00, 0x00, 0x00, 0x01, 0xc1, 0xff, 0xff, 0x00, 0x00, 0x00, 0x03, 0xe3, 0xff, 0xff, 0x80, 0x00, 0x00, 0x07, 0xff, 0xff, 0xef, 0xc0, 0x00, 0x00, 0x0f, 0xdf, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1f, 0xff, 0xc7, 0xff, 0xf0, 0x00, 0x00, 0x3f, 0xff, 0xc3, 0xff, 0xf8, 0x00, 0x00, 0x7f, 0xff, 0xe7, 0xff, 0xfc, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x00, 0x00, 0xfe, 0x7f, 0xff, 0xfe, 0x7f, 0x00, 0x00, 0xfe, 0x7f, 0xc1, 0xfe, 0x7f, 0x00, 0x00, 0xfc, 0xff, 0x80, 0xff, 0x7e, 0x00, 0x00, 0xf9, 0xff, 0x7f, 0x7f, 0xbc, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xce, 0x00, 0x00, 0x13, 0xff, 0xff, 0xff, 0xe7, 0x00, 0x00, 0x33, 0xff, 0xff, 0xff, 0xe7, 0x00, 0x00, 0x73, 0xff, 0xff, 0xff, 0xe7, 0x00, 0x00, 0x73, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x73, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x73, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xc7, 0x80, 0x00, 0x00, 0x00, 0x38, 0x00, 0x1f, 0xc0, 0x00, 0x00, 0x00, 0x7c, 0x00, 0x1f, 0xc0, 0x00, 0x00, 0x00, 0x7f, 0x00, 0x1f, 0xc0, 0x00, 0x00, 0x00, 0x7e, 0x00, 0x0f, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char sad_sad4 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xf3, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x3b, 0xf8, 0x07, 0x00, 0x00, 0x00, 0x00, 0x37, 0xfc, 0x0f, 0x80, 0x00, 0x00, 0x00, 0x2f, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x9f, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x3f, 0xfe, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x9f, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xcf, 0xf0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xcf, 0xf0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xcf, 0xf0, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xcf, 0xf0, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xcf, 0xf0, 0x00, 0x00, 0x7f, 0xfe, 0xff, 0xef, 0xf0, 0x00, 0x00, 0x7f, 0xfc, 0x7f, 0xf7, 0xe0, 0x00, 0x00, 0x7f, 0xfb, 0x8f, 0xf8, 0x00, 0x00, 0x00, 0x7f, 0xc7, 0xc3, 0xfc, 0x00, 0x00, 0x00, 0x3f, 0x87, 0xf1, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x61, 0xf3, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x1f, 0x0f, 0xff, 0xf0, 0x00, 0x00, 0x04, 0xff, 0xfe, 0xff, 0xf0, 0x00, 0x00, 0x01, 0xff, 0xfc, 0xff, 0xf8, 0x00, 0x00, 0x09, 0xff, 0xf9, 0xff, 0xfc, 0x80, 0x00, 0x09, 0xff, 0xf9, 0xdf, 0xfc, 0x80, 0x00, 0x01, 0xff, 0xfc, 0x1f, 0xfc, 0x00, 0x00, 0x01, 0xff, 0xfe, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0xff, 0xff, 0x1f, 0xf8, 0x00, 0x00, 0x00, 0x7f, 0xff, 0x0f, 0xc0, 0x00, 0x00, 0x3e, 0x7f, 0xfe, 0xf7, 0x80, 0x00, 0x00, 0x3f, 0xe3, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x1f, 0xc0, 0x07, 0xf8, 0x00, 0x00, 0x00, 0x0f, 0x80, 0x03, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char sad_sad5 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xf7, 0xf4, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xf8, 0x0e, 0x00, 0x00, 0x00, 0x00, 0x73, 0xfc, 0x1f, 0x00, 0x00, 0x00, 0x00, 0xe7, 0xff, 0xff, 0x80, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x7f, 0xc0, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x3f, 