Final Project Pictures and Video
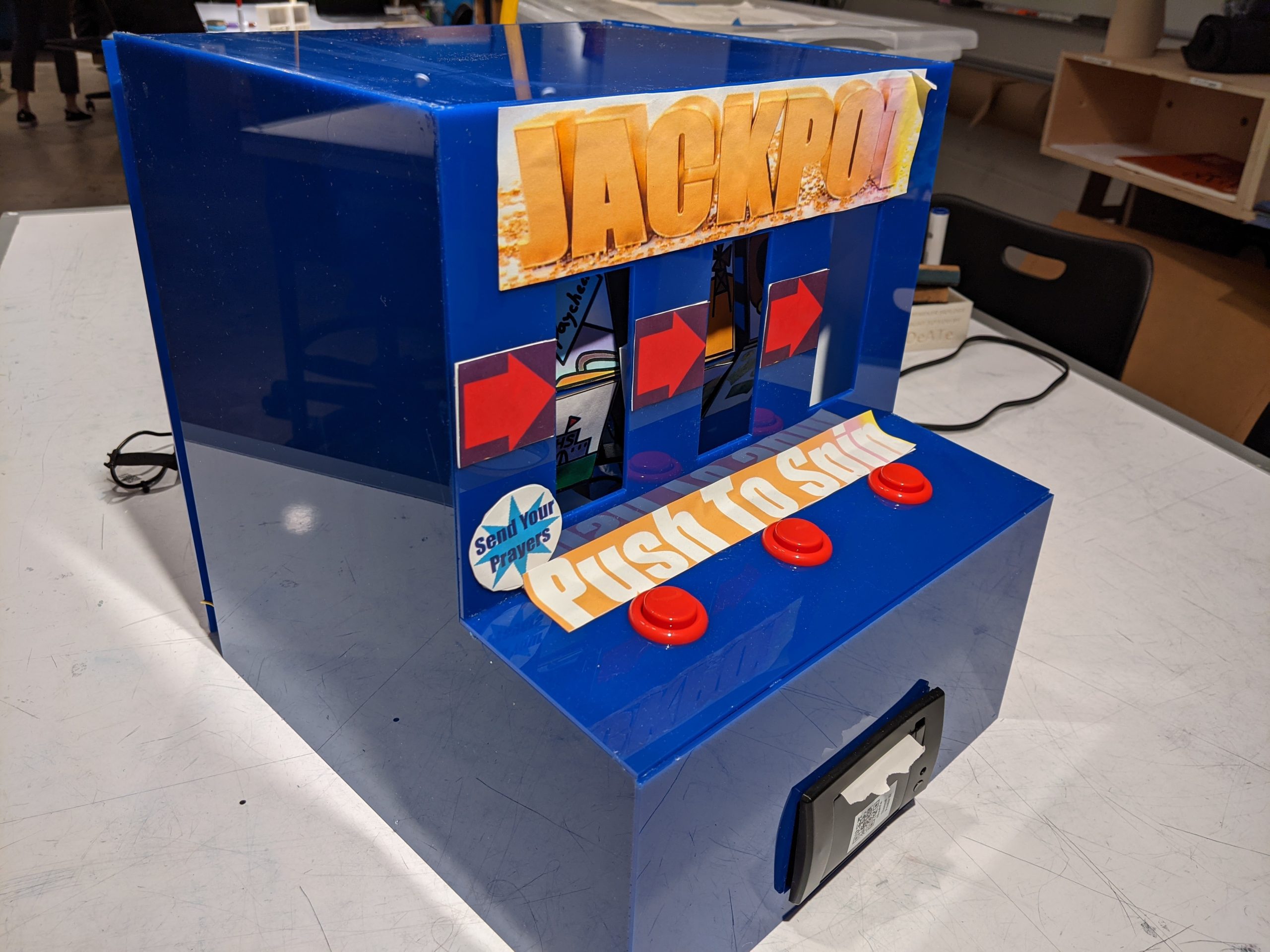
Side image of the slot machine
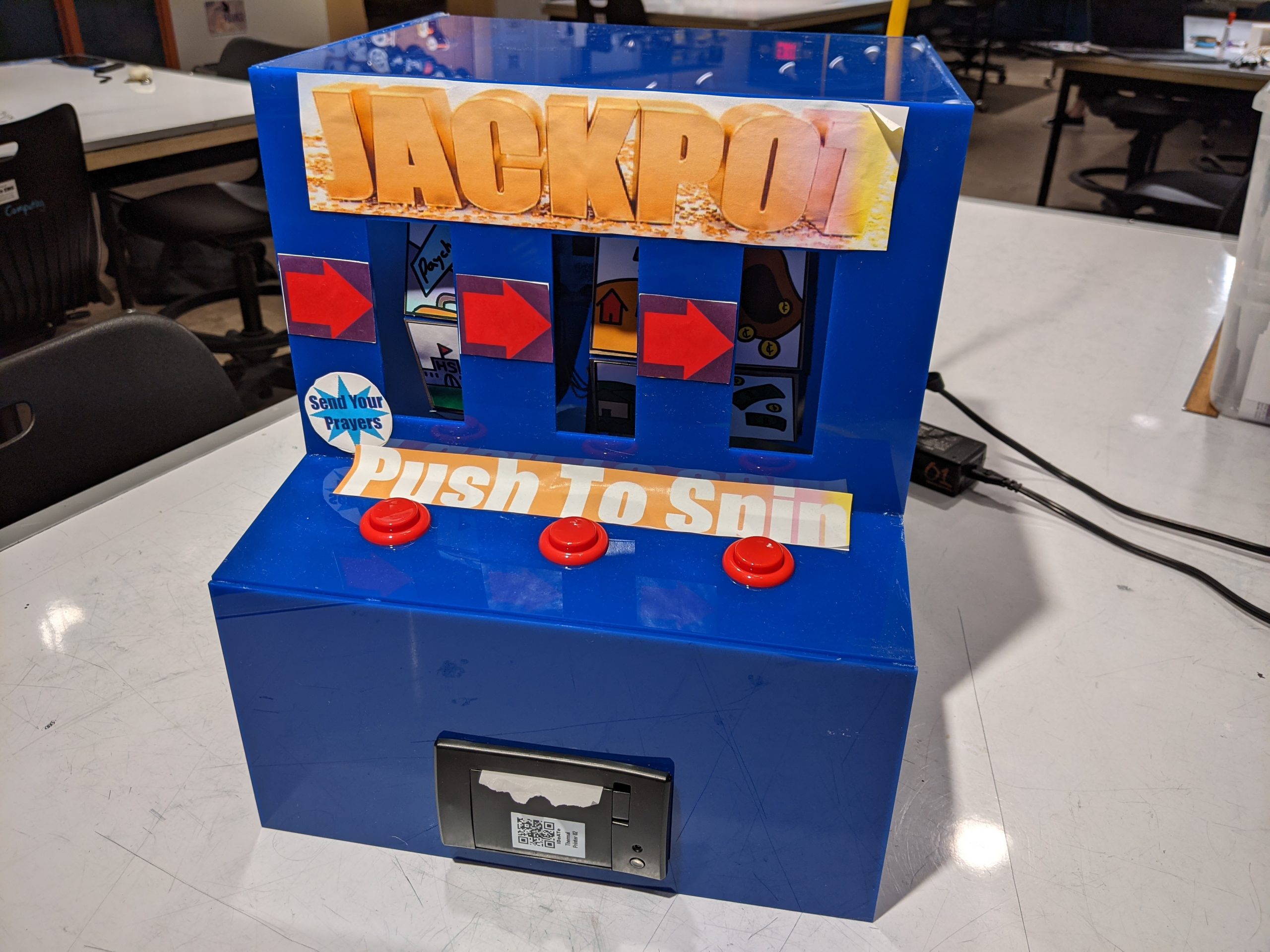
Front image of the slot machine
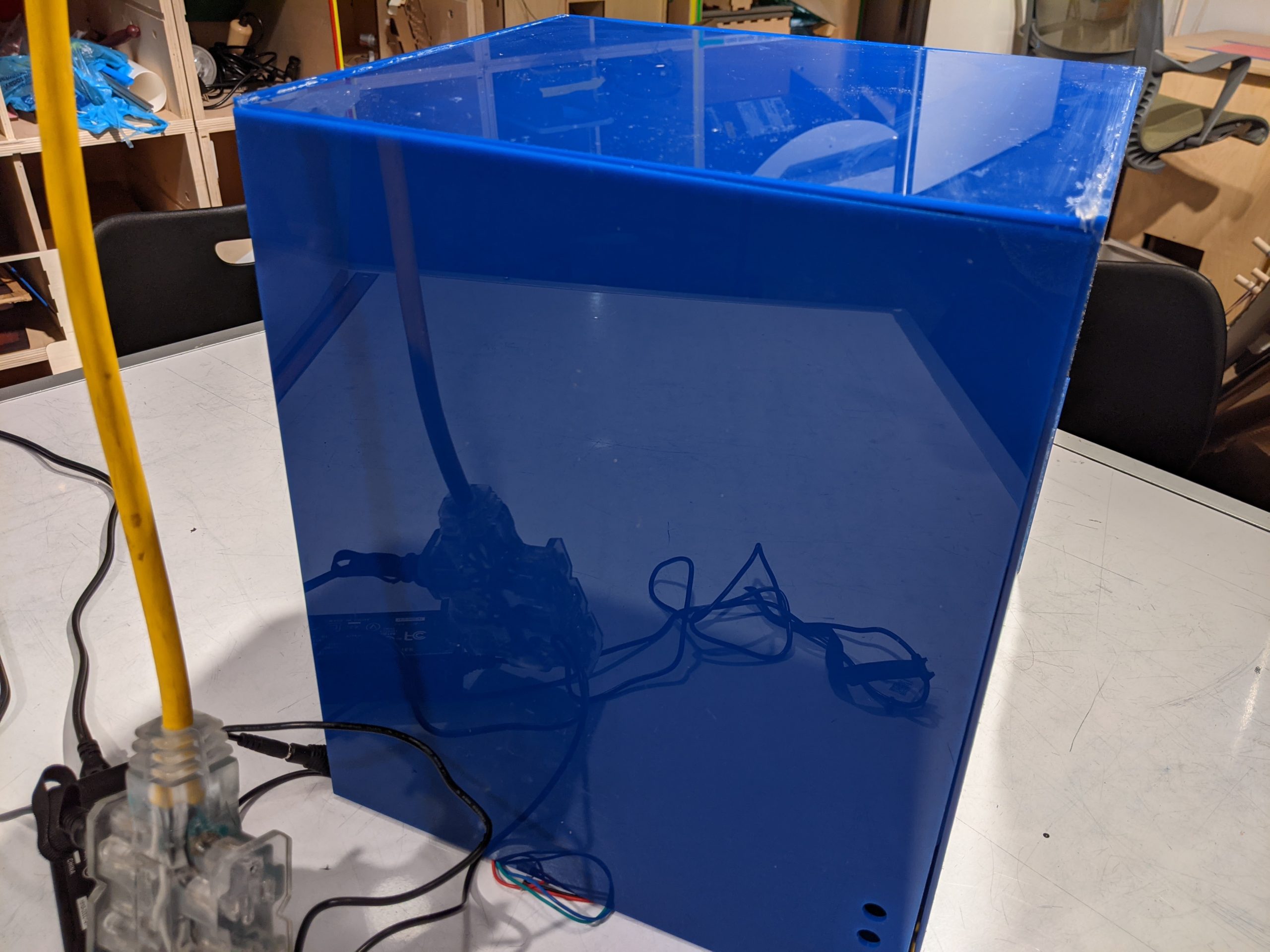
Back of it
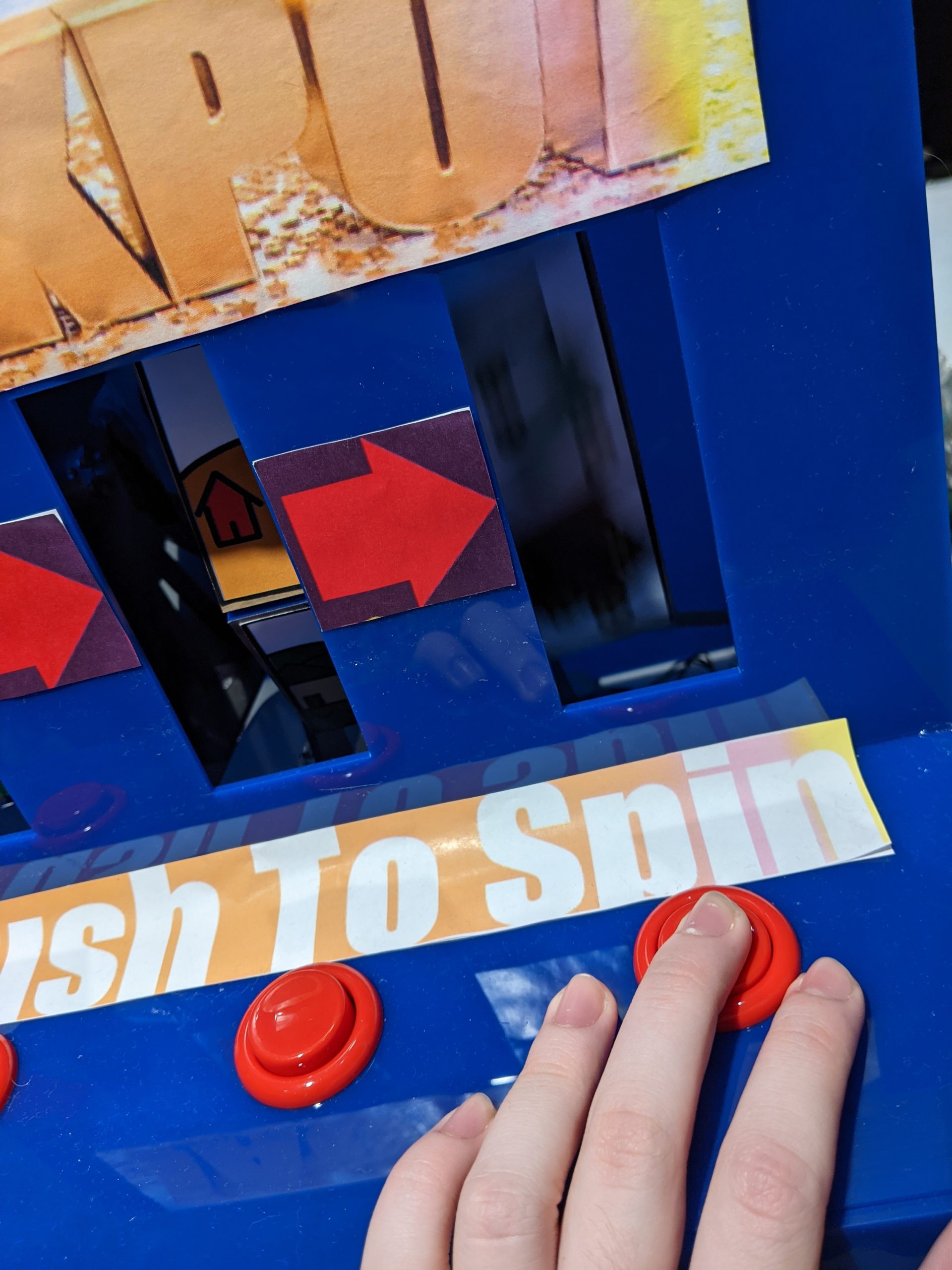
Holding down the button to spin the wheel
