Description
There is a small white box with a black switch on a white pillar. The small white box is connected to a black structure with 5 black and gold vases each containing water. The white box glows blue until the user flips the switch. Once the switch is flipped, the white box will flash green and give the user some time to position their hands. The user holds their hand over the white box and waits for the color scan. The waterfall will start running and lighter skin tones will cause the waterfall to run longer. When the water stops flowing, the box will glow blue again.
Project Reflection
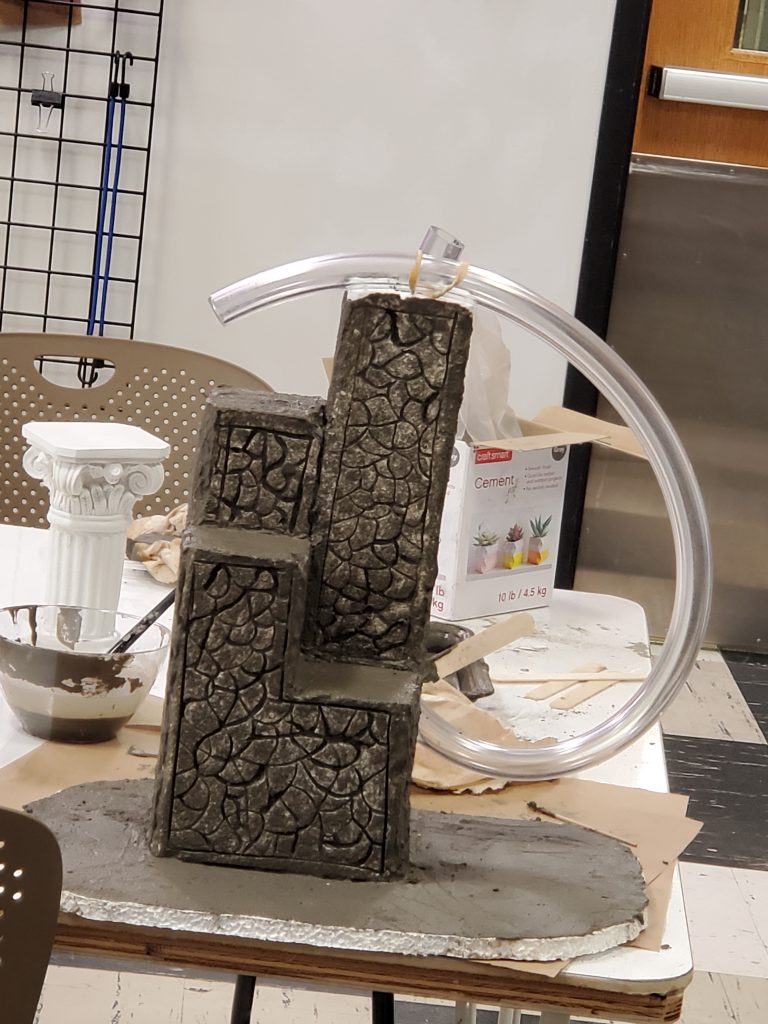
The waterfall base is coated in the first layer of concrete (works better than the original vases!!)
Success In Their Eyes is a project that challenges us to think about how colorism impacts our society and many others throughout the world. The idea developed from the past Jim Crow laws, segregated water fountains, as well as the use of skin lightening cream in several cultures. Throughout the creation, I faced several challenges, not only in the physical design but also in the way I wanted it to be perceived. I completed the technological part very early in the process, though calculating the lightness of color was a struggle. The examples from the Adafruit TCS library helped a lot and with some tweaking of the gain, it was functional! I was able to present the pump and scanner for our first checkpoint.
The biggest hurdle was creating the physical waterfall. I hadn’t given much thought to how the waterfall should look which was a mistake in the process. I finally settled on a Youtube video as my tutorial. I also watched other videos that used concrete to construct the vases, and I was inspired to try. Unfortunately, the process was problematic; the concrete mix was the wrong type and consistency which caused the vases not to dry. The backup plan was to use plastic mason jars and restructure them to the style I wanted. Next time I attempt working with concrete, I wouldn’t rush the research so I end up with something useable. The base was made out of styrofoam and creating it was fairly simple. The complication here was the tube. It wasn’t flexible enough to curve and fit in the base but a simple replacement solved that problem.
The pump and vases all were water tested and worked well individually. After putting them all together for the final product and adding water, I found out there was a major leak in the first and last vase. This was extremely disappointing since I spent so long creating the waterfall piece by piece. In the future, I would like to find a way to patch the problem areas, but recreating the whole waterfall might be easier.