0xe0, 0x00, 0x00, 0x00, 0x8f, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x07, 0xff, 0xff, 0xdf, 0xf0, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xcf, 0xf0, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xcf, 0xf0, 0x00, 0x00, 0x7f, 0xfc, 0x7f, 0xcf, 0xf0, 0x00, 0x00, 0x7f, 0xf8, 0x07, 0xcf, 0xf0, 0x00, 0x00, 0x7f, 0xf3, 0x83, 0xcf, 0xf0, 0x00, 0x00, 0x1f, 0xc7, 0xf1, 0xe7, 0xe0, 0x00, 0x00, 0x00, 0x0f, 0xf3, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xf3, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xf3, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x3f, 0xe0, 0xff, 0xf0, 0x00, 0x00, 0x04, 0xdf, 0xc8, 0xff, 0xf8, 0x00, 0x00, 0x0c, 0xe0, 0x13, 0xff, 0xfc, 0x00, 0x00, 0x0d, 0xf0, 0x33, 0xff, 0xfc, 0x00, 0x00, 0x0b, 0xff, 0xf3, 0xdf, 0xfc, 0x80, 0x00, 0x07, 0xff, 0xf8, 0x3f, 0xfc, 0x80, 0x00, 0x03, 0xff, 0xfc, 0x7f, 0xfc, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0xff, 0xff, 0x9f, 0xf8, 0x00, 0x00, 0x00, 0x7f, 0xff, 0x0f, 0xc0, 0x00, 0x00, 0x3e, 0x7f, 0xfe, 0xf7, 0x80, 0x00, 0x00, 0x3f, 0xe3, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x1f, 0xc0, 0x07, 0xf8, 0x00, 0x00, 0x00, 0x0f, 0x80, 0x03, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char sad_sad6 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x3b, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x07, 0xfd, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xfe, 0x1f, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0x0f, 0x00, 0x00, 0x00, 0x00, 0x5f, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x3f, 0xff, 0xff, 0x7f, 0xc0, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x03, 0xff, 0xff, 0x9f, 0xf8, 0x00, 0x00, 0x00, 0xff, 0xff, 0x9f, 0xf8, 0x00, 0x00, 0x00, 0x7e, 0x3f, 0xdf, 0xf8, 0x00, 0x00, 0x00, 0x3c, 0x1f, 0xcf, 0xf8, 0x00, 0x00, 0x00, 0x41, 0xe3, 0xcf, 0xf8, 0x00, 0x00, 0x00, 0xe3, 0xf7, 0xe7, 0xf0, 0x00, 0x00, 0x01, 0xff, 0xff, 0xf3, 0xe0, 0x00, 0x00, 0x09, 0xff, 0xfd, 0xf8, 0x00, 0x00, 0x00, 0x09, 0xff, 0xf9, 0xfc, 0x00, 0x00, 0x00, 0x09, 0xff, 0xf3, 0xff, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xe7, 0x7f, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xf0, 0xff, 0x80, 0x00, 0x00, 0x03, 0xff, 0xf9, 0xff, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x38, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x18, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0x08, 0x00, 0x00, 0x01, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0x83, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0x00, 0x00, 0x00, 0x00, 0x08, 0x00, 0x0f, 0x00, 0x00, 0x00, 0x00, 0x0f, 0x00, 0x1e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char sad_sad7 [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x1d, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x03, 0xfe, 0x7f, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0x07, 0x80, 0x00, 0x00, 0x00, 0x37, 0xff, 0x8f, 0x80, 0x00, 0x00, 0x00, 0x63, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x0f, 0xff, 0xff, 0x9f, 0xe0, 0x00, 0x00, 0x1f, 0xff, 0xff, 0x9f, 