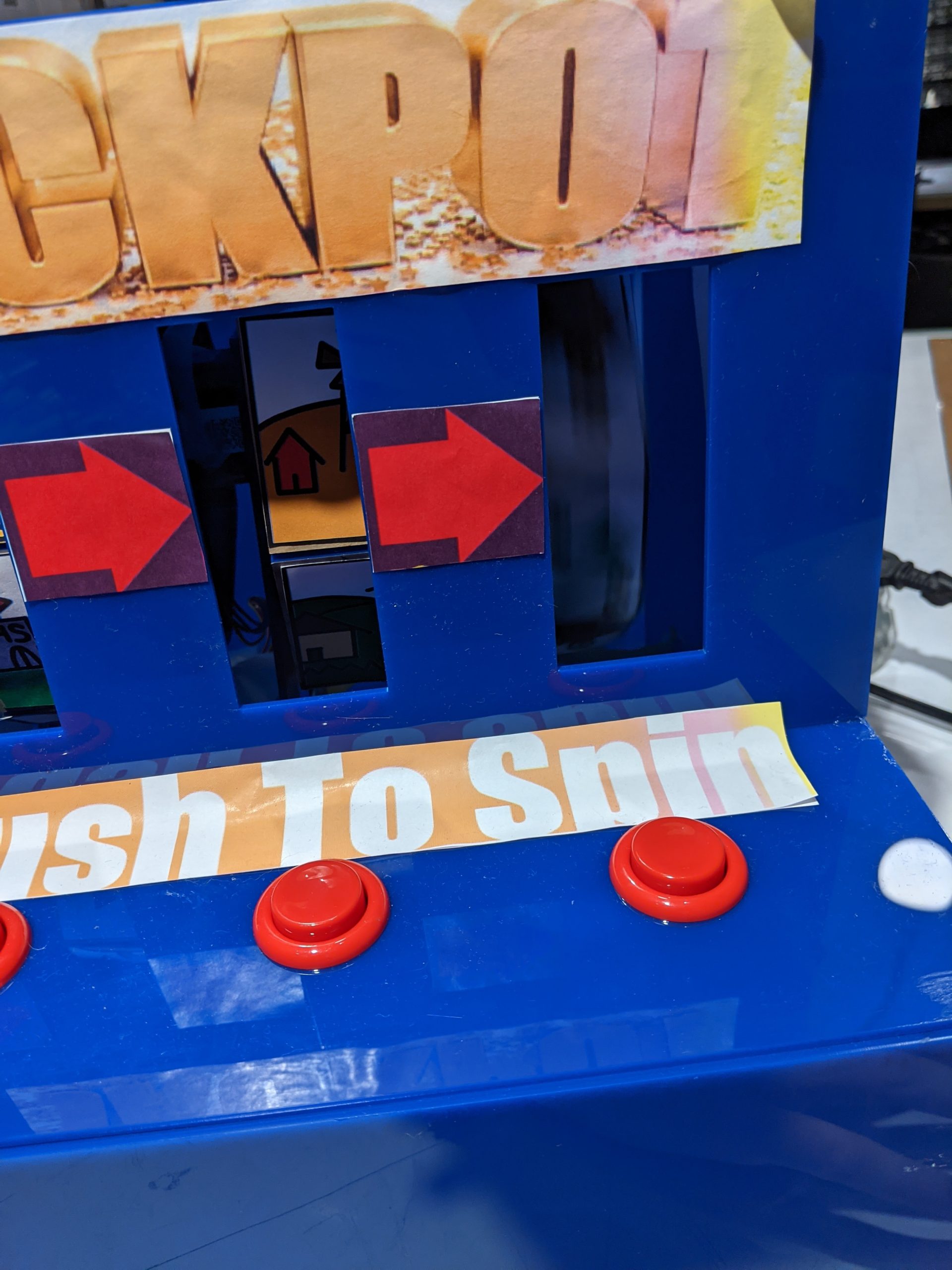
The wheel landing on it’s final position
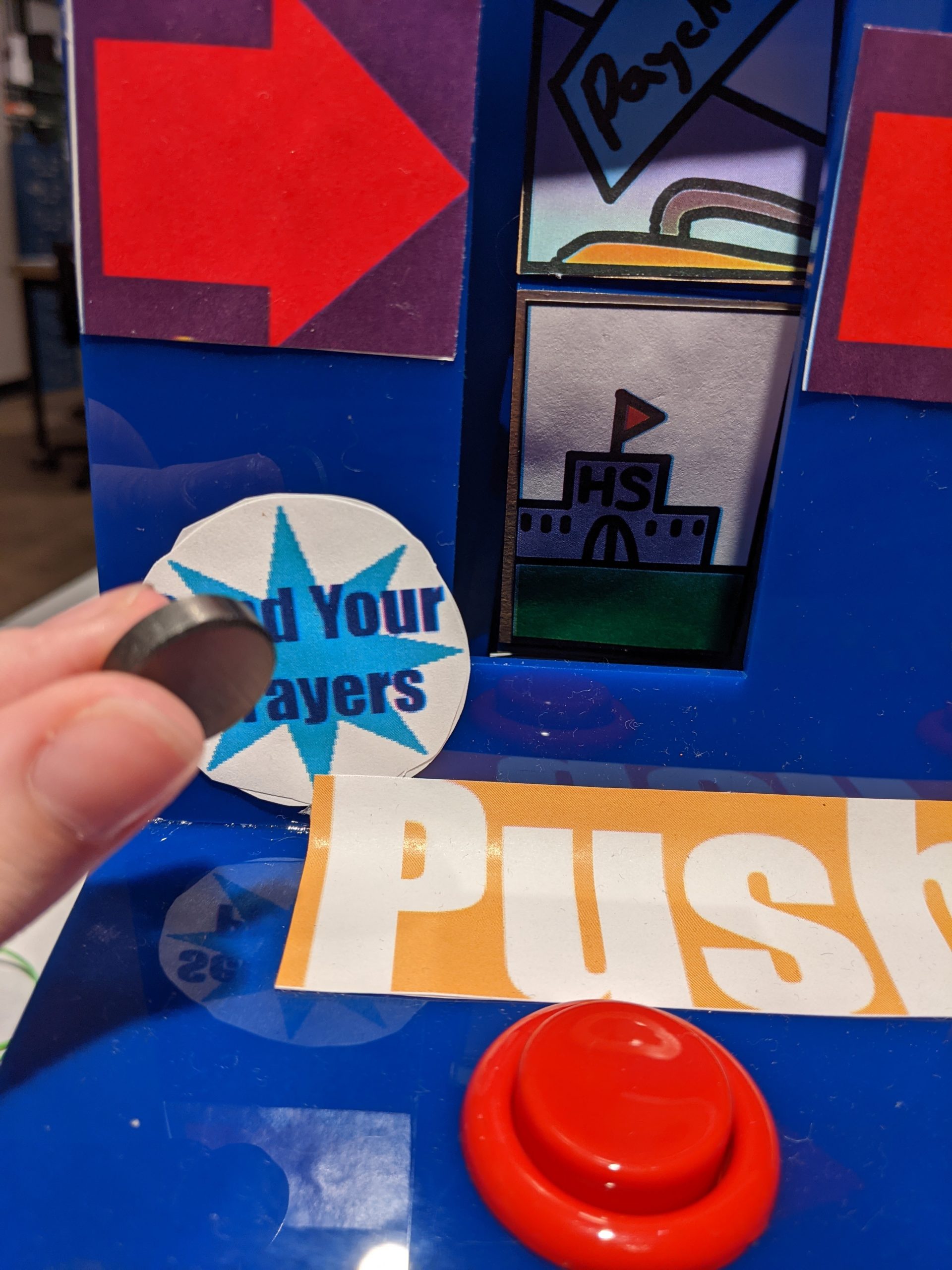
Waving the magnet around to change where the wheel lands
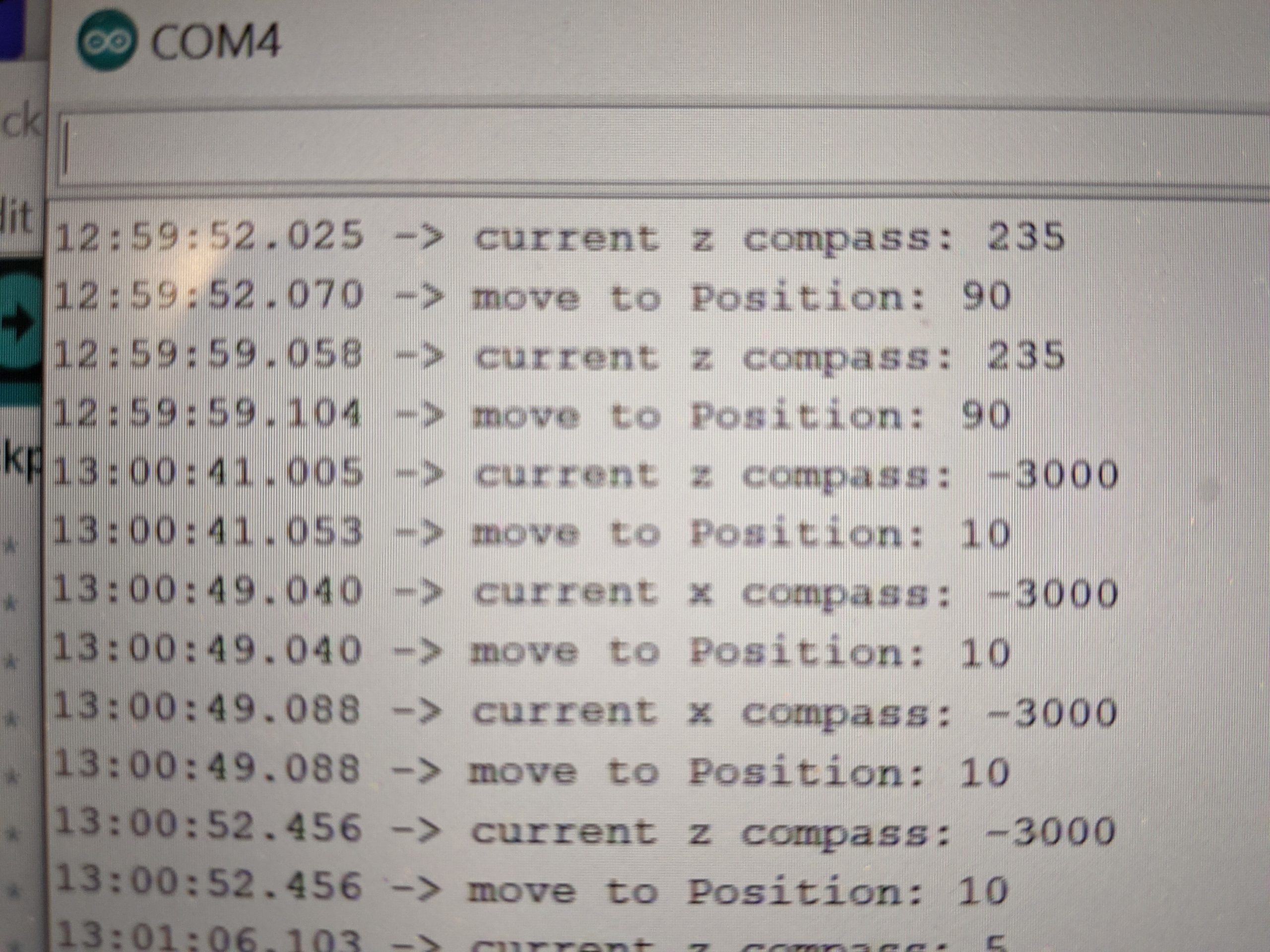
Computer output based off of the received input values
Narrative Description
The Jackpot of Life is a slot machine, but the user can rig it in their favor. A disproportionate amount of one’s life is determined by the circumstances of their birth, which is the idea behind my project. Each wheel correlates to some aspect of a person’s life, their family’s financial situation, where they are born, and their education level. The user presses a button and the wheel spins to land on some result. As I mentioned, though, it can be rigged. While holding down the button, one can “send their prayers,” which translates to waving a magnet in front of a sensor that measures magnetic fields. Based off of the value read from the magnet, the wheel spins to a specific position.
