This is a choker that can start recording autonomously when it detects the user humming unconsciously in the bathroom.
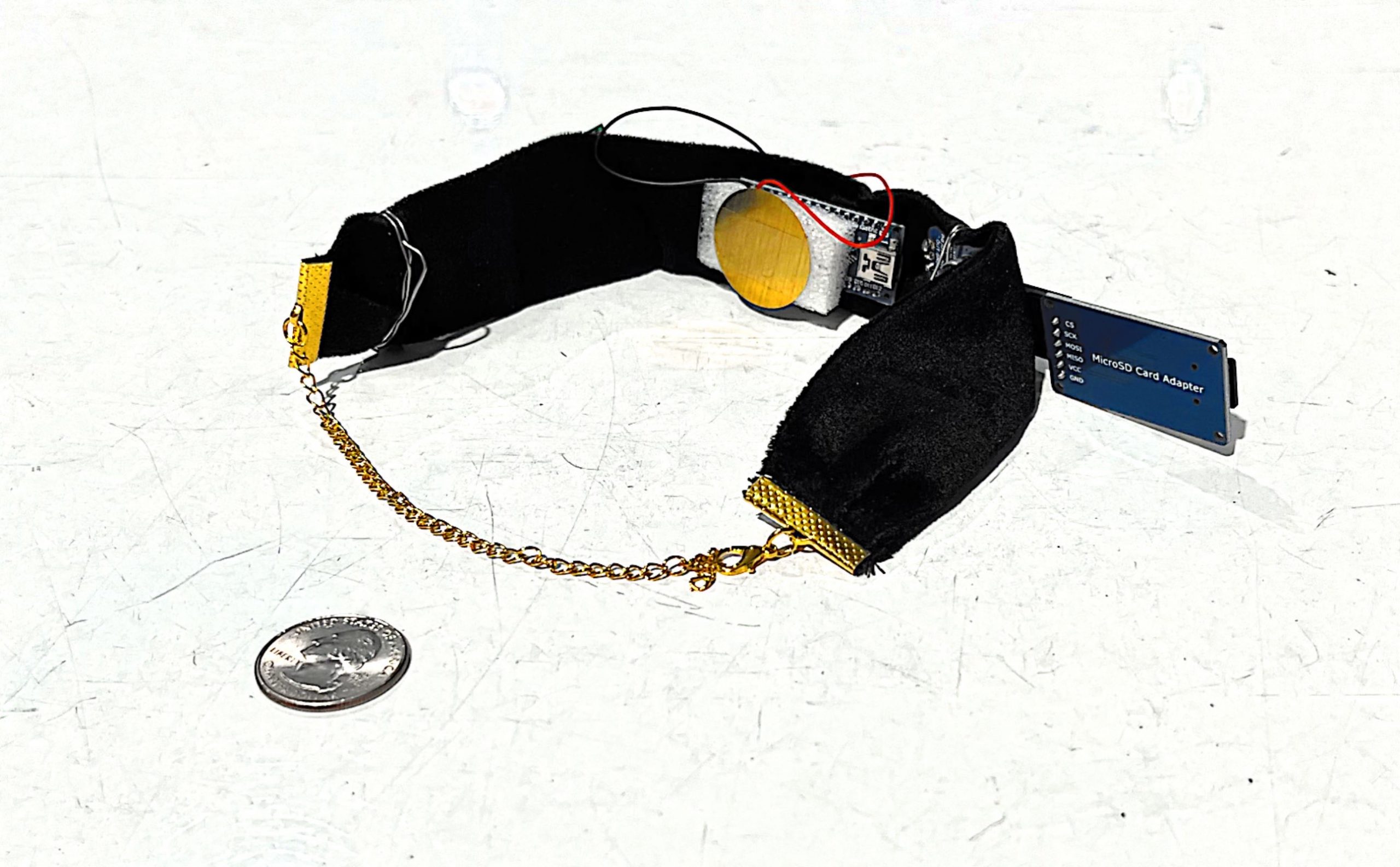
The choker compared to a quarter dollar coin