0xe0, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x03, 0xff, 0xff, 0xdf, 0xfc, 0x00, 0x00, 0x00, 0x7f, 0x07, 0xcf, 0xfc, 0x00, 0x00, 0x00, 0x3e, 0x03, 0xcf, 0xfc, 0x00, 0x00, 0x00, 0x00, 0xf8, 0xe7, 0xfc, 0x00, 0x00, 0x00, 0x01, 0xe0, 0xe7, 0xfc, 0x00, 0x00, 0x00, 0x1f, 0xc7, 0xf7, 0xfc, 0x00, 0x00, 0x00, 0x3c, 0x0f, 0xfb, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xfc, 0x00, 0x00, 0x00, 0x04, 0xc3, 0xfe, 0xfe, 0x00, 0x00, 0x00, 0x04, 0xff, 0xfd, 0xff, 0xe0, 0x00, 0x00, 0x01, 0xff, 0xfb, 0xff, 0xe0, 0x00, 0x00, 0x01, 0xff, 0xf3, 0x3f, 0xe0, 0x00, 0x00, 0x01, 0xff, 0xf8, 0x7f, 0xc0, 0x00, 0x00, 0x01, 0xff, 0xfc, 0xff, 0x80, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0x90, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0x90, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0x10, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc3, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x00, 0x07, 0x80, 0x07, 0x80, 0x00, 0x00, 0x00, 0x07, 0x80, 0x0f, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; // Array of all bitmaps for convenience. (Total bytes used to store images in PROGMEM = 3328) const unsigned char* sad_allArray[8] = { sad_sad0, sad_sad1, sad_sad2, sad_sad3, sad_sad4, sad_sad5, sad_sad6, sad_sad7 }; const unsigned char misc_cheer [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7c, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0xf7, 0xbf, 0xf8, 0x00, 0x00, 0x00, 0x01, 0xf3, 0x7f, 0xc0, 0x00, 0x00, 0x00, 0x1f, 0xf2, 0xf9, 0x80, 0x00, 0x00, 0x00, 0x3f, 0xf1, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf0, 0x1e, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x1f, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0xff, 0x86, 0x00, 0x00, 0x03, 0xff, 0xdf, 0xff, 0xc6, 0x00, 0x00, 0x07, 0xdf, 0x8f, 0xbf, 0xe6, 0x00, 0x03, 0xff, 0xff, 0x0f, 0xc3, 0xf0, 0x00, 0x03, 0xff, 0xff, 0x1f, 0x81, 0xf0, 0x00, 0x03, 0xfc, 0xff, 0xff, 0x3c, 0xf8, 0x00, 0x03, 0xf0, 0xef, 0xfe, 0x04, 0xfc, 0x00, 0x00, 0x00, 0xe7, 0xf8, 0x0c, 0xfc, 0x00, 0x00, 0x00, 0xe7, 0xf1, 0xbc, 0xfc, 0x00, 0x00, 0x00, 0xf0, 0x0f, 0xc1, 0xfc, 0x00, 0x00, 0x00, 0xf8, 0x1f, 0xe3, 0xfc, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x04, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x0c, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x3c, 0xff, 0xff, 0xff, 0xfd, 0xc0, 0x00, 0x38, 0xff, 0xff, 0xff, 0xfb, 0xc0, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xf7, 0xc0, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xe7, 0x80, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xff, 0x80, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; const unsigned char misc_dead [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0xff, 0xa0, 0x00, 0x00, 0x0e, 0xff, 0xfe, 0x3f, 0xc0, 0x00, 0x00, 0x00, 0xff, 0xff, 0x1f, 0xe0, 0x00, 0x00, 0x01, 0xfe, 0xff, 0xcf, 0xf0, 0x00, 0x00, 0x19, 0xff, 0x03, 0xcf, 0xf8, 0x00, 0x00, 0x18, 0xff, 0x07, 0xe7, 0xf8, 0x00, 0x00, 0x10, 0xff, 0x3f, 0xf3, 0xf8, 0x00, 0x00, 0x19, 0xf8, 0x0f, 0xf3, 0xf8, 0x00, 0x00, 0x1f, 0xfc, 0x0f, 0xfb, 0xfc, 0x00, 0x00, 0x1f, 0xff, 