Progress Images
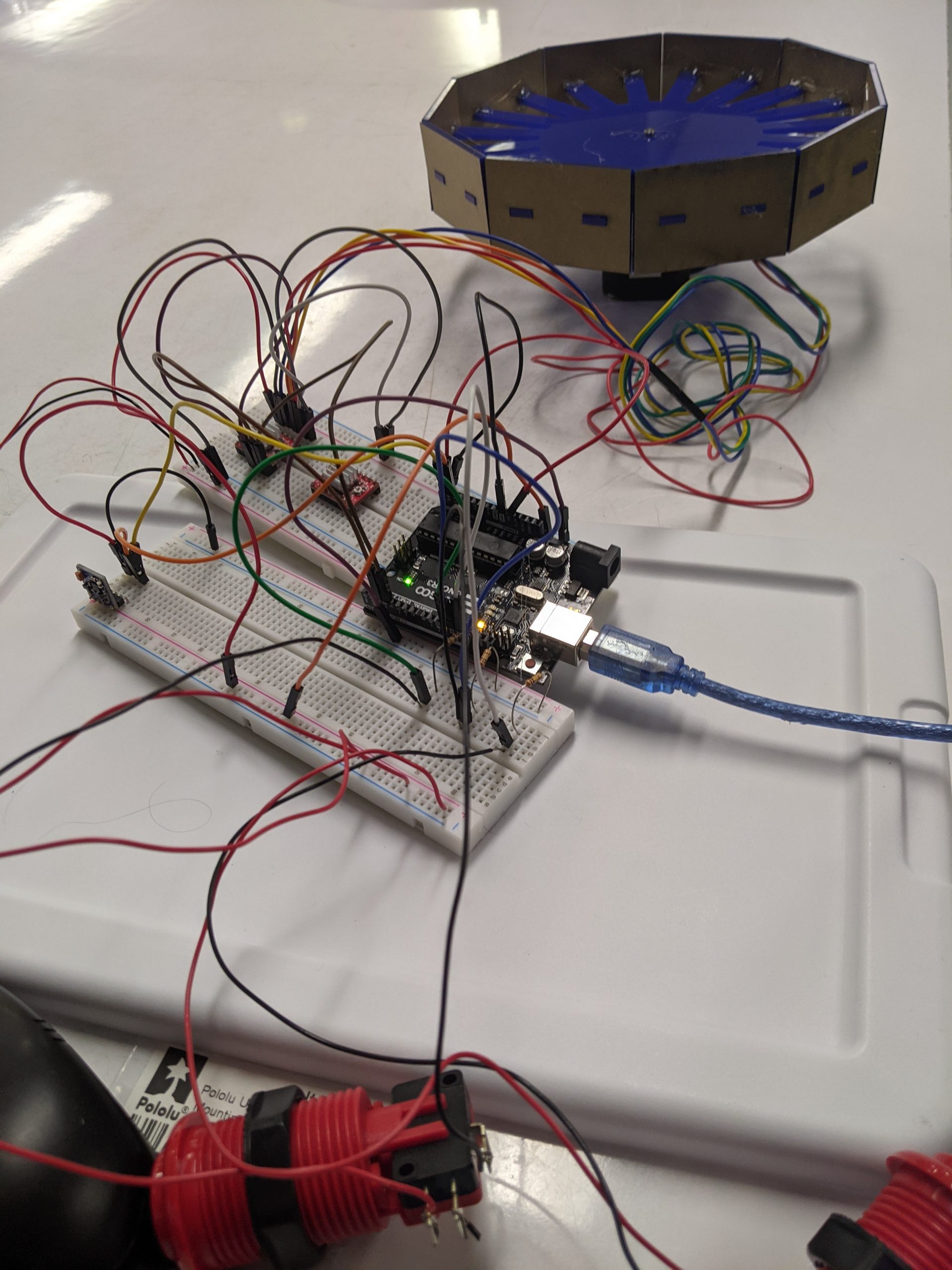
Wired and programmed the wheel to spin
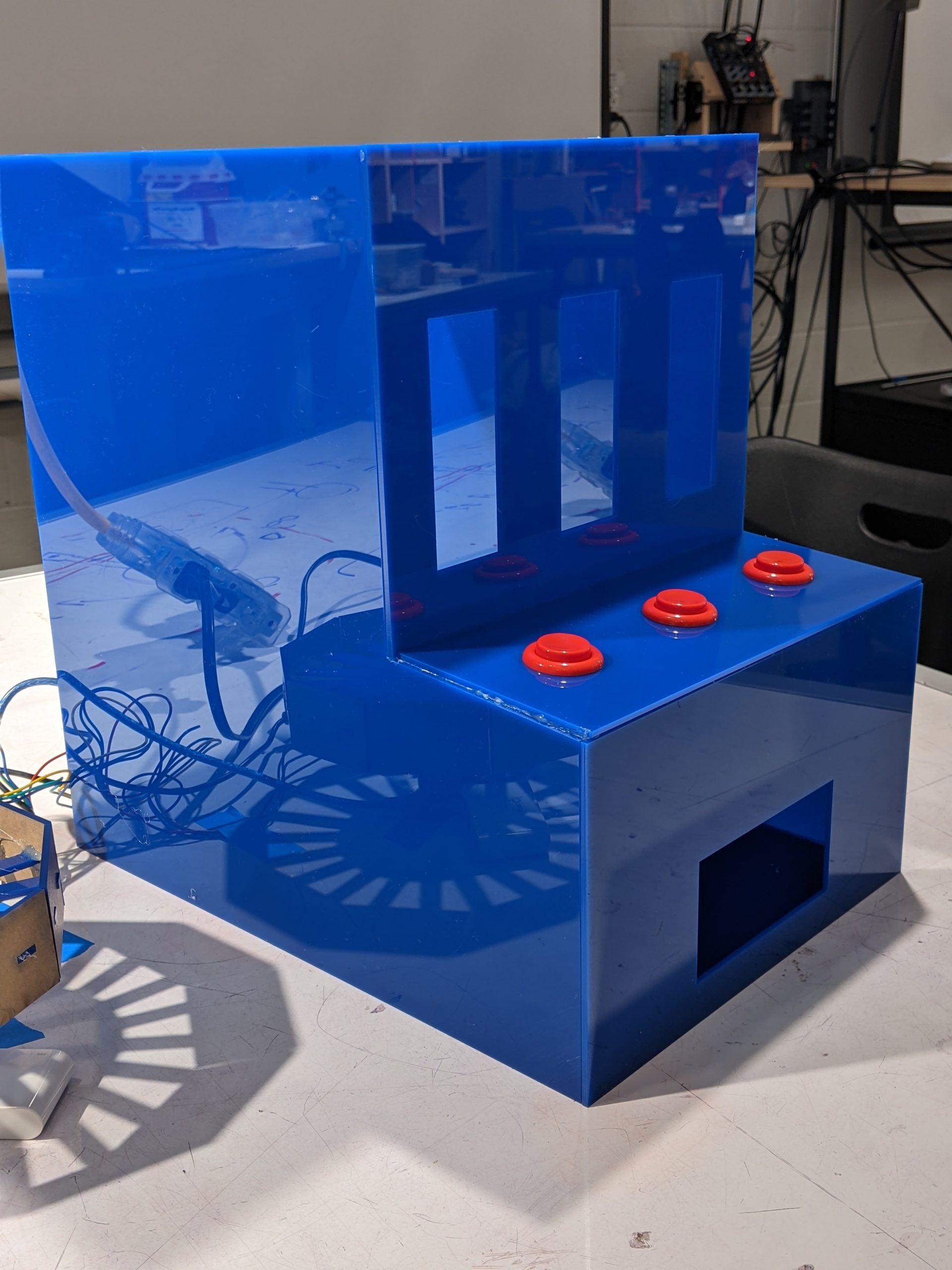