The last struggle was thinking of how users would experience this project. Originally, I wanted the users (most likely in a group) to play around with it and discuss why some people saw the waterfall flow longer. They would come to the realization that one of the visible differences in the group was the color of their skin, and the role it plays in how people are viewed. Additionally, I did not solve how to get users to present their hands without the color scanner being obvious. Given additional time, I would test the completed version with others and take into account the thoughts they have. While it would have been ideal to finish without issues, I loved every moment of fabricating this project and seeing it come to life.
Code
// Judenique Auguste // Success in Their Eyes // Creates an instances of the Adafruit Neopixels and Adafruit Color Sensor // The Neopixels are used to give the status of the waterfall through color // The Color Sensor is used to caluculate the brightness of the user's skin // A digital signal is sent to the pump which turns it on and off for a period of time // Use of Neopixel library example code and Color Sensor library example code #include <Wire.h> #include "Adafruit_TCS34725.h" #include <Adafruit_NeoPixel.h> #define pump 4 #define button 7 #define PIN 6 #define NUMPIXELS 8 #define BRIGHTNESS 50 int pumpState = LOW; int buttonState; Adafruit_NeoPixel pixels(NUMPIXELS, PIN, NEO_GRB + NEO_KHZ800); Adafruit_TCS34725 tcs = Adafruit_TCS34725(TCS34725_INTEGRATIONTIME_614MS , TCS34725_GAIN_16X); void setup() { Serial.begin(9600); pinMode(pump, OUTPUT); pinMode(button, INPUT_PULLUP); pixels.begin(); buttonState = digitalRead(button); if (tcs.begin()) { //Serial.println("Found sensor"); tcs.setInterrupt(true); pixels.begin(); pixels.setBrightness(BRIGHTNESS); buttonState = digitalRead(button); } else { Serial.println("No TCS34725 found ... check your connections"); while (1); } } void loop() { // fountain is idle for (int i = 0; i < NUMPIXELS; i++) { pixels.setPixelColor(i, pixels.Color(0, 0, 255)); pixels.show(); } float red, green, blue; if (buttonState != digitalRead(button)) { buttonState = digitalRead(button); pixels.clear(); // the wait period til scan to position int position_time = 0; while (position_time < 3) { for (int i = 0; i < NUMPIXELS; i++) { pixels.setPixelColor(i, pixels.Color(0, 255, 0)); pixels.show(); } delay(1000); for (int i = 0; i < NUMPIXELS; i++){ pixels.setPixelColor(i, pixels.Color(0, 0, 0)); pixels.show(); } delay(1000); position_time++; } tcs.setInterrupt(false); delay(200); tcs.getRGB(&red, &green, &blue); int colorTemp = tcs.calculateColorTemperature(red, green, blue); int lux = tcs.calculateLux(red, green, blue); tcs.setInterrupt(true); int lightness = 765 - (int(red) + int(green) + int(blue)); int brightness = map(lightness, 650, 0, 0, 255); int seconds_on = map(brightness, 0, 255, 6000, 25000); int new_time = seconds_on + 5000; Serial.print("bright:\t"); Serial.print(brightness); Serial.print("\tR:\t"); Serial.print(int(red)); Serial.print("\tG:\t"); Serial.print(int(green)); Serial.print("\tB:\t"); Serial.print(int(blue)); Serial.print("\n"); Serial.print("length of time:\t"); Serial.print(new_time); Serial.print("\n"); Serial.print("length of time2:\t"); Serial.print(seconds_on); Serial.println("\n"); pumpState = HIGH; digitalWrite(pump, pumpState); //length of time the pump will be on while((seconds_on*seconds_on) > 0) { rainbow(20); seconds_on -= 5000; } pumpState = LOW; digitalWrite(pump, pumpState); delay(10); } } void rainbow(uint8_t wait) { uint16_t i, j; for (j = 0; j < 256; j++) { for (i = 0; i < pixels.numPixels(); i ++) { pixels.setPixelColor(i, Wheel((i + j) & 255)); } pixels.show(); delay(wait); } } // Input a value 0 to 255 to get a color value. // The colours are a transition r - g - b - back to r. uint32_t Wheel(byte WheelPos) { WheelPos = 255 - WheelPos; if (WheelPos < 85) { return pixels.Color(255 - WheelPos * 3, 0, WheelPos * 3); } if (WheelPos < 170) { WheelPos -= 85; return pixels.Color(0, WheelPos * 3, 255 - WheelPos * 3); } WheelPos -= 170; return pixels.Color(WheelPos * 3, 255 - WheelPos * 3, 0); }
Online Resources
MakerCase – Easy Laser Cut Case Design
Overview | Adafruit Color Sensors | Adafruit Learning System