0x3f, 0xf9, 0xfc, 0x00, 0x00, 0xc7, 0xff, 0x3f, 0xf9, 0xfc, 0x00, 0x00, 0xe7, 0xff, 0xff, 0xfc, 0xfc, 0x00, 0x01, 0xff, 0xff, 0xfc, 0xfe, 0x4c, 0x00, 0x03, 0xff, 0xff, 0xfc, 0xfe, 0x61, 0x80, 0x03, 0xff, 0xff, 0xfc, 0xff, 0x71, 0x80, 0x03, 0xff, 0xff, 0xfc, 0xff, 0x39, 0x80, 0x01, 0xff, 0xff, 0xfe, 0x7f, 0x30, 0x00, 0x00, 0x3f, 0xf3, 0xf8, 0x7f, 0x00, 0x00, 0x00, 0x1f, 0xe1, 0xf0, 0x7f, 0x00, 0x00, 0x00, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xbf, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7c, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; // 'bone', 24x24px const unsigned char misc_bone [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x03, 0x80, 0x00, 0x03, 0xc0, 0x00, 0x01, 0xf8, 0x00, 0x01, 0xfc, 0x00, 0x03, 0xfc, 0x00, 0x02, 0x1c, 0x00, 0x08, 0x00, 0x00, 0x10, 0x00, 0x00, 0x60, 0x00, 0x3f, 0xc0, 0x00, 0x3f, 0x80, 0x00, 0x7f, 0x80, 0x00, 0x0f, 0x00, 0x00, 0x07, 0x00, 0x00, 0x03, 0x80, 0x00, 0x03, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; // 'ball', 24x24px const unsigned char misc_ball [] PROGMEM = { 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xb6, 0xff, 0xff, 0x00, 0x7f, 0xfe, 0x31, 0x3f, 0xf9, 0xf3, 0x9f, 0xf3, 0x33, 0xcf, 0xe6, 0x11, 0xcf, 0xf4, 0xdc, 0xcf, 0xe4, 0xce, 0x2f, 0xe4, 0xcf, 0xef, 0xe4, 0x1c, 0xef, 0xf3, 0x38, 0x4f, 0xfb, 0xf3, 0x0f, 0xfd, 0xe7, 0x1f, 0xfc, 0x0e, 0x3f, 0xff, 0x00, 0x7f, 0xff, 0xf7, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff }; char *tasks[] = {"empty","empty","empty","empty","empty","empty","empty","empty","empty","empty"}; unsigned long duetime[10]; int weight[10]; int type[10]; // 1 is health, 2 is happiness char *tasklist[] = {"Food", "Clean", "Break", "Sleep", "Exercise", "60-223", "67-262", "15-213", "05-392", "Research" }; int nextemptytask = 0; int numtasks = 0; int cursor = 0; int cursor2 = 0; const int butt1 = 4; const int butt2 = 3; const int butt3 = 2; const int speaker = 5; const int motor = 6; int butt1state = 0; int butt2state = 0; int butt3state = 0; int state = 0; /* 0 = idle 1 = input task (if they select sleep mode, it snoozes for 8 hours and doesn't draw anything) 2 = delete task 3 = set time for task 4 = sleep mode 5 = dead (press middle to reset) 6 = show tasks */ unsigned long idletime; unsigned long frametime; unsigned long buttontime; unsigned long countdown; unsigned long decrement; bool deathcountdown; // hour = 3600000 // minute = 60000 // this variable is changeable depending on how you want // to increment things unsigned long hour = 60000; int frame = 0; int happiness = 100; int health = 100; int animation = 0; int tasksdone = 0; void setup(void) { Serial.begin(9600); pinMode(butt1, INPUT); pinMode(butt2, INPUT); pinMode(butt3, INPUT); pinMode(motor, OUTPUT); digitalWrite(butt1, HIGH); digitalWrite(butt2, HIGH); digitalWrite(butt3, HIGH); state = 0; u8g.setFont(u8g_font_helvR08); idletime = millis(); frametime = millis(); buttontime = millis(); countdown = 0; delay(1000); animation = (rand() % (3 + 1)) * 2; // sets idle animation on a random animation for (int i = 0; i < 9; i++) { weight[i] = 