First prototype. Looks good.
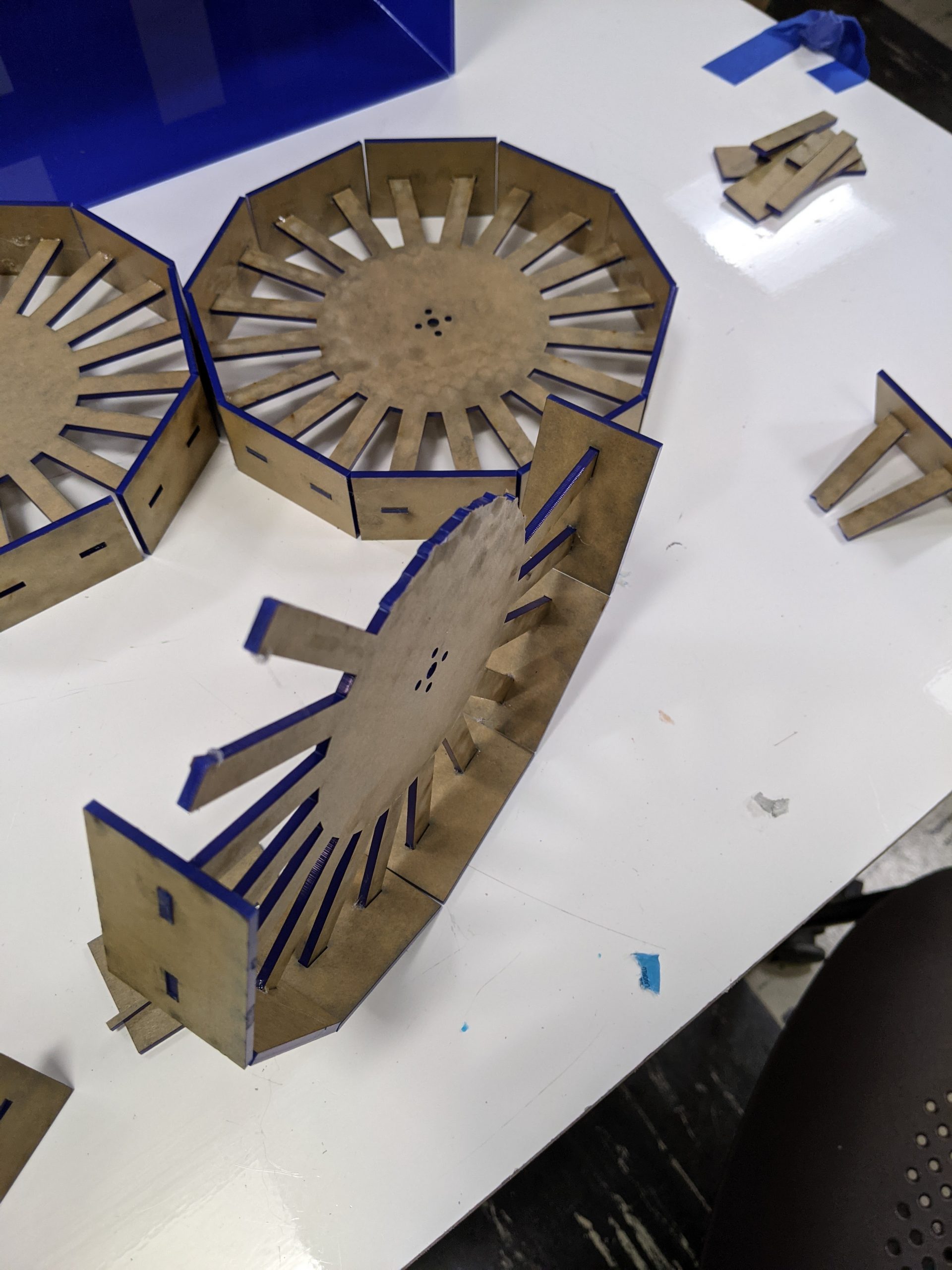
The day before my project is due, I drop my wheel and it breaks
