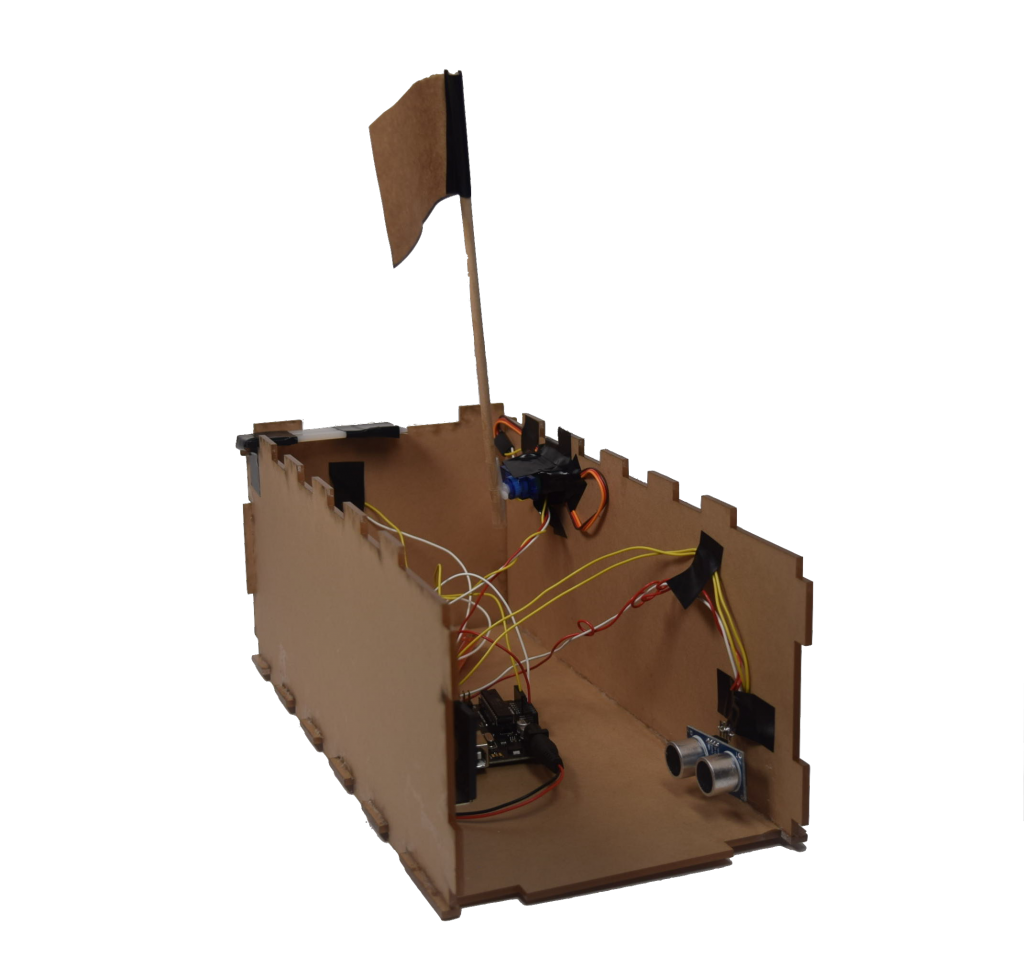
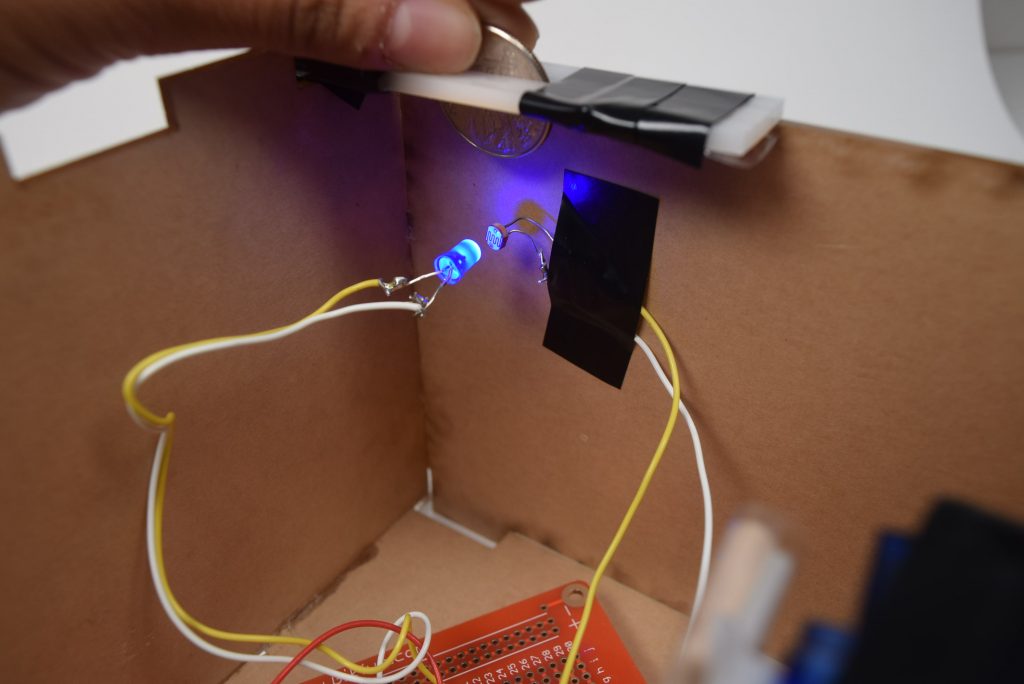
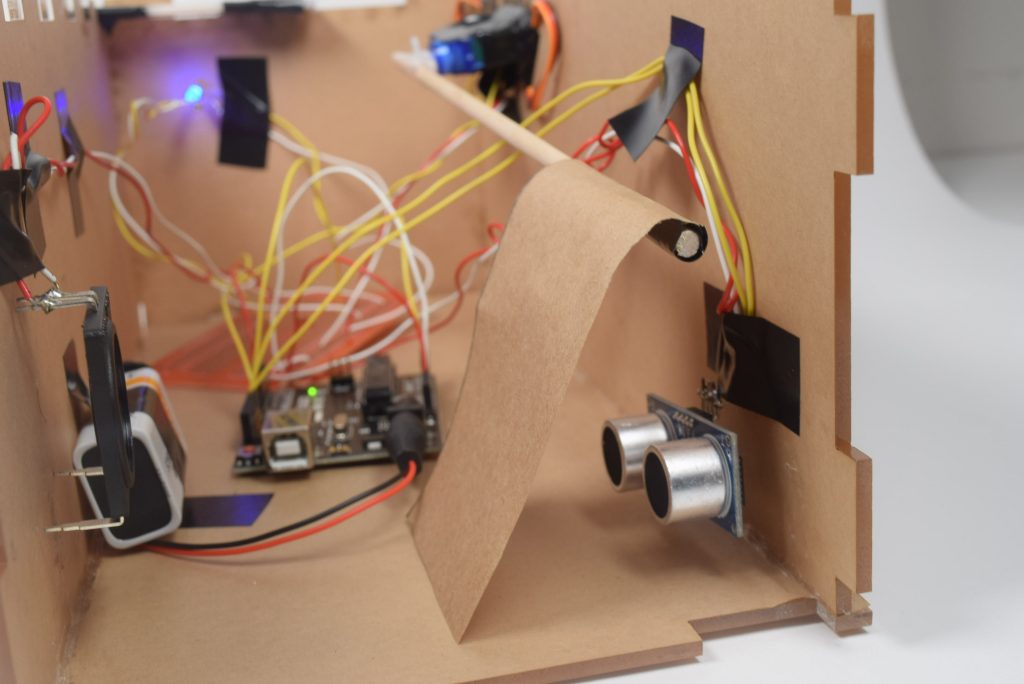
Demo Video
Basic functionality: The user drops a coin into the top of a box. This turns off an LED, which raises a small flag. This causes a short song to play, giving you a lot of satisfaction.