0; type[i] = 0; duetime[i] = 0; } } void loop(void) { u8g.setFont(u8g_font_helvR08); butt1state = digitalRead(butt1); butt2state = digitalRead(butt2); butt3state = digitalRead(butt3); bool butt1press = false; bool butt2press = false; bool butt3press = false; // sets if the buttons have been pressed if (butt1state == LOW && millis() - buttontime >= 250) { buttontime = millis(); butt1press = true; Serial.println("button 1 pressed"); } else if (butt2state == LOW && millis() - buttontime >= 250) { buttontime = millis(); butt2press = true; Serial.println("button 2 pressed"); } else if (butt3state == LOW && millis() - buttontime >= 250) { buttontime = millis(); butt3press = true; Serial.println("button 3 pressed"); } // the buddy starts dying if (state != 5 && !deathcountdown && (happiness == 0 || health == 0)) { playSad(); countdown = millis(); deathcountdown = true; } // this var can be changed depending on how long before something dies int deathcount = 10000 if (deathcountdown && millis() - countdown >= deathcount) { state = 5; countdown = 0; deathcountdown = false; } if (health >= 100) health = 100; if (happiness >= 100) happiness = 100; if (happiness <= 0) happiness = 0; if (health <= 0) health = 0; // goes through the modes checkDelete(); if (state == 0) { doIdle(butt1press, butt2press, butt3press); } else if (state == 1) { doInputTask(butt1press, butt2press, butt3press); } else if (state == 2) { doDeleteTask(butt1press, butt2press, butt3press); } else if (state == 3) { doSetTask(butt1press, butt2press, butt3press); } else if (state == 4) { doSleep(butt2press); // takes in the center button to wake up :D } else if (state == 5) { doDead(butt2press); // takes in the center button to reset } else if (state == 6) { doShowTasks(butt2press); // takes in the center button to reset } //decrements happiness and health if (millis() - decrement >= 5000) { decrement = millis(); happiness -= 1; health -= 1; } } // plays happy noises void playCheer(void){ // plays a little ditty digitalWrite(motor, HIGH); tone(speaker, NOTE_D5, 200); delay(200); noTone(speaker); tone(speaker, NOTE_E5, 200); delay(200); noTone(speaker); tone(speaker, NOTE_F5, 200); delay(200); noTone(speaker); tone(speaker, NOTE_FS5, 1000); delay(500*1.3); noTone(speaker); digitalWrite(motor, LOW); } //plays sad noises void playSad(void){ digitalWrite(motor, HIGH); tone(speaker, NOTE_D5, 1000); delay(1000); digitalWrite(motor, LOW); noTone(speaker); } //shows all the tasks void doShowTasks(int butt2press) { if (butt2press) { state = 0; } u8g.firstPage(); do { drawDeleteTasks(); } while(u8g.nextPage()); } // dead state void doDead(int butt2press) { // resets if butt2 is pressed if (butt2press) { state = 0; happiness = 90; health = 90; tasksdone -= 1; } u8g.firstPage(); do { u8g.drawStr( 0, 10, "Dead, dying, gone..."); u8g.drawBitmapP( 56, 10, 7, 56, misc_dead); } while(u8g.nextPage()); } // asleep state void doSleep(int butt2press) { // wakes it up if (butt2press) { state = 0; countdown = 0; health -= 10; // from being woken up if (health > 100) { health = 