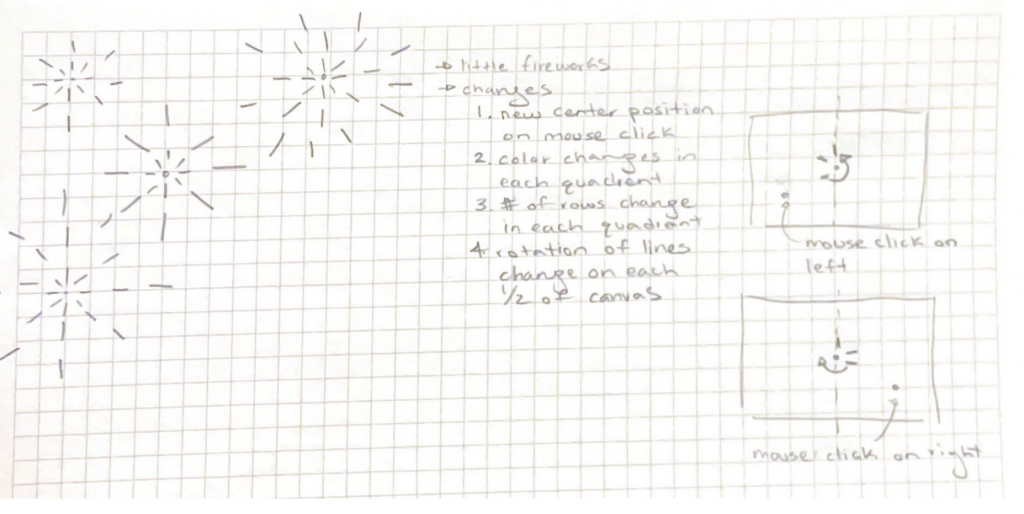
I began this project by writing down my main actions for the animation and what conditions caused them to change. After I got one part working, I slowly added more conditions and commands, ensuring they worked before continuing.
The animation starts with a simple circle of lines. The user can click on the screen to make the second row of lines appear. If the user clicks on the left half then the direction that the lines are drawn changes. Depending on the quadrant the user clicks in the number of rows and color will change.
sketch
// Emily Franco
// efranco
// Section C
//line distance from center
var fromCenter = 5;
//x and y positions of pts for lines
var ptX = 0;
var ptY = 0;
//default line length
var length = 5;
//current rotation degree
var deg = 0;
var rot = 5;
var dir = 1;
var degTwo = 0;
//tracks how many times mouse is clicked
var clicked = 0;
//tracks number of circle sets
var cirSet = 0;
//stores mouseX and mouseY when clicked
var mX =0;
var mY = 0;
//store previous tranlation to subtract from when new center is clicked
var transX;
var transY;
//num of rows around circle
var rows = 6;
//colors
var r = 255;
var g = 255;
var b = 0;
//color increment
var colorInt = 20;
function setup() {
createCanvas(600, 450);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
frameRate (70);
background ("black");
}
function draw() {
//change origin to center of canvas
transX = width/2;
transY = height/2;
translate (transX,transY);
//if mouse is clicke five times now center is
//at mouse position
if (cirSet==1){
translate (mX-transX,mY-transY);
transX = mX-transX;
transY = mY-transY;
}
//only reset at start of each set
if(clicked == rows){
//reset values
fromCenter = 5;
length = 5;
clicked = 0;
}
if (deg <= 360){
//rotate lines
rotate (radians(deg));
deg += rot*dir;
//if mouse is in left half turn clockwise
//if mouse is in right hald turn counterclockwise
//draw lines towards center of canvas
stroke (g,r,b);
line (ptX+fromCenter,ptY,ptX+fromCenter+length,ptY);
}
//line "chases white lines" and draws over them
push();
stroke (b,g,r);
rotate (radians(degTwo));
degTwo += rot*dir;
line (ptX+fromCenter,ptY,ptX+fromCenter+length,ptY);
//rect (ptX-1,ptY-length-fromCenter-1,2,length+2);
pop();
}
function mousePressed (){
//increase distance of lines from center
fromCenter += length + 10;
//increase length of lines
length+=10;
//if mouse clicked left of center draw counter clockwise
if (mouseX<=width/2){
dir = -1;
}else if (mouseX>=width/2){
dir = 1;
}
//if circle is complete set degree back to zero
//this will let next row start where previous row ended for only white lines
if (Math.abs(deg)>=360){
deg = 0;
}
//add one to clicked each time mouse pressed
clicked += 1;
//store x and y position for new center
if (clicked==rows){
cirSet = 1;
mX = mouseX;
mY = mouseY;
}
//number of rows per circle changed on first click of each set
if (clicked==1){
//number of rows per circle set changes in depending
//on the quadrant clicked in
if (mouseX<=width/2 & mouseY<=height/2){
rows = 3;
//blue
r = 255;
g = 0;
b = 0;
}if (mouseX>=width/2 & mouseY<=height/2){
rows = 4;
//yellow
r = 255;
g = 255;
b = 0;
}if (mouseX>=width/2 & mouseY>=height/2){
rows = 2;
//green
r = 128;
g = 255;
b = 0;
}if (mouseX<=width/2 & mouseY>=height/2){
rows = 6;
//orange
r = 255;
g = 128;
b = 0;
}
}
}