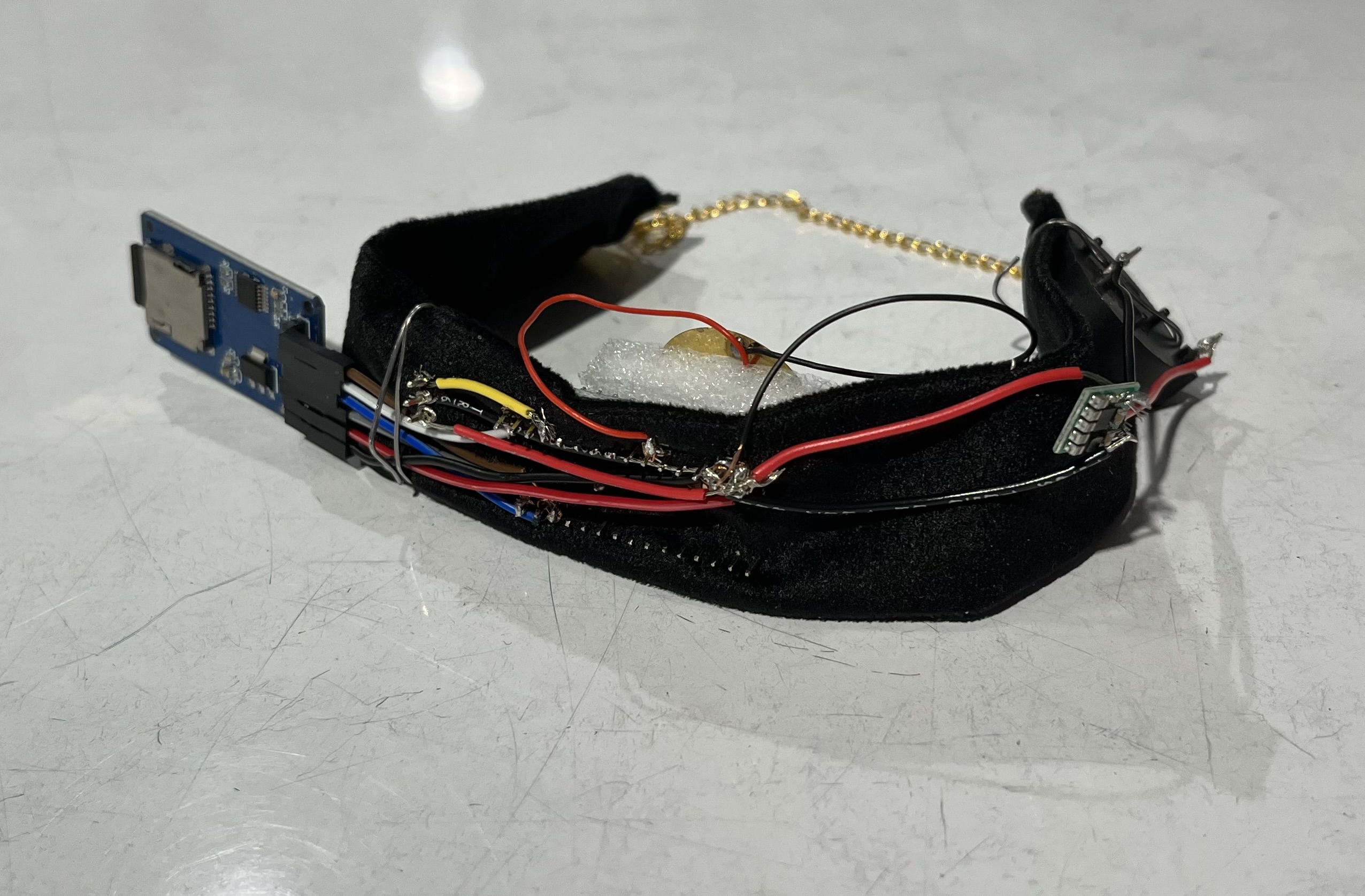
The front look of the choker
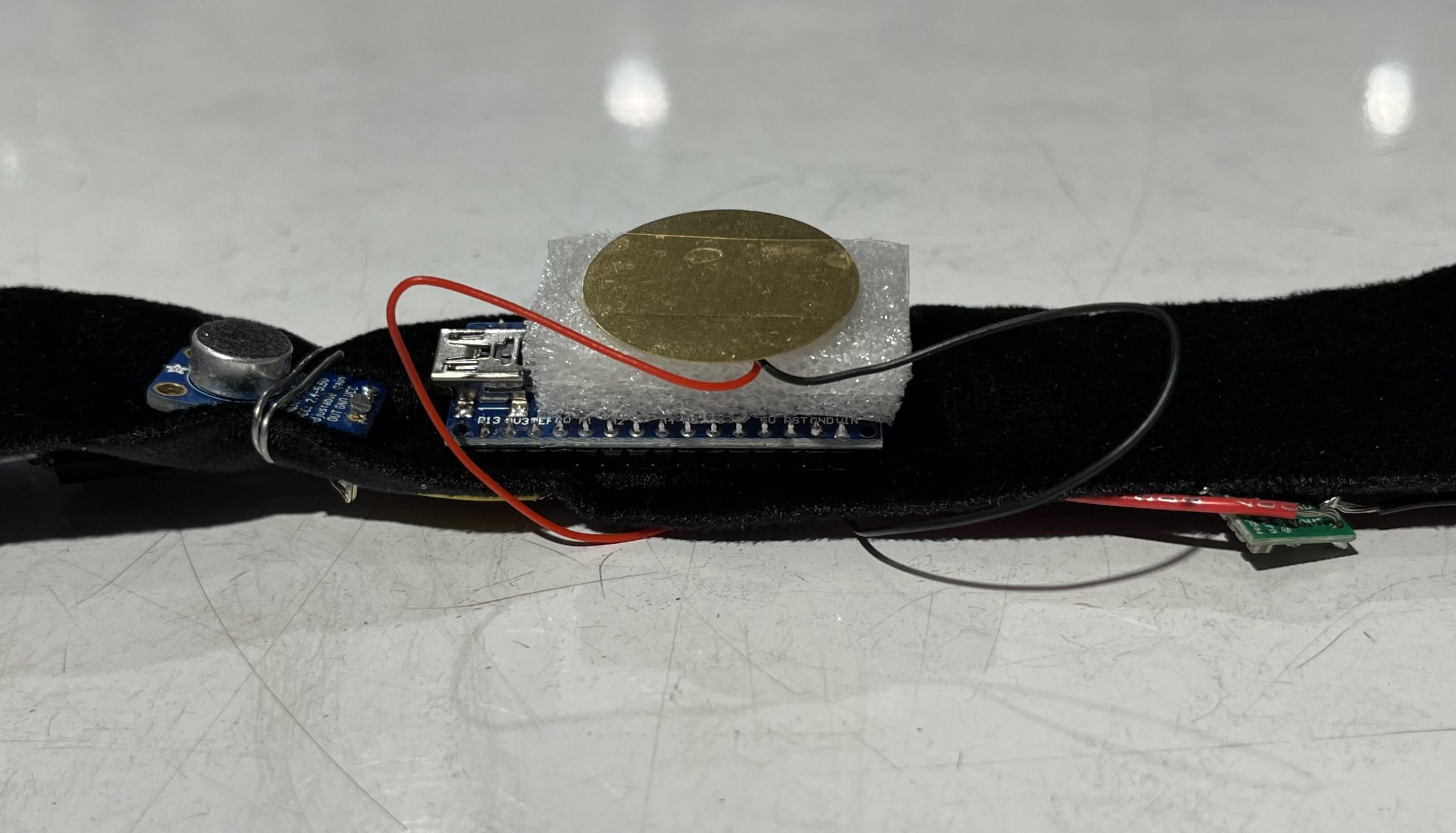
The piezo that detects the vibration on the neck and the microphone that detects and records sound
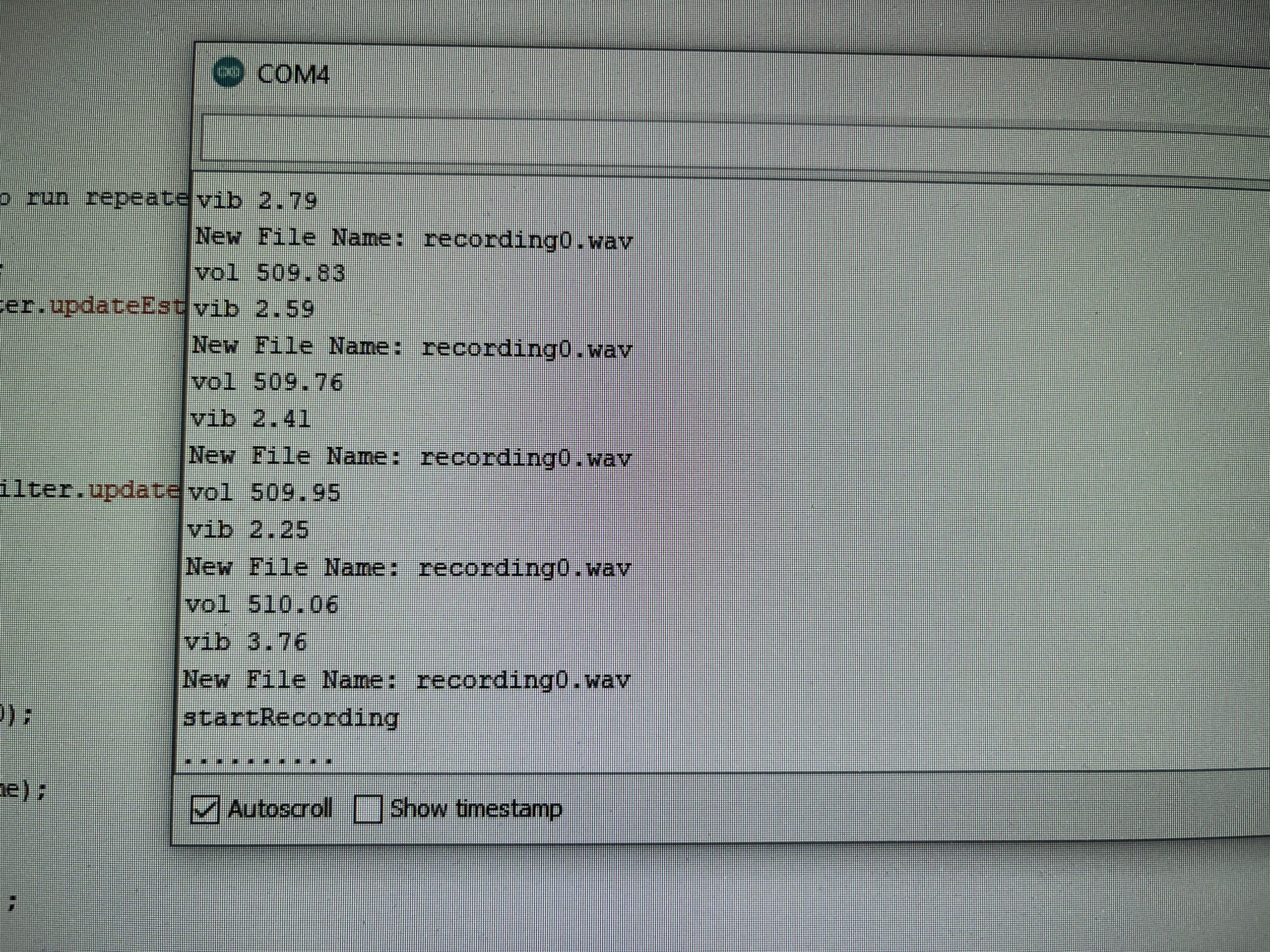