100; } beSad(); } // if it's 8 hours of sleep if (millis() - countdown == 28800000) { state = 0; countdown = 0; health += 15; if (health > 100) { health = 100; } } u8g.firstPage(); do { u8g.drawStr( 0, 10, "Asleep..."); u8g.drawBitmapP( 56, 10, 7, 56, health_sleep); } while(u8g.nextPage()); } // checks through the list of tasks to see if any of them are over void checkDelete(void) { for (int i = 0; i < numtasks; i++) { if (millis() >= duetime[i]) { Serial.println(tasks[i]); cursor = i; if (type[i] == 1) { health -= weight[i]; } else if (type[i] == 2) { happiness -= weight[i]; } deleteTask(); beSad(); tasksdone -= 1; } } } // deletes a task void deleteTask(void) { tasks[cursor] = "empty"; duetime[cursor] = 0; weight[cursor] = 0; type[cursor] = 0; for (int i = cursor; i < 9; i++) { tasks[i] = tasks[i + 1]; duetime[i] = duetime[i + 1]; weight[i] = weight[i + 1]; type[i] = type[i + 1]; } tasks[9] = "empty"; duetime[9] = 0; weight[9] = 0; type[9] = 0; cursor = 0; cursor2 = 0; nextemptytask -= 1; tasksdone += 1; numtasks -= 1; } // deletes a task void doDeleteTask(int butt1press, int butt2press, int butt3press) { if (nextemptytask == 0 && butt2press) { state = 0; u8g.firstPage(); do { u8g.drawStr( 24, 10, "No tasks!"); } while(u8g.nextPage()); } else { u8g.firstPage(); do { drawDeleteTasks(); } while(u8g.nextPage()); } if (nextemptytask != 0) { if (butt1press && cursor < numtasks - 1) { Serial.println("Pressed"); cursor += 1; } else if (butt3press && cursor > 0) { Serial.println("Pressed"); cursor -= 1; } else if (butt2press) { if (type[cursor] == 1) { health += weight[cursor]; // bonus for finishing an hour early if (millis() - duetime[cursor] > hour) { health += weight[cursor]; } if (health >= 100) { health = 100; } } else if (type[cursor] == 2) { happiness += weight[cursor]; // hour bonus if (millis() - duetime[cursor] > hour) { health += weight[cursor]; } if (happiness >= 100) { happiness = 100; } } deleteTask(); state = 0; // reverts to idle display u8g.firstPage(); do { u8g.drawBitmapP( 32, 10, 7, 56, misc_cheer); } while(u8g.nextPage()); playCheer(); } } } // Draws all the tasks to delete void drawDeleteTasks(void) { u8g.drawStr( 0, 10, "List of tasks:"); for (int i = 0; i < 5; i++) { u8g.drawStr(10, i * 10 + 24, tasks[i]); char time[10]; if (duetime[i] != 0) { itoa((duetime[i] - millis()) / 1000,time,10); u8g.drawStr(50, i * 10 + 24, time); } } for (int i = 5; i < 10; i++) { u8g.drawStr(70, (i - 5) * 10 + 24, tasks[i]); char time[10]; if (duetime[i] != 0) { itoa((duetime[i] - millis()) / 1000,time,10); u8g.drawStr(110, (i - 5) * 10 + 24, time); } } if (cursor < 5) { u8g.drawCircle(5, cursor * 10 + 20, 1); } else if (cursor >= 5) { u8g.drawCircle(55, (cursor-5) * 10 + 20, 1); } } // sets a time for a task void doSetTask(int butt1press, int butt2press, int butt3press) { if (butt2press) { unsigned long duet = millis() + (cursor2 * hour); Serial.println(duet); tasks[nextemptytask] = tasklist[cursor]; switch(cursor) { case 0: // food type[nextemptytask] = 1; weight[nextemptytask] = 10; duetime[nextemptytask] = duet; break; case 