Overview
I created a posture sensor to help me fix my back. It’s a light signal bracelet that tells you at which parts of your back is not in the correct posture.
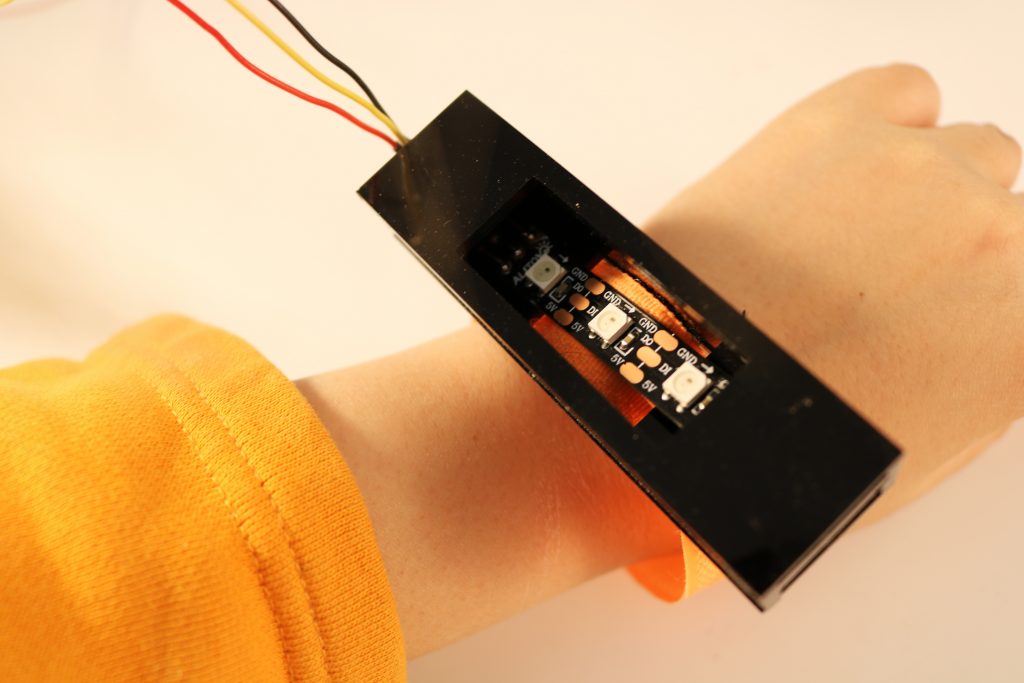
Velcro bracelet to wrap around wrist
The different lights will signal which part of the back is bad by turning into a red light.
Flex sensors are meant to be body taped to the back and the rest of the arduino is housed within a bag. It would have been better if there was some way to implement the flex sensors onto the back without having to tape because it does not work as well and it is uncomfortable.
This bag was made to clip onto the belt loops of your pants and the wires that exited the bag, to be taped onto your back and to the bracelet, would be hidden underneath your clothes. However, it was hard to put everything in the bag because wires would come out from the breadboard and every time I did try to put the arduino components in the bag, something always malfunctioned.
Because of my poor housing I tried to replicate how the device would work by taping the flex sensors to the back of a paper human cut out.
Process
Started off with a simple circuit and code from a tutorial that controlled the brightness of an a single LED light
Moved onto the light strips so I could later implement it into the rectangular watch.
I had difficulty trying to smooth the coding for the lights so that when the posture was good it would be green, but then progress to red depending on how bad the posture was.
Still having troubles with the sensitivity of the sensors in this video. This step is also when I decided I wanted to sense multiple parts of my back so I did two flex sensors sending data to different LED lights on the strip.
Discussion
This project got me really excited because I had a desire to make something that was more personal for me and could benefit me. During class critique, the guest person brought up major points to my design, which transitioned my thinking of this project from a school assigned project to a project creating an everyday object. He pointed out how it would be troublesome to tape to the sensors to one’s back everyday as well as having to hide the wires underneath the clothes. However, my fellow classmates liked my design (despite the lack of usability) of the bag, and brought up points on how it was easier to use for everyday life.
Bumps I encountered during this project were mainly the housing. If I were able to change how I thought during the process, I would have thought more on how to secure the wires better, which in turn could have caused the bag housing to work. Another problem that occurred was within the coding, where for some reason the flex sensors were reading at different values and I had just assumed that they were the same. To elaborate, one of them had a range from 700-900 while the other had a range from 200-500. Since I did not print the value of the second sensor, I did not know why the numbers I inputed into my code was not working, until after I started reading the second sensor. It taught me a valuable lesson to always print your values.
I would really like to go back to work on this project if I had time. Most likely, I would heat wrap the wires together to prevent having multiple wires tangle as well as using a different type of breadboard. Another thing I would still need to consider is how to attach the sensors to my back because even when I asked my table about any possible solutions, none were spoken. Unlike the double transducer where I struggled more with the coding because of my lack attention to the schematic and code structure, this project’s downfall was my physical housing. This was due to less planning on the physical and more focus on the coding.
Schematic
Code
// Fix Your Posture! // Nina Yoo /*Description : This project mounts two flex sensors onto one's back and notifies the user of their bad posture by emitting a red light from the LED*/ #include <PololuLedStrip.h> // Create an ledStrip object and specify the pin it will use. PololuLedStrip<5> ledStrip; // Create a buffer for holding the colors (3 bytes per color). #define LED_COUNT 60 rgb_color colors[LED_COUNT]; //Constants: const int flexPin2 = A1; const int flexPin = A0; //pin A0 to read analog input const int NUM_READINGS = 10; const int NUM_READINGS2 = 10; //Variables: int value; //save analog value int value2; int readings[NUM_READINGS]; int readIndex = 0; int total = 0; int average = 0; int readings2[NUM_READINGS2]; int readIndex2 = 0; int total2 = 0; int average2 = 0; void setup() { pinMode(flexPin, INPUT); pinMode(flexPin2, INPUT); Serial.begin(115200); for (int thisReading = 0; thisReading < NUM_READINGS; thisReading++) { readings[thisReading] = 0; } for (int thisReading2 = 0; thisReading2 < NUM_READINGS2; thisReading2++) { readings2[thisReading2] = 0; } } void loop() { total = total - readings[readIndex]; readings[readIndex] = analogRead(flexPin); total = total + readings [readIndex]; readIndex = readIndex + 1; if (readIndex >= NUM_READINGS) { readIndex = 0; } average = total / NUM_READINGS; Serial.println(average); total2 = total2 - readings2[readIndex2]; readings2[readIndex2] = analogRead(flexPin2); total2 = total2 + readings2[readIndex2]; readIndex2 = readIndex2 + 1; if (readIndex2 >= NUM_READINGS2) { readIndex2 = 0; } average2 = total2 / NUM_READINGS2; Serial.println(average2); value = analogRead(flexPin); Serial.print("flex1 = "); Serial.print(value); //Map value 0-1023 to 0-255 value = map(value, 700, 900, 0, 255); Serial.print("flex1 = "); Serial.println(value); analogWrite(ledPin, value); //Small delay value2 = analogRead(flexPin2); Serial.print("\tflex2 = "); Serial.print(value2); value2 = map(value2, 580, 850, 0, 255); Serial.print("\tflex2 = "); Serial.println(value2); analogWrite(ledPin, value2); colors[0] = rgb_color(value, 255 - value, 10); // Write the colors to the LED strip. ledStrip.write(colors, LED_COUNT); colors[2] = rgb_color(value2, 255 - value2, 10); ledStrip.write(colors, LED_COUNT); }
Comments are closed.