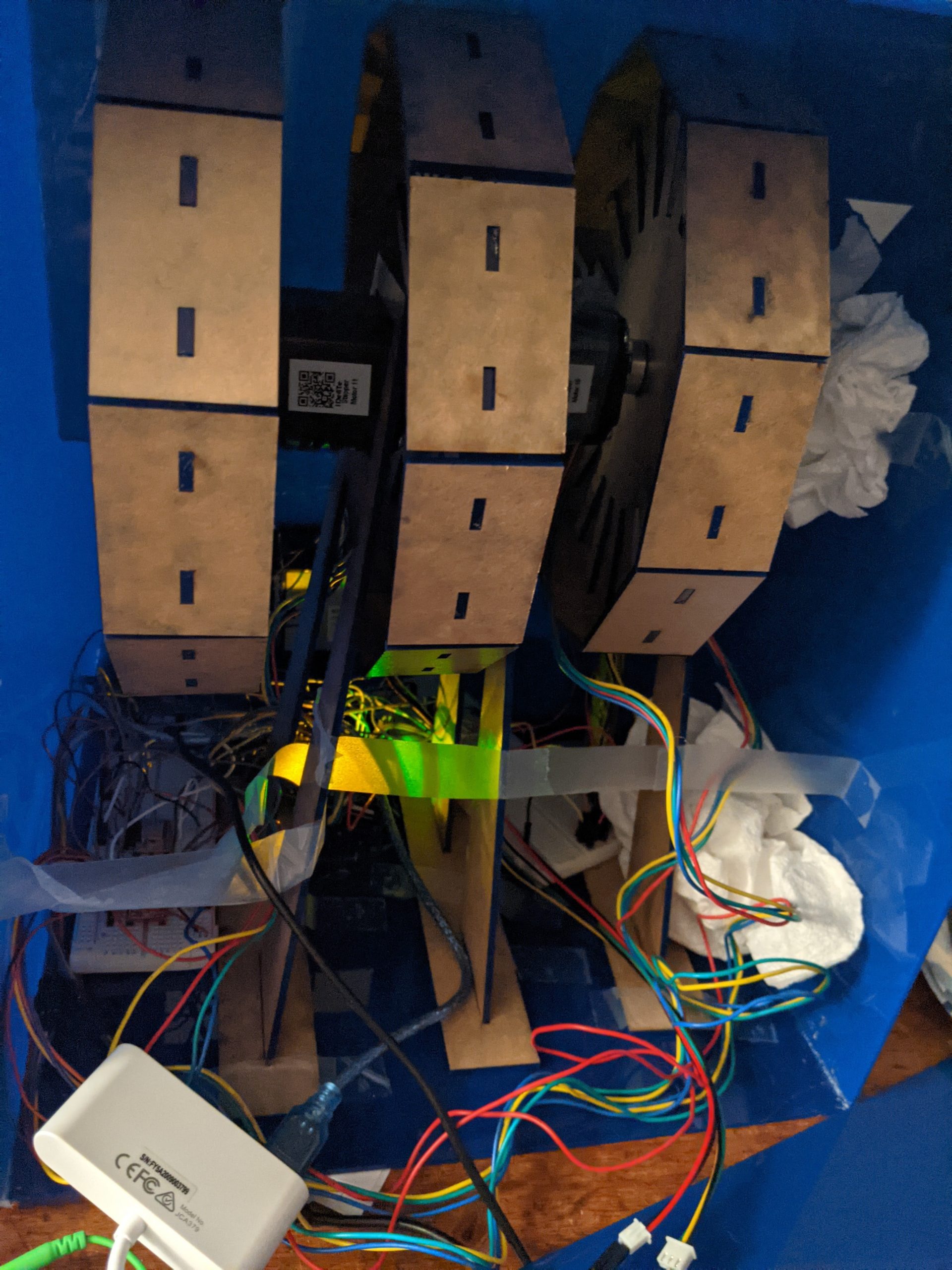
My desperate quick fix solution to set up the three wheels
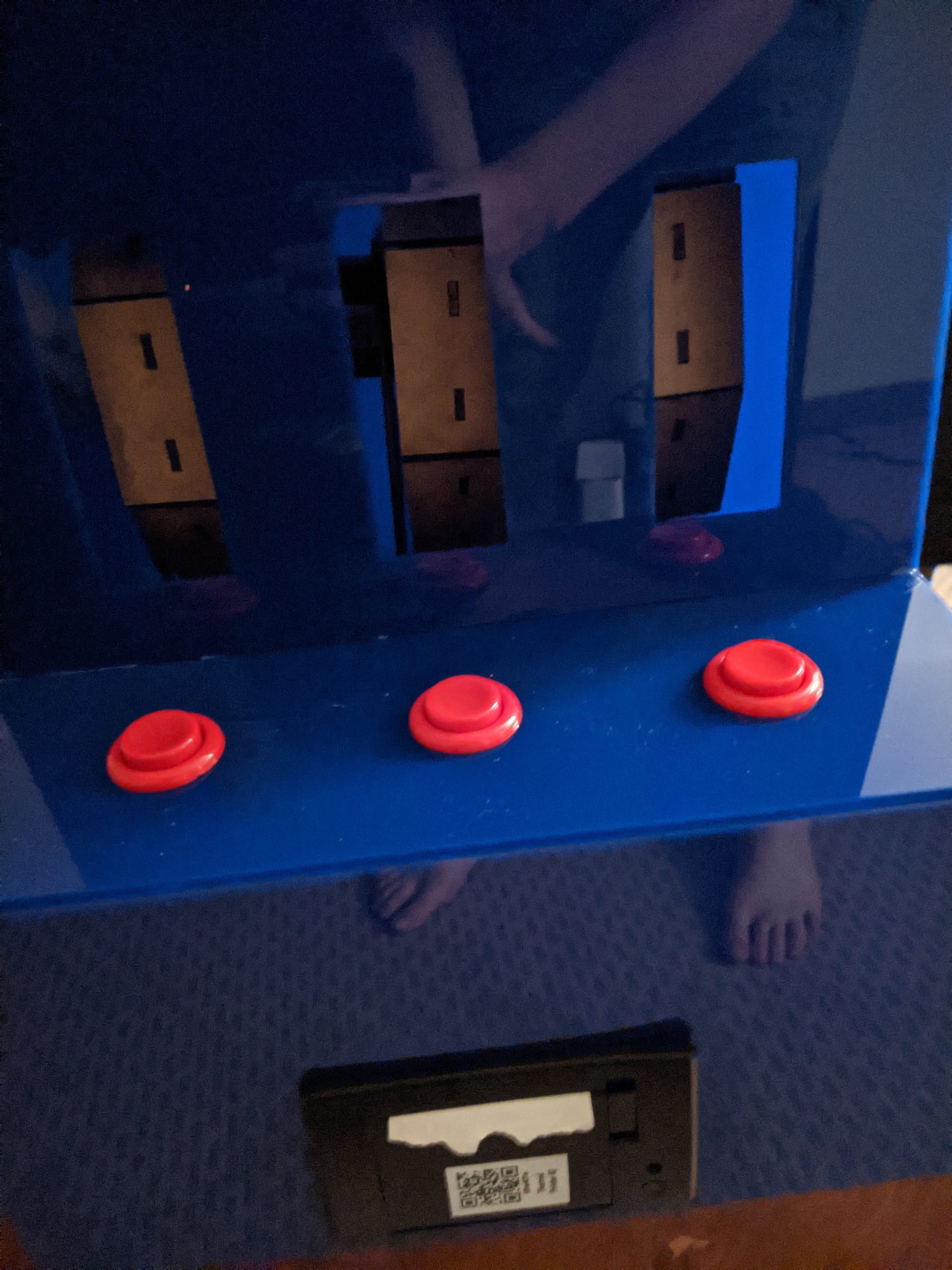
It works… to an extent.