1: // clean type[nextemptytask] = 1; weight[nextemptytask] = 5; duetime[nextemptytask] = duet; break; case 2: // break type[nextemptytask] = 1; weight[nextemptytask] = 5; duetime[nextemptytask] = duet; break; case 3: // sleep type[nextemptytask] = 1; weight[nextemptytask] = 15; duetime[nextemptytask] = duet; break; case 4: // exercise type[nextemptytask] = 1; weight[nextemptytask] = 5; duetime[nextemptytask] = duet; break; case 5: // 60-223 type[nextemptytask] = 2; weight[nextemptytask] = 10; duetime[nextemptytask] = duet; break; case 6: type[nextemptytask] = 2; weight[nextemptytask] = 10; duetime[nextemptytask] = duet; break; case 7: type[nextemptytask] = 2; weight[nextemptytask] = 15; duetime[nextemptytask] = duet; break; case 8: type[nextemptytask] = 2; weight[nextemptytask] = 10; duetime[nextemptytask] = duet; break; case 9: type[nextemptytask] = 2; weight[nextemptytask] = 10; duetime[nextemptytask] = duet; break; default : Serial.println("invalid cursor setting\n"); } cursor = 0; cursor2 = 0; nextemptytask += 1; numtasks += 1; happiness += 5; state = 0; // reverts to idle display } else if (butt1press && cursor2 < 24) { cursor2 += 1; } else if (butt3press && cursor2 >= 1) { cursor2 -= 1; } u8g.firstPage(); do { drawSetTasks(); } while(u8g.nextPage()); } // a timer for how long to do it // draws timing for tasks void drawSetTasks(void) { u8g.drawStr(10, 10, "Done by?"); char curse[7]; itoa(cursor2,curse,10); u8g.drawStr(56, 32, curse); u8g.drawStr(56, 50, "minutes"); } // inputs a new task void doInputTask(int butt1press, int butt2press, int butt3press) { if (butt2press) { state = 3; // if sleep was selected if (cursor == 3) { state = 4; cursor = 0; cursor2 = 0; } cursor2 = 1; } else if (butt1press && cursor != 9) { cursor += 1; } else if (butt3press && cursor != 0) { cursor -= 1; } u8g.firstPage(); do { drawInputScreen(); } while(u8g.nextPage()); } // draws the input screen for adding a new task void drawInputScreen(void) { u8g.drawStr( 0, 10, "Add a new task to your list!"); for (int i = 0; i < 5; i++) { u8g.drawStr(16, i * 10 + 24, tasklist[i]); } for (int i = 5; i < 10; i++) { u8g.drawStr(64, (i - 5) * 10 + 24, tasklist[i]); } if (cursor < 5) { u8g.drawCircle(10, cursor * 10 + 20, 1); } else if (cursor >= 5) { u8g.drawCircle(56, (cursor-5) * 10 + 20, 1); } } // default for being sad after having missed a task void beSad(void) { u8g.firstPage(); do { u8g.drawBitmapP( 56, 10, 7, 56, sad_allArray[4]); } while(u8g.nextPage()); playSad(); } // idle screen void doIdle(bool butt1press, bool butt2press, bool butt3press) { if (butt1press) { state = 1; Serial.println(state); } else if (butt2press) { state = 6; } else if (butt3press) { state = 2; Serial.println(state); } else if (millis() - idletime >= 5000) { idletime = millis(); animation = (rand() % (3 + 1)) * 2; } else if (millis() - frametime >= 500) { frame += 1; if (frame == 2) frame = 0; frametime = millis(); u8g.firstPage(); do { drawIdle(animation, frame); } while(u8g.nextPage()); } } // finds how close the next upcoming task is in milliseconds unsigned long