The choker doesn’t begin recording until the sound volume and the level of vibration both reach the thresholds
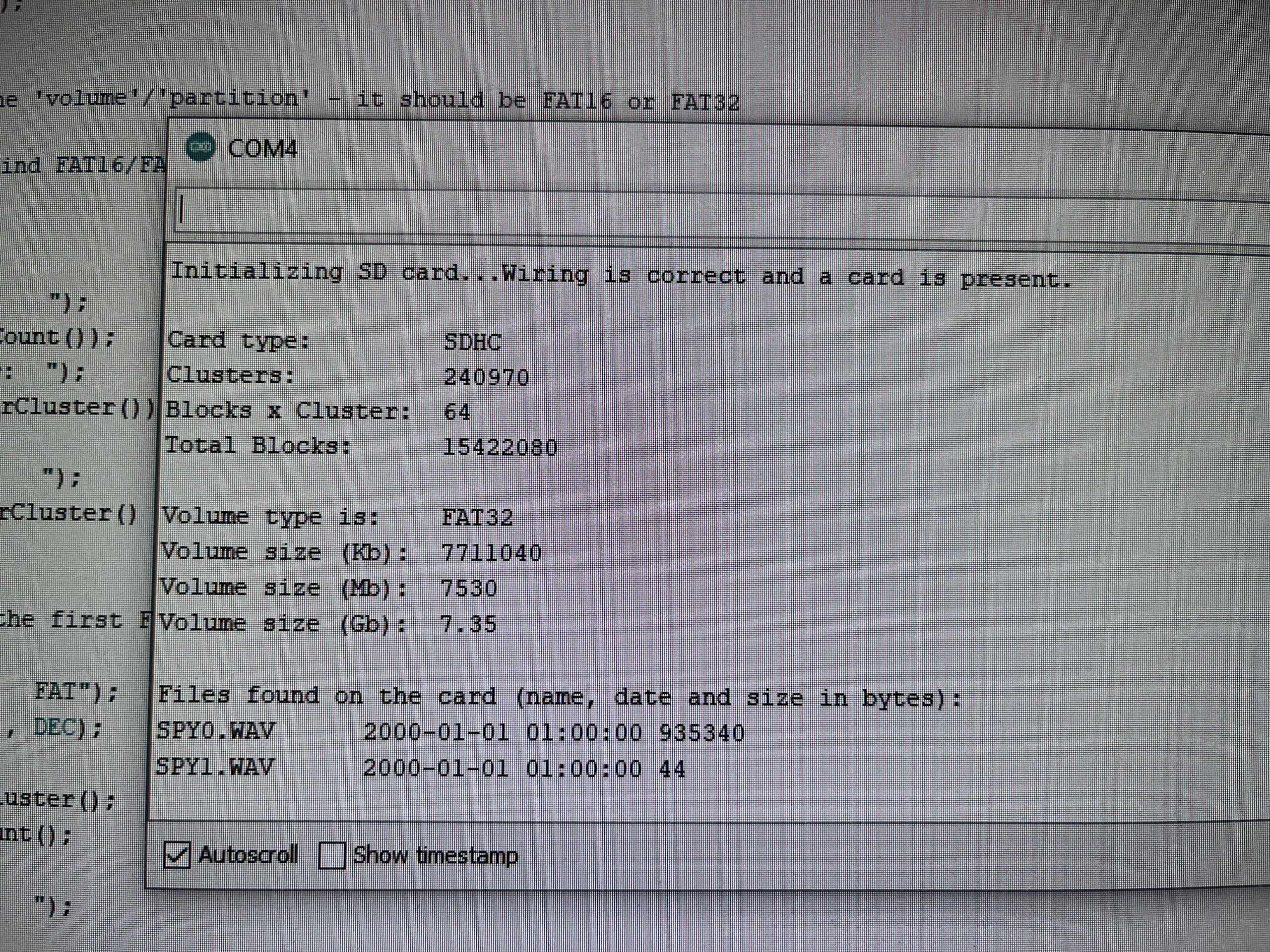
The audios will be saved to the SD card
Process
The first change I recorded is the choice of vibration detector, I was using a vibration sensor with a digital output at first, and it turned out that it cannot detect small vibration on the neck very well, then I changed it into a piezo.