Progress Images and Notes
Schematic
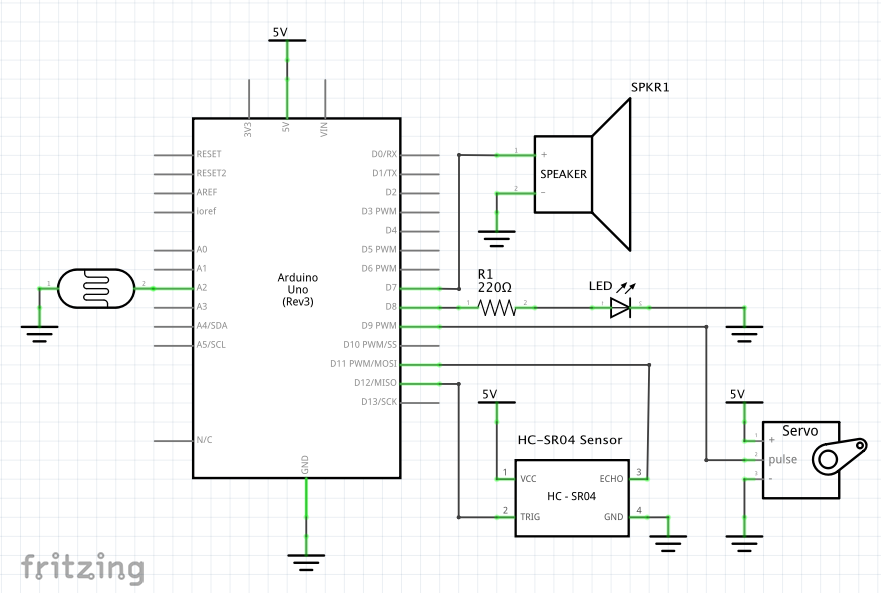
Code
/* Project 1 Eric Mendez (emendez) Tanvi Bhargava (tanvib) Description: The user drops a coin into the top of a box. This turns off an LED which is looking at a photoresistor.The light level goes below the defined threshold for the photoresistor which raises a small flag. The flag was covering a sonar sensor, but now the distance detected is over the defined threshold which causes a short song to play, giving you a lot of satisfaction. */ #include <Servo.h> #include <NewPing.h> #include <TimerFreeTone.h> // pin assignments const int TRIGGER_PIN = 12; const int ECHO_PIN = 11; const int MAX_DISTANCE = 200; //max distance we want for ping const int SERVO_PIN = 9; const int LED_PIN = 8; const int PHOTO_RESISTOR = A2; const int SOUND_PIN = 7; // declare servo and sonar from libraries NewPing sonar(TRIGGER_PIN, ECHO_PIN, MAX_DISTANCE); Servo flagMotor; // note intervals for song int quarter = 250; int eighth = 125; int sixteenth = eighth/2; int D = 587; int E = 659; int F = 698; int G = 784; int A = 880; int melody[] = { D, D, A, F, G, A, G, F, E, D}; int duration[] = { (quarter+eighth), eighth, eighth, eighth, (quarter*4), sixteenth, sixteenth, eighth, (quarter*4), (quarter*4) }; // declare variables used throughout loop int playedSong = 0; int ledState = 1; // 1 - on | 0 - off int lightLevel; //photoresistor int distance; void setup() { Serial.begin(9600); pinMode(LED_PIN, OUTPUT); pinMode(PHOTO_RESISTOR, INPUT_PULLUP); // eliminates need for pullup/pulldown resistor pinMode(SOUND_PIN, OUTPUT); flagMotor.attach(SERVO_PIN); flagMotor.write(90); digitalWrite(LED_PIN, HIGH); digitalWrite(SOUND_PIN, LOW); } void loop() { lightLevel = analogRead(PHOTO_RESISTOR); distance = sonar.ping_cm(); Serial.println(distance); if (lightLevel > 200) { // scale reversed since using input pullup: low -> high light level digitalWrite(LED_PIN, LOW); ledState = 0; // throw flag up flagMotor.write(170); } // case over sonar detection of flag if (ledState == 0 && distance > 10 && playedSong == 0) { // flag goes up and trigger sound Serial.println("play sound"); playedSong = 1; for (int thisNote = 0; thisNote < 10; thisNote++) { // Loop through the notes in the array. TimerFreeTone(SOUND_PIN, melody[thisNote], duration[thisNote]); // Play thisNote for its coresponding duration. delay(50); // Short delay between notes. } } }
Discussion
The base idea for this project was formed within seconds of us starting to brainstorm. Although we continued to consider other designs for double transducers, we eventually settled on our initial idea. Our extra thinking paid off, as some of the components we decided to use were different than originally planned. This saved us some time in deciding which parts were extraneous, and it helped choose more efficient components which saved us some hassle when it came to making the project function as a whole.
While constructing the project, we found it relatively straightforward to create the circuit and write the code so that each action occurred in sequence. However, I spent some more time constructing the box in Solidworks, laser cutting it, and gluing it together. Additionally, we put effort into making the setup functional and easy to use; for example, making sure the coin always fell between the LED and photoresistor.
We also learned some interesting things about the components we were using and how they worked internally. We found that we could not use the ultrasonic rangers and a speaker together due to timing issues within the libraries they both relied on. We had to find an alternate tone library for the speaker, which was called TimerFreeTone. It was similar, but it was necessary to enter both the frequencies and beat lengths in two separate, corresponding matrices. To make the song, I looked up 12-tet note frequencies, saved them as note-named variables, and recreated the tune by looking at the note progressions online.
Comments are closed.