nextTask(void) { if (numtasks == 0) { return 0; } int leastnum = 0; unsigned long least = duetime[0] - millis(); for (int i = 0; i < numtasks; i++) { if (duetime[i] - millis() < least) { least = duetime[i] - millis(); leastnum = i; } } return leastnum; } // draws the idle screen void drawIdle(int animation, int frame) { u8g.drawStr( 0, 10, "Happiness: "); char happy[7]; itoa(happiness, happy, 10); u8g.drawStr(54, 10, happy); u8g.drawStr( 0, 25, "Health: "); char hea[7]; itoa(health,hea,10); u8g.drawStr(35, 25, hea); u8g.drawStr(0, 40, "Next Task:"); unsigned long displayTime = 0; if (numtasks != 0) { int leastnum = nextTask(); displayTime = duetime[leastnum] - millis(); displayTime = displayTime / 1000; char distime[10]; itoa(displayTime,distime,10); u8g.drawStr(0, 50, tasks[leastnum]); u8g.drawStr(40, 50, distime); } else if (numtasks == 0) { char distime[10]; itoa(displayTime,distime,10); u8g.drawStr(0, 50, distime); } if (happiness > 50 && health >50 ) { u8g.drawBitmapP( 56, 10, 7, 56, happy_allArray[animation + frame]); } else if (happiness <= 50 || health < 50) { u8g.drawBitmapP( 56, 10, 7, 56, sad_allArray[animation + frame]); } if (tasksdone >= 5) { u8g.drawBitmapP(38, 40, 3, 24, misc_bone); } }
Pitches File Used:
#define NOTE_B0 31 #define NOTE_C1 33 #define NOTE_CS1 35 #define NOTE_D1 37 #define NOTE_DS1 39 #define NOTE_E1 41 #define NOTE_F1 44 #define NOTE_FS1 46 #define NOTE_G1 49 #define NOTE_GS1 52 #define NOTE_A1 55 #define NOTE_AS1 58 #define NOTE_B1 62 #define NOTE_C2 65 #define NOTE_CS2 69 #define NOTE_D2 73 #define NOTE_DS2 78 #define NOTE_E2 82 #define NOTE_F2 87 #define NOTE_FS2 93 #define NOTE_G2 98 #define NOTE_GS2 104 #define NOTE_A2 110 #define NOTE_AS2 117 #define NOTE_B2 123 #define NOTE_C3 131 #define NOTE_CS3 139 #define NOTE_D3 147 #define NOTE_DS3 156 #define NOTE_E3 165 #define NOTE_F3 175 #define NOTE_FS3 185 #define NOTE_G3 196 #define NOTE_GS3 208 #define NOTE_A3 220 #define NOTE_AS3 233 #define NOTE_B3 247 #define NOTE_C4 262 #define NOTE_CS4 277 #define NOTE_D4 294 #define NOTE_DS4 311 #define NOTE_E4 330 #define NOTE_F4 349 #define NOTE_FS4 370 #define NOTE_G4 392 #define NOTE_GS4 415 #define NOTE_A4 440 #define NOTE_AS4 466 #define NOTE_B4 494 #define NOTE_C5 523 #define NOTE_CS5 554 #define NOTE_D5 587 #define NOTE_DS5 622 #define NOTE_E5 659 #define NOTE_F5 698 #define NOTE_FS5 740 #define NOTE_G5 784 #define NOTE_GS5 831 #define NOTE_A5 880 #define NOTE_AS5 932 #define NOTE_B5 988 #define NOTE_C6 1047 #define NOTE_CS6 1109 #define NOTE_D6 1175 #define NOTE_DS6 1245 #define NOTE_E6 1319 #define NOTE_F6 1397 #define NOTE_FS6 1480 #define NOTE_G6 1568 #define NOTE_GS6 1661 #define NOTE_A6 1760 #define NOTE_AS6 1865 #define NOTE_B6 1976 #define NOTE_C7 2093 #define NOTE_CS7 2217 #define NOTE_D7 2349 #define NOTE_DS7 2489 #define NOTE_E7 2637 #define NOTE_F7 2794 #define NOTE_FS7 2960 #define NOTE_G7 3136 #define NOTE_GS7 3322 #define NOTE_A7 3520 #define NOTE_AS7 3729 #define NOTE_B7 3951 #define NOTE_C8 4186 #define NOTE_CS8 4435 #define NOTE_D8 4699 #define NOTE_DS8 4978 #define END -1