Process Reflection
I had a lot of problems during this project. I failed to account for a lot of the physical mechanics and innerworkings of the stands that hold up the wheels. To make matters worse, one of my wheels shattered into pieces. I had to use whatever I had to make things work. As a result, it isn’t stable at all and I had to use many unconventional materials to slap things together. The result is that it is kind of a mess. To make matters worse, it would randomly stop working at random intervals and I had to take time to fix it. I definitely didn’t give myself enough time to account for these problems.
In the end, I didn’t have the time to implement a lot of elements to the project that I was looking forward to. I didn’t have time to program and wire a receipt printer to print out the user’s results. I also didn’t have the time to make it visually appealing. I almost wish I didn’t print out any signage at all because it would have looked slightly less slapped together and more intentional if I hadn’t.
In the critique, many points were also brought up that I failed to consider early on in the project. I could’ve given more consideration to the relationship between the input and output and the connection to the overarching idea. My ultimate takeaway is that I should’ve done more detailed consideration and planning early on in the project to know how I would build the stands and box in general. I also should have put more work into the project early on to account for unexpected problems. I believe that I would not have struggled nearly as much if I had built a prototype stand for the prototype.
Code Submission
/* * * The Jackpot of Life * by Leah Walko * * Pin 11 -> left button * Pin 12 -> middle button * Pin 13 -> right button * Pin 2 -> left stepper step * Pin 3 -> left stepper direction * Pin 4 -> middle stepper step * Pin 5 -> middle stepper direction * Pin 6 -> right stepper step * Pin 7 -> right stepper direction * * read a xyz magnetic value ("Prayers") and turn it into * three Stepper value * * Sources: * QMC5883LCompass.h Library XYZ Example Sketch * Learn more at [https://github.com/mprograms/QMC5883Compas] * AccelStepper.h library example * */ // Pos: 10, 30, 50, 70, 90, 110, 130, 150, 170, 190 #include <QMC5883LCompass.h> #include <AccelStepper.h> const int LEFTBUTTONPIN = 11; const int MIDBUTTONPIN = 12; const int RIGHTBUTTONPIN = 13; const int LEFTSTEPPIN = 2; const int LEFTDIRPIN = 3; const int MIDSTEPPIN = 4; const int MIDDIRPIN = 5; const int RIGHTSTEPPIN = 6; const int RIGHTDIRPIN = 7; AccelStepper myMotorLeft(1, LEFTSTEPPIN, LEFTDIRPIN); AccelStepper myMotorMid(1, MIDSTEPPIN, MIDDIRPIN); AccelStepper myMotorRight(1, RIGHTSTEPPIN, RIGHTDIRPIN); QMC5883LCompass compass; int posX = 0; int posY = 0; int posZ = 0; int spin = 3000; int x, y, z; boolean leftIsSpinning = false; boolean leftGetResults = false; boolean midIsSpinning = false; boolean midGetResults = false; boolean rightIsSpinning = false; boolean rightGetResults = false; unsigned long timer = 0; const int INTERVAL = 1000; void setup() { // put your setup code here, to run once: Serial.begin(9600); compass.init(); pinMode(LEFTBUTTONPIN, INPUT); pinMode(MIDBUTTONPIN, INPUT); pinMode(RIGHTBUTTONPIN, INPUT); myMotorLeft.setMaxSpeed(1000); myMotorLeft.setAcceleration(500); myMotorLeft.setSpeed(1000); myMotorMid.setMaxSpeed(1000); myMotorMid.setAcceleration(500); myMotorMid.setSpeed(1000); myMotorRight.setMaxSpeed(1000); myMotorRight.setAcceleration(500); myMotorRight.setSpeed(1000); } void loop() { // put your main code here, to run repeatedly: if(digitalRead(LEFTBUTTONPIN) == !HIGH) { leftIsSpinning = true; } else { leftIsSpinning = false; } if(digitalRead(MIDBUTTONPIN) == !HIGH) { midIsSpinning = true; } else { midIsSpinning = false; } if(digitalRead(RIGHTBUTTONPIN) == !HIGH) { rightIsSpinning = true; } else { rightIsSpinning = false; } if(leftIsSpinning) { compass.read(); x = compass.getX(); myMotorLeft.runSpeed(); leftGetResults = true; } if(midIsSpinning) { compass.read(); y = compass.getY(); myMotorMid.runSpeed(); midGetResults = true; } if(rightIsSpinning) { compass.read(); z = compass.getZ(); myMotorRight.runSpeed(); rightGetResults = true; } if(leftGetResults && !leftIsSpinning) { if(x < -3000) { x = -3000; } else if (x > 3000) { x = 3000; } posX = map(x, -3000, 3000, 1, 10); posX = 20*posX - 10; Serial.print("current x compass: "); Serial.println(x); Serial.print("move to Position: "); Serial.println(posX); myMotorLeft.moveTo(posX); leftGetResults = false; } if(midGetResults && !midIsSpinning) { y = (y+x)/2; if(y < -3000) { y = -3000; } else if (y > 3000) { y = 3000; } posY = map(y, -3000, 3000, 1, 10); posY = 20*posY - 10; Serial.print("current y compass: "); Serial.println(y); Serial.print("move to Position: "); Serial.println(posY); myMotorMid.moveTo(posY); midGetResults = false; } if(rightGetResults && !rightIsSpinning) { y = (Z+y+x)/3; if(z < -3000) { z = -3000; } else if (z > 3000) { z = 3000; } posZ = map(z, -3000, 3000, 1, 10); posZ = 20*posZ - 10; Serial.print("current z compass: "); Serial.println(z); Serial.print("move to Position: "); Serial.println(posZ); myMotorRight.moveTo(posZ); rightGetResults = false; } if(myMotorLeft.distanceToGo() != 0) { myMotorLeft.run(); } else { myMotorLeft.setSpeed(1000); } if(myMotorMid.distanceToGo() != 0) { myMotorMid.run(); } else { myMotorMid.setSpeed(1000); } if(myMotorRight.distanceToGo() != 0) { myMotorRight.run(); } else { myMotorRight.setSpeed(1000); } }