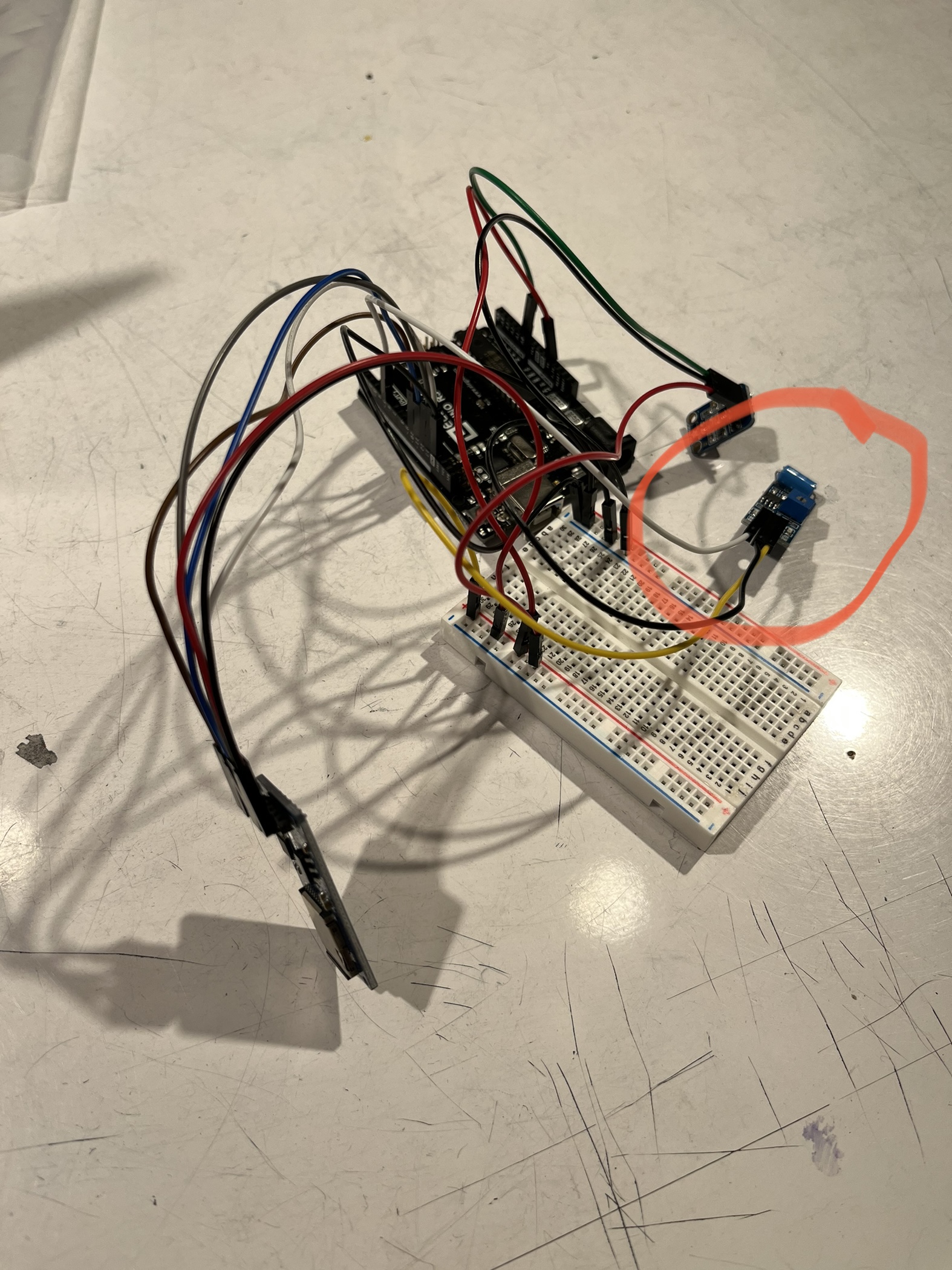
The circled device is the vibration sensor I was originally using
The second change is the overall appearance of the choker, I was thinking about only leaving the Arduino Nano outside, but in the end I flipped it over to make the choker more flexible to wear.
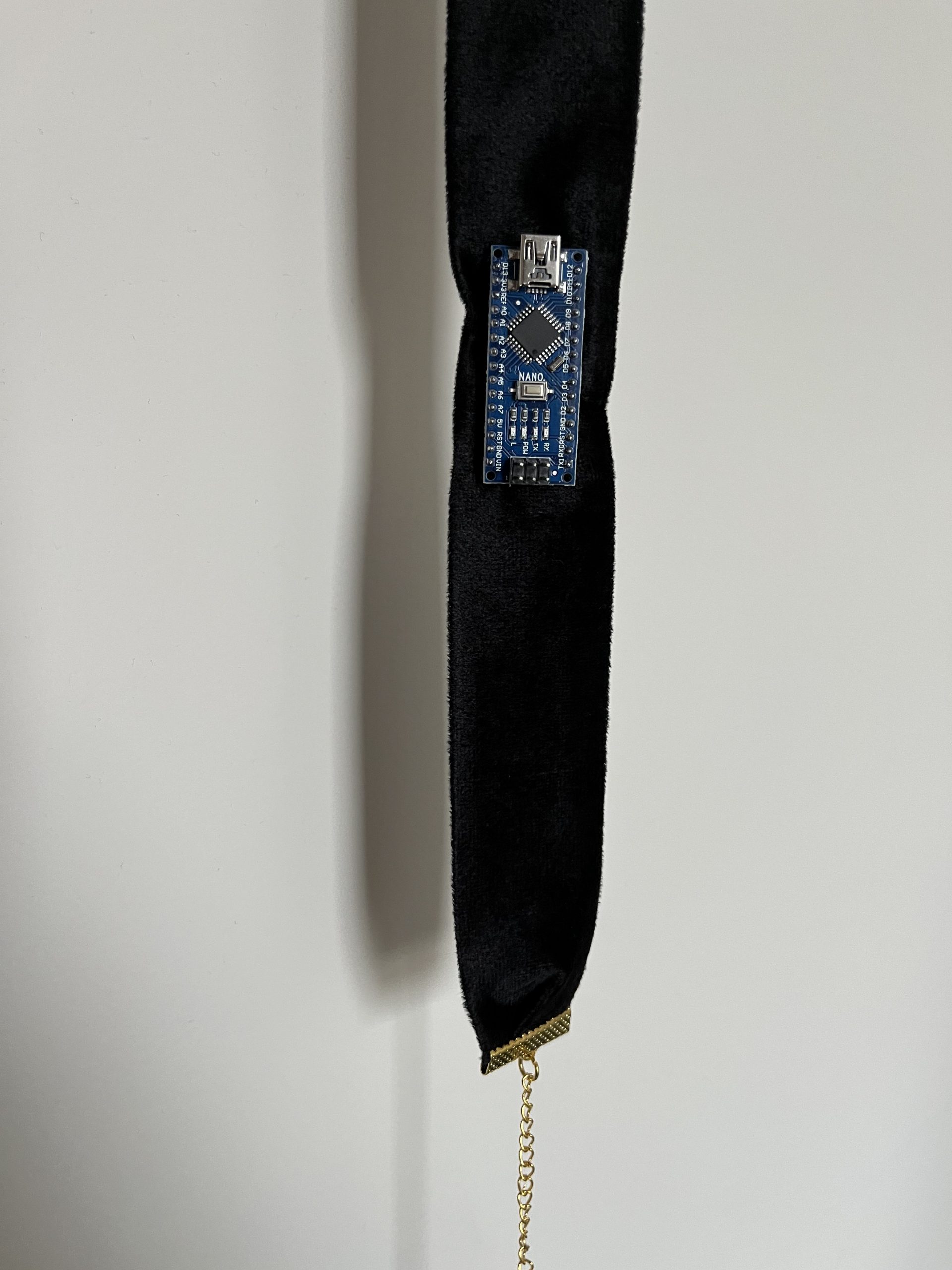
The original design – outside
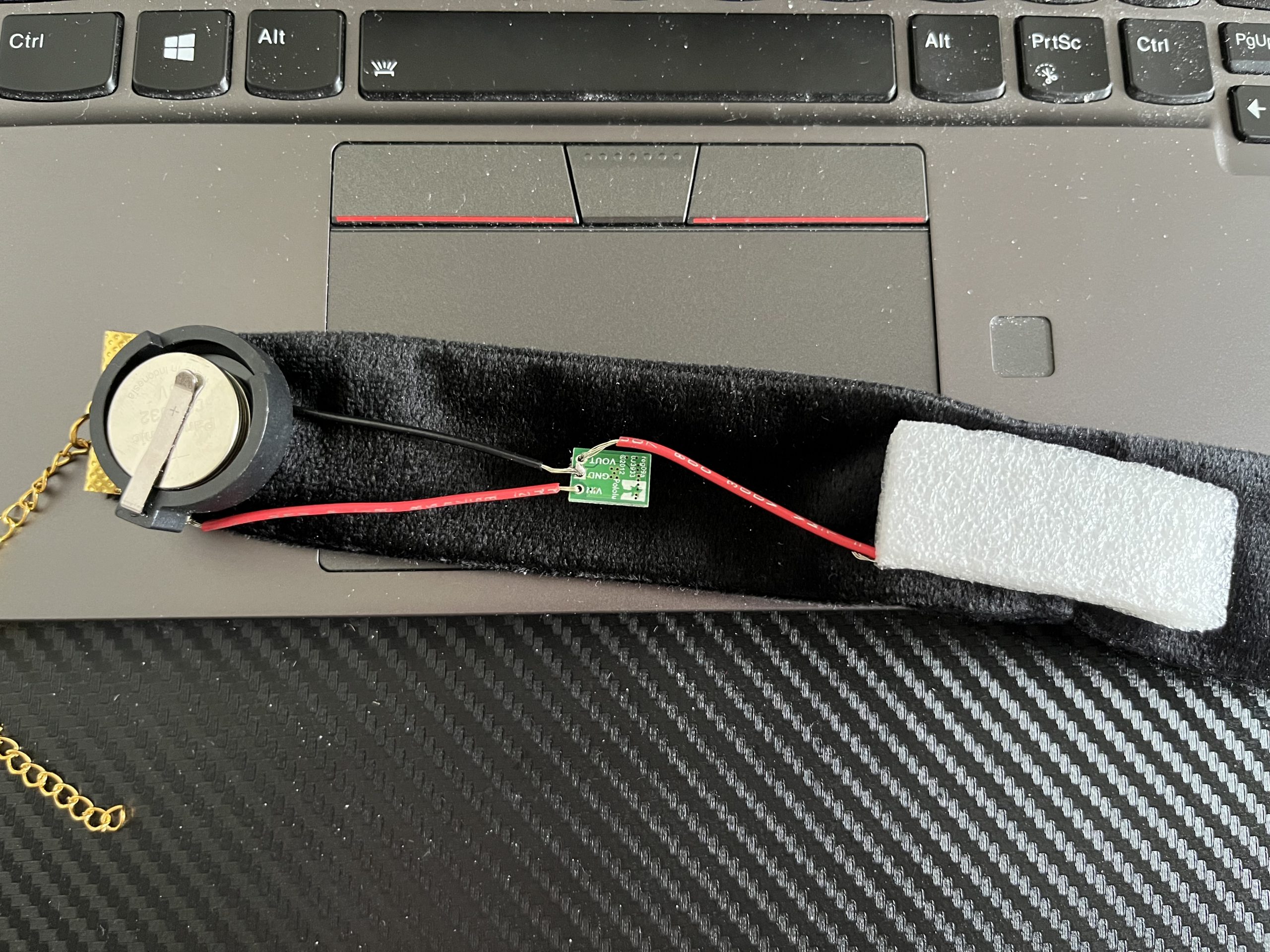
The origin design – inside
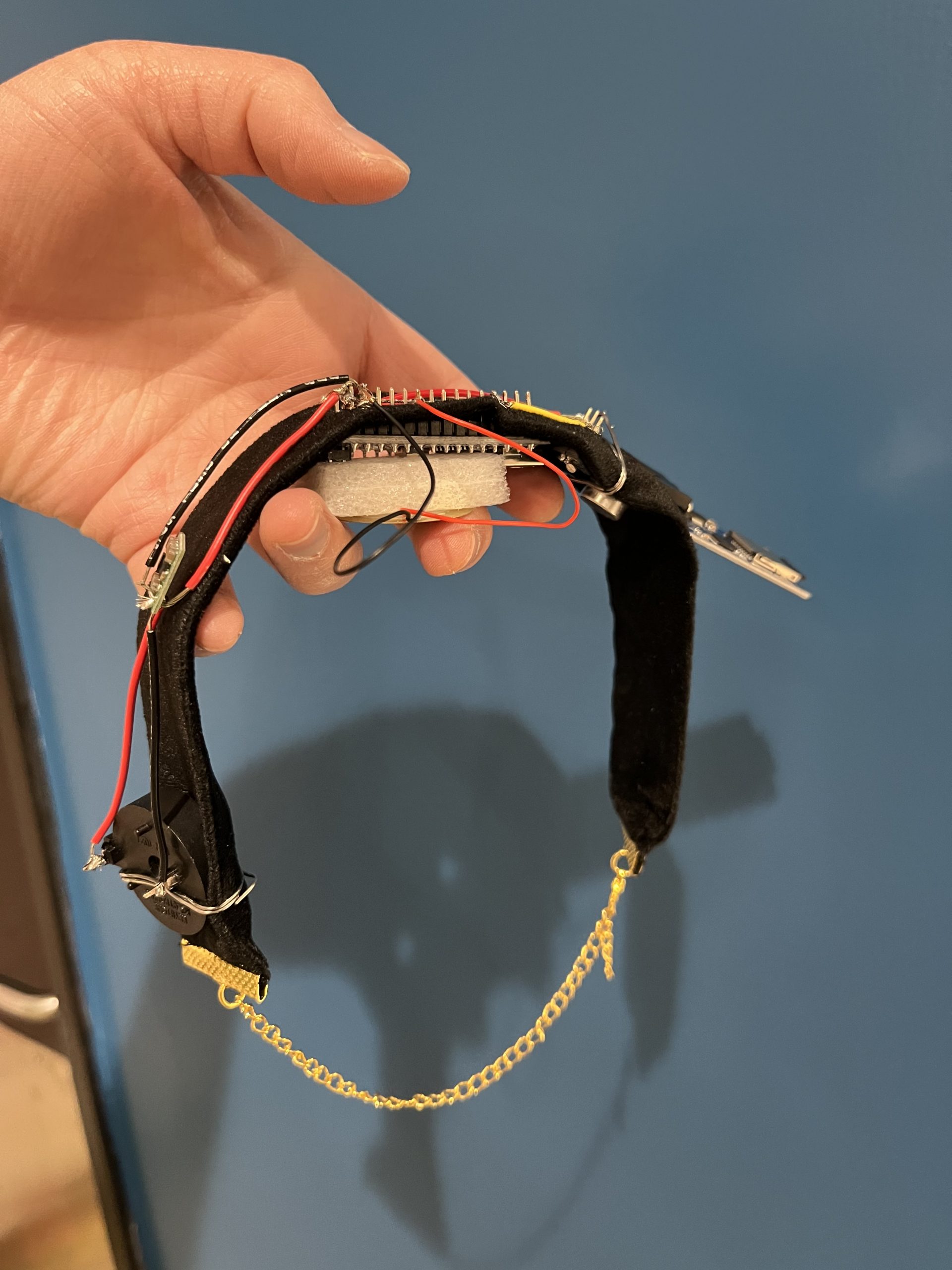
I flipped it over so that it’s really flexible and comfortable to wear
There are also changes in code that I forgot to record, I was using a naïve for loop calculating the average value to reduce noise but it didn’t work very well, later I use a Kalman filter instead.
Discussion
There are several comments regarding the appearance of the choker, some people are saying “the Arduino Nano as a ‘jewel’ is pretty neat looking, very high tech esthetic”, there are also people suggesting “hide the electronic board in the choker” or “hide all the electronics beneath some crystal/stone decor”. There are also comments regarding the user experience, people are saying “Softening the wires against your neck” and “Assembly and build is challenging to make robust and comfortable”. In the end, the choker turns out to be super cyberpunk due to me trying to make it comfortable to wear given limited hardware. Cyberpunk fans may like it while fans of traditional aesthetics may hate it. We don’t have wires in the lab that are soft enough, and the design of the SD card module make the choker super uncomfortable to wear if I attach the module to the choker. In fact, the original design is already a compromise, my idea is actually to make it into a modern silicon choker where the electronic components are all hidden in it, but due to limitations, this is how the final product looks like.
I’m personally not very satisfied about this project, because it doesn’t look very stable and I actually ran into an uploading issue when I finally decided to upload the code to Arduino Nano. There are some issues in the code as well, and I still cannot figure that out. A lot more extra work is needed in order to make it really satisfy me.
What I learned from this project is that it’s hard to deal with unfamiliar hardware and software libraries, my life would be much easier if I don’t try so many weird stuff. My original thought was just beyond the scope of this course, that’s not something I can finish using limited materials in such limited time. Apart from the hardware part, I was originally also thinking about writing code to make the choker actually distinguish between humming and other sound. I wanted to make something really useful that no other things can achieve the same result. My advice to my past self would be: don’t be too ambitious, why not just make a calorie tracker? You can definitely track it on your phone but it’s still a good little thing to have.
If I have chance to build another iteration of this project, I will debug the software first, and also acquire necessary materials to build a safe, stable, and good-looking choker. I will try to use Bluetooth instead of using SD card, and I will write code for distinguishing between music sound and noise.
Block Diagram
Schematic Diagram
Code
/* Music Composer Helper This code enables the choker to record for 3 minutes when the volume and vibration reach their thresholds, and save the audio to the SD card. */ /* Hardware Pinout Connection Arduino Nano SD Pin 5V ------------ VCC GND ----------- GND D10 ----------- CS D11 ----------- MOSI D12 ----------- MISO D13 ----------- SCK ________________________________________ Arduino Nano Microphone 5v ------------- VCC GND ------------ GND A0 ------------- Out ________________________________________ Arduino Nano Piezo GND ---------- negative A5 ----------- positive */ /* credit https://circuitdigest.com/microcontroller-projects/simple-arduino-voice- recorder-for-spy-bug-voice-recording */ #include <SimpleKalmanFilter.h> #include <TMRpcm.h>//the library for recording #include <SD.h> #include <SPI.h> #define SD_CSPin 10 #define vib_pin A5 #define mic_pin A0; const int sample_rate = 16000; int file_number = 0; int vib = 0; int vol = 0; unsigned int sample; float estimated_vol = 0; float estimated_vib = 0; char filePrefixname[50] = "rec"; char exten[10] = ".wav"; TMRpcm audio; /* SimpleKalmanFilter(e_mea, e_est, q); e_mea: Measurement Uncertainty e_est: Estimation Uncertainty q: Process Noise */ SimpleKalmanFilter volumeKalmanFilter(1, 1, 0.01); SimpleKalmanFilter vibrationKalmanFilter(1, 1, 0.01); // delay function for serial log. void wait_min(int mins) { int count = 0; int secs = mins * 60; while (1) { Serial.print('.'); delay(1000); count++; if (count == secs) { count = 0; break; } } Serial.println(); return ; } void setup() { Serial.begin(9600); //Sets up the pins pinMode(mic_pin, INPUT); pinMode(vib_pin, INPUT); //Checking SD card Serial.println("loading... SD card"); if (!SD.begin(SD_CSPin)) { Serial.println("An Error has occurred while mounting SD"); } while (!SD.begin(SD_CSPin)) { Serial.print("."); delay(500); } audio.CSPin = SD_CSPin; } void loop() { //print volume vol = analogRead(mic_pin); estimated_vol = volumeKalmanFilter.updateEstimate(vol); Serial.print("vol "); Serial.println(estimated_vol); //print vibration vib = analogRead(vib_pin); estimated_vib = vibrationKalmanFilter.updateEstimate(vib); Serial.print("vib "); Serial.println(estimated_vib); //print file name char fileSlNum[20] = ""; itoa(file_number, fileSlNum, 10); char file_name[50] = ""; strcat(file_name, filePrefixname); strcat(file_name, fileSlNum); strcat(file_name, exten); Serial.print("New File Name: "); Serial.println(file_name); //record if volume and vibration hit thresholds if (estimated_vol > 510 && estimated_vib > 1) { audio.startRecording(file_name, sample_rate, mic_pin); vol=0; vib=0; estimated_vol = 0; estimated_vib = 0; Serial.println("startRecording "); // record audio for 3mins wait_min(3); audio.stopRecording(file_name); Serial.println("stopRecording"); file_number++; } //clear values to avoid bugs vol=0; vib=0; estimated_vol = 0; estimated_vib = 0; }